text
stringlengths 2
104M
| meta
dict |
---|---|
try:
from setuptools import setup
except ImportError:
from distutils.core import setup
config = {
'description': 'pyfix',
'author': 'Tom Fewster',
'url': 'https://github.com/wannabegeek/PyFIX/',
'download_url': 'https://github.com/wannabegeek/PyFIX/',
'author_email': '[email protected].',
'version': '0.1',
'install_requires': [''],
'packages': ['pyfix', 'pyfix/FIX44'],
'scripts': [],
'name': 'pyfix'
}
setup(**config) | {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
# PyFIX [](https://travis-ci.org/wannabegeek/PyFIX)
Open Source implementation of a FIX (Financial Information eXchange) Engine implemented in Python
See here [http://fixprotocol.org/] for more information on what FIX is.
## Installation
This package requires Python 3 to run.
Install in the normal python way
```
python setup.py install
```
and it should install with no errors
## Usage
Using the module should be simple. There is an examples directory, which is the probably best place to start.
### Session Setup
Create an `EventManager` object instance, this handles all the timers and socket data required by the FIX engine, however, you can add to events to the manager if required.
Either you can create a `FIXClient` or a `FIXServer`. The Client initiates the connection and also initaiates the Logon sequence, a Server would sit there waiting for inbound connections, and expect a Logon message to be sent.
```python
self.eventMgr = EventManager()
self.client = FIXClient(self.eventMgr, "pyfix.FIX44", "TARGET", "SENDER")
# tell the client to start the connection sequence
self.client.start('localhost', int("9898"))
# enter the event loop waiting for something to happen
while True:
self.eventMgr.waitForEvent()
```
The argument "pyfix.FIX44" specified the module which is used as the protocol, this is dynamically loaded, to you can create and specify your own if required.
If you want to do something useful, other than just watching the session level bits work, you'll probably want to register for connection status changes (you'll need to do this be fore starting the event loop);
```python
self.client.addConnectionListener(self.onConnect, ConnectionState.CONNECTED)
self.client.addConnectionListener(self.onDisconnect, ConnectionState.DISCONNECTED)
```
The implementatino of thouse methods would be something like this;
```python
def onConnect(self, session):
logging.info("Established connection to %s" % (session.address(), ))
session.addMessageHandler(self.onLogin, MessageDirection.INBOUND, self.client.protocol.msgtype.LOGON)
def onDisconnect(self, session):
logging.info("%s has disconnected" % (session.address(), ))
session.removeMsgHandler(self.onLogin, MessageDirection.INBOUND, self.client.protocol.msgtype.LOGON)
```
in the code above, we are registering to be called back whenever we receive (`MessageDirection.INBOUND`) a logon request `MsgType[35]=A` on that session.
That is pretty much it for the session setup.
### Message construction and sending
Constructing a message is simple, and is just a matter of adding the fields you require.
The session level tags will be added when the message is encoded by the codec. Setting any of the following session tags will result in the tag being duplicated in the message
- BeginString
- BodyLength
- MsgType
- MsgSeqNo
- SendingTime
- SenderCompID
- TargetCompID
- CheckSum
Example of building a simple message
```python
def sendOrder(self, connectionHandler):
self.clOrdID = self.clOrdID + 1
# get the codec we are currently using for this session
codec = connectionHandler.codec
# create a new message
msg = FIXMessage(codec.protocol.msgtype.NEWORDERSINGLE)
# ...and add some data to it
msg.setField(codec.protocol.fixtags.Price, random.random() * 1000)
msg.setField(codec.protocol.fixtags.OrderQty, int(random.random() * 10000))
msg.setField(codec.protocol.fixtags.Symbol, "VOD.L")
msg.setField(codec.protocol.fixtags.SecurityID, "GB00BH4HKS39")
msg.setField(codec.protocol.fixtags.SecurityIDSource, "4")
msg.setField(codec.protocol.fixtags.Symbol, "VOD.L")
msg.setField(codec.protocol.fixtags.Account, "TEST")
msg.setField(codec.protocol.fixtags.HandlInst, "1")
msg.setField(codec.protocol.fixtags.ExDestination, "XLON")
msg.setField(codec.protocol.fixtags.Side, int(random.random() * 2))
msg.setField(codec.protocol.fixtags.ClOrdID, str(self.clOrdID))
msg.setField(codec.protocol.fixtags.Currency, "GBP")
# send the message on the session
connectionHandler.sendMsg(codec.pack(msg, connectionHandler.session))
```
A message (which is a subclass of `FIXContext`) can also hold instances of `FIXContext`, these will be treated as repeating groups. For example
```
msg = FIXMessage(codec.protocol.msgtype.NEWORDERSINGLE)
msg.setField(codec.protocol.fixtags.Symbol, "VOD.L")
msg.setField(codec.protocol.fixtags.SecurityID, "GB00BH4HKS39")
msg.setField(codec.protocol.fixtags.SecurityIDSource, "4")
rptgrp1 = FIXContext()
rptgrp1.setField(codec.protocol.fixtags.PartyID, "It's Me")
rptgrp1.setField(codec.protocol.fixtags.PartyIDSource, "1")
rptgrp1.setField(codec.protocol.fixtags.PartyRole, "2")
msg.addRepeatingGroup(codec.protocol.fixtags.NoPartyIDs, rptgrp1)
rptgrp2 = FIXContext()
rptgrp2.setField(codec.protocol.fixtags.PartyID, "Someone Else")
rptgrp2.setField(codec.protocol.fixtags.PartyIDSource, "2")
rptgrp2.setField(codec.protocol.fixtags.PartyRole, "8")
msg.addRepeatingGroup(codec.protocol.fixtags.NoPartyIDs, rptgrp2)
```
This will result in a message like the following
```
8=FIX.4.4|9=144|35=D|49=sender|56=target|34=1|52=20150619-11:08:54.000|55=VOD.L|48=GB00BH4HKS39|22=4|453=2|448=It's Me|447=1|452=2|448=Someone Else|447=2|452=8|10=073|
```
To send the message you need a handle on the session you want to use, this is provided to you in the callback methods. e.g. in the code above we registered for Logon callbacks using
```
session.addMessageHandler(self.onLogin, MessageDirection.INBOUND, self.client.protocol.msgtype.LOGON)
```
the signature for the callback is something like;
```
def onLogin(self, connectionHandler, msg):
logging.info("Logged in")
```
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
import logging
import socket
from pyfix.journaler import DuplicateSeqNoError
from pyfix.message import FIXMessage
from pyfix.session import FIXSession
from pyfix.connection import FIXEndPoint, ConnectionState, MessageDirection, FIXConnectionHandler
from pyfix.event import TimerEventRegistration
class FIXClientConnectionHandler(FIXConnectionHandler):
def __init__(self, engine, protocol, targetCompId, senderCompId, sock=None, addr=None, observer=None, targetSubId = None, senderSubId = None, heartbeatTimeout = 30):
FIXConnectionHandler.__init__(self, engine, protocol, sock, addr, observer)
self.targetCompId = targetCompId
self.senderCompId = senderCompId
self.targetSubId = targetSubId
self.senderSubId = senderSubId
self.heartbeatPeriod = float(heartbeatTimeout)
# we need to send a login request.
self.session = self.engine.getOrCreateSessionFromCompIds(self.targetCompId, self.senderCompId)
if self.session is None:
raise RuntimeError("Failed to create client session")
self.sendMsg(protocol.messages.Messages.logon())
def handleSessionMessage(self, msg):
protocol = self.codec.protocol
responses = []
recvSeqNo = msg[protocol.fixtags.MsgSeqNum]
msgType = msg[protocol.fixtags.MsgType]
targetCompId = msg[protocol.fixtags.TargetCompID]
senderCompId = msg[protocol.fixtags.SenderCompID]
if msgType == protocol.msgtype.LOGON:
if self.connectionState == ConnectionState.LOGGED_IN:
logging.warning("Client session already logged in - ignoring login request")
else:
try:
self.connectionState = ConnectionState.LOGGED_IN
self.heartbeatPeriod = float(msg[protocol.fixtags.HeartBtInt])
self.registerLoggedIn()
except DuplicateSeqNoError:
logging.error("Failed to process login request with duplicate seq no")
self.disconnect()
return
elif self.connectionState == ConnectionState.LOGGED_IN:
# compids are reversed here
if not self.session.validateCompIds(senderCompId, targetCompId):
logging.error("Received message with unexpected comp ids")
self.disconnect()
return
if msgType == protocol.msgtype.LOGOUT:
self.connectionState = ConnectionState.LOGGED_OUT
self.registerLoggedOut()
self.handle_close()
elif msgType == protocol.msgtype.TESTREQUEST:
responses.append(protocol.messages.Messages.heartbeat())
elif msgType == protocol.msgtype.RESENDREQUEST:
responses.extend(self._handleResendRequest(msg))
elif msgType == protocol.msgtype.SEQUENCERESET:
# we can treat GapFill and SequenceReset in the same way
# in both cases we will just reset the seq number to the
# NewSeqNo received in the message
newSeqNo = msg[protocol.fixtags.NewSeqNo]
if msg[protocol.fixtags.GapFillFlag] == "Y":
logging.info("Received SequenceReset(GapFill) filling gap from %s to %s" % (recvSeqNo, newSeqNo))
self.session.setRecvSeqNo(int(newSeqNo) - 1)
recvSeqNo = newSeqNo
else:
logging.warning("Can't process message, counterparty is not logged in")
return (recvSeqNo, responses)
class FIXClient(FIXEndPoint):
def __init__(self, engine, protocol, targetCompId, senderCompId, targetSubId = None, senderSubId = None, heartbeatTimeout = 30):
self.targetCompId = targetCompId
self.senderCompId = senderCompId
self.targetSubId = targetSubId
self.senderSubId = senderSubId
self.heartbeatTimeout = heartbeatTimeout
FIXEndPoint.__init__(self, engine, protocol)
def tryConnecting(self, type, closure):
try:
self.socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
logging.debug("Attempting Connection to " + self.host + ":" + str(self.port))
self.socket.connect((self.host, self.port))
if self.connectionRetryTimer is not None:
self.engine.eventManager.unregisterHandler(self.connectionRetryTimer)
self.connectionRetryTimer = None
self.connected()
except socket.error as why:
logging.error("Connection failed, trying again in 5s")
if self.connectionRetryTimer is None:
self.connectionRetryTimer = TimerEventRegistration(self.tryConnecting, 5.0)
self.engine.eventManager.registerHandler(self.connectionRetryTimer)
def start(self, host, port):
self.host = host
self.port = port
self.connections = []
self.connectionRetryTimer = None
self.tryConnecting(None, None)
def connected(self):
self.addr = (self.host, self.port)
logging.info("Connected to %s" % repr(self.addr))
connection = FIXClientConnectionHandler(self.engine, self.protocol, self.targetCompId, self.senderCompId, self.socket, self.addr, self, self.targetSubId, self.senderSubId, self.heartbeatTimeout)
self.connections.append(connection)
for handler in filter(lambda x: x[1] == ConnectionState.CONNECTED, self.connectionHandlers):
handler[0](connection)
def notifyDisconnect(self, connection):
FIXEndPoint.notifyDisconnect(self, connection)
self.tryConnecting(None, None)
def stop(self):
logging.info("Stopping client connections")
for connection in self.connections:
connection.disconnect()
self.socket.close()
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
import logging
class FIXSession:
def __init__(self, key, targetCompId, senderCompId):
self.key = key
self.senderCompId = senderCompId
self.targetCompId = targetCompId
self.sndSeqNum = 0
self.nextExpectedMsgSeqNum = 1
def validateCompIds(self, targetCompId, senderCompId):
return self.senderCompId == senderCompId and self.targetCompId == targetCompId
def allocateSndSeqNo(self):
self.sndSeqNum += 1
return str(self.sndSeqNum)
def validateRecvSeqNo(self, seqNo):
if self.nextExpectedMsgSeqNum < int(seqNo):
logging.warning("SeqNum from client unexpected (Rcvd: %s Expected: %s)" % (seqNo, self.nextExpectedMsgSeqNum))
return (False, self.nextExpectedMsgSeqNum)
else:
return (True, seqNo)
def setRecvSeqNo(self, seqNo):
# if self.nextExpectedMsgSeqNum != int(seqNo):
# logging.warning("SeqNum from client unexpected (Rcvd: %s Expected: %s)" % (seqNo, self.nextExpectedMsgSeqNum))
self.nextExpectedMsgSeqNum = int(seqNo) + 1
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
from pyfix.event import EventManager
from pyfix.journaler import Journaler
class FIXEngine(object):
def __init__(self, journalfile = None):
self.eventManager = EventManager()
self.journaller = Journaler(journalfile)
self.sessions = {}
# We load all sessions from the journal and add to our list
for session in self.journaller.sessions():
self.sessions[session.key] = session
def validateSession(self, targetCompId, senderCompId):
# this make any session we receive valid
return True
def shouldResendMessage(self, session, msg):
# we should resend all application messages
return True
def createSession(self, targetCompId, senderCompId):
if self.findSessionByCompIds(targetCompId, senderCompId) is None:
session = self.journaller.createSession(targetCompId, senderCompId)
self.sessions[session.key] = session
else:
raise RuntimeError("Failed to add session with duplicate key")
return session
def getSession(self, identifier):
try:
return self.sessions[identifier]
except KeyError:
return None
def findSessionByCompIds(self, targetCompId, senderCompId):
sessions = [x for x in self.sessions.values() if x.targetCompId == targetCompId and x.senderCompId == senderCompId]
if sessions is not None and len(sessions) != 0:
return sessions[0]
return None
def getOrCreateSessionFromCompIds(self, targetCompId, senderCompId):
session = self.findSessionByCompIds(targetCompId, senderCompId)
if session is None:
if self.validateSession(targetCompId, senderCompId):
session = self.createSession(targetCompId, senderCompId)
return session
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
from collections import OrderedDict
from enum import Enum
class MessageDirection(Enum):
INBOUND = 0
OUTBOUND = 1
class _FIXRepeatingGroupContainer:
def __init__(self):
self.groups = []
def addGroup(self, group, index):
if index == -1:
self.groups.append(group)
else:
self.groups.insert(index, group)
def removeGroup(self, index):
del self.groups[index]
def getGroup(self, index):
return self.groups[index]
def __str__(self):
return str(len(self.groups)) + "=>" + str(self.groups)
__repr__ = __str__
class FIXContext(object):
def __init__(self):
self.tags = OrderedDict()
def setField(self, tag, value):
self.tags[tag] = value
def removeField(self, tag):
try:
del self.tags[tag]
except KeyError:
pass
def getField(self, tag):
return self.tags[tag]
def addRepeatingGroup(self, tag, group, index=-1):
if tag in self.tags:
groupContainer = self.tags[tag]
groupContainer.addGroup(group, index)
else:
groupContainer = _FIXRepeatingGroupContainer()
groupContainer.addGroup(group, index)
self.tags[tag] = groupContainer
def removeRepeatingGroupByIndex(self, tag, index=-1):
if self.isRepeatingGroup(tag):
try:
if index == -1:
del self.tags[tag]
pass
else:
groups = self.tags[tag]
groups.removeGroup(index)
except KeyError:
pass
def getRepeatingGroup(self, tag):
if self.isRepeatingGroup(tag):
return (len(self.tags[tag].groups), self.tags[tag].groups)
return None
def getRepeatingGroupByTag(self, tag, identifierTag, identifierValue):
if self.isRepeatingGroup(tag):
for group in self.tags[tag].groups:
if identifierTag in group.tags:
if group.getField(identifierTag) == identifierValue:
return group
return None
def getRepeatingGroupByIndex(self, tag, index):
if self.isRepeatingGroup(tag):
return self.tags[tag].groups[index]
return None
def __getitem__(self, tag):
return self.getField(tag)
def __setitem__(self, tag, value):
self.setField(tag, value)
def isRepeatingGroup(self, tag):
return type(self.tags[tag]) is _FIXRepeatingGroupContainer
def __contains__(self, item):
return item in self.tags
def __str__(self):
r= ""
allTags = []
for tag in self.tags:
allTags.append("%s=%s" % (tag, self.tags[tag]))
r += "|".join(allTags)
return r
def __eq__(self, other):
# if our string representation looks the same, the objects are equivalent
return self.__str__() == other.__str__()
__repr__ = __str__
class FIXMessage(FIXContext):
def __init__(self, msgType):
self.msgType = msgType
FIXContext.__init__(self)
def setMsgType(self, msgType):
self.msgType = msgType
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
import sqlite3
import pickle
from pyfix.message import MessageDirection
from pyfix.session import FIXSession
class DuplicateSeqNoError(Exception):
pass
class Journaler(object):
def __init__(self, filename = None):
if filename is None:
self.conn = sqlite3.connect(":memory:")
else:
self.conn = sqlite3.connect(filename)
self.cursor = self.conn.cursor()
self.cursor.execute("CREATE TABLE IF NOT EXISTS message("
"seqNo INTEGER NOT NULL,"
"session TEXT NOT NULL,"
"direction INTEGER NOT NULL,"
"msg TEXT,"
"PRIMARY KEY (seqNo, session, direction))")
self.cursor.execute("CREATE TABLE IF NOT EXISTS session("
"sessionId INTEGER PRIMARY KEY AUTOINCREMENT,"
"targetCompId TEXT NOT NULL,"
"senderCompId TEXT NOT NULL,"
"outboundSeqNo INTEGER DEFAULT 0,"
"inboundSeqNo INTEGER DEFAULT 0,"
"UNIQUE (targetCompId, senderCompId))")
def sessions(self):
sessions = []
self.cursor.execute("SELECT sessionId, targetCompId, senderCompId, outboundSeqNo, inboundSeqNo FROM session")
for sessionInfo in self.cursor:
session = FIXSession(sessionInfo[0], sessionInfo[1], sessionInfo[2])
session.sndSeqNum = sessionInfo[3]
session.nextExpectedMsgSeqNum = sessionInfo[4] + 1
sessions.append(session)
return sessions
def createSession(self, targetCompId, senderCompId):
session = None
try:
self.cursor.execute("INSERT INTO session(targetCompId, senderCompId) VALUES(?, ?)", (targetCompId, senderCompId))
sessionId = self.cursor.lastrowid
self.conn.commit()
session = FIXSession(sessionId, targetCompId, senderCompId)
except sqlite3.IntegrityError:
raise RuntimeError("Session already exists for TargetCompId: %s SenderCompId: %s" % (targetCompId, senderCompId))
return session
def persistMsg(self, msg, session, direction):
msgStr = pickle.dumps(msg)
seqNo = msg["34"]
try:
self.cursor.execute("INSERT INTO message VALUES(?, ?, ?, ?)", (seqNo, session.key, direction.value, msgStr))
if direction == MessageDirection.OUTBOUND:
self.cursor.execute("UPDATE session SET outboundSeqNo=?", (seqNo,))
elif direction == MessageDirection.INBOUND:
self.cursor.execute("UPDATE session SET inboundSeqNo=?", (seqNo,))
self.conn.commit()
except sqlite3.IntegrityError as e:
raise DuplicateSeqNoError("%s is a duplicate" % (seqNo, ))
def recoverMsg(self, session, direction, seqNo):
try:
msgs = self.recoverMsgs(session, direction, seqNo, seqNo)
return msgs[0]
except IndexError:
return None
def recoverMsgs(self, session, direction, startSeqNo, endSeqNo):
self.cursor.execute("SELECT msg FROM message WHERE session = ? AND direction = ? AND seqNo >= ? AND seqNo <= ? ORDER BY seqNo", (session.key, direction.value, startSeqNo, endSeqNo))
msgs = []
for msg in self.cursor:
msgs.append(pickle.loads(msg[0]))
return msgs
def getAllMsgs(self, sessions = [], direction = None):
sql = "SELECT seqNo, msg, direction, session FROM message"
clauses = []
args = []
if sessions is not None and len(sessions) != 0:
clauses.append("session in (" + ','.join('?'*len(sessions)) + ")")
args.extend(sessions)
if direction is not None:
clauses.append("direction = ?")
args.append(direction.value)
if clauses:
sql = sql + " WHERE " + " AND ".join(clauses)
sql = sql + " ORDER BY rowid"
self.cursor.execute(sql, tuple(args))
msgs = []
for msg in self.cursor:
msgs.append((msg[0], pickle.loads(msg[1]), msg[2], msg[3]))
return msgs | {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
class TransactionResource(object):
def __init__(self, action):
self.action = action
def commit(self):
if self.action != None:
self.action()
class Transaction(TransactionResource):
def __init__(self):
self.resources = []
TransactionResource.__init__(self, None)
def addResource(self, resource):
self.resources.append(resource)
pass
def commit(self):
for resource in self.resources:
resource.commit()
class PriorityTransaction(TransactionResource):
def __init__(self):
self.resources = []
TransactionResource.__init__(self, None)
def addResource(self, resource, priority):
self.resources.append((priority, resource))
def commit(self):
# TODO: sort the resources...
# High --> Low (so you can always make something higher priority)
for resource in self.resources:
resource.commit()
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
import logging
import socket
from pyfix.journaler import DuplicateSeqNoError
from pyfix.session import FIXSession
from pyfix.connection import FIXEndPoint, ConnectionState, MessageDirection, FIXConnectionHandler
from pyfix.event import FileDescriptorEventRegistration, EventType
class FIXServerConnectionHandler(FIXConnectionHandler):
def __init__(self, engine, protocol, sock=None, addr=None, observer=None):
FIXConnectionHandler.__init__(self, engine, protocol, sock, addr, observer)
def handleSessionMessage(self, msg):
protocol = self.codec.protocol
recvSeqNo = msg[protocol.fixtags.MsgSeqNum]
msgType = msg[protocol.fixtags.MsgType]
targetCompId = msg[protocol.fixtags.TargetCompID]
senderCompId = msg[protocol.fixtags.SenderCompID]
responses = []
if msgType == protocol.msgtype.LOGON:
if self.connectionState == ConnectionState.LOGGED_IN:
logging.warning("Client session already logged in - ignoring login request")
else:
# compids are reversed here...
self.session = self.engine.getOrCreateSessionFromCompIds(senderCompId, targetCompId)
if self.session is not None:
try:
self.connectionState = ConnectionState.LOGGED_IN
self.heartbeatPeriod = float(msg[protocol.fixtags.HeartBtInt])
responses.append(protocol.messages.Messages.logon())
self.registerLoggedIn()
except DuplicateSeqNoError:
logging.error("Failed to process login request with duplicate seq no")
self.disconnect()
return
else:
logging.warning("Rejected login attempt for invalid session (SenderCompId: %s, TargetCompId: %s)" % (senderCompId, targetCompId))
self.disconnect()
return # we have to return here since self.session won't be valid
elif self.connectionState == ConnectionState.LOGGED_IN:
# compids are reversed here
if not self.session.validateCompIds(senderCompId, targetCompId):
logging.error("Received message with unexpected comp ids")
self.disconnect()
return
if msgType == protocol.msgtype.LOGOUT:
self.connectionState = ConnectionState.LOGGED_OUT
self.registerLoggedOut()
self.handle_close()
elif msgType == protocol.msgtype.TESTREQUEST:
responses.append(protocol.messages.Messages.heartbeat())
elif msgType == protocol.msgtype.RESENDREQUEST:
responses.extend(self._handleResendRequest(msg))
elif msgType == protocol.msgtype.SEQUENCERESET:
newSeqNo = msg[protocol.fixtags.NewSeqNo]
self.session.setRecvSeqNo(int(newSeqNo) - 1)
recvSeqNo = newSeqNo
else:
logging.warning("Can't process message, counterparty is not logged in")
return (recvSeqNo, responses)
class FIXServer(FIXEndPoint):
def __init__(self, engine, protocol):
FIXEndPoint.__init__(self, engine, protocol)
def start(self, host, port):
self.connections = []
self.socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
self.socket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
self.socket.bind((host, port))
self.socket.listen(5)
self.serverSocketRegistration = FileDescriptorEventRegistration(self.handle_accept, self.socket, EventType.READ)
logging.debug("Awaiting Connections " + host + ":" + str(port))
self.engine.eventManager.registerHandler(self.serverSocketRegistration)
def stop(self):
logging.info("Stopping server connections")
for connection in self.connections:
connection.disconnect()
self.serverSocketRegistration.fd.close()
self.engine.eventManager.unregisterHandler(self.serverSocketRegistration)
def handle_accept(self, type, closure):
pair = self.socket.accept()
if pair is not None:
sock, addr = pair
logging.info("Connection from %s" % repr(addr))
connection = FIXServerConnectionHandler(self.engine, self.protocol, sock, addr, self)
self.connections.append(connection)
for handler in filter(lambda x: x[1] == ConnectionState.CONNECTED, self.connectionHandlers):
handler[0](connection)
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
from datetime import datetime
import logging
from pyfix.message import FIXMessage, FIXContext
class EncodingError(Exception):
pass
class DecodingError(Exception):
pass
class RepeatingGroupContext(FIXContext):
def __init__(self, tag, repeatingGroupTags, parent):
self.tag = tag
self.repeatingGroupTags = repeatingGroupTags
self.parent = parent
FIXContext.__init__(self)
class Codec(object):
def __init__(self, protocol):
self.protocol = protocol
self.SOH = '\x01'
@staticmethod
def current_datetime():
return datetime.utcnow().strftime("%Y%m%d-%H:%M:%S.%f")[:-3]
def _addTag(self, body, t, msg):
if msg.isRepeatingGroup(t):
count, groups = msg.getRepeatingGroup(t)
body.append("%s=%s" % (t, count))
for group in groups:
for tag in group.tags:
self._addTag(body, tag, group)
else:
body.append("%s=%s" % (t, msg[t]))
def encode(self, msg, session):
# Create body
body = []
msgType = msg.msgType
body.append("%s=%s" % (self.protocol.fixtags.SenderCompID, session.senderCompId))
body.append("%s=%s" % (self.protocol.fixtags.TargetCompID, session.targetCompId))
seqNo = 0
if msgType == self.protocol.msgtype.SEQUENCERESET:
if self.protocol.fixtags.GapFillFlag in msg and msg[self.protocol.fixtags.GapFillFlag] == "Y":
# in this case the sequence number should already be on the message
try:
seqNo = msg[self.protocol.fixtags.MsgSeqNum]
except KeyError:
raise EncodingError("SequenceReset with GapFill='Y' must have the MsgSeqNum already populated")
else:
msg[self.protocol.fixtags.NewSeqNo] = session.allocateSndSeqNo()
seqNo = msg[self.protocol.fixtags.MsgSeqNum]
else:
# if we have the PossDupFlag set, we need to send the message with the same seqNo
if self.protocol.fixtags.PossDupFlag in msg and msg[self.protocol.fixtags.PossDupFlag] == "Y":
try:
seqNo = msg[self.protocol.fixtags.MsgSeqNum]
except KeyError:
raise EncodingError("Failed to encode message with PossDupFlay=Y but no previous MsgSeqNum")
else:
seqNo = session.allocateSndSeqNo()
body.append("%s=%s" % (self.protocol.fixtags.MsgSeqNum, seqNo))
body.append("%s=%s" % (self.protocol.fixtags.SendingTime, self.current_datetime()))
for t in msg.tags:
self._addTag(body, t, msg)
# Enable easy change when debugging
SEP = self.SOH
body = self.SOH.join(body) + self.SOH
# Create header
header = []
msgType = "%s=%s" % (self.protocol.fixtags.MsgType, msgType)
header.append("%s=%s" % (self.protocol.fixtags.BeginString, self.protocol.beginstring))
header.append("%s=%i" % (self.protocol.fixtags.BodyLength, len(body) + len(msgType) + 1))
header.append(msgType)
fixmsg = self.SOH.join(header) + self.SOH + body
cksum = sum([ord(i) for i in list(fixmsg)]) % 256
fixmsg = fixmsg + "%s=%0.3i" % (self.protocol.fixtags.CheckSum, cksum)
#print len(fixmsg)
return fixmsg + SEP
def decode(self, rawmsg):
#msg = rawmsg.rstrip(os.linesep).split(SOH)
try:
rawmsg = rawmsg.decode('utf-8')
msg = rawmsg.split(self.SOH)
msg = msg[:-1]
if len(msg) < 3: # at a minumum we require BeginString, BodyLength & Checksum
return (None, 0)
tag, value = msg[0].split('=', 1)
if tag != self.protocol.fixtags.BeginString:
logging.error("*** BeginString missing or not 1st field *** [" + tag + "]")
elif value != self.protocol.beginstring:
logging.error("FIX Version unexpected (Recv: %s Expected: %s)" % (value, self.protocol.beginstring))
tag, value = msg[1].split('=', 1)
msgLength = len(msg[0]) + len(msg[1]) + len('10=000') + 3
if tag != self.protocol.fixtags.BodyLength:
logging.error("*** BodyLength missing or not 2nd field *** [" + tag + "]")
else:
msgLength += int(value)
# do we have a complete message on the sockt
if msgLength > len(rawmsg):
return (None, 0)
else:
remainingMsgFragment = msgLength
# resplit our message
msg = rawmsg[:msgLength].split(self.SOH)
msg = msg[:-1]
decodedMsg = FIXMessage("UNKNOWN")
# logging.debug("\t-----------------------------------------")
# logging.debug("\t" + "|".join(msg))
repeatingGroups = []
repeatingGroupTags = self.protocol.fixtags.repeatingGroupIdentifiers()
currentContext = decodedMsg
for m in msg:
tag, value = m.split('=', 1)
t = None
try:
t = self.protocol.fixtags.tagToName(tag)
except KeyError:
logging.info("\t%s(Unknown): %s" % (tag, value))
t = "{unknown}"
if tag == self.protocol.fixtags.CheckSum:
cksum = ((sum([ord(i) for i in list(self.SOH.join(msg[:-1]))]) + 1) % 256)
if cksum != int(value):
logging.warning("\tCheckSum: %s (INVALID) expecting %s" % (int(value), cksum))
elif tag == self.protocol.fixtags.MsgType:
try:
msgType = self.protocol.msgtype.msgTypeToName(value)
decodedMsg.setMsgType(value)
except KeyError:
logging.error('*** MsgType "%s" not supported ***')
if tag in repeatingGroupTags: # found the start of a repeating group
if type(currentContext) is RepeatingGroupContext: # i.e. we are already in a repeating group
while repeatingGroups and tag not in currentContext.repeatingGroupTags:
currentContext.parent.addRepeatingGroup(currentContext.tag, currentContext)
currentContext = currentContext.parent
del repeatingGroups[-1] # pop the completed group off the stack
ctx = RepeatingGroupContext(tag, repeatingGroupTags[tag], currentContext)
repeatingGroups.append(ctx)
currentContext = ctx
elif repeatingGroups: # we have 1 or more repeating groups in progress & our tag isn't the start of a group
while repeatingGroups and tag not in currentContext.repeatingGroupTags:
currentContext.parent.addRepeatingGroup(currentContext.tag, currentContext)
currentContext = currentContext.parent
del repeatingGroups[-1] # pop the completed group off the stack
if tag in currentContext.tags:
# if the repeating group already contains this field, start the next
currentContext.parent.addRepeatingGroup(currentContext.tag, currentContext)
ctx = RepeatingGroupContext(currentContext.tag, currentContext.repeatingGroupTags, currentContext.parent)
del repeatingGroups[-1] # pop the completed group off the stack
repeatingGroups.append(ctx)
currentContext = ctx
# else add it to the current one
currentContext.setField(tag, value)
else:
# this isn't a repeating group field, so just add it normally
decodedMsg.setField(tag, value)
return (decodedMsg, remainingMsgFragment)
except UnicodeDecodeError as why:
logging.error("Failed to parse message %s" % (why, ))
return (None, 0)
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
import importlib
import sys
from pyfix.codec import Codec
from pyfix.journaler import DuplicateSeqNoError
from pyfix.message import FIXMessage, MessageDirection
from pyfix.session import *
from enum import Enum
from pyfix.event import FileDescriptorEventRegistration, EventType, TimerEventRegistration
class ConnectionState(Enum):
UNKNOWN = 0
DISCONNECTED = 1
CONNECTED = 2
LOGGED_IN = 3
LOGGED_OUT = 4
class FIXException(Exception):
class FIXExceptionReason(Enum):
NOT_CONNECTED = 0
DECODE_ERROR = 1
ENCODE_ERROR = 2
def __init__(self, reason, description = None):
super(Exception, self).__init__(description)
self.reason = reason
class SessionWarning(Exception):
pass
class SessionError(Exception):
pass
class FIXConnectionHandler(object):
def __init__(self, engine, protocol, sock=None, addr=None, observer=None):
self.codec = Codec(protocol)
self.engine = engine
self.connectionState = ConnectionState.CONNECTED
self.session = None
self.addr = addr
self.observer = observer
self.msgBuffer = b''
self.heartbeatPeriod = 30.0
self.msgHandlers = []
self.sock = sock
self.heartbeatTimerRegistration = None
self.expectedHeartbeatRegistration = None
self.socketEvent = FileDescriptorEventRegistration(self.handle_read, sock, EventType.READ)
self.engine.eventManager.registerHandler(self.socketEvent)
def address(self):
return self.addr
def disconnect(self):
self.handle_close()
def _notifyMessageObservers(self, msg, direction, persistMessage=True):
if persistMessage is True:
self.engine.journaller.persistMsg(msg, self.session, direction)
for handler in filter(lambda x: (x[1] is None or x[1] == direction) and (x[2] is None or x[2] == msg.msgType), self.msgHandlers):
handler[0](self, msg)
def addMessageHandler(self, handler, direction = None, msgType = None):
self.msgHandlers.append((handler, direction, msgType))
def removeMessageHandler(self, handler, direction = None, msgType = None):
remove = filter(lambda x: x[0] == handler and
(x[1] == direction or direction is None) and
(x[2] == msgType or msgType is None), self.msgHandlers)
for h in remove:
self.msgHandlers.remove(h)
def _sendHeartbeat(self):
self.sendMsg(self.codec.protocol.messages.Messages.heartbeat())
def _expectedHeartbeat(self, type, closure):
logging.warning("Expected heartbeat from peer %s" % (self.expectedHeartbeatRegistration ,))
self.sendMsg(self.codec.protocol.messages.Messages.test_request())
def registerLoggedIn(self):
self.heartbeatTimerRegistration = TimerEventRegistration(lambda type, closure: self._sendHeartbeat(), self.heartbeatPeriod)
self.engine.eventManager.registerHandler(self.heartbeatTimerRegistration)
# register timeout for 10% more than we expect
self.expectedHeartbeatRegistration = TimerEventRegistration(self._expectedHeartbeat, self.heartbeatPeriod * 1.10)
self.engine.eventManager.registerHandler(self.expectedHeartbeatRegistration)
def registerLoggedOut(self):
if self.heartbeatTimerRegistration is not None:
self.engine.eventManager.unregisterHandler(self.heartbeatTimerRegistration)
self.heartbeatTimerRegistration = None
if self.expectedHeartbeatRegistration is not None:
self.engine.eventManager.unregisterHandler(self.expectedHeartbeatRegistration)
self.expectedHeartbeatRegistration = None
def _handleResendRequest(self, msg):
protocol = self.codec.protocol
responses = []
beginSeqNo = msg[protocol.fixtags.BeginSeqNo]
endSeqNo = msg[protocol.fixtags.EndSeqNo]
if int(endSeqNo) == 0:
endSeqNo = sys.maxsize
logging.info("Received resent request from %s to %s", beginSeqNo, endSeqNo)
replayMsgs = self.engine.journaller.recoverMsgs(self.session, MessageDirection.OUTBOUND, beginSeqNo, endSeqNo)
gapFillBegin = int(beginSeqNo)
gapFillEnd = int(beginSeqNo)
for replayMsg in replayMsgs:
msgSeqNum = int(replayMsg[protocol.fixtags.MsgSeqNum])
if replayMsg[protocol.fixtags.MsgType] in protocol.msgtype.sessionMessageTypes:
gapFillEnd = msgSeqNum + 1
else:
if self.engine.shouldResendMessage(self.session, replayMsg):
if gapFillBegin < gapFillEnd:
# we need to send a gap fill message
gapFillMsg = FIXMessage(protocol.msgtype.SEQUENCERESET)
gapFillMsg.setField(protocol.fixtags.GapFillFlag, 'Y')
gapFillMsg.setField(protocol.fixtags.MsgSeqNum, gapFillBegin)
gapFillMsg.setField(protocol.fixtags.NewSeqNo, str(gapFillEnd))
responses.append(gapFillMsg)
# and then resent the replayMsg
replayMsg.removeField(protocol.fixtags.BeginString)
replayMsg.removeField(protocol.fixtags.BodyLength)
replayMsg.removeField(protocol.fixtags.SendingTime)
replayMsg.removeField(protocol.fixtags.SenderCompID)
replayMsg.removeField(protocol.fixtags.TargetCompID)
replayMsg.removeField(protocol.fixtags.CheckSum)
replayMsg.setField(protocol.fixtags.PossDupFlag, "Y")
responses.append(replayMsg)
gapFillBegin = msgSeqNum + 1
else:
gapFillEnd = msgSeqNum + 1
responses.append(replayMsg)
if gapFillBegin < gapFillEnd:
# we need to send a gap fill message
gapFillMsg = FIXMessage(protocol.msgtype.SEQUENCERESET)
gapFillMsg.setField(protocol.fixtags.GapFillFlag, 'Y')
gapFillMsg.setField(protocol.fixtags.MsgSeqNum, gapFillBegin)
gapFillMsg.setField(protocol.fixtags.NewSeqNo, str(gapFillEnd))
responses.append(gapFillMsg)
return responses
def handle_read(self, type, closure):
protocol = self.codec.protocol
try:
msg = self.sock.recv(8192)
if msg:
self.msgBuffer = self.msgBuffer + msg
(decodedMsg, parsedLength) = self.codec.decode(self.msgBuffer)
self.msgBuffer = self.msgBuffer[parsedLength:]
while decodedMsg is not None and self.connectionState != ConnectionState.DISCONNECTED:
self.processMessage(decodedMsg)
(decodedMsg, parsedLength) = self.codec.decode(self.msgBuffer)
self.msgBuffer = self.msgBuffer[parsedLength:]
if self.expectedHeartbeatRegistration is not None:
self.expectedHeartbeatRegistration.reset()
else:
logging.debug("Connection has been closed")
self.disconnect()
except ConnectionError as why:
logging.debug("Connection has been closed %s" % (why, ))
self.disconnect()
def handleSessionMessage(self, msg):
return -1
def processMessage(self, decodedMsg):
protocol = self.codec.protocol
beginString = decodedMsg[protocol.fixtags.BeginString]
if beginString != protocol.beginstring:
logging.warning("FIX BeginString is incorrect (expected: %s received: %s)", (protocol.beginstring, beginString))
self.disconnect()
return
msgType = decodedMsg[protocol.fixtags.MsgType]
try:
responses = []
if msgType in protocol.msgtype.sessionMessageTypes:
(recvSeqNo, responses) = self.handleSessionMessage(decodedMsg)
else:
recvSeqNo = decodedMsg[protocol.fixtags.MsgSeqNum]
# validate the seq number
(seqNoState, lastKnownSeqNo) = self.session.validateRecvSeqNo(recvSeqNo)
if seqNoState is False:
# We should send a resend request
logging.info("Requesting resend of messages: %s to %s" % (lastKnownSeqNo, 0))
responses.append(protocol.messages.Messages.resend_request(lastKnownSeqNo, 0))
# we still need to notify if we are processing Logon message
if msgType == protocol.msgtype.LOGON:
self._notifyMessageObservers(decodedMsg, MessageDirection.INBOUND, False)
else:
self.session.setRecvSeqNo(recvSeqNo)
self._notifyMessageObservers(decodedMsg, MessageDirection.INBOUND)
for m in responses:
self.sendMsg(m)
except SessionWarning as sw:
logging.warning(sw)
except SessionError as se:
logging.error(se)
self.disconnect()
except DuplicateSeqNoError:
try:
if decodedMsg[protocol.fixtags.PossDupFlag] == "Y":
logging.debug("Received duplicate message with PossDupFlag set")
except KeyError:
pass
finally:
logging.error("Failed to process message with duplicate seq no (MsgSeqNum: %s) (and no PossDupFlag='Y') - disconnecting" % (recvSeqNo, ))
self.disconnect()
def handle_close(self):
if self.connectionState != ConnectionState.DISCONNECTED:
logging.info("Client disconnected")
self.registerLoggedOut()
self.sock.close()
self.connectionState = ConnectionState.DISCONNECTED
self.msgHandlers.clear()
if self.observer is not None:
self.observer.notifyDisconnect(self)
self.engine.eventManager.unregisterHandler(self.socketEvent)
def sendMsg(self, msg):
if self.connectionState != ConnectionState.CONNECTED and self.connectionState != ConnectionState.LOGGED_IN:
raise FIXException(FIXException.FIXExceptionReason.NOT_CONNECTED)
encodedMsg = self.codec.encode(msg, self.session).encode('utf-8')
self.sock.send(encodedMsg)
if self.heartbeatTimerRegistration is not None:
self.heartbeatTimerRegistration.reset()
decodedMsg, junk = self.codec.decode(encodedMsg)
try:
self._notifyMessageObservers(decodedMsg, MessageDirection.OUTBOUND)
except DuplicateSeqNoError:
logging.error("We have sent a message with a duplicate seq no, failed to persist it (MsgSeqNum: %s)" % (decodedMsg[self.codec.protocol.fixtags.MsgSeqNum]))
class FIXEndPoint(object):
def __init__(self, engine, protocol):
self.engine = engine
self.protocol = importlib.import_module(protocol)
self.connections = []
self.connectionHandlers = []
def writable(self):
return True
def start(self, host, port):
pass
def stop(self):
pass
def addConnectionListener(self, handler, filter):
self.connectionHandlers.append((handler, filter))
def removeConnectionListener(self, handler, filter):
for s in self.connectionHandlers:
if s == (handler, filter):
self.connectionHandlers.remove(s)
def notifyDisconnect(self, connection):
self.connections.remove(connection)
for handler in filter(lambda x: x[1] == ConnectionState.DISCONNECTED, self.connectionHandlers):
handler[0](connection)
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
from enum import Enum
import datetime
import os
from select import select, error
import errno
import time
class EventType(Enum):
NONE = 0
READ = 1
WRITE = 2
TIMEOUT = 4
READWRITE = READ | WRITE
class EventRegistration(object):
def __init__(self, callback, closure=None):
self.callback = callback
self.closure = closure
class TimerEventRegistration(EventRegistration):
class TimeoutState(Enum):
NONE = 0
START = 1
PROGRESS = 2
def __init__(self, callback, timeout, closure=None):
EventRegistration.__init__(self, callback, closure)
self.timeout = timeout
self.timeoutState = TimerEventRegistration.TimeoutState.START
self.timeLeft = timeout
self.lastTime = None
def reset(self):
self.timeLeft = self.timeout
def __str__(self):
return "TimerEvent interval: %s, remaining: %s" % (self.timeout, self.timeLeft)
class FileDescriptorEventRegistration(EventRegistration):
def __init__(self, callback, fileDescriptor, eventType, closure=None):
EventRegistration.__init__(self, callback, closure)
self.fd = fileDescriptor
self.eventType = eventType
def __str__(self):
return "FileDescriptorEvent fd: %s, type: %s" % (self.fd, self.eventType.name)
class _Event(object):
def __init__(self, fd, filter):
self.fd = fd
self.filter = filter
class EventLoop(object):
def add(self, event):
pass
def remove(self, event):
pass
def run(self, timeout):
pass
class SelectEventLoop(EventLoop):
def __init__(self):
self.readSet = []
self.writeSet = []
def add(self, event):
if (event.filter.value & EventType.READ.value) == EventType.READ.value:
self.readSet.append(event.fd)
if (event.filter.value & EventType.WRITE.value) == EventType.WRITE.value:
self.writeSet.append(event.fd)
def remove(self, event):
if event.filter.value & EventType.READ.value == EventType.READ.value:
self.readSet.remove(event.fd)
if event.filter.value & EventType.WRITE.value == EventType.WRITE.value:
self.writeSet.remove(event.fd)
def run(self, timeout):
if len(self.readSet) == 0 and len(self.writeSet) ==0:
time.sleep(timeout)
return []
else:
while True:
try:
readReady, writeReady, exceptReady = select(self.readSet, self.writeSet, [], timeout)
events = []
for r in readReady:
events.append(_Event(r, EventType.READ))
for r in writeReady:
events.append(_Event(r, EventType.WRITE))
return events
except error as why:
if os.name == 'posix':
if why[0] != errno.EAGAIN and why[0] != errno.EINTR:
break
else:
if why[0] == errno.WSAEADDRINUSE:
break
class EventManager(object):
def __init__(self):
self.eventLoop = SelectEventLoop()
self.handlers = []
def waitForEvent(self):
self.waitForEventWithTimeout(None)
def waitForEventWithTimeout(self, timeout):
if not self.handlers:
raise RuntimeError("Failed to start event loop without any handlers")
timeout = self._setTimeout(timeout)
events = self.eventLoop.run(timeout)
self._serviceEvents(events)
def _setTimeout(self, timeout):
nowTime = datetime.datetime.utcnow()
duration = timeout
for handler in self.handlers:
if type(handler) is TimerEventRegistration:
if handler.timeoutState == TimerEventRegistration.TimeoutState.START:
handler.timeoutState = TimerEventRegistration.TimeoutState.PROGRESS
handler.lastTime = nowTime
if duration is None or handler.timeLeft < duration:
duration = handler.timeLeft
return duration
def _serviceEvents(self, events):
nowTime = datetime.datetime.utcnow()
for handler in self.handlers:
if isinstance(handler, FileDescriptorEventRegistration):
for event in events:
if event.fd == handler.fd:
type = handler.eventType.value & event.filter.value
if type != EventType.NONE:
handler.callback(type, handler.closure)
elif isinstance(handler, TimerEventRegistration):
if handler.timeoutState == TimerEventRegistration.TimeoutState.PROGRESS:
elapsedTime = nowTime - handler.lastTime
handler.timeLeft -= elapsedTime.total_seconds()
if handler.timeLeft <= 0.0:
handler.timeLeft = handler.timeout
handler.callback(EventType.TIMEOUT, handler.closure)
def registerHandler(self, handler):
if isinstance(handler, TimerEventRegistration):
pass
elif isinstance(handler, FileDescriptorEventRegistration):
self.eventLoop.add(_Event(handler.fd, handler.eventType))
else:
raise RuntimeError("Trying to register invalid handler")
self.handlers.append(handler)
def unregisterHandler(self, handler):
if self.isRegistered(handler):
self.handlers.remove(handler)
if isinstance(handler, FileDescriptorEventRegistration):
self.eventLoop.remove(_Event(handler.fd, handler.eventType))
def isRegistered(self, handler):
return handler in self.handlers
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
from pyfix.FIX44 import msgtype, messages
__author__ = 'tom'
beginstring = 'FIX.4.4'
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
from pyfix.FIX44 import msgtype, fixtags
from pyfix.message import FIXMessage
class Messages(object):
@staticmethod
def logon():
msg = FIXMessage(msgtype.LOGON)
msg.setField(fixtags.EncryptMethod, 0)
msg.setField(fixtags.HeartBtInt, 30)
return msg
@staticmethod
def logout():
msg = FIXMessage(msgtype.LOGOUT)
return msg
@staticmethod
def heartbeat():
msg = FIXMessage(msgtype.HEARTBEAT)
return msg
@staticmethod
def test_request():
msg = FIXMessage(msgtype.TESTREQUEST)
return msg
@staticmethod
def sequence_reset(respondingTo, isGapFill):
msg = FIXMessage(msgtype.SEQUENCERESET)
msg.setField(fixtags.GapFillFlag, 'Y' if isGapFill else 'N')
msg.setField(fixtags.MsgSeqNum, respondingTo[fixtags.BeginSeqNo])
return msg
#
# @staticmethod
# def sequence_reset(beginSeqNo, endSeqNo, isGapFill):
# msg = FIXMessage(msgtype.SEQUENCERESET)
# msg.setField(fixtags.GapFillFlag, 'Y' if isGapFill else 'N')
# msg.setField(fixtags.MsgSeqNum, respondingTo[fixtags.BeginSeqNo])
# return msg
@staticmethod
def resend_request(beginSeqNo, endSeqNo = '0'):
msg = FIXMessage(msgtype.RESENDREQUEST)
msg.setField(fixtags.BeginSeqNo, str(beginSeqNo))
msg.setField(fixtags.EndSeqNo, str(endSeqNo))
return msg | {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
Account = "1"
AdvId = "2"
AdvRefID = "3"
AdvSide = "4"
AdvTransType = "5"
AvgPx = "6"
BeginSeqNo = "7"
BeginString = "8"
BodyLength = "9"
CheckSum = "10"
ClOrdID = "11"
Commission = "12"
CommType = "13"
CumQty = "14"
Currency = "15"
EndSeqNo = "16"
ExecID = "17"
ExecInst = "18"
ExecRefID = "19"
ExecTransType = "20"
HandlInst = "21"
SecurityIDSource = "22"
IOIID = "23"
IOIOthSvc = "24"
IOIQltyInd = "25"
IOIRefID = "26"
IOIQty = "27"
IOITransType = "28"
LastCapacity = "29"
LastMkt = "30"
LastPx = "31"
LastQty = "32"
NoLinesOfText = "33"
MsgSeqNum = "34"
MsgType = "35"
NewSeqNo = "36"
OrderID = "37"
OrderQty = "38"
OrdStatus = "39"
OrdType = "40"
OrigClOrdID = "41"
OrigTime = "42"
PossDupFlag = "43"
Price = "44"
RefSeqNum = "45"
RelatdSym = "46"
Rule80A = "47"
SecurityID = "48"
SenderCompID = "49"
SenderSubID = "50"
SendingDate = "51"
SendingTime = "52"
Quantity = "53"
Side = "54"
Symbol = "55"
TargetCompID = "56"
TargetSubID = "57"
Text = "58"
TimeInForce = "59"
TransactTime = "60"
Urgency = "61"
ValidUntilTime = "62"
SettlType = "63"
SettlDate = "64"
SymbolSfx = "65"
ListID = "66"
ListSeqNo = "67"
TotNoOrders = "68"
ListExecInst = "69"
AllocID = "70"
AllocTransType = "71"
RefAllocID = "72"
NoOrders = "73"
AvgPxPrecision = "74"
TradeDate = "75"
ExecBroker = "76"
PositionEffect = "77"
NoAllocs = "78"
AllocAccount = "79"
AllocQty = "80"
ProcessCode = "81"
NoRpts = "82"
RptSeq = "83"
CxlQty = "84"
NoDlvyInst = "85"
DlvyInst = "86"
AllocStatus = "87"
AllocRejCode = "88"
Signature = "89"
SecureDataLen = "90"
SecureData = "91"
BrokerOfCredit = "92"
SignatureLength = "93"
EmailType = "94"
RawDataLength = "95"
RawData = "96"
PossResend = "97"
EncryptMethod = "98"
StopPx = "99"
ExDestination = "100"
CxlRejReason = "102"
OrdRejReason = "103"
IOIQualifier = "104"
WaveNo = "105"
Issuer = "106"
SecurityDesc = "107"
HeartBtInt = "108"
ClientID = "109"
MinQty = "110"
MaxFloor = "111"
TestReqID = "112"
ReportToExch = "113"
LocateReqd = "114"
OnBehalfOfCompID = "115"
OnBehalfOfSubID = "116"
QuoteID = "117"
NetMoney = "118"
SettlCurrAmt = "119"
SettlCurrency = "120"
ForexReq = "121"
OrigSendingTime = "122"
GapFillFlag = "123"
NoExecs = "124"
CxlType = "125"
ExpireTime = "126"
DKReason = "127"
DeliverToCompID = "128"
DeliverToSubID = "129"
IOINaturalFlag = "130"
QuoteReqID = "131"
BidPx = "132"
OfferPx = "133"
BidSize = "134"
OfferSize = "135"
NoMiscFees = "136"
MiscFeeAmt = "137"
MiscFeeCurr = "138"
MiscFeeType = "139"
PrevClosePx = "140"
ResetSeqNumFlag = "141"
SenderLocationID = "142"
TargetLocationID = "143"
OnBehalfOfLocationID = "144"
DeliverToLocationID = "145"
NoRelatedSym = "146"
Subject = "147"
Headline = "148"
URLLink = "149"
ExecType = "150"
LeavesQty = "151"
CashOrderQty = "152"
AllocAvgPx = "153"
AllocNetMoney = "154"
SettlCurrFxRate = "155"
SettlCurrFxRateCalc = "156"
NumDaysInterest = "157"
AccruedInterestRate = "158"
AccruedInterestAmt = "159"
SettlInstMode = "160"
AllocText = "161"
SettlInstID = "162"
SettlInstTransType = "163"
EmailThreadID = "164"
SettlInstSource = "165"
SettlLocation = "166"
SecurityType = "167"
EffectiveTime = "168"
StandInstDbType = "169"
StandInstDbName = "170"
StandInstDbID = "171"
SettlDeliveryType = "172"
SettlDepositoryCode = "173"
SettlBrkrCode = "174"
SettlInstCode = "175"
SecuritySettlAgentName = "176"
SecuritySettlAgentCode = "177"
SecuritySettlAgentAcctNum = "178"
SecuritySettlAgentAcctName = "179"
SecuritySettlAgentContactName = "180"
SecuritySettlAgentContactPhone = "181"
CashSettlAgentName = "182"
CashSettlAgentCode = "183"
CashSettlAgentAcctNum = "184"
CashSettlAgentAcctName = "185"
CashSettlAgentContactName = "186"
CashSettlAgentContactPhone = "187"
BidSpotRate = "188"
BidForwardPoints = "189"
OfferSpotRate = "190"
OfferForwardPoints = "191"
OrderQty2 = "192"
SettlDate2 = "193"
LastSpotRate = "194"
LastForwardPoints = "195"
AllocLinkID = "196"
AllocLinkType = "197"
SecondaryOrderID = "198"
NoIOIQualifiers = "199"
MaturityMonthYear = "200"
PutOrCall = "201"
StrikePrice = "202"
CoveredOrUncovered = "203"
CustomerOrFirm = "204"
MaturityDay = "205"
OptAttribute = "206"
SecurityExchange = "207"
NotifyBrokerOfCredit = "208"
AllocHandlInst = "209"
MaxShow = "210"
PegOffsetValue = "211"
XmlDataLen = "212"
XmlData = "213"
SettlInstRefID = "214"
NoRoutingIDs = "215"
RoutingType = "216"
RoutingID = "217"
Spread = "218"
Benchmark = "219"
BenchmarkCurveCurrency = "220"
BenchmarkCurveName = "221"
BenchmarkCurvePoint = "222"
CouponRate = "223"
CouponPaymentDate = "224"
IssueDate = "225"
RepurchaseTerm = "226"
RepurchaseRate = "227"
Factor = "228"
TradeOriginationDate = "229"
ExDate = "230"
ContractMultiplier = "231"
NoStipulations = "232"
StipulationType = "233"
StipulationValue = "234"
YieldType = "235"
Yield = "236"
TotalTakedown = "237"
Concession = "238"
RepoCollateralSecurityType = "239"
RedemptionDate = "240"
UnderlyingCouponPaymentDate = "241"
UnderlyingIssueDate = "242"
UnderlyingRepoCollateralSecurityType = "243"
UnderlyingRepurchaseTerm = "244"
UnderlyingRepurchaseRate = "245"
UnderlyingFactor = "246"
UnderlyingRedemptionDate = "247"
LegCouponPaymentDate = "248"
LegIssueDate = "249"
LegRepoCollateralSecurityType = "250"
LegRepurchaseTerm = "251"
LegRepurchaseRate = "252"
LegFactor = "253"
LegRedemptionDate = "254"
CreditRating = "255"
UnderlyingCreditRating = "256"
LegCreditRating = "257"
TradedFlatSwitch = "258"
BasisFeatureDate = "259"
BasisFeaturePrice = "260"
MDReqID = "262"
SubscriptionRequestType = "263"
MarketDepth = "264"
MDUpdateType = "265"
AggregatedBook = "266"
NoMDEntryTypes = "267"
NoMDEntries = "268"
MDEntryType = "269"
MDEntryPx = "270"
MDEntrySize = "271"
MDEntryDate = "272"
MDEntryTime = "273"
TickDirection = "274"
MDMkt = "275"
QuoteCondition = "276"
TradeCondition = "277"
MDEntryID = "278"
MDUpdateAction = "279"
MDEntryRefID = "280"
MDReqRejReason = "281"
MDEntryOriginator = "282"
LocationID = "283"
DeskID = "284"
DeleteReason = "285"
OpenCloseSettlFlag = "286"
SellerDays = "287"
MDEntryBuyer = "288"
MDEntrySeller = "289"
MDEntryPositionNo = "290"
FinancialStatus = "291"
CorporateAction = "292"
DefBidSize = "293"
DefOfferSize = "294"
NoQuoteEntries = "295"
NoQuoteSets = "296"
QuoteStatus = "297"
QuoteCancelType = "298"
QuoteEntryID = "299"
QuoteRejectReason = "300"
QuoteResponseLevel = "301"
QuoteSetID = "302"
QuoteRequestType = "303"
TotNoQuoteEntries = "304"
UnderlyingSecurityIDSource = "305"
UnderlyingIssuer = "306"
UnderlyingSecurityDesc = "307"
UnderlyingSecurityExchange = "308"
UnderlyingSecurityID = "309"
UnderlyingSecurityType = "310"
UnderlyingSymbol = "311"
UnderlyingSymbolSfx = "312"
UnderlyingMaturityMonthYear = "313"
UnderlyingMaturityDay = "314"
UnderlyingPutOrCall = "315"
UnderlyingStrikePrice = "316"
UnderlyingOptAttribute = "317"
UnderlyingCurrency = "318"
RatioQty = "319"
SecurityReqID = "320"
SecurityRequestType = "321"
SecurityResponseID = "322"
SecurityResponseType = "323"
SecurityStatusReqID = "324"
UnsolicitedIndicator = "325"
SecurityTradingStatus = "326"
HaltReason = "327"
InViewOfCommon = "328"
DueToRelated = "329"
BuyVolume = "330"
SellVolume = "331"
HighPx = "332"
LowPx = "333"
Adjustment = "334"
TradSesReqID = "335"
TradingSessionID = "336"
ContraTrader = "337"
TradSesMethod = "338"
TradSesMode = "339"
TradSesStatus = "340"
TradSesStartTime = "341"
TradSesOpenTime = "342"
TradSesPreCloseTime = "343"
TradSesCloseTime = "344"
TradSesEndTime = "345"
NumberOfOrders = "346"
MessageEncoding = "347"
EncodedIssuerLen = "348"
EncodedIssuer = "349"
EncodedSecurityDescLen = "350"
EncodedSecurityDesc = "351"
EncodedListExecInstLen = "352"
EncodedListExecInst = "353"
EncodedTextLen = "354"
EncodedText = "355"
EncodedSubjectLen = "356"
EncodedSubject = "357"
EncodedHeadlineLen = "358"
EncodedHeadline = "359"
EncodedAllocTextLen = "360"
EncodedAllocText = "361"
EncodedUnderlyingIssuerLen = "362"
EncodedUnderlyingIssuer = "363"
EncodedUnderlyingSecurityDescLen = "364"
EncodedUnderlyingSecurityDesc = "365"
AllocPrice = "366"
QuoteSetValidUntilTime = "367"
QuoteEntryRejectReason = "368"
LastMsgSeqNumProcessed = "369"
OnBehalfOfSendingTime = "370"
RefTagID = "371"
RefMsgType = "372"
SessionRejectReason = "373"
BidRequestTransType = "374"
ContraBroker = "375"
ComplianceID = "376"
SolicitedFlag = "377"
ExecRestatementReason = "378"
BusinessRejectRefID = "379"
BusinessRejectReason = "380"
GrossTradeAmt = "381"
NoContraBrokers = "382"
MaxMessageSize = "383"
NoMsgTypes = "384"
MsgDirection = "385"
NoTradingSessions = "386"
TotalVolumeTraded = "387"
DiscretionInst = "388"
DiscretionOffsetValue = "389"
BidID = "390"
ClientBidID = "391"
ListName = "392"
TotNoRelatedSym = "393"
BidType = "394"
NumTickets = "395"
SideValue1 = "396"
SideValue2 = "397"
NoBidDescriptors = "398"
BidDescriptorType = "399"
BidDescriptor = "400"
SideValueInd = "401"
LiquidityPctLow = "402"
LiquidityPctHigh = "403"
LiquidityValue = "404"
EFPTrackingError = "405"
FairValue = "406"
OutsideIndexPct = "407"
ValueOfFutures = "408"
LiquidityIndType = "409"
WtAverageLiquidity = "410"
ExchangeForPhysical = "411"
OutMainCntryUIndex = "412"
CrossPercent = "413"
ProgRptReqs = "414"
ProgPeriodInterval = "415"
IncTaxInd = "416"
NumBidders = "417"
BidTradeType = "418"
BasisPxType = "419"
NoBidComponents = "420"
Country = "421"
TotNoStrikes = "422"
PriceType = "423"
DayOrderQty = "424"
DayCumQty = "425"
DayAvgPx = "426"
GTBookingInst = "427"
NoStrikes = "428"
ListStatusType = "429"
NetGrossInd = "430"
ListOrderStatus = "431"
ExpireDate = "432"
ListExecInstType = "433"
CxlRejResponseTo = "434"
UnderlyingCouponRate = "435"
UnderlyingContractMultiplier = "436"
ContraTradeQty = "437"
ContraTradeTime = "438"
ClearingFirm = "439"
ClearingAccount = "440"
LiquidityNumSecurities = "441"
MultiLegReportingType = "442"
StrikeTime = "443"
ListStatusText = "444"
EncodedListStatusTextLen = "445"
EncodedListStatusText = "446"
PartyIDSource = "447"
PartyID = "448"
TotalVolumeTradedDate = "449"
TotalVolumeTraded = "450"
NetChgPrevDay = "451"
PartyRole = "452"
NoPartyIDs = "453"
NoSecurityAltID = "454"
SecurityAltID = "455"
SecurityAltIDSource = "456"
NoUnderlyingSecurityAltID = "457"
UnderlyingSecurityAltID = "458"
UnderlyingSecurityAltIDSource = "459"
Product = "460"
CFICode = "461"
UnderlyingProduct = "462"
UnderlyingCFICode = "463"
TestMessageIndicator = "464"
QuantityType = "465"
BookingRefID = "466"
IndividualAllocID = "467"
RoundingDirection = "468"
RoundingModulus = "469"
CountryOfIssue = "470"
StateOrProvinceOfIssue = "471"
LocaleOfIssue = "472"
NoRegistDtls = "473"
MailingDtls = "474"
InvestorCountryOfResidence = "475"
PaymentRef = "476"
DistribPaymentMethod = "477"
CashDistribCurr = "478"
CommCurrency = "479"
CancellationRights = "480"
MoneyLaunderingStatus = "481"
MailingInst = "482"
TransBkdTime = "483"
ExecPriceType = "484"
ExecPriceAdjustment = "485"
DateOfBirth = "486"
TradeReportTransType = "487"
CardHolderName = "488"
CardNumber = "489"
CardExpDate = "490"
CardIssNum = "491"
PaymentMethod = "492"
RegistAcctType = "493"
Designation = "494"
TaxAdvantageType = "495"
RegistRejReasonText = "496"
FundRenewWaiv = "497"
CashDistribAgentName = "498"
CashDistribAgentCode = "499"
CashDistribAgentAcctNumber = "500"
CashDistribPayRef = "501"
CashDistribAgentAcctName = "502"
CardStartDate = "503"
PaymentDate = "504"
PaymentRemitterID = "505"
RegistStatus = "506"
RegistRejReasonCode = "507"
RegistRefID = "508"
RegistDtls = "509"
NoDistribInsts = "510"
RegistEmail = "511"
DistribPercentage = "512"
RegistID = "513"
RegistTransType = "514"
ExecValuationPoint = "515"
OrderPercent = "516"
OwnershipType = "517"
NoContAmts = "518"
ContAmtType = "519"
ContAmtValue = "520"
ContAmtCurr = "521"
OwnerType = "522"
PartySubID = "523"
NestedPartyID = "524"
NestedPartyIDSource = "525"
SecondaryClOrdID = "526"
SecondaryExecID = "527"
OrderCapacity = "528"
OrderRestrictions = "529"
MassCancelRequestType = "530"
MassCancelResponse = "531"
MassCancelRejectReason = "532"
TotalAffectedOrders = "533"
NoAffectedOrders = "534"
AffectedOrderID = "535"
AffectedSecondaryOrderID = "536"
QuoteType = "537"
NestedPartyRole = "538"
NoNestedPartyIDs = "539"
TotalAccruedInterestAmt = "540"
MaturityDate = "541"
UnderlyingMaturityDate = "542"
InstrRegistry = "543"
CashMargin = "544"
NestedPartySubID = "545"
Scope = "546"
MDImplicitDelete = "547"
CrossID = "548"
CrossType = "549"
CrossPrioritization = "550"
OrigCrossID = "551"
NoSides = "552"
Username = "553"
Password = "554"
NoLegs = "555"
LegCurrency = "556"
TotNoSecurityTypes = "557"
NoSecurityTypes = "558"
SecurityListRequestType = "559"
SecurityRequestResult = "560"
RoundLot = "561"
MinTradeVol = "562"
MultiLegRptTypeReq = "563"
LegPositionEffect = "564"
LegCoveredOrUncovered = "565"
LegPrice = "566"
TradSesStatusRejReason = "567"
TradeRequestID = "568"
TradeRequestType = "569"
PreviouslyReported = "570"
TradeReportID = "571"
TradeReportRefID = "572"
MatchStatus = "573"
MatchType = "574"
OddLot = "575"
NoClearingInstructions = "576"
ClearingInstruction = "577"
TradeInputSource = "578"
TradeInputDevice = "579"
NoDates = "580"
AccountType = "581"
CustOrderCapacity = "582"
ClOrdLinkID = "583"
MassStatusReqID = "584"
MassStatusReqType = "585"
OrigOrdModTime = "586"
LegSettlType = "587"
LegSettlDate = "588"
DayBookingInst = "589"
BookingUnit = "590"
PreallocMethod = "591"
UnderlyingCountryOfIssue = "592"
UnderlyingStateOrProvinceOfIssue = "593"
UnderlyingLocaleOfIssue = "594"
UnderlyingInstrRegistry = "595"
LegCountryOfIssue = "596"
LegStateOrProvinceOfIssue = "597"
LegLocaleOfIssue = "598"
LegInstrRegistry = "599"
LegSymbol = "600"
LegSymbolSfx = "601"
LegSecurityID = "602"
LegSecurityIDSource = "603"
NoLegSecurityAltID = "604"
LegSecurityAltID = "605"
LegSecurityAltIDSource = "606"
LegProduct = "607"
LegCFICode = "608"
LegSecurityType = "609"
LegMaturityMonthYear = "610"
LegMaturityDate = "611"
LegStrikePrice = "612"
LegOptAttribute = "613"
LegContractMultiplier = "614"
LegCouponRate = "615"
LegSecurityExchange = "616"
LegIssuer = "617"
EncodedLegIssuerLen = "618"
EncodedLegIssuer = "619"
LegSecurityDesc = "620"
EncodedLegSecurityDescLen = "621"
EncodedLegSecurityDesc = "622"
LegRatioQty = "623"
LegSide = "624"
TradingSessionSubID = "625"
AllocType = "626"
NoHops = "627"
HopCompID = "628"
HopSendingTime = "629"
HopRefID = "630"
MidPx = "631"
BidYield = "632"
MidYield = "633"
OfferYield = "634"
ClearingFeeIndicator = "635"
WorkingIndicator = "636"
LegLastPx = "637"
PriorityIndicator = "638"
PriceImprovement = "639"
Price2 = "640"
LastForwardPoints2 = "641"
BidForwardPoints2 = "642"
OfferForwardPoints2 = "643"
RFQReqID = "644"
MktBidPx = "645"
MktOfferPx = "646"
MinBidSize = "647"
MinOfferSize = "648"
QuoteStatusReqID = "649"
LegalConfirm = "650"
UnderlyingLastPx = "651"
UnderlyingLastQty = "652"
SecDefStatus = "653"
LegRefID = "654"
ContraLegRefID = "655"
SettlCurrBidFxRate = "656"
SettlCurrOfferFxRate = "657"
QuoteRequestRejectReason = "658"
SideComplianceID = "659"
AcctIDSource = "660"
AllocAcctIDSource = "661"
BenchmarkPrice = "662"
BenchmarkPriceType = "663"
ConfirmID = "664"
ConfirmStatus = "665"
ConfirmTransType = "666"
ContractSettlMonth = "667"
DeliveryForm = "668"
LastParPx = "669"
NoLegAllocs = "670"
LegAllocAccount = "671"
LegIndividualAllocID = "672"
LegAllocQty = "673"
LegAllocAcctIDSource = "674"
LegSettlCurrency = "675"
LegBenchmarkCurveCurrency = "676"
LegBenchmarkCurveName = "677"
LegBenchmarkCurvePoint = "678"
LegBenchmarkPrice = "679"
LegBenchmarkPriceType = "680"
LegBidPx = "681"
LegIOIQty = "682"
NoLegStipulations = "683"
LegOfferPx = "684"
LegOrderQty = "685"
LegPriceType = "686"
LegQty = "687"
LegStipulationType = "688"
LegStipulationValue = "689"
LegSwapType = "690"
Pool = "691"
QuotePriceType = "692"
QuoteRespID = "693"
QuoteRespType = "694"
QuoteQualifier = "695"
YieldRedemptionDate = "696"
YieldRedemptionPrice = "697"
YieldRedemptionPriceType = "698"
BenchmarkSecurityID = "699"
ReversalIndicator = "700"
YieldCalcDate = "701"
NoPositions = "702"
PosType = "703"
LongQty = "704"
ShortQty = "705"
PosQtyStatus = "706"
PosAmtType = "707"
PosAmt = "708"
PosTransType = "709"
PosReqID = "710"
NoUnderlyings = "711"
PosMaintAction = "712"
OrigPosReqRefID = "713"
PosMaintRptRefID = "714"
ClearingBusinessDate = "715"
SettlSessID = "716"
SettlSessSubID = "717"
AdjustmentType = "718"
ContraryInstructionIndicator = "719"
PriorSpreadIndicator = "720"
PosMaintRptID = "721"
PosMaintStatus = "722"
PosMaintResult = "723"
PosReqType = "724"
ResponseTransportType = "725"
ResponseDestination = "726"
TotalNumPosReports = "727"
PosReqResult = "728"
PosReqStatus = "729"
SettlPrice = "730"
SettlPriceType = "731"
UnderlyingSettlPrice = "732"
UnderlyingSettlPriceType = "733"
PriorSettlPrice = "734"
NoQuoteQualifiers = "735"
AllocSettlCurrency = "736"
AllocSettlCurrAmt = "737"
InterestAtMaturity = "738"
LegDatedDate = "739"
LegPool = "740"
AllocInterestAtMaturity = "741"
AllocAccruedInterestAmt = "742"
DeliveryDate = "743"
AssignmentMethod = "744"
AssignmentUnit = "745"
OpenInterest = "746"
ExerciseMethod = "747"
TotNumTradeReports = "748"
TradeRequestResult = "749"
TradeRequestStatus = "750"
TradeReportRejectReason = "751"
SideMultiLegReportingType = "752"
NoPosAmt = "753"
AutoAcceptIndicator = "754"
AllocReportID = "755"
NoNested2PartyIDs = "756"
Nested2PartyID = "757"
Nested2PartyIDSource = "758"
Nested2PartyRole = "759"
Nested2PartySubID = "760"
BenchmarkSecurityIDSource = "761"
SecuritySubType = "762"
UnderlyingSecuritySubType = "763"
LegSecuritySubType = "764"
AllowableOneSidednessPct = "765"
AllowableOneSidednessValue = "766"
AllowableOneSidednessCurr = "767"
NoTrdRegTimestamps = "768"
TrdRegTimestamp = "769"
TrdRegTimestampType = "770"
TrdRegTimestampOrigin = "771"
ConfirmRefID = "772"
ConfirmType = "773"
ConfirmRejReason = "774"
BookingType = "775"
IndividualAllocRejCode = "776"
SettlInstMsgID = "777"
NoSettlInst = "778"
LastUpdateTime = "779"
AllocSettlInstType = "780"
NoSettlPartyIDs = "781"
SettlPartyID = "782"
SettlPartyIDSource = "783"
SettlPartyRole = "784"
SettlPartySubID = "785"
SettlPartySubIDType = "786"
DlvyInstType = "787"
TerminationType = "788"
NextExpectedMsgSeqNum = "789"
OrdStatusReqID = "790"
SettlInstReqID = "791"
SettlInstReqRejCode = "792"
SecondaryAllocID = "793"
AllocReportType = "794"
AllocReportRefID = "795"
AllocCancReplaceReason = "796"
CopyMsgIndicator = "797"
AllocAccountType = "798"
OrderAvgPx = "799"
OrderBookingQty = "800"
NoSettlPartySubIDs = "801"
NoPartySubIDs = "802"
PartySubIDType = "803"
NoNestedPartySubIDs = "804"
NestedPartySubIDType = "805"
NoNested2PartySubIDs = "806"
Nested2PartySubIDType = "807"
AllocIntermedReqType = "808"
UnderlyingPx = "810"
PriceDelta = "811"
ApplQueueMax = "812"
ApplQueueDepth = "813"
ApplQueueResolution = "814"
ApplQueueAction = "815"
NoAltMDSource = "816"
AltMDSourceID = "817"
SecondaryTradeReportID = "818"
AvgPxIndicator = "819"
TradeLinkID = "820"
OrderInputDevice = "821"
UnderlyingTradingSessionID = "822"
UnderlyingTradingSessionSubID = "823"
TradeLegRefID = "824"
ExchangeRule = "825"
TradeAllocIndicator = "826"
ExpirationCycle = "827"
TrdType = "828"
TrdSubType = "829"
TransferReason = "830"
AsgnReqID = "831"
TotNumAssignmentReports = "832"
AsgnRptID = "833"
ThresholdAmount = "834"
PegMoveType = "835"
PegOffsetType = "836"
PegLimitType = "837"
PegRoundDirection = "838"
PeggedPrice = "839"
PegScope = "840"
DiscretionMoveType = "841"
DiscretionOffsetType = "842"
DiscretionLimitType = "843"
DiscretionRoundDirection = "844"
DiscretionPrice = "845"
DiscretionScope = "846"
TargetStrategy = "847"
TargetStrategyParameters = "848"
ParticipationRate = "849"
TargetStrategyPerformance = "850"
LastLiquidityInd = "851"
PublishTrdIndicator = "852"
ShortSaleReason = "853"
QtyType = "854"
SecondaryTrdType = "855"
TradeReportType = "856"
AllocNoOrdersType = "857"
SharedCommission = "858"
ConfirmReqID = "859"
AvgParPx = "860"
ReportedPx = "861"
NoCapacities = "862"
OrderCapacityQty = "863"
NoEvents = "864"
EventType = "865"
EventDate = "866"
EventPx = "867"
EventText = "868"
PctAtRisk = "869"
NoInstrAttrib = "870"
InstrAttribType = "871"
InstrAttribValue = "872"
DatedDate = "873"
InterestAccrualDate = "874"
CPProgram = "875"
CPRegType = "876"
UnderlyingCPProgram = "877"
UnderlyingCPRegType = "878"
UnderlyingQty = "879"
TrdMatchID = "880"
SecondaryTradeReportRefID = "881"
UnderlyingDirtyPrice = "882"
UnderlyingEndPrice = "883"
UnderlyingStartValue = "884"
UnderlyingCurrentValue = "885"
UnderlyingEndValue = "886"
NoUnderlyingStips = "887"
UnderlyingStipType = "888"
UnderlyingStipValue = "889"
MaturityNetMoney = "890"
MiscFeeBasis = "891"
TotNoAllocs = "892"
LastFragment = "893"
CollReqID = "894"
CollAsgnReason = "895"
CollInquiryQualifier = "896"
NoTrades = "897"
MarginRatio = "898"
MarginExcess = "899"
TotalNetValue = "900"
CashOutstanding = "901"
CollAsgnID = "902"
CollAsgnTransType = "903"
CollRespID = "904"
CollAsgnRespType = "905"
CollAsgnRejectReason = "906"
CollAsgnRefID = "907"
CollRptID = "908"
CollInquiryID = "909"
CollStatus = "910"
TotNumReports = "911"
LastRptRequested = "912"
AgreementDesc = "913"
AgreementID = "914"
AgreementDate = "915"
StartDate = "916"
EndDate = "917"
AgreementCurrency = "918"
DeliveryType = "919"
EndAccruedInterestAmt = "920"
StartCash = "921"
EndCash = "922"
UserRequestID = "923"
UserRequestType = "924"
NewPassword = "925"
UserStatus = "926"
UserStatusText = "927"
StatusValue = "928"
StatusText = "929"
RefCompID = "930"
RefSubID = "931"
NetworkResponseID = "932"
NetworkRequestID = "933"
LastNetworkResponseID = "934"
NetworkRequestType = "935"
NoCompIDs = "936"
NetworkStatusResponseType = "937"
NoCollInquiryQualifier = "938"
TrdRptStatus = "939"
AffirmStatus = "940"
UnderlyingStrikeCurrency = "941"
LegStrikeCurrency = "942"
TimeBracket = "943"
CollAction = "944"
CollInquiryStatus = "945"
CollInquiryResult = "946"
StrikeCurrency = "947"
NoNested3PartyIDs = "948"
Nested3PartyID = "949"
Nested3PartyIDSource = "950"
Nested3PartyRole = "951"
NoNested3PartySubIDs = "952"
Nested3PartySubID = "953"
Nested3PartySubIDType = "954"
LegContractSettlMonth = "955"
LegInterestAccrualDate = "956"
tags = {}
for x in dir():
tags[str(globals()[x])] = x
def tagToName(n):
try:
return tags[n]
except KeyError:
return str(n)
def repeatingGroupIdentifiers():
return {
NoSecurityAltID : [SecurityAltID, SecurityAltIDSource],
NoMiscFees : [MiscFeeAmt, MiscFeeCurr, MiscFeeType, MiscFeeBasis],
NoClearingInstructions : [ClearingInstruction, ],
NoEvents : [EventType, EventDate, EventPx, EventText],
NoInstrAttrib : [InstrAttribType, InstrAttribValue],
NoLegSecurityAltID : [LegSecurityAltID, LegSecurityAltIDSource],
NoLegStipulations : [LegStipulationType, LegStipulationValue],
NoNestedPartyIDs : [NestedPartyID, NestedPartyIDSource, NestedPartyRole, NoNestedPartySubIDs],
NoNestedPartySubIDs : [NestedPartySubID, NestedPartySubIDType],
NoNested2PartyIDs : [Nested2PartyID, Nested2PartyIDSource, Nested2PartyRole, NoNested2PartySubIDs],
NoNested2PartySubIDs : [Nested2PartySubID, Nested2PartySubIDType],
NoNested3PartyIDs : [Nested3PartyID, Nested3PartyIDSource, Nested3PartyRole, NoNested3PartySubIDs],
NoNested3PartySubIDs : [Nested3PartySubID, Nested3PartySubIDType],
NoPartyIDs : [PartyID, PartyIDSource, PartyRole, NoPartySubIDs],
NoPartySubIDs : [PartySubID, PartySubIDType],
NoPosAmt : [PosAmtType, PosAmt],
NoPositions : [PosType, LongQty, ShortQty, PosQtyStatus],
NoDlvyInst : [SettlInstSource, DlvyInstType, NoSettlPartyIDs],
NoSettlPartyIDs : [SettlPartyID, SettlPartyIDSource, SettlPartyRole, NoSettlPartySubIDs],
NoSettlPartySubIDs : [SettlPartySubID, SettlPartySubIDType],
NoStipulations : [StipulationType, StipulationValue],
NoTrdRegTimestamps : [TrdRegTimestamp, TrdRegTimestampType, TrdRegTimestampOrigin],
NoUnderlyingSecurityAltID : [UnderlyingSecurityAltID, UnderlyingSecurityAltIDSource],
NoUnderlyingStips : [UnderlyingStipType, UnderlyingStipValue],
NoOrders : [ClOrdID, OrderID, SecondaryOrderID, SecondaryClOrdID, ListID, OrderQty, OrderAvgPx, OrderBookingQty],
NoExecs : [LastQty, ExecID, SecondaryExecID, LastPx, LastParPx, LastCapacity],
NoUnderlyings : [UnderlyingSymbol, UnderlyingSymbolSfx, UnderlyingSecurityID, UnderlyingSecurityIDSource, NoUnderlyingSecurityAltID, UnderlyingProduct, UnderlyingCFICode, UnderlyingSecurityType, UnderlyingSecuritySubType, UnderlyingMaturityMonthYear, UnderlyingMaturityDate, UnderlyingPutOrCall, UnderlyingCouponPaymentDate, UnderlyingIssueDate, UnderlyingRepoCollateralSecurityType, UnderlyingRepurchaseTerm, UnderlyingRepurchaseRate, UnderlyingFactor, UnderlyingCreditRating, UnderlyingInstrRegistry, UnderlyingCountryOfIssue, UnderlyingStateOrProvinceOfIssue, UnderlyingLocaleOfIssue, UnderlyingRedemptionDate, UnderlyingStrikePrice, UnderlyingStrikeCurrency, UnderlyingOptAttribute, UnderlyingContractMultiplier, UnderlyingCouponRate, UnderlyingSecurityExchange, UnderlyingIssuer, EncodedUnderlyingIssuerLen, EncodedUnderlyingIssuer, UnderlyingSecurityDesc, EncodedUnderlyingSecurityDescLen, EncodedUnderlyingSecurityDesc, UnderlyingCPProgram, UnderlyingCPRegType, UnderlyingCurrency, UnderlyingQty, UnderlyingPx, UnderlyingDirtyPrice, UnderlyingEndPrice, UnderlyingStartValue, UnderlyingCurrentValue, UnderlyingEndValue, NoUnderlyingStips],
NoAllocs : [AllocAccount, AllocAcctIDSource, MatchStatus, AllocPrice, AllocQty, IndividualAllocID, ProcessCode, NoNestedPartyIDs, NotifyBrokerOfCredit, AllocHandlInst, AllocText, EncodedAllocTextLen, EncodedAllocText, Commission, CommType, CommCurrency, FundRenewWaiv, AllocAvgPx, AllocNetMoney, SettlCurrAmt, AllocSettlCurrAmt, SettlCurrency, AllocSettlCurrency, SettlCurrFxRate, SettlCurrFxRateCalc, AllocAccruedInterestAmt, AllocInterestAtMaturity, NoMiscFees, NoClearingInstructions, AllocSettlInstType, SettlDeliveryType, StandInstDbType, StandInstDbName, StandInstDbID, NoDlvyInst],
NoLegs : [LegSymbol, LegSymbolSfx, LegSecurityID, LegSecurityIDSource, NoLegSecurityAltID, LegProduct, LegCFICode, LegSecurityType, LegSecuritySubType, LegMaturityMonthYear, LegMaturityDate, LegCouponPaymentDate, LegIssueDate, LegRepoCollateralSecurityType, LegRepurchaseTerm, LegRepurchaseRate, LegFactor, LegCreditRating, LegInstrRegistry, LegCountryOfIssue, LegStateOrProvinceOfIssue, LegLocaleOfIssue, LegRedemptionDate, LegStrikePrice, LegStrikeCurrency, LegOptAttribute, LegContractMultiplier, LegCouponRate, LegSecurityExchange, LegIssuer, EncodedIssuerLen, EncodedLegIssuer, LegSecurityDesc, EncodedLegSecurityDescLen, EncodedLegSecurityDesc, LegRatioQty, LegSide, LegCurrency, LegPool, LegDatedDate, LegContractSettlMonth, LegInterestAccrualDate]
} | {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
HEARTBEAT = "0"
TESTREQUEST = "1"
RESENDREQUEST = "2"
REJECT = "3"
SEQUENCERESET = "4"
LOGOUT = "5"
IOI = "6"
ADVERTISEMENT = "7"
EXECUTIONREPORT = "8"
ORDERCANCELREJECT = "9"
QUOTESTATUSREQUEST = "a"
LOGON = "A"
DERIVATIVESECURITYLIST = "AA"
NEWORDERMULTILEG = "AB"
MULTILEGORDERCANCELREPLACE = "AC"
TRADECAPTUREREPORTREQUEST = "AD"
TRADECAPTUREREPORT = "AE"
ORDERMASSSTATUSREQUEST = "AF"
QUOTEREQUESTREJECT = "AG"
RFQREQUEST = "AH"
QUOTESTATUSREPORT = "AI"
QUOTERESPONSE = "AJ"
CONFIRMATION = "AK"
POSITIONMAINTENANCEREQUEST = "AL"
POSITIONMAINTENANCEREPORT = "AM"
REQUESTFORPOSITIONS = "AN"
REQUESTFORPOSITIONSACK = "AO"
POSITIONREPORT = "AP"
TRADECAPTUREREPORTREQUESTACK = "AQ"
TRADECAPTUREREPORTACK = "AR"
ALLOCATIONREPORT = "AS"
ALLOCATIONREPORTACK = "AT"
CONFIRMATIONACK = "AU"
SETTLEMENTINSTRUCTIONREQUEST = "AV"
ASSIGNMENTREPORT = "AW"
COLLATERALREQUEST = "AX"
COLLATERALASSIGNMENT = "AY"
COLLATERALRESPONSE = "AZ"
NEWS = "B"
MASSQUOTEACKNOWLEDGEMENT = "b"
COLLATERALREPORT = "BA"
COLLATERALINQUIRY = "BB"
NETWORKCOUNTERPARTYSYSTEMSTATUSREQUEST = "BC"
NETWORKCOUNTERPARTYSYSTEMSTATUSRESPONSE = "BD"
USERREQUEST = "BE"
USERRESPONSE = "BF"
COLLATERALINQUIRYACK = "BG"
CONFIRMATIONREQUEST = "BH"
EMAIL = "C"
SECURITYDEFINITIONREQUEST = "c"
SECURITYDEFINITION = "d"
NEWORDERSINGLE = "D"
SECURITYSTATUSREQUEST = "e"
NEWORDERLIST = "E"
ORDERCANCELREQUEST = "F"
SECURITYSTATUS = "f"
ORDERCANCELREPLACEREQUEST = "G"
TRADINGSESSIONSTATUSREQUEST = "g"
ORDERSTATUSREQUEST = "H"
TRADINGSESSIONSTATUS = "h"
MASSQUOTE = "i"
BUSINESSMESSAGEREJECT = "j"
ALLOCATIONINSTRUCTION = "J"
BIDREQUEST = "k"
LISTCANCELREQUEST = "K"
BIDRESPONSE = "l"
LISTEXECUTE = "L"
LISTSTRIKEPRICE = "m"
LISTSTATUSREQUEST = "M"
XMLNONFIX = "n"
LISTSTATUS = "N"
REGISTRATIONINSTRUCTIONS = "o"
REGISTRATIONINSTRUCTIONSRESPONSE = "p"
ALLOCATIONINSTRUCTIONACK = "P"
ORDERMASSCANCELREQUEST = "q"
DONTKNOWTRADEDK = "Q"
QUOTEREQUEST = "R"
ORDERMASSCANCELREPORT = "r"
QUOTE = "S"
NEWORDERCROSS = "s"
SETTLEMENTINSTRUCTIONS = "T"
CROSSORDERCANCELREPLACEREQUEST = "t"
CROSSORDERCANCELREQUEST = "u"
MARKETDATAREQUEST = "V"
SECURITYTYPEREQUEST = "v"
SECURITYTYPES = "w"
MARKETDATASNAPSHOTFULLREFRESH = "W"
SECURITYLISTREQUEST = "x"
MARKETDATAINCREMENTALREFRESH = "X"
MARKETDATAREQUESTREJECT = "Y"
SECURITYLIST = "y"
QUOTECANCEL = "Z"
DERIVATIVESECURITYLISTREQUEST = "z"
sessionMessageTypes = [HEARTBEAT, TESTREQUEST, RESENDREQUEST, REJECT, SEQUENCERESET, LOGOUT, LOGON, XMLNONFIX]
tags = {}
for x in dir():
tags[str(globals()[x])] = x
def msgTypeToName(n):
try:
return tags[n]
except KeyError:
return str(n)
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
import unittest
from pyfix.connection import MessageDirection
from pyfix.journaler import Journaler
from pyfix.message import FIXMessage, FIXContext
from pyfix.session import FIXSession
class JournalerTests(unittest.TestCase):
def testAddExtractMsg(self):
journal = Journaler()
msg = FIXMessage("AB")
msg.setField("45", "dgd")
msg.setField("32", "aaaa")
msg.setField("323", "bbbb")
rptgrp1 = FIXContext()
rptgrp1.setField("611", "aaa")
rptgrp1.setField("612", "bbb")
rptgrp1.setField("613", "ccc")
msg.addRepeatingGroup("444", rptgrp1, 0)
session = FIXSession(1, "S1", "T1")
for i in range(0, 5):
msg.setField("34", str(i))
journal.persistMsg(msg, session, MessageDirection.OUTBOUND)
msg = journal.recoverMsg(session, MessageDirection.OUTBOUND, 1)
def testAddExtractMultipleMsgs(self):
journal = Journaler()
msg = FIXMessage("AB")
msg.setField("45", "dgd")
msg.setField("32", "aaaa")
msg.setField("323", "bbbb")
rptgrp1 = FIXContext()
rptgrp1.setField("611", "aaa")
rptgrp1.setField("612", "bbb")
rptgrp1.setField("613", "ccc")
msg.addRepeatingGroup("444", rptgrp1, 0)
session = FIXSession(1, "S1", "T1")
for i in range(0, 5):
msg.setField("34", str(i))
journal.persistMsg(msg, session, MessageDirection.OUTBOUND)
msgs = journal.recoverMsgs(session, MessageDirection.OUTBOUND, 0, 4)
for i in range(0, len(msgs)):
msg.setField("34", str(i))
self.assertEqual(msg, msgs[i])
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
import datetime
import unittest
from pyfix.event import EventManager, TimerEventRegistration
class EventTimerTests(unittest.TestCase):
def testTimerEvent(self):
mgr = EventManager()
endTime = None
t1 = TimerEventRegistration(lambda fire, closure: self.assertEqual(int((datetime.datetime.utcnow() - closure).total_seconds()), 1), 1.0, datetime.datetime.utcnow())
mgr.registerHandler(t1)
mgr.waitForEventWithTimeout(5.0)
def testTimerEventReset(self):
mgr = EventManager()
t1 = TimerEventRegistration(lambda fire, closure: self.assertEqual(int((datetime.datetime.utcnow() - closure).total_seconds()), 2), 1.0, datetime.datetime.utcnow())
mgr.registerHandler(t1)
mgr.registerHandler(TimerEventRegistration(lambda fire, closure: t1.reset(), 0.9))
for i in range(0, 3):
mgr.waitForEventWithTimeout(10.0)
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
import importlib
import datetime
import mock as mock
from pyfix.codec import Codec
from pyfix.message import FIXMessage, FIXContext
__author__ = 'tom'
import unittest
class FakeDate(datetime.datetime):
@classmethod
def utcnow(cls):
return cls(2015, 6, 19, 11, 8, 54)
class FIXCodecTests(unittest.TestCase):
def testDecode(self):
protocol = importlib.import_module("pyfix.FIX44")
codec = Codec(protocol)
inMsg = b'8=FIX.4.4\x019=817\x0135=J\x0134=953\x0149=FIX_ALAUDIT\x0156=BFUT_ALAUDIT\x0143=N\x0152=20150615-09:21:42.459\x0170=00000002664ASLO1001\x01626=2\x0110626=5\x0171=0\x0160=20150615-10:21:42\x01857=1\x0173=1\x0111=00000006321ORLO1\x0138=100.0\x01800=100.0\x01124=1\x0132=100.0\x0117=00000009758TRLO1\x0131=484.50\x0154=2\x0153=100.0\x0155=FTI\x01207=XEUE\x01454=1\x01455=EOM5\x01456=A\x01200=201506\x01541=20150619\x01461=FXXXXX\x016=484.50\x0174=2\x0175=20150615\x0178=2\x0179=TEST123\x0130009=12345\x01467=00000014901CALO1001\x019520=00000014898CALO1\x0180=33.0\x01366=484.50\x0181=0\x01153=484.50\x0110626=5\x0179=TEST124\x0130009=12345\x01467=00000014903CALO1001\x019520=00000014899CALO1\x0180=67.0\x01366=484.50\x0181=0\x01153=484.50\x0110626=5\x01453=3\x01448=TEST1\x01447=D\x01452=3\x01802=2\x01523=12345\x01803=3\x01523=TEST1\x01803=19\x01448=TEST1WA\x01447=D\x01452=38\x01802=4\x01523=Test1 Wait\x01803=10\x01523= \x01803=26\x01523=\x01803=3\x01523=TestWaCRF2\x01803=28\x01448=hagap\x01447=D\x01452=11\x01802=2\x01523=GB\x01803=25\x01523=BarCapFutures.FETService\x01803=24\x0110=033\x01'
msg, remaining = codec.decode(inMsg)
self.assertEqual("8=FIX.4.4|9=817|35=J|34=953|49=FIX_ALAUDIT|56=BFUT_ALAUDIT|43=N|52=20150615-09:21:42.459|70=00000002664ASLO1001|626=2|10626=5|71=0|60=20150615-10:21:42|857=1|73=1=>[11=00000006321ORLO1|38=100.0|800=100.0]|124=1=>[32=100.0|17=00000009758TRLO1|31=484.50]|54=2|53=100.0|55=FTI|207=XEUE|454=1=>[455=EOM5|456=A]|200=201506|541=20150619|461=FXXXXX|6=484.50|74=2|75=20150615|78=1=>[79=TEST123]|30009=12345|467=00000014903CALO1001|9520=00000014899CALO1|80=67.0|366=484.50|81=0|153=484.50|79=TEST124|453=3=>[448=TEST1|447=D|452=3|802=2=>[523=12345|803=3, 523=TEST1|803=19], 448=TEST1WA|447=D|452=38|802=4=>[523=Test1 Wait|803=10, 523= |803=26, 523=|803=3, 523=TestWaCRF2|803=28], 448=hagap|447=D|452=11|802=2=>[523=GB|803=25, 523=BarCapFutures.FETService|803=24]]|10=033", str(msg))
@mock.patch("pyfix.codec.datetime", FakeDate)
def testEncode(self):
from datetime import datetime
print("%s" % (datetime.utcnow()))
mock_session = mock.Mock()
mock_session.senderCompId = "sender"
mock_session.targetCompId = "target"
mock_session.allocateSndSeqNo.return_value = 1
protocol = importlib.import_module("pyfix.FIX44")
codec = Codec(protocol)
msg = FIXMessage(codec.protocol.msgtype.NEWORDERSINGLE)
msg.setField(codec.protocol.fixtags.Price, "123.45")
msg.setField(codec.protocol.fixtags.OrderQty, 9876)
msg.setField(codec.protocol.fixtags.Symbol, "VOD.L")
msg.setField(codec.protocol.fixtags.SecurityID, "GB00BH4HKS39")
msg.setField(codec.protocol.fixtags.SecurityIDSource, "4")
msg.setField(codec.protocol.fixtags.Symbol, "VOD.L")
msg.setField(codec.protocol.fixtags.Account, "TEST")
msg.setField(codec.protocol.fixtags.HandlInst, "1")
msg.setField(codec.protocol.fixtags.ExDestination, "XLON")
msg.setField(codec.protocol.fixtags.Side, 1)
msg.setField(codec.protocol.fixtags.ClOrdID, "abcdefg")
msg.setField(codec.protocol.fixtags.Currency, "GBP")
rptgrp1 = FIXContext()
rptgrp1.setField("611", "aaa")
rptgrp1.setField("612", "bbb")
rptgrp1.setField("613", "ccc")
msg.addRepeatingGroup("444", rptgrp1, 0)
rptgrp2 = FIXContext()
rptgrp2.setField("611", "zzz")
rptgrp2.setField("612", "yyy")
rptgrp2.setField("613", "xxx")
msg.addRepeatingGroup("444", rptgrp2, 1)
result = codec.encode(msg, mock_session)
expected = '8=FIX.4.4\x019=201\x0135=D\x0149=sender\x0156=target\x0134=1\x0152=20150619-11:08:54.000\x0144=123.45\x0138=9876\x0155=VOD.L\x0148=GB00BH4HKS39\x0122=4\x011=TEST\x0121=1\x01100=XLON\x0154=1\x0111=abcdefg\x0115=GBP\x01444=2\x01611=aaa\x01612=bbb\x01613=ccc\x01611=zzz\x01612=yyy\x01613=xxx\x0110=255\x01'
self.assertEqual(expected, result)
if __name__ == '__main__':
unittest.main()
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
import pickle
from pyfix.message import FIXMessage, FIXContext
__author__ = 'tom'
import unittest
class FIXMessageTests(unittest.TestCase):
def testMsgConstruction(self):
msg = FIXMessage("AB")
msg.setField("45", "dgd")
msg.setField("32", "aaaa")
msg.setField("323", "bbbb")
rptgrp1 = FIXContext()
rptgrp1.setField("611", "aaa")
rptgrp1.setField("612", "bbb")
rptgrp1.setField("613", "ccc")
msg.addRepeatingGroup("444", rptgrp1, 0)
rptgrp2 = FIXContext()
rptgrp2.setField("611", "zzz")
rptgrp2.setField("612", "yyy")
rptgrp2.setField("613", "xxx")
msg.addRepeatingGroup("444", rptgrp2, 1)
self.assertEqual("45=dgd|32=aaaa|323=bbbb|444=2=>[611=aaa|612=bbb|613=ccc, 611=zzz|612=yyy|613=xxx]", str(msg))
msg.removeRepeatingGroupByIndex("444", 1)
self.assertEqual("45=dgd|32=aaaa|323=bbbb|444=1=>[611=aaa|612=bbb|613=ccc]", str(msg))
msg.addRepeatingGroup("444", rptgrp2, 1)
rptgrp3 = FIXContext()
rptgrp3.setField("611", "ggg")
rptgrp3.setField("612", "hhh")
rptgrp3.setField("613", "jjj")
rptgrp2.addRepeatingGroup("445", rptgrp3, 0)
self.assertEqual("45=dgd|32=aaaa|323=bbbb|444=2=>[611=aaa|612=bbb|613=ccc, 611=zzz|612=yyy|613=xxx|445=1=>[611=ggg|612=hhh|613=jjj]]", str(msg))
grp = msg.getRepeatingGroupByTag("444", "612", "yyy")
self.assertEqual("611=zzz|612=yyy|613=xxx|445=1=>[611=ggg|612=hhh|613=jjj]", str(grp))
def testPickle(self):
msg = FIXMessage("AB")
msg.setField("45", "dgd")
msg.setField("32", "aaaa")
msg.setField("323", "bbbb")
rptgrp1 = FIXContext()
rptgrp1.setField("611", "aaa")
rptgrp1.setField("612", "bbb")
rptgrp1.setField("613", "ccc")
msg.addRepeatingGroup("444", rptgrp1, 0)
str = pickle.dumps(msg)
msg2 = pickle.loads(str)
self.assertEqual(msg, msg2)
if __name__ == '__main__':
unittest.main()
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
import argparse
import logging
from pyfix.journaler import Journaler
from pyfix.message import MessageDirection
def main():
logging.basicConfig(format='%(asctime)s %(message)s', level=logging.INFO)
parser = argparse.ArgumentParser(description='Examine the contents of the store file.')
group = parser.add_mutually_exclusive_group()
group.add_argument('-l', '--list', dest='list', action='store_true', help='list available streams')
group.add_argument('-s', '--sessions', dest='sessions', nargs='+', action='store', metavar=('s1', 's2'), help='session to examine')
parser.add_argument('filename', action='store', help='filename of the store file')
parser.add_argument('-d', '--direction', dest='direction', choices=['in', 'out', 'both'], action='store', default="both", help='filename of the store file')
args = parser.parse_args()
journal = Journaler(args.filename)
if args.list is True:
# list all sessions
row_format ="{:^15}|" * 3
separator_format ="{:->15}|" * 3
for session in journal.sessions():
print(row_format.format("Session Id", "TargetCompId", "SenderCompId"))
print(separator_format.format("", "", ""))
print(row_format.format(session.key, session.targetCompId, session.senderCompId))
print(separator_format.format("", "", ""))
else:
# list all messages in that stream
direction = None if args.direction == 'both' else MessageDirection.INBOUND if args.direction == "in" else MessageDirection.OUTBOUND
for (seqNo, msg, msgDirection, session) in journal.getAllMsgs(args.sessions, direction):
d = "---->" if msgDirection == MessageDirection.OUTBOUND.value else "<----"
print("{:>3} {:^5} [{:>5}] {}".format(session, d, seqNo, msg))
if __name__ == '__main__':
main()
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
from enum import Enum
import logging
from pyfix.connection import ConnectionState, MessageDirection
from pyfix.engine import FIXEngine
from pyfix.message import FIXMessage
from pyfix.server_connection import FIXServer
class Side(Enum):
buy = 1
sell = 2
class Server(FIXEngine):
def __init__(self):
FIXEngine.__init__(self, "server_example.store")
# create a FIX Server using the FIX 4.4 standard
self.server = FIXServer(self, "pyfix.FIX44")
# we register some listeners since we want to know when the connection goes up or down
self.server.addConnectionListener(self.onConnect, ConnectionState.CONNECTED)
self.server.addConnectionListener(self.onDisconnect, ConnectionState.DISCONNECTED)
# start our event listener indefinitely
self.server.start('', int("9898"))
while True:
self.eventManager.waitForEventWithTimeout(10.0)
# some clean up before we shut down
self.server.removeConnectionListener(self.onConnect, ConnectionState.CONNECTED)
self.server.removeConnectionListener(self.onConnect, ConnectionState.DISCONNECTED)
def validateSession(self, targetCompId, senderCompId):
logging.info("Received login request for %s / %s" % (senderCompId, targetCompId))
return True
def onConnect(self, session):
logging.info("Accepted new connection from %s" % (session.address(), ))
# register to receive message notifications on the session which has just been created
session.addMessageHandler(self.onLogin, MessageDirection.OUTBOUND, self.server.protocol.msgtype.LOGON)
session.addMessageHandler(self.onNewOrder, MessageDirection.INBOUND, self.server.protocol.msgtype.NEWORDERSINGLE)
def onDisconnect(self, session):
logging.info("%s has disconnected" % (session.address(), ))
# we need to clean up our handlers, since this session is disconnected now
session.removeMessageHandler(self.onLogin, MessageDirection.OUTBOUND, self.server.protocol.msgtype.LOGON)
session.removeMessageHandler(self.onNewOrder, MessageDirection.INBOUND, self.server.protocol.msgtype.NEWORDERSINGLE)
def onLogin(self, connectionHandler, msg):
codec = connectionHandler.codec
logging.info("[" + msg[codec.protocol.fixtags.SenderCompID] + "] <---- " + codec.protocol.msgtype.msgTypeToName(msg[codec.protocol.fixtags.MsgType]))
def onNewOrder(self, connectionHandler, request):
codec = connectionHandler.codec
try:
side = Side(int(request.getField(codec.protocol.fixtags.Side)))
logging.debug("<--- [%s] %s: %s %s %s@%s" % (codec.protocol.msgtype.msgTypeToName(request.getField(codec.protocol.fixtags.MsgType)), request.getField(codec.protocol.fixtags.ClOrdID), request.getField(codec.protocol.fixtags.Symbol), side.name, request.getField(codec.protocol.fixtags.OrderQty), request.getField(codec.protocol.fixtags.Price)))
# respond with an ExecutionReport Ack
msg = FIXMessage(codec.protocol.msgtype.EXECUTIONREPORT)
msg.setField(codec.protocol.fixtags.Price, request.getField(codec.protocol.fixtags.Price))
msg.setField(codec.protocol.fixtags.OrderQty, request.getField(codec.protocol.fixtags.OrderQty))
msg.setField(codec.protocol.fixtags.Symbol, request.getField(codec.protocol.fixtags.OrderQty))
msg.setField(codec.protocol.fixtags.SecurityID, "GB00BH4HKS39")
msg.setField(codec.protocol.fixtags.SecurityIDSource, "4")
msg.setField(codec.protocol.fixtags.Symbol, request.getField(codec.protocol.fixtags.Symbol))
msg.setField(codec.protocol.fixtags.Account, request.getField(codec.protocol.fixtags.Account))
msg.setField(codec.protocol.fixtags.HandlInst, "1")
msg.setField(codec.protocol.fixtags.OrdStatus, "0")
msg.setField(codec.protocol.fixtags.ExecType, "0")
msg.setField(codec.protocol.fixtags.LeavesQty, "0")
msg.setField(codec.protocol.fixtags.Side, request.getField(codec.protocol.fixtags.Side))
msg.setField(codec.protocol.fixtags.ClOrdID, request.getField(codec.protocol.fixtags.ClOrdID))
msg.setField(codec.protocol.fixtags.Currency, request.getField(codec.protocol.fixtags.Currency))
connectionHandler.sendMsg(msg)
logging.debug("---> [%s] %s: %s %s %s@%s" % (codec.protocol.msgtype.msgTypeToName(msg.msgType), msg.getField(codec.protocol.fixtags.ClOrdID), request.getField(codec.protocol.fixtags.Symbol), side.name, request.getField(codec.protocol.fixtags.OrderQty), request.getField(codec.protocol.fixtags.Price)))
except Exception as e:
msg = FIXMessage(codec.protocol.msgtype.EXECUTIONREPORT)
msg.setField(codec.protocol.fixtags.OrdStatus, "4")
msg.setField(codec.protocol.fixtags.ExecType, "4")
msg.setField(codec.protocol.fixtags.LeavesQty, "0")
msg.setField(codec.protocol.fixtags.Text, str(e))
msg.setField(codec.protocol.fixtags.ClOrdID, request.getField(codec.protocol.fixtags.ClOrdID))
connectionHandler.sendMsg(msg)
def main():
logging.basicConfig(format='%(asctime)s %(message)s', level=logging.DEBUG)
server = Server()
logging.info("All done... shutting down")
if __name__ == '__main__':
main()
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
import logging
class tag(object):
def __init__(self, name, value, values):
self.name = name
self.value = value
for k, v in values:
setattr(self, k, v)
def __eq__(self, other):
if type(other) == tag:
return other.name == self.name and other.value == self.value
else:
return self.value == other
def __str__(self):
return self.value
def __hash__(self):
return hash(self.name + self.value)
__repr__ = __str__
class msgtype(object):
pass
class tags(object):
def __init__(self):
self.tags = {}
self._addTag("MsgType", "35", [("ExecutionReport", "8"), ("NewOrderSingle", "D")])
def _addTag(self, key, value, values):
t = tag(key, value, values)
setattr(self, key, t)
self.tags[t] = key
class Demo(object):
def __init__(self):
t =tags()
logging.debug("%s = %s" % (t.tags[t.MsgType], t.MsgType))
logging.debug("ExecutionReport: %s" % (t.MsgType.ExecutionReport,))
def main():
logging.basicConfig(format='%(asctime)s %(message)s', level=logging.DEBUG)
server = Demo()
logging.info("All done... shutting down")
if __name__ == '__main__':
main()
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
from enum import Enum
import logging
import random
from pyfix.connection import ConnectionState, MessageDirection
from pyfix.client_connection import FIXClient
from pyfix.engine import FIXEngine
from pyfix.message import FIXMessage
from pyfix.event import TimerEventRegistration
class Side(Enum):
buy = 1
sell = 2
class Client(FIXEngine):
def __init__(self):
FIXEngine.__init__(self, "client_example.store")
self.clOrdID = 0
self.msgGenerator = None
# create a FIX Client using the FIX 4.4 standard
self.client = FIXClient(self, "pyfix.FIX44", "TARGET", "SENDER")
# we register some listeners since we want to know when the connection goes up or down
self.client.addConnectionListener(self.onConnect, ConnectionState.CONNECTED)
self.client.addConnectionListener(self.onDisconnect, ConnectionState.DISCONNECTED)
# start our event listener indefinitely
self.client.start('localhost', int("9898"))
while True:
self.eventManager.waitForEventWithTimeout(10.0)
# some clean up before we shut down
self.client.removeConnectionListener(self.onConnect, ConnectionState.CONNECTED)
self.client.removeConnectionListener(self.onConnect, ConnectionState.DISCONNECTED)
def onConnect(self, session):
logging.info("Established connection to %s" % (session.address(), ))
# register to receive message notifications on the session which has just been created
session.addMessageHandler(self.onLogin, MessageDirection.INBOUND, self.client.protocol.msgtype.LOGON)
session.addMessageHandler(self.onExecutionReport, MessageDirection.INBOUND, self.client.protocol.msgtype.EXECUTIONREPORT)
def onDisconnect(self, session):
logging.info("%s has disconnected" % (session.address(), ))
# we need to clean up our handlers, since this session is disconnected now
session.removeMessageHandler(self.onLogin, MessageDirection.INBOUND, self.client.protocol.msgtype.LOGON)
session.removeMessageHandler(self.onExecutionReport, MessageDirection.INBOUND, self.client.protocol.msgtype.EXECUTIONREPORT)
if self.msgGenerator:
self.eventManager.unregisterHandler(self.msgGenerator)
def sendOrder(self, connectionHandler):
self.clOrdID = self.clOrdID + 1
codec = connectionHandler.codec
msg = FIXMessage(codec.protocol.msgtype.NEWORDERSINGLE)
msg.setField(codec.protocol.fixtags.Price, "%0.2f" % (random.random() * 2 + 10))
msg.setField(codec.protocol.fixtags.OrderQty, int(random.random() * 100))
msg.setField(codec.protocol.fixtags.Symbol, "VOD.L")
msg.setField(codec.protocol.fixtags.SecurityID, "GB00BH4HKS39")
msg.setField(codec.protocol.fixtags.SecurityIDSource, "4")
msg.setField(codec.protocol.fixtags.Account, "TEST")
msg.setField(codec.protocol.fixtags.HandlInst, "1")
msg.setField(codec.protocol.fixtags.ExDestination, "XLON")
msg.setField(codec.protocol.fixtags.Side, int(random.random() * 2) + 1)
msg.setField(codec.protocol.fixtags.ClOrdID, str(self.clOrdID))
msg.setField(codec.protocol.fixtags.Currency, "GBP")
connectionHandler.sendMsg(msg)
side = Side(int(msg.getField(codec.protocol.fixtags.Side)))
logging.debug("---> [%s] %s: %s %s %s@%s" % (codec.protocol.msgtype.msgTypeToName(msg.msgType), msg.getField(codec.protocol.fixtags.ClOrdID), msg.getField(codec.protocol.fixtags.Symbol), side.name, msg.getField(codec.protocol.fixtags.OrderQty), msg.getField(codec.protocol.fixtags.Price)))
def onLogin(self, connectionHandler, msg):
logging.info("Logged in")
# lets do something like send and order every 3 seconds
self.msgGenerator = TimerEventRegistration(lambda type, closure: self.sendOrder(closure), 0.5, connectionHandler)
self.eventManager.registerHandler(self.msgGenerator)
def onExecutionReport(self, connectionHandler, msg):
codec = connectionHandler.codec
if codec.protocol.fixtags.ExecType in msg:
if msg.getField(codec.protocol.fixtags.ExecType) == "0":
side = Side(int(msg.getField(codec.protocol.fixtags.Side)))
logging.debug("<--- [%s] %s: %s %s %s@%s" % (codec.protocol.msgtype.msgTypeToName(msg.getField(codec.protocol.fixtags.MsgType)), msg.getField(codec.protocol.fixtags.ClOrdID), msg.getField(codec.protocol.fixtags.Symbol), side.name, msg.getField(codec.protocol.fixtags.OrderQty), msg.getField(codec.protocol.fixtags.Price)))
elif msg.getField(codec.protocol.fixtags.ExecType) == "4":
reason = "Unknown" if codec.protocol.fixtags.Text not in msg else msg.getField(codec.protocol.fixtags.Text)
logging.info("Order Rejected '%s'" % (reason,))
else:
logging.error("Received execution report without ExecType")
def main():
logging.basicConfig(format='%(asctime)s %(message)s', level=logging.DEBUG)
client = Client()
logging.info("All done... shutting down")
if __name__ == '__main__':
main()
| {
"repo_name": "wannabegeek/PyFIX",
"stars": "96",
"repo_language": "Python",
"file_name": "client_example.py",
"mime_type": "text/x-script.python"
} |
CC = cc -std=c99
CFLAGS = -Wall -Wextra -O3 -g3 -march=native
all: benchmark tests
benchmark: test/benchmark.c utf8.h test/utf8-encode.h test/bh-utf8.h
$(CC) $(CFLAGS) $(LDFLAGS) -o $@ test/benchmark.c $(LDLIBS)
tests: test/tests.c utf8.h test/utf8-encode.h
$(CC) $(CFLAGS) $(LDFLAGS) -o $@ test/tests.c $(LDLIBS)
bench: benchmark
./benchmark
check: tests
./tests
clean:
rm -f benchmark tests
| {
"repo_name": "skeeto/branchless-utf8",
"stars": "547",
"repo_language": "C",
"file_name": "utf8-encode.h",
"mime_type": "text/x-c"
} |
/* Branchless UTF-8 decoder
*
* This is free and unencumbered software released into the public domain.
*/
#ifndef UTF8_H
#define UTF8_H
#include <stdint.h>
/* Decode the next character, C, from BUF, reporting errors in E.
*
* Since this is a branchless decoder, four bytes will be read from the
* buffer regardless of the actual length of the next character. This
* means the buffer _must_ have at least three bytes of zero padding
* following the end of the data stream.
*
* Errors are reported in E, which will be non-zero if the parsed
* character was somehow invalid: invalid byte sequence, non-canonical
* encoding, or a surrogate half.
*
* The function returns a pointer to the next character. When an error
* occurs, this pointer will be a guess that depends on the particular
* error, but it will always advance at least one byte.
*/
static void *
utf8_decode(void *buf, uint32_t *c, int *e)
{
static const char lengths[] = {
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
0, 0, 0, 0, 0, 0, 0, 0, 2, 2, 2, 2, 3, 3, 4, 0
};
static const int masks[] = {0x00, 0x7f, 0x1f, 0x0f, 0x07};
static const uint32_t mins[] = {4194304, 0, 128, 2048, 65536};
static const int shiftc[] = {0, 18, 12, 6, 0};
static const int shifte[] = {0, 6, 4, 2, 0};
unsigned char *s = buf;
int len = lengths[s[0] >> 3];
/* Compute the pointer to the next character early so that the next
* iteration can start working on the next character. Neither Clang
* nor GCC figure out this reordering on their own.
*/
unsigned char *next = s + len + !len;
/* Assume a four-byte character and load four bytes. Unused bits are
* shifted out.
*/
*c = (uint32_t)(s[0] & masks[len]) << 18;
*c |= (uint32_t)(s[1] & 0x3f) << 12;
*c |= (uint32_t)(s[2] & 0x3f) << 6;
*c |= (uint32_t)(s[3] & 0x3f) << 0;
*c >>= shiftc[len];
/* Accumulate the various error conditions. */
*e = (*c < mins[len]) << 6; // non-canonical encoding
*e |= ((*c >> 11) == 0x1b) << 7; // surrogate half?
*e |= (*c > 0x10FFFF) << 8; // out of range?
*e |= (s[1] & 0xc0) >> 2;
*e |= (s[2] & 0xc0) >> 4;
*e |= (s[3] ) >> 6;
*e ^= 0x2a; // top two bits of each tail byte correct?
*e >>= shifte[len];
return next;
}
#endif
| {
"repo_name": "skeeto/branchless-utf8",
"stars": "547",
"repo_language": "C",
"file_name": "utf8-encode.h",
"mime_type": "text/x-c"
} |
# Branchless UTF-8 Decoder
Full article:
[A Branchless UTF-8 Decoder](http://nullprogram.com/blog/2017/10/06/)
## Example usage
```c
#define N (1 << 20) // 1 MiB
// input buffer with 3 bytes of zero padding
char buf[N+3];
char *end = buf + fread(buf, 1, N, stdin);
end[0] = end[1] = end[2] = 0;
// output buffer: parsed code points
int len = 0;
uint32_t cp[N];
int errors = 0;
for (char *p = buf; p < end;) {
int e;
p = utf8_decode(p, cp+len++, &e);
errors |= e;
}
if (errors) {
// decode failure
}
```
| {
"repo_name": "skeeto/branchless-utf8",
"stars": "547",
"repo_language": "C",
"file_name": "utf8-encode.h",
"mime_type": "text/x-c"
} |
#define _POSIX_C_SOURCE 200112L
#include <stdio.h>
#include <stdint.h>
#include <stdlib.h>
#include <signal.h>
#include <unistd.h> // alarm()
#include "../utf8.h"
#include "utf8-encode.h"
#include "bh-utf8.h"
#define SECONDS 6
#define BUFLEN 8 // MB
static uint32_t
pcg32(uint64_t *s)
{
uint64_t m = 0x9b60933458e17d7d;
uint64_t a = 0xd737232eeccdf7ed;
*s = *s * m + a;
int shift = 29 - (*s >> 61);
return *s >> shift;
}
/* Generate a random codepoint whose UTF-8 length is uniformly selected. */
static long
randchar(uint64_t *s)
{
uint32_t r = pcg32(s);
int len = 1 + (r & 0x3);
r >>= 2;
switch (len) {
case 1:
return r % 128;
case 2:
return 128 + r % (2048 - 128);
case 3:
return 2048 + r % (65536 - 2048);
case 4:
return 65536 + r % (131072 - 65536);
}
abort();
}
static volatile sig_atomic_t running;
static void
alarm_handler(int signum)
{
(void)signum;
running = 0;
}
/* Fill buffer with random characters, with evenly-distributed encoded
* lengths.
*/
static void *
buffer_fill(void *buf, size_t z)
{
uint64_t s = 0;
char *p = buf;
char *end = p + z;
while (p < end) {
long c;
do
c = randchar(&s);
while (IS_SURROGATE(c));
p = utf8_encode(p, c);
}
return p;
}
static unsigned char *
utf8_simple(unsigned char *s, long *c)
{
unsigned char *next;
if (s[0] < 0x80) {
*c = s[0];
next = s + 1;
} else if ((s[0] & 0xe0) == 0xc0) {
*c = ((long)(s[0] & 0x1f) << 6) |
((long)(s[1] & 0x3f) << 0);
if ((s[1] & 0xc0) != 0x80)
*c = -1;
next = s + 2;
} else if ((s[0] & 0xf0) == 0xe0) {
*c = ((long)(s[0] & 0x0f) << 12) |
((long)(s[1] & 0x3f) << 6) |
((long)(s[2] & 0x3f) << 0);
if ((s[1] & 0xc0) != 0x80 ||
(s[2] & 0xc0) != 0x80)
*c = -1;
next = s + 3;
} else if ((s[0] & 0xf8) == 0xf0 && (s[0] <= 0xf4)) {
*c = ((long)(s[0] & 0x07) << 18) |
((long)(s[1] & 0x3f) << 12) |
((long)(s[2] & 0x3f) << 6) |
((long)(s[3] & 0x3f) << 0);
if ((s[1] & 0xc0) != 0x80 ||
(s[2] & 0xc0) != 0x80 ||
(s[3] & 0xc0) != 0x80)
*c = -1;
next = s + 4;
} else {
*c = -1; // invalid
next = s + 1; // skip this byte
}
if (*c >= 0xd800 && *c <= 0xdfff)
*c = -1; // surrogate half
return next;
}
int
main(void)
{
double rate;
long errors, n;
size_t z = BUFLEN * 1024L * 1024;
unsigned char *buffer = malloc(z);
unsigned char *end = buffer_fill(buffer, z - 4);
/* Benchmark the branchless decoder */
running = 1;
signal(SIGALRM, alarm_handler);
alarm(SECONDS);
errors = n = 0;
do {
unsigned char *p = buffer;
int e = 0;
uint32_t c;
long count = 0;
while (p < end) {
p = utf8_decode(p, &c, &e);
errors += !!e; // force errors to be checked
count++;
}
if (p == end) // reached the end successfully?
n++;
} while (running);
rate = n * (end - buffer) / (double)SECONDS / 1024 / 1024;
printf("branchless: %f MB/s, %ld errors\n", rate, errors);
/* Benchmark Bjoern Hoehrmann's decoder */
running = 1;
signal(SIGALRM, alarm_handler);
alarm(SECONDS);
errors = n = 0;
do {
unsigned char *p = buffer;
uint32_t c;
uint32_t state = 0;
long count = 0;
for (; p < end; p++) {
if (!bh_utf8_decode(&state, &c, *p))
count++;
else if (state == UTF8_REJECT)
errors++; // force errors to be checked
}
if (p == end) // reached the end successfully?
n++;
} while (running);
rate = n * (end - buffer) / (double)SECONDS / 1024 / 1024;
printf("Hoehrmann: %f MB/s, %ld errors\n", rate, errors);
/* Benchmark simple decoder */
running = 1;
signal(SIGALRM, alarm_handler);
alarm(SECONDS);
errors = n = 0;
do {
unsigned char *p = buffer;
long c;
long count = 0;
while (p < end) {
p = utf8_simple(p, &c);
count++;
if (c < 0)
errors++;
}
if (p == end) // reached the end successfully?
n++;
} while (running);
rate = n * (end - buffer) / (double)SECONDS / 1024 / 1024;
printf("Simple: %f MB/s, %ld errors\n", rate, errors);
free(buffer);
}
| {
"repo_name": "skeeto/branchless-utf8",
"stars": "547",
"repo_language": "C",
"file_name": "utf8-encode.h",
"mime_type": "text/x-c"
} |
// Copyright (c) 2008-2009 Bjoern Hoehrmann <[email protected]>
// See http://bjoern.hoehrmann.de/utf-8/decoder/dfa/ for details.
#ifdef BH_ORIGINAL
#define UTF8_ACCEPT 0
#define UTF8_REJECT 1
static const uint8_t utf8d[] = {
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, // 00..1f
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, // 20..3f
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, // 40..5f
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, // 60..7f
1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9, // 80..9f
7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7, // a0..bf
8,8,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2, // c0..df
0xa,0x3,0x3,0x3,0x3,0x3,0x3,0x3,0x3,0x3,0x3,0x3,0x3,0x4,0x3,0x3, // e0..ef
0xb,0x6,0x6,0x6,0x5,0x8,0x8,0x8,0x8,0x8,0x8,0x8,0x8,0x8,0x8,0x8, // f0..ff
0x0,0x1,0x2,0x3,0x5,0x8,0x7,0x1,0x1,0x1,0x4,0x6,0x1,0x1,0x1,0x1, // s0..s0
1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,0,1,0,1,1,1,1,1,1, // s1..s2
1,2,1,1,1,1,1,2,1,2,1,1,1,1,1,1,1,1,1,1,1,1,1,2,1,1,1,1,1,1,1,1, // s3..s4
1,2,1,1,1,1,1,1,1,2,1,1,1,1,1,1,1,1,1,1,1,1,1,3,1,3,1,1,1,1,1,1, // s5..s6
1,3,1,1,1,1,1,3,1,3,1,1,1,1,1,1,1,3,1,1,1,1,1,1,1,1,1,1,1,1,1,1, // s7..s8
};
static uint32_t
bh_utf8_decode(uint32_t* state, uint32_t* codep, uint32_t byte) {
uint32_t type = utf8d[byte];
*codep = (*state != UTF8_ACCEPT) ?
(byte & 0x3fu) | (*codep << 6) :
(0xff >> type) & (byte);
*state = utf8d[256 + *state*16 + type];
return *state;
}
#else
#define UTF8_ACCEPT 0
#define UTF8_REJECT 12
static const uint8_t utf8d[] = {
// The first part of the table maps bytes to character classes that
// to reduce the size of the transition table and create bitmasks.
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1, 9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,
7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7, 7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,
8,8,2,2,2,2,2,2,2,2,2,2,2,2,2,2, 2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,
10,3,3,3,3,3,3,3,3,3,3,3,3,4,3,3, 11,6,6,6,5,8,8,8,8,8,8,8,8,8,8,8,
// The second part is a transition table that maps a combination
// of a state of the automaton and a character class to a state.
0,12,24,36,60,96,84,12,12,12,48,72, 12,12,12,12,12,12,12,12,12,12,12,12,
12, 0,12,12,12,12,12, 0,12, 0,12,12, 12,24,12,12,12,12,12,24,12,24,12,12,
12,12,12,12,12,12,12,24,12,12,12,12, 12,24,12,12,12,12,12,12,12,24,12,12,
12,12,12,12,12,12,12,36,12,36,12,12, 12,36,12,12,12,12,12,36,12,36,12,12,
12,36,12,12,12,12,12,12,12,12,12,12,
};
static uint32_t
bh_utf8_decode(uint32_t* state, uint32_t* codep, uint32_t byte) {
uint32_t type = utf8d[byte];
*codep = (*state != UTF8_ACCEPT) ?
(byte & 0x3fu) | (*codep << 6) :
(0xff >> type) & (byte);
*state = utf8d[256 + *state + type];
return *state;
}
#endif
| {
"repo_name": "skeeto/branchless-utf8",
"stars": "547",
"repo_language": "C",
"file_name": "utf8-encode.h",
"mime_type": "text/x-c"
} |
#include <stdio.h>
#include "../utf8.h"
#include "utf8-encode.h"
static int count_pass;
static int count_fail;
#define TEST(x, s, ...) \
do { \
if (x) { \
printf("\033[32;1mPASS\033[0m " s "\n", __VA_ARGS__); \
count_pass++; \
} else { \
printf("\033[31;1mFAIL\033[0m " s "\n", __VA_ARGS__); \
count_fail++; \
} \
} while (0)
int
main(void)
{
/* Make sure it can decode every character */
{
long failures = 0;
for (unsigned long i = 0; i < 0x10ffff; i++) {
if (!IS_SURROGATE(i)) {
int e;
uint32_t c;
unsigned char buf[8] = {0};
unsigned char *end = utf8_encode(buf, i);
unsigned char *res = utf8_decode(buf, &c, &e);
failures += end != res || c != i || e;
}
}
TEST(failures == 0, "decode all, errors: %ld", failures);
}
/* Reject everything outside of U+0000..U+10FFFF */
{
long failures = 0;
for (unsigned long i = 0x110000; i < 0x1fffff; i++) {
int e;
uint32_t c;
unsigned char buf[8] = {0};
utf8_encode(buf, i);
unsigned char *end = utf8_decode(buf, &c, &e);
failures += !e;
failures += end - buf != 4;
}
TEST(failures == 0, "out of range, errors: %ld", failures);
}
/* Does it reject all surrogate halves? */
{
long failures = 0;
for (unsigned long i = 0xd800; i <= 0xdfff; i++) {
int e;
uint32_t c;
unsigned char buf[8] = {0};
utf8_encode(buf, i);
utf8_decode(buf, &c, &e);
failures += !e;
}
TEST(failures == 0, "surrogate halves, errors: %ld", failures);
}
/* How about non-canonical encodings? */
{
int e;
uint32_t c;
unsigned char *end;
unsigned char buf2[8] = {0xc0, 0xA4};
end = utf8_decode(buf2, &c, &e);
TEST(e, "non-canonical len 2, 0x%02x", e);
TEST(end == buf2 + 2, "non-canonical recover 2, U+%04lx",
(unsigned long)c);
unsigned char buf3[8] = {0xe0, 0x80, 0xA4};
end = utf8_decode(buf3, &c, &e);
TEST(e, "non-canonical len 3, 0x%02x", e);
TEST(end == buf3 + 3, "non-canonical recover 3, U+%04lx",
(unsigned long)c);
unsigned char buf4[8] = {0xf0, 0x80, 0x80, 0xA4};
end = utf8_decode(buf4, &c, &e);
TEST(e, "non-canonical encoding len 4, 0x%02x", e);
TEST(end == buf4 + 4, "non-canonical recover 4, U+%04lx",
(unsigned long)c);
}
/* Let's try some bogus byte sequences */
{
int len, e;
uint32_t c;
/* Invalid first byte */
unsigned char buf0[4] = {0xff};
len = (unsigned char *)utf8_decode(buf0, &c, &e) - buf0;
TEST(e, "bogus [ff] 0x%02x U+%04lx", e, (unsigned long)c);
TEST(len == 1, "bogus [ff] recovery %d", len);
/* Invalid first byte */
unsigned char buf1[4] = {0x80};
len = (unsigned char *)utf8_decode(buf1, &c, &e) - buf1;
TEST(e, "bogus [80] 0x%02x U+%04lx", e, (unsigned long)c);
TEST(len == 1, "bogus [80] recovery %d", len);
/* Looks like a two-byte sequence but second byte is wrong */
unsigned char buf2[4] = {0xc0, 0x0a};
len = (unsigned char *)utf8_decode(buf2, &c, &e) - buf2;
TEST(e, "bogus [c0 0a] 0x%02x U+%04lx", e, (unsigned long)c);
TEST(len == 2, "bogus [c0 0a] recovery %d", len);
}
printf("%d fail, %d pass\n", count_fail, count_pass);
return count_fail != 0;
}
| {
"repo_name": "skeeto/branchless-utf8",
"stars": "547",
"repo_language": "C",
"file_name": "utf8-encode.h",
"mime_type": "text/x-c"
} |
#ifndef UTF8_ENCODE
#define UTF8_ENCODE
#define IS_SURROGATE(c) ((c) >= 0xD800U && (c) <= 0xDFFFU)
static void *
utf8_encode(void *buf, long c)
{
unsigned char *s = buf;
if (c >= (1L << 16)) {
s[0] = 0xf0 | (c >> 18);
s[1] = 0x80 | ((c >> 12) & 0x3f);
s[2] = 0x80 | ((c >> 6) & 0x3f);
s[3] = 0x80 | ((c >> 0) & 0x3f);
return s + 4;
} else if (c >= (1L << 11)) {
s[0] = 0xe0 | (c >> 12);
s[1] = 0x80 | ((c >> 6) & 0x3f);
s[2] = 0x80 | ((c >> 0) & 0x3f);
return s + 3;
} else if (c >= (1L << 7)) {
s[0] = 0xc0 | (c >> 6);
s[1] = 0x80 | ((c >> 0) & 0x3f);
return s + 2;
} else {
s[0] = c;
return s + 1;
}
}
#endif
| {
"repo_name": "skeeto/branchless-utf8",
"stars": "547",
"repo_language": "C",
"file_name": "utf8-encode.h",
"mime_type": "text/x-c"
} |
;;; eglot-jl.el --- Julia support for eglot -*- lexical-binding: t; -*-
;; Copyright (C) 2019 Adam Beckmeyer
;; Version: 2.1.1
;; Author: Adam Beckmeyer <[email protected]>
;; Maintainer: Adam Beckmeyer <[email protected]>
;; URL: https://github.com/non-Jedi/eglot-jl
;; Keywords: convenience, languages
;; Package-Requires: ((emacs "25.1") (eglot "1.4") (project "0.8.1") (cl-generic "1.0"))
;; License: CC0
;; This file is not part of GNU Emacs.
;;; License:
;; To the extent possible under law, Adam Beckmeyer has waived all
;; copyright and related or neighboring rights to eglot-jl. This
;; work is published from: United States.
;;; Commentary:
;; This package loads support for the Julia language server into eglot
;; and package.el. This provides IDE-like features for editing
;; julia-mode buffers. After installing this package, to load support
;; for the Julia language server, run eglot-jl-init. After that,
;; running the eglot function in a julia-mode buffer should work
;; properly.
;;; Code:
(require 'cl-generic)
(require 'eglot)
(require 'project)
(defconst eglot-jl-base (file-name-directory load-file-name))
(defgroup eglot-jl nil
"Interaction with LanguageServer.jl LSP server via eglot"
:prefix "eglot-jl-"
:group 'applications)
(defcustom eglot-jl-julia-command "julia"
"Command to run the Julia executable."
:type 'string)
(defcustom eglot-jl-julia-flags nil
"Extra flags to pass to the Julia executable."
:type '(repeat string))
(defcustom eglot-jl-depot ""
"Path or paths (space-separated) to Julia depots.
An empty string uses the default depot for ‘eglot-jl-julia-command’
when the JULIA_DEPOT_PATH environment variable is not set."
:type 'string)
(defcustom eglot-jl-language-server-project eglot-jl-base
"Julia project to run language server from.
The project should have LanguageServer and SymbolServer packages
available."
:type 'string)
;; Make project.el aware of Julia projects
(defun eglot-jl--project-try (dir)
"Return project instance if DIR is part of a julia project.
Otherwise returns nil"
(let ((root (or (locate-dominating-file dir "JuliaProject.toml")
(locate-dominating-file dir "Project.toml"))))
(and root (cons 'julia root))))
(cl-defmethod project-root ((project (head julia)))
(cdr project))
(defun eglot-jl--ls-invocation (_interactive)
"Return list of strings to be called to start the Julia language server."
`(,eglot-jl-julia-command
"--startup-file=no"
,(concat "--project=" eglot-jl-language-server-project)
,@eglot-jl-julia-flags
,(expand-file-name "eglot-jl.jl" eglot-jl-base)
,(file-name-directory (buffer-file-name))
,eglot-jl-depot))
;;;###autoload
(defun eglot-jl-init ()
"Load `eglot-jl' to use eglot with the Julia language server."
(interactive)
(add-hook 'project-find-functions #'eglot-jl--project-try)
(add-to-list 'eglot-server-programs
;; function instead of strings to find project dir at runtime
'((julia-mode julia-ts-mode) . eglot-jl--ls-invocation)))
(provide 'eglot-jl)
;;; eglot-jl.el ends here
| {
"repo_name": "non-Jedi/eglot-jl",
"stars": "52",
"repo_language": "Emacs Lisp",
"file_name": "COPYING",
"mime_type": "text/plain"
} |
# Usage:
# julia --project=path/to/eglot-jl path/to/eglot-jl/eglot-jl.jl [SOURCE_PATH] [DEPOT_PATH]
# For convenience, Pkg isn't included in eglot-jl
# Project.toml. Importing Pkg here relies on the standard library
# being available on LOAD_PATH
import Pkg
# Resolving the environment is necessary for cases where the shipped
# Manifest.toml is not compatible with the Julia version.
for _ in 1:2
try
Pkg.resolve(io=stderr)
@info "Environment successfully resolved"
break
catch err
# Downgrading from 1.6 to 1.5 sometimes causes temporary errors
@warn "Error while resolving the environment; retrying..." err
end
end
# In julia 1.4 this operation takes under a second. This can be
# crushingly slow in older versions of julia though.
Pkg.instantiate()
# Get the source path. In order of increasing priority:
# - default value: pwd()
# - command-line: ARGS[1]
src_path = length(ARGS) >= 1 ? ARGS[1] : pwd()
# Get the depot path. In order of increasing priority:
# - default value: ""
# - environment: ENV["JULIA_DEPOT_PATH"]
# - command-line: ARGS[2]
depot_path = get(ENV, "JULIA_DEPOT_PATH", "")
if length(ARGS) >= 2 && ARGS[2] != ""
depot_path = ARGS[2]
end
# Get the project environment from the source path
project_path = something(Base.current_project(src_path), Base.load_path_expand(LOAD_PATH[2])) |> dirname
# Make sure that we only load packages from this environment specifically.
empty!(LOAD_PATH)
push!(LOAD_PATH, "@")
using LanguageServer, SymbolServer
@info "Running language server" env=Base.load_path()[1] src_path project_path depot_path
server = LanguageServerInstance(stdin, stdout, project_path, depot_path)
run(server)
| {
"repo_name": "non-Jedi/eglot-jl",
"stars": "52",
"repo_language": "Emacs Lisp",
"file_name": "COPYING",
"mime_type": "text/plain"
} |
[deps]
LanguageServer = "2b0e0bc5-e4fd-59b4-8912-456d1b03d8d7"
SymbolServer = "cf896787-08d5-524d-9de7-132aaa0cb996"
[compat]
LanguageServer = "4"
julia = "1"
| {
"repo_name": "non-Jedi/eglot-jl",
"stars": "52",
"repo_language": "Emacs Lisp",
"file_name": "COPYING",
"mime_type": "text/plain"
} |
# This file is machine-generated - editing it directly is not advised
julia_version = "1.9.0"
manifest_format = "2.0"
project_hash = "697fe7aef15eb51d99a6890b570c6b02674ab66d"
[[deps.ArgTools]]
uuid = "0dad84c5-d112-42e6-8d28-ef12dabb789f"
version = "1.1.1"
[[deps.Artifacts]]
uuid = "56f22d72-fd6d-98f1-02f0-08ddc0907c33"
[[deps.Base64]]
uuid = "2a0f44e3-6c83-55bd-87e4-b1978d98bd5f"
[[deps.CSTParser]]
deps = ["Tokenize"]
git-tree-sha1 = "3ddd48d200eb8ddf9cb3e0189fc059fd49b97c1f"
uuid = "00ebfdb7-1f24-5e51-bd34-a7502290713f"
version = "3.3.6"
[[deps.CommonMark]]
deps = ["Crayons", "JSON", "PrecompileTools", "URIs"]
git-tree-sha1 = "532c4185d3c9037c0237546d817858b23cf9e071"
uuid = "a80b9123-70ca-4bc0-993e-6e3bcb318db6"
version = "0.8.12"
[[deps.Compat]]
deps = ["UUIDs"]
git-tree-sha1 = "7a60c856b9fa189eb34f5f8a6f6b5529b7942957"
uuid = "34da2185-b29b-5c13-b0c7-acf172513d20"
version = "4.6.1"
[deps.Compat.extensions]
CompatLinearAlgebraExt = "LinearAlgebra"
[deps.Compat.weakdeps]
Dates = "ade2ca70-3891-5945-98fb-dc099432e06a"
LinearAlgebra = "37e2e46d-f89d-539d-b4ee-838fcccc9c8e"
[[deps.Crayons]]
git-tree-sha1 = "249fe38abf76d48563e2f4556bebd215aa317e15"
uuid = "a8cc5b0e-0ffa-5ad4-8c14-923d3ee1735f"
version = "4.1.1"
[[deps.DataStructures]]
deps = ["Compat", "InteractiveUtils", "OrderedCollections"]
git-tree-sha1 = "d1fff3a548102f48987a52a2e0d114fa97d730f0"
uuid = "864edb3b-99cc-5e75-8d2d-829cb0a9cfe8"
version = "0.18.13"
[[deps.Dates]]
deps = ["Printf"]
uuid = "ade2ca70-3891-5945-98fb-dc099432e06a"
[[deps.Downloads]]
deps = ["ArgTools", "FileWatching", "LibCURL", "NetworkOptions"]
uuid = "f43a241f-c20a-4ad4-852c-f6b1247861c6"
version = "1.6.0"
[[deps.FileWatching]]
uuid = "7b1f6079-737a-58dc-b8bc-7a2ca5c1b5ee"
[[deps.Glob]]
git-tree-sha1 = "97285bbd5230dd766e9ef6749b80fc617126d496"
uuid = "c27321d9-0574-5035-807b-f59d2c89b15c"
version = "1.3.1"
[[deps.InteractiveUtils]]
deps = ["Markdown"]
uuid = "b77e0a4c-d291-57a0-90e8-8db25a27a240"
[[deps.JSON]]
deps = ["Dates", "Mmap", "Parsers", "Unicode"]
git-tree-sha1 = "31e996f0a15c7b280ba9f76636b3ff9e2ae58c9a"
uuid = "682c06a0-de6a-54ab-a142-c8b1cf79cde6"
version = "0.21.4"
[[deps.JSONRPC]]
deps = ["JSON", "UUIDs"]
git-tree-sha1 = "2756e5ffc7d46857e310a461aa366bbf7bbb673a"
uuid = "b9b8584e-8fd3-41f9-ad0c-7255d428e418"
version = "1.3.6"
[[deps.JuliaFormatter]]
deps = ["CSTParser", "CommonMark", "DataStructures", "Glob", "Pkg", "PrecompileTools", "Tokenize"]
git-tree-sha1 = "832629821a3c64bbabb8ad7f31fd62a37aad27be"
uuid = "98e50ef6-434e-11e9-1051-2b60c6c9e899"
version = "1.0.31"
[[deps.LanguageServer]]
deps = ["CSTParser", "JSON", "JSONRPC", "JuliaFormatter", "Markdown", "Pkg", "REPL", "StaticLint", "SymbolServer", "TestItemDetection", "Tokenize", "URIs", "UUIDs"]
git-tree-sha1 = "435e26f5767a756861bea4e406a109bee2442254"
uuid = "2b0e0bc5-e4fd-59b4-8912-456d1b03d8d7"
version = "4.4.0"
[[deps.LibCURL]]
deps = ["LibCURL_jll", "MozillaCACerts_jll"]
uuid = "b27032c2-a3e7-50c8-80cd-2d36dbcbfd21"
version = "0.6.3"
[[deps.LibCURL_jll]]
deps = ["Artifacts", "LibSSH2_jll", "Libdl", "MbedTLS_jll", "Zlib_jll", "nghttp2_jll"]
uuid = "deac9b47-8bc7-5906-a0fe-35ac56dc84c0"
version = "7.84.0+0"
[[deps.LibGit2]]
deps = ["Base64", "NetworkOptions", "Printf", "SHA"]
uuid = "76f85450-5226-5b5a-8eaa-529ad045b433"
[[deps.LibSSH2_jll]]
deps = ["Artifacts", "Libdl", "MbedTLS_jll"]
uuid = "29816b5a-b9ab-546f-933c-edad1886dfa8"
version = "1.10.2+0"
[[deps.Libdl]]
uuid = "8f399da3-3557-5675-b5ff-fb832c97cbdb"
[[deps.Logging]]
uuid = "56ddb016-857b-54e1-b83d-db4d58db5568"
[[deps.Markdown]]
deps = ["Base64"]
uuid = "d6f4376e-aef5-505a-96c1-9c027394607a"
[[deps.MbedTLS_jll]]
deps = ["Artifacts", "Libdl"]
uuid = "c8ffd9c3-330d-5841-b78e-0817d7145fa1"
version = "2.28.2+0"
[[deps.Mmap]]
uuid = "a63ad114-7e13-5084-954f-fe012c677804"
[[deps.MozillaCACerts_jll]]
uuid = "14a3606d-f60d-562e-9121-12d972cd8159"
version = "2022.10.11"
[[deps.NetworkOptions]]
uuid = "ca575930-c2e3-43a9-ace4-1e988b2c1908"
version = "1.2.0"
[[deps.OrderedCollections]]
git-tree-sha1 = "d321bf2de576bf25ec4d3e4360faca399afca282"
uuid = "bac558e1-5e72-5ebc-8fee-abe8a469f55d"
version = "1.6.0"
[[deps.Parsers]]
deps = ["Dates", "PrecompileTools", "UUIDs"]
git-tree-sha1 = "a5aef8d4a6e8d81f171b2bd4be5265b01384c74c"
uuid = "69de0a69-1ddd-5017-9359-2bf0b02dc9f0"
version = "2.5.10"
[[deps.Pkg]]
deps = ["Artifacts", "Dates", "Downloads", "FileWatching", "LibGit2", "Libdl", "Logging", "Markdown", "Printf", "REPL", "Random", "SHA", "Serialization", "TOML", "Tar", "UUIDs", "p7zip_jll"]
uuid = "44cfe95a-1eb2-52ea-b672-e2afdf69b78f"
version = "1.9.0"
[[deps.PrecompileTools]]
deps = ["Preferences"]
git-tree-sha1 = "259e206946c293698122f63e2b513a7c99a244e8"
uuid = "aea7be01-6a6a-4083-8856-8a6e6704d82a"
version = "1.1.1"
[[deps.Preferences]]
deps = ["TOML"]
git-tree-sha1 = "7eb1686b4f04b82f96ed7a4ea5890a4f0c7a09f1"
uuid = "21216c6a-2e73-6563-6e65-726566657250"
version = "1.4.0"
[[deps.Printf]]
deps = ["Unicode"]
uuid = "de0858da-6303-5e67-8744-51eddeeeb8d7"
[[deps.REPL]]
deps = ["InteractiveUtils", "Markdown", "Sockets", "Unicode"]
uuid = "3fa0cd96-eef1-5676-8a61-b3b8758bbffb"
[[deps.Random]]
deps = ["SHA", "Serialization"]
uuid = "9a3f8284-a2c9-5f02-9a11-845980a1fd5c"
[[deps.SHA]]
uuid = "ea8e919c-243c-51af-8825-aaa63cd721ce"
version = "0.7.0"
[[deps.Serialization]]
uuid = "9e88b42a-f829-5b0c-bbe9-9e923198166b"
[[deps.Sockets]]
uuid = "6462fe0b-24de-5631-8697-dd941f90decc"
[[deps.StaticLint]]
deps = ["CSTParser", "Serialization", "SymbolServer"]
git-tree-sha1 = "1152934b19a8a296db95ef6e1d454d4acc2aa79d"
uuid = "b3cc710f-9c33-5bdb-a03d-a94903873e97"
version = "8.1.0"
[[deps.SymbolServer]]
deps = ["InteractiveUtils", "LibGit2", "Markdown", "Pkg", "REPL", "SHA", "Serialization", "Sockets", "UUIDs"]
git-tree-sha1 = "d675e3a860523660421b1ca33543c06db2783a9b"
uuid = "cf896787-08d5-524d-9de7-132aaa0cb996"
version = "7.2.1"
[[deps.TOML]]
deps = ["Dates"]
uuid = "fa267f1f-6049-4f14-aa54-33bafae1ed76"
version = "1.0.3"
[[deps.Tar]]
deps = ["ArgTools", "SHA"]
uuid = "a4e569a6-e804-4fa4-b0f3-eef7a1d5b13e"
version = "1.10.0"
[[deps.TestItemDetection]]
deps = ["CSTParser"]
git-tree-sha1 = "c63abb8bf01ba3f0e5421760454d578ee9bd12ca"
uuid = "76b0de8b-5c4b-48ef-a724-914b33ca988d"
version = "0.2.0"
[[deps.Tokenize]]
git-tree-sha1 = "90538bf898832b6ebd900fa40f223e695970e3a5"
uuid = "0796e94c-ce3b-5d07-9a54-7f471281c624"
version = "0.5.25"
[[deps.URIs]]
git-tree-sha1 = "074f993b0ca030848b897beff716d93aca60f06a"
uuid = "5c2747f8-b7ea-4ff2-ba2e-563bfd36b1d4"
version = "1.4.2"
[[deps.UUIDs]]
deps = ["Random", "SHA"]
uuid = "cf7118a7-6976-5b1a-9a39-7adc72f591a4"
[[deps.Unicode]]
uuid = "4ec0a83e-493e-50e2-b9ac-8f72acf5a8f5"
[[deps.Zlib_jll]]
deps = ["Libdl"]
uuid = "83775a58-1f1d-513f-b197-d71354ab007a"
version = "1.2.13+0"
[[deps.nghttp2_jll]]
deps = ["Artifacts", "Libdl"]
uuid = "8e850ede-7688-5339-a07c-302acd2aaf8d"
version = "1.48.0+0"
[[deps.p7zip_jll]]
deps = ["Artifacts", "Libdl"]
uuid = "3f19e933-33d8-53b3-aaab-bd5110c3b7a0"
version = "17.4.0+0"
| {
"repo_name": "non-Jedi/eglot-jl",
"stars": "52",
"repo_language": "Emacs Lisp",
"file_name": "COPYING",
"mime_type": "text/plain"
} |
* eglot-jl
This is a simple package to make using [[https://github.com/julia-vscode/LanguageServer.jl][Julia's language server]] easier
with [[https://github.com/joaotavora/eglot][eglot]]. It can be installed from [[https://melpa.org/#/eglot-jl][melpa]] by [[https://melpa.org/#/getting-started][adding melpa to your
~package-archives~ ]] and doing =M-x package-install RET eglot-jl RET=
with Emacs 26.1+.
After installation, ~eglot-jl-init~ will load support for the Julia
language server into eglot and project.el. If ~eglot-jl-init~ has been
run in your emacs session, running ~eglot~ in a ~julia-mode~ buffer
will start a language server for the [[https://docs.julialang.org/en/v1.1/manual/code-loading/#Project-environments-1][Julia project]] to which the buffer
belongs. The first time ~eglot~ is run, the Julia language server will
be downloaded and installed into the default depot (e.g. =~/.julia=)
in its own isolated project environment. This may take a long time, so
~eglot-connect-timeout~ should be increased for this first run.
** Capabilities
Completion:
[[./images/completion.gif]]
Show help and signatures in the minibuffer:
[[./images/minibuffer_help.gif]]
Show docstring for symbol at point (~eglot-help-at-point~):
[[./images/eglot-help-at-point.gif]]
Jump to definitions of symbol at point (~xref-find-definitions~):
[[./images/xref-find-definitions.gif]]
Rename symbol at point (~eglot-rename~):
[[./images/eglot-rename.gif]]
Linting:
[[./images/lint.gif]]
Jump to symbol in document ~imenu~:
[[./images/imenu.gif]]
** FAQ
*** Why am I receiving ~Error running timer: (error "[eglot] Timed out")~ messages?
The most likely reason is that [[https://github.com/julia-vscode/SymbolServer.jl/issues/56][SymbolServer.jl takes a very long time
to process project dependencies]]. This is a one-time process that
shouldn't cause issues once the dependencies are cached, however it
can take over a minute to process each dependency. By default, eglot
will only wait wait 30 seconds for a language server to be ready; this
is controlled by =eglot-connect-timeout=.
To work around this issue, you can:
1. Set =eglot-connect-timeout= to a very high value.
- Progress of the SymbolServer can be monitored in the =*EGLOT
(ProjectName/julia-mode) stderr*= buffer.
2. Run the following, from your project directory:
#+begin_src sh
julia --project=path/to/eglot-jl/ path/to/eglot-jl/eglot-jl.jl
#+end_src
The SymbolServer is finished with caching dependencies when it
displays:
#+begin_quote
[ Info: Received new data from Julia Symbol Server.
#+end_quote
| {
"repo_name": "non-Jedi/eglot-jl",
"stars": "52",
"repo_language": "Emacs Lisp",
"file_name": "COPYING",
"mime_type": "text/plain"
} |
Creative Commons Legal Code
CC0 1.0 Universal
CREATIVE COMMONS CORPORATION IS NOT A LAW FIRM AND DOES NOT PROVIDE
LEGAL SERVICES. DISTRIBUTION OF THIS DOCUMENT DOES NOT CREATE AN
ATTORNEY-CLIENT RELATIONSHIP. CREATIVE COMMONS PROVIDES THIS
INFORMATION ON AN "AS-IS" BASIS. CREATIVE COMMONS MAKES NO WARRANTIES
REGARDING THE USE OF THIS DOCUMENT OR THE INFORMATION OR WORKS
PROVIDED HEREUNDER, AND DISCLAIMS LIABILITY FOR DAMAGES RESULTING FROM
THE USE OF THIS DOCUMENT OR THE INFORMATION OR WORKS PROVIDED
HEREUNDER.
Statement of Purpose
The laws of most jurisdictions throughout the world automatically confer
exclusive Copyright and Related Rights (defined below) upon the creator
and subsequent owner(s) (each and all, an "owner") of an original work of
authorship and/or a database (each, a "Work").
Certain owners wish to permanently relinquish those rights to a Work for
the purpose of contributing to a commons of creative, cultural and
scientific works ("Commons") that the public can reliably and without fear
of later claims of infringement build upon, modify, incorporate in other
works, reuse and redistribute as freely as possible in any form whatsoever
and for any purposes, including without limitation commercial purposes.
These owners may contribute to the Commons to promote the ideal of a free
culture and the further production of creative, cultural and scientific
works, or to gain reputation or greater distribution for their Work in
part through the use and efforts of others.
For these and/or other purposes and motivations, and without any
expectation of additional consideration or compensation, the person
associating CC0 with a Work (the "Affirmer"), to the extent that he or she
is an owner of Copyright and Related Rights in the Work, voluntarily
elects to apply CC0 to the Work and publicly distribute the Work under its
terms, with knowledge of his or her Copyright and Related Rights in the
Work and the meaning and intended legal effect of CC0 on those rights.
1. Copyright and Related Rights. A Work made available under CC0 may be
protected by copyright and related or neighboring rights ("Copyright and
Related Rights"). Copyright and Related Rights include, but are not
limited to, the following:
i. the right to reproduce, adapt, distribute, perform, display,
communicate, and translate a Work;
ii. moral rights retained by the original author(s) and/or performer(s);
iii. publicity and privacy rights pertaining to a person's image or
likeness depicted in a Work;
iv. rights protecting against unfair competition in regards to a Work,
subject to the limitations in paragraph 4(a), below;
v. rights protecting the extraction, dissemination, use and reuse of data
in a Work;
vi. database rights (such as those arising under Directive 96/9/EC of the
European Parliament and of the Council of 11 March 1996 on the legal
protection of databases, and under any national implementation
thereof, including any amended or successor version of such
directive); and
vii. other similar, equivalent or corresponding rights throughout the
world based on applicable law or treaty, and any national
implementations thereof.
2. Waiver. To the greatest extent permitted by, but not in contravention
of, applicable law, Affirmer hereby overtly, fully, permanently,
irrevocably and unconditionally waives, abandons, and surrenders all of
Affirmer's Copyright and Related Rights and associated claims and causes
of action, whether now known or unknown (including existing as well as
future claims and causes of action), in the Work (i) in all territories
worldwide, (ii) for the maximum duration provided by applicable law or
treaty (including future time extensions), (iii) in any current or future
medium and for any number of copies, and (iv) for any purpose whatsoever,
including without limitation commercial, advertising or promotional
purposes (the "Waiver"). Affirmer makes the Waiver for the benefit of each
member of the public at large and to the detriment of Affirmer's heirs and
successors, fully intending that such Waiver shall not be subject to
revocation, rescission, cancellation, termination, or any other legal or
equitable action to disrupt the quiet enjoyment of the Work by the public
as contemplated by Affirmer's express Statement of Purpose.
3. Public License Fallback. Should any part of the Waiver for any reason
be judged legally invalid or ineffective under applicable law, then the
Waiver shall be preserved to the maximum extent permitted taking into
account Affirmer's express Statement of Purpose. In addition, to the
extent the Waiver is so judged Affirmer hereby grants to each affected
person a royalty-free, non transferable, non sublicensable, non exclusive,
irrevocable and unconditional license to exercise Affirmer's Copyright and
Related Rights in the Work (i) in all territories worldwide, (ii) for the
maximum duration provided by applicable law or treaty (including future
time extensions), (iii) in any current or future medium and for any number
of copies, and (iv) for any purpose whatsoever, including without
limitation commercial, advertising or promotional purposes (the
"License"). The License shall be deemed effective as of the date CC0 was
applied by Affirmer to the Work. Should any part of the License for any
reason be judged legally invalid or ineffective under applicable law, such
partial invalidity or ineffectiveness shall not invalidate the remainder
of the License, and in such case Affirmer hereby affirms that he or she
will not (i) exercise any of his or her remaining Copyright and Related
Rights in the Work or (ii) assert any associated claims and causes of
action with respect to the Work, in either case contrary to Affirmer's
express Statement of Purpose.
4. Limitations and Disclaimers.
a. No trademark or patent rights held by Affirmer are waived, abandoned,
surrendered, licensed or otherwise affected by this document.
b. Affirmer offers the Work as-is and makes no representations or
warranties of any kind concerning the Work, express, implied,
statutory or otherwise, including without limitation warranties of
title, merchantability, fitness for a particular purpose, non
infringement, or the absence of latent or other defects, accuracy, or
the present or absence of errors, whether or not discoverable, all to
the greatest extent permissible under applicable law.
c. Affirmer disclaims responsibility for clearing rights of other persons
that may apply to the Work or any use thereof, including without
limitation any person's Copyright and Related Rights in the Work.
Further, Affirmer disclaims responsibility for obtaining any necessary
consents, permissions or other rights required for any use of the
Work.
d. Affirmer understands and acknowledges that Creative Commons is not a
party to this document and has no duty or obligation with respect to
this CC0 or use of the Work.
| {
"repo_name": "non-Jedi/eglot-jl",
"stars": "52",
"repo_language": "Emacs Lisp",
"file_name": "COPYING",
"mime_type": "text/plain"
} |
# Contribution Guidelines
Please note that this project is released with a [Contributor Code of Conduct](CODE-OF-CONDUCT.md). By participating in this project you agree to abide by its terms.
-
Ensure your pull request adheres to the following guidelines:
- Search previous suggestions before making a new one, as yours may be a duplicate.
- If you just created something, wait at least a couple of weeks before submitting.
- You should of course have read or used the thing you're submitting.
- Make an individual pull request for each suggestion.
- Use the following format: `[name](link) - Description.`
- Keep descriptions short and simple, but descriptive.
- Start the description with a capital and end with a full stop/period.
- Check your spelling and grammar.
- Make sure your text editor is set to remove trailing whitespace.
- Link additions should be added to the bottom of the relevant section.
- New categories or improvements to the existing categorization are welcome.
- Pull requests should have a useful title and include a link to the package and why it should be included.
Thank you for your suggestions!
### Updating your PR
A lot of times, making a PR adhere to the standards above can be difficult. If the maintainers notice anything that we'd like changed, we'll ask you to edit your PR before we merge it. There's no need to open a new PR, just edit the existing one. If you're not sure how to do that, [here is a guide](https://github.com/RichardLitt/docs/blob/master/amending-a-commit-guide.md) on the different ways you can update your PR so that we can merge it.
| {
"repo_name": "rajasegar/awesome-codemods",
"stars": "144",
"repo_language": "None",
"file_name": "main.yml",
"mime_type": "text/plain"
} |
# Contributor Covenant Code of Conduct
## Our Pledge
In the interest of fostering an open and welcoming environment, we as
contributors and maintainers pledge to making participation in our project and
our community a harassment-free experience for everyone, regardless of age, body
size, disability, ethnicity, gender identity and expression, level of experience,
nationality, personal appearance, race, religion, or sexual identity and
orientation.
## Our Standards
Examples of behavior that contributes to creating a positive environment
include:
* Using welcoming and inclusive language
* Being respectful of differing viewpoints and experiences
* Gracefully accepting constructive criticism
* Focusing on what is best for the community
* Showing empathy towards other community members
Examples of unacceptable behavior by participants include:
* The use of sexualized language or imagery and unwelcome sexual attention or
advances
* Trolling, insulting/derogatory comments, and personal or political attacks
* Public or private harassment
* Publishing others' private information, such as a physical or electronic
address, without explicit permission
* Other conduct which could reasonably be considered inappropriate in a
professional setting
## Our Responsibilities
Project maintainers are responsible for clarifying the standards of acceptable
behavior and are expected to take appropriate and fair corrective action in
response to any instances of unacceptable behavior.
Project maintainers have the right and responsibility to remove, edit, or
reject comments, commits, code, wiki edits, issues, and other contributions
that are not aligned to this Code of Conduct, or to ban temporarily or
permanently any contributor for other behaviors that they deem inappropriate,
threatening, offensive, or harmful.
## Scope
This Code of Conduct applies both within project spaces and in public spaces
when an individual is representing the project or its community. Examples of
representing a project or community include using an official project e-mail
address, posting via an official social media account, or acting as an appointed
representative at an online or offline event. Representation of a project may be
further defined and clarified by project maintainers.
## Enforcement
Instances of abusive, harassing, or otherwise unacceptable behavior may be
reported by contacting the project team at [email protected]. All
complaints will be reviewed and investigated and will result in a response that
is deemed necessary and appropriate to the circumstances. The project team is
obligated to maintain confidentiality with regard to the reporter of an incident.
Further details of specific enforcement policies may be posted separately.
Project maintainers who do not follow or enforce the Code of Conduct in good
faith may face temporary or permanent repercussions as determined by other
members of the project's leadership.
## Attribution
This Code of Conduct is adapted from the [Contributor Covenant][homepage], version 1.4,
available at [http://contributor-covenant.org/version/1/4][version]
[homepage]: http://contributor-covenant.org
[version]: http://contributor-covenant.org/version/1/4/
| {
"repo_name": "rajasegar/awesome-codemods",
"stars": "144",
"repo_language": "None",
"file_name": "main.yml",
"mime_type": "text/plain"
} |
# Awesome Codemods [](https://github.com/sindresorhus/awesome)
> A curated list of awesome codemod resources for various languages, libraries and frameworks
Please read the [contribution guidelines](CONTRIBUTING.md) before contributing.
## Contents
- [JavaScript](#javascript)
- [ESNext](#esnext)
- [Typescript](#typescript)
- [Ruby](#ruby)
- [Frameworks](#frameworks)
- [Libraries](#libraries)
- [Organization specific Codemods](#organization-specific-codemods)
- [Editor Plugins](#editor-plugins)
- [Misc](#misc)
- [Awesome Lists](#awesome-lists)
## JavaScript
- [js-codemod](https://github.com/cpojer/js-codemod/) - Codemod scripts to transform code to next generation JS.
- [js-transforms](https://github.com/jhgg/js-transforms) - Some documented codemod experiments to help you learn.
- [coffee-to-es2015-codemod](https://github.com/Hacker0x01/coffee-to-es2015-codemod) - A set of JSCodeshift transforms that will help you transform your CoffeeScript codebase to ES2015.
- [5to6-codemod](https://github.com/5to6/5to6-codemod) - A collection of codemods that allow you to transform your js code from ES5 to ES6.
- [es5-function-to-class-codemod](https://github.com/dhruvdutt/es5-function-to-class-codemod) - Transform ES5 Functions to ES6 Classes.
- [webpack-babel-codemod](https://github.com/agirton/webpack-babel-codemod) - Convert anonymous webpack commonjs require statements to es2015 import statements.
- [rm-debugger](https://www.npmjs.com/package/rm-debugger) - Codemod for removing `debugger;`.
- [AMD Transformer](https://bitbucket.org/atlassian/amd-codemod/src) - Converts JS into AMDified JS (by Atlassian).
- [undecorate-codemod](https://github.com/tizmagik/undecorate-codemod) - Transformers experimental ESNext decorators syntax to simple currying.
- [jest-codemods](https://github.com/skovhus/jest-codemods) - Codemods for migrating to Jest .
- [refactoring-codemods](https://github.com/jurassix/refactoring-codemods) - Refactoring support for JavaScript via jscodeshift codemods.
- [sinon-codemod](https://github.com/hurrymaplelad/sinon-codemod) - Codemod scripts that update Sinon APIs .
- [codemod-imports-sort](https://github.com/bfncs/codemod-imports-sort) - Sort ES6 imports by type.
- [JamieMason/codemods](https://github.com/JamieMason/codemods) - A collection of transforms for use with JSCodeshift.
- [eslint-transforms](https://github.com/eslint/eslint-transforms) - Codemods for the ESLint ecosystem.
- [js-codemods](https://github.com/yangshun/js-codemods) - Some codemod scripts to transform code for good styling.
- [class-props-codemod](https://github.com/zertosh/class-props-codemod) - Transform old-style assigned static properties to class static properties.
- [flow-comments-codemod](https://github.com/escaton/flow-comments-codemod) - Convert flowtype syntax to valid JS.
- [async-await-codemod](https://github.com/sgilroy/async-await-codemod) - Codemod script for migrating promise-based functions to use async/await syntax.
- [sort-class-members-codemod](https://github.com/pastelsky/sort-class-members-codemod) - A codemod for automatically fixing issues reported by eslint-plugin-sort-class-members.
- [relative-to-alias](https://github.com/s-yadav/relative-to-alias) - A codemod to do large-scale refactor of your relative path imports to alias.
- [transform-imports](https://github.com/suchipi/transform-imports) - Tools that make it easy to codemod imports/requires in your JS.
- [expect-js-to-assert](https://github.com/twada/expect-js-to-assert) - A jscodeshift codemod that transforms from expect.js to Node assert.
- [optional-chaining-codemod](https://github.com/NullVoxPopuli/optional-chaining-codemod)
- [chai-to-assert](https://github.com/twada/chai-to-assert) - A jscodeshift codemod that transforms from chai to Node assert.
- [nikgraf/js-codemod](https://github.com/nikgraf/js-codemod) - A collection of codemods.
- [amd-to-commonjs-codemod](https://github.com/skratchdot/amd-to-commonjs-codemod) - A codemod to transform amd style includes into commonjs includes.
- [js-codemod-import-absolute](https://github.com/bluedaniel/js-codemod-import-absolute) - Codemod to replace relative imports with absolute or custom paths.
- [relekang/codemods](https://github.com/relekang/codemods)
- [jest-expect-codemod](https://github.com/devenbansod/jest-expect-codemod) - CodeMods for migrating `chai.assert`, `chai.expect`, `assert` -based test assertions to jest's `expect` assertions.
- [vasco3/cuadrante-codemods](https://github.com/vasco3/cuadrante-codemods) - Converts ES6 imports to commonJS requires.
- [immutablejs-eraser-codemod](https://github.com/mariosanchez/immutablejs-eraser-codemod) - A codemod to rescue you from a Immutable.js abuse.
- [underscore-to-native](https://github.com/zackargyle/codemods) - Underscore to native codemods.
- [chai-to-jasmine](https://github.com/AlexJuarez/chai-to-jasmine) - A chai to jasmine codemod that includes additional transforms for jest migration.
- [node-dep-codemod](https://github.com/joyeecheung/node-dep-codemod) - A collection of JSCodeshift codemod scripts for migrating code that uses deprecated Node.js APIs.
- [styletron/codemods](https://github.com/styletron/codemods) - Codemods for styletron.
- [rxjs-codemod](https://github.com/sergi/rxjs-codemod) - Codemod scripts to transform older RxJS code to RxJS5-compatible code.
- [cleaner-codemods](https://github.com/peoplenarthax/cleaner-codemods) - Simple codemods to destructure prop types.
- [apitsummit-codemods](https://github.com/perjansson/aptisummit-codemods)
- [dsgkirkby/js-codemods](https://github.com/dsgkirkby/js-codemods) - Wrap switch statements in a code block.
- [EDITD/codemods](https://github.com/EDITD/codemods) - Radium to Glamour codemod.
- [bradencanderson/codemods](https://github.com/bradencanderson/codemods)
- [peakon/codemods](https://github.com/peakon/codemods) - I18n Context, Locale, No immutable class codemods.
- [knoopx/codemods](https://github.com/knoopx/codemods)
- [autobots](https://github.com/geekjuice/autobots) - Codemods for great good!.
- [ce-codemods](https://github.com/dogoku/ce-codemods) - Codemods for custom elements.
- [bseber/codemods](https://github.com/bseber/codemods) - Codemods for Jasmine refactoring.
- [strudel-codemod](https://github.com/strudeljs/strudel-codemod) - Strudel codemod scripts.
- [co-to-async](https://github.com/albinekb/co-to-async) - Take the step from co.wrap to async/await automagically.
- [DrewML/codemods](https://github.com/DrewML/codemods)
- [skratchdot-codemods](https://github.com/skratchdot/skratchdot-codemods) - A collection of utility codemods.
- [aws-sdk-js-codemod](https://github.com/awslabs/aws-sdk-js-codemod) - Codemod scripts to update AWS SDK for JavaScript APIs.
- [codeshift-community](https://github.com/CodeshiftCommunity/CodeshiftCommunity) - Community-owned global registry and documentation hub for codemods. Write & run codemodes, share them with your friends.
## ESNext
- [5to6](https://github.com/jamischarles/5to6) - A collection of codemods that allow you to transform your js code from ES5 to ES6.
- [async-await-codemod](https://github.com/vivek12345/async-await-codemod) - Codemod to add try catch to all the async await statements.
- [codemod-get-to-optional-member-expression](https://github.com/eschaefer/codemod-get-to-optional-member-expressions) - Change Lodash `get` functions to ES7 optional member expressions.
- [idx-to-optional-chaining](https://github.com/cdlewis/idx-to-optional-chaining) - JSCodeShift codemod that transforms usage of idx to the draft optional chaining standard.
- [generator2async-codemod](https://github.com/shimohq/generator2async-codemod)
- [lebab-as-babel-plugins](https://github.com/rajatvijay/lebab-as-babel-plugins) - Codemod to transform ES5 code to ES6/7 and uses babel plugins.
## Typescript
- [ts-codemod](https://github.com/tusharmath/ts-codemod) - Typescript based codemods.
- [tscodeshift](https://github.com/KnisterPeter/tscodeshift) - A tscodeshift is a toolkit for running codemods over multiple TS files.
- [flowToTs](https://github.com/MarcoPolo/flowToTs) - Flow to Typescript codemods.
- [codemod-cli-ts](https://github.com/jmdejno/codemod-cli-ts) - CLI for generating codemods written in Typescript.
- [tsmod](https://github.com/WolkSoftware/tsmod) - Refactor TypScript code programmatically using codemods.
- [js-to-typescript-codemod](https://github.com/mattlewis92/js-to-typescript-codemod) - A simple codemod for helping migrate from babel to typescript. Converts default imports to wildcards.
- [riceburn](https://github.com/kenotron/riceburn) - A Typescript, JSON, and text file Code Mod Utility.
- [flowshift](https://github.com/albertywu/flowshift) - Flow to typescript codemods.
- [ts-codemod-scripts](https://github.com/buildo/ts-codemod-scripts) - Collection of basic JS/React codemod scripts to prepare for TS on a codebase.
- [type-import-codemod](https://github.com/IanVS/type-import-codemod) - Combine type and value imports using Typescript 4.5 type modifier syntax.
## Ruby
- [Ruby AST Explorer](https://github.com/rajasegar/ruby-ast-explorer) - AST Explorer for Ruby.
- [codeshift](https://github.com/rajasegar/codeshift) - JSCodeshift equivalent for Ruby.
- [cybertron](https://github.com/rajasegar/cybertron) - Codemod CLI to bootstrap Ruby codemods (transforms).
- [ruby_crystal_codemod](https://github.com/DocSpring/ruby_crystal_codemod) - A codemod / transpiler that can help you convert Ruby into Crystal.
## Frameworks
### React.js
- [react-codemod](https://github.com/reactjs/react-codemod) - React codemod scripts to update React APIs.
- [rackt-codemod](https://github.com/reactjs/rackt-codemod) - Codemod scripts for Rackt libraries.
- [ast-18n](https://github.com/sibelius/ast-i18n) - Easily migrate your existing React codebase to use i18n.
- [codemod-react-proptypes-to-flow](https://github.com/jamiebuilds/codemod-react-proptypes-to-flow)
- [proptypes-to-flow](https://github.com/mikhail-hatsilau/proptypes-to-flow-codemod) - Codemod to tranform react proptypes to flow.
- [react-hot-loader-codemod](https://github.com/sibelius/react-hot-loader-codemod)
- [mst-codemod-to-0.10](https://github.com/mobxjs/mst-codemod-to-0.10) - A codemod to migrate to MobX-State-Tree 0.10 from previous versions.
- [babel-plugin-codemod-react-css-modules](https://github.com/Craga89/babel-plugin-codemod-react-css-modules) - Converts React components using imported CSS stylesheets to equivalent CSS Modules syntax.
- [metal-to-react](https://github.com/bryceosterhaus/metal-to-react) - Codemods for migrating metal-jsx to react.
- [rn-update-deprecated-modules](https://github.com/lucasbento/rn-update-deprecated-modules) - Codemod to update import declarations as per react-native > 0.59.x deprecations.
- [babel-plugin-hyperscript-to-jsx](https://github.com/RIP21/babel-plugin-hyperscript-to-jsx) - This plugin transforms react-hyperscript into JSX. Intended to be used as codemod.
- [cjsx-codemod](https://github.com/jsdf/cjsx-codemod) - A codemod for migrating off of coffee-react CJSX.
- [over_react_codemod](https://github.com/Workiva/over_react_codemod) - Codemods to help consumers of over_react automate the migration of UI component code.
- [yannvr/codemods](https://github.com/yannvr/codemods) - JS/React transforms because life is too short.
- [js2tsx](https://github.com/sjy/js2tsx) - A toolkit provide some codemod scripts based on jscodeshift to migrating react code base to typescript.
- [react-native-paper-codemod](https://github.com/callstack/react-native-paper-codemod)
- [react-codemod-pure-component-to-class](https://github.com/orzarchi/react-codemod-pure-component-to-class) - A react codemod to transform stateless/pure/functional components to class components.
- [denvned/codemod](https://github.com/denvned/codemod) - Relay Mutation - didResolveProps.
- [mukeshsoni/codemods](https://github.com/mukeshsoni/codemods) - Adds a data-test-id attribute to all jsx html elements.
- [js-react-codemods](https://github.com/nicholas-b-carter/js-react-codemods) - A boilerplate of JS 5/6/7 transforms for react/redux/js/etc.
- [react-with-hooks-removal-codemod](https://github.com/polizz/react-with-hooks-removal-codemod) - Remove the react-with-hooks library code when React 16.7.0 is released.
- [react-native-fix-inline-styles](https://github.com/ignacioola/react-native-fix-inline-styles) - Fix inline styles in react native components.
- [react-style-px-suffix-codemod](https://github.com/conorhastings/react-style-px-suffix-codemod) - Append px to shorthand values in style objects in react in prep for react 15 warning.
### Ember.js
- [ember-codemods](https://github.com/ember-codemods) - Official organization for Ember.js Codemods.
- [ember-watson](https://github.com/abuiles/ember-watson) - An Ember.js codemod to make upgrades automatic.
- [test-selectors-codemod](https://github.com/lorcan/test-selectors-codemod) - A codemode for fixing the ember-test-selectors testSelector helper deprecation.
- [ember-i18n-to-intl-migrator](https://github.com/DockYard/ember-i18n-to-intl-migrator) - Migrate ember-i18n to ember-intl .
- [lil-codemods](https://github.com/jmdejno/lil-codemods) - Ember codemods.
- [jmdejno/ember-codemods](https://github.com/jmdejno/ember-codemods) - Ember code Transforms.
- [react-destructuring-assignment-codemod](https://github.com/thibaudcolas/react-destructuring-assignment-codemod) - A WIP jscodeshift codemod to destructure assignments of props, state, and context.
- [legacy-tests-codemod](https://github.com/patocallaghan/legacy-tests-codemod) - A collection of codemod's for legacy-tests-codemod.
- [ember-action-codemods](https://github.com/lennyburdette/ember-action-codemods) - Codemods for converting uses of action to the {{on}} modifier.
- [ember-k-codemod](https://github.com/cibernox/ember-k-codemod) - Removes all usages of Ember.K.
- [ember-computed-decorators-codemod](https://github.com/bwittenbrook3/ember-computed-decorators-codemod) - Codemod to update ember-computed-decorators to ember-decorators.
- [ember-cli-mirage-faker-codemod](https://github.com/caseywatts/ember-cli-mirage-faker-codemod)
- [ember-component-jquery](https://github.com/patocallaghan/ember-component-jquery) - A codemod for migrating Ember Component code from `this.$()` to `$(this.element)`.
### Preact.js
- [preact-codemod](https://github.com/vutran/preact-codemod) - Shave some bytes by using Preact.
### Vue.js
- [vue-codemods](https://github.com/SergioCrisostomo/vue-codemods) - Collection of codemod scripts that help update and refactor Vue and JavaScript files.
### Angular.js
- [angular-codemods](https://github.com/arthurflachs/angular-codemods) - Codemods for refactoring legacy angular applications.
## Libraries
### Lodash
- [lodash-codemods](https://github.com/jfmengels/lodash-codemods) - Codemods to simplify upgrading Lodash versions.
- [lodash-to-lodash-amd-codemods](https://github.com/OliverJAsh/lodash-to-lodash-amd-codemods) - Lodash to [lodash-amd](https://github.com/lodash/lodash-amd) codemods.
- [optional-chaining-codemod](https://github.com/villesau/optional-chaining-codemod) - Codemod to migrate from Lodash get and logical and expressions to optional chaining.
- [js-transforms](https://github.com/gunar/js-transforms) - Codemod to replace lodash for lodash/fp.
- [modular-lodash-codemod](https://github.com/dgrijuela/modular-lodash-codemod) - Makes all your lodash imports modular.
- [kevinbarabash/codemods](https://github.com/kevinbarabash/codemods) - Lodash/Underscore to native.
### Mocha
- [mocha-to-jest-codemod](https://github.com/paularmstrong/mocha-to-jest-codemod) - Convert Mochan TDD with Chai assert tests to Jest.
- [mocha2ava-codemod](https://github.com/shimohq/mocha2ava-codemod) - A tranformer for migrating tests from Mocha to Ava.
### AVA
- [jscodeshift-ava-tester](https://github.com/jfmengels/jscodeshift-ava-tester) - Codeshift wrapper to write smaller and better tests for your codemods using AVA.
### Styled Components
- [styled-components-codemods](https://github.com/styled-components/styled-components-codemods) - Automatic codemods to upgrade your styled-components code to newer versions.
- [styled-components-v3-to-v4-codemod](https://github.com/RIP21/styled-components-v3-to-v4-codemod) - Codemod to migrate deprecated .extend API in favor of only styled functions.
### react-router
- [react-router-v4-codemods](https://github.com/rajasegar/react-router-v4-codemods) - Codemods for migrating react-router from v3 to v4.
- [react-router-v6-codemods](https://github.com/rajasegar/react-router-v6-codemods) - Codemods for migrating react-router from v5 to v6.
- [@putout/plugin-react-router](https://github.com/coderaiser/putout/tree/master/packages/plugin-react-router) - Putout plugin adds ability to migrate to latest version of react router.
### material-ui
- [@mui/codemod](https://github.com/mui/material-ui/tree/master/packages/mui-codemod) - A collection of codemod scripts based for use with jscodeshift that help update MUI APIs.
### ant-design
- [codemod-v4](https://github.com/ant-design/codemod-v4) - Codemod cli for antd v4 upgrade.
- [antd-codemod](https://github.com/ant-design/antd-codemod) - Antd codemod scripts.
- [codemod-v5](https://github.com/ant-design/codemod-v5) - Codemod cli for antd v5 upgrade.
## Organization specific Codemods
This is the list of codemods used by a particular organization for their code transformations.
- [@freshworks/ember-codemods](https://github.com/freshdesk/ember-freshdesk-codemods) - A collection of codemods used in Freshworks.
- [shopify-codemod](https://github.com/shopify-graveyard/shopify-codemod) - A collection of Codemods written with JSCodeshift that will help update our old, crusty JavaScript to nice, clean JavaScript.
- [uber-codemods](https://github.com/uber-web/uber-codemods) - Because Code Changes and Evolves.
- [artsy/codemods](https://github.com/artsy/codemods) - Various codemods used around Artsy.
- [tune-codemods](https://github.com/TUNE-Archive/tune-codemods) - A collection of codemods we use at tune.
- [yapp-codemods](https://github.com/yappbox/yapp-codemods) - Yapp's codemods.
- [civicsource/codemod](https://github.com/civicsource/codemod/)
- [salesforce/lwc-codemod](https://github.com/salesforce/lwc-codemod) - Codemods for Lightning Web Components.
## Editor Plugins
- [atom-codemod](https://github.com/rosswarren/atom-codemod) - Atom plugin for running codemods.
- [vscodemod](https://github.com/mikaelbr/vscodemod) - VSCode extension for doing codemod on selected text.
- [nmn/atom-codemod](https://github.com/nmn/atom-codemod) - Simple commands to apply specific Babel plugins/codemods on your code.
## Misc
- [ratchet](https://github.com/mskelton/ratchet) - Codemod to convert React PropTypes to TypeScript types.
- [flow-codemod](https://github.com/flowtype/flow-codemod) - Jscodeshift-powered [email protected] to [email protected] transformations .
- [mithril-codemods](https://github.com/MithrilJS/mithril-codemods)
- [webpack-codemods](https://github.com/okonet/webpack-codemods) - JS Codemod to automatically convert webpack config from v1 to v2.
- [js-codemods](https://github.com/entria/js-codemods) - Node.js/JavaScript codemods used at @entria.
- [jasmine-to-chai-codemod](https://github.com/vansosnin/jasmine-to-chai-codemod) - Codemod for jscodeshift to migrate your tests from Jasmine to Chai syntax.
- [closure-codemod](https://github.com/toshi-toma/closure-codemod) - Closure codemod scripts.
- [titanium-codemods](https://github.com/ewanharris/titanium-codemods) - Codemod scripts for Titanium Applications.
- [next-codemod](https://github.com/zeit/next-codemod) - Codemod transformations to help upgrade Next.js codebases.
- [date-fns-upgrade-codemod](https://github.com/date-fns/date-fns-upgrade-codemod) - Code mods for upgrading date-fns versions.
- [graphql2ts](https://github.com/sibelius/graphql2ts) - Transform .graphql to graphql-js typescript.
- [gen-codemod](https://github.com/noahsug/gen-codemod) - Generate codemods by specifying your starting -> desired JavaScript.
- [babel-plugin-localize](https://github.com/amerani/babel-plugin-localize) - Codemod to localize static strings.
- [generator-codemod](https://github.com/jamestalmage/generator-codemod) - A generator to create codemods quickly.
- [codemodes-tycoon](https://github.com/myshov/codemodes-tycoon) - Codemods from Tycoon.
- [pkg-upgrader](https://github.com/benmonro/pkg-upgrader) - Easily build codemod CLIs using jscodeshift. fork of lib-upgrader.
- [can-migrate](https://github.com/canjs/can-migrate) - CLI & codemod scripts for upgrading to CanJS 3, 4 and 5.
- [create-codemod-app](https://github.com/dangreenisrael/create-codemod-app) - Create Codemod App, a codemod generator and runner.
- [babel-plugin-glamorous-to-emotion](https://github.com/TejasQ/babel-plugin-glamorous-to-emotion) - A codemod to migrate existing React or Preact codebases from glamorous to emotion.
- [rxdart_codemod](https://github.com/brianegan/rxdart_codemod) - A collection of codemods to upgrade your RxDart code from one version to the next.
- [viewtools/codemods](https://github.com/viewstools/codemods) - Helpers to migrate your code to newer versions of Views Tools.
- [PHP-Codeshift](https://github.com/Atanamo/PHP-Codeshift) - A small PHP toolkit for running codemods (code transformations) over multiple PHP files.
- [gnome-shell-extension-es6-class-codemod](https://github.com/zhanghai/gnome-shell-extension-es6-class-codemod) - A jscodeshift transform that helps migrating GNOME Shell extensions to 3.32.
- [direct-import-codemod](https://github.com/limpbrains/direct-import-codemod) - Use direct imports to save JS bundle size.
- [d3-upgrade-codemods](https://github.com/expobrain/d3-upgrade-codemods) - Codemods to upgrade d3 from version 3.x.
- [generator-jscodeshif](https://github.com/scalvert/generator-jscodeshift) - A yeoman generator for a jscodeshift codemod.
- [tds-codemod](https://github.com/telus/tds-codemod) - TELUS Design System.
- [gsa-codeshift](https://github.com/bjoernricks/gsa-codeshift) - GSA codemod scripts.
- [bottender-codemod](https://github.com/bottenderjs/bottender-codemod) - Bottender codemod scripts.
- [mrm](https://github.com/sapegin/mrm) - Codemods for your project config files.
- [webdriverio/codemod](https://github.com/webdriverio/codemod) - A codemod to transform Protractor into WebdriverIO tests.
- [strapi/codemods](https://github.com/strapi/codemods) - CLI to help you migrate your Strapi applications & plugins from v3 to v4.
- [django-codemod](https://github.com/browniebroke/django-codemod) - A tool to automatically fix Django deprecations.
## Awesome Lists
- [awesome-ast](https://github.com/cowchimp/awesome-ast)
- [awesome-jscodeshift](https://github.com/sejoker/awesome-jscodeshift)
| {
"repo_name": "rajasegar/awesome-codemods",
"stars": "144",
"repo_language": "None",
"file_name": "main.yml",
"mime_type": "text/plain"
} |
on:
- push
- pull_request
jobs:
lint:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
with:
fetch-depth: 0
- uses: actions/setup-node@v1
with:
node-version: 12
- run: npm install --global awesome-lint
- run: awesome-lint
| {
"repo_name": "rajasegar/awesome-codemods",
"stars": "144",
"repo_language": "None",
"file_name": "main.yml",
"mime_type": "text/plain"
} |
services:
allhands22:
image: "${TESTING_IMAGE}"
postgres:
image: postgres
environment:
POSTGRES_USER: postgres
POSTGRES_PASSWORD: postgres
volumes:
- ./healthchecks:/healthchecks
healthcheck:
test: /healthchecks/postgres-healthcheck
interval: "5s"
sut:
image: "${TESTING_IMAGE}"
depends_on:
allhands22:
condition: service_started
postgres:
condition: service_healthy
# run all your tests here against the allhands22 service
command: curl --fail http://allhands22:80 || exit 1 | {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
# sample dockerfile for testing docker builds
FROM nginx:1.20-alpine as base
RUN apk add --no-cache curl
WORKDIR /test
COPY . .
#########################
FROM base as test
#layer test tools and assets on top as optional test stage
RUN apk add --no-cache apache2-utils
#########################
FROM base as final
# this layer gets built by default unless you set target to test
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
# Automation with Docker for CI Workflows
[](https://github.com/bretfisher/docker-ci-automation/actions/workflows/call-super-linter.yaml)
> For Docker Community All Hands 2022
[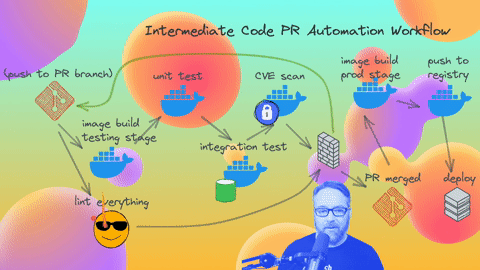](https://www.youtube.com/watch?v=aZzV6X7XhyI)
Watch the walkthrough of this repo: [https://www.youtube.com/watch?v=aZzV6X7XhyI](https://www.youtube.com/watch?v=aZzV6X7XhyI)
See this repositories' [`.github/workflows`](.github/workflows) directory for the below example workflows, ordered by number, simple to complex.
These examples are focused on five of Docker's [official GitHub Actions](https://github.com/marketplace?type=actions&query=publisher%3Adocker+).
These examples are based on three workflow diagrams on progressively more complex automation pipelines:
1. [Basic code PR automation workflow](diagrams/basic-code-pr.png)
2. [Intermediate code PR automation workflow](diagrams/intermediate-code-pr.png)
3. [Advanced code PR automation workflow](diagrams/advanced-code-pr.png)
I also have a [LIVE course on learning GitHub Actions for DevOps automation and Argo CD](https://bret.courses/autodeploy) for GitOps-style deployments.
## Example Workflows
1. Basic Docker build
2. Adding BuildKit cache
3. Adding multi-platform builds
4. Adding metadata to images
5. Adding comments with image tags to PRs
6. Adding CVE scanning
7. Adding CVE security reporting
8. Adding unit testing
9. Adding integration testing
10. Adding Kubernetes smoke tests
11. Adding job parallelizing for mucho speed
## GitHub Actions shown in these examples
- [Docker Login](https://github.com/marketplace/actions/docker-login)
- [Docker Setup Buildx](https://github.com/marketplace/actions/docker-setup-buildx)
- [Docker Setup QEMU](https://github.com/marketplace/actions/docker-setup-qemu)
- [Docker Metadata](https://github.com/marketplace/actions/docker-metadata-action)
- [Docker Build and Push](https://github.com/marketplace/actions/build-and-push-docker-images)
- [Aqua Security Trivy CVE Scan](https://github.com/marketplace/actions/aqua-security-trivy)
- [Super-Linter](https://github.com/marketplace/actions/super-linter)
- [Setup k3d](https://github.com/marketplace/actions/absaoss-k3d-action)
- [Find Comment](https://github.com/marketplace/actions/find-comment)
- [Create or Update Comment](https://github.com/marketplace/actions/create-or-update-comment)
## This repository is part of my example DevOps repos on GitHub Actions
- [bretfisher/github-actions-templates](https://github.com/BretFisher/github-actions-templates) - Main reusable templates repository
- [bretfisher/super-linter-workflow](https://github.com/BretFisher/super-linter-workflow) - Reusable linter workflow
- [bretfisher/docker-build-workflow](https://github.com/BretFisher/docker-build-workflow)- Reusable docker build workflow
- (you are here) [bretfisher/docker-ci-automation](https://github.com/BretFisher/docker-ci-automation) - Step by step video and example of a Docker CI workflow
- [My full list of container examples and tools](https://github.com/bretfisher)
## More reading
[Docker Build/Push Action advanced examples](https://github.com/docker/build-push-action/tree/master/docs/advanced)
[My full list of container examples and tools](https://github.com/bretfisher)
## 🎉🎉🎉 Join my container DevOps community 🎉🎉🎉
- [My "Vital DevOps" Discord server](https://devops.fan)
- [My weekly YouTube Live show](https://bret.live)
- [My courses and coupons](https://www.bretfisher.com/courses)
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
# To get started with Dependabot version updates, you'll need to specify which
# package ecosystems to update and where the package manifests are located.
# Please see the documentation for all configuration options:
# https://docs.github.com/github/administering-a-repository/configuration-options-for-dependency-updates
version: 2
updates:
# Maintain dependencies for GitHub Actions
- package-ecosystem: "github-actions"
directory: "/"
schedule:
interval: "daily"
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
name: 04 Build with Metadata
on:
push:
branches:
- main
pull_request:
jobs:
build-image:
name: Build Images
runs-on: ubuntu-latest
steps:
- name: Set up QEMU
uses: docker/setup-qemu-action@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
#NEW: (START) ##########################################################
- name: Docker meta
id: docker_meta
uses: docker/metadata-action@v4
with:
images: bretfisher/docker-ci-automation
flavor: |
latest=false
tags: |
type=raw,value=04
type=raw,value=latest,enable=${{ endsWith(github.ref, github.event.repository.default_branch) }}
type=ref,event=pr
type=ref,event=branch
type=semver,pattern={{version}}
#NEW: (END) ############################################################
- name: Docker build
uses: docker/build-push-action@v4
with:
#NEW: (START) ##########################################################
push: true
tags: ${{ steps.docker_meta.outputs.tags }}
labels: ${{ steps.docker_meta.outputs.labels }}
#NEW: (END) ############################################################
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64,linux/arm64,linux/arm/v7
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
name: 09 Build and Integration Test
on:
push:
branches:
- main
pull_request:
jobs:
build-image:
name: Build Images
runs-on: ubuntu-latest
steps:
- name: Checkout git repo
uses: actions/checkout@v3
- name: Set up QEMU
uses: docker/setup-qemu-action@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Build and export to Docker
uses: docker/build-push-action@v4
with:
push: false
load: true # Export to Docker Engine rather than pushing to a registry
tags: ${{ github.run_id }}
target: test
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64
#NEW: (START) ############################################################
# for more advanced tests, use docker compose with `depends_on`
# NOTE: GHA and other CIs can also run dependency containers on their own
# GHA `services:` can do this if you're trying to avoid docker compose
- name: Test healthcheck in Docker Compose
run: |
export TESTING_IMAGE="${{ github.run_id }}"
docker compose -f docker-compose.test.yml up --exit-code-from sut
#NEW: (END) ############################################################
- name: Docker Metadata for Final Image Build
id: docker_meta
uses: docker/metadata-action@v4
with:
images: bretfisher/docker-ci-automation
flavor: |
latest=false
tags: |
type=raw,value=09
# comment these out on all but 04-add-metadata.yaml to avoid overwriting image tags
# type=raw,value=latest,enable=${{ endsWith(github.ref, github.event.repository.default_branch) }}
# type=ref,event=pr
# type=ref,event=branch
# type=semver,pattern={{version}}
- name: Docker Build and Push to Docker Hub
uses: docker/build-push-action@v4
with:
push: true
tags: ${{ steps.docker_meta.outputs.tags }}
labels: ${{ steps.docker_meta.outputs.labels }}
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64,linux/arm64,linux/arm/v7
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
# template source: https://github.com/bretfisher/super-linter-workflow/blob/main/templates/call-super-linter.yaml
name: Lint Code Base
on:
push:
branches: [main]
pull_request:
jobs:
call-super-linter:
name: Call Super-Linter
permissions:
contents: read # clone the repo to lint
statuses: write #read/write to repo custom statuses
### use Reusable Workflows to call my workflow remotely
### https://docs.github.com/en/actions/learn-github-actions/reusing-workflows
### you can also call workflows from inside the same repo via file path
#FIXME: customize uri to point to your own linter repository
uses: bretfisher/super-linter-workflow/.github/workflows/reusable-super-linter.yaml@main
### Optional settings examples
# with:
### For a DevOps-focused repository. Prevents some code-language linters from running
### defaults to false
# devops-only: false
### A regex to exclude files from linting
### defaults to empty
# filter-regex-exclude: html/.*
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
name: 03 Build with Multi-Platform
on:
push:
branches:
- main
pull_request:
jobs:
build-image:
name: Build Images
runs-on: ubuntu-latest
steps:
#NEW: (START) ##########################################################
- name: Set up QEMU
uses: docker/setup-qemu-action@v2
#NEW: (END) ############################################################
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Docker build
uses: docker/build-push-action@v4
with:
push: ${{ github.event_name != 'pull_request' }}
tags: bretfisher/docker-ci-automation:03
cache-from: type=gha
cache-to: type=gha,mode=max
#NEW: (START) ##########################################################
platforms: linux/amd64,linux/arm64,linux/arm/v7
#NEW: (END) ############################################################
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
name: 07 Build and Scan + Report
on:
push:
branches:
- main
pull_request:
jobs:
build-image:
name: Build Images
runs-on: ubuntu-latest
#NEW: (START) ##########################################################
permissions:
contents: read # for actions/checkout to fetch code
security-events: write # for github/codeql-action/upload-sarif to upload SARIF results
#NEW: (END) ############################################################
steps:
#NEW: (START) ##########################################################
- name: Checkout git repo
uses: actions/checkout@v3
#NEW: (END) ############################################################
- name: Set up QEMU
uses: docker/setup-qemu-action@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Build and export to Docker
uses: docker/build-push-action@v4
with:
push: false
load: true # Export to Docker Engine rather than pushing to a registry
tags: ${{ github.run_id }}
target: test
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64
- name: Run Trivy for all CVEs (non-blocking)
uses: aquasecurity/trivy-action@master
with:
image-ref: ${{ github.run_id }}
format: table
exit-code: 0
#NEW: (START) ##########################################################
- name: Run Trivy for HIGH,CRITICAL CVEs and report (blocking)
uses: aquasecurity/trivy-action@master
with:
image-ref: ${{ github.run_id }}
exit-code: 1
ignore-unfixed: true
vuln-type: 'os,library'
severity: 'HIGH,CRITICAL'
format: 'sarif'
output: 'trivy-results.sarif'
- name: Upload Trivy scan results to GitHub Security tab
uses: github/codeql-action/upload-sarif@v2
if: always()
with:
sarif_file: 'trivy-results.sarif'
#NEW: (END) ############################################################
- name: Docker Metadata for Final Image Build
id: docker_meta
uses: docker/metadata-action@v4
with:
images: bretfisher/docker-ci-automation
flavor: |
latest=false
tags: |
type=raw,value=07
# comment these out on all but 04-add-metadata.yaml to avoid overwriting image tags
# type=raw,value=latest,enable=${{ endsWith(github.ref, github.event.repository.default_branch) }}
# type=ref,event=pr
# type=ref,event=branch
# type=semver,pattern={{version}}
- name: Docker Build and Push to Docker Hub
uses: docker/build-push-action@v4
with:
push: true
tags: ${{ steps.docker_meta.outputs.tags }}
labels: ${{ steps.docker_meta.outputs.labels }}
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64,linux/arm64,linux/arm/v7
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
name: 10 Build and Smoke Test
on:
push:
branches:
- main
pull_request:
jobs:
build-image:
name: Build Images
runs-on: ubuntu-latest
permissions:
packages: write # needed to push docker image to ghcr.io
steps:
- name: Checkout git repo
uses: actions/checkout@v3
- name: Set up QEMU
uses: docker/setup-qemu-action@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
#NEW: (START) ############################################################
- name: Login to ghcr.io registry
uses: docker/login-action@v2
with:
registry: ghcr.io
username: ${{ github.actor }}
password: ${{ secrets.GITHUB_TOKEN }}
- name: Build and Push to GHCR
uses: docker/build-push-action@v4
with:
push: true
tags: ghcr.io/bretfisher/docker-ci-automation:${{ github.run_id }}
target: test
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64
- uses: AbsaOSS/k3d-action@v2
with:
cluster-name: "test-cluster-1"
args: >-
--agents 1
--no-lb
--k3s-arg "--no-deploy=traefik,servicelb,metrics-server@server:*"
- name: Smoke test deployment in k3d Kubernetes
run: |
kubectl create secret docker-registry regcred \
--docker-server=https://ghcr.io \
--docker-username=${{ github.actor }} \
--docker-password=${{ secrets.GITHUB_TOKEN }}
export TESTING_IMAGE=ghcr.io/bretfisher/docker-ci-automation:"$GITHUB_RUN_ID"
envsubst < manifests/deployment.yaml | kubectl apply -f -
kubectl rollout status deployment myapp
kubectl exec deploy/myapp -- curl --fail localhost
#NEW: (END) ############################################################
- name: Docker Metadata for Final Image Build
id: docker_meta
uses: docker/metadata-action@v4
with:
images: bretfisher/docker-ci-automation,ghcr.io/bretfisher/docker-ci-automation
flavor: |
latest=false
tags: |
type=raw,value=10
# comment these out on all but 04-add-metadata.yaml to avoid overwriting image tags
# type=raw,value=latest,enable=${{ endsWith(github.ref, github.event.repository.default_branch) }}
# type=ref,event=pr
# type=ref,event=branch
# type=semver,pattern={{version}}
- name: Docker Build and Push to Docker Hub
uses: docker/build-push-action@v4
with:
push: true
tags: ${{ steps.docker_meta.outputs.tags }}
labels: ${{ steps.docker_meta.outputs.labels }}
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64,linux/arm64,linux/arm/v7
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
name: 06 Build and Scan
on:
push:
branches:
- main
pull_request:
jobs:
build-image:
name: Build Images
runs-on: ubuntu-latest
steps:
- name: Set up QEMU
uses: docker/setup-qemu-action@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Build and export to Docker
uses: docker/build-push-action@v4
with:
push: false
load: true # Export to Docker Engine rather than pushing to a registry
tags: ${{ github.run_id }}
target: test
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64
#NEW: (START) ##########################################################
- name: Run Trivy for all CVEs (non-blocking)
uses: aquasecurity/trivy-action@master
with:
image-ref: ${{ github.run_id }}
exit-code: 0
format: table
#NEW: (END) ############################################################
- name: Docker Metadata for Final Image Build
id: docker_meta
uses: docker/metadata-action@v4
with:
images: bretfisher/docker-ci-automation
flavor: |
latest=false
tags: |
type=raw,value=06
# comment these out on all but 04-add-metadata.yaml to avoid overwriting image tags
# type=raw,value=latest,enable=${{ endsWith(github.ref, github.event.repository.default_branch) }}
# type=ref,event=pr
# type=ref,event=branch
# type=semver,pattern={{version}}
- name: Docker Build and Push to Docker Hub
uses: docker/build-push-action@v4
with:
push: true
tags: ${{ steps.docker_meta.outputs.tags }}
labels: ${{ steps.docker_meta.outputs.labels }}
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64,linux/arm64,linux/arm/v7
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
name: 08 Build and Unit Test
on:
push:
branches:
- main
pull_request:
jobs:
build-image:
name: Build Images
runs-on: ubuntu-latest
steps:
- name: Set up QEMU
uses: docker/setup-qemu-action@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
#NEW: (START) ##########################################################
- name: Build and export to Docker
uses: docker/build-push-action@v4
with:
push: false
load: true # Export to Docker Engine rather than pushing to a registry
tags: ${{ github.run_id }}
target: test
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64
# for simple tests (npm test, etc.) just run a local image in docker
- name: Unit Testing in Docker
run: |
docker run --rm ${{ github.run_id }} echo "run test commands here"
#NEW: (END) ############################################################
- name: Docker Metadata for Final Image Build
id: docker_meta
uses: docker/metadata-action@v4
with:
images: bretfisher/docker-ci-automation
flavor: |
latest=false
tags: |
type=raw,value=08
# comment these out on all but 04-add-metadata.yaml to avoid overwriting image tags
# type=raw,value=latest,enable=${{ endsWith(github.ref, github.event.repository.default_branch) }}
# type=ref,event=pr
# type=ref,event=branch
# type=semver,pattern={{version}}
- name: Docker Build and Push to Docker Hub
uses: docker/build-push-action@v4
with:
push: true
tags: ${{ steps.docker_meta.outputs.tags }}
labels: ${{ steps.docker_meta.outputs.labels }}
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64,linux/arm64,linux/arm/v7
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
name: 02 Build with BuildKit Cache
on:
push:
branches:
- main
pull_request:
jobs:
build-image:
name: Build Image
runs-on: ubuntu-latest
steps:
#NEW: (START) ##########################################################
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
#NEW: (END) ############################################################
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Docker build
uses: docker/build-push-action@v4
with:
push: ${{ github.event_name != 'pull_request' }}
tags: bretfisher/docker-ci-automation:02
#NEW: (START) ##########################################################
cache-from: type=gha
cache-to: type=gha,mode=max
#NEW: (END) ############################################################ | {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
name: 05 Build and Comment
on:
push:
branches:
- main
pull_request:
jobs:
build-image:
name: Build Images
runs-on: ubuntu-latest
#NEW: (START) ##########################################################
permissions:
pull-requests: write # needed to create and update comments in PRs
#NEW: (END) ############################################################
steps:
- name: Set up QEMU
uses: docker/setup-qemu-action@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Docker meta
id: docker_meta
uses: docker/metadata-action@v4
with:
images: bretfisher/docker-ci-automation
flavor: |
latest=false
tags: |
type=raw,value=05
type=raw,value=latest,enable=${{ endsWith(github.ref, github.event.repository.default_branch) }}
type=ref,event=pr
type=ref,event=branch
type=semver,pattern={{version}}
- name: Docker build
uses: docker/build-push-action@v4
with:
push: false # false for this demo to prevent overwriting image tags of other examples
tags: ${{ steps.docker_meta.outputs.tags }}
labels: ${{ steps.docker_meta.outputs.labels }}
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64,linux/arm64,linux/arm/v7
#NEW: (START) ##########################################################
# If PR, put image tags in the PR comments
# from https://github.com/marketplace/actions/create-or-update-comment
- name: Find comment for image tags
uses: peter-evans/find-comment@v2
if: github.event_name == 'pull_request'
id: fc
with:
issue-number: ${{ github.event.pull_request.number }}
comment-author: 'github-actions[bot]'
body-includes: Docker image tag(s) pushed
# If PR, put image tags in the PR comments
- name: Create or update comment for image tags
uses: peter-evans/create-or-update-comment@v3
if: github.event_name == 'pull_request'
with:
comment-id: ${{ steps.fc.outputs.comment-id }}
issue-number: ${{ github.event.pull_request.number }}
body: |
Docker image tag(s) pushed:
```text
${{ steps.docker_meta.outputs.tags }}
```
Labels added to images:
```text
${{ steps.docker_meta.outputs.labels }}
```
edit-mode: replace
#NEW: (END) ############################################################
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
name: 99 Parallelize Jobs
on:
push:
branches:
- main
pull_request:
jobs:
# FIRST JOB #######################################################################
build-test-image:
name: Build Image for Testing
runs-on: ubuntu-latest
permissions:
packages: write # needed to push docker image to ghcr.io
steps:
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Login to ghcr.io registry
uses: docker/login-action@v2
with:
registry: ghcr.io
username: ${{ github.actor }}
password: ${{ secrets.GITHUB_TOKEN }}
- name: Build and Push to GHCR
uses: docker/build-push-action@v4
with:
push: true
tags: ghcr.io/bretfisher/docker-ci-automation:${{ github.run_id }}
target: test
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64
# NEXT JOB #######################################################################
test-unit:
name: Unit tests in Docker
needs: [build-test-image]
runs-on: ubuntu-latest
permissions:
packages: read
steps:
- name: Login to ghcr.io registry
uses: docker/login-action@v2
with:
registry: ghcr.io
username: ${{ github.actor }}
password: ${{ secrets.GITHUB_TOKEN }}
- name: Unit Testing in Docker
run: docker run --rm ghcr.io/bretfisher/docker-ci-automation:"$GITHUB_RUN_ID" echo "run test commands here"
# NEXT JOB #######################################################################
test-integration:
name: Integration tests in Compose
needs: [build-test-image]
runs-on: ubuntu-latest
permissions:
packages: read
steps:
- name: Checkout git repo
uses: actions/checkout@v3
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Login to ghcr.io registry
uses: docker/login-action@v2
with:
registry: ghcr.io
username: ${{ github.actor }}
password: ${{ secrets.GITHUB_TOKEN }}
- name: Test healthcheck in Docker Compose
run: |
export TESTING_IMAGE=ghcr.io/bretfisher/docker-ci-automation:"$GITHUB_RUN_ID"
echo Testing image: "$TESTING_IMAGE"
docker compose -f docker-compose.test.yml up --exit-code-from sut
# NEXT JOB #######################################################################
test-k3d:
name: Test Deployment in Kubernetes
needs: [build-test-image]
runs-on: ubuntu-latest
permissions:
packages: read
steps:
- name: Checkout git repo
uses: actions/checkout@v3
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Login to ghcr.io registry
uses: docker/login-action@v2
with:
registry: ghcr.io
username: ${{ github.actor }}
password: ${{ secrets.GITHUB_TOKEN }}
- uses: AbsaOSS/k3d-action@v2
with:
cluster-name: "test-cluster-1"
args: >-
--agents 1
--no-lb
--k3s-arg "--no-deploy=traefik,servicelb,metrics-server@server:*"
- name: Smoke test deployment in k3d Kubernetes
run: |
kubectl create secret docker-registry regcred \
--docker-server=https://ghcr.io \
--docker-username=${{ github.actor }} \
--docker-password=${{ secrets.GITHUB_TOKEN }}
export TESTING_IMAGE=ghcr.io/bretfisher/docker-ci-automation:"$GITHUB_RUN_ID"
envsubst < manifests/deployment.yaml | kubectl apply -f -
kubectl rollout status deployment myapp
kubectl exec deploy/myapp -- curl --fail localhost
# NEXT JOB #######################################################################
scan-image:
name: Scan Image with Trivy
needs: [build-test-image]
runs-on: ubuntu-latest
permissions:
contents: read # for actions/checkout to fetch code
packages: read # needed to pull docker image to ghcr.io
security-events: write # for github/codeql-action/upload-sarif to upload SARIF results
steps:
- name: Checkout git repo
uses: actions/checkout@v3
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Login to ghcr.io registry
uses: docker/login-action@v2
with:
registry: ghcr.io
username: ${{ github.actor }}
password: ${{ secrets.GITHUB_TOKEN }}
- name: Pull image to scan
run: docker pull ghcr.io/bretfisher/docker-ci-automation:"$GITHUB_RUN_ID"
- name: Run Trivy for all CVEs (non-blocking)
uses: aquasecurity/trivy-action@master
with:
image-ref: ghcr.io/bretfisher/docker-ci-automation:${{ github.run_id }}
format: table
exit-code: 0
# NOTE: disabled to avoid running twice and overwriting 07-add-cve-scanning-adv.yaml
# - name: Run Trivy for HIGH,CRITICAL CVEs and report (blocking)
# uses: aquasecurity/trivy-action@master
# with:
# image-ref: ghcr.io/bretfisher/docker-ci-automation:${{ github.run_id }}
# exit-code: 1
# ignore-unfixed: true
# vuln-type: 'os,library'
# severity: 'HIGH,CRITICAL'
# format: 'sarif'
# output: 'trivy-results.sarif'
# - name: Upload Trivy scan results to GitHub Security tab
# uses: github/codeql-action/upload-sarif@v1
# if: always()
# with:
# sarif_file: 'trivy-results.sarif'
# NEXT JOB #######################################################################
build-final-image:
name: Build Final Image
needs: [test-unit, test-integration, test-k3d, scan-image]
runs-on: ubuntu-latest
permissions:
packages: write # needed to push docker image to ghcr.io
pull-requests: write # needed to create and update comments in PRs
steps:
- name: Set up QEMU
uses: docker/setup-qemu-action@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Login to ghcr.io registry
uses: docker/login-action@v2
with:
registry: ghcr.io
username: ${{ github.actor }}
password: ${{ secrets.GITHUB_TOKEN }}
- name: Docker Metadata for Final Image Build
id: docker_meta
uses: docker/metadata-action@v4
with:
images: bretfisher/docker-ci-automation,ghcr.io/bretfisher/docker-ci-automation
flavor: |
latest=false
tags: |
type=raw,value=99
# comment these out on all but 04-add-metadata.yaml to avoid overwriting image tags
# type=raw,value=latest,enable=${{ endsWith(github.ref, github.event.repository.default_branch) }}
# type=ref,event=pr
# type=ref,event=branch
# type=semver,pattern={{version}}
- name: Docker Build and Push to GHCR and Docker Hub
uses: docker/build-push-action@v4
with:
push: true
tags: ${{ steps.docker_meta.outputs.tags }}
labels: ${{ steps.docker_meta.outputs.labels }}
cache-from: type=gha
cache-to: type=gha,mode=max
platforms: linux/amd64,linux/arm64,linux/arm/v7
# If PR, put image tags in the PR comments
# from https://github.com/marketplace/actions/create-or-update-comment
# These are commented out to avoid conflicting with 05-add-comments.yaml example
# - name: Find comment for image tags
# uses: peter-evans/find-comment@v1
# if: github.event_name == 'pull_request'
# id: fc
# with:
# issue-number: ${{ github.event.pull_request.number }}
# comment-author: 'github-actions[bot]'
# body-includes: Docker image tag(s) pushed
# # If PR, put image tags in the PR comments
# - name: Create or update comment for image tags
# uses: peter-evans/create-or-update-comment@v1
# if: github.event_name == 'pull_request'
# with:
# comment-id: ${{ steps.fc.outputs.comment-id }}
# issue-number: ${{ github.event.pull_request.number }}
# body: |
# Docker image tag(s) pushed:
# ```text
# ${{ steps.docker_meta.outputs.tags }}
# ```
# Labels added to images:
# ```text
# ${{ steps.docker_meta.outputs.labels }}
# ```
# edit-mode: replace
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
---
name: 01 Basic Docker Build
# simplest workflow possible
on:
push:
branches:
- main
pull_request:
jobs:
build-image:
name: Build Image
runs-on: ubuntu-latest
steps:
- name: Login to Docker Hub
uses: docker/login-action@v2
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Docker build
uses: docker/build-push-action@v4
with:
push: ${{ github.event_name != 'pull_request' }}
tags: bretfisher/docker-ci-automation:latest,bretfisher/docker-ci-automation:01
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
# kubernetes deployment
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
labels:
app: myapp
spec:
replicas: 1
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
imagePullSecrets:
- name: regcred
containers:
- name: myapp
image: "${TESTING_IMAGE}"
imagePullPolicy: IfNotPresent
| {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
#!/bin/bash
set -eo pipefail
host="$(hostname -i || echo '127.0.0.1')"
user="${POSTGRES_USER:-postgres}"
db="${POSTGRES_DB:-$POSTGRES_USER}"
export PGPASSWORD="${POSTGRES_PASSWORD:-}"
args=(
# force postgres to not use the local unix socket (test "external" connectibility)
--host "$host"
--username "$user"
--dbname "$db"
--quiet --no-align --tuples-only
)
if select="$(echo 'SELECT 1' | psql "${args[@]}")" && [ "$select" = '1' ]; then
exit 0
fi
exit 1 | {
"repo_name": "BretFisher/docker-ci-automation",
"stars": "177",
"repo_language": "Shell",
"file_name": "postgres-healthcheck",
"mime_type": "text/x-shellscript"
} |
<div align="center">
<br>
<img src="https://user-images.githubusercontent.com/12294525/44203609-77d50800-a147-11e8-98f0-f2403527abdc.png" width="600px" />
</div>
<br>
<p align="center">
A boilerplate for Scalable Cross-Platform Desktop Apps based on <a href="http://electron.atom.io/">Electron</a>, <a href="https://facebook.github.io/react/">React</a>, <a href="https://github.com/reactjs/redux">Redux</a>, <a href="https://github.com/reactjs/react-router">React Router</a>, <a href="http://webpack.github.io/docs/">Webpack</a> and <a href="https://github.com/gaearon/react-hot-loader">React Hot Loader</a> for rapid application development (HMR).
</p>
<div align="center">
<br>
<img src="https://forthebadge.com/images/badges/built-with-love.svg" />
<img src="https://forthebadge.com/images/badges/made-with-javascript.svg" />
<img src="https://forthebadge.com/images/badges/for-you.svg" />
</div>
<br>
<div align="center">
<a href="https://facebook.github.io/react/"><img src="./internals/img/react-padded-90.png" /></a>
<a href="https://webpack.github.io/"><img src="./internals/img/webpack-padded-90.png" /></a>
<a href="http://redux.js.org/"><img src="./internals/img/redux-padded-90.png" /></a>
<a href="https://github.com/ReactTraining/react-router"><img src="./internals/img/react-router-padded-90.png" /></a>
<a href="https://flowtype.org/"><img src="./internals/img/flow-padded-90.png" /></a>
<a href="http://eslint.org/"><img src="./internals/img/eslint-padded-90.png" /></a>
<a href="https://facebook.github.io/jest/"><img src="./internals/img/jest-padded-90.png" /></a>
<a href="https://yarnpkg.com/"><img src="./internals/img/yarn-padded-90.png" /></a>
</div>
<hr>
<br>
<div align="center">
[![Build Status][travis-image]][travis-url]
[![Appveyor Build Status][appveyor-image]][appveyor-url]
[![Dependency Status][david_img]][david_site]
[![DevDependency Status][david_img_dev]][david_site_dev]
[![Github Tag][github-tag-image]][github-tag-url]
[](https://gitter.im/electron-react-boilerplate/Lobby?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
[](#backers)
[](#sponsors)
</div>
<div align="center">

</div>
## Install
- **If you have installation or compilation issues with this project, please see [our debugging guide](https://github.com/electron-react-boilerplate/electron-react-boilerplate/issues/400)**
First, clone the repo via git:
```bash
git clone --depth 1 --single-branch --branch master https://github.com/electron-react-boilerplate/electron-react-boilerplate.git your-project-name
```
And then install the dependencies with yarn.
```bash
$ cd your-project-name
$ yarn
```
## Run
Start the app in the `dev` environment. This starts the renderer process in [**hot-module-replacement**](https://webpack.js.org/guides/hmr-react/) mode and starts a webpack dev server that sends hot updates to the renderer process:
```bash
$ yarn dev
```
If you don't need autofocus when your files was changed, then run `dev` with env `START_MINIMIZED=true`:
```bash
$ START_MINIMIZED=true yarn dev
```
## Packaging
To package apps for the local platform:
```bash
$ yarn package
```
To package apps for all platforms:
First, refer to the [Multi Platform Build docs](https://www.electron.build/multi-platform-build) for dependencies.
Then,
```bash
$ yarn package-all
```
To package apps with options:
```bash
$ yarn package --[option]
```
To run End-to-End Test
```bash
$ yarn build-e2e
$ yarn test-e2e
# Running e2e tests in a minimized window
$ START_MINIMIZED=true yarn build-e2e
$ yarn test-e2e
```
:bulb: You can debug your production build with devtools by simply setting the `DEBUG_PROD` env variable:
```bash
DEBUG_PROD=true yarn package
```
## CSS Modules
This boilerplate is configured to use [css-modules](https://github.com/css-modules/css-modules) out of the box.
All `.css` file extensions will use css-modules unless it has `.global.css`.
If you need global styles, stylesheets with `.global.css` will not go through the
css-modules loader. e.g. `app.global.css`
If you want to import global css libraries (like `bootstrap`), you can just write the following code in `.global.css`:
```css
@import '~bootstrap/dist/css/bootstrap.css';
```
## SASS support
If you want to use Sass in your app, you only need to import `.sass` files instead of `.css` once:
```js
import './app.global.scss';
```
## Static Type Checking
This project comes with Flow support out of the box! You can annotate your code with types, [get Flow errors as ESLint errors](https://github.com/amilajack/eslint-plugin-flowtype-errors), and get [type errors during runtime](https://github.com/codemix/flow-runtime) during development. Types are completely optional.
## Dispatching redux actions from main process
See [#118](https://github.com/electron-react-boilerplate/electron-react-boilerplate/issues/118) and [#108](https://github.com/electron-react-boilerplate/electron-react-boilerplate/issues/108)
## How to keep your project updated with the boilerplate
If your application is a fork from this repo, you can add this repo to another git remote:
```sh
git remote add upstream https://github.com/electron-react-boilerplate/electron-react-boilerplate.git
```
Then, use git to merge some latest commits:
```sh
git pull upstream master
```
## Maintainers
- [Vikram Rangaraj](https://github.com/vikr01)
- [Amila Welihinda](https://github.com/amilajack)
- [C. T. Lin](https://github.com/chentsulin)
- [Jhen-Jie Hong](https://github.com/jhen0409)
## Backers
Support us with a monthly donation and help us continue our activities. [[Become a backer](https://opencollective.com/electron-react-boilerplate#backer)]
<a href="https://opencollective.com/electron-react-boilerplate/backer/0/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/0/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/1/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/1/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/2/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/2/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/3/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/3/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/4/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/4/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/5/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/5/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/6/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/6/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/7/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/7/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/8/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/8/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/9/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/9/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/10/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/10/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/11/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/11/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/12/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/12/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/13/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/13/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/14/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/14/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/15/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/15/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/16/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/16/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/17/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/17/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/18/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/18/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/19/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/19/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/20/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/20/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/21/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/21/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/22/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/22/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/23/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/23/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/24/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/24/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/25/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/25/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/26/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/26/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/27/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/27/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/28/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/28/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/backer/29/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/backer/29/avatar.svg"></a>
## Sponsors
Become a sponsor and get your logo on our README on Github with a link to your site. [[Become a sponsor](https://opencollective.com/electron-react-boilerplate#sponsor)]
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/0/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/0/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/1/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/1/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/2/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/2/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/3/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/3/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/4/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/4/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/5/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/5/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/6/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/6/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/7/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/7/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/8/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/8/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/9/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/9/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/10/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/10/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/11/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/11/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/12/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/12/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/13/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/13/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/14/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/14/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/15/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/15/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/16/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/16/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/17/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/17/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/18/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/18/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/19/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/19/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/20/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/20/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/21/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/21/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/22/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/22/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/23/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/23/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/24/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/24/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/25/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/25/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/26/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/26/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/27/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/27/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/28/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/28/avatar.svg"></a>
<a href="https://opencollective.com/electron-react-boilerplate/sponsor/29/website" target="_blank"><img src="https://opencollective.com/electron-react-boilerplate/sponsor/29/avatar.svg"></a>
## License
MIT © [Electron React Boilerplate](https://github.com/electron-react-boilerplate)
[npm-image]: https://img.shields.io/npm/v/electron-react-boilerplate.svg?style=flat-square
[github-tag-image]: https://img.shields.io/github/tag/electron-react-boilerplate/electron-react-boilerplate.svg
[github-tag-url]: https://github.com/electron-react-boilerplate/electron-react-boilerplate/releases/latest
[travis-image]: https://travis-ci.com/electron-react-boilerplate/electron-react-boilerplate.svg?branch=master
[travis-url]: https://travis-ci.com/electron-react-boilerplate/electron-react-boilerplate
[appveyor-image]: https://ci.appveyor.com/api/projects/status/github/electron-react-boilerplate/electron-react-boilerplate?svg=true
[appveyor-url]: https://ci.appveyor.com/project/electron-react-boilerplate/electron-react-boilerplate/branch/master
[david_img]: https://img.shields.io/david/electron-react-boilerplate/electron-react-boilerplate.svg
[david_site]: https://david-dm.org/electron-react-boilerplate/electron-react-boilerplate
[david_img_dev]: https://david-dm.org/electron-react-boilerplate/electron-react-boilerplate/dev-status.svg
[david_site_dev]: https://david-dm.org/electron-react-boilerplate/electron-react-boilerplate?type=dev
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
/* eslint global-require: off */
const developmentEnvironments = ['development', 'test'];
const developmentPlugins = [require('react-hot-loader/babel')];
const productionPlugins = [
require('babel-plugin-dev-expression'),
// babel-preset-react-optimize
require('@babel/plugin-transform-react-constant-elements'),
require('@babel/plugin-transform-react-inline-elements'),
require('babel-plugin-transform-react-remove-prop-types')
];
module.exports = api => {
// see docs about api at https://babeljs.io/docs/en/config-files#apicache
const development = api.env(developmentEnvironments);
return {
presets: [
[
require('@babel/preset-env'),
{
targets: { electron: require('electron/package.json').version },
useBuiltIns: 'usage'
}
],
require('@babel/preset-flow'),
[require('@babel/preset-react'), { development }]
],
plugins: [
// Stage 0
require('@babel/plugin-proposal-function-bind'),
// Stage 1
require('@babel/plugin-proposal-export-default-from'),
require('@babel/plugin-proposal-logical-assignment-operators'),
[require('@babel/plugin-proposal-optional-chaining'), { loose: false }],
[
require('@babel/plugin-proposal-pipeline-operator'),
{ proposal: 'minimal' }
],
[
require('@babel/plugin-proposal-nullish-coalescing-operator'),
{ loose: false }
],
require('@babel/plugin-proposal-do-expressions'),
// Stage 2
[require('@babel/plugin-proposal-decorators'), { legacy: true }],
require('@babel/plugin-proposal-function-sent'),
require('@babel/plugin-proposal-export-namespace-from'),
require('@babel/plugin-proposal-numeric-separator'),
require('@babel/plugin-proposal-throw-expressions'),
// Stage 3
require('@babel/plugin-syntax-dynamic-import'),
require('@babel/plugin-syntax-import-meta'),
[require('@babel/plugin-proposal-class-properties'), { loose: true }],
require('@babel/plugin-proposal-json-strings'),
...(development ? developmentPlugins : productionPlugins)
]
};
};
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
# 0.17.1 (2018.11.20)
- Fix `yarn test-e2e` and testcafe for single package.json structure
- Fixes incorrect path in `yarn start` script
- Bumped deps
- Bump g++ in travis
- Change clone arguments to clone only master
- Change babel config to target current electron version
For full change list, see https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/2021
# 0.17.0 (2018.10.30)
- upgraded to `babel@7` (thanks to @vikr01 🎉🎉🎉)
- migrated from [two `package.json` structure](https://www.electron.build/tutorials/two-package-structure) (thanks to @HyperSprite!)
- initial auto update support (experimental)
- migrate from greenkeeper to [renovate](https://renovatebot.com)
- added issue template
- use `babel-preset-env` to target current electron version
- add [opencollective](https://opencollective.com/electron-react-boilerplate-594) banner message display in postinstall script (help support ERB 🙏)
- fix failing ci issues
# 0.16.0 (2018.10.3)
- removed unused dependencies
- migrate from `react-redux-router` to `connect-react-router`
- move webpack configs to `./webpack` dir
- use `g++` on travis when testing linux
- migrate from `spectron` to `testcafe` for e2e tests
- add linting support for config styles
- changed stylelint config
- temporarily disabled flow in appveyor to make ci pass
- added necessary infra to publish releases from ci
# 0.15.0 (2018.8.25)
- Performance: cache webpack uglify results
- Feature: add start minimized feature
- Feature: lint and fix styles with prettier and stylelint
- Feature: add greenkeeper support
# 0.14.0 (2018.5.24)
- Improved CI timings
- Migrated README commands to yarn from npm
- Improved vscode config
- Updated all dependencies to latest semver
- Fix `electron-rebuild` script bug
- Migrated to `mini-css-extract-plugin` from `extract-text-plugin`
- Added `optimize-css-assets-webpack-plugin`
- Run `prettier` on json, css, scss, and more filetypes
# 0.13.3 (2018.5.24)
- Add git precommit hook, when git commit will use `prettier` to format git add code
- Add format code function in `lint-fix` npm script which can use `prettier` to format project js code
# 0.13.2 (2018.1.31)
- Hot Module Reload (HMR) fixes
- Bumped all dependencies to latest semver
- Prevent error propagation of `CheckNativeDeps` script
# 0.13.1 (2018.1.13)
- Hot Module Reload (HMR) fixes
- Bumped all dependencies to latest semver
- Fixed electron-rebuild script
- Fixed tests scripts to run on all platforms
- Skip redux logs in console in test ENV
# 0.13.0 (2018.1.6)
#### Additions
- Add native dependencies check on postinstall
- Updated all dependencies to latest semver
# 0.12.0 (2017.7.8)
#### Misc
- Removed `babel-polyfill`
- Renamed and alphabetized npm scripts
#### Breaking
- Changed node dev `__dirname` and `__filename` to node built in fn's (https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/1035)
- Renamed `app/bundle.js` to `app/renderer.prod.js` for consistency
- Renamed `dll/vendor.js` to `dll/renderer.dev.dll.js` for consistency
#### Additions
- Enable node_modules cache on CI
# 0.11.2 (2017.5.1)
Yay! Another patch release. This release mostly includes refactorings and router bug fixes. Huge thanks to @anthonyraymond!
⚠️ Windows electron builds are failing because of [this issue](https://github.com/electron/electron/issues/9321). This is not an issue with the boilerplate ⚠️
#### Breaking
- **Renamed `./app/main.development.js` => `./app/main.{dev,prod}.js`:** [#963](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/963)
#### Fixes
- **Fixed reloading when not on `/` path:** [#958](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/958) [#949](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/949)
#### Additions
- **Added support for stylefmt:** [#960](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/960)
# 0.11.1 (2017.4.23)
You can now debug the production build with devtools like so:
```
DEBUG_PROD=true npm run package
```
🎉🎉🎉
#### Additions
- **Added support for debugging production build:** [#fab245a](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/941/commits/fab245a077d02a09630f74270806c0c534a4ff95)
#### Bug Fixes
- **Fixed bug related to importing native dependencies:** [#933](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/933)
#### Improvements
- **Updated all deps to latest semver**
# 0.11.0 (2017.4.19)
Here's the most notable changes since `v0.10.0`. Its been about a year since a release has been pushed. Expect a new release to be published every 3-4 weeks.
#### Breaking Changes
- **Dropped support for node < 6**
- **Refactored webpack config files**
- **Migrate to two-package.json project structure**
- **Updated all devDeps to latest semver**
- **Migrated to Jest:** [#768](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/768)
- **Migrated to `react-router@4`**
- **Migrated to `electron-builder@4`**
- **Migrated to `webpack@2`**
- **Migrated to `react-hot-loader@3`**
- **Changed default live reload server PORT to `1212` from `3000`**
#### Additions
- **Added support for Yarn:** [#451](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/451)
- **Added support for Flow:** [#425](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/425)
- **Added support for stylelint:** [#911](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/911)
- **Added support for electron-builder:** [#876](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/876)
- **Added optional support for SASS:** [#880](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/880)
- **Added support for eslint-plugin-flowtype:** [#911](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/911)
- **Added support for appveyor:** [#280](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/280)
- **Added support for webpack dlls:** [#860](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/860)
- **Route based code splitting:** [#884](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/884)
- **Added support for Webpack Bundle Analyzer:** [#922](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/922)
#### Improvements
- **Parallelize renderer and main build processes when running `npm run build`**
- **Dynamically generate electron app menu**
- **Improved vscode integration:** [#856](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/856)
#### Bug Fixes
- **Fixed hot module replacement race condition bug:** [#917](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/917) [#920](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/920)
# 0.10.0 (2016.4.18)
#### Improvements
- **Use Babel in main process with Webpack build:** [#201](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/201)
- **Change targets to built-in support by webpack:** [#197](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/197)
- **use es2015 syntax for webpack configs:** [#195](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/195)
- **Open application when webcontent is loaded:** [#192](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/192)
- **Upgraded dependencies**
#### Bug fixed
- **Fix `npm list electron-prebuilt` in package.js:** [#188](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/188)
# 0.9.0 (2016.3.23)
#### Improvements
- **Added [redux-logger](https://github.com/fcomb/redux-logger)**
- **Upgraded [react-router-redux](https://github.com/reactjs/react-router-redux) to v4**
- **Upgraded dependencies**
- **Added `npm run dev` command:** [#162](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/162)
- **electron to v0.37.2**
#### Breaking Changes
- **css module as default:** [#154](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/154).
- **set default NODE_ENV to production:** [#140](https://github.com/electron-react-boilerplate/electron-react-boilerplate/issues/140)
# 0.8.0 (2016.2.17)
#### Bug fixed
- **Fix lint errors**
- **Fix Webpack publicPath for production builds**: [#119](https://github.com/electron-react-boilerplate/electron-react-boilerplate/issues/119).
- **package script now chooses correct OS icon extension**
#### Improvements
- **babel 6**
- **Upgrade Dependencies**
- **Enable CSS source maps**
- **Add json-loader**: [#128](https://github.com/electron-react-boilerplate/electron-react-boilerplate/issues/128).
- **react-router 2.0 and react-router-redux 3.0**
# 0.7.1 (2015.12.27)
#### Bug fixed
- **Fixed npm script on windows 10:** [#103](https://github.com/electron-react-boilerplate/electron-react-boilerplate/issues/103).
- **history and react-router version bump**: [#109](https://github.com/electron-react-boilerplate/electron-react-boilerplate/issues/109), [#110](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/110).
#### Improvements
- **electron 0.36**
# 0.7.0 (2015.12.16)
#### Bug fixed
- **Fixed process.env.NODE_ENV variable in webpack:** [#74](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/74).
- **add missing object-assign**: [#76](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/76).
- **packaging in npm@3:** [#77](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/77).
- **compatibility in windows:** [#100](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/100).
- **disable chrome debugger in production env:** [#102](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/102).
#### Improvements
- **redux**
- **css-modules**
- **upgrade to react-router 1.x**
- **unit tests**
- **e2e tests**
- **travis-ci**
- **upgrade to electron 0.35.x**
- **use es2015**
- **check dev engine for node and npm**
# 0.6.5 (2015.11.7)
#### Improvements
- **Bump style-loader to 0.13**
- **Bump css-loader to 0.22**
# 0.6.4 (2015.10.27)
#### Improvements
- **Bump electron-debug to 0.3**
# 0.6.3 (2015.10.26)
#### Improvements
- **Initialize ExtractTextPlugin once:** [#64](https://github.com/electron-react-boilerplate/electron-react-boilerplate/issues/64).
# 0.6.2 (2015.10.18)
#### Bug fixed
- **Babel plugins production env not be set properly:** [#57](https://github.com/electron-react-boilerplate/electron-react-boilerplate/issues/57).
# 0.6.1 (2015.10.17)
#### Improvements
- **Bump electron to v0.34.0**
# 0.6.0 (2015.10.16)
#### Breaking Changes
- **From react-hot-loader to react-transform**
# 0.5.2 (2015.10.15)
#### Improvements
- **Run tests with babel-register:** [#29](https://github.com/electron-react-boilerplate/electron-react-boilerplate/issues/29).
# 0.5.1 (2015.10.12)
#### Bug fixed
- **Fix #51:** use `path.join(__dirname` instead of `./`.
# 0.5.0 (2015.10.11)
#### Improvements
- **Simplify webpack config** see [#50](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/50).
#### Breaking Changes
- **webpack configs**
- **port changed:** changed default port from 2992 to 3000.
- **npm scripts:** remove `start-dev` and `dev-server`. rename `hot-dev-server` to `hot-server`.
# 0.4.3 (2015.9.22)
#### Bug fixed
- **Fix #45 zeromq crash:** bump version of `electron-prebuilt`.
# 0.4.2 (2015.9.15)
#### Bug fixed
- **run start-hot breaks chrome refresh(CTRL+R) (#42)**: bump `electron-debug` to `0.2.1`
# 0.4.1 (2015.9.11)
#### Improvements
- **use electron-prebuilt version for packaging (#33)**
# 0.4.0 (2015.9.5)
#### Improvements
- **update dependencies**
# 0.3.0 (2015.8.31)
#### Improvements
- **eslint-config-airbnb**
# 0.2.10 (2015.8.27)
#### Features
- **custom placeholder icon**
#### Improvements
- **electron-renderer as target:** via [webpack-target-electron-renderer](https://github.com/chentsulin/webpack-target-electron-renderer)
# 0.2.9 (2015.8.18)
#### Bug fixed
- **Fix hot-reload**
# 0.2.8 (2015.8.13)
#### Improvements
- **bump electron-debug**
- **babelrc**
- **organize webpack scripts**
# 0.2.7 (2015.7.9)
#### Bug fixed
- **defaultProps:** fix typos.
# 0.2.6 (2015.7.3)
#### Features
- **menu**
#### Bug fixed
- **package.js:** include webpack build.
# 0.2.5 (2015.7.1)
#### Features
- **NPM Script:** support multi-platform
- **package:** `--all` option
# 0.2.4 (2015.6.9)
#### Bug fixed
- **Eslint:** typo, [#17](https://github.com/electron-react-boilerplate/electron-react-boilerplate/issues/17) and improve `.eslintrc`
# 0.2.3 (2015.6.3)
#### Features
- **Package Version:** use latest release electron version as default
- **Ignore Large peerDependencies**
#### Bug fixed
- **Npm Script:** typo, [#6](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/6)
- **Missing css:** [#7](https://github.com/electron-react-boilerplate/electron-react-boilerplate/pull/7)
# 0.2.2 (2015.6.2)
#### Features
- **electron-debug**
#### Bug fixed
- **Webpack:** add `.json` and `.node` to extensions for imitating node require.
- **Webpack:** set `node_modules` to externals for native module support.
# 0.2.1 (2015.5.30)
#### Bug fixed
- **Webpack:** #1, change build target to `atom`.
# 0.2.0 (2015.5.30)
#### Features
- **Ignore:** `test`, `tools`, `release` folder and devDependencies in `package.json`.
- **Support asar**
- **Support icon**
# 0.1.0 (2015.5.27)
#### Features
- **Webpack:** babel, react-hot, ...
- **Flux:** actions, api, components, containers, stores..
- **Package:** darwin (osx), linux and win32 (windows) platform.
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
image: Visual Studio 2017
platform:
- x64
environment:
matrix:
- nodejs_version: 10
cache:
- '%LOCALAPPDATA%/Yarn'
- node_modules
- flow-typed
- '%USERPROFILE%\.electron'
matrix:
fast_finish: true
build: off
version: '{build}'
shallow_clone: true
clone_depth: 1
install:
- ps: Install-Product node $env:nodejs_version x64
- set CI=true
- yarn
test_script:
- yarn package-ci
- yarn lint
# - yarn flow
- yarn test
- yarn build-e2e
- yarn test-e2e
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
# whirlpool-client-gui development
## Developers usage
### Install
- npm: sudo apt install npm
- yarn: sudo npm install -g yarn (or see www.yarnpkg.com)
```
cd whirlpool-gui
yarn
```
### Start in debug mode
GUI will look for a local CLI jar in from `DL_PATH_LOCAL` directory.
Edit this path for your own needs.
```
cd whirlpool-gui
yarn dev
```
### Start in standard mode
GUI will automatically download CLI.
```
cd whirlpool-gui
yarn start
```
### Build
```
cd whirlpool-gui
yarn package
```
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
[](https://travis-ci.org/Samourai-Wallet/whirlpool-client-cli)
[](https://jitpack.io/#Samourai-Wallet/whirlpool-client-cli)
# whirlpool-client-gui
# This repository is now maintained at https://code.samourai.io/whirlpool/whirlpool-gui
Desktop GUI for [Whirlpool](https://github.com/Samourai-Wallet/Whirlpool) by Samourai-Wallet.
## Requirements
- Java 8+ (OpenJDK 8+ recommended)
- A pairing payload obtained from Samourai Wallet (Android)
## Files
Whirlpool files are stored in ```userData``` which varies depending on your OS:
- MacOS: ```~/Library/Application Support/whirlpool-gui```
- Windows: ```%APPDATA%/whirlpool-gui```
- Linux: ```$XDG_CONFIG_HOME/whirlpool-gui``` or ```~/.config/whirlpool-gui```
#### Logs
- logs for GUI: ```whirlpool-gui.log```
- logs for CLI (when local): ```whirlpool-cli.log```
#### Configuration file
- CLI configuration: ```whirlpool-cli-config.properties```
#### System files
- CLI state for wallet: ```whirlpool-cli-state-xxx.properties```
- CLI state for utxos: ```whirlpool-cli-utxos-xxx.properties```
## Resources
- [whirlpool](https://github.com/Samourai-Wallet/Whirlpool)
- [whirlpool-protocol](https://github.com/Samourai-Wallet/whirlpool-protocol)
- [whirlpool-client](https://github.com/Samourai-Wallet/whirlpool-client)
- [whirlpool-server](https://github.com/Samourai-Wallet/whirlpool-server)
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Whirlpool - Samourai Wallet - GUI</title>
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no" />
<link rel="icon" href="../resources/samourai.ico" />
<script>
(function() {
if (!process.env.HOT) {
const link = document.createElement('link');
link.rel = 'stylesheet';
link.href = './dist/style.css';
// HACK: Writing the script path should be done with webpack
document.getElementsByTagName('head')[0].appendChild(link);
}
}());
</script>
</head>
<body>
<div id="root" class="h-100"></div>
<script>
{
const scripts = [];
// Dynamically insert the DLL script in development env in the
// renderer process
if (process.env.NODE_ENV === 'development') {
scripts.push('../dll/renderer.dev.dll.js');
}
// Dynamically insert the bundled app script in the renderer process
const port = process.env.PORT || 1212;
scripts.push(
(process.env.HOT)
? 'http://localhost:' + port + '/dist/renderer.dev.js'
: './dist/renderer.prod.js'
);
document.write(
scripts
.map(script => `<script defer src="${script}"><\/script>`)
.join('')
);
}
</script>
</body>
</html>
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import { version } from '../package.json';
import { computeLogPath, logger } from './utils/logger';
import { CliApiService } from './mainProcess/cliApiService';
import electron from 'electron';
export const IS_DEV = (process.env.NODE_ENV === 'development')
/* shared with mainProcess */
const API_VERSION = '0.10';
export const cliApiService = new CliApiService(API_VERSION)
export const GUI_VERSION = version;
export const DEFAULT_CLI_LOCAL = true;
export const DEFAULT_CLIPORT = 8899;
export const VERSIONS_URL = "https://raw.githubusercontent.com/Samourai-Wallet/whirlpool-runtimes/master/CLI.json"
export const IPC_CLILOCAL = {
GET_STATE: 'cliLocal.getState',
STATE: 'cliLocal.state',
RELOAD: 'cliLocal.reload',
DELETE_CONFIG: 'cliLocal.deleteConfig'
};
export const IPC_CAMERA = {
REQUEST: 'camera.request',
GRANTED: 'camera.granted',
DENIED: 'camera.denied'
};
export const CLILOCAL_STATUS = {
DOWNLOADING: 'DOWNLOADING',
ERROR: 'ERROR',
READY: 'READY'
};
export const WHIRLPOOL_SERVER = {
TESTNET: 'Whirlpool TESTNET',
MAINNET: 'Whirlpool MAINNET',
INTEGRATION: 'Whirlpool INTEGRATION'
};
export const TX0_FEE_TARGET = {
BLOCKS_2: {
value: 'BLOCKS_2',
label: 'High priority · in 2 blocks'
},
BLOCKS_6: {
value: 'BLOCKS_6',
label: 'Medium priority · in 6 blocks'
},
BLOCKS_24: {
value: 'BLOCKS_24',
label: 'Low priority · in 24 blocks'
}
}
export const STORE_CLILOCAL = 'cli.local';
export const CLI_LOG_FILE = computeLogPath('whirlpool-cli.log');
export const CLI_LOG_ERROR_FILE = computeLogPath('whirlpool-cli.error.log');
export const GUI_LOG_FILE = logger.getFile();
export const GUI_CONFIG_FILENAME = 'config.json';
export const CLI_CONFIG_FILENAME = 'whirlpool-cli-config.properties';
const app = electron.app || electron.remote.app;
export const APP_USERDATA = app.getPath('userData')
// accept CLI self-signed certificate
app.commandLine.appendSwitch('ignore-certificate-errors');
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import React from 'react';
import { render } from 'react-dom';
import { AppContainer as ReactHotAppContainer } from 'react-hot-loader';
import Root from './containers/Root';
import { configureStore, history } from './store/configureStore';
import './app.global.css';
const store = configureStore();
const AppContainer = process.env.PLAIN_HMR ? Fragment : ReactHotAppContainer;
render(
<AppContainer>
<Root store={store} history={history} />
</AppContainer>,
document.getElementById('root')
);
if (module.hot) {
module.hot.accept('./containers/Root', () => {
// eslint-disable-next-line global-require
const NextRoot = require('./containers/Root').default;
render(
<AppContainer>
<NextRoot store={store} history={history} />
</AppContainer>,
document.getElementById('root')
);
});
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
/*
* @NOTE: Prepend a `~` to css file paths that are in your node_modules
* See https://github.com/webpack-contrib/sass-loader#imports
*/
@import '~bootstrap/dist/css/bootstrap.min.css';
@import './css/bootstrap-dashboard.css';
@import './css/samourai.css';
@import './css/dashboard.css';
.premixPage .btn-sm {
line-height: 1em !important;
}
th.clipboard {
width: 20px;
}
th.hash {
width: 190px;
}
th.path {
width: 60px;
}
th.account {
width: 70px;
}
th.confirmations, th.value, th.poolId, th.mixsDone, th.utxoStatus {
width: 95px;
}
td.utxoMessage {
overflow: auto;
max-width: 180px;
}
.progress-bar {
font-weight: bold;
}
.navbar {
color: #fff !important;
}
.stats {
padding-top: 1em;
}
.tablescroll {
overflow-y: scroll;
}
.table-utxos {
border-left: 1px solid #700606;
}
.table-utxos .table-sm td {
padding: 0.2rem;
}
.table-utxos .btn-sm {
line-height: 1em;
}
.table-utxos th.utxoControls {
width: 7.5em;
}
.table-utxos th a {
cursor: pointer;
}
.table-utxos tr.utxo-disabled {
opacity: 0.5;
}
.table-hover tbody tr.utxo-disabled:hover {
background: none;
}
.table-utxos .clipboard-icon {
visibility: hidden;
cursor: pointer;
transition: opacity 0.2s;
}
.table-utxos .clipboard-icon:active {
opacity: 0.3;
outline: none;
}
.table-utxos tbody tr:hover .clipboard-icon {
visibility: visible;
}
.modal-body canvas.qr {
margin: 0.6em 0 0.2em 0;
}
.modal-zpub {
word-break: break-word;
}
.sidebar-sticky {
text-align: center;
}
.sidebar-sticky .nav {
text-align: left;
}
.zpubLink {
float: right;
font-size: 0.9em;
font-weight: bold;
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
// @flow
import { app, Menu, shell, BrowserWindow } from 'electron';
export default class MenuBuilder {
mainWindow: BrowserWindow;
constructor(mainWindow: BrowserWindow) {
this.mainWindow = mainWindow;
}
buildMenu() {
if (
process.env.NODE_ENV === 'development' ||
process.env.DEBUG_PROD === 'true'
) {
this.setupDevelopmentEnvironment();
}
const template =
process.platform === 'darwin'
? this.buildDarwinTemplate()
: this.buildDefaultTemplate();
const menu = Menu.buildFromTemplate(template);
Menu.setApplicationMenu(menu);
return menu;
}
setupDevelopmentEnvironment() {
this.mainWindow.openDevTools();
this.mainWindow.webContents.on('context-menu', (e, props) => {
const { x, y } = props;
Menu.buildFromTemplate([
{
label: 'Inspect element',
click: () => {
this.mainWindow.inspectElement(x, y);
}
}
]).popup(this.mainWindow);
});
}
buildDarwinTemplate() {
const subMenuAbout = {
label: 'whirlpool-gui',
submenu: [
{
label: 'About Samourai-Wallet',
selector: 'orderFrontStandardAboutPanel:'
},
{ type: 'separator' },
{ label: 'Services', submenu: [] },
{ type: 'separator' },
{
label: 'Hide whirlpool-gui',
accelerator: 'Command+H',
selector: 'hide:'
},
{
label: 'Hide Others',
accelerator: 'Command+Shift+H',
selector: 'hideOtherApplications:'
},
{ label: 'Show All', selector: 'unhideAllApplications:' },
{ type: 'separator' },
{
label: 'Quit',
accelerator: 'Command+Q',
click: () => {
app.quit();
}
}
]
};
const subMenuEdit = {
label: 'Edit',
submenu: [
{ label: 'Undo', accelerator: 'Command+Z', selector: 'undo:' },
{ label: 'Redo', accelerator: 'Shift+Command+Z', selector: 'redo:' },
{ type: 'separator' },
{ label: 'Cut', accelerator: 'Command+X', selector: 'cut:' },
{ label: 'Copy', accelerator: 'Command+C', selector: 'copy:' },
{ label: 'Paste', accelerator: 'Command+V', selector: 'paste:' },
{
label: 'Select All',
accelerator: 'Command+A',
selector: 'selectAll:'
}
]
};
const subMenuViewDev = {
label: 'View',
submenu: [
{
label: 'Reload',
accelerator: 'Command+R',
click: () => {
this.mainWindow.webContents.reload();
}
},
{
label: 'Toggle Full Screen',
accelerator: 'Ctrl+Command+F',
click: () => {
this.mainWindow.setFullScreen(!this.mainWindow.isFullScreen());
}
},
{
label: 'Toggle Developer Tools',
accelerator: 'Alt+Command+I',
click: () => {
this.mainWindow.toggleDevTools();
}
}
]
};
const subMenuViewProd = {
label: 'View',
submenu: [
{
label: 'Toggle Full Screen',
accelerator: 'Ctrl+Command+F',
click: () => {
this.mainWindow.setFullScreen(!this.mainWindow.isFullScreen());
}
}
]
};
const subMenuWindow = {
label: 'Window',
submenu: [
{
label: 'Minimize',
accelerator: 'Command+M',
selector: 'performMiniaturize:'
},
{ label: 'Close', accelerator: 'Command+W', selector: 'performClose:' },
{ type: 'separator' },
{ label: 'Bring All to Front', selector: 'arrangeInFront:' }
]
};
const subMenuHelp = {
label: 'Help',
submenu: [
{
label: 'Samourai Wallet',
click() {
shell.openExternal('https://www.samouraiwallet.com');
}
},
{
label: 'GitHub repository',
click() {
shell.openExternal(
'https://github.com/Samourai-Wallet/whirlpool-gui/'
);
}
}
]
};
const subMenuView =
process.env.NODE_ENV === 'development' ? subMenuViewDev : subMenuViewProd;
return [subMenuAbout, subMenuEdit, subMenuView, subMenuWindow, subMenuHelp];
}
buildDefaultTemplate() {
const templateDefault = [
{
label: '&File',
submenu: [
{
label: '&Open',
accelerator: 'Ctrl+O'
},
{
label: '&Close',
accelerator: 'Ctrl+W',
click: () => {
this.mainWindow.close();
}
}
]
},
{
label: '&View',
submenu:
process.env.NODE_ENV === 'development'
? [
{
label: '&Reload',
accelerator: 'Ctrl+R',
click: () => {
this.mainWindow.webContents.reload();
}
},
{
label: 'Toggle &Full Screen',
accelerator: 'F11',
click: () => {
this.mainWindow.setFullScreen(
!this.mainWindow.isFullScreen()
);
}
},
{
label: 'Toggle &Developer Tools',
accelerator: 'Alt+Ctrl+I',
click: () => {
this.mainWindow.toggleDevTools();
}
}
]
: [
{
label: 'Toggle &Full Screen',
accelerator: 'F11',
click: () => {
this.mainWindow.setFullScreen(
!this.mainWindow.isFullScreen()
);
}
}
]
},
{
label: 'Help',
submenu: [
{
label: 'Learn More',
click() {
shell.openExternal('http://electron.atom.io');
}
},
{
label: 'Documentation',
click() {
shell.openExternal(
'https://github.com/atom/electron/tree/master/docs#readme'
);
}
},
{
label: 'Community Discussions',
click() {
shell.openExternal('https://discuss.atom.io/c/electron');
}
},
{
label: 'Search Issues',
click() {
shell.openExternal('https://github.com/atom/electron/issues');
}
}
]
}
];
return templateDefault;
}
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
/* eslint global-require: off */
/**
* This module executes inside of electron's main process. You can start
* electron renderer process from here and communicate with the other processes
* through IPC.
*
* When running `yarn build` or `yarn build-main`, this file is compiled to
* `./app/main.prod.js` using webpack. This gives us some performance wins.
*
* @flow
*/
import { app, BrowserWindow, ipcMain, systemPreferences } from 'electron';
import { autoUpdater } from 'electron-updater';
import log from 'electron-log';
import MenuBuilder from './menu';
import { CliLocal } from './mainProcess/cliLocal';
import fs from "fs";
import { GUI_LOG_FILE, IPC_CAMERA } from './const';
import guiConfig from './mainProcess/guiConfig';
export default class AppUpdater {
constructor() {
log.transports.file.level = 'info';
autoUpdater.logger = log;
autoUpdater.checkForUpdatesAndNotify();
}
}
let mainWindow = null;
if (process.env.NODE_ENV === 'production') {
const sourceMapSupport = require('source-map-support');
sourceMapSupport.install();
}
if (
process.env.NODE_ENV === 'development' ||
process.env.DEBUG_PROD === 'true'
) {
require('electron-debug')();
}
const installExtensions = async () => {
const installer = require('electron-devtools-installer');
const forceDownload = !!process.env.UPGRADE_EXTENSIONS;
const extensions = ['REACT_DEVELOPER_TOOLS', 'REDUX_DEVTOOLS'];
return Promise.all(
extensions.map(name => installer.default(installer[name], forceDownload))
).catch(console.log);
};
// prevent multiple processes
const gotTheLock = app.requestSingleInstanceLock()
if (!gotTheLock) {
console.error("Already running => exit")
app.quit()
}
else {
app.on('second-instance', (event, commandLine, workingDirectory) => {
// Someone tried to run a second instance, we should focus our window.
if (mainWindow) {
if (mainWindow.isMinimized()) mainWindow.restore()
mainWindow.focus()
}
})
/**
* Add event listeners...
*/
app.on('window-all-closed', () => {
app.quit();
});
app.on('ready', async () => {
if (
process.env.NODE_ENV === 'development' ||
process.env.DEBUG_PROD === 'true'
) {
await installExtensions();
}
mainWindow = new BrowserWindow({
show: false,
width: 1280,
height: 728,
webPreferences: {
nodeIntegration: true
}
});
// GUI proxy
try {
const guiProxy = guiConfig.getGuiProxy()
if (guiProxy) {
// use proxy
const session = mainWindow.webContents.session
console.log('Using guiProxy:'+guiProxy)
session.setProxy({
proxyRules: guiProxy
})
}
} catch(e) {
console.error(e)
}
ipcMain.on(IPC_CAMERA.REQUEST, async (event) => {
if (process.platform !== 'darwin' || systemPreferences.getMediaAccessStatus("camera") !== "granted") {
event.reply(IPC_CAMERA.GRANTED)
} else {
const granted = await systemPreferences.askForMediaAccess("camera");
if (granted) {
event.reply(IPC_CAMERA.GRANTED)
} else {
event.reply(IPC_CAMERA.DENIED)
}
}
});
// init cliLocal
const guiLogStream = fs.createWriteStream(GUI_LOG_FILE, { flags: 'a' })
new CliLocal(ipcMain, mainWindow.webContents, guiLogStream)
mainWindow.loadURL(`file://${__dirname}/app.html`);
// @TODO: Use 'ready-to-show' event
// https://github.com/electron/electron/blob/master/docs/api/browser-window.md#using-ready-to-show-event
mainWindow.webContents.on('did-finish-load', () => {
if (!mainWindow) {
throw new Error('"mainWindow" is not defined');
}
if (process.env.START_MINIMIZED) {
mainWindow.minimize();
} else {
mainWindow.show();
mainWindow.focus();
}
});
mainWindow.on('closed', () => {
mainWindow = null;
});
const menuBuilder = new MenuBuilder(mainWindow);
menuBuilder.buildMenu();
// Remove this if your app does not use auto updates
// eslint-disable-next-line
//new AppUpdater();
});
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
// @flow
export const MIX_START = 'MIX_START';
export const MIX_STOP = 'MIX_STOP';
export const MIX_SET = 'MIX_SET';
export const mixActions = {
start: () => {
return {
type: MIX_START
}
},
stop: () => {
return {
type: MIX_STOP
}
},
set: (mix) => {
return {
type: MIX_SET,
payload: mix
}
}
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
// @flow
export const WALLET_SET = 'WALLET_SET';
export const walletActions = {
set: (wallet) => {
return {
type: WALLET_SET,
payload: wallet
}
}
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
// @flow
export const CLI_SET = 'CLI_SET';
export const cliActions = {
set: (cli) => {
return {
type: CLI_SET,
payload: cli
}
}
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
// @flow
export const GUI_SET = 'GUI_SET';
export const guiActions = {
set: (gui) => {
return {
type: GUI_SET,
payload: gui
}
}
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
// @flow
export const POOLS_SET = 'POOLS_SET';
export const poolsActions = {
set: (pools) => {
return {
type: POOLS_SET,
payload: pools
}
}
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
body {
font-size: .875rem;
}
.feather {
width: 16px;
height: 16px;
vertical-align: text-bottom;
}
/*
* Sidebar
*/
.sidebar {
position: fixed;
top: 0;
bottom: 0;
left: 0;
z-index: 100; /* Behind the navbar */
padding: 48px 0 0; /* Height of navbar */
box-shadow: inset -1px 0 0 rgba(0, 0, 0, .1);
}
.sidebar-sticky {
position: relative;
top: 0;
height: calc(100vh - 48px);
padding-top: .5rem;
overflow-x: hidden;
overflow-y: auto; /* Scrollable contents if viewport is shorter than content. */
}
@supports ((position: -webkit-sticky) or (position: sticky)) {
.sidebar-sticky {
position: -webkit-sticky;
position: sticky;
}
}
.sidebar .nav-link {
font-weight: 500;
color: #333;
}
.sidebar .nav-link .feather {
margin-right: 4px;
color: #999;
}
.sidebar .nav-link.active {
color: #007bff;
}
.sidebar .nav-link:hover .feather,
.sidebar .nav-link.active .feather {
color: inherit;
}
.sidebar-heading {
font-size: .75rem;
text-transform: uppercase;
}
/*
* Content
*/
[role="main"] {
padding-top: 55px; /* Space for fixed navbar */
}
/*
* Navbar
*/
.navbar-brand-col {
box-shadow: inset -1px 0 0 rgba(0, 0, 0, .25);
}
.navbar .form-control {
padding: .75rem 1rem;
border-width: 0;
border-radius: 0;
}
.form-control-dark {
color: #fff;
background-color: rgba(255, 255, 255, .1);
border-color: rgba(255, 255, 255, .1);
}
.form-control-dark:focus {
border-color: transparent;
box-shadow: 0 0 0 3px rgba(255, 255, 255, .25);
}
/*
* Utilities
*/
.border-top { border-top: 1px solid #e5e5e5; }
.border-bottom { border-bottom: 1px solid #e5e5e5; }
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
html,body{height:100%;}
.btn-primary {
background-color: #700606;
border-color: #700606;
}
.btn-primary:hover {
background-color: #b71c1c;
border-color: #b71c1c;
}
.btn-primary.disabled,
.btn-primary:disabled {
background-color: #aaa;
}
.btn-border {
border-color: #700606;
}
.text-primary {
color: #700606 !important;
}
.form-control:focus {
border-color: #b71c1c;
}
.page-middle {
margin-top: 10%;
}
code {
color: #b71c1c;
}
.custom-control-input:checked~.custom-control-label::before {
border-color: #b71c1c;
background-color: #b71c1c;
}
.dropdown-item.active,
.dropdown-item:active {
background-color: #700606 !important;
}
.container-fluid a.dropdown-item.active:hover {
color: #fff !important;
}
.progress-bar.progressBarSamourai {
background-color: #700606 !important;
}
.progress-bar.progressBarSamourai.connecting {
background-color: #888 !important;
}
.cliStatus {
text-align:center;
margin-top:-1em;
margin-left:3em;
font-size:0.8em;
color:#CCC;
}
.cliStatus > .icon {
margin: 0 0.5em;
}
.footerNav {
position:absolute;
bottom:2.5em;
left:1em;
color:#AAA;
}
.navbar .torIcon svg {
width:12px;
height:12px;
}
.torIconConnected {
color: green;
}
.torIconConnected svg path {
fill: green;
}
.torIconConnecting svg path {
fill: orange;
}
.torIconConnecting {
color: orange;
}
.torIconDisabled svg path {
fill: #CCC
}
.loginLocalStatus {
float:left;
margin-top:-0.5em;
}
/* status */
.status {
position: absolute;
left: 253px;
bottom: 0;
z-index: 999999;
}
.status .item {
width: 800px;
font-size: 0.8em;
background-color: #f0f2f5;
}
.status .item .label {
font-weight: bold;
}
.status .item .errorMessage {
font-weight: bold;
color: red;
}
.mixStatus {
text-align: left;
}
.mixStatus strong {
font-size: 1.1em;
}
.mixThreads {
font-size: 0.9em;
padding-top: 0.3em;
color: #222;
}
.mixThreads > .row {
height: 5em;
}
.mixThreads > .row > .col-sm-3, .mixThreads .item .progress {
height: 2.1em;
}
.mixThreads .progress-bar {
font-weight: normal;
}
.mixThreads .item {
position: relative;
margin-bottom: 0.1em;
padding: 0 8px;
}
.mixThreads .item .label {
position: absolute;
top: 0.1em;
text-align:center;
width: 100%;
line-height: 1em;
}
/* DepositPage */
.modal-tx0 {
width: 80%;
max-width: none !important;
}
.modal-status {
width: 100%;
}
.utxoPoolSelector .dropdown-item,
.utxoMixsTargetSelector .dropdown-item {
padding: 0 0.4em;
}
/* login */
.form-signin {
width: 100%;
max-width: 330px;
padding: 15px;
margin: auto;
}
.form-signin .checkbox {
font-weight: 400;
}
.form-signin .form-control {
position: relative;
box-sizing: border-box;
height: auto;
padding: 10px;
font-size: 16px;
}
.form-signin .form-control:focus {
z-index: 2;
}
.form-signin input[type="password"] {
margin-bottom: 10px;
}
/* seedSelector */
.clearWord {
cursor: not-allowed;
}
/* statusPage */
.logs {
font-size:0.8em;
padding:1em;
border: 1px solid #000;
height: 16em;
overflow: auto;
white-space: pre;
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
@font-face{
font-family:'katana';
src:url('fonts/katana/katana.eot');
src:url('fonts/katana/katana.eot?#iefix') format('embedded-opentype'),url('fonts/katana/katana.woff') format('woff'),url('fonts/katana/katana.ttf') format('truetype'),url('fonts/katana/katana.svg#katana') format('svg');
font-weight:normal;
font-style:normal}
.branding {
display: inline-block;
margin: 8px 0 0 0;
}
.branding .brand-logo {
color: #fff;
background: #c12727;
width: 55px;
height: 55px;
position: relative;
border-radius: 50%;
display: inline-block;
font-size: 2.1rem;
padding:0;
}
.branding .product-title {
color: #fff;
display: block;
float: right;
margin: 10px 0 0 10px;
font-size: 1.55rem;
}
.branding a:hover {
text-decoration: none !important;
}
.svglogo {
display: inline-block;
font-family: 'katana';
font-style: normal;
font-weight: normal;
line-height: 1;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
color: #00038;
}
span.logo {
position: absolute;
right: 0;
left: 6px;
top: 3px;
border: 0;
font-family: -apple-system, BlinkMacSystemFont, "Segoe UI", Roboto, Oxygen-Sans, Ubuntu, Cantarell, "Helvetica Neue", sans-serif;
font-size: 2.4rem;
color: #fff;
padding-top: .25rem;
padding-bottom: .15rem;
}
.icon-samourai-logo-trans::before {
content: '\0042';
display: inline-block;
font-family: 'katana';
font-style: normal;
font-weight: normal;
line-height: 1;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
}
.sidebar .nav-link.active, .container-fluid a {
color: #700606;
}
.container-fluid a:hover {
color: #b71c1c;
}
/* navbar */
[role="main"] {
padding-top: 0px !important;
}
.navbar {
height:80px;
}
.sidebar {
padding-top: 80px !important; /* Space for fixed navbar */
}
.main-container {
padding-top: 80px !important; /* Space for fixed navbar */
padding-bottom: 60px;
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import { APP_USERDATA, IS_DEV } from '../const';
import cliVersion from './cliVersion';
import { logger } from '../utils/logger';
import guiConfig from './guiConfig';
export const API_MODES = {
RELEASE: 'RELEASE',
LOCAL: 'LOCAL',
QA: 'QA'
}
const DL_PATH_LOCAL = '/zl/workspaces/whirlpool/whirlpool-client-cli4/target/'
export class CliApiService {
constructor (apiVersion) {
let apiMode = guiConfig.getApiMode()
if (IS_DEV) {
// use local jar when started with "yarn dev"
apiMode = API_MODES.LOCAL
}
this.apiMode = apiMode
this.apiVersion = apiVersion
logger.info('Initializing CliApiService: '+this.getVersionName())
}
getApiMode() {
return this.apiMode
}
getApiVersion() {
return this.apiVersion
}
isApiModeRelease() {
return this.apiMode === API_MODES.RELEASE
}
isApiModeLocal() {
return this.apiMode === API_MODES.LOCAL
}
useChecksum() {
// skip checksum verification when started with "yarn dev"
return !IS_DEV
}
getCliPath() {
if (this.isApiModeLocal()) {
// local CLI
return DL_PATH_LOCAL
}
// standard CLI download path
return APP_USERDATA
}
async fetchCliApi() {
if (this.isApiModeLocal()) {
// use local jar
return {
cliVersion: 'develop-SNAPSHOT',
filename: 'whirlpool-client-cli-develop-SNAPSHOT-run.jar',
url: false,
checksum: false
}
}
const fetchVersion = this.isApiModeRelease() ? this.apiVersion : this.getApiMode()
try {
let cliApi = await cliVersion.fetchCliApi(fetchVersion)
logger.info('Using CLI_API ' + fetchVersion, cliApi)
const projectUrl = fetchVersion === API_MODES.QA ? 'SamouraiDev/QA/releases/download/CLI-' + cliApi.CLI_VERSION : 'Samourai-Wallet/whirlpool-client-cli/releases/download/' + cliApi.CLI_VERSION
return {
cliVersion: cliApi.CLI_VERSION,
filename: 'whirlpool-client-cli-' + cliApi.CLI_VERSION + '-run.jar',
url: 'https://github.com/'+projectUrl + '/whirlpool-client-cli-' + cliApi.CLI_VERSION + '-run.jar',
checksum: cliApi.CLI_CHECKSUM
}
} catch(e) {
logger.error("Could not fetch CLI_API "+fetchVersion, e)
throw e
}
}
getVersionName() {
let version = this.apiVersion
if (!this.isApiModeRelease()) {
version += " ("+this.apiMode+")"
}
return version
}
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import { logger } from '../utils/logger';
import fs from 'fs';
import electron from 'electron';
import Store from 'electron-store';
import { STORE_CLILOCAL } from '../const';
// for some reason cliApiService.API_MODES is undefined here
const API_MODES = {
RELEASE: 'RELEASE',
LOCAL: 'LOCAL',
QA: 'QA'
}
const CONFIG_DEFAULT = {
API_MODE: API_MODES.RELEASE
}
// for some reason const.APP_USERDATA is undefined here...
const app = electron.app || electron.remote.app;
const APP_USERDATA = app.getPath('userData')
const GUI_CONFIG_FILENAME = 'whirlpool-gui-config.json';
const GUI_CONFIG_FILEPATH = APP_USERDATA+'/'+GUI_CONFIG_FILENAME
const STORE_CLIURL = "cli.url"
const STORE_APIKEY = "cli.apiKey"
const STORE_GUI_PROXY = "gui.proxy"
const STORE_GUICONFIG_VERSION = "guiConfig.version"
const GUI_CONFIG_VERSION = 1
class GuiConfig {
constructor() {
this.store = new Store()
this.cfg = this.loadConfig()
this.checkUpgradeGui()
}
loadConfig() {
let config = undefined
try {
config = JSON.parse(fs.readFileSync(GUI_CONFIG_FILEPATH, 'utf8'));
} catch(e) {
}
if (!this.validate(config)) {
logger.info("Using GUI configuration: default")
config = CONFIG_DEFAULT
this.hasConfig = false
} else {
logger.info("Using GUI configuration: "+GUI_CONFIG_FILEPATH)
this.hasConfig = true
}
return config
}
checkUpgradeGui() {
const fromGuiConfigVersion = this.store.get(STORE_GUICONFIG_VERSION)
logger.info("fromGuiConfigVersion=" + fromGuiConfigVersion + ", GUI_CONFIG_VERSION=" + GUI_CONFIG_VERSION)
if (!fromGuiConfigVersion || fromGuiConfigVersion !== GUI_CONFIG_VERSION) {
this.upgradeGui(fromGuiConfigVersion)
this.store.set(STORE_GUICONFIG_VERSION, GUI_CONFIG_VERSION)
}
}
upgradeGui(fromGuiConfigVersion) {
logger.info("Upgrading GUI: " + fromGuiConfigVersion + " -> " + GUI_CONFIG_VERSION)
// VERSION 1: use HTTPS
if (!fromGuiConfigVersion || fromGuiConfigVersion < 1) {
// move CLIURL to HTTPS
const cliUrl = this.getCliUrl()
logger.info("cliUrl=" + cliUrl)
if (cliUrl && cliUrl.indexOf('http://') !== -1) {
const cliUrlHttps = cliUrl.replace('http://', 'https://')
logger.info("Updating cliUrl: " + cliUrl + ' -> ' + cliUrlHttps)
this.setCliUrl(cliUrlHttps)
}
}
}
validate(config) {
if (config) {
if (config.API_MODE && API_MODES[config.API_MODE]) {
// valid
return true
}
// invalid
logger.error("guiConfig is invalid: "+GUI_CONFIG_FILEPATH)
}
// or not existing
return false
}
getApiMode() {
return this.cfg.API_MODE
}
hasConfig() {
return this.hasConfig
}
getConfigFile() {
return GUI_CONFIG_FILEPATH
}
// CLI CONFIG
setCliUrl(cliUrl) {
logger.info('guiConfig: set cliUrl='+cliUrl)
this.store.set(STORE_CLIURL, cliUrl)
}
setCliApiKey(apiKey) {
this.store.set(STORE_APIKEY, apiKey)
}
setCliLocal(cliLocal) {
this.store.set(STORE_CLILOCAL, cliLocal)
}
resetCliConfig() {
this.store.delete(STORE_CLIURL)
this.store.delete(STORE_APIKEY)
this.store.delete(STORE_CLILOCAL)
}
getCliUrl() {
return this.store.get(STORE_CLIURL)
}
getCliApiKey() {
return this.store.get(STORE_APIKEY)
}
getCliLocal() {
return this.store.get(STORE_CLILOCAL)
}
// GUI CONFIG
setGuiProxy(guiProxy) {
logger.info('guiConfig: set guiProxy='+guiProxy)
this.store.set(STORE_GUI_PROXY, guiProxy)
}
getGuiProxy() {
return this.store.get(STORE_GUI_PROXY)
}
}
const guiConfig = new GuiConfig()
export default guiConfig
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import { download } from 'electron-dl';
import tcpPortUsed from 'tcp-port-used';
import fs from 'fs';
import { exec, spawn } from 'child_process';
import Store from 'electron-store';
import AwaitLock from 'await-lock';
import ps from 'ps-node';
import {
CLI_CONFIG_FILENAME,
CLI_LOG_ERROR_FILE,
CLI_LOG_FILE,
cliApiService,
CLILOCAL_STATUS,
DEFAULT_CLI_LOCAL,
DEFAULT_CLIPORT,
IPC_CLILOCAL,
IS_DEV,
STORE_CLILOCAL
} from '../const';
import { logger } from '../utils/logger';
import crypto from 'crypto';
const START_TIMEOUT = 10000
const ARG_CLI_GUI = '--whirlpool-cli-gui'
export class CliLocal {
constructor(ipcMain, window) {
this.state = {}
this.ipcMain = ipcMain
this.window = window
this.store = new Store()
this.ipcMutex = new AwaitLock()
this.onIpcMain = this.onIpcMain.bind(this)
this.ipcMain.on(IPC_CLILOCAL.RELOAD, () => this.onIpcMain(IPC_CLILOCAL.RELOAD))
this.ipcMain.on(IPC_CLILOCAL.GET_STATE, () => this.onIpcMain(IPC_CLILOCAL.GET_STATE));
this.ipcMain.on(IPC_CLILOCAL.DELETE_CONFIG, () => this.onIpcMain(IPC_CLILOCAL.DELETE_CONFIG));
this.findCliProcesses = this.findCliProcesses.bind(this)
this.handleExit()
this.reload()
}
async onIpcMain(id) {
await this.ipcMutex.acquireAsync();
try {
switch(id) {
case IPC_CLILOCAL.RELOAD: await this.reload(true); break
case IPC_CLILOCAL.GET_STATE: await this.onGetState(true); break
case IPC_CLILOCAL.DELETE_CONFIG: await this.onDeleteConfig(true); break
}
} finally {
this.ipcMutex.release();
}
}
handleExit() {
// kill CLI on exit
process.stdin.resume();//so the program will not close instantly
const exitHandler = () => {
logger.info("whirlpool-gui is terminating.")
this.stop(true) // kill immediately
process.exit()
}
//do something when app is closing
process.on('exit', exitHandler);
//catches ctrl+c event
process.on('SIGINT', exitHandler);
// catches "kill pid" (for example: nodemon restart)
process.on('SIGUSR1', exitHandler);
process.on('SIGUSR2', exitHandler);
//catches uncaught exceptions
process.on('uncaughtException', exitHandler);
}
async onGetState(gotMutex=false) {
if (!gotMutex) {
await this.ipcMutex.acquireAsync();
}
try {
this.pushState()
} finally {
if (!gotMutex) {
this.ipcMutex.release();
}
}
}
resetState() {
this.state = {
valid: undefined,
started: undefined,
status: undefined,
info: undefined,
error: undefined,
progress: undefined,
cliApi: undefined
}
}
async reload(gotMutex=false) {
logger.info("CLI reloading...")
await this.stop(gotMutex)
this.resetState()
await this.refreshState(true, gotMutex)
}
async onDeleteConfig(gotMutex=false) {
const cliConfigPath = cliApiService.getCliPath()+'/'+CLI_CONFIG_FILENAME
logger.info("CLI deleting local config... "+cliConfigPath)
await this.stop(gotMutex)
// delete local config
if (fs.existsSync(cliConfigPath)) {
try {
await fs.unlinkSync(cliConfigPath)
} catch (e) {
logger.error("unable to unlink " + cliConfigPath, e)
}
}
this.resetState()
}
getStoreOrSetDefault(key, defaultValue) {
let value = this.store.get(key)
if (value === undefined) {
this.store.set(key, defaultValue)
value = defaultValue
}
return value
}
getCliFilename() {
return this.state.cliApi.filename
}
getCliUrl() {
return this.state.cliApi.url
}
getCliChecksum() {
return this.state.cliApi.checksum
}
isCliLocal() {
return this.getStoreOrSetDefault(STORE_CLILOCAL, DEFAULT_CLI_LOCAL)
}
async refreshState(downloadIfMissing=true, gotMutex=false) {
if (cliApiService.isApiModeLocal()) {
downloadIfMissing = false
}
const javaInstalled = await this.isJavaInstalled()
if (!javaInstalled) {
this.state.valid = false
this.state.error = 'Please install JAVA 8+ (OpenJDK recommended)'
this.updateState(CLILOCAL_STATUS.ERROR)
await this.stop(gotMutex)
return
}
try {
this.state.cliApi = await cliApiService.fetchCliApi()
} catch(e) {
logger.error("Could not fetch CLI_API", e)
this.state.valid = false
this.state.error = 'Could not fetch CLI_API'
this.updateState(CLILOCAL_STATUS.ERROR)
await this.stop(gotMutex)
return
}
const myThis = this
this.state.valid = await this.verifyChecksum()
if (!this.state.valid) {
if (this.state.started) {
logger.info("CLI is invalid, stopping.")
await this.stop(gotMutex)
}
if (downloadIfMissing) {
if (this.state.status === CLILOCAL_STATUS.DOWNLOADING || this.state.status === CLILOCAL_STATUS.ERROR) {
console.log('refreshState: download skipped, status='+this.state.status+')')
return
}
// download
this.download(this.getCliUrl()).then(() => {
logger.info('CLI: download success')
myThis.state.info = undefined
myThis.state.error = undefined
myThis.state.progress = undefined
myThis.refreshState(false)
}).catch(e => {
logger.error('CLI: Download error', e)
myThis.state.info = undefined
myThis.state.error = 'Download error'
myThis.state.progress = undefined
myThis.updateState(CLILOCAL_STATUS.ERROR)
})
} else {
logger.error('CLI: download failed')
this.state.info = undefined
this.state.error = 'Could not download CLI'
myThis.state.progress = undefined
this.updateState(CLILOCAL_STATUS.ERROR)
}
} else {
this.state.error = undefined
this.updateState(CLILOCAL_STATUS.READY)
if (!this.state.started && this.isCliLocal()) {
await this.start(gotMutex)
}
}
}
async isJavaInstalled() {
try {
const result = await this.execPromise('java -version')
console.log("[CLI_LOCAL] java seems installed. "+result)
return true
} catch (e) {
console.error("[CLI_LOCAL] java is NOT installed: "+e.message)
return false
}
}
execPromise(command) {
return new Promise(function(resolve, reject) {
exec(command, (error, stdout, stderr) => {
if (error) {
reject(error);
return;
}
resolve(stdout.trim());
});
});
}
async start(gotMutex=false) {
if (!gotMutex) {
await this.ipcMutex.acquireAsync();
}
try {
if (!this.state.valid) {
console.error("[CLI_LOCAL] start skipped: not valid")
return
}
if (this.state.started) {
console.error("[CLI_LOCAL] start skipped: already started")
return
}
const myThis = this
await tcpPortUsed.waitUntilFreeOnHost(DEFAULT_CLIPORT, 'localhost', 1000, START_TIMEOUT)
.then(() => {
// port is available => start proc
myThis.state.started = new Date().getTime()
myThis.pushState()
const cmd = 'java'
const args = ['-jar', myThis.getCliFilename(), '--listen', '--debug', ARG_CLI_GUI]
if (IS_DEV) {
args.push('--debug-client')
}
myThis.startProc(cmd, args, cliApiService.getCliPath(), CLI_LOG_FILE, CLI_LOG_ERROR_FILE)
}, (e) => {
// port in use => cannot start proc
logger.error("[CLI_LOCAL] cannot start: port "+DEFAULT_CLIPORT+" already in use")
// lookup running processes
myThis.findCliProcesses(cliProcesses => {
if (cliProcesses.length > 0) {
for (const i in cliProcesses) {
const cliProcess = cliProcesses[i]
logger.debug('Foud CLI proces => killing', cliProcess);
ps.kill(cliProcess.pid, err => {
if (err) {
logger.error('Kill ' + cliProcess.pid + ' FAILED', err)
} else {
logger.debug('Kill ' + cliProcess.pid + ' SUCCESS');
}
});
}
// retry start
this.state.error = undefined
this.updateState(CLILOCAL_STATUS.READY)
myThis.start(true)
}
})
myThis.state.error = 'CLI cannot start: port '+DEFAULT_CLIPORT+' already in use'
myThis.updateState(CLILOCAL_STATUS.ERROR)
});
} finally {
if (!gotMutex) {
this.ipcMutex.release();
}
}
}
findCliProcesses(callback) {
callback = callback.bind(this)
return ps.lookup({
command: 'java',
psargs: 'ux'
}, function(err, resultList ) {
if (err) {
throw new Error( err );
}
const processes = []
resultList.forEach(function( process ){
if( process ){
if (process.arguments && process.arguments.indexOf('-jar') !== -1 && process.arguments.indexOf(ARG_CLI_GUI) !== -1) {
processes.push(process)
}
}
});
callback(processes)
});
}
startProc(cmd, args, cwd, logFile, logErrorFile) {
const cliLog = fs.createWriteStream(logFile, {flags: 'a'})
const cliLogError = fs.createWriteStream(logErrorFile, {flags: 'a'})
const myThis = this
const cmdStr = cmd+' '+args.join(' ')
cliLog.write('[CLI_LOCAL] => start: '+cmdStr+' (cwd='+cwd+')\n')
cliLogError.write('[CLI_LOCAL] => start: '+cmdStr+' (cwd='+cwd+')\n')
logger.info('[CLI_LOCAL] => start: '+cmdStr+' (cwd='+cwd+')')
try {
this.cliProc = spawn(cmd, args, { cwd: cwd })
this.cliProc.on('error', function(err) {
cliLog.write('[CLI_LOCAL][ERROR] => ' + err + '\n')
cliLogError.write('[CLI_LOCAL][ERROR] => ' + err + '\n')
logger.error('[CLI_LOCAL] => ', err)
myThis.state.info = undefined
myThis.state.error = ''+err
myThis.state.progress = undefined
myThis.updateState(CLILOCAL_STATUS.ERROR)
})
this.cliProc.on('exit', (code) => {
let reloading = false
if (code == 0) {
// finishing normal
cliLog.write('[CLI_LOCAL] => terminated without error.\n')
cliLogError.write('[CLI_LOCAL] => terminated without error.\n')
logger.info('[CLI_LOCAL] => terminated without error.')
} else {
// finishing with error
if (code === 143) {
// reloading? TODO
reloading = true
cliLog.write('[CLI_LOCAL] => terminated for reloading...\n')
cliLogError.write('[CLI_LOCAL] => terminated for reloading...\n')
logger.error('[CLI_LOCAL] => terminated for reloading...')
} else {
cliLog.write('[CLI_LOCAL][ERROR] => terminated with error: ' + code + '\n')
cliLogError.write('[CLI_LOCAL][ERROR] => terminated with error: ' + code + '\n')
logger.error('[CLI_LOCAL][ERROR] => terminated with error: ' + code + '. Check logs for details')
myThis.state.info = undefined
myThis.state.error = 'CLI terminated with error: '+code+'. Check logs for details.'
myThis.state.progress = undefined
myThis.updateState(CLILOCAL_STATUS.ERROR)
}
}
if (!reloading) {
myThis.stop(true, false) // just update state
}
})
this.cliProc.stdout.on('data', function(data) {
const dataStr = data.toString()
const dataLine = dataStr.substring(0, (dataStr.length - 1))
console.log('[CLI_LOCAL] ' + dataLine);
cliLog.write(data)
// log java errors to CLI error file
if (dataStr.match(/ ERROR ([0-9]+) \-\-\- /g)) {
console.error('[CLI_LOCAL][ERROR] ' + dataLine);
logger.error('[CLI_LOCAL][ERROR] ' + dataLine)
cliLogError.write('[ERROR]' + data)
}
});
this.cliProc.stderr.on('data', function(data) {
const dataStr = data.toString()
const dataLine = dataStr.substring(0, (dataStr.length - 1))
console.error('[CLI_LOCAL][ERROR] ' + dataLine);
logger.error('[CLI_LOCAL][ERROR] ' + dataLine)
cliLog.write('[ERROR]' + data)
cliLogError.write('[ERROR]' + data)
//myThis.state.error = dataLine
//myThis.updateState(CLILOCAL_STATUS.ERROR)
});
} catch(e) {
myThis.stop(true)
}
}
async stop(gotMutex=false, kill=true) {
if (!gotMutex) {
await this.ipcMutex.acquireAsync();
}
try {
if (!this.state.started) {
console.error("CliLocal: stop skipped: not started")
return
}
this.state.started = false
this.pushState()
if (this.cliProc && kill) {
logger.info('CLI stop')
this.cliProc.stdout.pause()
this.cliProc.stderr.pause()
this.cliProc.kill()
}
} finally {
if (!gotMutex) {
this.ipcMutex.release();
}
}
}
async verifyChecksum() {
const dlPathFile = cliApiService.getCliPath()+'/'+this.getCliFilename()
const expectedChecksum = this.getCliChecksum()
try {
const checksum = await this.sha256File(dlPathFile)
if (!checksum) {
logger.error('CLI not found: '+dlPathFile)
return false;
}
if (cliApiService.useChecksum() && checksum !== expectedChecksum) {
logger.error('CLI is invalid: '+dlPathFile+', '+checksum+' vs '+expectedChecksum)
return false;
}
logger.debug('CLI is valid: ' + dlPathFile)
return true
} catch(e) {
logger.error('CLI not found: '+dlPathFile)
return false;
}
}
async download(url) {
// delete existing file if any
const dlPathFile = cliApiService.getCliPath()+'/'+this.getCliFilename()
if (fs.existsSync(dlPathFile)) {
logger.verbose('CLI overwriting '+dlPathFile)
try {
await fs.unlinkSync(dlPathFile)
} catch (e) {
logger.error("unable to unlink " + dlPathFile, e)
}
}
this.updateState(CLILOCAL_STATUS.DOWNLOADING)
const onProgress = progress => {
logger.verbose('CLI downloading, progress='+progress)
const progressPercent = parseInt(progress * 100)
this.state.progress = progressPercent
this.updateState(CLILOCAL_STATUS.DOWNLOADING)
}
logger.info('CLI downloading: '+url)
return download(this.window, url, {directory: cliApiService.getCliPath(), onProgress: onProgress.bind(this)})
}
updateState(status) {
this.state.status = status
this.pushState()
}
pushState () {
console.log('cliLocal: pushState',this.state)
this.window.send(IPC_CLILOCAL.STATE, this.state)
}
sha256File(filename, algorithm = 'sha256') {
return new Promise((resolve, reject) => {
let shasum = crypto.createHash(algorithm);
try {
let s = fs.ReadStream(filename)
s.on('data', function (data) {
shasum.update(data)
})
// making digest
s.on('end', function () {
const hash = shasum.digest('hex')
return resolve(hash);
})
s.on('error', function () {
return reject('file read error: '+filename);
})
} catch (error) {
return reject('checksum failed');
}
});
}
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import fetch from 'node-fetch';
import { VERSIONS_URL } from '../const';
class CliVersion {
constructor() {
}
fetchVersions() {
return fetch(VERSIONS_URL, {cache: "no-store"})
.then(res => res.json())
}
fetchCliApi(cliApi) {
return this.fetchVersions().then(json => {
console.log('cliVersions',json)
return json.CLI_API[cliApi]
})
}
}
const cliVersion = new CliVersion()
export default cliVersion
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import ifNot from 'if-not-running';
import { GUI_VERSION } from '../const';
import cliService from './cliService';
import backendService from './backendService';
import guiConfig from '../mainProcess/guiConfig';
import { API_MODES } from '../mainProcess/cliApiService';
const REFRESH_RATE = 1800000; //30min
class GuiUpdateService {
constructor () {
this.setState = undefined
this.state = undefined
this.refreshTimeout = undefined
}
init (state, setState) {
ifNot.run('guiUpdateService:init', () => {
this.setState = setState
if (this.state === undefined) {
console.log('guiState: init...')
if (state !== undefined) {
console.log('guiState: load', Object.assign({}, state))
this.state = state
}
} else {
console.log('guiState: already initialized')
}
})
}
start() {
if (this.refreshTimeout === undefined) {
this.refreshTimeout = setInterval(this.fetchState.bind(this), REFRESH_RATE)
return this.fetchState()
}
return Promise.resolve()
}
stop() {
if (this.refreshTimeout) {
clearInterval(this.refreshTimeout)
}
this.refreshTimeout = undefined
this.state = {}
}
isReady() {
return this.state && this.state.guiLast
}
// getUpdate
getGuiUpdate () {
if (!this.isReady() || !cliService.isConnected() || guiConfig.getApiMode() !== API_MODES.RELEASE) {
return undefined
}
const apiMode = guiConfig.getApiMode()
const serverName = apiMode === API_MODES.QA ? API_MODES.QA : cliService.getServerName()
if (serverName && this.state.guiLast[serverName] && this.state.guiLast[serverName] !== GUI_VERSION) {
// update available
return this.state.guiLast[serverName]
}
// no update available
return undefined
}
fetchState () {
return ifNot.run('guiUpdateService:fetchState', () => {
// fetch GUI_LAST
return backendService.gui.versions()
.then(json => {
// set state
if (this.state === undefined) {
console.log('guiUpdateService: initializing new state')
this.state = {
guiLast: json.GUI.LAST
}
} else {
console.log('guiUpdateService: updating existing state', Object.assign({}, this.state))
this.state.guiLast = json.GUI.LAST
}
this.pushState()
})
})
}
pushState () {
this.setState(this.state)
}
}
const guiUpdateService = new GuiUpdateService()
export default guiUpdateService
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import uniqueId from 'lodash/uniqueId';
import { statusActions } from './statusActions';
const STATUS_SUCCESS_CLEAR = 10000
class Status {
constructor () {
}
executeWithStatus (mainLabel, label, executor, dispatch, itemId, silent=false) {
if (itemId === undefined) {
itemId = uniqueId()
}
if (!silent) {
dispatch(statusActions.add(itemId, mainLabel, label, executor))
}
const promise = executor()
// important: keep promise variable unchanged to return initial promise
promise
.then(() => {
if (!silent) {
dispatch(statusActions.success(itemId))
setTimeout(() => dispatch(statusActions.clear(itemId)),
STATUS_SUCCESS_CLEAR
)
}
})
.catch(error => {
console.error(`failed: ${label}`, error)
dispatch(statusActions.error(itemId, error.message))
})
return promise
}
}
const status = new Status()
export default status
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import cliService from './cliService';
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome';
import * as Icons from '@fortawesome/free-solid-svg-icons';
import * as React from 'react';
import { shell } from 'electron';
const AMOUNT_PRECISION = 4
const BTC_TO_SAT = 100000000
export const TX0_MIN_CONFIRMATIONS = 0
export const MIXSTARGET_UNLIMITED = 0
export const DATETIME_FORMAT = 'YYYY-MM-DD HH:mm:ss'
export const WHIRLPOOL_ACCOUNTS = {
DEPOSIT: 'DEPOSIT',
PREMIX: 'PREMIX',
POSTMIX: 'POSTMIX'
}
export const UTXO_STATUS = {
READY: 'READY',
STOP: 'STOP',
TX0: 'TX0',
TX0_FAILED: 'TX0_FAILED',
TX0_SUCCESS: 'TX0_SUCCESS',
MIX_QUEUE: 'MIX_QUEUE',
MIX_STARTED: 'MIX_STARTED',
MIX_SUCCESS: 'MIX_SUCCESS',
MIX_FAILED: 'MIX_FAILED'
}
export const MIXABLE_STATUS = {
MIXABLE: 'MIXABLE',
UNCONFIRMED: 'UNCONFIRMED',
HASH_MIXING: 'HASH_MIXING',
NO_POOL: 'NO_POOL'
}
export const CLI_STATUS = {
NOT_INITIALIZED: 'NOT_INITIALIZED',
NOT_READY: 'NOT_READY',
READY: 'READY'
}
class Utils {
constructor () {
}
fetch(input, init, json=false) {
return fetch(input, init).then(resp => {
if (!resp) {
const message = 'Fetch failed'
console.error('fetch error:', message)
return Promise.reject(new Error(message))
}
if (!resp.ok) {
if (resp.body) {
return resp.json().then(responseError => {
const message = responseError.message ? responseError.message : 'Error ' + resp.status
console.error('fetch error: ' + message, responseError)
return Promise.reject(new Error(message))
})/*.catch(e => { // no json response
const message = 'Error '+resp.status
console.error('fetch error: '+message, e)
return Promise.reject(new Error(message))
})*/
} else {
const message = 'Error '+resp.status
console.error('fetch error: '+message, e)
return Promise.reject(new Error(message))
}
}
if (json) {
return resp.json()
}
return resp
})
}
sumUtxos (utxos) {
return utxos.reduce(function (sum, utxo) {
return sum + utxo.value;
}, 0);
}
scale (value, precision) {
const factor = Math.pow(10, precision)
return Math.floor(value * factor) / factor
}
toBtc (sats, scale = false) {
if (sats === 0) {
return 0
}
let valueBtc = sats / BTC_TO_SAT
if (scale === true) valueBtc = this.scale(valueBtc, AMOUNT_PRECISION)
return valueBtc
}
linkExplorer(utxo) {
if (cliService.isTestnet()) {
return 'https://blockstream.info/testnet/tx/'+utxo.hash
}
return 'https://oxt.me/transaction/'+utxo.hash
}
statusIcon(utxo) {
if (utxo.status === UTXO_STATUS.MIX_STARTED) {
return <FontAwesomeIcon icon={Icons.faPlay} size='xs' color='green' title='MIXING'/>
}
if (utxo.status === UTXO_STATUS.TX0) {
return <FontAwesomeIcon icon={Icons.faPlay} size='xs' color='green' title='TX0'/>
}
if (utxo.status === UTXO_STATUS.TX0_FAILED) {
return <FontAwesomeIcon icon={Icons.faSquare} size='xs' color='red' title='TX0 FAILED'/>
}
if (utxo.status === UTXO_STATUS.MIX_FAILED) {
return <FontAwesomeIcon icon={Icons.faSquare} size='xs' color='red' title='MIX FAILED'/>
}
if ((utxo.account === WHIRLPOOL_ACCOUNTS.POSTMIX && (utxo.mixsTargetOrDefault != MIXSTARGET_UNLIMITED || utxo.mixsDone >= utxo.mixsTargetOrDefault) && utxo.mixableStatus === MIXABLE_STATUS.MIXABLE) || utxo.status === UTXO_STATUS.MIX_SUCCESS) {
return <FontAwesomeIcon icon={Icons.faCheck} size='xs' color='green' title='MIXED'/>
}
switch(utxo.mixableStatus) {
case MIXABLE_STATUS.MIXABLE:
return <FontAwesomeIcon icon={Icons.faCircle} size='xs' color='green' title='Ready to mix'/>
case MIXABLE_STATUS.HASH_MIXING:
return <FontAwesomeIcon icon={Icons.faEllipsisH} size='xs' color='orange' title='Another utxo from same hash is currently mixing'/>
case MIXABLE_STATUS.NO_POOL:
return <span></span>
case MIXABLE_STATUS.UNCONFIRMED:
return <FontAwesomeIcon icon={Icons.faClock} size='xs' color='orange' title='Unconfirmed'/>
}
return undefined
}
statusLabel(utxo) {
const icon = this.statusIcon(utxo)
const text = this.statusLabelText(utxo)
return <span>{icon?icon:''} {text?text:''}</span>
}
statusLabelText(utxo) {
switch(utxo.status) {
case UTXO_STATUS.READY:
if (utxo.account === WHIRLPOOL_ACCOUNTS.POSTMIX && (utxo.mixsTargetOrDefault != MIXSTARGET_UNLIMITED || utxo.mixsDone >= utxo.mixsTargetOrDefault)) {
return 'MIXED'
}
if (utxo.mixableStatus === MIXABLE_STATUS.NO_POOL) {
return undefined
}
return 'READY'
case UTXO_STATUS.STOP: return 'STOPPED'
case UTXO_STATUS.TX0: return 'TX0'
case UTXO_STATUS.TX0_SUCCESS: return 'TX0:SUCCESS'
case UTXO_STATUS.TX0_FAILED: return 'TX0:ERROR'
case UTXO_STATUS.MIX_QUEUE: return 'QUEUE'
case UTXO_STATUS.MIX_STARTED: return 'MIXING'
case UTXO_STATUS.MIX_SUCCESS: return 'MIXED'
case UTXO_STATUS.MIX_FAILED: return 'MIX:ERROR'
default: return '?'
}
}
utxoMessage(utxo) {
if (utxo.error) {
return utxo.error
}
return utxo.message
}
utxoPathChain(utxo) {
if (!utxo.path) {
return undefined
}
var matches = utxo.path.match(/M\/([0-9]+)\/[0-9]+$/)
return parseInt(matches[1])
}
isUtxoPostmixSpending(utxo) {
if (utxo.account === WHIRLPOOL_ACCOUNTS.POSTMIX) {
const utxoPathChain = this.utxoPathChain(utxo)
if (utxoPathChain && utxoPathChain !== 0) {
return true
}
}
return false
}
isUtxoReadOnly(utxo) {
// POSTMIX spendings (chains <> 0) as read only
if (this.isUtxoPostmixSpending(utxo)) {
return true
}
return false
}
mixsTargetLabel(mixsTarget) {
return mixsTarget !== MIXSTARGET_UNLIMITED ? mixsTarget : '∞'
}
torIcon(width=20) {
return <svg width={width} version="1.1" viewBox="0 0 32 32" xmlns="http://www.w3.org/2000/svg">
<g transform="translate(-58.12 -303.3)">
<path d="m77.15 303.3c-1.608 1.868-0.09027 2.972-0.9891 4.84 1.514-2.129 5.034-2.862 7.328-3.643-3.051 2.72-5.457 6.326-8.489 9.009l-1.975-0.8374c-0.4647-4.514-1.736-4.705 4.125-9.369z" fillRule="evenodd"/>
<path d="m74.04 313.1 2.932 0.9454c-0.615 2.034 0.3559 2.791 0.9472 3.123 1.324 0.7332 2.602 1.49 3.619 2.412 1.916 1.75 3.004 4.21 3.004 6.812 0 2.578-1.183 5.061-3.169 6.717-1.868 1.561-4.446 2.223-6.953 2.223-1.561 0-2.956-0.0708-4.47-0.5677-3.453-1.159-6.031-4.115-6.244-7.663-0.1893-2.767 0.4257-4.872 2.578-7.072 1.111-1.159 2.563-2.749 4.1-3.813 0.757-0.5204 1.119-1.191-0.4183-3.958l1.28-1.076 2.795 1.918-2.352-0.3007c0.1656 0.2366 1.189 0.7706 1.284 1.078 0.2128 0.8751-0.1911 1.771-0.3804 2.149-0.9696 1.75-1.86 2.275-3.066 3.268-2.129 1.75-4.27 2.836-4.01 7.637 0.1183 2.365 1.433 5.295 4.2 6.643 1.561 0.757 2.859 1.189 4.68 1.284 1.632 0.071 4.754-0.8988 6.457-2.318 1.821-1.514 2.838-3.808 2.838-6.149 0-2.365-0.9461-4.612-2.72-6.197-1.017-0.9223-2.696-2.034-3.737-2.625-1.041-0.5912-2.782-2.06-2.356-3.645z"/>
<path d="m73.41 316.6c-0.186 1.088-0.4177 3.117-0.8909 3.917-0.3293 0.5488-0.4126 0.8101-0.7846 1.094-1.09 1.535-1.45 1.761-2.132 4.552-0.1447 0.5914-0.3832 1.516-0.2591 2.107 0.372 1.703 0.6612 2.874 1.316 4.103 0 0 0.1271 0.1217 0.1271 0.169 0.6821 0.9225 0.6264 1.05 2.665 2.246l-0.06204 0.3313c-1.55-0.4729-2.604-0.9591-3.41-2.024 0-0.0236-0.1513-0.1558-0.1513-0.1558-0.868-1.135-1.753-2.788-2.021-4.546-0.1447-0.7097-0.0769-1.341 0.08833-2.075 0.7026-2.885 1.415-4.093 2.744-5.543 0.3514-0.2601 0.6704-0.6741 1.001-1.092 0.4859-0.6764 1.462-2.841 1.814-4.189z"/>
<path d="m74.09 318.6c0.0237 1.04 0.0078 3.036 0.3389 3.796 0.0945 0.2599 0.3274 1.414 0.9422 2.794 0.4258 0.96 0.5418 1.933 0.6128 2.193 0.2838 1.14-0.4002 3.086-0.8734 4.906-0.2364 0.98-0.6051 1.773-1.371 2.412l0.2796 0.3593c0.5204-0.02 1.954-1.096 2.403-2.416 0.757-2.24 1.328-3.317 0.9729-5.797-0.0473-0.2402-0.2094-1.134-0.6588-2.014-0.6622-1.34-1.474-2.614-1.592-2.874-0.213-0.4198-1.007-2.119-1.054-3.359z"/>
<path d="m74.88 313.9 0.9727 0.4962c-0.09145 0.6403 0.04572 2.059 0.686 2.424 2.836 1.761 5.512 3.683 6.565 5.604 3.751 6.771-2.63 13.04-8.143 12.44 2.996-2.219 4.428-6.583 3.307-11.55-0.4574-1.944-1.729-3.893-2.987-5.883-0.545-0.9768-0.3547-2.188-0.4006-3.538z" fillRule="evenodd"/>
<rect x="73.07" y="312.8" width="1" height="22"/>
</g>
</svg>
}
openExternal(url) {
shell.openExternal(url)
}
checkedIcon(checked) {
return <FontAwesomeIcon icon={checked?Icons.faCheck:Icons.faTimes} color={checked?'green':'red'} />
}
}
const utils = new Utils()
export default utils
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import ifNot from 'if-not-running';
import moment from 'moment';
import backendService from './backendService';
import { TX0_MIN_CONFIRMATIONS, WHIRLPOOL_ACCOUNTS } from './utils';
import poolsService from './poolsService';
import walletService from './walletService';
const REFRESH_RATE = 3000;
class MixService {
constructor () {
this.setState = undefined
this.state = undefined
this.refreshTimeout = undefined
}
init (state, setState) {
ifNot.run('mixService:init', () => {
this.setState = setState
if (this.state === undefined) {
console.log('mixState: init...')
if (state !== undefined) {
console.log('mixState: load', Object.assign({}, state))
this.state = state
}
} else {
console.log('mixState: already initialized')
}
})
}
start() {
if (this.refreshTimeout === undefined) {
this.refreshTimeout = setInterval(this.fetchState.bind(this), REFRESH_RATE)
return this.fetchState()
}
return Promise.resolve()
}
stop() {
if (this.refreshTimeout) {
clearInterval(this.refreshTimeout)
}
this.refreshTimeout = undefined
this.state = {}
}
isReady() {
return this.state && this.state.mix
}
// controls global
startMix() {
return backendService.mix.start().then(() => this.fetchState())
}
stopMix() {
return backendService.mix.stop().then(() => this.fetchState())
}
// controls utxo
getPoolsForTx0(utxo) {
return poolsService.getPoolsForTx0(utxo.value)
}
isTx0Possible(utxo) {
return (utxo.account === WHIRLPOOL_ACCOUNTS.DEPOSIT || utxo.account === WHIRLPOOL_ACCOUNTS.PREMIX)
&& (utxo.status === 'READY' || utxo.status === 'TX0_FAILED')
&& utxo.confirmations >= TX0_MIN_CONFIRMATIONS
&& this.getPoolsForTx0(utxo).length > 0
}
setPoolId(utxo, poolId) {
utxo.poolId = poolId
return this.configure(utxo)
}
setMixsTarget(utxo, mixsTarget) {
utxo.mixsTarget = mixsTarget
return this.configure(utxo)
}
configure(utxo) {
return backendService.utxo.configure(utxo.hash, utxo.index, utxo.poolId, utxo.mixsTarget).then(() => walletService.fetchState())
}
tx0(utxo, feeTarget, poolId, mixsTarget) {
return backendService.utxo.tx0(utxo.hash, utxo.index, feeTarget, poolId, mixsTarget).then(() => walletService.fetchState())
}
startMixUtxo(utxo) {
return backendService.utxo.startMix(utxo.hash, utxo.index).then(() => this.refresh())
}
refresh() {
const walletState = walletService.fetchState()
const state = this.fetchState()
return Promise.all([walletState, state])
}
stopMixUtxo(utxo) {
return backendService.utxo.stopMix(utxo.hash, utxo.index).then(() => this.refresh())
}
getPoolsForMix(utxo) {
const liquidity = utxo.account === WHIRLPOOL_ACCOUNTS.POSTMIX
return poolsService.getPoolsForMix(utxo.value, liquidity)
}
isStartMixPossible(utxo) {
return (utxo.account === WHIRLPOOL_ACCOUNTS.PREMIX || utxo.account === WHIRLPOOL_ACCOUNTS.POSTMIX)
&& (utxo.status === 'MIX_FAILED' || utxo.status === 'READY')
&& this.getPoolsForMix(utxo).length > 0
}
isStopMixPossible(utxo) {
return (utxo.account === WHIRLPOOL_ACCOUNTS.PREMIX || utxo.account === WHIRLPOOL_ACCOUNTS.POSTMIX)
&& (utxo.status === 'MIX_QUEUE' || utxo.status === 'MIX_STARTED' || utxo.status === 'MIX_SUCCESS')
}
// state
computeLastActivity(utxo) {
if (!utxo.lastActivityElapsed) {
return undefined
}
const fetchElapsed = new Date().getTime()-this.state.mix.fetchTime
return moment.duration(fetchElapsed + utxo.lastActivityElapsed).humanize()
}
isStarted () {
return this.state.mix.started;
}
getNbMixing() {
return this.state.mix.nbMixing;
}
getNbQueued() {
return this.state.mix.nbQueued;
}
getThreads() {
return this.state.mix.threads;
}
fetchState () {
return ifNot.run('mixService:fetchState', () => {
// fetchState backend
return backendService.mix.fetchState().then(mix => {
mix.fetchTime = new Date().getTime()
// set state
if (this.state === undefined) {
console.log('mixService: initializing new state')
this.state = {
mix: mix
}
} else {
// new state object
const currentState = Object.assign({}, this.state)
console.log('mixService: updating existing state', currentState)
currentState.mix = mix
this.state = currentState
}
this.pushState()
})
})
}
pushState () {
this.setState(this.state)
}
}
const mixService = new MixService()
export default mixService
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import ifNot from 'if-not-running';
import backendService from './backendService';
import { logger } from '../utils/logger';
import cliService from './cliService';
import { cliLocalService } from './cliLocalService';
export class CliConfigService {
constructor (setState=()=>{}) {
this.setState = setState
this.state = {
cliConfig: undefined
}
this.load()
}
// state
getCliConfig() {
if (!this.state.cliConfig) {
return undefined
}
return this.state.cliConfig;
}
getServer() {
if (!this.state.cliConfig) {
return undefined
}
return this.state.cliConfig.server;
}
save (cliConfig) {
return backendService.cli.setConfig(cliConfig).then(() => {
logger.info('Save CLI configuration: success')
cliLocalService.reload()
cliService.fetchState()
}).catch(e => {
logger.error('Save CLI configuration: failed', e)
throw e
})
}
load () {
return ifNot.run('cliConfigService:fetchState', () => {
// fetchState backend
return backendService.cli.getConfig().then(cliConfigResponse => {
const cliConfig = cliConfigResponse.config
console.log('cliConfigService.load',cliConfig)
// set state
if (this.state === undefined) {
console.log('cliConfigService: initializing new state')
this.state = {
cliConfig: cliConfig
}
} else {
// new state object
const currentState = Object.assign({}, this.state)
console.log('cliConfigService: updating existing state', currentState)
currentState.cliConfig = cliConfig
this.state = currentState
}
this.pushState()
})
})
}
pushState () {
this.setState(this.state.cliConfig)
}
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import * as React from 'react';
import ifNot from 'if-not-running';
import { logger } from '../utils/logger';
import backendService from './backendService';
import utils, { CLI_STATUS } from './utils';
import mixService from './mixService';
import walletService from './walletService';
import poolsService from './poolsService';
import { cliLocalService } from './cliLocalService';
import { DEFAULT_CLI_LOCAL } from '../const';
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome';
import * as Icons from '@fortawesome/free-solid-svg-icons';
import guiUpdateService from './guiUpdateService';
import routes from '../constants/routes';
import { Link } from 'react-router-dom';
import guiConfig from '../mainProcess/guiConfig';
const REFRESH_RATE = 6000;
class CliService {
constructor () {
this.setState = undefined
this.state = undefined
this.refreshTimeout = undefined
this.servicesStarted = false
this.cliUrl = undefined
this.apiKey = undefined
this.cliLocal = undefined
this.loadConfig()
}
init (state, setState) {
ifNot.run('cliService:init', () => {
this.setState = setState
if (this.state === undefined) {
console.log('cliState: init...')
if (state !== undefined) {
console.log('cliState: load', Object.assign({}, state))
this.state = state
}
} else {
console.log('cliState: already initialized')
}
})
}
start() {
if (this.refreshTimeout === undefined) {
this.refreshTimeout = setInterval(this.fetchState.bind(this), REFRESH_RATE)
return this.fetchState()
}
}
startServices() {
if (!this.servicesStarted) {
this.servicesStarted = true
mixService.start()
walletService.start()
poolsService.start()
}
}
stopServices() {
if (this.servicesStarted) {
this.servicesStarted = false
mixService.stop()
walletService.stop()
poolsService.stop()
}
}
isConnected() {
return this.state && this.state.cli
}
isConfigured() {
return this.cliUrl && this.apiKey
}
isCliLocal() {
return this.cliLocal
}
// cli API
getCliUrl() {
return this.cliUrl
}
getApiKey() {
return this.apiKey
}
testCliUrl(cliUrl, apiKey) {
return this.doFetchState(cliUrl, apiKey).then(cliState => {
return cliState.cliStatus === CLI_STATUS.READY
})
}
fetchStateError(error) {
if (error.message === 'Failed to fetch') {
error = Error('Could not connect to CLI (may take a few seconds to start...)')
}
return error
}
initializeCli(cliUrl, apiKey, cliLocal, pairingPayload, tor, dojo) {
return backendService.cli.init(cliUrl, apiKey, pairingPayload, tor, dojo).then(result => {
const apiKey = result.apiKey
// save configuration
this.saveConfig(cliUrl, apiKey, cliLocal)
})
}
saveConfig(cliUrl, apiKey, cliLocal) {
this.cliUrl = cliUrl
this.apiKey = apiKey
guiConfig.setCliUrl(cliUrl)
guiConfig.setCliApiKey(apiKey)
this.setCliLocal(cliLocal)
this.start()
}
loadConfig() {
this.cliUrl = guiConfig.getCliUrl()
this.apiKey = guiConfig.getCliApiKey()
this.cliLocal = guiConfig.getCliLocal()
console.log('cliService.loadConfig: cliUrl='+this.cliUrl)
if (!this.isConfigured()) {
logger.info('cliService is not configured.')
this.setCliLocal(DEFAULT_CLI_LOCAL)
}
}
setCliLocal(cliLocal) {
logger.info("cliService.setCliLocal: "+cliLocal)
this.cliLocal = cliLocal
guiConfig.setCliLocal(cliLocal)
if (!cliLocal) {
cliLocalService.deleteConfig()
}
cliLocalService.reload()
}
getResetLabel() {
let resetLabel = 'GUI'
if (this.isCliLocal()) {
resetLabel += ' + CLI config'
}
return resetLabel
}
resetConfig() {
if (this.isCliLocal()) {
// reset CLI first
cliLocalService.deleteConfig()
}
this.doResetGUIConfig()
}
doResetGUIConfig() {
// reset GUI
guiConfig.resetCliConfig()
this.cliUrl = undefined
this.apiKey = undefined
this.setCliLocal(DEFAULT_CLI_LOCAL)
// force refresh
this.updateState({
cli: undefined,
cliUrlError: undefined,
cliLocalState: undefined
})
cliLocalService.reload()
}
login(seedPassphrase) {
return backendService.cli.login(seedPassphrase).then(cliState => {
this.updateState({
cli: cliState,
cliUrlError: undefined
})
})
}
logout() {
return backendService.cli.logout().then(cliState => {
this.updateState({
cli: cliState,
cliUrlError: undefined
})
})
}
// state
getCliUrlError() {
return this.state ? this.state.cliUrlError : undefined
}
getCliStatus() {
if (!this.isConnected()) {
return undefined
}
return this.state.cli.cliStatus;
}
getCliMessage() {
if (!this.isConnected()) {
return undefined
}
return this.state.cli.cliMessage;
}
getNetwork() {
if (!this.isConnected()) {
return undefined
}
return this.state.cli.network;
}
getServerUrl() {
if (!this.isConnected()) {
return undefined
}
return this.state.cli.serverUrl;
}
getServerName() {
if (!this.isConnected()) {
return undefined
}
return this.state.cli.serverName;
}
isTor() {
if (!this.isConnected()) {
return undefined
}
return this.state.cli.tor;
}
isDojo() {
if (!this.isConnected()) {
return undefined
}
return this.state.cli.dojo;
}
isDojoPossible() {
if (!this.isConnected()) {
return undefined
}
return this.getDojoUrl() ? true : false
}
getDojoUrl() {
if (!this.isConnected()) {
return undefined
}
return this.state.cli.dojoUrl;
}
isTestnet() {
return this.getNetwork() === 'test'
}
isCliStatusReady() {
return this.isConnected() && this.state.cli.cliStatus === CLI_STATUS.READY
}
isCliStatusNotInitialized() {
return this.isConnected() && this.state.cli.cliStatus === CLI_STATUS.NOT_INITIALIZED
}
isLoggedIn() {
return this.isCliStatusReady() && this.state.cli.loggedIn
}
getTorProgress() {
return this.isCliStatusReady() && this.state.cli.torProgress
}
getTorProgressIcon() {
const torProgress = this.getTorProgress()
if (!torProgress) {
return undefined
}
const connected = torProgress === 100
return <span className={'torIcon torIcon'+(cliService.isTor() ? (connected?'Connected':'Connecting'):'Disabled')} title={'Tor is '+(cliService.isTor() ?(connected?'CONNECTED':'CONNECTING '+torProgress+'%'):'DISABLED')}>
{utils.torIcon()}
{!connected && <span>{torProgress+'%'}</span>}
</span>
}
getDojoIcon() {
if (!this.isConnected() || !this.isDojo()) {
return undefined
}
return <span className='dojoIcon' title={'DOJO is ENABLED: '+this.getDojoUrl()}><FontAwesomeIcon icon={Icons.faHdd} color='green'/></span>
}
setCliLocalState(cliLocalState) {
const newState = {cliLocalState: cliLocalState}
if (cliLocalState !== undefined && cliLocalState.error) {
// forward local CLI error
newState.cliUrlError = cliLocalState.error
newState.cli = undefined
}
this.updateState(newState)
}
doFetchState(cliUrl=undefined, apiKey=undefined) {
return backendService.cli.fetchState(cliUrl, apiKey).catch(e => {
throw this.fetchStateError(e)
})
}
fetchState () {
if (this.isCliLocal()) {
cliLocalService.fetchState()
if (!cliLocalService.isStarted()) {
return Promise.reject("local CLI not started yet")
}
}
return ifNot.run('cliService:fetchState', () => {
// fetchState backend
return this.doFetchState().then(cliState => {
this.updateState({
cli: cliState,
cliUrlError: undefined
})
// start guiUpdateService
guiUpdateService.start()
// notify services
if (this.isLoggedIn()) {
this.startServices()
} else {
this.stopServices()
}
}).catch(e => {
// notify services
this.updateState({
cli: undefined,
cliUrlError: e.message
})
this.stopServices()
})
})
}
updateState(newState) {
// set state
if (this.state === undefined) {
console.log('cliService: initializing new state')
this.state = newState
} else {
// new state object
const currentState = Object.assign({}, this.state)
console.log('cliService: updating existing state', currentState)
for (const key in newState) {
currentState[key] = newState[key]
}
this.state = currentState
}
this.pushState()
}
pushState () {
this.setState(this.state)
}
getStatusIcon(format) {
if (cliService.isCliStatusReady()) {
// connected & ready
const status = 'Connected to CLI'
return format(<FontAwesomeIcon icon={Icons.faWifi} color='green' title={status} />, status)
}
if (cliService.getCliUrlError()) {
// not connected
const status = 'Disconnected from CLI'
return format(<FontAwesomeIcon icon={Icons.faWifi} color='red' title={status} />, status)
}
// connected & initialization required
if (cliService.isCliStatusNotInitialized()) {
const status = 'Connected to CLI, initialization required'
return format(<FontAwesomeIcon icon={Icons.faWifi} color='orange' title={status}/>, status)
}
// connected & not ready
if (cliService.isConnected()) {
let cliMessage = cliService.getCliMessage()
if (!cliMessage) {
cliMessage = 'starting...'
}
const status = 'Connected to CLI, which is not ready: '+cliMessage
return format(<FontAwesomeIcon icon={Icons.faWifi} color='lightgreen' title={status}/>, status)
}
}
getLoginStatusIcon(format) {
if (cliService.isLoggedIn()) {
// logged in
const status = 'Wallet opened'
return format(<a href='#' title={status} onClick={()=>cliService.logout()} className='icon'><FontAwesomeIcon icon={Icons.faSignOutAlt} color='#CCC'/></a>, status)
}
const status = 'Wallet closed'
return format(<Link to={routes.HOME} title={status} className='icon'><FontAwesomeIcon icon={Icons.faLock} color='#CCC' /></Link>, status)
}
}
const cliService = new CliService()
export default cliService
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import utils from './utils';
import status from './status';
import cliService from './cliService';
import { cliApiService, VERSIONS_URL } from '../const';
const HEADER_API_VERSION = 'apiVersion'
const HEADER_API_KEY = 'apiKey'
class BackendService {
constructor () {
this.dispatch = undefined
}
init (dispatch) {
this.dispatch = dispatch
}
computeHeaders (apiKey=undefined) {
const headers = {
'Content-Type': 'application/json'
}
headers[HEADER_API_VERSION] = cliApiService.getApiVersion()
if (apiKey === undefined) {
apiKey = cliService.getApiKey()
}
headers[HEADER_API_KEY] = apiKey
return headers
}
fetchBackend (url, method, jsonBody=undefined, parseJson=false, cliUrl=undefined, apiKey=undefined) {
if (!cliUrl) {
cliUrl = cliService.getCliUrl()
}
return utils.fetch(cliUrl + url, {
method,
headers: this.computeHeaders(apiKey),
body: jsonBody !== undefined ? JSON.stringify(jsonBody) : undefined
}, parseJson)
}
fetchBackendAsJson (url, method, jsonBody=undefined, cliUrl=undefined, apiKey=undefined) {
return this.fetchBackend(url, method, jsonBody, true, cliUrl, apiKey)
}
withStatus (mainLabel, label, executor, itemId, silent=false) {
console.log(`=> req backend: ${mainLabel}: ${label}`)
return status.executeWithStatus(
mainLabel,
label,
executor,
this.dispatch,
itemId,
silent
)
}
cli = {
fetchState: (cliUrl=undefined, apiKey=undefined) => {
return this.withStatus('CLI', 'Fetch state', () =>
this.fetchBackendAsJson('/rest/cli', 'GET', undefined, cliUrl, apiKey)
, 'cli.status', true)
},
init: (cliUrl, apiKey, pairingPayload, tor, dojo) => {
return this.withStatus('CLI', 'Initial setup', () =>
this.fetchBackendAsJson('/rest/cli/init', 'POST', {
pairingPayload: pairingPayload,
tor: tor,
dojo: dojo
}, cliUrl, apiKey)
, 'cli.init')
},
login: (seedPassphrase) => {
return this.withStatus('CLI', 'Authenticate', () =>
this.fetchBackendAsJson('/rest/cli/login', 'POST', {
seedPassphrase: seedPassphrase
})
, 'cli.login')
},
logout: () => {
return this.withStatus('CLI', 'Authenticate', () =>
this.fetchBackendAsJson('/rest/cli/logout', 'POST')
, 'cli.logout')
},
getConfig: () => {
return this.withStatus('CLI', 'Fetch configuration', () =>
this.fetchBackendAsJson('/rest/cli/config', 'GET')
, 'cli.getConfig')
},
setConfig: (config) => {
return this.withStatus('CLI', 'Save configuration', () =>
this.fetchBackend('/rest/cli/config', 'POST', {
config: config
})
, 'cli.setConfig')
},
resetConfig: () => {
return this.withStatus('CLI', 'Reset configuration', () =>
this.fetchBackend('/rest/cli/config', 'DELETE')
, 'cli.resetConfig')
}
};
pools = {
fetchPools: (tx0FeeTarget=undefined) => {
return this.withStatus('Pools', 'Fetch pools', () =>
this.fetchBackendAsJson('/rest/pools'+(tx0FeeTarget!=undefined?'?tx0FeeTarget='+tx0FeeTarget:''), 'GET')
, 'pools.fetchPools', true)
}
};
wallet = {
fetchUtxos: () => {
return this.withStatus('Wallet', 'Fetch utxos', () =>
this.fetchBackendAsJson('/rest/utxos', 'GET')
, 'wallet.fetchUtxos', true)
},
fetchDeposit: (increment) => {
return this.withStatus('Wallet', 'Fetch deposit address', () =>
this.fetchBackendAsJson('/rest/wallet/deposit?increment='+increment, 'GET')
, 'wallet.fetchDeposit')
}
};
mix = {
fetchState: () => {
return this.withStatus('Mix', 'Fetch state', () =>
this.fetchBackendAsJson('/rest/mix', 'GET')
, 'mix.fetchState', true)
},
start: () => {
return this.withStatus('Mix', 'Start mixing', () =>
this.fetchBackend('/rest/mix/start', 'POST')
, 'mix.start')
},
stop: () => {
return this.withStatus('Mix', 'Stop mixing', () =>
this.fetchBackend('/rest/mix/stop', 'POST')
, 'mix.stop')
}
};
utxo = {
tx0Preview: (hash, index, feeTarget, poolId) => {
return this.withStatus('Utxo', 'Preview tx0', () =>
this.fetchBackendAsJson('/rest/utxos/'+hash+':'+index+'/tx0Preview', 'POST', {
feeTarget: feeTarget,
poolId: poolId
})
)
},
tx0: (hash, index, feeTarget, poolId, mixsTarget) => {
return this.withStatus('Utxo', 'New tx0', () =>
this.fetchBackendAsJson('/rest/utxos/'+hash+':'+index+'/tx0', 'POST', {
feeTarget: feeTarget,
poolId: poolId,
mixsTarget: mixsTarget
})
)
},
configure: (hash, index, poolId, mixsTarget) => {
return this.withStatus('Utxo', 'Configure utxo', () =>
this.fetchBackendAsJson('/rest/utxos/'+hash+':'+index, 'POST', {
poolId: poolId,
mixsTarget: mixsTarget
})
)
},
startMix: (hash, index) => {
return this.withStatus('Utxo', 'Start mixing', () =>
this.fetchBackend('/rest/utxos/'+hash+':'+index+'/startMix', 'POST')
)
},
stopMix: (hash, index) => {
return this.withStatus('Utxo', 'Stop mixing', () =>
this.fetchBackend('/rest/utxos/'+hash+':'+index+'/stopMix', 'POST')
)
},
};
gui = {
versions: () => {
return this.withStatus('GUI', 'Check for updates', () => {
const headers = {
cache: "no-store"
}
return utils.fetch(VERSIONS_URL, { method: 'GET', headers: headers, cache: "no-store" }, true)
},
'gui.versions', true
)
}
}
}
const backendService = new BackendService()
export default backendService
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import sjcl from 'sjcl';
const CRYPT_KEY_LENGTH = 256
const CRYPT_TAG_LENGTH = 128
const CRYPT_ITERATIONS = 10000
class EncryptUtils {
constructor () {
}
encrypt(key, message) {
// AES
const cipherText = sjcl.encrypt(key, message, {mode: "gcm", ks: CRYPT_KEY_LENGTH, ts: CRYPT_TAG_LENGTH, iter: CRYPT_ITERATIONS})
// minify
const parsed = JSON.parse(cipherText);
const result = {
iv: parsed.iv,
salt: parsed.salt,
ct: parsed.ct
}
return result;
}
/*decrypt(key, minimalCipherText) {
// decode base64
const parsed = JSON.parse(atob(minimalCipherText));
// decode minify
const cipherText = Object.assign(parsed, {mode:"ccm",iter:10000,ks:128,ts:64,v:1,cipher:"aes",adata:""});
const cipherTextStr = JSON.stringify(cipherText);
// AES
const plaintext = sjcl.decrypt(key, cipherTextStr)
return plaintext
}*/
}
const encryptUtils = new EncryptUtils()
export default encryptUtils
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import ifNot from 'if-not-running';
import moment from 'moment';
import backendService from './backendService';
const REFRESH_RATE = 10000;
class WalletService {
constructor () {
this.setState = undefined
this.state = undefined
this.refreshTimeout = undefined
}
init (state, setState) {
ifNot.run('walletService:init', () => {
this.setState = setState
if (this.state === undefined) {
console.log('walletState: init...')
if (state !== undefined) {
console.log('walletState: load', Object.assign({}, state))
this.state = state
}
} else {
console.log('walletState: already initialized')
}
})
}
start() {
if (this.refreshTimeout === undefined) {
this.refreshTimeout = setInterval(this.fetchState.bind(this), REFRESH_RATE)
return this.fetchState()
}
return Promise.resolve()
}
stop() {
if (this.refreshTimeout) {
clearInterval(this.refreshTimeout)
}
this.refreshTimeout = undefined
this.state = {}
}
isReady() {
return this.state && this.state.wallet
}
// wallet
computeLastActivity(utxo) {
if (!utxo.lastActivityElapsed) {
return undefined
}
const fetchElapsed = new Date().getTime()-this.state.wallet.fetchTime
return moment.duration(fetchElapsed + utxo.lastActivityElapsed).humanize()
}
getUtxosDeposit () {
return this.state.wallet.deposit.utxos;
}
getUtxosPremix () {
return this.state.wallet.premix.utxos;
}
getUtxosPostmix () {
return this.state.wallet.postmix.utxos;
}
getBalanceDeposit () {
return this.state.wallet.deposit.balance
}
getBalancePremix () {
return this.state.wallet.premix.balance
}
getBalancePostmix () {
return this.state.wallet.postmix.balance
}
getZpubDeposit() {
return this.state.wallet.deposit.zpub
}
getZpubPremix() {
return this.state.wallet.premix.zpub
}
getZpubPostmix() {
return this.state.wallet.postmix.zpub
}
fetchState () {
return ifNot.run('walletService:fetchState', () => {
// fetchState backend
return backendService.wallet.fetchUtxos().then(wallet => {
wallet.fetchTime = new Date().getTime()
// set state
if (this.state === undefined) {
console.log('walletService: initializing new state')
this.state = {
wallet: wallet
}
} else {
console.log('walletService: updating existing state', Object.assign({}, this.state))
this.state.wallet = wallet
}
this.pushState()
})
})
}
// deposit
fetchDepositAddress (increment=false) {
return backendService.wallet.fetchDeposit(increment).then(depositResponse => {
const depositAddress = depositResponse.depositAddress
return depositAddress;
})
}
fetchDepositAddressDistinct(distinctAddress, increment=false) {
return this.fetchDepositAddress(increment).then(depositAddress => {
if (depositAddress === distinctAddress) {
return this.fetchDepositAddressDistinct(distinctAddress, true).then(distinctDepositAddress => {
return distinctDepositAddress
})
}
return depositAddress;
})
}
pushState () {
this.setState(this.state)
}
}
const walletService = new WalletService()
export default walletService
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
export const ACTION_APP_STATUS_ITEM_ADD = 'app/App/ACTION_APP_STATUS_ITEM_ADD'
export const ACTION_APP_STATUS_ITEM_SUCCESS =
'app/App/ACTION_APP_STATUS_ITEM_SUCCESS'
export const ACTION_APP_STATUS_ITEM_ERROR =
'app/App/ACTION_APP_STATUS_ITEM_ERROR'
export const ACTION_APP_STATUS_ITEM_CLEAR =
'app/App/ACTION_APP_STATUS_ITEM_CLEAR'
export const ACTION_APP_STATUS_ITEM_RETRY =
'app/App/ACTION_APP_STATUS_ITEM_RETRY'
export const statusActions = {
add: (id, mainLabel, label, executor) => {
return {
type: ACTION_APP_STATUS_ITEM_ADD,
payload: {
id: id,
mainLabel: mainLabel,
label: label,
executor: executor,
success: false,
error: false,
errorMessage: undefined
}
}
},
success: id => {
return {
type: ACTION_APP_STATUS_ITEM_SUCCESS,
payload: {
id: id
}
}
},
error: (id, errorMessage) => {
return {
type: ACTION_APP_STATUS_ITEM_ERROR,
payload: {
id: id,
errorMessage: errorMessage
}
}
},
clear: id => {
return {
type: ACTION_APP_STATUS_ITEM_CLEAR,
payload: {
id: id
}
}
},
retry: id => {
return {
type: ACTION_APP_STATUS_ITEM_RETRY,
payload: {
id: id
}
}
}
}
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import ifNot from 'if-not-running';
import moment from 'moment';
import backendService from './backendService';
const REFRESH_RATE = 30000;
class PoolsService {
constructor () {
this.setState = undefined
this.state = undefined
this.refreshTimeout = undefined
}
init (state, setState) {
ifNot.run('poolsService:init', () => {
this.setState = setState
if (this.state === undefined) {
console.log('poolsState: init...')
if (state !== undefined) {
console.log('poolsState: load', Object.assign({}, state))
this.state = state
}
} else {
console.log('poolsState: already initialized')
}
})
}
start() {
if (this.refreshTimeout === undefined) {
this.refreshTimeout = setInterval(this.fetchState.bind(this), REFRESH_RATE)
return this.fetchState()
}
return Promise.resolve()
}
stop() {
if (this.refreshTimeout) {
clearInterval(this.refreshTimeout)
}
this.refreshTimeout = undefined
this.state = {}
}
isReady() {
return this.state && this.state.pools
}
// pools
getPools () {
if (!this.isReady()) {
return []
}
return this.state.pools.pools;
}
fetchPoolsForTx0(utxoBalance, tx0FeeTarget) {
return backendService.pools.fetchPools(tx0FeeTarget).then(poolsResponse => poolsResponse.pools.filter(pool => utxoBalance >= pool.tx0BalanceMin))
}
getPoolsForTx0(utxoBalance) {
return this.getPools().filter(pool => utxoBalance >= pool.tx0BalanceMin)
}
getPoolsForMix(utxoBalance, liquidity) {
if (liquidity) {
return this.getPools().filter(pool => utxoBalance == pool.denomination)
}
return this.getPools().filter(pool => utxoBalance >= pool.mustMixBalanceMin && utxoBalance <= pool.mustMixBalanceMax)
}
findPool(poolId) {
const results = this.getPools().filter(pool => poolId === pool.poolId)
return (results.length > 0 ? results[0] : undefined)
}
computePoolProgress(pool) {
if (!pool) {
return undefined
}
return pool.nbConfirmed / pool.mixAnonymitySet * 100
}
fetchState () {
return ifNot.run('poolsService:fetchState', () => {
// fetchState backend
return backendService.pools.fetchPools().then(pools => {
pools.fetchTime = new Date().getTime()
// set state
if (this.state === undefined) {
console.log('poolsService: initializing new state')
this.state = {
pools: pools
}
} else {
console.log('poolsService: updating existing state', Object.assign({}, this.state))
this.state.pools = pools
}
this.pushState()
})
})
}
pushState () {
this.setState(this.state)
}
}
const poolsService = new PoolsService()
export default poolsService
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import * as React from 'react';
import { ipcRenderer } from 'electron';
import { ProgressBar } from 'react-bootstrap';
import { CLILOCAL_STATUS, IPC_CLILOCAL, cliApiService } from '../const';
import cliService from './cliService';
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome';
import * as Icons from '@fortawesome/free-solid-svg-icons';
import moment from 'moment';
class CliLocalService {
constructor() {
this.state = undefined
ipcRenderer.on(IPC_CLILOCAL.STATE, this.onState.bind(this))
}
onState(event, cliLocalState) {
console.log('cliLocalService.onState', cliLocalState)
this.state = cliLocalState
cliService.setCliLocalState(cliLocalState)
}
fetchState() {
console.log('CliLocalService: fetchState')
ipcRenderer.send(IPC_CLILOCAL.GET_STATE)
}
reload() {
console.log('CliLocalService: reload')
ipcRenderer.send(IPC_CLILOCAL.RELOAD)
}
deleteConfig() {
console.log('CliLocalService: reset local CLI')
ipcRenderer.send(IPC_CLILOCAL.DELETE_CONFIG)
}
isValid() {
return this.state !== undefined && this.state.valid
}
getInfo() {
return this.state !== undefined && this.state.info
}
getError() {
return this.state !== undefined && this.state.error
}
getProgress() {
return this.state !== undefined && this.state.progress
}
isStatusUnknown() {
return this.state !== undefined && this.state.status === undefined
}
isStatusDownloading() {
return this.state !== undefined && this.state.status === CLILOCAL_STATUS.DOWNLOADING
}
isStarted() {
return this.state !== undefined && this.state.started
}
getStartTime() {
return this.state ? this.state.started : undefined
}
isStatusError() {
return this.state !== undefined && this.state.status === CLILOCAL_STATUS.ERROR
}
getStatusIcon(format) {
let infoError = ""
if (cliLocalService.getError()) {
infoError = cliLocalService.getError()+'. '
}
if (cliLocalService.getInfo()) {
infoError += cliLocalService.getInfo()
}
// downloading
if (cliLocalService.isStatusDownloading()) {
const progress = this.getProgress()
const status = 'Downloading CLI '+cliLocalService.getCliVersionStr()+'... '+(progress?progress+'%':'')
const progressBar = progress ? <ProgressBar animated now={progress} label={progress+'%'} title={status}/> : <span></span>
return format(progressBar, status)
}
// error
if (cliLocalService.isStatusError()) {
const status = 'CLI error: '+infoError
return format(<FontAwesomeIcon icon={Icons.faCircle} color='red' title={status}/>, status)
}
if (cliLocalService.isStarted()) {
// started
const status = 'CLI running for '+moment.duration(new Date().getTime()-cliLocalService.getStartTime()).humanize()
return format(<FontAwesomeIcon icon={Icons.faPlay} color='green' title={status} size='xs'/>, status)
}
if (cliLocalService.isStatusUnknown()) {
// unknown
const status = 'CLI is initializing. '+infoError
return format(<FontAwesomeIcon icon={Icons.faCircle} color='red' title={status} size='xs'/>, status)
}
if (!cliLocalService.isValid()) {
// invalid
const status = 'CLI executable is not valid. '+infoError
return format(<FontAwesomeIcon icon={Icons.faCircle} color='red' title={status} size='xs'/>, status)
}
// valid but stopped
const status = 'CLI is not running. '+infoError
return format(<FontAwesomeIcon icon={Icons.faStop} color='orange' title={status} />, status)
}
hasCliApi() {
return this.state && this.state.cliApi
}
getCliVersion() {
if (!this.hasCliApi()) {
return undefined
}
return this.state.cliApi.cliVersion
}
getCliVersionStr() {
let version = this.getCliVersion() ? this.getCliVersion() : '?'
if (!cliApiService.isApiModeRelease()) {
// apiMode
version += ' ['+cliApiService.getApiMode()+']'
// checksum
if (cliApiService.useChecksum()) {
const checksum = this.getCliChecksum() ? this.getCliChecksum() : '?'
version += ' (' + checksum + ')'
}
}
return version
}
getCliFilename() {
if (!this.hasCliApi()) {
return undefined
}
return this.state.cliApi.filename
}
getCliUrl() {
if (!this.hasCliApi()) {
return undefined
}
return this.state.cliApi.url
}
getCliChecksum() {
if (!this.hasCliApi()) {
return undefined
}
return this.state.cliApi.checksum
}
}
export const cliLocalService = new CliLocalService()
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
class ModalService {
constructor () {
this.setState = undefined
}
init (setState) {
this.setState = setState
}
openTx0(utxo) {
this.setState({
modalTx0: utxo
})
}
openDeposit() {
this.setState({
modalDeposit: true
})
}
openZpub(account, zpub) {
this.setState({
modalZpub: {
account,
zpub
}
})
}
close() {
this.setState({
modalTx0: false,
modalDeposit: false,
modalZpub: false
});
}
}
const modalService = new ModalService()
export default modalService
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
import React from 'react';
import utils from '../../services/utils';
/* eslint-disable react/prefer-stateless-function */
class LinkExternal extends React.PureComponent {
render () {
return (
<a href='#' onClick={e => {utils.openExternal(this.props.href);e.preventDefault()}}>{this.props.children}</a>
)
}
}
export default LinkExternal
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
/**
*
* Status
*
*/
import React from 'react';
import { Dropdown, DropdownButton } from 'react-bootstrap';
import mixService from '../../services/mixService';
import { MIXSTARGET_UNLIMITED } from '../../services/utils';
import utils from '../../services/utils';
/* eslint-disable react/prefer-stateless-function */
class UtxoMixsTargetSelector extends React.PureComponent {
render () {
const utxo = this.props.utxo
const targets = [1, 2, 3, 5, 10, 25, 50, 100, MIXSTARGET_UNLIMITED]
return (
<DropdownButton size='sm' variant="default" title={utxo.mixsDone+' / '+utils.mixsTargetLabel(utxo.mixsTargetOrDefault)} className='utxoMixsTargetSelector'>
{targets.map(value => {
value = parseInt(value)
const label = utils.mixsTargetLabel(value)
return <Dropdown.Item key={value} active={value === utxo.mixsTarget} onClick={() => mixService.setMixsTarget(utxo, value)}>{label}</Dropdown.Item>
})}
</DropdownButton>
)
}
}
export default UtxoMixsTargetSelector
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
/**
*
* Status
*
*/
import React from 'react';
import mixService from '../../services/mixService';
import { WHIRLPOOL_ACCOUNTS } from '../../services/utils';
import { Dropdown, DropdownButton } from 'react-bootstrap';
/* eslint-disable react/prefer-stateless-function */
class UtxoPoolSelector extends React.PureComponent {
computePools(utxo) {
if (utxo.account === WHIRLPOOL_ACCOUNTS.DEPOSIT) {
return mixService.getPoolsForTx0(utxo)
}
return mixService.getPoolsForMix(utxo)
}
computePoolLabel(poolId) {
return poolId ? poolId : 'no pool'
}
render () {
const utxo = this.props.utxo
const pools = this.computePools(utxo);
const activeLabel = this.computePoolLabel(utxo.poolId)
if (pools.length < 2 && (!this.props.noPool || !utxo.poolId)) {
// single choice available
return <span>{this.computePoolLabel(utxo.poolId ? utxo.poolId : undefined)}</span>
}
return (
<DropdownButton size='sm' variant="default" title={activeLabel} className='utxoPoolSelector'>
{pools.map((pool,i) => {
const poolLabel = this.computePoolLabel(pool.poolId)
return <Dropdown.Item key={i} active={utxo.poolId === pool.poolId} onClick={() => mixService.setPoolId(utxo, pool.poolId)}>{poolLabel}</Dropdown.Item>
})}
{(this.props.noPool || !utxo.poolId) && <Dropdown.Divider />}
{(this.props.noPool || !utxo.poolId) && <Dropdown.Item active={!utxo.poolId} onClick={() => mixService.setPoolId(utxo, undefined)}>{this.computePoolLabel(undefined)}</Dropdown.Item>}
</DropdownButton>
)
}
}
export default UtxoPoolSelector
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
/**
*
* Status
*
*/
import React, { useCallback, useMemo, useState } from 'react';
import _ from 'lodash';
import mixService from '../../services/mixService';
import * as Icon from 'react-feather';
import utils, { MIXABLE_STATUS, UTXO_STATUS, WHIRLPOOL_ACCOUNTS } from '../../services/utils';
import LinkExternal from '../Utils/LinkExternal';
import UtxoMixsTargetSelector from './UtxoMixsTargetSelector';
import UtxoPoolSelector from './UtxoPoolSelector';
import modalService from '../../services/modalService';
const UtxoControls = React.memo(({ utxo }) => {
return (
<div>
{utxo.account === WHIRLPOOL_ACCOUNTS.DEPOSIT && mixService.isTx0Possible(utxo) && <button className='btn btn-sm btn-primary' title='Send to Premix' onClick={() => modalService.openTx0(utxo)}>Premix <Icon.ArrowRight size={12}/></button>}
{mixService.isStartMixPossible(utxo) && utxo.mixableStatus === MIXABLE_STATUS.MIXABLE && <button className='btn btn-sm btn-primary' title='Start mixing' onClick={() => mixService.startMixUtxo(utxo)}>Mix <Icon.Play size={12} /></button>}
{mixService.isStartMixPossible(utxo) && utxo.mixableStatus !== MIXABLE_STATUS.MIXABLE && <button className='btn btn-sm btn-border' title='Add to queue' onClick={() => mixService.startMixUtxo(utxo)}><Icon.Plus size={12} />queue</button>}
{mixService.isStopMixPossible(utxo) && utxo.status === UTXO_STATUS.MIX_QUEUE && <button className='btn btn-sm btn-border' title='Remove from queue' onClick={() => mixService.stopMixUtxo(utxo)}><Icon.Minus size={12} />queue</button>}
{mixService.isStopMixPossible(utxo) && utxo.status !== UTXO_STATUS.MIX_QUEUE && <button className='btn btn-sm btn-primary' title='Stop mixing' onClick={() => mixService.stopMixUtxo(utxo)}>Stop <Icon.Square size={12} /></button>}
</div>
)
});
/* eslint-disable react/prefer-stateless-function */
const UtxosTable = ({ controls, account, utxos }) => {
const copyToClipboard = useCallback((text) => {
const el = document.createElement('textarea');
el.value = text;
el.setAttribute('readonly', '');
el.style.position = 'absolute';
el.style.left = '-9999px';
document.body.appendChild(el);
el.select();
document.execCommand('copy');
document.body.removeChild(el);
}, []);
const [sortBy, setSortBy] = useState('lastActivityElapsed');
const [ascending, setAscending] = useState(true);
const handleSetSort = useCallback((key) => {
if (sortBy === key) {
setAscending(!ascending);
} else {
setAscending(true);
}
setSortBy(key);
}, [sortBy, ascending]);
const sortedUtxos = useMemo(() => {
const sortedUtxos = _.sortBy(utxos, sortBy);
if (!ascending) {
return _.reverse(sortedUtxos);
}
return sortedUtxos;
}, [utxos, sortBy, ascending]);
const renderSort = sort => sortBy === sort && (ascending ? "▲" : "▼")
return (
<div className='table-utxos'>
<table className="table table-sm table-hover">
<thead>
<tr>
<th scope="col" className='clipboard'/>
{account && <th scope="col" className='account'>
<a onClick={() => handleSetSort('account')}>
Account {renderSort('account')}
</a>
</th>}
<th scope="col" className='hash'>
<a onClick={() => handleSetSort('hash')}>
UTXO {renderSort('hash')}
</a>
</th>
<th scope="col" className='path'>
<a onClick={() => handleSetSort('path')}>
Path {renderSort('path')}
</a>
</th>
<th scope="col" className='confirmations'>
<a onClick={() => handleSetSort('confirmations')}>
Confirms {renderSort('confirmations')}
</a>
</th>
<th scope="col" className='value'>
<a onClick={() => handleSetSort('value')}>
Amount {renderSort('value')}
</a>
</th>
<th scope="col" className='poolId'>
<a onClick={() => handleSetSort('poolId')}>
Pool {renderSort('poolId')}
</a>
</th>
<th scope="col" className='mixsDone'>
<a onClick={() => handleSetSort('mixsDone')}>
Mixs {renderSort('mixsDone')}
</a>
</th>
<th scope="col" className='utxoStatus'>
<a onClick={() => handleSetSort('status')}>
Status {renderSort('status')}
</a>
</th>
<th scope="col" colSpan={2}>
<a onClick={() => handleSetSort('lastActivityElapsed')}>
Last activity {renderSort('lastActivityElapsed')}
</a>
</th>
{controls && <th scope="col" className='utxoControls' />}
</tr>
</thead>
<tbody>
{sortedUtxos.map((utxo, i) => {
const lastActivity = mixService.computeLastActivity(utxo);
const utxoReadOnly = utils.isUtxoReadOnly(utxo);
const allowNoPool = utxo.account === WHIRLPOOL_ACCOUNTS.DEPOSIT;
return (
<tr key={i} className={utxoReadOnly ? 'utxo-disabled' : ''}>
<td>
<span title='Copy TX ID'>
<Icon.Clipboard
className='clipboard-icon'
tabIndex={0}
size={18}
onClick={() => copyToClipboard(utxo.hash)}
/>
</span>
</td>
{account && <td><small>{utxo.account}</small></td>}
<td>
<small>
<span title={utxo.hash + ':' + utxo.index}>
<LinkExternal href={utils.linkExplorer(utxo)}>
{utxo.hash.substring(0, 20)}...{utxo.hash.substring(utxo.hash.length - 5)}:{utxo.index}
</LinkExternal>
</span>
</small>
</td>
<td>
<small>{utxo.path}</small>
</td>
<td>
<small>{utxo.confirmations > 0 ? (
<span title="confirmations">{utxo.confirmations}</span>
) : (
<strong>unconfirmed</strong>
)}
</small>
</td>
<td>{utils.toBtc(utxo.value)}</td>
<td>
{!utxoReadOnly && <UtxoPoolSelector utxo={utxo} noPool={allowNoPool}/>}
</td>
<td>
{!utxoReadOnly && <UtxoMixsTargetSelector utxo={utxo}/>}
</td>
<td>
{!utxoReadOnly && <span className='text-primary'>{utils.statusLabel(utxo)}</span>}
</td>
<td className='utxoMessage'>
{!utxoReadOnly && <small>{utils.utxoMessage(utxo)}</small>}
</td>
<td>
{!utxoReadOnly && <small>{lastActivity ? lastActivity : '-'}</small>}
</td>
<td>
{!utxoReadOnly && controls && <UtxoControls utxo={utxo}/>}
</td>
</tr>
);
})}
</tbody>
</table>
</div>
);
};
export default UtxosTable
| {
"repo_name": "Samourai-Wallet/whirlpool-gui",
"stars": "56",
"repo_language": "JavaScript",
"file_name": "module_vx.x.x.js",
"mime_type": "text/plain"
} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.