text
stringlengths 184
4.48M
|
---|
# ---
# jupyter:
# jupytext:
# formats: ipynb,py:light
# text_representation:
# extension: .py
# format_name: light
# format_version: '1.5'
# jupytext_version: 1.15.0
# kernelspec:
# display_name: Python 3 (ipyflow)
# language: python
# name: ipyflow
# ---
# # CytoTable object graph analysis
#
# This notebook explores how CytoTable objects operate. The work is related to [CytoTable#75](https://github.com/cytomining/CytoTable/issues/75).
# +
import gc
import pathlib
import sys
import cytotable
import pandas as pd
gc.set_debug(gc.DEBUG_LEAK)
cytotable.convert(
source_path="../examples/data/all_cellprofiler.sqlite",
dest_path="./examples/data/test-result.parquet",
dest_datatype="parquet",
preset="cellprofiler_sqlite_pycytominer",
)
collected = gc.collect()
if gc.garbage:
print(f"Memory leak detected: {len(gc.garbage)} objects")
df = pd.DataFrame(
[
{
"id": id(obj),
"type": type(obj),
"refcount": sys.getrefcount(obj),
"repr": repr(obj),
"size": sys.getsizeof(obj),
}
for obj in gc.garbage
]
)
df.head()
# -
# create a list of files to reference
list_of_sqlite_files = [
"../examples/data/all_cellprofiler.sqlite",
]
cytotable.convert(
source_path="../examples/data/all_cellprofiler.sqlite",
dest_path="./examples/data/test-result.parquet",
dest_datatype="parquet",
preset="cellprofiler_sqlite_pycytominer",
)
# +
collected = gc.collect()
if gc.garbage:
print(f"Memory leak detected: {len(gc.garbage)} objects")
df = pd.DataFrame(
[
{
"id": id(obj),
"type": type(obj),
"refcount": sys.getrefcount(obj),
"repr": repr(obj),
"size": sys.getsizeof(obj),
}
for obj in gc.garbage
]
)
df.head()
# -
df.sort_values(by=["size", "refcount"], ascending=False).drop_duplicates(
subset="id"
).head(30)
df[
~df["repr"].str.contains("AppFuture") & ~df["repr"].str.contains("deque")
].sort_values(by=["size", "refcount"], ascending=False).drop_duplicates(
subset="id"
).head(
30
).to_csv(
"leaks.csv"
)
df.sort_values(by="refcount", ascending=False).drop_duplicates(subset="id")[
"type"
].value_counts() |
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Employee Info</title>
</head>
<body>
<table>
<tr>
<th colspan="6">Employee Info</th>
</tr>
<tr>
<th>emp_d</th>
<th>emp_name</th>
<th>age</th>
<th>sex</th>
<th>email</th>
<th>options</th>
</tr>
<tr th:each="employee : ${page.list}">
<td th:text="${employee.empId}"></td>
<td th:text="${employee.empName}"></td>
<td th:text="${employee.age}"></td>
<td th:text="${employee.sex}"></td>
<td th:text="${employee.email}"></td>
<td><a href="">delete</a> <a href="">update</a></td>
</tr>
<tr>
<td colspan="6"><span th:if="${page.hasPreviousPage}"> <a th:href="@{/emp/page/1}">首页</a> <a
th:href="@{'/emp/page/'+${page.prePage}}">上一页</a> </span> <span
th:each="num : ${page.navigatepageNums}"> <a th:if="${page.pageNum==num}"
th:href="@{'/emp/page/'+${num}}"
th:text="'['+${num}+']'" style="color: red;"></a> <a
th:if="${page.pageNum!=num}" th:href="@{'/emp/page/'+${num}}" th:text="${num} "></a> </span> <span
th:if="${page.hasNextPage}"> <a th:href="@{'/emp/page/'+${page.nextPage}}">下一页</a> <a
th:href="@{'/emp/page/'+${page.pages}}">末页</a> </span></td>
</tr>
</table>
</body>
</html> |
import React, { useState } from "react";
import {
Box,
Card,
CardActions,
CardContent,
Collapse,
Button,
Typography,
Rating,
useTheme,
useMediaQuery,
} from "@mui/material";
import Header from "components/Header";
// import { useGetCse_dsQuery } from "state/api";
import { useGetItQuery } from "state/api";
const IT = ({
_id,
BranchName,
Year,
Section,
TotalStrength,
}) => {
const theme = useTheme();
const [isExpanded, setIsExpanded] = useState(false);
return (
<Card
sx={{
backgroundImage: "none",
backgroundColor: theme.palette.background.alt,
borderRadius: "0.55rem",
}}
>
<CardContent>
<Typography
sx={{ fontSize: 14 }}
color={theme.palette.secondary[700]}
gutterBottom
>
{/* {category} */}
{BranchName}
</Typography>
<Typography variant="h5" component="div">
Total Strength: {Number(TotalStrength)}
</Typography>
<Typography sx={{ mb: "1.5rem" }} color={theme.palette.secondary[400]}>
{Section}
</Typography>
<Rating value={Year} readOnly />
{/* <Typography variant="body2">{description}</Typography> */}
</CardContent>
<CardActions>
<Button
variant="primary"
size="small"
onClick={() => setIsExpanded(!isExpanded)}
>
See More
</Button>
</CardActions>
<Collapse
in={isExpanded}
timeout="auto"
unmountOnExit
sx={{
color: theme.palette.neutral[300],
}}
>
<CardContent>
<Typography>id: {_id}</Typography>
<Typography>Year number: {Year}</Typography>
<Typography>
{/* Yearly Sales This Year: {stat.yearlyClassesTotal} */}
</Typography>
<Typography>
{/* Yearly Units Sold This Year: {stat.yearlyTotalConducted} */}
</Typography>
</CardContent>
</Collapse>
</Card>
);
};
const It = () => {
const { data, isLoading } = useGetItQuery();
console.log("data", data)
const isNonMobile = useMediaQuery("(min-width: 1000px)");
return (
<Box m="1.5rem 2.5rem">
<Header title="IT" subtitle="Information Technology Branch" />
{data || !isLoading ? (
<Box
mt="20px"
display="grid"
gridTemplateColumns="repeat(4, minmax(0, 1fr))"
justifyContent="space-between"
rowGap="20px"
columnGap="1.33%"
sx={{
"& > div": { gridColumn: isNonMobile ? undefined : "span 4" },
}}
>
{data.map(
({
_id,
BranchName,
Year,
Section,
TotalStrength,
}) => (
<IT
// key={_id}
_id={_id}
BranchName={BranchName}
Year={Year}
Section={Section}
TotalStrength={TotalStrength}
/>
)
)}
</Box>
) : (
<>Loading...</>
)}
</Box>
);
};
export default It; |
"use client";
import supabase from "@/database/supabaseClient";
import React, { useEffect, useState } from "react";
import { Database } from "../../../types/supabase";
import Image from "next/image";
import Line from "../(components)/Line";
type Fixture = Database["public"]["Tables"]["fix"]["Row"];
interface Images {
[teamName: string]: string; // Maps a team name (string) to an image source (string)
}
const images: Images = {
Houdu: "/images/img1.png",
Ngapu: "/images/img2.png",
Khouchi: "/images/img3.png",
Kapamodzü: "/images/img4.png",
Referee: "/images/img5.png",
Admin: "/images/img6.gif",
};
function FixtureRow({ id, sort, team_A, team_B }: Fixture) {
// Check if team_name is not null, and if it's a valid key in images
const teamImageSrcA = team_A && images[team_A] ? images[team_A] : "";
const teamImageSrcB = team_B && images[team_B] ? images[team_B] : "";
const altTextA = team_A || "Default Alt Text";
const altTextB = team_B || "Default Alt Text";
return (
<tr>
<td className="px-1 md:px-4 py-4 text-sm text-gray-300 whitespace-nowrap text-center">
{team_A}
</td>
<td className="px-1 md:px-4 py-4 text-sm text-gray-300 whitespace-nowrap">
<Image
src={teamImageSrcA}
alt={altTextA}
width={60}
height={60}
className="rounded-full bg-white p-1"
></Image>
</td>
<td className="px-1 md:px-4 py-4 text-sm text-gray-300 whitespace-nowrap text-center">VS</td>
<td className="px-1 md:px-4 py-4 text-sm text-gray-300 whitespace-nowrap text-center">
<Image
src={teamImageSrcB}
alt={altTextB}
width={60}
height={60}
className="rounded-full bg-white p-1"
></Image>
</td>
<td className="px-1 md:px-4 py-4 text-sm text-gray-300 whitespace-nowrap text-center">
{team_B}
</td>
</tr>
);
}
function FixtureTable({ fix }: { fix: Fixture[] }) {
return (
<div className="overflow-x-auto bg-gray-800 shadow sm:rounded-lg">
<table className="min-w-full divide-y divide-gray-700">
<thead>
<tr>
<th className="px-4 py-3 bg-gray-700 text-center text-xs text-gray-300 uppercase tracking-wider">
Team 1
</th>
<th className="px-4 py-3 bg-gray-700 text-center text-xs text-gray-300 uppercase tracking-wider">
Image
</th>
<th className="px-4 py-3 bg-gray-700 text-center text-xs text-gray-300 uppercase tracking-wider">
-
</th>
<th className="px-4 py-3 bg-gray-700 text-center text-xs text-gray-300 uppercase tracking-wider">
Image
</th>
<th className="px-4 py-3 bg-gray-700 text-center text-xs text-gray-300 uppercase tracking-wider">
Team 2
</th>
</tr>
</thead>
<tbody className="divide-y divide-gray-700">
{fix.map((fix) => (
<FixtureRow key={fix.id} {...fix} />
))}
</tbody>
</table>
</div>
);
}
export default function Page() {
const [foot, setFoot] = useState<Fixture[] | null>(null);
const [mvolley, setMvolley] = useState<Fixture[] | null>(null);
const [fvolley, setFvolley] = useState<Fixture[] | null>(null);
const [mbasket, setMbasket] = useState<Fixture[] | null>(null);
const [fbasket, setFbasket] = useState<Fixture[] | null>(null);
const [fetchError, setFetchError] = useState<string | null>(null);
useEffect(() => {
const fetchFoot = async () => {
const { data, error } = await supabase
.from("fix")
.select()
.eq("sport", "⚽ Football");
if (error) {
setFetchError("could not fetch the data");
setFoot(null);
console.log(error);
}
if (data) {
setFoot(data);
setFetchError(null);
}
};
fetchFoot();
}, []);
useEffect(() => {
const fetchMvolley = async () => {
const { data, error } = await supabase
.from("fix")
.select()
.eq("sport", "🏐 Volleyball")
.eq("gender", "Men");
if (error) {
setFetchError("could not fetch the data");
setMvolley(null);
console.log(error);
}
if (data) {
setMvolley(data);
setFetchError(null);
}
};
fetchMvolley();
}, []);
useEffect(() => {
const fetchFvolley = async () => {
const { data, error } = await supabase
.from("fix")
.select()
.eq("sport", "🏐 Volleyball")
.eq("gender", "Women");
if (error) {
setFetchError("could not fetch the data");
setFvolley(null);
console.log(error);
}
if (data) {
setFvolley(data);
setFetchError(null);
}
};
fetchFvolley();
}, []);
useEffect(() => {
const fetchMbasket = async () => {
const { data, error } = await supabase
.from("fix")
.select()
.eq("sport", "🏀 Basketball")
.eq("gender", "Men");
if (error) {
setFetchError("could not fetch the data");
setMbasket(null);
console.log(error);
}
if (data) {
setMbasket(data);
setFetchError(null);
}
};
fetchMbasket();
}, []);
useEffect(() => {
const fetchFbasket = async () => {
const { data, error } = await supabase
.from("fix")
.select()
.eq("sport", "🏀 Basketball")
.eq("gender", "Women");
if (error) {
setFetchError("could not fetch the data");
setFbasket(null);
console.log(error);
}
if (data) {
setFbasket(data);
setFetchError(null);
}
};
fetchFbasket();
}, []);
return (
<div className="flex flex-col gap-16">
<div className="text-center w-full pt-16 text-3xl font-semibold">
<div>Fixture</div>
<div className="text-sm font-normal mt-3">Get to know who is against who</div>
</div>
<div className="w-4/5 mx-auto"><Line /></div>
<div>
{foot && mvolley && fvolley && mbasket && fbasket && (
<div className="grid grid-cols-1 lg:grid-cols-2 xl:grid-cols-3 gap-20 mb-20 px-10">
<div className="flex flex-col justify-center items-center gap-6">
<div className="bg-white rounded-full text-black w-fit py-2 px-6">Men's Football</div>
<div><FixtureTable fix={foot}/></div>
</div>
<div className="flex flex-col justify-center items-center gap-6">
<div className="bg-white rounded-full text-black w-fit py-2 px-6">Men's Volleyball</div>
<div><FixtureTable fix={mvolley}/></div>
</div>
<div className="flex flex-col justify-center items-center gap-6">
<div className="bg-white rounded-full text-black w-fit py-2 px-6">Women's Volleyball</div>
<div><FixtureTable fix={fvolley}/></div>
</div>
<div className="flex flex-col justify-center items-center gap-6">
<div className="bg-white rounded-full text-black w-fit py-2 px-6">Men's Basketball</div>
<div><FixtureTable fix={mbasket}/></div>
</div>
<div className="flex flex-col justify-center items-center gap-6">
<div className="bg-white rounded-full text-black w-fit py-2 px-6">Women's Basketball</div>
<div><FixtureTable fix={fbasket}/></div>
</div>
</div>
)}
</div>
</div>
);
} |
# File name: app.R
# Path: './app.R'
# Author: N. Chan
# Purpose: Runs a Shiny R app to interactively view a histogram from input data
source(paste0(getwd(), "/scripts/01_loaddata.R"))
# Define UI
ui <- fluidPage(
titlePanel("Histogram-Density Plot Viewer"),
sidebarLayout(
sidebarPanel(
h5("Prepared by Nathan Chan"),
h6("for the Shopify Summer 2022 Data Science Intern Challenge"),
br(),
helpText("Select your file and specify the settings below."),
fileInput(inputId = "xlsxfile", "Select Excel File", accept = ".xlsx", multiple = FALSE),
selectInput("selectedcolumn", "Choose Column (must be numeric)", choices = c("")),
br(),
textOutput("displaymax"),
tags$head(tags$style("#displaymax{color: blue; font-style: bold;}")),
textOutput("displaymin"),
tags$head(tags$style("#displaymin{color: blue; font-style: bold;}")),
textOutput("displayrechist"),
tags$head(tags$style("#displayrechist{color: blue; font-style: bold;}")),
br(),
helpText("Specify plot limits."),
numericInput("selectedmax", "X-axis Maximum", value = 1),
numericInput("selectedmin", "X-axis Minimum", value = 0),
br(),
helpText("Specify a histogram bin size between the minimum and maximum of the column specified."),
numericInput("selectedbinsize", "Histogram Bin Size", value = 1),
helpText("Specify a density plot scale factor between the minimum and maximum of the column specified. It is recommended to start with the same value as the recommended histogram bin size above and adjust from there."),
numericInput("selecteddensize", "Density Plot Scale Factor", value = 1)
# sliderInput("selectedbinsize", "Specify Histogram Bin Size", min = 0, max = 10, value = 5, step = 1),
# sliderInput("selecteddensize", "Specify Density Plot Scale Factor", min = 0, max = 10, value = 5, step = 1),
# sliderInput("selectedmax", "Specify X-axis Maximum", min = 0, max = 10, value = 5, step = 1),
# sliderInput("selectedmin", "Specify X-axis Minimum", min = 0, max = 10, value = 5, step = 1)
),
mainPanel(
tabsetPanel(
tabPanel(
title = "Data",
dataTableOutput(outputId = "displaydataTable")
),
tabPanel(
title = "Metrics",
dataTableOutput(outputId = "displaymetrics")
),
tabPanel(
title = "Plot",
plotlyOutput(outputId = "displayplot",
width = "100%",
height = "100%")
)
))
)
)
server <- function(input, output, session) {
xlsxdata <- reactive({
req(input$xlsxfile)
read_excel(input$xlsxfile$datapath)
})
output$displaymetrics <- renderDataTable({
req(input$xlsxfile)
req(input$selectedcolumn)
xlsxdata <- xlsxdata()
summ_stats(xlsxdata[[input$selectedcolumn]])
})
output$displaydataTable <- renderDataTable({xlsxdata()})
output$displaymax <- renderText({
req(input$xlsxfile)
req(input$selectedcolumn)
xlsxdata <- xlsxdata()
selectedcolumn <- as.character(input$selectedcolumn)
maxval <- max(xlsxdata[, selectedcolumn])
paste0("Maximum: ", maxval)
})
output$displaymin <- renderText({
req(input$xlsxfile)
req(input$selectedcolumn)
xlsxdata <- xlsxdata()
selectedcolumn <- as.character(input$selectedcolumn)
minval <- min(xlsxdata[, selectedcolumn])
paste0("Minimum: ", minval)
})
output$displayrechist <- renderText({
req(input$xlsxfile)
req(input$selectedcolumn)
xlsxdata_max <- input$selectedmax
xlsxdata_min <- input$selectedmin
xlsxdata_range <- xlsxdata_max - xlsxdata_min
paste0("Recommended Histogram Bin Size: ", xlsxdata_range * 0.01)
})
output$displayplot <- renderPlotly({
req(input$xlsxfile)
req(input$selectedcolumn)
req(input$selectedbinsize)
xlsxdata <- xlsxdata()
selectedcolumn <- input$selectedcolumn
summarystats <- summ_stats(xlsxdata[[selectedcolumn]])
binwidth <- input$selectedbinsize
binwidth2 <- input$selecteddensize
xmin <- input$selectedmin
xmax <- input$selectedmax
p <-
ggplot(data = xlsxdata, aes_string(x = selectedcolumn)) +
geom_histogram(
aes(color = "Histogram"),
fill = "springgreen4",
size = 0,
binwidth = binwidth) +
geom_density(
# Density plots are usually constrained within [0,1]. However, ggplot
# requires that the y-axis of plots have the same scale. This is a
# workaround to let our density plot display properly.
aes(y = ..density.. * nrow(xlsxdata) * binwidth2, color = "Density Plot"),
fill = "springgreen4",
size = 0,
alpha = 0.3
) +
geom_vline(
aes(xintercept = summarystats[which(summarystats == "Arithmetic Mean"), 2], color = "Arithmetic Mean"),
linetype = "longdash",
size = 0.25,
) +
geom_vline(
aes(xintercept = summarystats[which(summarystats == "Median"), 2], color = "Median"),
linetype = "dotdash",
size = 0.25,
) +
geom_vline(
aes(xintercept = summarystats[which(summarystats == "Mode"), 2], color = "Mode"),
linetype = "dotted",
size = 0.25,
) +
geom_vline(
aes(xintercept = summarystats[which(summarystats == "Geometric Mean"), 2], color = "Geometric Mean"),
linetype = "twodash",
size = 0.25,
) +
geom_vline(
aes(xintercept = summarystats[which(summarystats == "Harmonic Mean"), 2], color = "Harmonic Mean"),
linetype = "dashed",
size = 0.25,
) +
labs(
y = "Count"
) +
scale_x_continuous(
labels = function(x)
format(x, scientific = FALSE),
guide = guide_legend(),
limits = c(xmin, xmax)
) +
scale_color_manual(
name = "",
values = c(
"Histogram" = "springgreen4",
"Density Plot" = "springgreen4",
"Arithmetic Mean" = "red",
"Median" = "blue",
"Mode" = "orange",
"Geometric Mean" = "green",
"Harmonic Mean" = "grey"
)
) +
theme_classic() +
theme(plot.title = element_text(hjust = 0.5),
axis.text.x = element_text(angle = 45, hjust = 1))
ggplotly(p) %>%
layout(
legend = list(
orientation = "h", x = 0.5, y = -0.25, xanchor = "center"))
})
observe({
req(input$xlsxfile)
xlsxdata <- xlsxdata()
updateSelectInput(
session = session,
inputId = "selectedcolumn",
choices = colnames(xlsxdata[, sapply(xlsxdata, is.numeric)])
)
})
observe({
req(input$xlsxfile)
req(input$selectedcolumn)
xlsxdata <- xlsxdata()
selectedcolumn <- as.character(input$selectedcolumn)
xlsxdata_max <- range(xlsxdata[, selectedcolumn])[2]
xlsxdata_min <- range(xlsxdata[, selectedcolumn])[1]
xlsxdata_range <- xlsxdata_max - xlsxdata_min
xlsxdata_rec <- (input$selectedmax - input$selectedmin) * 0.01
updateNumericInput(
session = session,
inputId = "selectedbinsize",
max = xlsxdata_range * 0.1,
min = xlsxdata_range * 0.001,
value = xlsxdata_rec,
step = xlsxdata_range * 0.001
)
updateNumericInput(
session = session,
inputId = "selecteddensize",
max = xlsxdata_range * 0.1,
min = xlsxdata_range * 0.0001,
value = xlsxdata_rec,
step = xlsxdata_range * 0.0001
)
# updateSliderInput(
# session = session,
# inputId = "selectedbinsize",
# max = xlsxdata_range * 0.1,
# min = xlsxdata_range * 0.001,
# value = xlsxdata_range * 0.01,
# step = xlsxdata_range * 0.001
# )
# updateSliderInput(
# session = session,
# inputId = "selecteddensize",
# max = xlsxdata_range * 0.1,
# min = xlsxdata_range * 0.0001,
# value = xlsxdata_range * 0.01,
# step = xlsxdata_range * 0.0001
# )
})
observe({
req(input$xlsxfile)
req(input$selectedcolumn)
xlsxdata <- xlsxdata()
selectedcolumn <- as.character(input$selectedcolumn)
xlsxdata_max <- range(xlsxdata[, selectedcolumn])[2]
xlsxdata_min <- range(xlsxdata[, selectedcolumn])[1]
xlsxdata_range <- xlsxdata_max - xlsxdata_min
updateNumericInput(
session = session,
inputId = "selectedmax",
max = xlsxdata_max,
min = xlsxdata_min,
value = xlsxdata_max,
step = xlsxdata_range * 0.001
)
updateNumericInput(
session = session,
inputId = "selectedmin",
max = xlsxdata_max,
min = xlsxdata_min,
value = xlsxdata_min,
step = xlsxdata_range * 0.001
)
# updateSliderInput(
# session = session,
# inputId = "selectedmax",
# max = xlsxdata_max,
# min = xlsxdata_min,
# value = xlsxdata_max,
# step = xlsxdata_range * 0.001
# )
# updateSliderInput(
# session = session,
# inputId = "selectedmin",
# max = xlsxdata_max,
# min = xlsxdata_min,
# value = xlsxdata_min,
# step = xlsxdata_range * 0.001
# )
})
}
shinyApp(ui, server) |
import { useState, useEffect } from "react";
import { useNavigate, useParams } from "react-router-dom";
import { FiSearch, FiUpload } from "react-icons/fi";
import { Container, Form } from "./styles";
import { Header } from "../../components/Header";
import { BackButton } from "../../components/BackButton";
import { FileInput } from "../../components/FileInput";
import { Input } from "../../components/Input";
import { Select } from "../../components/Select";
import { IngredientItem } from "../../components/IngredientItem";
import { Textarea } from "../../components/Textarea";
import { Button } from "../../components/Button";
import { Footer } from "../../components/Footer";
import { api } from "../../services/api";
export function Edit() {
const [data, setData] = useState(null);
const [updatedImage, setUpdatedImage] = useState(null);
const [name, setName] = useState("");
const [category, setCategory] = useState("meal");
const [price, setPrice] = useState(0);
const [description, setDescription] = useState("");
const [ingredients, setIngredients] = useState([]);
const [newIngredient, setNewIngredient] = useState("");
const params = useParams();
const navigate = useNavigate();
function handleChangeMealImage(event) {
const file = event.target.files[0];
setUpdatedImage(file);
}
function handleAddIngredient() {
setIngredients((prevState) => [...prevState, newIngredient]);
setNewIngredient("");
}
function handleRemoveIngredient(deleted) {
setIngredients((prevState) =>
prevState.filter((ingredient) => ingredient !== deleted)
);
}
async function handleUpdateMeal() {
try {
const formData = new FormData();
formData.append("image", updatedImage);
formData.append("name", name);
formData.append("category", category);
formData.append("ingredients", JSON.stringify(ingredients));
formData.append("price", price);
formData.append("description", description);
await api.put(`/meals/${params.id}`, formData);
alert("Prato atualizado com sucesso!");
navigate(-1);
} catch (error) {
if (error.response) {
return alert(error.response.data.message);
} else {
return alert(
"Não foi possível atualizar o prato. Tente novamente mais tarde."
);
}
}
}
async function handleDeleteMeal() {
try {
await api.delete(`/meals/${params.id}`);
alert("Prato excluído com sucesso!");
navigate("/");
} catch (error) {
if (error.response) {
return alert(error.response.data.message);
} else {
return alert(
"Não foi possível excluir o prato. Tente novamente mais tarde."
);
}
}
}
useEffect(() => {
async function fetchMeal() {
const response = await api.get(`/meals/${params.id}`);
setData(response.data);
const { name, category, ingredients, price, description } = response.data;
setName(name);
setCategory(category);
setIngredients(ingredients.map((ingredient) => ingredient.name));
setPrice(price);
setDescription(description);
}
fetchMeal();
}, []);
return (
<Container>
<Header>
<Input
icon={FiSearch}
type="search"
placeholder="Busque por pratos ou ingredientes"
/>
</Header>
<main>
<BackButton title="voltar" to="/" />
<h1>Editar prato</h1>
<Form>
<div className="image-wrapper">
<p>Imagem do prato</p>
<FileInput
id="image"
icon={FiUpload}
label="Imagem do prato"
onChange={handleChangeMealImage}
/>
</div>
<Input
id="name"
type="text"
label="Nome"
placeholder="Ex.: Salada Ceasar"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<Select
id="category"
label="Categoria"
value={category}
onChange={(e) => setCategory(e.target.value)}
>
<option value="meal">Refeição</option>
<option value="dessert">Sobremesa</option>
<option value="beverage">Bebida</option>
</Select>
<div className="ingredients-wrapper">
<label>Ingredientes</label>
<div className="ingredients">
{ingredients.map((ingredient, index) => (
<IngredientItem
key={String(index)}
value={ingredient}
onClick={() => handleRemoveIngredient(ingredient)}
/>
))}
<IngredientItem
isNew
id="ingredients"
label="Ingredientes"
placeholder="Adicionar"
onChange={(e) => setNewIngredient(e.target.value)}
value={newIngredient}
onClick={handleAddIngredient}
/>
</div>
</div>
<Input
id="price"
type="number"
label="Preço"
placeholder="Ex.: R$ 00,00"
value={price}
onChange={(e) => setPrice(e.target.value)}
/>
<div className="description-wrapper">
<Textarea
id="description"
label="Descrição"
placeholder="Fale brevemente sobre o prato, seus ingredientes e composição"
defaultValue={description}
onChange={(e) => setDescription(e.target.value)}
/>
</div>
<div className="button-wrapper">
<Button
className="delete-button"
title="Excluir prato"
onClick={handleDeleteMeal}
/>
<Button title="Salvar alterações" onClick={handleUpdateMeal} />
</div>
</Form>
</main>
<Footer />
</Container>
);
} |
<template>
<div class="main-cont grey lighten-5">
<div class="title-img-cont" data-aos="zoom-in">
<img :src="GeneralHelpers.requireImage('title.png')" />
</div>
<v-card class="card" data-aos="zoom-in" data-aos-delay="300">
<v-card-text class="card-body">
<v-scroll-x-reverse-transition>
<div v-show="cardView === VIEW_MAIN">
<div class="mb-3">
Keep track of your
<strong>Nuzlockes</strong>
and
<strong>Soulinks</strong>
!
</div>
<div v-if="currentUser">
<v-btn @click="setCardView(VIEW_CREATE_SHEET)">Create Sheet</v-btn>
</div>
<div v-if="currentUser">
<v-btn @click="setCardView(VIEW_JOIN_SHEET)">Join Sheet</v-btn>
</div>
<div v-if="currentUser">
<v-btn
@click="showUserPreferenceDialog = true"
:disabled="!hasSavedSheets"
>
View my sheets
</v-btn>
</div>
<div v-if="!currentUser">
<v-btn
@click="onSignInOrOffBtn(false, authProviders.google)"
:disabled="isSigningIn"
:loading="isSigningIn"
>
<i class="mdi mdi-google"></i>
Sign In
</v-btn>
</div>
<div v-if="currentUser">
<div class="profile-pic">
Signed in as:
<br />
<img :src="currentUser.photoURL" crossorigin="" />
<div>
<strong>{{ currentUser.email }}</strong>
<br />
<span
class="link"
v-if="currentUser"
@click="onSignInOrOffBtn(false, authProviders.google)"
>
(Sign Out)
</span>
</div>
</div>
</div>
</div>
</v-scroll-x-reverse-transition>
<v-scroll-x-reverse-transition>
<div v-show="cardView === VIEW_CREATE_SHEET">
<v-form v-model="createSheetForm.isValid" ref="createSheetForm">
<v-text-field
class="v-input-small mb-5"
v-model="createSheetForm.name"
outlined
label="Your name"
:rules="createSheetForm.nameRules"
prepend-icon="mdi-account"
hide-details
required
></v-text-field>
<v-text-field
class="v-input-small mb-5"
v-model="createSheetForm.title"
outlined
label="Sheet title"
:rules="createSheetForm.titleRules"
prepend-icon="mdi-pencil-box-outline"
hide-details
required
></v-text-field>
<v-select
class="v-input-small mb-5"
v-model="createSheetForm.pokemonGen"
:items="pokemonGames"
:menu-props="{ top: false, offsetY: true }"
prepend-icon="mdi-pokeball"
label="Pokémon Game"
:rules="createSheetForm.pokemonGenRules"
outlined
hide-details
required
></v-select>
<v-select
class="v-input-small mb-5"
v-model="createSheetForm.isPrivate"
:items="isPrivateOptions"
item-text="text"
item-value="value"
:menu-props="{ top: false, offsetY: true }"
prepend-icon="mdi-lock"
label="Visibility"
outlined
hide-details
required
></v-select>
<div class="d-flex justify-space-between mt-8">
<v-btn @click="setCardView(VIEW_MAIN)">Back</v-btn>
<v-btn
:disabled="!createSheetForm.isValid"
:loading="creatingSheet"
@click="createSheet"
color="primary"
>
Create
</v-btn>
</div>
</v-form>
</div>
</v-scroll-x-reverse-transition>
<v-scroll-x-reverse-transition>
<div v-show="cardView === VIEW_JOIN_SHEET">
<v-form v-model="joinSheetForm.isValid" ref="joinSheetForm">
<v-text-field
class="v-input-small"
v-model="joinSheetForm.code"
outlined
label="Sheet Code"
:rules="joinSheetForm.codeRules"
required
:error="joinSheetForm.error"
></v-text-field>
<div class="d-flex justify-space-between">
<v-btn
:disabled="!joinSheetForm.isValid"
:loading="joiningSheet"
@click="joinSheet"
>
Join Sheet
</v-btn>
<v-btn @click="setCardView(VIEW_MAIN)">Back</v-btn>
</div>
</v-form>
</div>
</v-scroll-x-reverse-transition>
</v-card-text>
</v-card>
<div class="my-4 home-footer">
<strong>Made by</strong>
<v-btn
class="ml-2"
icon
href="https://www.linkedin.com/in/cvillalobosgtz/"
target="_blank"
x-small
>
<v-icon>mdi-linkedin</v-icon>
</v-btn>
-
<v-btn icon href="https://github.com/charliemike3124" target="_blank" x-small>
<v-icon>mdi-github</v-icon>
</v-btn>
</div>
<v-dialog v-model="showUserPreferenceDialog">
<UserSheets @closeDialog="showUserPreferenceDialog = false"></UserSheets>
</v-dialog>
<!-- Snackbar -->
<v-snackbar v-model="snackbar.show">
{{ snackbar.text }}
<template v-slot:action="{ attrs }">
<v-btn
:color="snackbar.color"
text
v-bind="attrs"
icon
@click="snackbar.show = false"
>
<v-icon>mdi-close</v-icon>
</v-btn>
</template>
</v-snackbar>
</div>
</template>
<script>
import FirebaseAuth from "@/services/FirebaseAuth";
import { mapState, mapActions } from "vuex";
import { User } from "../resources/models";
import { Constants, PokemonGens } from "../resources/constants";
import UserSheets from "../components/userSheets.vue";
export default {
name: "Home",
computed: {
...mapState("sheets", ["savedSheets", "currentUser", "userPreference"]),
hasSavedSheets() {
return this.userPreference?.savedSheets?.length || false;
},
},
components: {
UserSheets,
},
data() {
return {
showUserPreferenceDialog: false,
isSigningIn: false,
cardView: "main",
VIEW_MAIN: "main",
VIEW_CREATE_SHEET: "createSheet",
VIEW_JOIN_SHEET: "joinSheet",
pokemonGames: PokemonGens.names,
isPrivateOptions: [
{ text: "Private", value: true },
{ text: "Public", value: false },
],
createSheetForm: {
isValid: false,
title: "",
titleRules: [(v) => !!v || "Enter a title!"],
name: "",
nameRules: [(v) => !!v || "Enter a name!"],
pokemonGen: null,
pokemonGenRules: [(v) => !!v || "Select a game!"],
isPrivate: true,
},
joinSheetForm: {
isValid: false,
code: "",
codeRules: [(v) => !!v || "Enter a Code!"],
error: false,
JOIN_TRY_DELAY: 3000,
},
joiningSheet: false,
creatingSheet: false,
newSheetTitle: "",
snackbar: {
show: false,
text: "",
color: "",
},
authProviders: {
google: Constants.AUTH_PROVIDERS.GOOGLE,
facebook: Constants.AUTH_PROVIDERS.FACEBOOK,
twitter: Constants.AUTH_PROVIDERS.TWITTER,
email: Constants.AUTH_PROVIDERS.EMAIL,
},
};
},
methods: {
...mapActions("sheets", ["LoadUserPreferences", "SetCurrentUser"]),
...mapActions("nuzlocke", ["InitializeSheetDataList", "JoinSheet"]),
async createSheet() {
this.creatingSheet = true;
const documentId = await this.InitializeSheetDataList([
this.createSheetForm.title,
[
User(
this.currentUser?.uid,
this.createSheetForm.name,
this.currentUser?.email,
this.currentUser?.photoURL
),
],
this.createSheetForm.pokemonGen,
this.createSheetForm.isPrivate
]);
this.$router.push({
name: "Sheet",
params: { code: documentId },
});
},
async joinSheet() {
this.joiningSheet = true;
const sheetExists = await this.JoinSheet(this.joinSheetForm.code);
if (sheetExists === Constants.JOIN_SHEET_ERRORS.DOES_NOT_EXIST) {
this.showSnackbar("There are no sheets with that code!");
} else if (sheetExists === Constants.JOIN_SHEET_ERRORS.NO_ACCESS) {
this.showSnackbar("You have no access to that sheet.");
} else if (!!sheetExists) {
this.$router.push({
name: "Sheet",
params: { code: this.joinSheetForm.code },
});
}
this.joiningSheet = false;
},
async onSignInOrOffBtn(fromJoinSheetBtn = false, authProvider) {
this.isSigningIn = true;
if (!this.currentUser) {
//Handle Sign In
const user = await FirebaseAuth.SignInWithPopupAsync(authProvider);
if (user) {
this.SetCurrentUser(user);
if (!!fromJoinSheetBtn) {
this.setCardView(this.VIEW_JOIN_SHEET);
}
}
} else {
//Handle Sign Out
await FirebaseAuth.SignOutAsync();
this.SetCurrentUser(null);
}
this.isSigningIn = false;
},
setCardView(view) {
if (view === this.VIEW_JOIN_SHEET && !this.currentUser) {
this.showSnackbar("Sign in first before joining a sheet!");
} else {
this.cardView = "";
setTimeout(() => {
this.cardView = view;
}, 300);
}
},
showSnackbar(text) {
this.snackbar.show = true;
this.snackbar.text = text;
this.joinSheetForm.error = true;
setTimeout(() => {
this.joinSheetForm.error = false;
}, this.joinSheetForm.JOIN_TRY_DELAY);
},
},
async mounted() {
await FirebaseAuth.CheckForSignedInUser(this.SetCurrentUser);
await this.LoadUserPreferences();
},
};
</script>
<style lang="less">
@import (less) "../styles/views/home.less";
</style> |
import pandas as pd
import os
from orangePlatform import logger
import joblib
from numpy import array
from keras.models import Sequential
from keras.layers import LSTM, Dense, Flatten, TimeDistributed
from keras.layers import ConvLSTM2D
import numpy as np
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.callbacks import EarlyStopping
import yaml
from orangePlatform.entity.config_entity import Model_TrainerConfig
def create_lstm_model_3_layers(num_lstm_units, learning_rate, nsteps):
n_seq = 5
n_steps = int(nsteps // n_seq)
model = Sequential()
model.add(ConvLSTM2D(filters=num_lstm_units, kernel_size=(1, 2), activation='relu', padding='same', input_shape=(n_seq, 1, n_steps, 1), return_sequences=True))
model.add(ConvLSTM2D(filters=num_lstm_units//2, kernel_size=(1, 2), activation='relu', padding='same', return_sequences=True))
model.add(ConvLSTM2D(filters=num_lstm_units//4, kernel_size=(1, 2), activation='relu', padding='same'))
model.add(Flatten())
model.add(Dense(1))
optimizer = Adam(learning_rate=learning_rate)
model.compile(optimizer=optimizer, loss='mse')
return model
def split_sequence(sequence, n_steps):
X, y = list(), list()
for i in range(len(sequence)):
# find the end of this pattern
end_ix = i + n_steps
# check if we are beyond the sequence
if end_ix > len(sequence)-1:
break
# gather input and output parts of the pattern
seq_x, seq_y = sequence[i:end_ix], sequence[end_ix]
X.append(seq_x)
y.append(seq_y)
return np.array(X), np.array(y)
class BestModelTrainer:
def __init__(self, config: Model_TrainerConfig):
self.config = config
def train(self):
train_data = pd.read_csv(self.config.train_data_path)
test_data = pd.read_csv(self.config.test_data_path)
train=train_data.values
test=test_data.values
hyperparams = {
'num_lstm_units': [256],
'learning_rate': [0.001],
'epochs': [100],
'early_stopping_patience': [10],
'nsteps': [10]
}
best_param={'num_lstm_units': 256, 'learning_rate': 0.001, 'epochs': 180, 'early_stopping_patience': 10, 'nsteps': 15}
loss= 1e+20
# Hyperparameter search loop
for num_lstm_units in hyperparams['num_lstm_units']:
for learning_rate in hyperparams['learning_rate']:
for epochs in hyperparams['epochs']:
for early_stopping_patience in hyperparams['early_stopping_patience']:
for nsteps in hyperparams['nsteps']:
# Create and train the LSTM model
model = create_lstm_model_3_layers(num_lstm_units, learning_rate, nsteps)
X, y = split_sequence(train, nsteps) # You need to define split_sequence
n_features = 1
n_seq =5
n_steps = int(nsteps // n_seq)
X = X.reshape((X.shape[0], n_seq, 1, n_steps, 1))
X_val, y_val = split_sequence(test, nsteps)
X_val = X_val.reshape((X_val.shape[0], n_seq, 1, n_steps, 1))
# Define early stopping callback
early_stopping = EarlyStopping(monitor='val_loss', patience=early_stopping_patience, restore_best_weights=True)
# Train the model and log metrics using MLflow
history = model.fit(X, y, epochs=epochs, validation_data=(X_val, y_val), callbacks=[early_stopping])
if history.history['val_loss'][-1] < loss:
loss = history.history['val_loss'][-1]
best_param={'num_lstm_units': num_lstm_units, 'learning_rate': learning_rate, 'epochs': epochs, 'early_stopping_patience': early_stopping_patience, 'nsteps': nsteps}
# Load the existing params.yaml file
with open('params.yaml', 'r') as file:
params = yaml.safe_load(file)
# Update the parameters in the params dictionary
params['ConvLSTM2D'].update(best_param)
# Write the updated parameters back to the params.yaml file
with open('params.yaml', 'w') as file:
yaml.dump(params, file, default_flow_style=False) |
<script setup>
import { ref, onMounted } from 'vue';
import { useRoute } from 'vue-router';
import { useStore } from 'vuex';
import UserCard from '@/components/UserCard';
const store = useStore();
const route = useRoute();
const username = ref('');
const userFollowers = ref([]);
const isDataReady = ref(false);
const getUserFollowers = async () => {
let response;
try {
response = await store.state.axiosInstance.get(`${store.state.apiBaseURL}/v1/users/${username.value}/followers`, {
headers: {
'Authorization': `Bearer ${store.state.accessToken.token}`
}
});
} catch(_) { }
const { data } = response.data;
userFollowers.value = data.reverse();
};
onMounted(async () => {
username.value = route.params.username;
await getUserFollowers();
isDataReady.value = true;
});
</script>
<template>
<div>
<div class="px-3" v-if="isDataReady">
<div v-if="!userFollowers[0]" class="flex justify-center pt-10">
<div class="text-center">
<i class="bi bi-wind text-6xl text-neutral-500"></i>
<p class="mt-2 text-neutral-500 font-semibold">This user doesn't have a follower yet</p>
</div>
</div>
<div v-else>
<UserCard v-for="user, i in userFollowers" :key="i" :user="user"/>
</div>
</div>
</div>
</template> |
public class RecordTest {
interface I { }
record Middle(String field) { }
record A(Middle afield) implements I { }
record B(Middle bfield) implements I { }
public static String sink(String s) { return s; }
public static void test(boolean inp) {
I i = inp ? new A(new Middle("A")) : new B(new Middle("B"));
switch(i) {
case A(Middle(String field)):
sink(field);
break;
case B(Middle(String field)):
sink(field);
break;
default:
break;
}
switch(i) {
case A(Middle(String field)) -> sink(field);
case B(Middle(String field)) -> sink(field);
default -> { }
}
var x = switch(i) {
case A(Middle(String field)):
yield sink(field);
case B(Middle(String field)):
yield sink(field);
default:
yield "Default case";
};
var y = switch(i) {
case A(Middle(String field)) -> sink(field);
case B(Middle(String field)) -> sink(field);
default -> "Default case";
};
}
} |
<?php
/*
* Copyright 2012 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* Credentials object used for OAuth 2.0 Signed JWT assertion grants.
*
* @author Chirag Shah <[email protected]>
*/
class Google_AssertionCredentials {
const MAX_TOKEN_LIFETIME_SECS = 3600;
public $serviceAccountName;
public $scopes;
public $privateKey;
public $privateKeyPassword;
public $assertionType;
public $prn;
/**
*
* @param
* $serviceAccountName
* @param $scopes array
* List of scopes
* @param
* $privateKey
* @param string $privateKeyPassword
* @param string $assertionType
* @param bool|string $prn
* The email address of the user for which the
* application is requesting delegated access.
*/
public function __construct($serviceAccountName, $scopes, $privateKey, $privateKeyPassword = 'notasecret', $assertionType = 'http://oauth.net/grant_type/jwt/1.0/bearer', $prn = false) {
$this->serviceAccountName = $serviceAccountName;
$this->scopes = is_string ( $scopes ) ? $scopes : implode ( ' ', $scopes );
$this->privateKey = $privateKey;
$this->privateKeyPassword = $privateKeyPassword;
$this->assertionType = $assertionType;
$this->prn = $prn;
}
public function generateAssertion() {
$now = time ();
$jwtParams = array (
'aud' => Google_OAuth2::OAUTH2_TOKEN_URI,
'scope' => $this->scopes,
'iat' => $now,
'exp' => $now + self::MAX_TOKEN_LIFETIME_SECS,
'iss' => $this->serviceAccountName
);
if ($this->prn !== false) {
$jwtParams ['prn'] = $this->prn;
}
return $this->makeSignedJwt ( $jwtParams );
}
/**
* Creates a signed JWT.
*
* @param array $payload
* @return string The signed JWT.
*/
private function makeSignedJwt($payload) {
$header = array (
'typ' => 'JWT',
'alg' => 'RS256'
);
$segments = array (
Google_Utils::urlSafeB64Encode ( json_encode ( $header ) ),
Google_Utils::urlSafeB64Encode ( json_encode ( $payload ) )
);
$signingInput = implode ( '.', $segments );
$signer = new Google_P12Signer ( $this->privateKey, $this->privateKeyPassword );
$signature = $signer->sign ( $signingInput );
$segments [] = Google_Utils::urlSafeB64Encode ( $signature );
return implode ( ".", $segments );
}
} |
index.html
<!DOCTYPE html>
<html lang="es">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<!-- Google fonts -->
<link
href="https://fonts.googleapis.com/css2?family=Montserrat&display=swap"
rel="stylesheet"
/>
<!-- CSS Bootstrap -->
<link
href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity="sha384-KK94CHFLLe+nY2dmCWGMq91rCGa5gtU4mk92HdvYe+M/SXH301p5ILy+dN9+nJOZ"
crossorigin="anonymous"
/>
<!-- Mi CSS -->
<link rel="stylesheet" href="css/style.css" />
<title>After</title>
</head>
<body>
<header>
<nav class="navbar navbar-expand-lg navbar--blue">
<div class="container">
<a class="navbar-brand" href="#">Navbar</a>
<button
class="navbar-toggler"
type="button"
data-bs-toggle="collapse"
data-bs-target="#navbarSupportedContent"
aria-controls="navbarSupportedContent"
aria-expanded="false"
aria-label="Toggle navigation"
>
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul class="navbar-nav me-auto mb-2 mb-lg-0">
<li class="nav-item">
<a class="nav-link active" aria-current="page" href="#"
>Inicio</a
>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Servicios</a>
</li>
<li class="nav-item">
<a class="nav-link">Contacto</a>
</li>
</ul>
<form class="d-flex" role="search">
<input
class="form-control me-2"
type="search"
placeholder="Search"
aria-label="Search"
/>
<button class="btn btn-outline-success" type="submit">
Search
</button>
</form>
</div>
</div>
</nav>
</header>
<main class="bg-light container">
<h1 class="text-center py-5 display-6">Lorem ipsum dolor sit.</h1>
<p class="lead text-center">
Lorem ipsum, dolor sit amet consectetur adipisicing elit. Voluptates,
numquam cupiditate aspernatur mollitia et nostrum?
</p>
<div class="row pt-5">
<div class="col-12 col-md-4">
<h2>6000</h2>
<p class="text-muted">Lorem ipsum dolor sit amet.</p>
</div>
<div class="col-md-4">
<h2>8.4</h2>
<p class="text-muted">Lorem ipsum dolor sit amet.</p>
</div>
<div class="col-md-4">
<h2>75%</h2>
<p class="text-muted">Lorem ipsum dolor sit amet.</p>
</div>
</div>
<div class="pb-5">
<div
class="progress"
role="progressbar"
aria-label="Basic example"
aria-valuenow="25"
aria-valuemin="0"
aria-valuemax="100"
>
<div class="progress-bar" style="width: 25%"></div>
</div>
</div>
</main>
<footer class="text-center my-5">
<p>
Lorem, ipsum dolor sit amet consectetur adipisicing elit. Ratione soluta
cum dolore repellat, nemo quae.
</p>
</footer>
<script
src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"
integrity="sha384-ENjdO4Dr2bkBIFxQpeoTz1HIcje39Wm4jDKdf19U8gI4ddQ3GYNS7NTKfAdVQSZe"
crossorigin="anonymous"
></script>
</body>
</html> |
import { IProduct } from './IProduct';
export class Product implements IProduct {
//#region Fields
id: number;
name: string;
description: string;
imageURL: string;
price: number;
quantity: number;
//#endregion
//#region Constructor
/**
* Product Constructor
*/
constructor(
_id: number,
_name: string,
_description: string,
_imageURL: string,
_price: number,
_quantity: number
) {
this.id = _id;
this.name = _name;
this.description = _description;
this.imageURL = _imageURL;
this.price = _price;
this.quantity = _quantity;
}
//#endregion
}
export let productList: Product[] = [
{
id: 1,
name: 'Mac',
description: 'Macintosh',
imageURL:
'https://www.hardsoftcomputers.co.uk/wp-content/uploads/2023/03/mac.png',
price: 1000,
quantity: 2,
},
{
id: 2,
name: 'Samsung',
description: 'Samsung Phone',
imageURL:
'https://img.global.news.samsung.com/in/wp-content/uploads/2022/03/SM-A536_Galaxy-A53-5G_Awesome-Peach_Front.jpg',
price: 500,
quantity: 3,
},
{
id: 3,
name: 'IPhone',
description: 'Apple Mobile',
imageURL:
'https://m.media-amazon.com/images/I/71yzJoE7WlL._AC_UF1000,1000_QL80_.jpg',
price: 500,
quantity: 0,
},
{
id: 4,
name: 'TV',
description: 'Television',
imageURL:
'https://cdn.webshopapp.com/shops/256009/files/374440369/xiaomi-xiaomi-mi-tv-p1-32-inch.jpg',
price: 15000,
quantity: 1,
},
{
id: 5,
name: 'PS5',
description: 'Playstation Five',
imageURL:
'https://jawalplus.com/wp-content/uploads/2020/11/sony-ps5-digital-edition.jpg',
price: 2500,
quantity: 6,
},
{
id: 6,
name: 'Camera',
description: 'Canon Camera',
imageURL:
'https://petapixel.com/assets/uploads/2022/09/canon-5d-mark-iv-dslr.jpg',
price: 6000,
quantity: 4,
},
{
id: 7,
name: 'Mac',
description: 'Macintosh',
imageURL:
'https://www.hardsoftcomputers.co.uk/wp-content/uploads/2023/03/mac.png',
price: 8080,
quantity: 3,
},
]; |
import { Fragment, useState } from 'react';
import { FormattedMessage } from 'react-intl';
import {
Autocomplete,
Button,
FormControl,
FormControlLabel,
FormHelperText,
Grid,
InputLabel,
Menu,
MenuItem,
Radio,
RadioGroup,
Select,
Stack,
TextField
} from '@mui/material';
import { useCustomerFormStore } from '../customer-form-store';
import { DeleteFilled, MoreOutlined, PlusCircleFilled, PlusOutlined } from '@ant-design/icons';
import { DesktopDatePicker, LocalizationProvider } from '@mui/x-date-pickers';
import { AdapterDayjs } from '@mui/x-date-pickers/AdapterDayjs';
import { getPetCategoryList } from 'pages/customer/service';
import MainCard from 'components/MainCard';
import IconButton from 'components/@extended/IconButton';
import FormPetCategory from '../components/FormPetCategory';
const TabPetInformation = () => {
const customerPets = useCustomerFormStore((state) => state.customerPets);
const petCategoryList = useCustomerFormStore((state) => state.petCategoryList);
const onDeletePets = (i) => {
useCustomerFormStore.setState((prevState) => {
let newData = [...prevState.customerPets];
newData[i].command = 'del';
return { customerPets: newData, customerFormTouch: true };
});
};
const onAddPets = () => {
useCustomerFormStore.setState((prevState) => {
return {
customerPets: [
...prevState.customerPets,
{
id: '',
petName: '',
petCategoryId: null,
races: '',
condition: '',
petGender: '',
isSteril: '',
birthDateType: '', // dateBirth || monthAndYear
dateOfBirth: null,
petMonth: '',
petYear: '',
color: '',
command: '',
error: {
petNameErr: '',
petCategoryErr: '',
conditionErr: '',
petGenderErr: '',
isSterilErr: ''
}
}
],
customerFormTouch: true
};
});
};
const onCheckValidation = () => {};
const onFieldHandler = (event, i) => {
if (event.target.name === 'petYear' && +event.target.value > 20) return;
useCustomerFormStore.setState((prevState) => {
let newData = [...prevState.customerPets];
newData[i][event.target.name] = event.target.value;
return { customerPets: newData, customerFormTouch: true };
});
onCheckValidation();
};
const onDropdownHandler = (selected, procedure, i) => {
useCustomerFormStore.setState((prevState) => {
let newData = [...prevState.customerPets];
newData[i][procedure] = selected ? selected : null;
return { customerPets: newData, customerFormTouch: true };
});
onCheckValidation();
};
const [openMenu, setOpenMenu] = useState(); // el: null
const [openFormPetCategory, setOpenFormPetCategory] = useState(false);
const onCloseFormPetCategory = async (val) => {
if (val) {
const getData = await getPetCategoryList();
useCustomerFormStore.setState({ petCategoryList: getData });
}
setOpenFormPetCategory(false);
};
return (
<>
<MainCard
title={<FormattedMessage id="pet-information" />}
secondary={
<Stack direction="row" justifyContent="flex-end">
<IconButton
variant="light"
color="secondary"
id="basic-button"
aria-controls={openMenu ? 'basic-menu' : undefined}
aria-haspopup="true"
aria-expanded={openMenu ? 'true' : undefined}
onClick={(e) => setOpenMenu(e?.currentTarget)}
>
<MoreOutlined />
</IconButton>
<Menu
id="basic-menu"
anchorEl={openMenu}
open={Boolean(openMenu)}
onClose={() => setOpenMenu()}
MenuListProps={{
'aria-labelledby': 'basic-button'
}}
anchorOrigin={{
vertical: 'bottom',
horizontal: 'right'
}}
transformOrigin={{
vertical: 'top',
horizontal: 'right'
}}
>
<MenuItem onClick={() => setOpenFormPetCategory(true)}>
<PlusCircleFilled style={{ color: '#1890ff' }} /> <FormattedMessage id="pet-category" />
</MenuItem>
</Menu>
</Stack>
}
>
<Grid container spacing={3}>
{customerPets.map(
(dt, i) =>
dt.command !== 'del' && (
<Fragment key={i}>
<Grid item xs={12} sm={11}>
<Grid container spacing={3}>
<Grid item xs={12} sm={4}>
<Stack spacing={1}>
<InputLabel htmlFor="name">{<FormattedMessage id="name" />}</InputLabel>
<TextField
fullWidth
id="petName"
name="petName"
value={dt.petName}
onChange={(event) => onFieldHandler(event, i)}
inputProps={{ maxLength: 100 }}
error={Boolean(dt.error.petNameErr && dt.error.petNameErr.length > 0)}
helperText={dt.error.petNameErr}
/>
</Stack>
</Grid>
<Grid item xs={12} sm={4}>
<Stack spacing={1}>
<InputLabel>
<FormattedMessage id="pet-category" />
</InputLabel>
<Autocomplete
id="pet-category"
options={petCategoryList}
value={dt.petCategoryId}
isOptionEqualToValue={(option, val) => val === '' || option.value === val.value}
onChange={(_, value) => onDropdownHandler(value, 'petCategoryId', i)}
renderInput={(params) => (
<TextField
{...params}
error={Boolean(dt.error.petCategoryErr && dt.error.petCategoryErr.length > 0)}
helperText={dt.error.petCategoryErr}
variant="outlined"
/>
)}
/>
</Stack>
</Grid>
<Grid item xs={12} sm={4}>
<Stack spacing={1}>
<InputLabel htmlFor="race">{<FormattedMessage id="race" />}</InputLabel>
<TextField
fullWidth
id="races"
name="races"
value={dt.races}
onChange={(event) => onFieldHandler(event, i)}
inputProps={{ maxLength: 100 }}
/>
</Stack>
</Grid>
<Grid item xs={12} sm={4}>
<Stack spacing={1}>
<InputLabel htmlFor="condition">{<FormattedMessage id="condition" />}</InputLabel>
<TextField
fullWidth
id="condition"
name="condition"
value={dt.condition}
onChange={(event) => onFieldHandler(event, i)}
inputProps={{ maxLength: 100 }}
error={Boolean(dt.error.conditionErr && dt.error.conditionErr.length > 0)}
helperText={dt.error.conditionErr}
/>
</Stack>
</Grid>
<Grid item xs={12} sm={4}>
<Stack spacing={1}>
<InputLabel htmlFor="gender">
<FormattedMessage id="gender" />
</InputLabel>
<FormControl sx={{ m: 1, minWidth: 120 }}>
<Select
id={`petGender${i}`}
name="petGender"
value={dt.petGender}
onChange={(event) => onFieldHandler(event, i)}
>
<MenuItem value="">
<em>
<FormattedMessage id="select-gender" />
</em>
</MenuItem>
<MenuItem value={'J'}>Jantan</MenuItem>
<MenuItem value={'B'}>Betina</MenuItem>
</Select>
{dt.error.petGenderErr.length > 0 && <FormHelperText error> {dt.error.petGenderErr} </FormHelperText>}
</FormControl>
</Stack>
</Grid>
<Grid item xs={12} sm={4}>
<Stack spacing={1}>
<InputLabel htmlFor="sterile">
<FormattedMessage id="sterile" />
</InputLabel>
<FormControl sx={{ m: 1, minWidth: 120 }}>
<Select id={`isSteril${i}`} name="isSteril" value={dt.isSteril} onChange={(event) => onFieldHandler(event, i)}>
<MenuItem value="">
<em>
<FormattedMessage id="select" />
</em>
</MenuItem>
<MenuItem value={'1'}>
<FormattedMessage id="yes" />
</MenuItem>
<MenuItem value={'0'}>
<FormattedMessage id="no" />
</MenuItem>
</Select>
{dt.error.isSterilErr.length > 0 && <FormHelperText error> {dt.error.isSterilErr} </FormHelperText>}
</FormControl>
</Stack>
</Grid>
<Grid item xs={12} sm={4}>
<Stack spacing={1}>
<Stack spacing={1} flexDirection="row" alignItems="center">
<InputLabel htmlFor="birth-date">
<FormattedMessage id="birth-date" />
</InputLabel>
<RadioGroup
name="birthDateType"
value={dt.birthDateType} // birthDate || monthAndYear
style={{ flexDirection: 'row', height: '20px', marginLeft: '16px', marginTop: '0px' }}
onChange={(e) => {
useCustomerFormStore.setState((prevState) => {
let newData = [...prevState.customerPets];
newData[i].birthDateType = e.target.value;
newData[i].dateOfBirth = null;
newData[i].petMonth = '';
newData[i].petYear = '';
return { customerPets: newData, customerFormTouch: true };
});
}}
>
<FormControlLabel
value="birthDate"
control={<Radio />}
label={<FormattedMessage id="birth-date" />}
style={{ height: '20px' }}
/>
<FormControlLabel
value="monthAndYear"
control={<Radio />}
label={<FormattedMessage id="month-and-year" />}
style={{ height: '20px' }}
/>
</RadioGroup>
</Stack>
{dt.birthDateType === 'birthDate' && (
<Fragment>
<LocalizationProvider dateAdapter={AdapterDayjs}>
<DesktopDatePicker
value={dt.dateOfBirth}
onChange={(selected) => onDropdownHandler(selected, 'dateOfBirth', i)}
renderInput={(params) => <TextField {...params} />}
/>
</LocalizationProvider>
</Fragment>
)}
{dt.birthDateType === 'monthAndYear' && (
<Fragment>
<Stack spacing={1} flexDirection={'row'} gap={'10px'}>
<TextField
fullWidth
label={<FormattedMessage id="year" />}
id={`petYear${i}`}
name="petYear"
value={dt.petYear}
onChange={(event) => onFieldHandler(event, i)}
inputProps={{ min: 0, max: 20 }}
/>
<FormControl sx={{ m: 1, minWidth: '120px' }} style={{ marginTop: 'unset' }}>
<Select
id={`petMonth${i}`}
name="petMonth"
value={dt.petMonth}
onChange={(event) => onFieldHandler(event, i)}
>
<MenuItem value="">
<em>
<FormattedMessage id="select-month" />
</em>
</MenuItem>
{[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12].map((dt, idx) => (
<MenuItem value={dt} key={idx}>
{dt}
</MenuItem>
))}
</Select>
</FormControl>
</Stack>
</Fragment>
)}
</Stack>
</Grid>
<Grid item xs={12} sm={4}>
<Stack spacing={1}>
<InputLabel htmlFor="color">{<FormattedMessage id="color" />}</InputLabel>
<TextField fullWidth id="color" name="color" value={dt.color} onChange={(event) => onFieldHandler(event, i)} />
</Stack>
</Grid>
</Grid>
</Grid>
{customerPets.length > 1 && (
<Grid item xs={12} sm={1}>
<IconButton size="large" color="error" onClick={() => onDeletePets(i)}>
<DeleteFilled />
</IconButton>
</Grid>
)}
</Fragment>
)
)}
</Grid>
<Button variant="contained" onClick={onAddPets} startIcon={<PlusOutlined />} style={{ marginTop: '20px' }}>
Add
</Button>
</MainCard>
<FormPetCategory open={openFormPetCategory} onClose={onCloseFormPetCategory} />
</>
);
};
export default TabPetInformation; |
package com.myproject.query;
import com.alibaba.fastjson.JSON;
import com.myproject.core.consts.ConstMsg;
import com.myproject.core.consts.ConstUtils;
import com.myproject.core.consts.ICodes;
import com.myproject.core.utils.LocaleMessageSourceService;
import java.io.Serializable;
/**
* 通用AJAX请求后的结果数据
*/
public class Ret implements Serializable{
private static final long serialVersionUID = 8777098949345414562L;
public static final String quote = "\"";
/**
* 业务处理是否成功的状态
*/
private boolean success = true;
/**
* 全局消息码
*/
private int code = ICodes.SUCCESS;
/**
* 消息(根据消息常量的配置或国际化语言配置自动获取)
*/
private String message = "";
/**
* 附加消息 : 额外返回的消息
*/
private String info = "";
/**
* 返回的数据
*/
private Object data = null;
public static Ret me() {
return new Ret();
}
public Ret() {
this.code = ICodes.SUCCESS;
}
public Ret(int code) {
this.code = code;
}
public Ret(boolean isSuccess, int code) {
this.success = isSuccess;
this.code = code;
}
public Ret(boolean success, int code, Object data) {
this.data = data;
this.success = success;
this.code = code;
}
public Ret setSuccess(boolean isSuccess) {
this.success = isSuccess;
if (!this.success)
this.code = ICodes.FAILED;
return this;
}
public boolean isSuccess() {
return this.success;
}
public Ret setCode(int code) {
this.code = code;
if(ICodes.FAILED==code){
this.success = false;
}else if (ICodes.SUCCESS == code) {
this.success = true;
}
return this;
}
public int getCode() {
return this.code;
}
/**
* 会根据消息码查找全局消息常量,并返回对应的消息内容(如果有国际化要求,会去找国际化配置中的消息)
* @return
*/
public String getMessage() {
ConstMsg msgConst = ConstUtils.me().getMsgConst(code);
String _msg = null;
try{
_msg = LocaleMessageSourceService.getMessage(msgConst.getKeyCode(),null,msgConst.getName());
}catch (Exception e) {
e.printStackTrace();
}
if (_msg == null)
_msg = "";
message = _msg;
return message;
}
public String getInfo() {
return this.info;
}
public Ret setInfo(String info) {
this.info = info;
return this;
}
public Ret setData(Object data) {
this.data = data;
return this;
}
public Object getData() {
return this.data;
}
public String toString() {
StringBuffer sb = new StringBuffer();
sb.append("{");
sb.append(quote + "code" + quote + ":");
sb.append(code);
sb.append("," + quote + "success" + quote + ":");
sb.append(success);
sb.append("," + quote + "message" + quote + ":");
sb.append(quote + encode(this.getMessage()) + quote);
sb.append("," + quote + "info" + quote + ":");
sb.append(quote + encode(info) + quote);
if (null != this.data) {
sb.append("," + quote + "data" + quote + ":");
if (data instanceof String) {
sb.append(data);
} else {
sb.append(JSON.toJSONString(data));
}
}
sb.append("}");
return sb.toString();
}
private String encode(String text) {
if (null == text)
return "";
String _text = text.replace("\"", "“");
return _text;
}
} |
import axios from "axios";
import Comment from "../../../DB/models/comment.model.js";
import Reply from "../../../DB/models/reply.model.js";
/**
* * destructure data from body and authUser and query
* * check if the reply for (comment or reply)
* * check if comment is already exists
* * check if reply is already exists
* * create reply
* * response successfully
*/
export const addReply = async (req, res, next) => {
// * destructure data from body and authUser and query
const { _id } = req.authUser;
const { content, onModel } = req.body;
const { replyOnId } = req.query;
// * check if the reply for (comment or reply)
if (onModel === "Comment") {
// * check if comment is already exists
const comment = await Comment.findById(replyOnId);
if (!comment) return next("Comment not found", { cause: 404 });
} else if (onModel === "Reply") {
// * check if reply is already exists
const checkReply = await Reply.findById(replyOnId);
if (!checkReply) return next("Reply not found", { cause: 404 });
}
// * create reply
const reply = await Reply.create({
content,
addedBy: _id,
onModel,
replyOnId,
});
// * response successfully
res.status(201).json({
success: true,
message: "reply created successfully",
data: reply,
});
};
/**
* * destructure data from query and authUser
* * delete comment
* * delete all replies for this reply
* * response successfully
*/
export const deleteReply = async (req, res, next) => {
// * destructure data from query and authUser
const { _id } = req.authUser;
const { replyId } = req.query;
// * delete comment
const reply = await Reply.findOneAndDelete({
_id: replyId,
addedBy: _id,
});
if (!reply) {
return next("Comment not deleted", { cause: 400 });
}
// * delete all replies for this reply
await Reply.deleteMany({ replyOnId: replyId });
// * response successfully
res.status(200).json({ success: true, message: "reply deleted" });
};
/**
* * destructure data from query and authUser and body
* * check if reply is already existing
* * update reply
* * save reply
* * response successfully
*/
export const updateReply = async (req, res, next) => {
// * destructure data from query and authUser and body
const { content } = req.body;
const { replyId } = req.query;
const { _id } = req.authUser;
// * check if reply is already existing
const reply = await Reply.findOne({ _id: replyId, addedBy: _id });
if (!reply) {
return next("reply not found", { cause: 404 });
}
// * update reply
reply.content = content;
// * save reply
await reply.save();
// * response successfully
res.status(200).json({
success: true,
message: "comment updated successfully",
data: reply,
});
};
/**
* * destructure data from params and body and headers
* * call like API
*/
export const likeReply = async (req, res, next) => {
// * destructure data from query and body and params
const { replyId } = req.params;
const { onModel } = req.body;
const { accesstoken } = req.headers;
// * call like API
axios({
method: "post",
url: `http://localhost:3000/like/addLike/${replyId}`,
data: {
onModel,
},
headers: {
accesstoken,
},
})
.then((response) => {
res.status(200).json({ response: response.data });
})
.catch((err) => {
res.status(500).json({ catch: err.data });
});
}; |
package resources
import (
"context"
"fmt"
"log"
redis "github.com/go-redis/redis/v8"
"github.com/tmzt/config-api/models"
"github.com/tmzt/config-api/util"
"gorm.io/gorm"
)
type PlatformPermissionsResource struct {
logger *log.Logger
rdb *redis.Client
db *gorm.DB
}
func NewPlatformPermissionsResource(rdb *redis.Client, db *gorm.DB) *PlatformPermissionsResource {
logger := log.New(log.Writer(), "RootUserPermissionsResource: ", log.LstdFlags|log.Lshortfile)
return &PlatformPermissionsResource{logger, rdb, db}
}
func (r *PlatformPermissionsResource) GetRootUserPermissions(userId util.UserId) (*models.PlatformPermissionsDetail, error) {
ormRootPermissions := &models.PlatformPermissionsORM{}
// Check for cached version
cacheObj := &models.PlatformPermissionsCache{}
cacheKey := fmt.Sprintf("platform_user_permissions:%s", userId)
if err := util.GetCache(context.Background(), r.rdb, cacheKey, *cacheObj); err == nil {
log.Println("Returning cached platform user permissions detail")
return cacheObj.Detail, nil
}
res := r.db.
Where("user_id = ?", string(userId)).
Find(ormRootPermissions)
if res.Error != nil {
log.Println("Returning empty platform user permissions detail")
return &models.PlatformPermissionsDetail{}, nil
}
detail := ormRootPermissions.Detail()
// Cache
r.rdb.Set(context.Background(), "platform_user_permissions:"+string(userId), detail, 0)
return detail, nil
}
func (r *PlatformPermissionsResource) UpsertRootUserPermissions(userId util.UserId, updatePermissions *models.PlatformPermissionsDetail) error {
err := r.db.Transaction(func(tx *gorm.DB) error {
ormRootUserPermissions := models.PlatformPermissionsORM{}
accountId := util.ROOT_ACCOUNT_ID
res := r.db.
Where("account_id = ? AND user_id = ?", accountId, string(userId)).
// Only used if record does not exist
Attrs(&models.PlatformPermissionsORM{
Id: util.NewUUID(),
}).
// Always updated
Assign(&models.PlatformPermissionsORM{
AccountId: string(accountId),
UserId: string(userId),
RootUser: updatePermissions.PlatformUser,
InternalUser: updatePermissions.InternalUser,
}).
FirstOrCreate(&ormRootUserPermissions)
if res.Error != nil {
return res.Error
}
// Cache the result
util.SetCache(r.rdb, ormRootUserPermissions.Cache())
return nil
})
if err != nil {
log.Println("Error updating platform user permissions: ", err)
return err
}
return nil
}
func (r *PlatformPermissionsResource) IsPlatformAdmin(userId util.UserId) bool {
detail, err := r.GetRootUserPermissions(userId)
if err != nil {
log.Println("Error getting platform user permissions: ", err)
return false
}
return detail.PlatformAdmin
}
func (r *PlatformPermissionsResource) IsPlatformUser(userId util.UserId) bool {
detail, err := r.GetRootUserPermissions(userId)
if err != nil {
log.Println("Error getting platform user permissions: ", err)
return false
}
return detail.PlatformUser
}
func (r *PlatformPermissionsResource) IsInternalUser(userId util.UserId) bool {
detail, err := r.GetRootUserPermissions(userId)
if err != nil {
log.Println("Error getting platform user permissions: ", err)
return false
}
return detail.InternalUser
} |
----
## 1. 节点类型
不同角色的节点
- `Master eligible / Data / Ingest / Coordinating / Machine Learning`
在开发环境中,一个节点可承担多种角色
在生产环境中
- 根据数据量,写入和查询的吞吐量,选择适合的部署方式
- 建议设置单一角色的节点(dedicated node)
## 2. 节点参数配置
一个节点在默认情况下会同时扮演: `master eligible` ,`data node` 和 `ingest node`
| 节点类型 | 配置参数 | 默认值 |
|-------------------|-------------|-------------------------|
| master eligible | node.master | true |
| data | node.data | true |
| ingest | node.ingest | true |
| coodrinating only | 无 | 设置上面三个参数全部为 false |
| machine learning | node.ml | true (需要 enable x-pack) |
## 3. 单一职责的节点
一个节点只承担一个角色
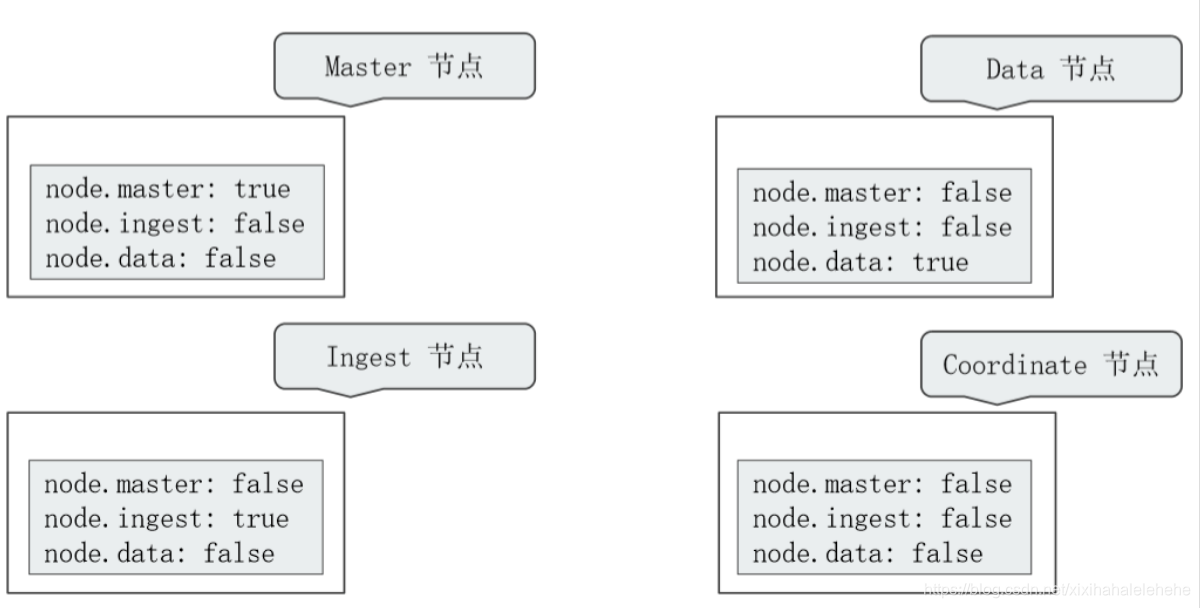
## 4. 单一角色:职责分离的好处
Dedicated master eligible nodes:负责集群状态(cluster state)的管理
- 使用低配置的 CPU ,RAM 和磁盘
Dedicated data nodes: 负责数据存储及处理客户端请求
- 使用高配置的 CPU,RAM 和磁盘
Dedicated ingest nodes: 负责数据处理
- 使用高配置的 CPU ; 中等配置的 RAM; 低配置的磁盘
## 5. Dedicate Coordinating Only Node (Client Node)
配置:将 Master ,Data ,Ingest 都配置成 Flase
- Medium / High CUP; Medium / High RAM;Low Disk
生产环境中,建议为一些大的集群配置 Coordinating Only Nodes
- 扮演 Load Balancers。 降低 Master 和 Data Nodes 的负载
- 负载搜索结果的 Gather / Reduce
- 有时候无法预知客户端会发生怎样的请求
大量占用内存的结合操作,一个深度聚合可能引发 OOM
## 6. Dedicate Master Node
从高可用 & 避免脑裂的角色出发
- 一般在生产环境中配置 3 台
- 一个集群只有 1 台活跃的主节点
- 负载分片管理,索引创建,集群管理等操作
如果和数据节点或者 Coordinate 节点混合部署
- 数据节点相对有比较大的内存占用
- Coordinate 节点有时候可能会有开销很高的查询,导致 OOM
- 这些都有可能影响 Master 节点,导致集群的不稳定
## 7. 基本部署:增减节点,水平扩展
当磁盘容量无法满足需求时,可以增加数据节点;磁盘读写压力大时,增加数据节点
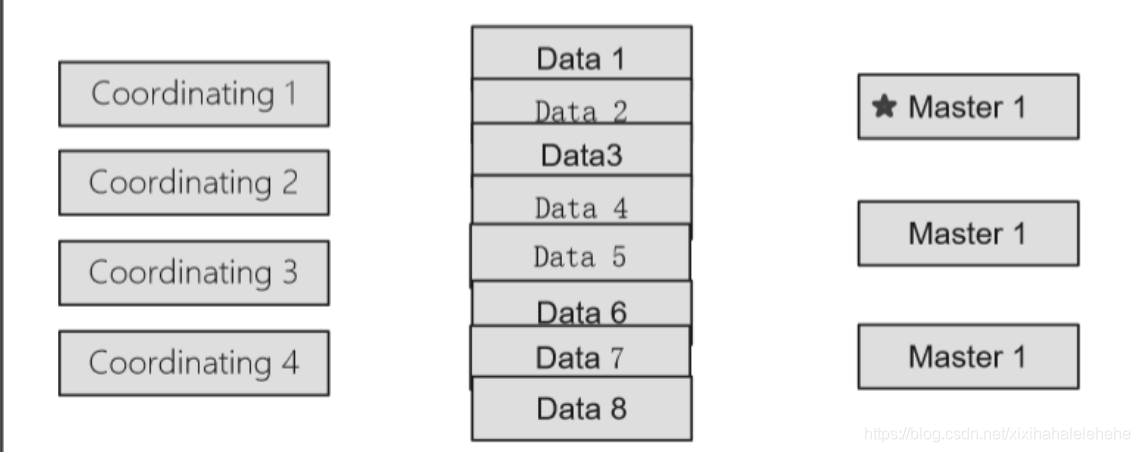
## 8. 水平扩展:Coordinating Only Node
当系统中有大量的复杂查询及聚合时候,增加 Coordinating 节点,增加查询的性能
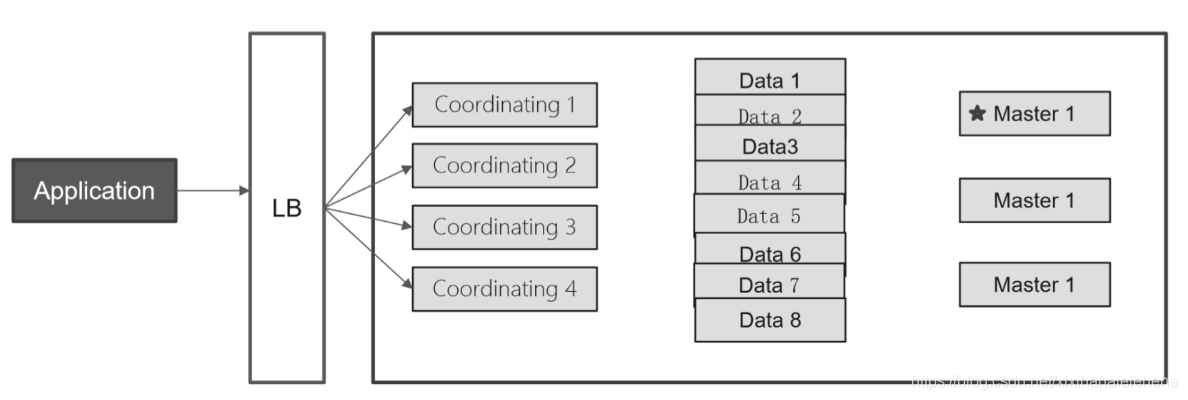
## 9. 读写分离
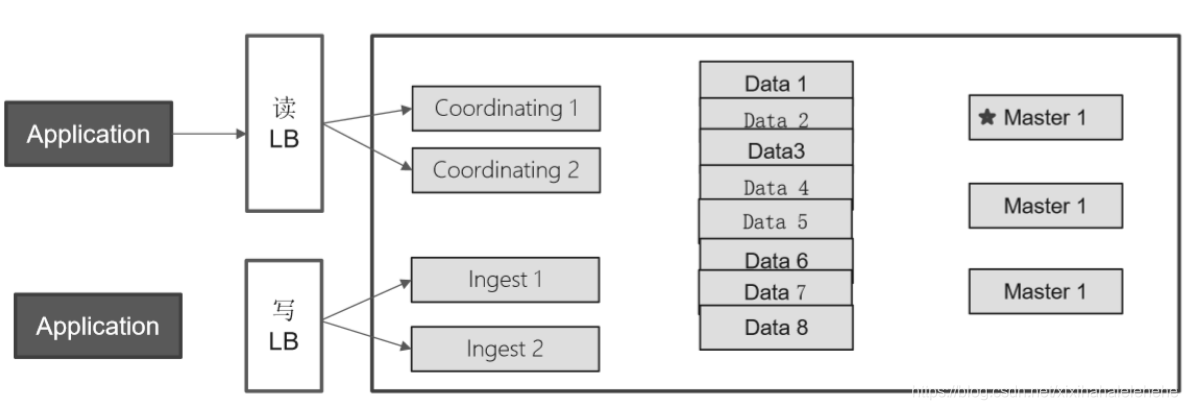
## 10. 在集群里部署 Kibana
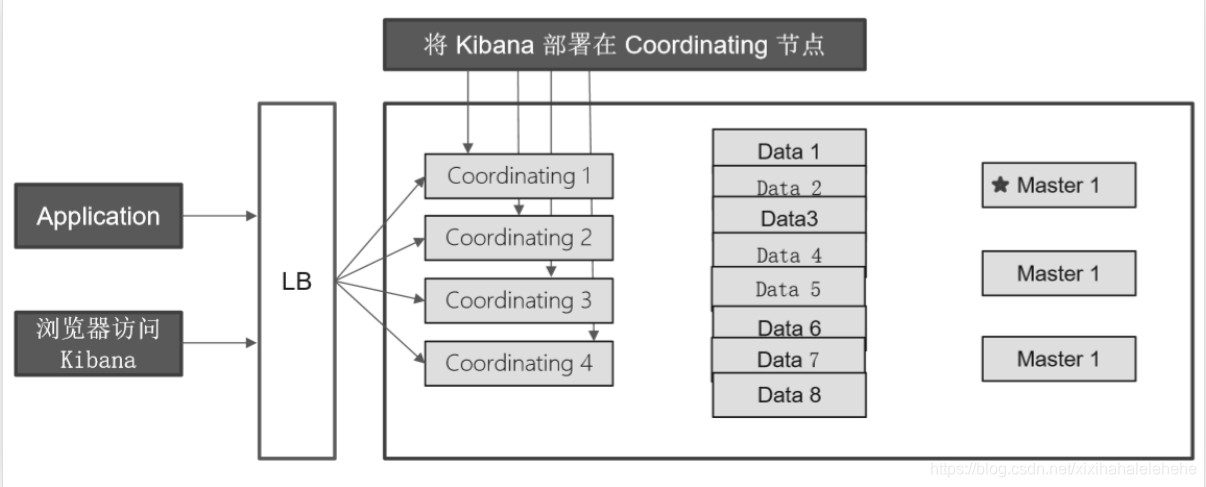
## 11. 异地多活的部署
集群处在三个数据中心;数据三写;GTM 分发读请求
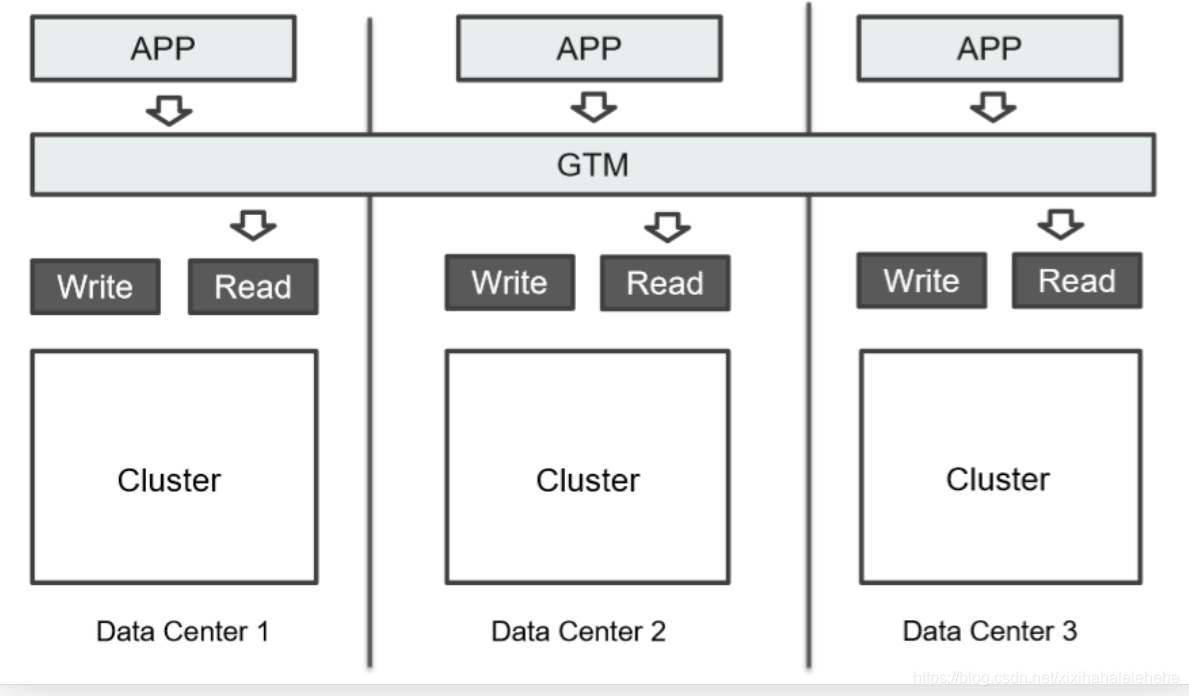 |
package com.softserve.itacademy.rest;
import com.softserve.itacademy.dto.TaskDto;
import com.softserve.itacademy.dto.TaskTransformer;
import com.softserve.itacademy.model.Task;
import com.softserve.itacademy.service.StateService;
import com.softserve.itacademy.service.TaskService;
import com.softserve.itacademy.service.ToDoService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.web.bind.annotation.*;
import javax.validation.Valid;
@RestController
@RequestMapping("/api/tasks")
public class TaskRestController {
private final TaskService taskService;
private final ToDoService todoService;
private final StateService stateService;
@Autowired
public TaskRestController(TaskService taskService, ToDoService todoService, StateService stateService) {
this.taskService = taskService;
this.todoService = todoService;
this.stateService = stateService;
}
@PostMapping({"/{todoId}"})
@PreAuthorize("@toDoRestController.getToDoReadingLevel(#todoId) <= 1 || hasAuthority('ADMIN')")
public TaskDto create(@PathVariable long todoId, @Valid @RequestBody TaskDto taskDto) {
Task task = TaskTransformer.convertToEntity(
taskDto,
todoService.readById(todoId),
stateService.getByName("New")
);
task = taskService.create(task);
taskDto = TaskTransformer.convertToDto(task);
return taskDto;
}
@GetMapping("/{taskId}")
@PreAuthorize("hasAuthority('ADMIN')")
public TaskDto read(@PathVariable long taskId) {
Task task = taskService.readById(taskId);
return TaskTransformer.convertToDto(task);
}
@PutMapping("/{todoId}/{taskId}")
@PreAuthorize("@toDoRestController.getToDoReadingLevel(#todoId) <= 1 || hasAuthority('ADMIN')")
public TaskDto update(@PathVariable long todoId, @PathVariable long taskId, @Valid @RequestBody TaskDto taskDto) {
if (taskId != taskDto.getId()) {
throw new IllegalArgumentException("id cannot be changed");
}
Task task = TaskTransformer.convertToEntity(
taskDto,
todoService.readById(taskDto.getTodoId()),
stateService.readById(taskDto.getStateId())
);
task = taskService.update(task);
taskDto = TaskTransformer.convertToDto(task);
return taskDto;
}
@DeleteMapping("/{todoId}/{taskId}")
@PreAuthorize("@toDoRestController.getToDoReadingLevel(#todoId) <= 1 || hasAuthority('ADMIN')")
public void delete(@PathVariable long todoId, @PathVariable long taskId) {
taskService.delete(taskId);
}
} |
import { describe, expect, it } from 'vitest';
import { AgentRuntimeErrorType } from '@/libs/agent-runtime';
import { ChatErrorType } from '@/types/fetch';
import { createErrorResponse } from './errorResponse';
describe('createErrorResponse', () => {
// 测试各种错误类型的状态码
it('returns a 401 status for NoOpenAIAPIKey error type', () => {
const errorType = ChatErrorType.NoOpenAIAPIKey;
const response = createErrorResponse(errorType);
expect(response.status).toBe(401);
});
it('returns a 401 status for InvalidAccessCode error type', () => {
const errorType = ChatErrorType.InvalidAccessCode;
const response = createErrorResponse(errorType as any);
expect(response.status).toBe(401);
});
// 测试包含Invalid的错误类型
it('returns a 401 status for Invalid error type', () => {
const errorType = 'InvalidTestError';
const response = createErrorResponse(errorType as any);
expect(response.status).toBe(401);
});
it('returns a 403 status for LocationNotSupportError error type', () => {
const errorType = AgentRuntimeErrorType.LocationNotSupportError;
const response = createErrorResponse(errorType);
expect(response.status).toBe(403);
});
describe('Provider Biz Error', () => {
it('returns a 471 status for OpenAIBizError error type', () => {
const errorType = AgentRuntimeErrorType.OpenAIBizError;
const response = createErrorResponse(errorType);
expect(response.status).toBe(471);
});
it('returns a 471 status for ProviderBizError error type', () => {
const errorType = AgentRuntimeErrorType.ProviderBizError;
const response = createErrorResponse(errorType);
expect(response.status).toBe(471);
});
it('returns a 470 status for AgentRuntimeError error type', () => {
const errorType = AgentRuntimeErrorType.AgentRuntimeError;
const response = createErrorResponse(errorType);
expect(response.status).toBe(470);
});
it('returns a 471 status for OpenAIBizError error type', () => {
const errorType = AgentRuntimeErrorType.OpenAIBizError;
const response = createErrorResponse(errorType as any);
expect(response.status).toBe(471);
});
});
// 测试状态码不在200-599范围内的情况
it('logs an error when the status code is not a number or not in the range of 200-599', () => {
const errorType = 'Unknown Error';
const consoleSpy = vi.spyOn(console, 'error');
try {
createErrorResponse(errorType as any);
} catch (e) {}
expect(consoleSpy).toHaveBeenCalled();
consoleSpy.mockRestore();
});
// 测试默认情况
it('returns the same error type as status for unknown error types', () => {
const errorType = 500; // 假设500是一个未知的错误类型
const response = createErrorResponse(errorType as any);
expect(response.status).toBe(errorType);
});
// 测试返回的Response对象是否包含正确的body和errorType
it('returns a Response object with the correct body and errorType', () => {
const errorType = ChatErrorType.NoOpenAIAPIKey;
const body = { message: 'No API key provided' };
const response = createErrorResponse(errorType, body);
return response.json().then((data) => {
expect(data).toEqual({
body,
errorType,
});
});
});
// 测试没有提供body时,返回的Response对象的body是否为undefined
it('returns a Response object with an undefined body when no body is provided', () => {
const errorType = ChatErrorType.NoOpenAIAPIKey;
const response = createErrorResponse(errorType);
return response.json().then((data) => {
expect(data.body).toBeUndefined();
});
});
}); |
<<<<<<< HEAD
#618
'''
Python | Check if a given string is binary or not
'''
# Using set
def check(string):
p = set(string)
s = {'0','1'}
if s == p or p == {'0'} or p == {'1'}:
print("Yes")
else:
print("No")
if __name__ == "__main__":
string = "101010000111"
check(string)
############################################################################################################
#619
'''
simple iteration
'''
def check2(string):
t = '01'
count = 0
for char in string:
if char not in t:
count = 1
break
else:
pass
if count:
print("No")
else:
print("Yes")
if __name__ == "__main__":
string = "00012011101001010"
check2(string)
#############################################################################################################
#620
'''
Regular expression
'''
import re
sampleInput = "1001010"
c = re.compile('[^01]')
if(len(c.findall(sampleInput))):
print("No")
else:
print("Yes")
##############################################################################################################
#621
'''
Using exception handling and int
'''
def check(string):
try:
int(string,2)
except ValueError:
return "No"
return "Yes"
if __name__ == "__main__":
string1 = "101011001111"
string2 = "201000001"
print(check(string1))
print(check(string2))
##############################################################################################################
#622
'''
Using count() function
'''
string = "1010001010101"
if(string.count('0')+string.count('1')==len(string)):
print("Yes")
else:
print("No")
##############################################################################################################
#623
'''
Using replace() and len() methods
'''
string = '1010201010101'
binary = '01'
for i in binary:
string = string.replace(i,"")
if(len(string) == 0):
print("Yes")
else:
print("No")
#################################################################################################################
#624
'''
Using all()
'''
def check(string):
if all((letter in "01") for letter in string):
return "Yes"
return "No"
if __name__ == "__main__":
string1 = "101011001111"
string2 = "201000001"
print(check(string1))
print(check(string2))
#############################################################################################################
#625
'''
Using issubset() method
'''
def is_binary_string(s):
unique_chars = {c for c in s}
return unique_chars.issubset({'0','1'})
if __name__ == "__main__":
string1 = "101011001111"
string2 = "201000001"
print(is_binary_string(string1))
print(is_binary_string(string2))
###############################################################################################################
#626
'''
Using re.search()
'''
import re
def is_binary_string(str):
regex = r"[^01]"
if re.search(regex,str):
return False
else:
return True
print(is_binary_string(string1))
print(is_binary_string(string2))
###############################################################################################################
=======
#618
'''
Python | Check if a given string is binary or not
'''
# Using set
def check(string):
p = set(string)
s = {'0','1'}
if s == p or p == {'0'} or p == {'1'}:
print("Yes")
else:
print("No")
if __name__ == "__main__":
string = "101010000111"
check(string)
############################################################################################################
#619
'''
simple iteration
'''
def check2(string):
t = '01'
count = 0
for char in string:
if char not in t:
count = 1
break
else:
pass
if count:
print("No")
else:
print("Yes")
if __name__ == "__main__":
string = "00012011101001010"
check2(string)
#############################################################################################################
#620
'''
Regular expression
'''
import re
sampleInput = "1001010"
c = re.compile('[^01]')
if(len(c.findall(sampleInput))):
print("No")
else:
print("Yes")
##############################################################################################################
#621
'''
Using exception handling and int
'''
def check(string):
try:
int(string,2)
except ValueError:
return "No"
return "Yes"
if __name__ == "__main__":
string1 = "101011001111"
string2 = "201000001"
print(check(string1))
print(check(string2))
##############################################################################################################
#622
'''
Using count() function
'''
string = "1010001010101"
if(string.count('0')+string.count('1')==len(string)):
print("Yes")
else:
print("No")
##############################################################################################################
#623
'''
Using replace() and len() methods
'''
string = '1010201010101'
binary = '01'
for i in binary:
string = string.replace(i,"")
if(len(string) == 0):
print("Yes")
else:
print("No")
#################################################################################################################
#624
'''
Using all()
'''
def check(string):
if all((letter in "01") for letter in string):
return "Yes"
return "No"
if __name__ == "__main__":
string1 = "101011001111"
string2 = "201000001"
print(check(string1))
print(check(string2))
#############################################################################################################
#625
'''
Using issubset() method
'''
def is_binary_string(s):
unique_chars = {c for c in s}
return unique_chars.issubset({'0','1'})
if __name__ == "__main__":
string1 = "101011001111"
string2 = "201000001"
print(is_binary_string(string1))
print(is_binary_string(string2))
###############################################################################################################
#626
'''
Using re.search()
'''
import re
def is_binary_string(str):
regex = r"[^01]"
if re.search(regex,str):
return False
else:
return True
print(is_binary_string(string1))
print(is_binary_string(string2))
###############################################################################################################
>>>>>>> 411c1dae0476b61d9837f2ef36555e5ad68e8cf4 |
# 南墙简介
[](https://github.com/Safe3/uuWAF)
[](https://github.com/Safe3/uuWAF/discussions)
> **南墙**WEB应用防火墙(简称:`uuWAF`)一款社区驱动的免费、高性能、高扩展顶级Web应用安全防护产品。
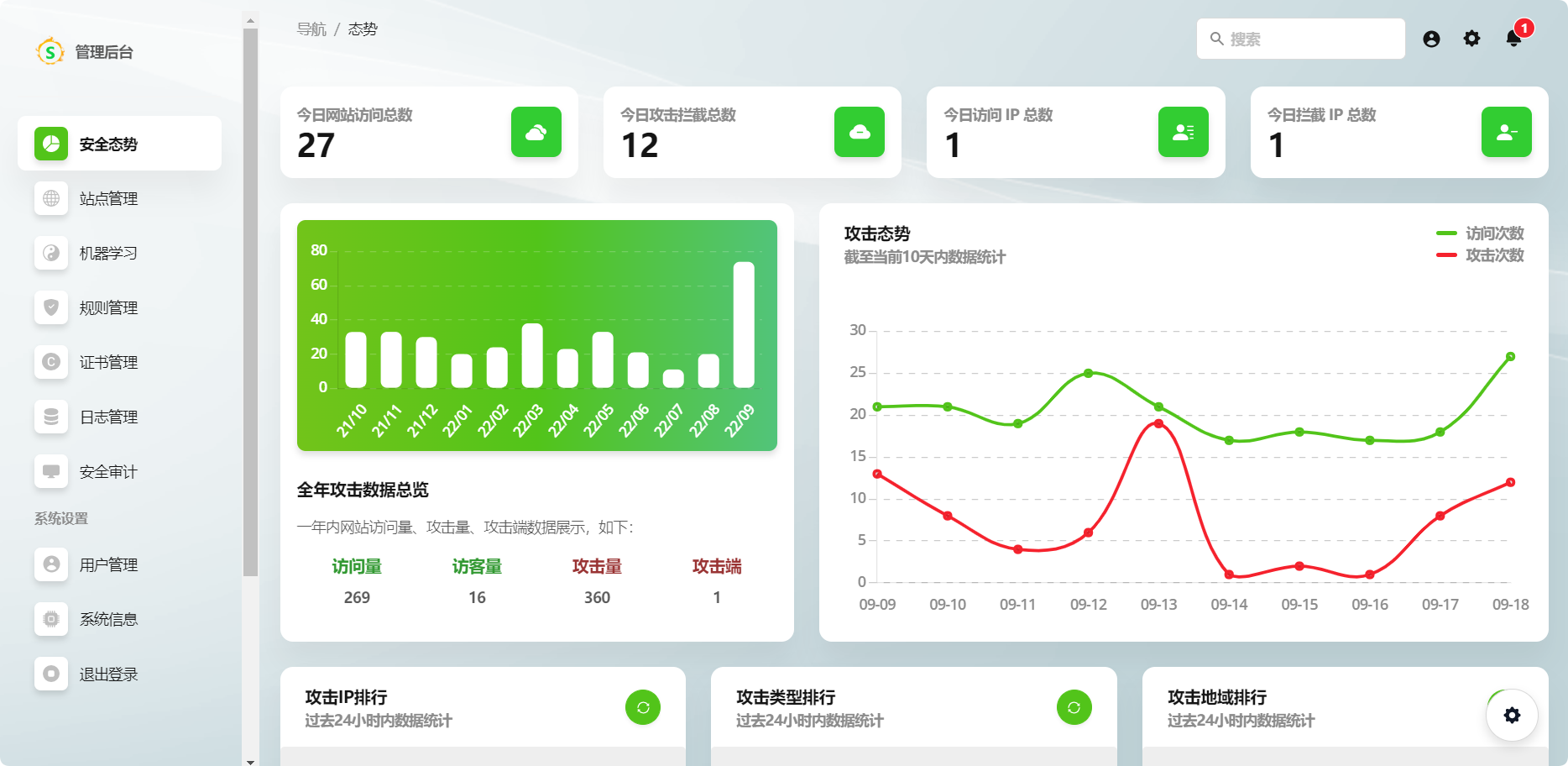
🏠安装及使用请访问官网: https://waf.uusec.com/
:heavy_exclamation_mark:注意:南墙 暂不开源,直接下载编译好的二进制文件安装即可,github仓库内主要为社区贡献的规则,每次 uuWAF 发布将自动更新。
## :dart: 技术优势
- :libra: 先进语义引擎
南墙采用业界领先的`SQL、XSS、RCE、LFI` 4种基于语义分析的检测引擎,结合多种深度解码引擎可对`base64、json、form-data`等HTTP内容真实还原,从而有效抵御各种绕过WAF的攻击方式,并且相比传统正则匹配具备准确率高、误报率低、效率高等特点,管理员无需维护庞杂的规则库,即可拦截多种攻击类型。
- :ophiuchus: 智能0day防御
南墙创新性的运用机器学习技术,使用**异常检测算法**对http正常与攻击流量进行区分识别,并对正常流量进行白名单威胁建模。通过**机器学习算法**自动学习正常流量中的参数特征,并转化成对应的参数白名单规则库,可以在面对各种突发0day漏洞时,无需添加规则即可拦截攻击,免除网站管理者一出现漏洞就需挑灯夜战升级的痛苦。
- :gemini: 高级规则引擎
南墙积极运用`nginx`和`luajit`的高性能、高灵活性特点,除了提供对普通用户友好性较好的传统规则创建模式,还提供了高扩展性、高灵活性的lua脚本规则编写功能,使得有一定编程功底的高级安全管理员可以创造出一系列传统WAF所不能实现的高级漏洞防护规则,用户可以编写一系列插件来扩展WAF现有功能。从而使得在拦截一些复杂漏洞时,可以更加得心应手。
## :rocket: 一键安装
南墙为你提供了强大灵活的扩展和安全规则的编写API,在管理后台发布后所有规则无需重启立即生效,远超市面上大部分免费WAF产品如`ModSecurity`,规则展示如下:
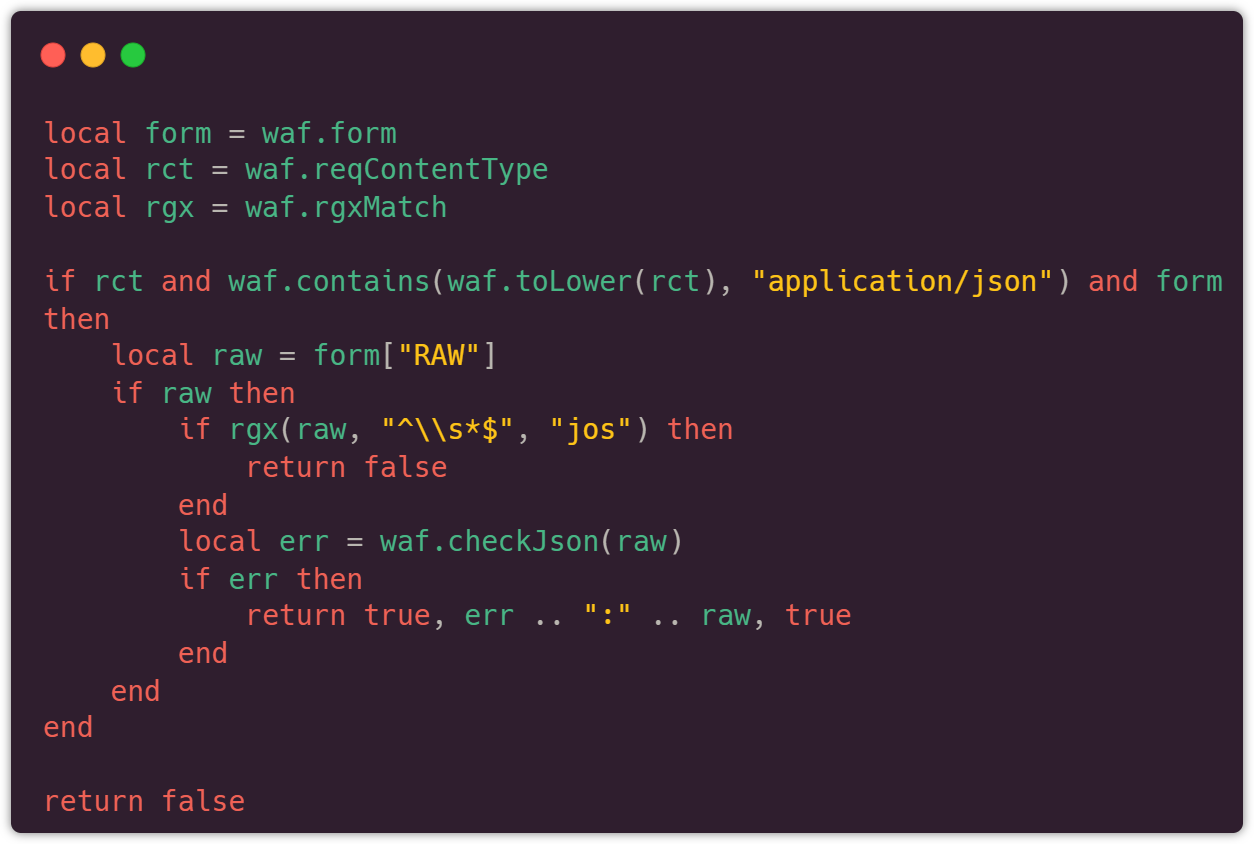
🏠请访问官网: https://waf.uusec.com/ 下载 南墙WAF使用说明书 了解规则API详情
南墙安装及其简便,通常在几分钟内即可安装完毕,具体耗时视网络下载情况而定。
注意:请尽量选择一台纯净Linux x86_64环境的服务器安装,因为安装过程会卸载旧的MySQL数据库并重新安装,如果没有备份,可造成旧的MySQL数据丢失,并且南墙采用云WAF反向代理模式,默认需要使用80、443端口。
> 主机版安装方式如下:
```bash
sudo yum install -y ca-certificates
curl https://waf.uusec.com/waf-install -o waf-install && sudo bash ./waf-install && rm -f ./waf-install
```
安装成功后会显示 “ 恭喜您,安装成功!”
> Docker版安装方式如下:
```bash
curl https://waf.uusec.com/waf.tgz -o waf.tgz && tar -zxf waf.tgz && sudo bash ./waf/uuwaf.sh
```
> 1Panel安装方式如下:
1Panel 是新一代的 Linux 服务器运维管理面板,下载 https://1panel.cn/ 后在应用商店中找到南墙安装。
> 快速入门:
1. 登录后台:访问https://ip:4443 ,ip为安装南墙的服务器ip地址,用户名admin,密码wafadmin。
2. 添加站点:进入站点管理菜单,点击添加站点按钮,按提示添加站点域名与网站服务器ip。
3. 添加TLS证书:进入证书管理菜单,点击添加证书按钮,上传第二步中域名的https证书和私钥文件。
4. 修改域名DNS指向:到域名服务商管理后台把域名DNS A记录的ip地址改为南墙服务器ip地址。
5. 测试连通性:访问站点域名查看网站是否能够打开,查看返回的http header头server字段是否为uuWAF。
## :gift_heart: 贡献名单
如何贡献?参照: https://waf.uusec.com/#/guide/contribute
这里感谢puhui222、Kingdom为南墙所做的贡献!
## :kissing_heart: 加入讨论
欢迎各位就 南墙 的各种bug或功能需求及使用问题,在如下渠道参与讨论
- 问题提交:https://github.com/Safe3/uuWAF/issues
- 讨论社区:https://github.com/Safe3/uuWAF/discussions
- 官方 QQ 群:11500614
- 官方微信群:微信扫描以下二维码加入
<img src="https://waf.uusec.com/_media/weixin.jpg" alt="微信群" height="200px" /> |
/* function example() {
const x = 3.14;
if(true) {
const y = 12;
}
console.log(x)
console.log(y)
}
example()
console.log(x)
console.log(y) */
// Hoisting
// console.log(a)
// const a = 10
// console.log(a)
/* function calculator(a,b,callback) {
return callback(a, b);
}
function add(a, b) {
return a+b
}
function mul(a, b) {
return a*b
}
var res = calculator(5, 3, mul);
console.log(res)
var res = calculator(5, 3, add);
console.log(res) */
// CALLBACK HELL
/* const placeorder = (callback) => {
setTimeout(()=>{
console.log("order placed");
callback();
}, 2000)
}
const startProduction = (callback) => {
setTimeout(() => {
console.log("production started")
callback();
}, 5000)
}
const printId = (callback) => {
setTimeout(() => {
console.log("id printed")
callback();
}, 5000)
}
const productName = (callback) => {
setTimeout(() => {
console.log("productName printed")
callback();
}, 5000)
}
const productDesc = () => {
console.log("productDesc printed")
}
console.log('ordering product')
placeorder(() => {
console.log('in production');
startProduction(() => {
console.log("printing item started");
printId(() => {
productName(() => {
productDesc();
console.log(`day ended`);
})
})
})
}) */
const placeorder = (callback) => {
setTimeout(() => {
console.log("Order placed");
callback();
}, 2000);
};
const bookticket = (callback) => {
setTimeout(() => {
console.log("Ticket booked");
callback();
}, 1000);
};
const boardingpass = (callback) => {
setTimeout(() => {
console.log("Boarding pass issued");
callback();
}, 2000);
};
const securitycheck = (callback) => {
setTimeout(() => {
console.log("security checked pass");
callback();
}, 2000);
};
const selfcheck = (callback) => {
setTimeout(() => {
console.log("self checked pass");
callback();
}, 2000);
};
const navigationpanel = (callback) => {
setTimeout(() => {
console.log("navigation panel crossed");
callback();
}, 2000);
};
const buspickup = (callback) => {
setTimeout(() => {
console.log("pickup bus reached");
callback();
}, 2000);
};
const airplanetakeoff = (callback) => {
setTimeout(() => {
console.log("airplane takeoff");
callback();
}, 2000);
};
const aircraft = (callback) => {
setTimeout(() => {
console.log("aircraft");
callback();
}, 2000);
};
const destination = () => {
console.log("detination reached");
};
console.log("booking ticket");
placeorder(() => {
bookticket(() => {
boardingpass(() => {
securitycheck(() => {
selfcheck(() => {
navigationpanel(() => {
buspickup(() => {
airplanetakeoff(() => {
aircraft(() => {
destination();
console.log("journey completed");
});
});
});
});
});
});
});
});
}); |
import torch
import torchvision.models as models
from sklearn.metrics import precision_recall_fscore_support as score
def train(params, DataClass, model, criterion, device = "cpu"):
optimizer = torch.optim.SGD(model.parameters(), lr = 0.01)
scheduler = torch.optim.lr_scheduler.CosineAnnealingLR(optimizer, T_max=10, eta_min=0)
model.to(device)
for epoch in range(params['num_epochs']):
model.train()
running_loss = 0.0
for _, metadata in enumerate(DataClass.dataloader['train']):
inputs = metadata[0]
labels = metadata[1]
inputs, labels = inputs.to(device), labels.to(device)
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item() * inputs.size(0)
scheduler.step()
epoch_loss = running_loss / len(DataClass.dataset['train'])
print(f"Epoch {epoch+1}/{params['num_epochs']}, Loss: {epoch_loss:.4f}")
return model
def test(DataClass, model, device):
model.eval()
model.to(device)
y_pred = torch.tensor([], device=device)
y_true = torch.tensor([], device=device)
with torch.no_grad():
for _, metadata in enumerate(DataClass.dataloader['test']):
inputs = metadata[0]
labels = metadata[1]
inputs, labels = inputs.to(device), labels.to(device)
outputs = model(inputs)
_, predicted = torch.max(outputs, 1)
#Append to the mega-list
y_pred = torch.cat((y_pred, predicted), dim = 0)
y_true = torch.cat((y_true, labels), dim = 0)
accuracy = (y_pred == y_true).sum().item() / y_true.size(0)
print(f"Accuracy on test set: {accuracy:.4f}")
y_true = y_true.cpu()
y_pred = y_pred.cpu()
sc = score(y_true, y_pred)
#verbose prints
print(f"Accuracy on test set: {accuracy:.4f}")
print('Precision: {}'.format(sc[0]))
print('Recall: {}'.format(sc[1]))
print('F1-Score: {}'.format(sc[2])) |
<template>
<!-- Auth Modal -->
<div class="fixed z-10 inset-0 overflow-y-auto" id="modal" :class="hiddenClass">
<div
class="flex items-end justify-center min-h-screen pt-4 px-4 pb-20 text-center sm:block sm:p-0"
>
<div class="fixed inset-0 transition-opacity">
<div class="absolute inset-0 bg-gray-800 opacity-75"></div>
</div>
<!-- This element is to trick the browser into centering the modal contents. -->
<span class="hidden sm:inline-block sm:align-middle sm:h-screen">​</span>
<div
class="inline-block align-bottom bg-white rounded-lg text-left overflow-hidden shadow-xl transform transition-all sm:my-8 sm:align-middle sm:max-w-lg sm:w-full"
>
<!-- Add margin if you want to see some of the overlay behind the modal-->
<div class="py-4 text-left px-6">
<!--Title-->
<div class="flex justify-between items-center pb-4">
<p class="text-2xl font-bold">Your Account</p>
<!-- Modal Close Button -->
<div class="modal-close cursor-pointer z-50" @click="modalVisibility = false">
<i class="fas fa-times"></i>
</div>
</div>
<!-- Tabs -->
<ul class="flex flex-wrap mb-4">
<li class="flex-auto text-center">
<a
class="block rounded py-3 px-4 transition"
href="#"
@click.prevent="tab = 'login'"
:class="{
'hover:text-white text-white bg-blue-600': tab === 'login',
'hover:text-blue-600': tab === 'register'
}"
>Login</a
>
</li>
<li class="flex-auto text-center">
<a
class="block rounded py-3 px-4 transition"
href="#"
@click.prevent="tab = 'register'"
:class="{
'hover:text-white text-white bg-blue-600': tab === 'register',
'hover:text-blue-600': tab === 'login'
}"
>Register</a
>
</li>
</ul>
<!-- Login Form -->
<form v-show="tab === 'login'">
<!-- Email -->
<div class="mb-3">
<label class="inline-block mb-2">Email</label>
<input
type="email"
class="block w-full py-1.5 px-3 text-gray-800 border border-gray-300 transition duration-500 focus:outline-none focus:border-black rounded"
placeholder="Enter Email"
/>
</div>
<!-- Password -->
<div class="mb-3">
<label class="inline-block mb-2">Password</label>
<input
type="password"
class="block w-full py-1.5 px-3 text-gray-800 border border-gray-300 transition duration-500 focus:outline-none focus:border-black rounded"
placeholder="Password"
/>
</div>
<button
type="submit"
class="block w-full bg-purple-600 text-white py-1.5 px-3 rounded transition hover:bg-purple-700"
>
Submit
</button>
</form>
<!-- Registration Form -->
<vee-form v-show="tab === 'register'" :validation-schema="schema">
<!-- Name -->
<div class="mb-3">
<label class="inline-block mb-2">Name</label>
<vee-field
type="text"
name="name"
class="block w-full py-1.5 px-3 text-gray-800 border border-gray-300 transition duration-500 focus:outline-none focus:border-black rounded"
placeholder="Enter Name"
/>
<error-message class="text-red-600" name="name" />
</div>
<!-- Email -->
<div class="mb-3">
<label class="inline-block mb-2">Email</label>
<vee-field
name="email"
type="email"
class="block w-full py-1.5 px-3 text-gray-800 border border-gray-300 transition duration-500 focus:outline-none focus:border-black rounded"
placeholder="Enter Email"
/>
<error-message class="text-red-600" name="email" />
</div>
<!-- Age -->
<div class="mb-3">
<label class="inline-block mb-2">Age</label>
<vee-field
name="age"
type="number"
class="block w-full py-1.5 px-3 text-gray-800 border border-gray-300 transition duration-500 focus:outline-none focus:border-black rounded"
/>
<error-message class="text-red-600" name="age" />
</div>
<!-- Password -->
<div class="mb-3">
<label class="inline-block mb-2">Password</label>
<vee-field
name="password"
type="password"
class="block w-full py-1.5 px-3 text-gray-800 border border-gray-300 transition duration-500 focus:outline-none focus:border-black rounded"
placeholder="Password"
/>
<error-message class="text-red-600" name="password" />
</div>
<!-- Confirm Password -->
<div class="mb-3">
<label class="inline-block mb-2">Confirm Password</label>
<vee-field
name="confirm_password"
type="password"
class="block w-full py-1.5 px-3 text-gray-800 border border-gray-300 transition duration-500 focus:outline-none focus:border-black rounded"
placeholder="Confirm Password"
/>
<error-message class="text-red-600" name="confirm_password" />
</div>
<!-- Country -->
<div class="mb-3">
<label class="inline-block mb-2">Country</label>
<select
class="block w-full py-1.5 px-3 text-gray-800 border border-gray-300 transition duration-500 focus:outline-none focus:border-black rounded"
>
<option value="USA">USA</option>
<option value="Mexico">Mexico</option>
<option value="Germany">Germany</option>
</select>
</div>
<!-- TOS -->
<div class="mb-3 pl-6">
<input type="checkbox" class="w-4 h-4 float-left -ml-6 mt-1 rounded" />
<label class="inline-block">Accept terms of service</label>
</div>
<button
type="submit"
class="block w-full bg-purple-600 text-white py-1.5 px-3 rounded transition hover:bg-purple-700"
>
Submit
</button>
</vee-form>
</div>
</div>
</div>
</div>
</template>
<script>
import { mapState, mapWritableState } from 'pinia'
import useModalStore from '@/stores/modal'
export default {
name: 'AppAuth',
data() {
return {
tab: 'login',
schema: {
name: 'required|min:3|max:100|alphaSpaces',
email: 'required|min:3|max:100|email',
age: 'required|min_value:18|max_value:100',
password: 'required|min:3|max:100',
confirm_password: 'confirmed:@password',
country: '',
tos: ''
}
}
},
computed: {
...mapState(useModalStore, ['hiddenClass']),
...mapWritableState(useModalStore, {
modalVisibility: 'isOpen'
})
}
}
</script> |
from typing import NamedTuple
class Employee(NamedTuple):
"""Represents an employee."""
name: str
id: int = 3
def __repr__(self) -> str:
return f'<Employee: {self.name!r}, id={self.id}>'
x = Employee('Bob', 5)
print(x)
print(x.name) |
import React from "react";
import { AiOutlineClose } from "react-icons/ai";
import ModalContainer from "./ModalContainer";
//Component which handles the the writers modal
export default function WritersModal({
profiles = [],//array having all the writers details
visible,//visibility of modal
onClose,
onRemoveClick,//function accepts id and removes writer with that id from writers array
}) {
return (
<ModalContainer ignoreContainer onClose={onClose} visible={visible}>
<div className="space-y-2 dark:bg-primary bg-white rounded max-w-[45rem] max-h-[40rem] overflow-auto p-2 custom-scroll-bar">
{profiles.map(({ id, name, avatar }) => {
return (
<div
key={id}
className="flex space-x-3 dark:bg-secondary bg-white drop-shadow-md rounded"
>
<img
className="w-16 h-16 aspect-square rounded object-cover"
src={avatar}
alt={name}
/>
<p className="w-full font-semibold dark:text-white text-primary">
{name}
</p>
<button
onClick={() => onRemoveClick(id)}
className="dark:text-white text-primary hover:opacity-80 transition p-2"
>
<AiOutlineClose />
</button>
</div>
);
})}
</div>
</ModalContainer>
);
} |
/* Given a linked list, the task is to find the sum of all subsets of a linked list.
Examples:
Input: 2 -> 3 -> NULL
Output: 10
Explanation:
All non-empty subsets are {2}, {3} and {2, 3}
Total sum = 2 + 3 + (2 + 3) = 10
*/
#include<iostream>
#include<cmath>
using namespace std;
struct Node {
int data;
struct Node* next;
};
void push(struct Node **head_ref, int new_data) {
struct Node* new_node = (struct Node*) malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = NULL;
if(*head_ref == NULL) {
*head_ref = new_node;
return;
}
struct Node* temp = *head_ref;
while(temp->next!=NULL) {
temp = temp->next;
}
temp->next = new_node;
}
void printLinkedList(struct Node* temp) {
while(temp!=NULL) {
cout << temp->data << " ";
temp = temp->next;
}
cout << endl;
}
int sum(struct Node* head, int n) {
int sum = 0, result;
struct Node *temp = head;
while(temp!=NULL) {
sum += temp->data;
temp = temp->next;
}
result = sum * pow(2, n-1);
return result;
}
int main() {
struct Node* head = NULL;
int n;
cout << "Enter the number of elements" << endl;
cin >> n;
cout << "Enter the elements" << endl;
int val;
for(int i = 0 ; i < n; i++) {
cin >> val;
push(&head, val);
}
cout << "Initial Linked List" << endl;
printLinkedList(head);
cout << "The sum of all subsets is " << sum(head, n) << endl;
return 0;
} |
package com.mercadolivro.controller.request
import com.mercadolivro.validation.EmailAvailable
import javax.validation.constraints.Email
import javax.validation.constraints.NotEmpty
data class PostCustomerRequest (
@field:NotEmpty(message = "Field name must be informed")
var name: String,
@EmailAvailable
@field:Email(message = "Field email must be valid")
var email: String,
@field:NotEmpty(message = "Inform the password")
var password : String
) |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>typeof & instanceof</title>
</head>
<body>
<h3 style="text-align: center">
typeof 对于对象来说,除了函数都会显示 object,所以说 typeof 并不能准确判断变量到底是什么类型,<br>
如果我们想判断一个对象的正确类型,这时候可以考虑使用 instanceof,因为内部机制是通过 原型链 来判断的
</h3>
</body>
<script>
// 判断 foo 是否是 Foo 类的实例 , 并且是否是其父类型的实例
function Aoo() {
}
function Foo() {
}
Foo.prototype = new Aoo();//JavaScript 原型继承
var foo = new Foo();
console.log(foo instanceof Foo)//true
console.log(foo instanceof Aoo)//true
// 复杂用法
console.log(Object instanceof Object);//true
console.log(Function instanceof Function);//true
console.log(Number instanceof Number);//false
console.log(String instanceof String);//false
console.log(Function instanceof Object);//true
console.log(Foo instanceof Function);//true
console.log(Foo instanceof Foo);//false
function instance_of(L, R) {//L 表示左表达式,R 表示右表达式
if (typeof(L) != 'object') return false
let O = R.prototype;// 取 R 的显示原型
L = L.__proto__;// 取 L 的隐式原型
while (true) {
if (L === null) return false;
if (O === L) return true; // 这里重点:当 O 严格等于 L 时,返回 true
L = L.__proto__;
}
}
console.log(instance_of(Object, Object))
</script>
</html> |
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package Controller;
import DAO.PostTypeDAO;
import Model.PostType;
import java.io.IOException;
import java.io.PrintWriter;
import jakarta.servlet.ServletException;
import jakarta.servlet.annotation.WebServlet;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
/**
*
* @author DUY
*/
@WebServlet(name = "UpdatePostTypeController", urlPatterns = {"/UpdatePostTypeController"})
public class UpdatePostTypeController extends HttpServlet {
private static final String ERROR = "configPage.jsp";
private static final String SUCCESS = "configPage.jsp";
/**
* Processes requests for both HTTP <code>GET</code> and <code>POST</code>
* methods.
*
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
protected void processRequest(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
String url = ERROR;
PostTypeDAO dao = new PostTypeDAO();
PostType postType = new PostType();
try {
String postType_name = request.getParameter("postType_name_update");
int postType_id = Integer.parseInt(request.getParameter("postType_id"));
if (postType_name != null && !postType_name.isEmpty()) {
boolean checkExists = dao.isPostTypeExists(postType_name);
if (checkExists) {
request.setAttribute("POSTTYPE_ERROR", "Thể loại bài viết đã tồn tại!");
} else {
postType.setPostTypeId(postType_id);
postType.setPostTypeName(postType_name);
boolean check = dao.updatePostType(postType);
if (check) {
url = SUCCESS;
}
}
}
} catch (Exception e) {
log("Error at UpdatePostTypeController: " + e.toString());
} finally {
request.getRequestDispatcher(url).forward(request, response);
}
}
// <editor-fold defaultstate="collapsed" desc="HttpServlet methods. Click on the + sign on the left to edit the code.">
/**
* Handles the HTTP <code>GET</code> method.
*
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
processRequest(request, response);
}
/**
* Handles the HTTP <code>POST</code> method.
*
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
processRequest(request, response);
}
/**
* Returns a short description of the servlet.
*
* @return a String containing servlet description
*/
@Override
public String getServletInfo() {
return "Short description";
}// </editor-fold>
} |
import React, { useState } from "react";
import { updateUserAvatarAPI } from "../api/userApi";
import { useNavigate } from "react-router-dom";
import CountDown from "../utils/CountDown";
function UpdateAvatar() {
const navigate = useNavigate()
const [modelOpen,setModelOpen] = useState(false)
const [avatar,setAvatar] = useState(null)
const [check,setCheck] = useState(false)
const closeModal=()=>{
setModelOpen(false)
}
function handleAvatar(e){
const file = e.target.files[0]
if(file){
setAvatar(file)
}
}
function handleSubmit(e){
e.preventDefault()
if(check){
if(avatar){
setModelOpen(true)
const formData = new FormData()
formData.append("newAvatar",avatar)
updateUserAvatarAPI(formData)
.then((data)=>{
console.log(data)
const accessToken = localStorage.getItem('accessToken')
const refreshToken = localStorage.getItem('refreshToken')
localStorage.clear()
localStorage.setItem('user',JSON.stringify(data.data))
localStorage.setItem('refreshToken',refreshToken)
localStorage.setItem('accessToken',accessToken)
navigate("/profile")
window.location.reload()
})
.catch((error)=>{
console.log("error", error)
})
}
}
}
return (
<div>
{/* COUNTDOWN */}
{modelOpen && <CountDown onClose={closeModal} />}
{/* header */}
<div className="flex justify-center items-center mt-4 mb-10">
<h3 className="bg-black p-3 font-lg rounded-lg text-white font-semibold">
Update Profile Avatar
</h3>
</div>
<form class="w-[90%] md:w-[70%] mx-auto ">
<div>
<label
class="block mb-2 text-sm font-medium text-gray-900 dark:text-white"
for="user_avatar"
>
Upload New Avatar Image
</label>
<input
class="block w-full text-sm text-gray-900 border border-gray-300 rounded-lg cursor-pointer bg-gray-50 dark:text-gray-400 focus:outline-none dark:bg-gray-700 dark:border-gray-600 dark:placeholder-gray-400"
aria-describedby="user_avatar_help"
id="user_avatar"
type="file"
onChange={handleAvatar}
required
/>
<div
class="mt-1 text-sm text-gray-500 dark:text-gray-300"
id="user_avatar_help"
>
A profile picture is useful to confirm you are logged into your
account
</div>
</div>
<div class="flex items-start mb-5 mt-5 ">
<div class="flex items-center h-5">
<input
id="terms"
type="checkbox"
value=""
class="w-4 h-4 border border-gray-300 rounded bg-gray-50 focus:ring-3 focus:ring-blue-300 dark:bg-gray-700 dark:border-gray-600 dark:focus:ring-blue-600 dark:ring-offset-gray-800 dark:focus:ring-offset-gray-800"
required
onChange={(e)=>setCheck(!check)}
/>
</div>
<label
for="terms"
class="ms-2 text-sm font-medium text-gray-900 dark:text-gray-300"
>
I want to{" "}
<a
href="#"
class="text-blue-600 hover:underline dark:text-blue-500"
>
update avatar
</a>
</label>
</div>
<button
onClick={handleSubmit}
type="submit"
class="text-white bg-blue-700 hover:bg-blue-800 focus:ring-4 focus:outline-none focus:ring-blue-300 font-medium rounded-lg text-sm px-5 py-2.5 text-center dark:bg-blue-600 dark:hover:bg-blue-700 dark:focus:ring-blue-800"
>
Submit
</button>
</form>
</div>
);
}
export default UpdateAvatar; |
import arc from "@architect/functions"
import bcrypt from "bcryptjs"
import invariant from "tiny-invariant"
import { getUserAssetCount } from "./asset.server"
import { getMenteeCount } from "./mentee.server"
export enum Role {
BUDDY = "0",
HR = "1",
PRESIDENT = "2",
ADMIN = "3",
}
interface Contractor {
agreementStartDate: string
agreementEndDate: string
}
export interface User extends Contractor {
id: `User#${User["email"]}`
email: string
firstName: string
lastName: string
faculty: string
role: Role
}
type UserId = User["id"]
type UserEmail = User["email"]
type UnsavedUser = Omit<User, "id">
export type Password = { password: string }
function email2UserId(email: UserEmail): UserId {
return `User#${email}`
}
export async function getUserListItems(): Promise<User[]> {
const db = await arc.tables()
const result = await db.users.scan({})
return result.Items
}
export async function getUserById(id: UserId): Promise<User | null> {
const db = await arc.tables()
const result = await db.users.query({
KeyConditionExpression: "id = :id",
ExpressionAttributeValues: { ":id": id },
})
const record: User | null = result.Items[0]
return record
}
export async function getUserByEmail(email: UserEmail) {
const userId = email2UserId(email)
return getUserById(userId)
}
async function getUserPasswordByEmail(
email: UserEmail,
): Promise<Password | null> {
const userId = email2UserId(email)
const db = await arc.tables()
const result = await db.passwords.query({
KeyConditionExpression: "userId = :userId",
ExpressionAttributeValues: { ":userId": userId },
})
const [record] = result.Items
return record ?? null
}
export async function createUser({
password,
email,
...userProps
}: UnsavedUser & Password): Promise<User> {
const lowerEmail = email.toLowerCase()
const userId = email2UserId(lowerEmail)
const hashedPassword = await bcrypt.hash(password, 10)
const db = await arc.tables()
await db.passwords.put({
userId,
password: hashedPassword,
})
await db.users.put({
id: userId,
email: lowerEmail,
...userProps,
})
const user = await getUserById(userId)
invariant(user, `User not found after being created. This should not happen`)
return user
}
export async function isEmailUnique(email: UserEmail): Promise<boolean> {
const user = await getUserByEmail(email)
return !user
}
export async function updateUser(updatedUser: Omit<User, "id">): Promise<User> {
const db = await arc.tables()
return await db.users.put({
id: email2UserId(updatedUser.email),
...updatedUser,
})
}
export async function deleteUser(id: UserId): Promise<void> {
const db = await arc.tables()
await Promise.all([
db.passwords.delete({ userId: id }),
db.users.delete({ id }),
])
}
export async function deleteUserByEmail(email: UserEmail): Promise<void> {
const userId = email2UserId(email)
await deleteUser(userId)
}
export async function verifyLogin(
email: UserEmail,
password: Password["password"],
): Promise<User | null> {
// we save all emails as lowercase in the db
const maybeEmailInDb = email.toLowerCase()
const userPassword = await getUserPasswordByEmail(maybeEmailInDb)
if (!userPassword) {
return null
}
const isValid = await bcrypt.compare(password, userPassword.password)
return isValid ? await getUserByEmail(maybeEmailInDb) : null
}
export async function getBuddyById(id: UserId): Promise<User | null> {
const buddy = await getUserById(id)
if (!buddy) {
return null
}
const isBuddyActive = new Date(buddy.agreementEndDate) > new Date()
return isBuddyActive ? buddy : null
}
export async function canUserDeleteUser({
loggedInUser,
userToDelete,
}: {
loggedInUser: User
userToDelete: User
}): Promise<{
canDelete: boolean
reason: string
}> {
const isNotLoggedInUser = loggedInUser.id !== userToDelete.id
if (!isNotLoggedInUser) {
return {
canDelete: false,
reason: "You can not delete yourself",
}
}
const isNotAdmin = userToDelete.role !== Role.ADMIN
if (!isNotAdmin) {
return {
canDelete: false,
reason: "You can not delete an admin",
}
}
const isHigher = loggedInUser.role > userToDelete.role
if (!isHigher) {
return {
canDelete: false,
reason: "You can only delete users with a lower role than you",
}
}
const hasMentees = (await getMenteeCount({ buddyId: userToDelete.id })) > 0
if (hasMentees) {
return {
canDelete: false,
reason: "You can not delete a user with active mentees",
}
}
const hasAssets = (await getUserAssetCount(userToDelete.id)) > 0
if (hasAssets) {
return {
canDelete: false,
reason: "You can not delete a user who has assets",
}
}
return {
canDelete: true,
reason: "",
}
}
export function isUserId(value: any): value is UserId {
return typeof value === "string" && value.startsWith("User#")
} |
// Khai báo một mảng trống ;
// Khai báo một mảng có số phần tử lớn hơn 5
// Tìm độ dài của mảng của bạn
// Lấy mục đầu tiên, mục giữa và mục cuối cùng của mảng
// Khai báo một mảng có tên làmixDataTypes , đặt các kiểu dữ liệu khác nhau vào mảng và tìm độ dài của mảng. Kích thước mảng phải lớn hơn 5
// Khai báo một biến mảng tên itCompanies và gán giá trị ban đầu Facebook, Google, Microsoft, Apple, IBM, Oracle và Amazon
// In mảng bằng console.log()
// In số lượng công ty trong mảng
// In công ty đầu tiên, công ty giữa và công ty cuối cùng
// In ra từng công ty
// Thay đổi từng tên công ty thành chữ hoa và in chúng ra
// In mảng như một câu: Facebook, Google, Microsoft, Apple, IBM,Oracle và Amazon là những công ty CNTT lớn.
// Kiểm tra xem một công ty nhất định có tồn tại trong mảng itCompanies hay không. Nếu nó tồn tại thì trả lại công ty, nếu không thì trả lại không tìm thấy công ty
// Lọc ra các công ty có nhiều hơn một chữ 'o' mà không có phương pháp lọc
// Sắp xếp mảng bằng phương thức Sort()
// Đảo ngược mảng bằng phương thức Reverse()
// Loại bỏ 3 công ty đầu tiên khỏi mảng
// Cắt bỏ 3 công ty cuối cùng khỏi mảng
// Loại bỏ các công ty CNTT trung gian khỏi mảng
// Xóa công ty CNTT đầu tiên khỏi mảng
// Xóa công ty CNTT trung gian hoặc các công ty khỏi mảng
// Xóa công ty CNTT cuối cùng khỏi mảng
// Xóa tất cả các công ty CNTT
const countries = [
"Albania",
"Bolivia",
"Canada",
"Denmark",
"Ethiopia",
"Finland",
"Germany",
"Hungary",
"Ireland",
"Japan",
"Kenya",
];
const webTechs = [
"HTML",
"CSS",
"JavaScript",
"React",
"Redux",
"Node",
"MongoDB",
];
const arr = [];
const lemon = ["Cá Chép", "Cá Lục", "Cá Rô", "Cá Chắm", "Cá Phi"];
console.log(lemon.length);
console.log(lemon.slice(0, 1));
console.log(lemon.slice(2, 3));
console.log(lemon.slice(4, 5));
const mixedDataTypes = [
"SuperIdol",
241,
true,
{ sudo: ["Liên Minh", "Huyền Thoại"] },
[
["Tiền", "Thuốc"],
[258, 264, 214],
],
];
console.log(mixedDataTypes.length);
const itCompanies = [
"Facebook",
"Google",
"Microsoft",
"Apple",
"IBM",
"Oracle và Amazon",
];
console.log(itCompanies);
console.log(itCompanies.length);
console.log(itCompanies.slice(0, 1));
console.log(itCompanies.slice(3, 4));
console.log(itCompanies.slice(6, 7));
console.log(itCompanies[3]); //123456
console.log(itCompanies.join() + "là những công ty CNTT lớn nhất");
let congty = itCompanies.indexOf("Apple");
if (congty != -1) {
console.log("Công Ty");
} else {
console.log("Công Ty Không Tồn Tại");
}
console.log(itCompanies.sort());
console.log(itCompanies.reverse());
console.log(itCompanies.slice(0, 3));
console.log(itCompanies.slice(2, 5));
console.log(itCompanies.slice(4, 7));
console.log(itCompanies.shift());
console.log(itCompanies.splice(2, 1));
console.log(itCompanies.pop()); |
#include <stdlib.h>
#include "lists.h"
/**
* list_len - Prints the number of elements in a linked list
*
* @h: Head node pointer
*
* Return: Number of nodes
*/
size_t list_len(const list_t *h)
{
size_t num = 0;
while (h)
{
h = h->next;
num++;
}
return (num);
} |
/*
* SPDX-FileCopyrightText: (C) Copyright 2023 Regione Piemonte
*
* SPDX-License-Identifier: EUPL-1.2 */
package it.csi.epay.epaypacatalogsrv.business.dispatcher;
import java.util.HashMap;
import java.util.Map;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.LogManager;
import org.springframework.aop.framework.AopProxyUtils;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.stereotype.Component;
import it.csi.epay.epaypacatalogsrv.dto.ParentInput;
import it.csi.epay.epaypacatalogsrv.dto.ParentOutput;
import it.csi.epay.epaypacatalogsrv.exception.DuplicatedOperationHandlerException;
import it.csi.epay.epaypacatalogsrv.exception.MissingOperationHandlerException;
@Component
public class OperationHandlerRegister implements ApplicationContextAware {
private final Logger logger = LogManager.getLogger ( this.getClass () );
private static ApplicationContext context;
@SuppressWarnings ( "rawtypes" )
private Map<String, OperationHandlerWrapper> operationHandlerCache = new HashMap<> ();
public static ApplicationContext getApplicationContext () {
return OperationHandlerRegister.context;
}
@Override
public void setApplicationContext ( ApplicationContext context ) throws BeansException {
OperationHandlerRegister.context = context;
}
@SuppressWarnings ( { "rawtypes", "unchecked" } )
public OperationHandlerWrapper findOperationHandler ( Class<? extends ParentInput> TI, Class<? extends ParentOutput> TO ) {
String key = "OH" + ( TI.getName () + ";O=" + TO.getName () ).hashCode ();
if ( !operationHandlerCache.containsKey ( key ) ) {
logger.info ( "resolving operation handler for types: \nINPUT = " + TI.getName () + "\nOUTPUT = " + TO.getName () );
Map<String, Object> beans = context.getBeansWithAnnotation ( Operation.class );
OperationHandlerWrapper handler = null;
for ( Object bean: beans.values () ) {
Operation annotation = AopProxyUtils.ultimateTargetClass ( bean ).getAnnotation ( Operation.class );
if ( annotation.consumes () == TI && annotation.produces () == TO ) {
if ( handler == null ) {
handler = new OperationHandlerWrapper ( (OperationHandler) bean, annotation );
} else {
String errorMessage = "OperationHandler non univoco per i tipi INPUT = " + TI.getName () + ", OUTPUT = " + TO.getName ();
logger.error ( errorMessage );
throw new DuplicatedOperationHandlerException ( errorMessage );
}
}
}
if ( handler == null ) {
// prova a cercare per nome
if ( TI.getSimpleName ().endsWith ( "Input" ) && TO.getSimpleName ().endsWith ( "Output" ) ) {
String radiceInput = TI.getSimpleName ().substring ( 0, TI.getSimpleName ().length () - 5 );
String radiceOutput = TO.getSimpleName ().substring ( 0, TO.getSimpleName ().length () - 6 );
if ( radiceInput.equals ( radiceOutput ) ) {
String nomeOperationHandler = radiceInput.substring ( 0, 1 ).toLowerCase () + radiceInput.substring ( 1 ) + "Operation";
logger.debug ( "risolto nome operation temptative da nome classi: " + nomeOperationHandler );
try {
Object bean = context.getBean ( nomeOperationHandler );
logger.debug ( "lookup del bean riuscito: " + bean.getClass ().getName () );
handler = new OperationHandlerWrapper ( (OperationHandler) bean, null );
} catch ( Exception e ) {
logger.debug ( "lookup del bean fallito", e );
}
} else {
logger.warn ( "Attenzione: i nomi delle classi INPUT / OUTPUT non seguono la naming convention corretta: " + TI.getName () + ", "
+ TO.getName () );
}
}
}
if ( handler == null ) {
String errorMessage = "nessun OperationHandler registrato per i tipi INPUT = " + TI.getName () + ", OUTPUT = " + TO.getName ();
logger.error ( errorMessage );
throw new MissingOperationHandlerException ( errorMessage );
}
logger.info ( "resolved operation handler " + handler.getClass ().getName () );
operationHandlerCache.put ( key, handler );
return handler;
} else {
return operationHandlerCache.get ( key );
}
}
public class OperationHandlerWrapper<TI, TO> {
private OperationHandler<TI, TO> handler;
private Operation annotation;
public OperationHandlerWrapper ( OperationHandler<TI, TO> handler, Operation annotation ) {
super ();
this.handler = handler;
this.annotation = annotation;
}
public OperationHandler<TI, TO> getHandler () {
return handler;
}
public Operation getAnnotation () {
return annotation;
}
}
} |
# Prelude Macros
These are the main macros defined by the prelude.
## Definition Forms
### define
``` scheme
(define (id . args) body ...)
=> (define-values (id)
(lambda% args body ...))
(define id expr)
=> (define-values (id) expr)
```
### def
``` scheme
(def (id . args) body ...)
=> (define-values (id)
(lambda args body ...))
(def ((head . rest) . args) body ...)
=> (def (head . rest)
(lambda args body ...))
(def id expr)
=> (define-values (id) expr)
```
Compared with `define`, which defines lambdas with the core Scheme `lambda%` form,
`def` uses the extended `lambda` form, and supports curried definitions.
### def*
``` scheme
(def* id
(args body ....) ...)
=>
(def id
(case-lambda (args body ...) ...))
```
::: tip Examples:
``` scheme
> (def* foo
(() ['no-arg])
((x) ['one-arg x])
(rest ['more-than-one rest]))
> (foo 1)
(one-arg 1)
> (foo 1 2)
(more-than-one (1 2))
```
:::
### defvalues
``` scheme
(defvalues (id ...) expr)
=> (define-values (id ...) expr)
```
### defsyntax
``` scheme
(defsyntax (id . args) body ...)
=> (define-syntax id (lambda args body ...))
(defsyntax id expr)
=> (define-syntax id expr)
```
### defrules
``` scheme
(defrules id (keyword-id ...)
(pat [fender] body) ...)
=> (defsyntax id
(syntax-rules (keyword-id ...)
(pat [fender] body) ...))
```
### defalias
``` scheme
(defalias id alias-id)
=> (define-alias id alias-id)
```
## Binding Forms
### let*-values
``` scheme
(let*-values (((id ...) expr) rest ...) body ...)
=> (let-values (((id) expr))
(let*-values rest body ...))
(let*-values () body ...)
=> (let-values () body ...)
```
### let
``` scheme
(let id ((var expr) ...) body ...)
=> ((letrec (id (lambda% (var ...) body ...))) expr ...)
(let bind body ...)
=> (let-values (bind-values) body ...)
(let (bind ...) body ...)
=> (let-values (bind-values ...) body ...)
<bind>:
((values id ...) expr)
(id expr)
<bind-values>:
(((id ...) expr))
```
### let*
``` scheme
(let* (bind rest ...) body ...)
=> (let bind (let* (rest ...) body ...))
(let* () body ...)
=> (let () body ...)
```
### letrec letrec*
``` scheme
(letrec[*] bind body ...)
=> (letrec[*]-values (bind-values) body ...)
(letrec[*] (bind ...) body ...)
=> (letrec[*]-values (bind-values ...) body ...)
```
### lambda
``` scheme
(lambda (arg ...) body ...)
(lambda (arg ... . id) body)
<arg>:
id ; required argument
(id default) ; optional argument
key: (id default) ; keyword argument
```
The extended lambda form that supports optional and keyword arguments.
### set!
``` scheme
(set! id expr)
(set! setq-macro-id expr)
=> apply setq-macro expander
(set! (setf-macro-id . args) . rest)
=> apply setf-macro expander
(set! (getf-id arg ...) expr)
=> (getf-id-set! arg ... expr)
```
And when you got bindings, you want your mutator too.
As they say, _mostly functional_.
## Common Syntactic Sugar
### and or
``` scheme
(and expr ...)
(or expr ...)
```
### case cond
``` scheme
(cond cond-clause ...)
(case case-clause ...)
```
The well known conditional macros; `case` has its extended form supporting `=>`
dispatch.
### when unless
``` scheme
(when test expr ...)
=> (if test (begin expr ...) #!void)
(unless test expr ...)
=> (if test #!void (begin expr ...))
```
### do do-while
``` scheme
(do ((var init step ...) ...)
(test fini ...)
body ...)
(do-while hd (test fini ...) body ...)
=> (do hd ((not test) fini ...) body ...)
```
The common iteration macro and its inverted form.
### begin0
``` scheme
(begin0 expr rest ...)
=> (let (val expr) rest ... val)
```
### rec
``` scheme
(rec id expr)
=> (letrec ((id expr)) id)
(rec (values id ...) expr)
=> (letrec ((values id ...) expr) (values id ...))
(rec (id . args) body ...)
=> (letrec (id (lambda args body ...)) id)
```
Short recursive definition form.
### alet alet* and-let*
``` scheme
(alet bind body ...)
(alet (bind ...) body ...)
(alet* bind body ...)
(alet* (bind ...) body ...)
(defalias and-let* alet*)
```
Anaphoric lets which short circuit to `#f` if any of the bindings is `#f`.
::: tip Examples:
``` scheme
> (alet ((a [1 2])
(b #f))
[a b])
#f
> (alet ((a [1 2])
(b #t))
[a b])
((1 2) #t)
```
:::
### @list
``` scheme
(@list)
=> '()
(@list :: tl)
=> tl
(@list xs ellipsis)
=> xs
(@list xs ellipsis . rest)
=> (foldr cons (@list . rest) xs)
(@list x . xs)
=> (cons x (@list . xs)))
(@list . tl)
=> tl
```
This is the reader macro for `[...]`.
### quasiquote
``` scheme
(quasiquote expr)
```
### delay
``` scheme
(delay expr)
```
The promise to eval `expr`.
### cut
``` scheme
(cut arg ...)
```
if you don't know how this works, stop and read the [SRFI](https://srfi.schemers.org/srfi-26/srfi-26.html).
Most useful little macro ever.
::: tip Examples:
``` scheme
> (filter (cut < <> 10) [1 10 2 20])
(1 2)
> (def fn (cut list 'a 'b))
> (fn)
(a b)
```
:::
### parameterize
``` scheme
(parameterize ((paremter-id expr) ...) body ...)
```
::: tip Examples:
``` scheme
> (def foo (make-parameter #f))
> (foo)
#f
> (parameterize ((foo 100))
(foo))
100
```
:::
### let/cc let/esc
``` scheme
(let/cc id body ...)
=> (call/cc (lambda (id) body ...))
(let/esc id body ...)
=> (call/esc (lambda (id) body ...))
```
`call/esc` is really the same thing as `call/cc` in Gerbil on Gambit.
### unwind-protect
``` scheme
(unwind-protect body postlude)
```
### @bytes
```scheme
(@bytes "...")
=> (quote #u8(...))
```
Converts a utf8 encoded string to a u8vector at expansion time.
### syntax-error
``` scheme
(syntax-error message detail ...)
```
Raises a syntax error; used for meaningful error reporting in syntax-rules macros.
## Runtime Aliases
### car-set! cdr-set!
```scheme
(defalias car-set! set-car!)
(defalias cdr-set! set-cdr!)
```
### string-ref-set!
```scheme
(defalias string-ref-set! string-set!)
```
### *vector-ref-set!
```scheme
(defalias vector-ref-set! vector-set!)
(defalias s8vector-ref-set! s8vector-set!)
(defalias u8vector-ref-set! u8vector-set!)
(defalias s16vector-ref-set! s16vector-set!)
(defalias u16vector-ref-set! u16vector-set!)
(defalias s32vector-ref-set! s32vector-set!)
(defalias u32vector-ref-set! u32vector-set!)
(defalias s64vector-ref-set! s64vector-set!)
(defalias u64vector-ref-set! u64vector-set!)
(defalias f32vector-ref-set! f32vector-set!)
(defalias f64vector-ref-set! f64vector-set!)
```
These binding enable you to use `set!` with `*vector-ref`.
::: tip Examples
```scheme
> (def foo (vector 1 2 3))
> (set! (vector-ref foo 1) 4)
> foo
#(1 4 3)
```
:::
### call/values call/parameters
```scheme
(defalias call/values call-with-values)
(defalias call/parameters call-with-parameters)
```
### randeom-bytes random-source-make-bytes
```scheme
(defalias random-bytes random-u8vector)
(defalias random-source-make-bytes random-source-make-u8vectors))
```
## MOP Macros
### defclass-type defstruct-type
``` scheme
(defclass-type id super make instance? type-body ...)
(defstruct-type id super make instance? type-body ...)
<type-body>:
name: id ; type name
id: id ; type id
constructor: method-id ; constructor method id
properties: expr ; additional type properties
slots: ((id getf setf) ...) ; class slots
mixin: ((id getf setf) ...) ; mixin class slots
struct: <boolean> ; #t for structs
final: <boolean> ; final class?
metaclass: id ; metaclass type
```
Low level class type definition facilities.
### defclass defstruct define-class define-struct
``` scheme
(defclass id (slot ...) typedef-option ...)
(defclass (id super ...) (slot ...) typedef-option ...)
(defstruct id (slot ...) typedef-option ...)
(defstruct (id super ...) (slot ...) typedef-option ...)
(defalias define-class defclass)
(defalias define-struct defstruct)
<typedef-option>:
name: id ; type name
id: id ; type id
constructor: id ; constructor method id
final: <boolean> ; #t for final types
struct: <boolean> ; #t for structs
transparent: <boolean> ; #t for equality checks and printability
equal: <boolean> or <list> ; a list of slots to consider for equal? checks, #t for all fields
print: <boolean> or <list> ; a list of slots to print, #t for all fields
metaclass: id ; metaclass type
```
Canonical class type definition macro.
### defmethod
``` scheme
(defmethod {method-id type}
expr
[rebind: <boolean>])
```
Defines a method `method-id` for type `type`, which must be
a class type.
The `:std/generic` library extends the form for generic methods.
### @method
``` scheme
{obj.id arg ...}
{id obj arg ...}
(@method id obj arg ...)
=> (call-method obj 'id arg ...)
```
This is the reader macro for `{...}`, the method invocation operator.
### @ (slot reference)
``` scheme
(@ obj id)
=> (slot-ref obj 'id)
(@ obj id rest ...)
=> (@ (@ obj id) rest ...)
```
Dynamic slot reference macro.
### @-set!
``` scheme
(set! (@ obj id ...) val)
=> (@-set! obj id ... val)
(@-set! obj id val)
=> (slot-set! obj 'id val)
(@-set! obj id rest ... last val)
=> (@-set! (@ obj id rest ...) last val)
```
Dynamic slot mutation macro.
### System Classes
#### :t
```scheme
(defsystem-class-info :t t::t () true)
```
#### :object
```scheme
(defsystem-class-info :object object::t (t::t) true)
```
#### :immediate
```scheme
(defsystem-class-info :immediate immediate::t (t::t) immediate?)
```
#### :boolean
```scheme
(defsystem-class-info char::t (immediate::t) char?)
```
#### :boolean
```scheme
(defsystem-class-info :boolean boolean::t (immediate::t) boolean?)
```
#### :atom
```scheme
(defsystem-class-info :atom atom::t (immediate::t) atom?)
```
#### :void
```scheme
(defsystem-class-info :void void::t (atom::t) void?)
```
#### :eof
```scheme
(defsystem-class-info :eof eof::t (atom::t) eof-object?)
```
#### :true
```scheme
(defsystem-class-info :true true::t (boolean::t atom::t) true?)
```
#### :false
```scheme
(defsystem-class-info :false false::t (boolean::t atom::t) not)
```
#### :special
```scheme
(defsystem-class-info :special special::t (atom::t) special?)
```
#### :number
```scheme
(defsystem-class-info :number number::t (t::t) number?)
```
#### :real
```scheme
(defsystem-class-info :real real::t (number::t) real?)
```
#### :integer
```scheme
(defsystem-class-info :integer integer::t (real::t) integer?)
```
#### :fixnum
```scheme
(defsystem-class-info :fixnum fixnum::t (integer::t immediate::t) fixnum?)
```
#### :bignum
```scheme
(defsystem-class-info :bignum bignum::t (integer::t) ##bignum?)
```
#### :ratnum
```scheme
(defsystem-class-info :ratnum ratnum::t (real::t) ##ratnum?)
```
#### :flonum
```scheme
(defsystem-class-info :flonum flonum::t (real::t) flonum?)
```
#### :cpxnum
```scheme
(defsystem-class-info :cpxnum cpxnum::t (number::t) ##cpxnum?)
```
#### :symbolic
```scheme
(defsystem-class-info :symbolic symbolic::t (t::t) symbolic?)
```
#### :symbol
```scheme
(defsystem-class-info :symbol symbol::t (symbolic::t) symbol?)
```
#### :keyword
```scheme
(defsystem-class-info :keyword keyword::t (symbolic::t) keyword?)
```
#### :list
```scheme
(defsystem-class-info :list list::t (t::t) list?)
```
#### :pair
```scheme
(defsystem-class-info :pair pair::t (list::t) pair?)
```
#### :null
```scheme
(defsystem-class-info :null null::t (list::t atom::t) null?)
```
#### :sequence
```scheme
(defsystem-class-info :sequence sequence::t (t::t) sequence?)
```
#### :vector
```scheme
(defsystem-class-info :vector vector::t (sequence::t) vector?)
```
#### :string
```scheme
(defsystem-class-info :string string::t (sequence::t) string?)
```
#### :hvector
```scheme
(defsystem-class-info :hvector hvector::t (sequence::t) hvector?)
```
#### :u8vector
```scheme
(defsystem-class-info :u8vector u8vector::t (hvector::t) u8vector?)
```
#### :s8vector
```scheme
(defsystem-class-info :s8vector s8vector::t (hvector::t) s8vector?)
```
#### :u16vector
```scheme
(defsystem-class-info :u16vector u16vector::t (hvector::t) u16vector?)
```
#### :s16vector
```scheme
(defsystem-class-info :s16vector s16vector::t (hvector::t) s16vector?)
```
#### :u32vector
```scheme
(defsystem-class-info :u32vector u32vector::t (hvector::t) u32vector?)
```
#### :s32vector
```scheme
(defsystem-class-info :s32vector s32vector::t (hvector::t) s32vector?)
```
#### :u64vector
```scheme
(defsystem-class-info :u64vector u64vector::t (hvector::t) u64vector?)
```
#### :s64vector
```scheme
(defsystem-class-info :s64vector s64vector::t (hvector::t) s64vector?)
```
#### :f32vector
```scheme
(defsystem-class-info :f32vector f32vector::t (hvector::t) f32vector?)
```
#### :f64vector
```scheme
(defsystem-class-info :f64vector f64vector::t (hvector::t) f64vector?)
```
#### :values
```scheme
(defsystem-class-info :values values::t (t::t) ##values?)
```
#### :box
```scheme
(defsystem-class-info :box box::t (t::t) box?)
```
#### :frame
```scheme
(defsystem-class-info :frame frame::t (t::t) ##frame?)
```
#### :continuation
```scheme
(defsystem-class-info :continuation continuation::t (t::t) continuation?)
```
#### :promise
```scheme
(defsystem-class-info :promise promise::t (t::t) promise?)
```
#### :weak
```scheme
(defsystem-class-info :weak weak::t (t::t) weak?)
```
#### :foreign
```scheme
(defsystem-class-info :foreign foreign::t (t::t) foreign?)
```
#### :procedure
```scheme
(defsystem-class-info :procedure procedure::t (t::t) procedure?)
```
#### :time
```scheme
(defsystem-class-info :time time::t (t::t) time?)
```
#### :thread
```scheme
(defsystem-class-info :thread thread::t (t::t) thread?)
```
#### :thread-group
```scheme
(defsystem-class-info :thread-group thread-group::t (t::t) thread-group?)
```
#### :mutex
```scheme
(defsystem-class-info :mutex mutex::t (t::t) mutex?)
```
#### :condvar
```scheme
(defsystem-class-info :condvar condvar::t (t::t) condvar?)
```
#### :port
```scheme
(defsystem-class-info :port port::t (t::t) port?)
```
#### :object-port
```scheme
(defsystem-class-info :object-port object-port::t (port::t) object-port?)
```
#### :character-port
```scheme
(defsystem-class-info :character-port character-port::t (object-port::t) character-port?)
```
#### :byte-port
```scheme
(defsystem-class-info :byte-port byte-port::t (character-port::t) byte-port?)
```
#### :device-port
```scheme
(defsystem-class-info :device-port device-port::t (byte-port::t) device-port?)
```
#### :vector-port
```scheme
(defsystem-class-info :vector-port vector-port::t (object-port::t) vector-port?)
```
#### :string-port
```scheme
(defsystem-class-info :string-port string-port::t (character-port::t) string-port?)
```
#### :u8vector-port
```scheme
(defsystem-class-info :u8vector-port u8vector-port::t (byte-port::t) u8vector-port?)
```
#### :raw-device-port
```scheme
(defsystem-class-info :raw-device-port raw-device-port::t (port::t) raw-device-port?)
```
#### :tcp-server-port
```scheme
(defsystem-class-info :tcp-server-port tcp-server-port::t (object-port::t) tcp-server-port?)
```
#### :udp-port
```scheme
(defsystem-class-info :udp-port udp-port::t (object-port::t) udp-port?)
```
#### :directory-port
```scheme
(defsystem-class-info :directory-port directory-port::t (object-port::t) directory-port?)
```
#### :event-queue-port
```scheme
(defsystem-class-info :event-queue-port event-queue-port::t (object-port::t) event-queue-port?)
```
#### :table
```scheme
(defsystem-class-info :table table::t (t::t) table?)
```
#### :readenv
```scheme
(defsystem-class-info :readenv readenv::t (t::t) readenv?)
```
#### :writeenv
```scheme
(defsystem-class-info :writeenv writeenv::t (t::t) writeenv?)
```
#### :readtable
```scheme
(defsystem-class-info :readtable readtable::t (t::t) readtable?)
```
#### :processor
```scheme
(defsystem-class-info :processor processor::t (t::t) processor?)
```
#### :vm
```scheme
(defsystem-class-info :vm vm::t (t::t) vm?)
```
#### :file-info
```scheme
(defsystem-class-info :file-info file-info::t (t::t) file-info?)
```
#### :socket-info
```scheme
(defsystem-class-info :socket-info socket-info::t (t::t) socket-info?)
```
#### :address-info
```scheme
(defsystem-class-info :address-info address-info::t (t::t) address-info?)
```
## Pattern Matching
### match
``` scheme
(match expr
(pattern body ...) ...
[(else body ...)])
<pattern>:
(? test) ; predicate test with the `?` predicate constructor
(? test pattern) ; test and match a pattern
(? test => pattern) ; test and match a pattern on the value of the test
(? test :: proc => pattern) ; test and match with a filter
(and pattern ...) ; match all patterns
(or pattern ...) ; match any pattern
(not pattern) ; negated match
(cons pattern1 pattern2) ; destructure a pair like cons
(cons* pattern ... pattern-tail) ; destructure a list like cons*
[pattern ...] ;
(@list pattern ...) ; destructure a list like @list
(box pattern) ;
#&pattern ; destructure a box
(values pattern ...) ; destructure a values tuple
(vector pattern ...) ;
#(pattern ...) ; destructure a vector
(struct-id pattern ...) ; destructure a struct
(class-id (slot pattern) ...) ; destructure a class
(eq? val) ; match eq? to val
(eqv? val) ; match eqv? to val
(equal? val) ; match equal? to val
(quote expr) ; match eq?/eqv?/equal? to a quoted value
(quasiquote datum) ; destructure with quasiquote
(apply getf pattern) ; applicative destructuring
(match-macro arg ...) ; apply match macro expander
_ ; match any and ignore
id ; match any and bind to id
datum ; match eq?/eqv?/equal? to a datum
(match <> (match-pattern body ...) ...)
=> (lambda (obj) (match obj (match-pattern body ...) ...))
(match <...> (match-pattern body ...) ...)
=> (lambda args (match args (match-pattern body ...) ...))
```
The fundamental destructuring pattern match macro; you've seen many and this one is
very much like them.
::: tip Examples:
``` scheme
> (def lst [1 2 3])
> (match lst
([0 1 c] "starts with 0 1")
([a _ c] (list c a))
(_ #f))
(3 1)
> (let loop ((rest [1 2]))
(match rest
([v . rest] (displayln v) (loop rest))
([] (displayln 'end))))
1
2
end
> (def foo (match <>
((? (and number? inexact?)) 'inexact)
((? number?) 'number)
((and (? string?) (not "")) 'string)
(_ 'other)))
> (foo 10)
number
> (foo 2.0)
inexact
> (foo "")
other
> (foo "bar")
string
```
:::
### match*
``` scheme
(match* (expr ...)
((pattern ...) body) ...)
```
Matches multiple objects in sequence.
::: tip Examples:
``` scheme
> (match* (#t [1 2])
((#f [a 0]) 'a)
((#t [a]) 'b)
((#t [_ b]) 'c)
(else 'd))
c
```
:::
### with with*
``` scheme
(with (pattern expr) body ...)
=> (match expr (pattern body ...))
(with ((pattern expr) ...) body ...)
=> (match* (expr ...) ((pattern ...) body ...))
(with () body ...)
=> (let () body ...)
(with* (hd rest ...) body ...)
=> (with hd (with* (rest ...) body ...))
(with* () body ...)
=> (let () body ...)
```
Short-form destructuring bind macros.
::: tip Examples:
``` scheme
> (with ([a b . c] (iota 4))
(list a b c))
(0 1 (2 3))
```
:::
### ? (predicate constructor)
``` scheme
(? (and pred ...) obj)
=> (and (? pred obj) ...)
(? (or pred ...) obj)
=> (or (? pred obj) ...)
(? (not pred) obj)
=> (not (? pred obj))
(? pred obj)
=> (pred obj)
(? pred)
=> (lambda (obj) (? pred obj))
(? pred => K)
=> (lambda (obj)
(alet (val (? pred obj))
(K val)))
(? pred :: proc)
=> (lambda (obj)
(and (? pred obj)
(proc obj)))
(? pred :: proc => K)
=> (lambda (obj)
(and (? pred obj)
(K (proc obj))))
```
The predicate constructor macro.
::: tip Examples:
``` scheme
> (? (and number? fixnum?) 1000)
#t
> (? (and number? fixnum?))
#<procedure #1>
```
:::
### defsyntax-for-match
``` scheme
(defsyntax-for-match id match-macro-expr [macro-expr])
```
Defines a match macro expander with name `id`, with optionally a regular expander for the
same identifier.
## Macros for Syntax
The following macros are only available for syntax (phi = 1).
### syntax-case syntax syntax/loc
``` scheme
(syntax-case stx (keyword-id ...)
(pat [fender] body) ...)
(syntax expr)
(syntax/loc src-stx expr)
```
The well-known `syntax` and `syntax-case` macros, first defined in "Extending the Scope
of Syntactic Abstraction" by Waddell and Dybvig and popularized by Racket.
`syntax/loc` is like syntax, only it assigns the source location to that of `src-stx`
### syntax-rules
``` scheme
(syntax-rules (keyword-id ...)
(pat [fender] expr) ...)
```
The familiar `syntax-rules` macro from R5RS, extended with pattern fenders like `syntax-case`
and meaningful underscores.
### with-syntax with-syntax*
``` scheme
(with-syntax ((pat expr) ...) body)
(with-syntax* ((bind expr) ...) body)
<bind>:
(values id ...) ; value binding
pat ; syntax binding
```
The common `with-syntax` macro is widely used in Racket.
Its sequence form `with-syntax*` is like a sequence of `with-syntax`, with the Gerbilic
allowance for value bindings with `let*` semantics.
### identifier-rules
``` scheme
(identifier-rules (keyword-id)
(pat [fender] expr) ...)
```
Variant of `syntax-rules` that constructs a setq macro and not a plain macro expander.
## Module Sugar
### require
``` scheme
(require feature ...)
```
Fails with a syntax error if the `cond-expand` features `feature ...` are not satisfied.
### defsyntax-for-import defsyntax-for-export defsyntax-for-import-export
``` scheme
(defsyntax-for-import id expr)
(defsyntax-for-import (id . args) body ...)
=> (defsyntax-for-import id (lambda args body ...))
(defsyntax-for-export id expr)
(defsyntax-for-export (id . args) body ...)
=> (defsyntax-for-export id (lambda args body ...))
(defsyntax-for-import-export id expr)
(defsyntax-for-import-export (id . args) body ...)
=> (defsyntax-for-import-export id (lambda args body ...))
```
Define import and export macro expanders.
### for-syntax for-template
``` scheme
(import (for-syntax import-spec ...))
(export (for-syntax export-spec ...))
(import (for-template import-spec ...))
(export (for-template export-spec ...))
```
Import/export expanders that switch the phase of the import/export;
`for-syntax` switches by +1, `for-template` switches by -1, just like
the phi: directive.
### only-in
``` scheme
(import (only-in import-spec id ...))
```
Import expander; only import identifiers `id ...` from a set.
### except-in except-out
``` scheme
(import (except-in import-spec id ...))
(export (except-out export-spec id ...))
```
Import and export expander; filter identifiers `id ...` from a set.
### rename-in rename-out
``` scheme
(import (rename-in import-spec (id new-id) ...))
(export (rename-out export-spec (id new-id) ...))
```
Import and export expander; rename specific identifiers in a set.
### prefix-in prefix-out
``` scheme
(import (prefix-in import-spec prefix-id))
(export (prefix-out export-spec prefix-id))
```
Import and export expander; rename a set by applying a prefix.
### struct-out
``` scheme
(export (struct-out struct-id ...))
```
Export expander; export all identifiers related with structs `struct-id ...`
### group-in
``` scheme
(import (group-in prefix mod ...))
mod := id
| (id mod ...)
```
Imports a group of common prefix library modules.
Examples:
```
(import (group-in :std/misc queue rbtree))
= (import :std/misc/queue :std/misc/rbtree)
(import (group-in :std (misc queue rbtree) (net bio)))
= (import :std/misc/queue :std/misc/rbtree :std/net/bio)
(import (group-in :std sugar (srfi 1 113 133)))
= (import :std/sugar :std/srfi/1 :std/srfi/113 :std/srfi/133)
```
## Special Evaluation Forms
### eval-when-compile
``` scheme
(eval-when-compile expr)
```
Evaluates `expr` when expanding in compiler context. |
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './Component/App.jsx'
import { createBrowserRouter, RouterProvider } from "react-router-dom";
import HomePage from './Component/HomePage.jsx'
import Shop from './Component/Shop.jsx'
import Cart from './Component/Cart.jsx';
const Router = () => {
const router = createBrowserRouter([
{
path: "/",
element: <App />,
children: [
{ index: true, element: <HomePage /> },
{ path: "shop/:name", element: <Shop /> },
{ path: "shop/", element: <Shop /> },
{ path: "cart/", element: <Cart /> },
]
}
]);
return <RouterProvider router={router} />
}
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<Router />
</React.StrictMode>
) |
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
import java.util.ArrayList;
import java.util.Random;
/**
*
* @author Thomas
*/
public class JokeManager {
private ArrayList<String> jokesList;
public JokeManager(){
this.jokesList = new ArrayList<>();
}
public void addJoke(String joke){
this.jokesList.add(joke);
}
public String drawJoke(){
Random randomDraw = new Random();
if(jokesList.isEmpty()){
return "Jokes are in short supply.";
} else {
int index = randomDraw.nextInt(jokesList.size());
return jokesList.get(index);
}
}
public void printJokes(){
for(String joke:jokesList){
System.out.println(joke);
}
}
} |
<template>
<v-container fluid pb-0>
<!-- <pre>{{ itemModel }}</pre> -->
<!-- <pre>{{ localItem }}</pre> -->
<v-row
v-for="(model, index) in itemModel"
:key="`rowModel-${index}`"
dense
class="align-center mb-2"
>
<v-col cols="3" class="text-right pr-3">
<span>
{{ model.text }} :
</span>
</v-col>
<v-col cols="6">
<!-- <pre>{{ model }}</pre> -->
<v-text-field
v-if="model.field_type === 'str'"
filled
hide-details="auto"
clearable
v-model="localItem[modelKey(model)]"
dense
@input="updateItemDebounced()"
/>
<v-textarea
v-else-if="model.field_type === 'longStr'"
filled
rows="3"
hide-details="auto"
v-model="localItem[modelKey(model)]"
dense
@input="updateItemDebounced()"
/>
<v-textarea
v-else-if="model.field_type === 'json'"
filled
rows="3"
hide-details="auto"
v-model="localItem[modelKey(model)]"
dense
@input="updateItemDebounced()"
/>
<v-text-field
v-else
filled
hide-details="auto"
clearable
v-model="localItem[modelKey(model)]"
dense
@input="updateItemDebounced()"
/>
</v-col>
<v-col cols="3" class="text-left">
<v-btn
small
plain
color="grey darken-2"
class="px-0"
>
<v-icon
x-small
>
{{ fieldIcon(model.field_type) }}
</v-icon>
</v-btn>
<span class="text-caption">
{{ $t(fieldText(model.field_type)) }}
</span>
</v-col>
</v-row>
</v-container>
</template>
<script>
import { mapState, mapGetters } from 'vuex'
import { FindFieldIcon, FindFieldText } from '@/utils/utilsFields'
export default {
name: 'ModalFields',
props: [
'item',
'itemModel',
'itemType',
'apiUrl',
'action',
'updateCurrentDataset',
'onlyLocalUpdate',
],
data () {
return {
localItem: undefined,
dialog: false,
tab: null,
}
},
watch: {
item (next) {
this.localItem = this.item
}
},
computed: {
...mapState({
log: (state) => state.log,
}),
...mapGetters({
getUserWorkspaceById: 'workspaces/getUserItemById',
headerUser: 'user/headerUser'
}),
},
beforeMount () {
// this.log && console.log('C-ModalFields > beforeMount > this.apiUrl :' , this.apiUrl)
// this.log && console.log('C-ModalFields > beforeMount > this.item :' , this.item)
this.localItem = this.item
// this.log && console.log('C-ModalFields > beforeMount > this.localItem :' , this.localItem)
},
methods: {
fieldIcon (type) {
return FindFieldIcon(type)
},
fieldText(type) {
return FindFieldText(type)
},
modelKey(model) {
const key = this.onlyLocalUpdate ? model.value : model.field_code
return key
},
updateItemDebounced() {
// cancel pending call
clearTimeout(this._timerId)
// delay new call 500ms
this._timerId = setTimeout(() => {
this.updateItem()
}, 500)
},
updateItem() {
if (this.action === 'update'){
// this.log && console.log('C-ModalFields > updateItem > this.apiUrl :' , this.apiUrl)
let itemPayload = this.localItem
// this.log && console.log('C-ModalFields > updateItem > itemPayload :' , itemPayload)
// this.log && console.log('C-ModalFields > updateItem > this.itemModel :' , this.itemModel)
// don't forget ux if update workspace
if (this.itemType === 'workspaces') {
let currentWs = this.getUserWorkspaceById(this.item.id)
itemPayload.datasets = currentWs.datasets
}
if (!this.onlyLocalUpdate) {
this.$axios
.put(`${this.apiUrl}/${this.item.id}`, itemPayload, this.headerUser)
.then(resp => {
// this.log && console.log('C-ModalFields > updateItem > resp.data : ', resp.data)
this.$store.dispatch(`${this.itemType}/updateUserItem`, {data: resp.data, temp: this.onlyLocalUpdate})
if (this.updateCurrentDataset) {
this.$store.dispatch(`${this.itemType}/setCurrentItem`, resp.data)
}
})
}
}
},
}
}
</script> |
from scipy.spatial import Delaunay
import numpy as np
from pprint import pprint
def alpha_shape(points, alpha, only_outer=True):
"""
Compute the alpha shape (concave hull) of a set of points.
:param points: np.array of shape (n,2) points.
:param alpha: alpha value.
:param only_outer: boolean value to specify if we keep only the outer border
or also inner edges.
:return: set of (i,j) pairs representing edges of the alpha-shape. (i,j) are
the indices in the points array.
"""
assert points.shape[0] > 3, "Need at least four points"
def add_edge(edges, i, j):
"""
Add a line between the i-th and j-th points,
if not in the list already
"""
if (i, j) in edges or (j, i) in edges:
# already added
assert (j, i) in edges, "Can't go twice over same directed edge right?"
if only_outer:
# if both neighboring triangles are in shape, it is not a boundary edge
edges.remove((j, i))
return
edges.add((i, j))
tri = Delaunay(points)
edges = set()
# Loop over triangles:
# ia, ib, ic = indices of corner points of the triangle
for ia, ib, ic in tri.simplices:
pa = points[ia]
pb = points[ib]
pc = points[ic]
# Computing radius of triangle circumcircle
# www.mathalino.com/reviewer/derivation-of-formulas/derivation-of-formula-for-radius-of-circumcircle
a = np.sqrt((pa[0] - pb[0]) ** 2 + (pa[1] - pb[1]) ** 2)
b = np.sqrt((pb[0] - pc[0]) ** 2 + (pb[1] - pc[1]) ** 2)
c = np.sqrt((pc[0] - pa[0]) ** 2 + (pc[1] - pa[1]) ** 2)
s = (a + b + c) / 2.0
area = np.sqrt(s * (s - a) * (s - b) * (s - c))
circum_r = a * b * c / (4.0 * area)
if circum_r < alpha:
add_edge(edges, ia, ib)
add_edge(edges, ib, ic)
add_edge(edges, ic, ia)
return edges
if __name__ == "__main__":
from matplotlib.pyplot import *
# Constructing the input point data
np.random.seed(0)
x = 3.0 * np.random.rand(2000)
y = 2.0 * np.random.rand(2000) - 1.0
inside = ((x ** 2 + y ** 2 > 1.0) & ((x - 3) ** 2 + y ** 2 > 1.0) & ((x - 1.5) ** 2 + y ** 2 > 0.09))
points = np.vstack([x[inside], y[inside]]).T
print("points.shape: {0}".format(points.shape))
# Computing the alpha shape
edges = alpha_shape(points, alpha=0.25, only_outer=True)
pprint(edges)
# Plotting the output
figure()
axis('equal')
plot(points[:, 0], points[:, 1], '.')
for i, j in edges:
plot(points[[i, j], 0], points[[i, j], 1])
show() |
import React from 'react'
function Cart(props) {
return (
<>
{props.counter <= 0 ? (
<div className='fixed top-28 z-[30] ml-5 w-[90%] rounded-md bg-neutral-white text-sm shadow-2xl mobile:top-32 md:top-[6.3rem] md:right-8 md:w-[54%] lg:w-[35%] desktop:w-[25%]'>
<h3 className='p-5 font-bold text-neutral-very_dark-blue'>Cart</h3>
<hr />
<div className='flex items-center justify-center py-14 mobile:py-20'>
<p className='font-bold text-neutral-dark_grayish-blue'>
Your cart is empty.
</p>
</div>
</div>
) : (
<div className='fixed top-28 z-[30] ml-5 w-[90%] rounded-md bg-neutral-white text-sm shadow-2xl mobile:top-32 md:top-[6.3rem] md:right-8 md:w-[54%] lg:w-[35%] desktop:w-[25%]'>
<h3 className='p-5 font-bold text-neutral-very_dark-blue'>Cart</h3>
<hr />
<div className='px-5 pb-5'>
<div className='flex items-center justify-around gap-5 py-7 '>
<img
alt='Product Image'
className='w-14 rounded-lg'
src='/images/image-product-1-thumbnail.jpg'
/>
<div className='flex flex-col gap-1'>
<p className='font-semibold text-neutral-dark_grayish-blue'>
Autumn Limited Edition...
</p>
<p className='font-bold text-neutral-dark_grayish-blue'>
$125.00 x {props.counter}{' '}
<span className='font-bold text-neutral-black'>
${(props.counter * 125.0).toFixed(2)}
</span>
</p>
</div>
<img alt='Delete Icon' src='/images/icon-delete.svg' />
</div>
<div className='flex cursor-pointer justify-center rounded-lg bg-primary-orange p-4 font-semibold shadow-primary-orange'>
<button className='font-bold text-neutral-white'>Checkout</button>
</div>
</div>
</div>
)}
</>
)
}
export default Cart |
<template>
<form @submit.prevent="handleSubmit">
<error-message :errors="errors"/>
<div class="form-floating mb-3">
<input-with-validation
:id="'email-validation'"
:name="'Электронная почта'"
:errors="errors"
:value="'email'"
v-model="formData.email"
/>
</div>
<div class="form-floating mb-3">
<input-with-validation
:id="'password-validation'"
:type="'password'"
:name="'Пароль'"
:errors="errors"
:value="'password'"
v-model="formData.password"
/>
</div>
<button class="w-100 btn btn-primary btn-block mb-4" type="submit">
<div v-if="isLoading" class="spinner-grow spinner-grow-sm" role="status">
<span class="visually-hidden">Loading...</span>
</div>
<template v-else>Войти</template>
</button>
<template v-if="isPasswordIncorrect">
<div class="text-center">
<p><router-link :to="{ name: 'AuthUserResetPasswordRequest' }">Восстановить доступ</router-link></p>
</div>
</template>
<div class="text-center">
<p>Не зарегистрированы? <router-link :to="{ name: 'AuthRegistrationRequest' }">Регистрация</router-link></p>
</div>
</form>
</template>
<script lang="ts">
import { defineComponent, ref, reactive } from 'vue'
import { useRouter } from 'vue-router'
import api from '../../../api'
import { LoginDto } from '@/models/auth'
import {Error, ErrorCode} from '@/models/error'
import InputWithValidation from '../../../components/UI/blocks/input-with-validation.vue'
import ErrorMessage from '../../../components/UI/message/error-message.vue'
export default defineComponent({
components: {
InputWithValidation,
ErrorMessage
},
setup () {
const router = useRouter()
const isLoading = ref(false)
const formData = reactive<LoginDto>({})
const errors = ref<Error[]>([])
const errorMessage = ref('')
const isPasswordIncorrect = ref(false)
const handleSubmit = async () => {
isLoading.value = true
errors.value = []
errorMessage.value = ''
isPasswordIncorrect.value = false
try {
const response = await api.post('v1/auth/login/request', formData)
const accessToken = response['accessToken']
localStorage.setItem('accessToken', accessToken)
await router.push({ name: 'AppIndex' })
isLoading.value = false
} catch (e: any) {
isLoading.value = false
if (e instanceof Response) {
const result = await e.json()
errors.value = result.errors
if (errors.value[0].code === ErrorCode.PasswordIncorrect) {
isPasswordIncorrect.value = true
}
} else {
errors.value = [{ message: 'Неизвестная ошибка' }]
}
}
}
return {
isLoading,
formData,
errors,
errorMessage,
isPasswordIncorrect,
handleSubmit
}
}
});
</script> |
class Solution {
/* Kadane's algorithm
Basically a DP to find the max subarray sum including i
when adding nums[i], and if the sum becomes negative, because subarray is
contiguous, number before and including i just can't be part of the max
subarry so we can basically the sum seen so far and see if can find a bigger
sum later
*/
public:
int maxSubArray(vector<int>& nums) {
int sum = nums[0];
int maxSum = sum;
for (int i = 1; i < nums.size(); ++i) {
if (sum < 0) sum = 0;
sum += nums[i];
maxSum = std::max(maxSum, sum);
}
return maxSum;
}
}; |
import {
Form,
FormControl,
FormField,
FormItem,
FormLabel,
} from "@/components/ui/form";
import { useDebouncedCallback } from "use-debounce";
import { Input } from "@/components/ui/input";
import { zodResolver } from "@hookform/resolvers/zod";
import { useForm } from "react-hook-form";
import { z } from "zod";
import { useUpdateCurrentUserProfile } from "./use-update-current-user-profile";
const playerProfileSchema = z.object({
username: z.string().min(1),
});
export const ProfileForm = ({
defaultValues,
}: {
defaultValues: z.infer<typeof playerProfileSchema>;
}) => {
const updateCurrentUserProfile = useUpdateCurrentUserProfile();
const form = useForm<z.infer<typeof playerProfileSchema>>({
defaultValues,
resolver: zodResolver(playerProfileSchema),
});
const updateProfile = useDebouncedCallback(() => {
const profile = form.getValues();
return updateCurrentUserProfile.mutate(profile);
}, 500);
return (
<Form {...form}>
<form onChange={updateProfile}>
<FormField
control={form.control}
name="username"
render={({ field }) => (
<FormItem>
<FormLabel className="sr-only">Username</FormLabel>
<FormControl>
<Input placeholder={defaultValues.username} {...field} />
</FormControl>
</FormItem>
)}
/>
</form>
</Form>
);
}; |
// Copyright © Blockchain Luxembourg S.A. All rights reserved.
import RxCocoa
import RxSwift
import UIKit
final class DigitPadButtonView: UIView {
// MARK: - UI Properties
@IBOutlet private var backgroundView: UIView!
@IBOutlet private var button: UIButton!
// MARK: - Rx
private let disposeBag = DisposeBag()
// MARK: - Injected
var viewModel: DigitPadButtonViewModel! {
didSet {
backgroundView.layer.cornerRadius = viewModel.background.cornerRadius
switch viewModel.content {
case .image(type: let image, tint: let color):
button.setImage(image.image, for: .normal)
button.imageView?.tintColor = color
case .label(text: let value, tint: let color):
button.setTitle(value, for: .normal)
button.setTitleColor(color, for: .normal)
case .none:
break
}
button.accessibility = viewModel.content.accessibility
// Bind button taps to the view model tap relay
button.rx.tap
.bindAndCatch(weak: self) { (self) in
self.viewModel.tap()
}
.disposed(by: disposeBag)
}
}
// MARK: - Setup
override init(frame: CGRect) {
super.init(frame: frame)
setup()
}
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
setup()
}
private func setup() {
fromNib(in: .module)
button.titleLabel?.font = .main(.medium, 32)
backgroundView.clipsToBounds = true
backgroundColor = .clear
}
// MARK: - Button touches
@IBAction private func touchDown() {
backgroundView.backgroundColor = viewModel.background.highlightColor
}
@IBAction private func touchUp() {
backgroundView.backgroundColor = .clear
}
} |
import React from 'react'
import { Formik, Form, useField } from 'formik'
import * as Yup from 'yup'
const MyTextInput = ({ label, ...props }) => {
// useField() returns [formik.getFieldProps(), formik.getFieldMeta()]
// which we can spread on <input>. We can use field meta to show an error message
// if the field is invalid and it has been touched (i.e. visited)
const [field, meta] = useField(props)
return (
<>
<label htmlFor={props.id || props.name}>{label}</label>
<input className='text-input' {...field} {...props} />
{meta.touched && meta.error ? (
<div className='error'>{meta.error}</div>
) : null}
</>
)
}
// const MyCheckBox = ({ children, ...props }) => {
// // React treat radios and checkbox inputs differently other input types, select, and textarea.
// // Formik does this too! When you specify `type` to useField(), it will
// // return the correct bag of props for you -- a `checked` prop will be included
// // in `field` alongside `name`, `value`, `onChange`, and `onBlur`
// const [field, meta] = useField({ ...props, type: 'checkbox' })
// return (
// <div>
// <label className='checkbox-input'>
// <input type='checkbox' {...field} {...props} />
// {children}
// </label>
// {meta.touched && meta.error ? (
// <div className='error'>{meta.error}</div>
// ) : null}
// </div>
// )
// }
// const MySelect = ({ label, ...props }) => {
// const [field, meta] = useField(props)
// return (
// <div>
// <label htmlFor={prop.id || props.name}>{label}</label>
// <select {...meta} {...props} />
// {meta.touched && meta.error ? (
// <div className='error'>{meta.error}</div>
// ) : null}
// </div>
// )
// }
const SignupFormFifth = () => {
return (
<>
<h1>Subscribe!</h1>
<Formik
initialValues={{
firstName: '',
lastName: '',
email: '',
acceptedTerms: false, // added for our checkbox
jobType: '', // added for our select
}}
validationSchema={Yup.object({
firstName: Yup.string()
.max(15, 'Must be 15 characters or less.')
.required('Required.'),
lastName: Yup.string()
.max(20, 'Must be 20 characters or less.')
.required('Required.'),
email: Yup.string()
.email('Invalid email address.')
.required('Required.'),
acceptedTerms: Yup.boolean()
.required('Required')
.oneOf([true], 'You must accepts the terms and conditions.'),
jobType: Yup.string()
.oneOf(
['designer', 'development', 'product', 'other'],
'Invalid Job Type.'
)
.required('Required.'),
})}
onSubmit={(values) => {
alert(JSON.stringify(values, null, 2))
}}
>
<MyTextInput
label='First Name'
type='text'
id='firstName'
name='firstName'
placeholder='Jane'
/>
{/* <MyTextInput
label='Last Name'
type='text'
id='lastName'
name='lastName'
placeholder='Doe'
/> */}
{/* <MyTextInput
label='Email address'
type='email'
id='email'
name='email'
placeholder='[email protected]'
/> */}
{/* <MySelect label='Job Type' name='jobType'>
<option value=''>Select a job type</option>
<option value='designer'>Designer</option>
<option value='development'>Development</option>
<option value='product'>Product Manager</option>
<option value='other'>Other</option>
</MySelect> */}
{/* <MyCheckBox name='acceptedTerms'>
I accept the terms and conditions
</MyCheckBox> */}
<button type='submit'>Submit</button>
</Formik>
</>
)
}
export default SignupFormFifth |
package com.ezteam.applocker.viewmodel
import android.app.Application
import android.content.Context
import android.graphics.BitmapFactory
import android.os.Environment
import androidx.lifecycle.MutableLiveData
import androidx.lifecycle.viewModelScope
import com.ezteam.applocker.item.ItemAlbumRestoreImages
import com.ezteam.applocker.item.ItemImageRestore
import com.ezteam.applocker.utils.Config
import com.ezteam.applocker.utils.FileUtils
import com.ezteam.baseproject.viewmodel.BaseViewModel
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.launch
import java.io.File
class RestoreImageViewModel(application: Application) : BaseViewModel(application) {
private val listPhoto = mutableListOf<ItemImageRestore>()
// private val listAlbumRestorePhoto = mutableListOf<ItemAlbumRestoreImages>()
val position: MutableLiveData<Int> = MutableLiveData()
var albumRestorePhotoLiveData: MutableLiveData<ItemAlbumRestoreImages?> = MutableLiveData()
var listAlbumRestorePhotoLiveData: MutableLiveData<MutableList<ItemAlbumRestoreImages>?> =
MutableLiveData()
fun getImageRestore(context: Context) {
// listAlbumRestorePhoto.clear()
listPhoto.clear()
val strArr = Environment.getExternalStorageDirectory().absolutePath
viewModelScope.launch(Dispatchers.IO) {
getSdCardImage(context)
val arr = File(strArr).listFiles()
if (!arr.isNullOrEmpty()) {
checkFileOfDirectoryImage(strArr, arr)
}
// listAlbumRestorePhoto.sortWith { a, b -> if (a.lastModified > b.lastModified) 1 else if (a.lastModified < b.lastModified) -1 else 0 }
// viewModelScope.launch(Dispatchers.Main) {
// listAlbumRestorePhotoLiveData.value = listAlbumRestorePhoto
// }
}
}
private fun getSdCardImage(context: Context) {
val externalStoragePaths = FileUtils.getExternalStorageDirectories(context)
if (externalStoragePaths.size > 0) {
for (path in externalStoragePaths) {
val file = File(path)
if (file.exists()) {
val subFiles = file.listFiles()
if (!subFiles.isNullOrEmpty()) {
checkFileOfDirectoryImage(path, subFiles)
}
}
}
}
}
private fun checkFileOfDirectoryImage(temp: String, fileArr: Array<File>) {
for (i in fileArr.indices) {
if (fileArr[i].isDirectory) {
val temp_sub = fileArr[i].path
val mfileArr = File(fileArr[i].path).listFiles()
if (!mfileArr.isNullOrEmpty()) {
checkFileOfDirectoryImage(temp_sub, mfileArr)
}
} else {
val options: BitmapFactory.Options = BitmapFactory.Options()
options.inJustDecodeBounds = true
BitmapFactory.decodeFile(fileArr[i].path, options)
if (!(options.outWidth==-1||options.outHeight==-1)) {
if (fileArr[i].path.endsWith(".jpg")
||fileArr[i].path.endsWith(".jpeg")
||fileArr[i].path.endsWith(".png")
||fileArr[i].path.endsWith(".gif")
) {
val file = File(fileArr[i].path)
val file_size = file.length()
if (file_size > 10000) {
try {
listPhoto.add(
ItemImageRestore(
fileArr[i].path,
file.lastModified(),
file_size
)
)
} catch (e: ArrayIndexOutOfBoundsException) {
}
}
} else {
val file = File(fileArr[i].path)
val fileSize = file.length()
if (fileSize > 50000) {
try {
listPhoto.add(
ItemImageRestore(
fileArr[i].path,
file.lastModified(),
fileSize
)
)
} catch (e: ArrayIndexOutOfBoundsException) {
}
}
}
}
}
}
if (listPhoto.isNotEmpty()&&!temp.contains(FileUtils.getPathSave(Config.RESTORE_IMAGE))) {
val listImage = listPhoto.toMutableList()
// listImage.sortWith { a, b ->
// if (a.lastModified > b.lastModified) 1 else if (a.lastModified < b.lastModified) -1 else 0
// }
viewModelScope.launch(Dispatchers.Main) {
albumRestorePhotoLiveData.value = ItemAlbumRestoreImages(
temp,
File(temp).lastModified(),
listImage
)
}
listPhoto.clear()
}
}
} |
#!/usr/bin/env node
/**
* Module dependencies.
*/
var XDate = require('xdate');
var app = require('../app');
var debug = require('debug')('aws-socket.io-elb-proto:server');
var http = require('http');
/**
* Get port from environment and store in Express.
*/
var port = normalizePort(process.env.PORT || '80');
app.set('port', port);
/**
* Create HTTP server.
*/
var server = http.createServer(app);
var io = require('socket.io')(server);
/**
* Listen on provided port, on all network interfaces.
*/
server.listen(port);
server.on('error', onError);
server.on('listening', onListening);
console.log('Server listening on port: ' + port);
/**
* Normalize a port into a number, string, or false.
*/
function normalizePort(val) {
var port = parseInt(val, 10);
if (isNaN(port)) {
// named pipe
return val;
}
if (port >= 0) {
// port number
return port;
}
return false;
}
/**
* Event listener for HTTP server "error" event.
*/
function onError(error) {
if (error.syscall !== 'listen') {
throw error;
}
var bind = typeof port === 'string'
? 'Pipe ' + port
: 'Port ' + port;
// handle specific listen errors with friendly messages
switch (error.code) {
case 'EACCES':
console.error(bind + ' requires elevated privileges');
process.exit(1);
break;
case 'EADDRINUSE':
console.error(bind + ' is already in use');
process.exit(1);
break;
default:
throw error;
}
}
/**
* Event listener for HTTP server "listening" event.
*/
function onListening() {
var addr = server.address();
var bind = typeof addr === 'string'
? 'pipe ' + addr
: 'port ' + addr.port;
debug('Listening on ' + bind);
}
io.on('connection', function (socket) {
console.log('client connected');
socket.on('disconnect', function () {
console.log('user disconnected');
});
var emitInterval = setInterval(function() {
socket.emit('tick', new XDate().toUTCString());
}, 1000);
}); |
/*
* Copyright (c) 2021-2022 Huawei Device Co., Ltd.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
#include "audio_service_dump.h"
using namespace std;
namespace OHOS {
namespace AudioStandard {
constexpr int STRING_BUFFER_SIZE = 4096;
template <typename...Args>
void AppendFormat(std::string& out, const char* fmt, Args&& ... args)
{
char buf[STRING_BUFFER_SIZE] = {0};
int len = ::sprintf_s(buf, sizeof(buf), fmt, args...);
if (len <= 0) {
AUDIO_ERR_LOG("snprintf_s error : buffer allocation fails");
return;
}
out += buf;
}
AudioServiceDump::AudioServiceDump() : mainLoop(nullptr),
api(nullptr),
context(nullptr),
isMainLoopStarted_(false),
isContextConnected_(false)
{
AUDIO_DEBUG_LOG("AudioServiceDump ctor");
InitDumpFuncMap();
}
AudioServiceDump::~AudioServiceDump()
{
ResetPAAudioDump();
}
void AudioServiceDump::InitDumpFuncMap()
{
dumpFuncMap[u"-h"] = &AudioServiceDump::HelpInfoDump;
dumpFuncMap[u"-p"] = &AudioServiceDump::PlaybackStreamDump;
dumpFuncMap[u"-rs"] = &AudioServiceDump::RecordStreamDump;
dumpFuncMap[u"-m"] = &AudioServiceDump::HDFModulesDump;
dumpFuncMap[u"-d"] = &AudioServiceDump::DevicesInfoDump;
dumpFuncMap[u"-c"] = &AudioServiceDump::CallStatusDump;
dumpFuncMap[u"-rm"] = &AudioServiceDump::RingerModeDump;
dumpFuncMap[u"-v"] = &AudioServiceDump::StreamVolumesDump;
dumpFuncMap[u"-a"] = &AudioServiceDump::AudioFocusInfoDump;
dumpFuncMap[u"-az"] = &AudioServiceDump::AudioInterruptZoneDump;
dumpFuncMap[u"-g"] = &AudioServiceDump::GroupInfoDump;
dumpFuncMap[u"-e"] = &AudioServiceDump::EffectManagerInfoDump;
dumpFuncMap[u"-vi"] = &AudioServiceDump::StreamVolumeInfosDump;
dumpFuncMap[u"-md"] = &AudioServiceDump::MicrophoneDescriptorsDump;
}
void AudioServiceDump::ResetPAAudioDump()
{
lock_guard<mutex> lock(ctrlMutex_);
if (mainLoop && (isMainLoopStarted_ == true)) {
pa_threaded_mainloop_stop(mainLoop);
}
if (context) {
pa_context_set_state_callback(context, nullptr, nullptr);
if (isContextConnected_ == true) {
AUDIO_INFO_LOG("[AudioServiceDump] disconnect context!");
pa_context_disconnect(context);
}
pa_context_unref(context);
}
if (mainLoop) {
pa_threaded_mainloop_free(mainLoop);
}
isMainLoopStarted_ = false;
isContextConnected_ = false;
mainLoop = nullptr;
context = nullptr;
api = nullptr;
}
int32_t AudioServiceDump::Initialize()
{
int error = PA_ERR_INTERNAL;
mainLoop = pa_threaded_mainloop_new();
if (mainLoop == nullptr) {
return AUDIO_DUMP_INIT_ERR;
}
api = pa_threaded_mainloop_get_api(mainLoop);
if (api == nullptr) {
ResetPAAudioDump();
return AUDIO_DUMP_INIT_ERR;
}
context = pa_context_new(api, "AudioServiceDump");
if (context == nullptr) {
ResetPAAudioDump();
return AUDIO_DUMP_INIT_ERR;
}
pa_context_set_state_callback(context, PAContextStateCb, mainLoop);
if (pa_context_connect(context, nullptr, PA_CONTEXT_NOFAIL, nullptr) < 0) {
error = pa_context_errno(context);
AUDIO_ERR_LOG("context connect error: %{public}s", pa_strerror(error));
ResetPAAudioDump();
return AUDIO_DUMP_INIT_ERR;
}
isContextConnected_ = true;
pa_threaded_mainloop_lock(mainLoop);
if (pa_threaded_mainloop_start(mainLoop) < 0) {
AUDIO_ERR_LOG("Audio Service not started");
pa_threaded_mainloop_unlock(mainLoop);
ResetPAAudioDump();
return AUDIO_DUMP_INIT_ERR;
}
isMainLoopStarted_ = true;
while (true) {
pa_context_state_t state = pa_context_get_state(context);
if (state == PA_CONTEXT_READY) {
break;
}
if (!PA_CONTEXT_IS_GOOD(state)) {
error = pa_context_errno(context);
AUDIO_ERR_LOG("context bad state error: %{public}s", pa_strerror(error));
pa_threaded_mainloop_unlock(mainLoop);
ResetPAAudioDump();
return AUDIO_DUMP_INIT_ERR;
}
pa_threaded_mainloop_wait(mainLoop);
}
pa_threaded_mainloop_unlock(mainLoop);
return AUDIO_DUMP_SUCCESS;
}
void AudioServiceDump::OnTimeOut()
{
pa_threaded_mainloop_lock(mainLoop);
pa_threaded_mainloop_signal(mainLoop, 0);
pa_threaded_mainloop_unlock(mainLoop);
}
bool AudioServiceDump::IsEndWith(const std::string &mainStr, const std::string &toMatch)
{
if (mainStr.size() >= toMatch.size() &&
mainStr.compare(mainStr.size() - toMatch.size(), toMatch.size(), toMatch) == 0) {
return true;
}
return false;
}
bool AudioServiceDump::IsValidModule(const std::string moduleName)
{
if (moduleName.rfind("fifo", 0) == SUCCESS) {
return false; // Module starts with fifo, Not valid module
}
if (IsEndWith(moduleName, "monitor")) {
return false; // Module ends with monitor, Not valid module
}
return true;
}
bool AudioServiceDump::IsStreamSupported(AudioStreamType streamType)
{
switch (streamType) {
case STREAM_MUSIC:
case STREAM_RING:
case STREAM_VOICE_CALL:
case STREAM_VOICE_ASSISTANT:
case STREAM_WAKEUP:
return true;
default:
return false;
}
}
const std::string AudioServiceDump::GetStreamName(AudioStreamType streamType)
{
string name;
switch (streamType) {
case STREAM_VOICE_ASSISTANT:
name = "VOICE_ASSISTANT";
break;
case STREAM_VOICE_CALL:
name = "VOICE_CALL";
break;
case STREAM_SYSTEM:
name = "SYSTEM";
break;
case STREAM_RING:
name = "RING";
break;
case STREAM_MUSIC:
name = "MUSIC";
break;
case STREAM_ALARM:
name = "ALARM";
break;
case STREAM_NOTIFICATION:
name = "NOTIFICATION";
break;
case STREAM_BLUETOOTH_SCO:
name = "BLUETOOTH_SCO";
break;
case STREAM_DTMF:
name = "DTMF";
break;
case STREAM_TTS:
name = "TTS";
break;
case STREAM_ACCESSIBILITY:
name = "ACCESSIBILITY";
break;
case STREAM_ULTRASONIC:
name = "ULTRASONIC";
break;
case STREAM_WAKEUP:
name = "WAKEUP";
break;
default:
name = "UNKNOWN";
}
const string streamName = name;
return streamName;
}
const std::string AudioServiceDump::GetSourceName(SourceType sourceType)
{
string name;
switch (sourceType) {
case SOURCE_TYPE_INVALID:
name = "INVALID";
break;
case SOURCE_TYPE_MIC:
name = "MIC";
break;
case SOURCE_TYPE_VOICE_RECOGNITION:
name = "VOICE_RECOGNITION";
break;
case SOURCE_TYPE_ULTRASONIC:
name = "ULTRASONIC";
break;
case SOURCE_TYPE_VOICE_COMMUNICATION:
name = "VOICE_COMMUNICATION";
break;
case SOURCE_TYPE_WAKEUP:
name = "WAKEUP";
break;
default:
name = "UNKNOWN";
}
const string sourceName = name;
return sourceName;
}
const std::string AudioServiceDump::GetStreamUsgaeName(StreamUsage streamUsage)
{
string usage;
switch (streamUsage) {
case STREAM_USAGE_MEDIA:
usage = "MEDIA";
break;
case STREAM_USAGE_VOICE_COMMUNICATION:
usage = "VOICE_COMMUNICATION";
break;
case STREAM_USAGE_NOTIFICATION_RINGTONE:
usage = "NOTIFICATION_RINGTONE";
break;
case STREAM_USAGE_VOICE_ASSISTANT:
usage = "VOICE_ASSISTANT";
break;
default:
usage = "STREAM_USAGE_UNKNOWN";
}
const string streamUsageName = usage;
return streamUsageName;
}
const std::string AudioServiceDump::GetContentTypeName(ContentType contentType)
{
string content;
switch (contentType) {
case CONTENT_TYPE_SPEECH:
content = "SPEECH";
break;
case CONTENT_TYPE_MUSIC:
content = "MUSIC";
break;
case CONTENT_TYPE_MOVIE:
content = "MOVIE";
break;
case CONTENT_TYPE_SONIFICATION:
content = "SONIFICATION";
break;
case CONTENT_TYPE_RINGTONE:
content = "RINGTONE";
break;
default:
content = "UNKNOWN";
}
const string contentTypeName = content;
return contentTypeName;
}
const std::string AudioServiceDump::GetDeviceTypeName(DeviceType deviceType)
{
string device;
switch (deviceType) {
case DEVICE_TYPE_EARPIECE:
device = "EARPIECE";
break;
case DEVICE_TYPE_SPEAKER:
device = "SPEAKER";
break;
case DEVICE_TYPE_WIRED_HEADSET:
device = "WIRED_HEADSET";
break;
case DEVICE_TYPE_WIRED_HEADPHONES:
device = "WIRED_HEADPHONES";
break;
case DEVICE_TYPE_BLUETOOTH_SCO:
device = "BLUETOOTH_SCO";
break;
case DEVICE_TYPE_BLUETOOTH_A2DP:
device = "BLUETOOTH_A2DP";
break;
case DEVICE_TYPE_MIC:
device = "MIC";
break;
case DEVICE_TYPE_WAKEUP:
device = "WAKEUP";
break;
case DEVICE_TYPE_NONE:
device = "NONE";
break;
case DEVICE_TYPE_INVALID:
device = "INVALID";
break;
default:
device = "UNKNOWN";
}
const string deviceTypeName = device;
return deviceTypeName;
}
const std::string AudioServiceDump::GetConnectTypeName(ConnectType connectType)
{
string connectName;
switch (connectType) {
case OHOS::AudioStandard::CONNECT_TYPE_LOCAL:
connectName = "LOCAL";
break;
case OHOS::AudioStandard::CONNECT_TYPE_DISTRIBUTED:
connectName = "REMOTE";
break;
default:
connectName = "UNKNOWN";
break;
}
const string connectTypeName = connectName;
return connectTypeName;
}
const std::string AudioServiceDump::GetDeviceVolumeTypeName(DeviceVolumeType deviceType)
{
string device;
switch (deviceType) {
case EARPIECE_VOLUME_TYPE:
device = "EARPIECE";
break;
case SPEAKER_VOLUME_TYPE:
device = "SPEAKER";
break;
case HEADSET_VOLUME_TYPE:
device = "HEADSET";
break;
default:
device = "UNKNOWN";
}
const string deviceTypeName = device;
return deviceTypeName;
}
void AudioServiceDump::PlaybackStreamDump(std::string &dumpString)
{
char s[PA_SAMPLE_SPEC_SNPRINT_MAX];
dumpString += "Playback Streams\n";
AppendFormat(dumpString, "- %d Playback stream (s) available:\n", audioData_.streamData.sinkInputs.size());
for (auto it = audioData_.streamData.sinkInputs.begin(); it != audioData_.streamData.sinkInputs.end(); it++) {
InputOutputInfo sinkInputInfo = *it;
AppendFormat(dumpString, " Stream %d\n", it - audioData_.streamData.sinkInputs.begin() + 1);
AppendFormat(dumpString, " - Stream Id: %s\n", (sinkInputInfo.sessionId).c_str());
AppendFormat(dumpString, " - Application Name: %s\n", ((sinkInputInfo.applicationName).c_str()));
AppendFormat(dumpString, " - Process Id: %s\n", (sinkInputInfo.processId).c_str());
AppendFormat(dumpString, " - User Id: %u\n", sinkInputInfo.userId);
char *inputSampleSpec = pa_sample_spec_snprint(s, sizeof(s), &(sinkInputInfo.sampleSpec));
AppendFormat(dumpString, " - Stream Configuration: %s\n", inputSampleSpec);
dumpString += " - Status:";
dumpString += (sinkInputInfo.corked) ? "STOPPED/PAUSED" : "RUNNING";
AppendFormat(dumpString, "\n - Stream Start Time: %s\n", (sinkInputInfo.sessionStartTime).c_str());
dumpString += "\n";
}
}
void AudioServiceDump::RecordStreamDump(std::string &dumpString)
{
char s[PA_SAMPLE_SPEC_SNPRINT_MAX];
dumpString += "Record Streams \n";
AppendFormat(dumpString, "- %d Record stream (s) available:\n", audioData_.streamData.sourceOutputs.size());
for (auto it = audioData_.streamData.sourceOutputs.begin(); it != audioData_.streamData.sourceOutputs.end(); it++) {
InputOutputInfo sourceOutputInfo = *it;
AppendFormat(dumpString, " Stream %d\n", it - audioData_.streamData.sourceOutputs.begin() + 1);
AppendFormat(dumpString, " - Stream Id: %s\n", (sourceOutputInfo.sessionId).c_str());
AppendFormat(dumpString, " - Application Name: %s\n", (sourceOutputInfo.applicationName).c_str());
AppendFormat(dumpString, " - Process Id: %s\n", sourceOutputInfo.processId.c_str());
AppendFormat(dumpString, " - User Id: %d\n", sourceOutputInfo.userId);
char *outputSampleSpec = pa_sample_spec_snprint(s, sizeof(s), &(sourceOutputInfo.sampleSpec));
AppendFormat(dumpString, " - Stream Configuration: %s\n", outputSampleSpec);
dumpString += " - Status:";
dumpString += (sourceOutputInfo.corked) ? "STOPPED/PAUSED" : "RUNNING";
AppendFormat(dumpString, "\n - Stream Start Time: %s\n", (sourceOutputInfo.sessionStartTime).c_str());
dumpString += "\n";
}
}
void AudioServiceDump::HDFModulesDump(std::string &dumpString)
{
char s[PA_SAMPLE_SPEC_SNPRINT_MAX];
dumpString += "\nHDF Input Modules\n";
AppendFormat(dumpString, "- %d HDF Input Modules (s) available:\n", audioData_.streamData.sourceDevices.size());
for (auto it = audioData_.streamData.sourceDevices.begin(); it != audioData_.streamData.sourceDevices.end(); it++) {
SinkSourceInfo sourceInfo = *it;
AppendFormat(dumpString, " Module %d\n", it - audioData_.streamData.sourceDevices.begin() + 1);
AppendFormat(dumpString, " - Module Name: %s\n", (sourceInfo.name).c_str());
char *hdfOutSampleSpec = pa_sample_spec_snprint(s, sizeof(s), &(sourceInfo.sampleSpec));
AppendFormat(dumpString, " - Module Configuration: %s\n\n", hdfOutSampleSpec);
}
dumpString += "HDF Output Modules\n";
AppendFormat(dumpString, "- %d HDF Output Modules (s) available:\n", audioData_.streamData.sinkDevices.size());
for (auto it = audioData_.streamData.sinkDevices.begin(); it != audioData_.streamData.sinkDevices.end(); it++) {
SinkSourceInfo sinkInfo = *it;
AppendFormat(dumpString, " Module %d\n", it - audioData_.streamData.sinkDevices.begin() + 1);
AppendFormat(dumpString, " - Module Name: %s\n", (sinkInfo.name).c_str());
char *hdfInSampleSpec = pa_sample_spec_snprint(s, sizeof(s), &(sinkInfo.sampleSpec));
AppendFormat(dumpString, " - Module Configuration: %s\n\n", hdfInSampleSpec);
}
}
void AudioServiceDump::CallStatusDump(std::string &dumpString)
{
dumpString += "\nAudio Scene:";
switch (audioData_.policyData.callStatus) {
case AUDIO_SCENE_DEFAULT:
dumpString += "DEFAULT";
break;
case AUDIO_SCENE_RINGING:
dumpString += "RINGING";
break;
case AUDIO_SCENE_PHONE_CALL:
dumpString += "PHONE_CALL";
break;
case AUDIO_SCENE_PHONE_CHAT:
dumpString += "PHONE_CHAT";
break;
default:
dumpString += "UNKNOWN";
}
dumpString += "\n";
}
void AudioServiceDump::RingerModeDump(std::string &dumpString)
{
dumpString += "Ringer Mode:";
switch (audioData_.policyData.ringerMode) {
case RINGER_MODE_NORMAL:
dumpString += "NORMAL";
break;
case RINGER_MODE_SILENT:
dumpString += "SILENT";
break;
case RINGER_MODE_VIBRATE:
dumpString += "VIBRATE";
break;
default:
dumpString += "UNKNOWN";
}
dumpString += "\n";
}
void AudioServiceDump::StreamVolumesDump (string &dumpString)
{
dumpString += "\nStream Volumes\n";
AppendFormat(dumpString, " [StreamName]: [Volume]\n");
for (auto it = audioData_.policyData.streamVolumes.cbegin(); it != audioData_.policyData.streamVolumes.cend();
++it) {
AppendFormat(dumpString, " - %s: %d\n", GetStreamName(it->first).c_str(), it->second);
}
dumpString += "\n";
return;
}
void AudioServiceDump::AudioFocusInfoDump(string &dumpString)
{
dumpString += "\nAudio In Focus Info:\n";
uint32_t invalidSessionID = static_cast<uint32_t>(-1);
std::list<std::pair<AudioInterrupt, AudioFocuState>> audioFocusInfoList = audioData_.policyData.audioFocusInfoList;
AppendFormat(dumpString, "- %d Audio Focus Info (s) available:\n", audioFocusInfoList.size());
for (auto iter = audioFocusInfoList.begin(); iter != audioFocusInfoList.end(); ++iter) {
if ((iter->first).sessionID == invalidSessionID) {
continue;
}
AppendFormat(dumpString, " - Session Id: %d\n", (iter->first).sessionID);
AppendFormat(dumpString, " - AudioFocus isPlay Id: %d\n", (iter->first).audioFocusType.isPlay);
AppendFormat(dumpString, " - Stream Name: %s\n",
GetStreamName((iter->first).audioFocusType.streamType).c_str());
AppendFormat(dumpString, " - Source Name: %s\n",
GetSourceName((iter->first).audioFocusType.sourceType).c_str());
AppendFormat(dumpString, " - AudioFocus State: %d\n", iter->second);
dumpString += "\n";
}
return;
}
void AudioServiceDump::AudioInterruptZoneDump(string &dumpString)
{
dumpString += "\nAudioInterrupt Zone:\n";
AppendFormat(dumpString, "- %d AudioInterruptZoneDump (s) available:\n",
audioData_.policyData.audioInterruptZonesMapDump.size());
for (const auto&[zoneID, audioInterruptZoneDump] : audioData_.policyData.audioInterruptZonesMapDump) {
if (zoneID < 0) {
continue;
}
AppendFormat(dumpString, " - Zone ID: %d\n", zoneID);
AppendFormat(dumpString, " - Pids size: %d\n", audioInterruptZoneDump->pids.size());
for (auto pid : audioInterruptZoneDump->pids) {
AppendFormat(dumpString, " - pid: %d\n", pid);
}
AppendFormat(dumpString, " - Interrupt callback size: %d\n",
audioInterruptZoneDump->interruptCbSessionIDsMap.size());
AppendFormat(dumpString, " - The sessionIDs as follow:\n");
for (auto sessionID : audioInterruptZoneDump->interruptCbSessionIDsMap) {
AppendFormat(dumpString, " - SessionID: %d -- have interrupt callback.\n", sessionID);
}
AppendFormat(dumpString, " - Audio policy client proxy callback size: %d\n",
audioInterruptZoneDump->audioPolicyClientProxyCBClientPidMap.size());
AppendFormat(dumpString, " - The clientPids as follow:\n");
for (auto pid : audioInterruptZoneDump->audioPolicyClientProxyCBClientPidMap) {
AppendFormat(dumpString, " - ClientPid: %d -- have audiopolicy client proxy callback.\n", pid);
}
std::list<std::pair<AudioInterrupt, AudioFocuState>> audioFocusInfoList
= audioInterruptZoneDump->audioFocusInfoList;
AppendFormat(dumpString, " - %d Audio Focus Info (s) available:\n", audioFocusInfoList.size());
uint32_t invalidSessionID = static_cast<uint32_t>(-1);
for (auto iter = audioFocusInfoList.begin(); iter != audioFocusInfoList.end(); ++iter) {
if ((iter->first).sessionID == invalidSessionID) {
continue;
}
AppendFormat(dumpString, " - Pid: %d\n", (iter->first).pid);
AppendFormat(dumpString, " - Session ID: %d\n", (iter->first).sessionID);
AppendFormat(dumpString, " - AudioFocus isPlay Id: %d\n", (iter->first).audioFocusType.isPlay);
AppendFormat(dumpString, " - Stream Name: %s\n",
GetStreamName((iter->first).audioFocusType.streamType).c_str());
AppendFormat(dumpString, " - Source Name: %s\n",
GetSourceName((iter->first).audioFocusType.sourceType).c_str());
AppendFormat(dumpString, " - AudioFocus State: %d\n", iter->second);
dumpString += "\n";
}
dumpString += "\n";
}
return;
}
void AudioServiceDump::GroupInfoDump(std::string& dumpString)
{
dumpString += "\nGroupInfo:\n";
AppendFormat(dumpString, "- %d Group Infos (s) available :\n", audioData_.policyData.groupInfos.size());
for (auto it = audioData_.policyData.groupInfos.begin(); it != audioData_.policyData.groupInfos.end(); it++) {
GroupInfo groupInfo = *it;
AppendFormat(dumpString, " Group Infos %d\n", it - audioData_.policyData.groupInfos.begin() + 1);
AppendFormat(dumpString, " - ConnectType(0 for Local, 1 for Remote): %d\n", groupInfo.type);
AppendFormat(dumpString, " - Name: %s\n", groupInfo.groupName.c_str());
AppendFormat(dumpString, " - Id: %d\n", groupInfo.groupId);
}
}
void AudioServiceDump::DevicesInfoDump(string& dumpString)
{
dumpString += "\nInput Devices:\n";
AppendFormat(dumpString, "- %d Input Devices (s) available :\n", audioData_.policyData.inputDevices.size());
for (auto it = audioData_.policyData.inputDevices.begin(); it != audioData_.policyData.inputDevices.end(); it++) {
DevicesInfo devicesInfo = *it;
AppendFormat(dumpString, " device %d\n", it - audioData_.policyData.inputDevices.begin() + 1);
AppendFormat(dumpString, " - device type:%s\n", GetDeviceTypeName(devicesInfo.deviceType).c_str());
AppendFormat(dumpString, " - connect type:%s\n", GetConnectTypeName(devicesInfo.conneceType).c_str());
}
dumpString += "\nOutput Devices:\n";
AppendFormat(dumpString, "- %d Output Devices (s) available :\n", audioData_.policyData.outputDevices.size());
for (auto it = audioData_.policyData.outputDevices.begin(); it != audioData_.policyData.outputDevices.end(); it++) {
DevicesInfo devicesInfo = *it;
AppendFormat(dumpString, " device %d\n", it - audioData_.policyData.outputDevices.begin() + 1);
AppendFormat(dumpString, " - device type:%s\n", GetDeviceTypeName(devicesInfo.deviceType).c_str());
AppendFormat(dumpString, " - connect type:%s\n", GetConnectTypeName(devicesInfo.conneceType).c_str());
}
AppendFormat(dumpString, "\nHighest priority output device: %s",
GetDeviceTypeName(audioData_.policyData.priorityOutputDevice).c_str());
AppendFormat(dumpString, "\nHighest priority input device: %s \n\n",
GetDeviceTypeName(audioData_.policyData.priorityInputDevice).c_str());
}
static void EffectManagerInfoDumpPart(string& dumpString, const AudioData &audioData_)
{
int32_t count;
// xml -- Preprocess
AppendFormat(dumpString, "- %d preProcess (s) available :\n",
audioData_.policyData.oriEffectConfig.preProcess.size());
for (Preprocess x : audioData_.policyData.oriEffectConfig.preProcess) {
AppendFormat(dumpString, " preProcess stream = %s \n", x.stream.c_str());
count = 0;
for (string modeName : x.mode) {
count++;
AppendFormat(dumpString, " - modeName%d = %s \n", count, modeName.c_str());
for (Device deviceInfo : x.device[count - 1]) {
AppendFormat(dumpString, " - device type = %s \n", deviceInfo.type.c_str());
AppendFormat(dumpString, " - device chain = %s \n", deviceInfo.chain.c_str());
}
}
dumpString += "\n";
}
// xml -- Postprocess
AppendFormat(dumpString, "- %d postProcess (s) available :\n",
audioData_.policyData.oriEffectConfig.preProcess.size());
for (Postprocess x : audioData_.policyData.oriEffectConfig.postProcess) {
AppendFormat(dumpString, " postprocess stream = %s \n", x.stream.c_str());
count = 0;
for (string modeName : x.mode) {
count++;
AppendFormat(dumpString, " - modeName%d = %s \n", count, modeName.c_str());
for (Device deviceInfo : x.device[count - 1]) {
AppendFormat(dumpString, " - device type = %s \n", deviceInfo.type.c_str());
AppendFormat(dumpString, " - device chain = %s \n", deviceInfo.chain.c_str());
}
}
dumpString += "\n";
}
}
void AudioServiceDump::EffectManagerInfoDump(string& dumpString)
{
int count = 0;
dumpString += "\nEffect Manager INFO\n";
AppendFormat(dumpString, " XML version:%f \n", audioData_.policyData.oriEffectConfig.version);
// xml -- Library
AppendFormat(dumpString, "- %d library (s) available :\n", audioData_.policyData.oriEffectConfig.libraries.size());
for (Library x : audioData_.policyData.oriEffectConfig.libraries) {
count++;
AppendFormat(dumpString, " library%d\n", count);
AppendFormat(dumpString, " - library name = %s \n", x.name.c_str());
AppendFormat(dumpString, " - library path = %s \n", x.path.c_str());
dumpString += "\n";
}
// xml -- effect
count = 0;
AppendFormat(dumpString, "- %d effect (s) available :\n", audioData_.policyData.oriEffectConfig.effects.size());
for (Effect x : audioData_.policyData.oriEffectConfig.effects) {
count++;
AppendFormat(dumpString, " effect%d\n", count);
AppendFormat(dumpString, " - effect name = %s \n", x.name.c_str());
AppendFormat(dumpString, " - effect libraryName = %s \n", x.libraryName.c_str());
dumpString += "\n";
}
// xml -- effectChain
count = 0;
AppendFormat(dumpString, "- %d effectChain (s) available :\n",
audioData_.policyData.oriEffectConfig.effectChains.size());
for (EffectChain x : audioData_.policyData.oriEffectConfig.effectChains) {
count++;
AppendFormat(dumpString, " effectChain%d\n", count);
AppendFormat(dumpString, " - effectChain name = %s \n", x.name.c_str());
int countEffect = 0;
for (string effectUnit : x.apply) {
countEffect++;
AppendFormat(dumpString, " - effectUnit%d = %s \n", countEffect, effectUnit.c_str());
}
dumpString += "\n";
}
EffectManagerInfoDumpPart(dumpString, audioData_);
// successful lib
count = 0;
AppendFormat(dumpString, "- %d available Effect (s) available :\n",
audioData_.policyData.availableEffects.size());
for (Effect x : audioData_.policyData.availableEffects) {
count++;
AppendFormat(dumpString, " available Effect%d\n", count);
AppendFormat(dumpString, " - available Effect%d name = %s \n", count, x.name.c_str());
AppendFormat(dumpString, " - available Effect%d libraryName = %s \n", count, x.libraryName.c_str());
dumpString += "\n";
}
}
void AudioServiceDump::DataDump(string &dumpString)
{
PlaybackStreamDump(dumpString);
RecordStreamDump(dumpString);
HDFModulesDump(dumpString);
DevicesInfoDump(dumpString);
CallStatusDump(dumpString);
RingerModeDump(dumpString);
StreamVolumesDump(dumpString);
AudioFocusInfoDump(dumpString);
AudioInterruptZoneDump(dumpString);
GroupInfoDump(dumpString);
EffectManagerInfoDump(dumpString);
StreamVolumeInfosDump(dumpString);
MicrophoneDescriptorsDump(dumpString);
}
void AudioServiceDump::ArgDataDump(std::string &dumpString, std::queue<std::u16string>& argQue)
{
dumpString += "Audio Data Dump:\n\n";
if (argQue.empty()) {
DataDump(dumpString);
return;
}
while (!argQue.empty()) {
std::u16string para = argQue.front();
if (para == u"-h") {
dumpString.clear();
(this->*dumpFuncMap[para])(dumpString);
return;
} else if (dumpFuncMap.count(para) == 0) {
dumpString.clear();
AppendFormat(dumpString, "Please input correct param:\n");
HelpInfoDump(dumpString);
return;
} else {
(this->*dumpFuncMap[para])(dumpString);
}
argQue.pop();
}
}
void AudioServiceDump::HelpInfoDump(string &dumpString)
{
AppendFormat(dumpString, "usage:\n");
AppendFormat(dumpString, " -h\t\t\t|help text for hidumper audio\n");
AppendFormat(dumpString, " -p\t\t\t|dump playback streams\n");
AppendFormat(dumpString, " -rs\t\t\t|dump record Streams\n");
AppendFormat(dumpString, " -m\t\t\t|dump hdf input modules\n");
AppendFormat(dumpString, " -d\t\t\t|dump input devices & output devices\n");
AppendFormat(dumpString, " -c\t\t\t|dump audio scene(call status)\n");
AppendFormat(dumpString, " -rm\t\t\t|dump ringer mode\n");
AppendFormat(dumpString, " -v\t\t\t|dump stream volumes\n");
AppendFormat(dumpString, " -a\t\t\t|dump audio in focus info\n");
AppendFormat(dumpString, " -az\t\t\t|dump audio in interrupt zone info\n");
AppendFormat(dumpString, " -g\t\t\t|dump group info\n");
AppendFormat(dumpString, " -e\t\t\t|dump effect manager info\n");
AppendFormat(dumpString, " -vi\t\t\t|dump volume config of streams\n");
AppendFormat(dumpString, " -md\t\t\t|dump available microphone descriptors\n");
}
void AudioServiceDump::AudioDataDump(PolicyData &policyData, string &dumpString,
std::queue<std::u16string>& argQue)
{
if (mainLoop == nullptr || context == nullptr) {
AUDIO_ERR_LOG("Audio Service Not running");
return;
}
pa_threaded_mainloop_lock(mainLoop);
pa_operation *operation = nullptr;
operation = pa_context_get_sink_info_list(context, AudioServiceDump::PASinkInfoCallback, (void *)(this));
while (pa_operation_get_state(operation) == PA_OPERATION_RUNNING) {
pa_threaded_mainloop_wait(mainLoop);
}
pa_operation_unref(operation);
operation = pa_context_get_sink_input_info_list(context, AudioServiceDump::PASinkInputInfoCallback, (void *)this);
while (pa_operation_get_state(operation) == PA_OPERATION_RUNNING) {
pa_threaded_mainloop_wait(mainLoop);
}
pa_operation_unref(operation);
operation = pa_context_get_source_info_list(context, AudioServiceDump::PASourceInfoCallback, (void *)this);
while (pa_operation_get_state(operation) == PA_OPERATION_RUNNING) {
pa_threaded_mainloop_wait(mainLoop);
}
pa_operation_unref(operation);
operation = pa_context_get_source_output_info_list(context,
AudioServiceDump::PASourceOutputInfoCallback, (void *)this);
while (pa_operation_get_state(operation) == PA_OPERATION_RUNNING) {
pa_threaded_mainloop_wait(mainLoop);
}
pa_operation_unref(operation);
pa_threaded_mainloop_unlock(mainLoop);
audioData_.policyData = policyData;
ArgDataDump(dumpString, argQue);
return;
}
void AudioServiceDump::PAContextStateCb(pa_context *context, void *userdata)
{
pa_threaded_mainloop *mainLoop = (pa_threaded_mainloop *)userdata;
switch (pa_context_get_state(context)) {
case PA_CONTEXT_READY:
case PA_CONTEXT_TERMINATED:
case PA_CONTEXT_FAILED:
pa_threaded_mainloop_signal(mainLoop, 0);
break;
case PA_CONTEXT_UNCONNECTED:
case PA_CONTEXT_CONNECTING:
case PA_CONTEXT_AUTHORIZING:
case PA_CONTEXT_SETTING_NAME:
default:
break;
}
return;
}
void AudioServiceDump::PASinkInfoCallback(pa_context *c, const pa_sink_info *i, int eol, void *userdata)
{
AudioServiceDump *asDump = (AudioServiceDump *)userdata;
if (asDump == nullptr) {
AUDIO_ERR_LOG("Failed to get sink information");
return;
}
pa_threaded_mainloop *mainLoop = (pa_threaded_mainloop *)asDump->mainLoop;
if (eol < 0) {
AUDIO_ERR_LOG("Failed to get sink information: %{public}s", pa_strerror(pa_context_errno(c)));
return;
}
if (eol) {
pa_threaded_mainloop_signal(mainLoop, 0);
return;
}
SinkSourceInfo sinkInfo;
if (i->name != nullptr) {
string sinkName(i->name);
if (IsValidModule(sinkName)) {
(sinkInfo.name).assign(sinkName);
sinkInfo.sampleSpec = i->sample_spec;
asDump->audioData_.streamData.sinkDevices.push_back(sinkInfo);
}
}
}
void AudioServiceDump::PASinkInputInfoCallback(pa_context *c, const pa_sink_input_info *i, int eol, void *userdata)
{
AudioServiceDump *asDump = (AudioServiceDump *)userdata;
if (asDump == nullptr) {
AUDIO_ERR_LOG("Failed to get sink input information");
return;
}
pa_threaded_mainloop *mainLoop = (pa_threaded_mainloop *)asDump->mainLoop;
if (eol < 0) {
AUDIO_ERR_LOG("Failed to get sink input information: %{public}s", pa_strerror(pa_context_errno(c)));
return;
}
if (eol) {
pa_threaded_mainloop_signal(mainLoop, 0);
return;
}
InputOutputInfo sinkInputInfo;
sinkInputInfo.sampleSpec = i->sample_spec;
sinkInputInfo.corked = i->corked;
if (i->proplist !=nullptr) {
const char *applicationname = pa_proplist_gets(i->proplist, "application.name");
const char *processid = pa_proplist_gets(i->proplist, "application.process.id");
const char *user = pa_proplist_gets(i->proplist, "application.process.user");
const char *sessionid = pa_proplist_gets(i->proplist, "stream.sessionID");
const char *sessionstarttime = pa_proplist_gets(i->proplist, "stream.startTime");
if (applicationname != nullptr) {
string applicationName(applicationname);
(sinkInputInfo.applicationName).assign(applicationName);
}
if (processid != nullptr) {
string processId(processid);
(sinkInputInfo.processId).assign(processId);
}
if (user != nullptr) {
struct passwd *p;
if ((p = getpwnam(user)) != nullptr) {
sinkInputInfo.userId = uint32_t(p->pw_uid);
}
}
if (sessionid != nullptr) {
string sessionId(sessionid);
(sinkInputInfo.sessionId).assign(sessionId);
}
if (sessionstarttime != nullptr) {
string sessionStartTime(sessionstarttime);
(sinkInputInfo.sessionStartTime).assign(sessionStartTime);
}
}
asDump->audioData_.streamData.sinkInputs.push_back(sinkInputInfo);
}
void AudioServiceDump::PASourceInfoCallback(pa_context *c, const pa_source_info *i, int eol, void *userdata)
{
AudioServiceDump *asDump = (AudioServiceDump *)userdata;
if (asDump == nullptr) {
AUDIO_ERR_LOG("Failed to get source information");
return;
}
pa_threaded_mainloop *mainLoop = (pa_threaded_mainloop *)asDump->mainLoop;
if (eol < 0) {
AUDIO_ERR_LOG("Failed to get source information: %{public}s", pa_strerror(pa_context_errno(c)));
return;
}
if (eol) {
pa_threaded_mainloop_signal(mainLoop, 0);
return;
}
SinkSourceInfo sourceInfo;
if (i->name != nullptr) {
string sourceName(i->name);
if (IsValidModule(sourceName)) {
(sourceInfo.name).assign(sourceName);
sourceInfo.sampleSpec = i->sample_spec;
asDump->audioData_.streamData.sourceDevices.push_back(sourceInfo);
}
}
}
void AudioServiceDump::PASourceOutputInfoCallback(pa_context *c, const pa_source_output_info *i, int eol,
void *userdata)
{
AudioServiceDump *asDump = (AudioServiceDump *)userdata;
if (asDump == nullptr) {
AUDIO_ERR_LOG("Failed to get source output information");
return;
}
pa_threaded_mainloop *mainLoop = (pa_threaded_mainloop *)asDump->mainLoop;
if (eol < 0) {
AUDIO_ERR_LOG("Failed to get source output information: %{public}s", pa_strerror(pa_context_errno(c)));
return;
}
if (eol) {
pa_threaded_mainloop_signal(mainLoop, 0);
return;
}
InputOutputInfo sourceOutputInfo;
sourceOutputInfo.sampleSpec = i->sample_spec;
sourceOutputInfo.corked = i->corked;
if (i->proplist !=nullptr) {
const char *applicationname = pa_proplist_gets(i->proplist, "application.name");
const char *processid = pa_proplist_gets(i->proplist, "application.process.id");
const char *user = pa_proplist_gets(i->proplist, "application.process.user");
const char *sessionid = pa_proplist_gets(i->proplist, "stream.sessionID");
const char *sessionstarttime = pa_proplist_gets(i->proplist, "stream.startTime");
if (applicationname != nullptr) {
string applicationName(applicationname);
(sourceOutputInfo.applicationName).assign(applicationName);
}
if (processid != nullptr) {
string processId(processid);
(sourceOutputInfo.processId).assign(processId);
}
if (user != nullptr) {
struct passwd *p;
if ((p = getpwnam(user)) != nullptr) {
sourceOutputInfo.userId = uint32_t(p->pw_uid);
}
}
if (sessionid != nullptr) {
string sessionId(sessionid);
(sourceOutputInfo.sessionId).assign(sessionId);
}
if (sessionstarttime != nullptr) {
string sessionStartTime(sessionstarttime);
(sourceOutputInfo.sessionStartTime).assign(sessionStartTime);
}
}
asDump->audioData_.streamData.sourceOutputs.push_back(sourceOutputInfo);
}
void AudioServiceDump::DeviceVolumeInfosDump(std::string& dumpString, DeviceVolumeInfoMap &deviceVolumeInfos)
{
for (auto iter = deviceVolumeInfos.cbegin(); iter != deviceVolumeInfos.cend(); ++iter) {
AppendFormat(dumpString, " %s : {", GetDeviceVolumeTypeName(iter->first).c_str());
auto volumePoints = iter->second->volumePoints;
for (auto volPoint = volumePoints.cbegin(); volPoint != volumePoints.cend(); ++volPoint) {
AppendFormat(dumpString, "[%d, %d]", volPoint->index, volPoint->dbValue);
if (volPoint + 1 != volumePoints.cend()) {
dumpString += ", ";
}
}
dumpString += "}\n";
}
AppendFormat(dumpString, "\n");
}
void AudioServiceDump::StreamVolumeInfosDump(std::string& dumpString)
{
dumpString += "\nVolume config of streams:\n";
for (auto it = audioData_.policyData.streamVolumeInfos.cbegin();
it != audioData_.policyData.streamVolumeInfos.cend(); ++it) {
AppendFormat(dumpString, " %s: ", GetStreamName(it->first).c_str());
auto streamVolumeInfo = it->second;
AppendFormat(dumpString, "minLevel = %d ", streamVolumeInfo->minLevel);
AppendFormat(dumpString, "maxLevel = %d ", streamVolumeInfo->maxLevel);
AppendFormat(dumpString, "defaultLevel = %d\n", streamVolumeInfo->defaultLevel);
DeviceVolumeInfosDump(dumpString, streamVolumeInfo->deviceVolumeInfos);
}
}
void AudioServiceDump::MicrophoneDescriptorsDump(std::string& dumpString)
{
dumpString += "\nAvailable MicrophoneDescriptors:\n";
for (auto it = audioData_.policyData.availableMicrophones.begin();
it != audioData_.policyData.availableMicrophones.end(); ++it) {
AppendFormat(dumpString, "id:%d \n", (*it)->micId_);
AppendFormat(dumpString, "device type:%d \n", (*it)->deviceType_);
AppendFormat(dumpString, "group id:%d \n", (*it)->groupId_);
AppendFormat(dumpString, "sensitivity:%d \n", (*it)->sensitivity_);
AppendFormat(dumpString, "position:%f %f %f (x, y, z)\n",
(*it)->position_.x, (*it)->position_.y, (*it)->position_.z);
AppendFormat(dumpString, "orientation:%f %f %f (x, y, z)\n",
(*it)->orientation_.x, (*it)->orientation_.y, (*it)->orientation_.z);
}
}
} // namespace AudioStandard
} // namespace OHOS |
import { Articles } from "@/components/dashboard/articles";
import { ArticlesEmpty } from "@/components/dashboard/articles-empty";
import { auth } from "auth";
import { redirect } from "next/navigation";
const getArticlesByPage = async (accessToken: string, id: string) => {
const res = await fetch(
process.env.NEXT_PUBLIC_API_URL + "/api/articles?page=" + id,
{
headers: {
"Content-Type": "application/json",
Authorization: `Bearer ${accessToken}`,
},
}
);
if (!res.ok) {
return [];
}
return await res.json();
};
export default async function ArticlePage({
params,
}: {
params: { page: string };
}) {
if (parseInt(params.page) > 2) redirect("/articles/2");
const session = await auth();
const {
jwt: { access },
} = session;
const { data: articles } = await getArticlesByPage(access, params.page);
return (
<>
{articles && articles.length === 0 ? (
<ArticlesEmpty />
) : (
<Articles articles={articles} page={params.page} />
)}
</>
);
} |
#define _CRT_SECURE_NO_WARNINGS
#include <locale.h>
#include <iostream>
#include <string.h>
using namespace std;
const int MAX_CLIENTS = 100; // Максимальное количество клиентов
struct Deposit {
int accountNumber;
char* lastName;
char* firstName;
double balance;
};
void addNewAccount(Deposit accounts[], int& count) {
if (count < MAX_CLIENTS) {
Deposit newAccount;
cout << "Введите номер счета: ";
cin >> newAccount.accountNumber;
char lastName[50];
char firstName[50];
cout << "Введите фамилию клиента: ";
cin >> lastName;
newAccount.lastName = new char[strlen(lastName) + 1];
strcpy(newAccount.lastName, lastName);
cout << "Введите имя клиента: ";
cin >> firstName;
newAccount.firstName = new char[strlen(firstName) + 1];
strcpy(newAccount.firstName, firstName);
cout << "Введите сумму на счете: ";
cin >> newAccount.balance;
accounts[count++] = newAccount;
}
else {
cout << "Достигнуто максимальное количество клиентов." << endl;
}
}
void printAccounts(const Deposit accounts[], int count) {
cout << "Номер счета\tФамилия\t\tИмя\t\tСумма на счете" << endl;
for (int i = 0; i < count; ++i) {
cout << accounts[i].accountNumber << "\t\t"
<< accounts[i].lastName << "\t\t"
<< accounts[i].firstName << "\t\t"
<< accounts[i].balance << endl;
}
}
void findAccountsByBalance(const Deposit accounts[], int count, double threshold) {
cout << "Счета с суммой больше " << threshold << ":" << endl;
for (int i = 0; i < count; ++i) {
if (accounts[i].balance > threshold) {
cout << accounts[i].accountNumber << "\t\t"
<< accounts[i].lastName << "\t\t"
<< accounts[i].firstName << "\t\t"
<< accounts[i].balance << endl;
}
}
}
void findAccountsByClient(const Deposit accounts[], int count, const char* lastName, const char* firstName) {
cout << "Счета клиента " << firstName << " " << lastName << ":" << endl;
for (int i = 0; i < count; ++i) {
if (strcmp(accounts[i].lastName, lastName) == 0 && strcmp(accounts[i].firstName, firstName) == 0) {
cout << accounts[i].accountNumber << "\t\t"
<< accounts[i].lastName << "\t\t"
<< accounts[i].firstName << "\t\t"
<< accounts[i].balance << endl;
}
}
}
int main() {
setlocale(0, "Russian");
Deposit bankClients[MAX_CLIENTS];
int clientCount = 0;
int choice;
double threshold;
char lastName[50];
char firstName[50];
do {
cout << "\nВыберите действие:\n";
cout << "1. Добавить новый счет\n";
cout << "2. Распечатать информацию о счетах\n";
cout << "3. Найти счета с суммой больше заданной\n";
cout << "4. Найти счета заданного клиента\n";
cout << "0. Выйти\n";
cout << "Ваш выбор: ";
cin >> choice;
switch (choice) {
case 1:
addNewAccount(bankClients, clientCount);
break;
case 2:
printAccounts(bankClients, clientCount);
break;
case 3:
cout << "Введите пороговую сумму: ";
cin >> threshold;
findAccountsByBalance(bankClients, clientCount, threshold);
break;
case 4:
cout << "Введите фамилию клиента: ";
cin >> lastName;
cout << "Введите имя клиента: ";
cin >> firstName;
findAccountsByClient(bankClients, clientCount, lastName, firstName);
break;
case 0:
cout << "Конец\n";
break;
default:
cout << "Некорректный ввод\n";
}
} while (choice != 0);
for (int i = 0; i < clientCount; ++i) {
delete[] bankClients[i].lastName;
delete[] bankClients[i].firstName;
}
return 0;
} |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.Json.Serialization;
namespace FlexionV2.Logic
{
public class Layer
{
private double _widthAtCenter;
[JsonInclude]
public double WidthAtCenter
{
get =>_widthAtCenter;
set { if (value > 0) { _widthAtCenter = value; } }
}
private double _widthOnSide;
[JsonInclude]
public double WidthOnSide
{
get =>_widthOnSide;
set { if (value > 0) { _widthOnSide = value; } }
}
private double _heightAtCenter;
[JsonInclude]
public double HeightAtCenter
{
get =>_heightAtCenter;
set { if (value > 0) { _heightAtCenter = value; } }
}
private double _heightOnSide;
[JsonInclude]
public double HeightOnSide
{
get =>_heightOnSide;
set { if (value > 0) { _heightOnSide = value; } }
}
[JsonInclude]
public Material Material { get; set; }
[JsonConstructor]
public Layer() { }
/// <summary>
/// Cr�e une nouvelle couche avec la mati�re donn�e et une longueur et un largeur uniforme.
/// </summary>
/// <param name="material">Mati�re de la couche</param>
/// <param name="width">Largeur au centre et sur les c�t� de la couche</param>
/// <param name="height">Hauteur au centre et sur les c�t� de la couche</param>
public Layer(Material material, double width, double height)
{
Material = material;
WidthAtCenter=width;
WidthOnSide=width;
HeightAtCenter=height;
HeightOnSide = height;
}
/// <summary>
/// Cr�e une nouvelle couche avec la mati�re donn�e et une longueur et un largeur non-uniforme.
/// </summary>
/// <param name="material">Mati�re de la couche</param>
/// <param name="widthCenter">Largeur au centre de la couche</param>
/// <param name="widthSide">Largeur sur les c�t� de la couche</param>
/// <param name="heightCenter">Hauteur au centre de la couche</param>
/// <param name="heightSide">Hauteur sur les c�t� de la couche</param>
public Layer(Material material, double widthCenter, double widthSide, double heightCenter, double heightSide)
{
Material = material;
WidthAtCenter = widthCenter;
WidthOnSide=widthSide;
HeightAtCenter=heightCenter;
HeightOnSide=heightSide;
}
/// <summary>
/// Retourne un r�sum� de la couche
/// </summary>
/// <returns>Le nom de la mati�re, la largeur au centre, la largeur sur les c�t�s, la hauteur au centre, la hauteur sur les c�t�s</returns>
public override string ToString()
{
return $"{Material.Nom} M={WidthAtCenter * 1000}x{HeightAtCenter * 1000} C={WidthOnSide * 1000}x{HeightOnSide * 1000}";
}
public double[] Base(double longueur, double eref, double[] xs)
{
double l1 = (4 * WidthOnSide - 4 * WidthAtCenter) / Math.Pow(longueur, 2);
double[] l2 = AdditionalMath.OperationDoubleArray(xs, longueur / 2, AdditionalMath.Operation.Minus);
l2 = AdditionalMath.OperationDoubleArray(l2, 2, AdditionalMath.Operation.Power);
double[] baseArea = AdditionalMath.OperationDoubleArray(l2, l1, AdditionalMath.Operation.Multiplication);
baseArea = AdditionalMath.OperationDoubleArray(baseArea, WidthAtCenter, AdditionalMath.Operation.Plus);
double divider = eref / Material.E;
return AdditionalMath.OperationDoubleArray(baseArea, divider, AdditionalMath.Operation.Divided);
}
private IEnumerable<double> Width(double longueur, double eref, IEnumerable<double> xs)
{
double l1 = (4 * WidthOnSide - 4 * WidthAtCenter) / Math.Pow(longueur, 2);
List<double> L2 = xs.Select(x => Math.Pow(x - longueur / 2, 2)).ToList();
List<double> Lf = L2.Select(l2 => l1 * l2 + WidthAtCenter).ToList();
return Lf.Select(lf => lf / eref * Material.E).ToList();
}
public List<double> Height(double longueur, IEnumerable<double> xs)
{
double e1 = (4 * HeightOnSide - 4 * HeightAtCenter) / Math.Pow(longueur, 2);
List<double> e2 = xs.Select(x => Math.Pow(x - longueur / 2, 2)).ToList();
return e2.Select(l2 => e1 * l2 + HeightAtCenter).ToList();
}
public List<double> Surface(double length, double eref, double[] xs)
{
IEnumerable<double> widths = Width(length, eref, xs);
List<double> heights = Height(length, xs);
return widths.Select((t, i) => t * heights[i]).ToList();
}
}
} |
'use client';
import { ArrowLeftIcon, ArrowRightIcon } from '@heroicons/react/24/outline';
import clsx from 'clsx';
import Link from 'next/link';
import { generatePagination } from '@/app/lib/utils';
import { usePathname, useSearchParams } from 'next/navigation';
export default function Pagination({ totalPages }: { totalPages: number }) {
const pathname = usePathname();
const searchParams = useSearchParams();
const currentPage = Number(searchParams.get('page')) || 1;
const createPageURL = (pageNumber: number | string) => {
const params = new URLSearchParams(searchParams);
params.set('page', pageNumber.toString());
return `${pathname}?${params.toString()}`;
};
// NOTE: comment in this code when you get to this point in the course
const allPages = generatePagination(currentPage, totalPages);
return (
<>
{/* NOTE: comment in this code when you get to this point in the course */}
<div className="inline-flex">
<PaginationArrow
direction="left"
href={createPageURL(currentPage - 1)}
isDisabled={currentPage <= 1}
/>
<div className="flex -space-x-px">
{allPages.map((page, index) => {
let position: 'first' | 'last' | 'single' | 'middle' | undefined;
if (index === 0) position = 'first';
if (index === allPages.length - 1) position = 'last';
if (allPages.length === 1) position = 'single';
if (page === '...') position = 'middle';
return (
<PaginationNumber
key={page}
href={createPageURL(page)}
page={page}
position={position}
isActive={currentPage === page}
/>
);
})}
</div>
<PaginationArrow
direction="right"
href={createPageURL(currentPage + 1)}
isDisabled={currentPage >= totalPages}
/>
</div>
</>
);
}
function PaginationNumber({
page,
href,
isActive,
position,
}: {
page: number | string;
href: string;
position?: 'first' | 'last' | 'middle' | 'single';
isActive: boolean;
}) {
const className = clsx(
'flex h-10 w-10 items-center justify-center text-sm border',
{
'rounded-l-md': position === 'first' || position === 'single',
'rounded-r-md': position === 'last' || position === 'single',
'z-10 bg-blue-600 border-blue-600 text-white': isActive,
'hover:bg-gray-100': !isActive && position !== 'middle',
'text-gray-300': position === 'middle',
},
);
return isActive || position === 'middle' ? (
<div className={className}>{page}</div>
) : (
<Link href={href} className={className}>
{page}
</Link>
);
}
function PaginationArrow({
href,
direction,
isDisabled,
}: {
href: string;
direction: 'left' | 'right';
isDisabled?: boolean;
}) {
const className = clsx(
'flex h-10 w-10 items-center justify-center rounded-md border',
{
'pointer-events-none text-gray-300': isDisabled,
'hover:bg-gray-100': !isDisabled,
'mr-2 md:mr-4': direction === 'left',
'ml-2 md:ml-4': direction === 'right',
},
);
const icon =
direction === 'left' ? (
<ArrowLeftIcon className="w-4" />
) : (
<ArrowRightIcon className="w-4" />
);
return isDisabled ? (
<div className={className}>{icon}</div>
) : (
<Link className={className} href={href}>
{icon}
</Link>
);
} |
package com.taller.votos.entities;
import lombok.Data;
import javax.persistence.Entity;
import javax.persistence.Table;
import javax.validation.constraints.Email;
import javax.validation.constraints.Size;
import javax.validation.constraints.NotNull;
import javax.persistence.Id;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Column;
@Entity
@Data
@Table(name="admins")
public class AdminEntity {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
@Column(name = "id")
private Long id;
@Column(name = "name", nullable = false, length = 40)
@NotNull(message = "El nombre no puede estar vacío")
@Size(min = 1, max = 40)
private String name;
@Column(name = "email", nullable = false, unique = true)
@Email(message = "El campo debe ser un correo válido")
private String email;
@Column(name = "password", nullable = false)
@NotNull(message = "La contraseña no puede estar vacía")
private String password;
} |
import { EntityType, SeatType, Side } from "sketchbook/core/enums"
import * as Utils from "sketchbook/core/FunctionLibrary"
import { IControllable } from "sketchbook/core/interfaces"
import { SpringSimulator } from "sketchbook/physics"
import * as THREE from "three"
import { Object3D } from "three"
import { Character } from "../Character"
import { VehicleSeat } from "../vehicles/VehicleSeat"
import { Driving } from "./Driving"
import { Sitting } from "./Sitting"
import { CharacterStateBase } from "./_stateLibrary"
export class EnteringVehicle extends CharacterStateBase {
private vehicle: IControllable
private animData: any
private seat: VehicleSeat
private initialPositionOffset: THREE.Vector3 = new THREE.Vector3()
private startPosition: THREE.Vector3 = new THREE.Vector3()
private endPosition: THREE.Vector3 = new THREE.Vector3()
private startRotation: THREE.Quaternion = new THREE.Quaternion()
private endRotation: THREE.Quaternion = new THREE.Quaternion()
private factorSimulator: SpringSimulator
constructor(character: Character, seat: VehicleSeat, entryPoint: Object3D) {
super(character)
this.canFindVehiclesToEnter = false
this.vehicle = seat.vehicle
this.seat = seat
const side = Utils.detectRelativeSide(entryPoint, seat.seatPointObject)
this.animData = this.getEntryAnimations(seat.vehicle.entityType)
this.playAnimation(this.animData[side], 0.1)
this.character.resetVelocity()
this.character.tiltContainer.rotation.z = 0
this.character.setPhysicsEnabled(false)
; (this.seat.vehicle as unknown as THREE.Object3D).attach(this.character)
this.startPosition.copy(entryPoint.position)
this.startPosition.y += 0.53
this.endPosition.copy(seat.seatPointObject.position)
this.endPosition.y += 0.6
this.initialPositionOffset
.copy(this.startPosition)
.sub(this.character.position)
this.startRotation.copy(this.character.quaternion)
this.endRotation.copy(this.seat.seatPointObject.quaternion)
this.factorSimulator = new SpringSimulator(60, 10, 0.5)
this.factorSimulator.target = 1
}
public update(timeStep: number): void {
super.update(timeStep)
if (this.animationEnded(timeStep)) {
this.character.occupySeat(this.seat)
this.character.setPosition(
this.endPosition.x,
this.endPosition.y,
this.endPosition.z
)
if (this.seat.type === SeatType.Driver) {
if (this.seat.door) this.seat.door.physicsEnabled = true
this.character.setState(new Driving(this.character, this.seat))
} else if (this.seat.type === SeatType.Passenger) {
this.character.setState(new Sitting(this.character, this.seat))
}
} else {
if (this.seat.door) {
this.seat.door.physicsEnabled = false
this.seat.door.rotation = 1
}
let factor = THREE.MathUtils.clamp(
this.timer / (this.animationLength - this.animData.end_early),
0,
1
)
let sineFactor = Utils.easeInOutSine(factor)
this.factorSimulator.simulate(timeStep)
let currentPosOffset = new THREE.Vector3().lerpVectors(
this.initialPositionOffset,
new THREE.Vector3(),
this.factorSimulator.position
)
let lerpPosition = new THREE.Vector3().lerpVectors(
this.startPosition.clone().sub(currentPosOffset),
this.endPosition,
sineFactor
)
this.character.setPosition(lerpPosition.x, lerpPosition.y, lerpPosition.z)
THREE.Quaternion.slerp(
this.startRotation,
this.endRotation,
this.character.quaternion,
this.factorSimulator.position
)
}
}
private getEntryAnimations(type: EntityType): any {
switch (type) {
case EntityType.Airplane:
return {
[Side.Left]: "enter_airplane_left",
[Side.Right]: "enter_airplane_right",
end_early: 0.3
}
default:
return {
[Side.Left]: "sit_down_left",
[Side.Right]: "sit_down_right",
end_early: 0.0
}
}
}
} |
/*
* @Author: YauCheun [email protected]
* @Date: 2024-03-02 16:33:42
* @LastEditors: YauCheun [email protected]
* @LastEditTime: 2024-03-02 16:35:59
* @FilePath: \nestjs-study\src\upload\upload.module.ts
* @Description: 这是默认设置,请设置`customMade`, 打开koroFileHeader查看配置 进行设置: https://github.com/OBKoro1/koro1FileHeader/wiki/%E9%85%8D%E7%BD%AE
*/
import { Module } from '@nestjs/common';
import { UploadService } from './upload.service';
import { UploadController } from './upload.controller';
//文件上传需要的包
import { MulterModule } from '@nestjs/platform-express';
import { diskStorage } from 'multer';
import { join, extname } from 'path';
@Module({
//里面有register 和 registerAsync 两个方法,前者是同步的,后者是异步的
imports: [MulterModule.register({
//图片上传完要存放的位置
storage: diskStorage({
destination: join(__dirname, '../images'),//存放的文件路径
filename: (req, file, callback) => {
//重新定义文件名,file.originalname 文件的原始名称
// extname 获取文件后缀
const fileName = `${new Date().getTime() + extname(file.originalname)}`;
//返回新的名称,参数1是错误,这里用null就好
return callback(null, fileName)
}
}),
}
)],
controllers: [UploadController],
providers: [UploadService]
})
export class UploadModule { } |
package com.example.gjucampus
import android.os.Bundle
import androidx.fragment.app.Fragment
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.Button
import android.widget.EditText
import android.widget.Toast
import com.google.firebase.auth.FirebaseAuth
import com.google.firebase.auth.FirebaseAuthException
import com.google.firebase.auth.EmailAuthProvider
class Password_change : Fragment() {
private lateinit var etCurrentPassword: EditText
private lateinit var etNewPassword: EditText
private lateinit var etConfirmPassword: EditText
private lateinit var btnChangePassword: Button
override fun onCreateView(
inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
val view = inflater.inflate(R.layout.fragment_password_change, container, false)
etCurrentPassword = view.findViewById(R.id.current_pass)
etNewPassword = view.findViewById(R.id.update_pass)
etConfirmPassword = view.findViewById(R.id.confirmPassEt)
btnChangePassword = view.findViewById(R.id.button)
btnChangePassword.setOnClickListener {
val currentPassword = etCurrentPassword.text.toString()
val newPassword = etNewPassword.text.toString()
val confirmPassword = etConfirmPassword.text.toString()
if (newPassword == confirmPassword) {
changePassword(currentPassword, newPassword)
} else {
showToast("New password and confirm password do not match")
}
}
return view
}
private fun changePassword(currentPassword: String, newPassword: String) {
val auth = FirebaseAuth.getInstance()
val user = auth.currentUser
val credential = user?.email?.let {
EmailAuthProvider.getCredential(it, currentPassword)
}
if (credential != null) {
user?.reauthenticate(credential)?.addOnCompleteListener { reauthTask ->
if (reauthTask.isSuccessful) {
user.updatePassword(newPassword).addOnCompleteListener { updateTask ->
if (updateTask.isSuccessful) {
showToast("Password updated successfully")
} else {
val exception = updateTask.exception as? FirebaseAuthException
showToast("Password update failed: ${exception?.message}")
}
}
} else {
showToast("Reauthentication failed")
}
}
}
}
private fun showToast(message: String) {
Toast.makeText(requireContext(), message, Toast.LENGTH_SHORT).show()
}
} |
#!/usr/bin/env python
#
# A library that provides a Python interface to the Telegram Bot API
# Copyright (C) 2015-2021
# Leandro Toledo de Souza <[email protected]>
#
# This program is free software: you can redistribute it and/or modify
# it under the terms of the GNU Lesser Public License as published by
# the Free Software Foundation, either version 3 of the License, or
# (at your option) any later version.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU Lesser Public License for more details.
#
# You should have received a copy of the GNU Lesser Public License
# along with this program. If not, see [http://www.gnu.org/licenses/].
"""This module contains an object that represents a location to which a chat is connected."""
from typing import TYPE_CHECKING, Any, Optional
from telegram import TelegramObject
from telegram.utils.types import JSONDict
from .files.location import Location
if TYPE_CHECKING:
from telegram import Bot
class ChatLocation(TelegramObject):
"""This object represents a location to which a chat is connected.
Objects of this class are comparable in terms of equality. Two objects of this class are
considered equal, if their :attr:`location` is equal.
Args:
location (:class:`telegram.Location`): The location to which the supergroup is connected.
Can't be a live location.
address (:obj:`str`): Location address; 1-64 characters, as defined by the chat owner
**kwargs (:obj:`dict`): Arbitrary keyword arguments.
Attributes:
location (:class:`telegram.Location`): The location to which the supergroup is connected.
address (:obj:`str`): Location address, as defined by the chat owner
"""
def __init__(
self,
location: Location,
address: str,
**_kwargs: Any,
):
self.location = location
self.address = address
self._id_attrs = (self.location,)
@classmethod
def de_json(cls, data: Optional[JSONDict], bot: 'Bot') -> Optional['ChatLocation']:
data = cls.parse_data(data)
if not data:
return None
data['location'] = Location.de_json(data.get('location'), bot)
return cls(bot=bot, **data) |
# Clear workspace
rm(list = ls())
# Setup
################################################################################
# Packages
library(tidyverse)
# Directories
indir <- "/Users/cfree/Dropbox/ca_set_gillnet_bycatch/confidential/obs_state/raw"
outdir <- "/Users/cfree/Dropbox/ca_set_gillnet_bycatch/confidential/obs_state/processed"
plotdir <- "/Users/cfree/Dropbox/ca_set_gillnet_bycatch/confidential/obs_state/figures"
keydir <- "data/keys"
# Read data
data_orig <- read.csv(file.path(indir, "GNC8389.csv"), as.is=T, na.strings="")
# Read keys
port_key <- readRDS(file.path(keydir, "CDFW_port_key.Rds"))
spp_key <- readRDS(file.path(keydir, "CDFW_species_key.Rds"))
spp_key2 <- readRDS("/Users/cfree/Dropbox/ca_set_gillnet_bycatch/confidential/obs_federal/processed/SWFSC_observer_program_spp_key.Rds")
# Format data
################################################################################
# Format data
data <- data_orig %>%
# Rename
janitor::clean_names("snake") %>%
rename(vessel_id=fgno,
set_num=setno,
spp_code_chr=species,
n_caught=tcat,
n_discarded_dead=ddead,
n_discarded_alive=dlive,
n_damaged=nodam,
n_kept=nokept,
n_kept_sublegal=nokeptsl,
n_sold=nosold) %>%
# Format date
mutate(date=lubridate::ymd(date)) %>%
# Format number discarded alive
mutate(n_discarded_alive=ifelse(n_discarded_alive %in% c("m", "M"), 0, n_discarded_alive),
n_discarded_alive=as.numeric(n_discarded_alive)) %>%
# Format number damaged
mutate(n_damaged=ifelse(n_damaged %in% c("S", "M"), 0, n_damaged),
n_damaged=as.numeric(n_damaged)) %>%
# Format number kept
mutate(n_kept=ifelse(n_kept %in% c("S") | is.na(n_kept), 0, n_kept),
n_kept=as.numeric(n_kept)) %>%
# Format number kept sublegal
mutate(n_kept_sublegal=ifelse(is.na(n_kept_sublegal), 0, n_kept_sublegal)) %>%
# Format number sold
mutate(n_sold=ifelse(is.na(n_sold), 0, n_sold)) %>%
# Add species name (fix a few codes first)
mutate(spp_code_chr=recode(spp_code_chr,
"PPn"="pPN",
"aV"="aVE")) %>%
left_join(spp_key2 %>% select(spp_code, comm_name), by=c("spp_code_chr"="spp_code")) %>%
# Fill in missing common name
mutate(comm_name=case_when(spp_code_chr=="152" ~ "Spiny dogfish shark",
TRUE ~ comm_name)) %>%
# Add set id
mutate(set_id=paste(date, vessel_id, set_num, sep="-")) %>%
# Check data
mutate(n_caught_calc=n_discarded_dead+n_discarded_alive+n_kept+n_kept_sublegal+n_sold, # n_damaged is redundant
n_check=n_caught-n_caught_calc) %>%
# select(-c(n_caught_calc, n_check)) %>%
# Arrange
select(date, vessel_id, set_num, set_id, spp_code_chr, comm_name, everything()) %>%
arrange(date, vessel_id, set_num, set_id, comm_name)
# Inspect data
################################################################################
# Inspect
str(data)
freeR::complete(data)
# Data
range(data$date)
# File links
sort(unique(data$m_file_link))
sort(unique(data$s_file_link))
# Inspect species
data_spp <- data %>%
# Unique species
select(spp_code_chr, comm_name) %>%
unique() %>%
# Arrange
arrange(spp_code_chr)
data_spp$spp_code_chr
# See if any species are duplicated within a set
check1 <- data %>%
count(set_id, comm_name) %>%
filter(n>1)
# Finalize data
################################################################################
# Finalize
data_out <- data %>%
# Simplify
select(-c(m_file_link, s_file_link, n_caught_calc, n_check)) %>%
# Summarize results across set and species
group_by(date, vessel_id, set_num, set_id, spp_code_chr, comm_name) %>%
summarize(across(where(is.numeric), sum), .groups = 'drop')
# Inspect
freeR::complete(data_out)
# Export data
################################################################################
# Export
saveRDS(data_out, file=file.path(outdir, "CDFW_1983_1989_gillnet_observer_data.Rds")) |
function [source] = sourceanalysis(cfg, data, baseline);
% SOURCEANALYSIS performs beamformer dipole analysis on EEG or MEG data
% after preprocessing and a timelocked or frequency analysis
%
% Use as either
% [source] = sourceanalysis(cfg, freq)
% [source] = sourceanalysis(cfg, timelock)
%
% where the data in freq or timelock should be organised in a structure
% as obtained from the FREQANALYSIS or TIMELOCKANALYSIS function. The
% configuration "cfg" is a structure containing information about
% source positions and other options.
%
% The different source reconstruction algorithms that are implemented
% are
% cfg.method = 'lcmv' linear constrained minimum variance beamformer
% 'sam' synthetic aperture magnetometry
% 'dics' dynamic imaging of coherent sources
% 'pcc' partial cannonical correlation/coherence
% 'mne' minimum norm estimation
% 'loreta' minimum norm estimation with smoothness constraint
% 'rv' scan residual variance with single dipole
% 'music' multiple signal classification
% 'mvl' multivariate Laplace source localization
% The DICS and PCC methods are for frequency domain data, all other methods
% are for time domain data.
%
% The positions of the sources can be specified as a regular 3-D
% grid that is aligned with the axes of the head coordinate system
% cfg.grid.xgrid = vector (e.g. -20:1:20) or 'auto' (default = 'auto')
% cfg.grid.ygrid = vector (e.g. -20:1:20) or 'auto' (default = 'auto')
% cfg.grid.zgrid = vector (e.g. 0:1:20) or 'auto' (default = 'auto')
% cfg.grid.resolution = number (e.g. 1 cm) for automatic grid generation
% Alternatively the position of a few sources at locations of interest can
% be specified, for example obtained from an anatomical or functional MRI
% cfg.grid.pos = Nx3 matrix with position of each source
% cfg.grid.dim = [Nx Ny Nz] vector with dimensions in case of 3-D grid (optional)
% cfg.grid.inside = vector with indices of the sources inside the brain (optional)
% cfg.grid.outside = vector with indices of the sources outside the brain (optional)
% You can also use the PREPARE_LEADFIELD function to create a grid with
% dipole positions and with precomputed leadfields.
%
% The following strategies are supported to obtain statistics for the source parameters using
% multiple trials in the data, either directly or through a resampling-based approach
% cfg.singletrial = 'no' or 'yes' construct filter from average, apply to single trials
% cfg.rawtrial = 'no' or 'yes' construct filter from single trials, apply to single trials
% cfg.jackknife = 'no' or 'yes' jackknife resampling of trials
% cfg.pseudovalue = 'no' or 'yes' pseudovalue resampling of trials
% cfg.bootstrap = 'no' or 'yes' bootstrap resampling of trials
% cfg.numbootstrap = number of bootstrap replications (e.g. number of original trials)
% If none of these options is specified, the average over the trials will
% be computed prior to computing the source reconstruction.
%
% To obtain statistics over the source parameters between two conditions, you
% can also use a resampling procedure that reshuffles the trials over both
% conditions. In that case, you should call the function with two datasets
% containing single trial data like
% [source] = sourceanalysis(cfg, freqA, freqB)
% [source] = sourceanalysis(cfg, timelockA, timelockB)
% and you should specify
% cfg.randomization = 'no' or 'yes'
% cfg.permutation = 'no' or 'yes'
% cfg.numrandomization = number, e.g. 500
% cfg.numpermutation = number, e.g. 500 or 'all'
%
% You should specify the volume conductor model with
% cfg.hdmfile = string, file containing the volume conduction model
% or alternatively
% cfg.vol = structure with volume conduction model
%
% If the sensor information is not contained in the data itself you should
% also specify the sensor information using
% cfg.gradfile = string, file containing the gradiometer definition
% cfg.elecfile = string, file containing the electrode definition
% or alternatively
% cfg.grad = structure with gradiometer definition
% cfg.elec = structure with electrode definition
%
% If you have not specified a grid with pre-computed leadfields,
% the leadfield for each grid location will be computed on the fly.
% In that case you can modify the leadfields by reducing the rank
% (i.e. remove the weakest orientation), or by normalizing each
% column.
% cfg.reducerank = 'no', or number (default = 3 for EEG, 2 for MEG)
% cfg.normalize = 'no' or 'yes' (default = 'no')
%
% Other configuration options are
% cfg.channel = Nx1 cell-array with selection of channels (default = 'all'),
% see CHANNELSELECTION for details
% cfg.frequency = single number (in Hz)
% cfg.latency = single number in seconds, for time-frequency analysis
% cfg.lambda = number or empty for automatic default
% cfg.refchan = reference channel label (for coherence)
% cfg.refdip = reference dipole location (for coherence)
% cfg.supchan = suppressed channel label(s)
% cfg.supdip = suppressed dipole location(s)
% cfg.keeptrials = 'no' or 'yes'
% cfg.keepleadfield = 'no' or 'yes'
% cfg.projectnoise = 'no' or 'yes'
% cfg.keepfilter = 'no' or 'yes'
% cfg.keepcsd = 'no' or 'yes'
% cfg.keepmom = 'no' or 'yes'
% cfg.feedback = 'no', 'text', 'textbar', 'gui' (default = 'text')
%
% See also SOURCEDESCRIPTIVES, SOURCESTATISTICS, PREPARE_LEADFIELD
% Undocumented local options:
% cfg.numcomponents
% cfg.refchannel
% cfg.trialweight = 'equal' or 'proportional'
% cfg.powmethod = 'lambda1' or 'trace'
%
% This function depends on PREPARE_DIPOLE_GRID which has the following options:
% cfg.grid.xgrid (default set in PREPARE_DIPOLE_GRID: cfg.grid.xgrid = 'auto'), documented
% cfg.grid.ygrid (default set in PREPARE_DIPOLE_GRID: cfg.grid.ygrid = 'auto'), documented
% cfg.grid.zgrid (default set in PREPARE_DIPOLE_GRID: cfg.grid.zgrid = 'auto'), documented
% cfg.grid.resolution, documented
% cfg.grid.pos, documented
% cfg.grid.dim, documented
% cfg.grid.inside, documented
% cfg.grid.outside, documented
% cfg.mri
% cfg.mriunits
% cfg.smooth
% cfg.sourceunits
% cfg.threshold
% cfg.symmetry
%
% This function depends on PREPARE_FREQ_MATRICES which has the following options:
% cfg.channel (default set in SOURCEANALYSIS: cfg.channel = 'all'), documented
% cfg.dicsfix
% cfg.frequency, documented
% cfg.latency, documented
% cfg.refchan, documented
%
% This function depends on PREPARE_RESAMPLED_DATA which has the following options:
% cfg.jackknife (default set in SOURCEANALYSIS: cfg.jackknife = 'no'), documented
% cfg.numbootstrap, documented
% cfg.numcondition (set in SOURCEANALYSIS: cfg.numcondition = 2)
% cfg.numpermutation (default set in SOURCEANALYSIS: cfg.numpermutation = 100), documented
% cfg.numrandomization (default set in SOURCEANALYSIS: cfg.numrandomization = 100), documented
% cfg.permutation (default set in SOURCEANALYSIS: cfg.permutation = 'no'), documented
% cfg.pseudovalue (default set in SOURCEANALYSIS: cfg.pseudovalue = 'no'), documented
% cfg.randomization (default set in SOURCEANALYSIS: cfg.randomization = 'no'), documented
%
% This function depends on PREPARE_VOL_SENS which has the following options:
% cfg.channel, (default set in SOURCEANALYSIS: cfg.channel = 'all'), documented
% cfg.elec, documented
% cfg.elecfile, documented
% cfg.grad, documented
% cfg.gradfile, documented
% cfg.hdmfile, documented
% cfg.order
% cfg.vol, documented
%
% This function depends on PREPARE_LEADFIELD which has the following options:
% cfg.feedback, (default set in SOURCEANALYSIS: cfg.feedback = 'text'), documented
% cfg.grid, documented
% cfg.lbex
% cfg.normalize (default set in SOURCEANALYSIS), documented
% cfg.previous
% cfg.reducerank (default set in SOURCEANALYSIS), documented
% cfg.sel50p
% cfg.version
% Copyright (c) 2003-2008, Robert Oostenveld, F.C. Donders Centre
%
% $Log: not supported by cvs2svn $
% Revision 1.140 2009/06/04 13:37:28 marvger
% changed mvlap name to mvl to make it consistent with literature
%
% Revision 1.139 2009/05/20 16:53:30 marvger
% added support for mvlap method (tentative)
%
% Revision 1.138 2009/03/26 13:32:12 roboos
% added SAM as beamformer method
% fixed bug in the default assignment of reducerank, which for some MEG systems caused the reducerank default to be 3 instead of 2 (which is preferred)
%
% Revision 1.137 2009/03/11 11:27:34 roboos
% added a comment and removed a now obsolete channelselection call
%
% Revision 1.136 2009/03/06 13:02:59 sashae
% changed default cfg.projectnoise='yes' to 'no'
%
% Revision 1.135 2009/02/06 10:53:10 jansch
% added experimental possibility to apply prewhitening. fixed incorrect
% conjugate transposition and replaced by explicit transpose()
%
% Revision 1.134 2009/02/05 10:22:07 roboos
% better support for single-trial data, thanks to Vladimir
%
% Revision 1.133 2009/01/20 13:01:31 sashae
% changed configtracking such that it is only enabled when BOTH explicitly allowed at start
% of the fieldtrip function AND requested by the user
% in all other cases configtracking is disabled
%
% Revision 1.132 2009/01/19 12:29:51 roboos
% preallocate the fake covariance matrix in case it is absent in the data
% ensure that length(size(cov))==2 in case of Ntrials==1 (i.e. when keeptrials=yes and only one trial in the data)
%
% Revision 1.131 2008/11/21 13:21:35 sashae
% added call to checkconfig at start and end of fucntion
%
% Revision 1.130 2008/10/02 15:32:21 sashae
% replaced call to createsubcfg with checkconfig
%
% Revision 1.129 2008/09/26 12:42:18 sashae
% checkconfig: checks if the input cfg is valid for this function
%
% Revision 1.128 2008/09/22 20:17:44 roboos
% added call to fieldtripdefs to the begin of the function
%
% Revision 1.127 2008/07/15 19:56:44 roboos
% moved cfg details for dipole grid to subcfg (cfg.grid)subcfg (cfg.grid.xxx)
%
% Revision 1.126 2008/07/07 12:37:42 roboos
% fixed bug in channelordering in case sens.label and data.label were inconsistent
%
% Revision 1.125 2008/04/10 08:03:11 roboos
% renamed the fieldtrip/private/prepare_vol_sens function into prepare_headmodel
%
% Revision 1.124 2007/05/15 07:01:29 roboos
% updated help
%
% Revision 1.123 2007/04/03 15:37:07 roboos
% renamed the checkinput function to checkdata
%
% Revision 1.122 2007/03/30 17:05:40 ingnie
% checkinput; only proceed when input data is allowed datatype
%
% Revision 1.121 2007/03/27 11:05:19 ingnie
% changed call to fixdimord in call to checkinput
%
% Revision 1.120 2007/03/05 15:31:37 roboos
% added empty defaults for refchan/supchan in case of method=pcc
%
% Revision 1.119 2006/10/19 15:19:14 roboos
% switched to using beamformer_lcmv and beamformer_dics for the specific methods
%
% Revision 1.118 2006/10/12 13:04:34 roboos
% updated documentation
%
% Revision 1.117 2006/10/04 08:10:51 roboos
% changed default for cfg.reducerank, new default is 2 for MEG and 3 for EEG
%
% Revision 1.116 2006/10/04 07:10:07 roboos
% updated documentation
%
% Revision 1.115 2006/10/02 13:01:21 roboos
% also recognize rpttap as part of the dimord
%
% Revision 1.114 2006/08/16 08:23:36 roboos
% updated documentation
%
% Revision 1.113 2006/07/05 10:34:52 roboos
% minor change in documentation
%
% Revision 1.112 2006/07/05 10:32:14 roboos
% minor change in documentation
%
% Revision 1.111 2006/07/05 10:26:11 roboos
% updated documentation, the cfg.xgrid/ygrid/zgrid options have moved to cfg.grid substructure
% removed default 'auto' values for xgrid/ygrid/zgrid (were similar to the default values in prepare_dipole_grid)
%
% Revision 1.110 2006/07/04 17:06:23 ingnie
% updated documentation
%
% Revision 1.109 2006/07/04 16:04:50 roboos
% renamed option 'jacknife' into 'jackknife' for consistency, maintain backward compatibility with cfgs and old data
%
% Revision 1.108 2006/06/26 09:26:03 ingnie
% fixed bug (forgotten "end")
%
% Revision 1.107 2006/06/22 12:27:41 roboos
% optionally pass the covariance as input argument to MUSIC
%
% Revision 1.106 2006/06/20 16:25:56 ingnie
% updated documentation
%
% Revision 1.105 2006/06/14 11:55:07 roboos
% switched from construct_optarg() subfunction to the new createsubcfg function
%
% Revision 1.104 2006/06/13 14:48:09 ingnie
% updated documentation
%
% Revision 1.103 2006/05/23 10:15:31 roboos
% Changed the initial construction of the dipole grid, either with or
% without precomputed leadfields (there was a case in which the automatic
% grid would not be tight). Improved documentation around the disabled
% section for singletrial=yes. Some other small changes.
%
% Revision 1.102 2006/05/10 08:14:09 roboos
% removed support for parallelization on cluster, only precompute leadfields
% if not already present, implemented subfunction construct_optarg() for
% clean and consistent construction of key-value arguments for the low-level
% inverse methods, allow additional arguments to be passed transparently
% to the low-level inverse methods by means of cfg.xxx.key=value, where
% xxx=pcc/lcmv/dics/rv/music/loreta/mne
%
% Revision 1.101 2006/05/03 15:10:11 roboos
% only define submethod in the case of method=dics
%
% Revision 1.100 2006/04/20 09:58:34 roboos
% updated documentation
%
% Revision 1.99 2006/04/12 08:38:01 ingnie
% updated documentation
%
% Revision 1.98 2006/04/10 16:33:46 ingnie
% updated documentation
%
% Revision 1.97 2006/04/06 16:17:10 ingnie
% updated documentation
%
% Revision 1.96 2006/03/22 07:53:06 roboos
% small change in documentation
%
% Revision 1.95 2006/03/09 08:19:06 roboos
% apply fixdimord to the baseline condition data if present
%
% Revision 1.94 2006/02/24 16:41:39 roboos
% changed foi and toi into freq and time for frequency data
%
% Revision 1.93 2006/02/23 10:28:16 roboos
% changed dimord strings for consistency, changed toi and foi into time and freq, added fixdimord where neccessary
%
% Revision 1.92 2006/02/07 22:21:01 roboos
% changed the xgrid/ygrid/zgrid and dim in the output source structure (from cfg to grid), they can be different from the cfg since prepare_dipole_grid will make the box tight
%
% Revision 1.91 2006/02/07 20:08:23 roboos
% changed all occurences of a dimord with chancmb (was previous sgncmb) into chan
%
% Revision 1.90 2006/02/01 12:26:00 roboos
% made all uses of dimord consistent with the common definition of data dimensions, see the fixdimord() function
%
% Revision 1.89 2006/01/24 20:51:57 roboos
% fixed obvious typo
%
% Revision 1.88 2006/01/24 20:02:50 roboos
% renamed dics_cohrefdip and cohrefchan into cfg.method=dics (backwards compatible)
% added extra local variable "submethod" that indicates the details for dics
%
% Revision 1.87 2006/01/24 14:28:33 roboos
% small change in documentation
%
% Revision 1.86 2005/11/24 16:25:16 roboos
% added average or single trial ERF to the input of PCC beamformer in timedomain
%
% Revision 1.85 2005/11/16 09:07:03 roboos
% added option cfg.powmethod to determine whether lambda1 or trace is used in beamformer
%
% Revision 1.84 2005/11/08 11:07:13 roboos
% added support for normalize and reducerank, by passing these options on to the beamformer subfunction
%
% Revision 1.83 2005/09/29 00:45:00 roboos
% added multiple signal classification as reconstruction technique for timelocked data
%
% Revision 1.82 2005/10/25 08:42:56 roboos
% changed the detection of either frequency or timelock data using flags (isfreq, iscomp, istimelock) instead of by renaming the input data object to freq/timelock
% renamed the freq/timelock variable throughout the code into "data"
% added support for freq/comp data to the non-beamforming timelocked source reconstruction methods
%
% Revision 1.81 2005/10/14 15:47:03 roboos
% add an identity covariance matrix to timelocked data if not present
% some cosmetic changes
%
% Revision 1.80 2005/10/05 11:14:11 roboos
% updated documentation
% added support for LORETA (still experimental)
% removed all code that referred to unimplemented parallelization for some of the reconstruction methods
%
% Revision 1.79 2005/09/05 06:36:56 jansch
% keep cumtapcnt of freq-input in output
%
% Revision 1.78 2005/08/16 13:15:55 jansch
% *** empty log message ***
%
% Revision 1.77 2005/08/16 12:43:03 jansch
% included possibility to have 'rpttap_sgncmb_frq' as input
%
% Revision 1.76 2005/06/29 12:46:29 roboos
% the following changes were done on a large number of files at the same time, not all of them may apply to this specific file
% - added try-catch around the inclusion of input data configuration
% - moved cfg.version, cfg.previous and the assignment of the output cfg to the end
% - changed help comments around the configuration handling
% - some changes in whitespace
%
% Revision 1.75 2005/06/17 09:01:13 roboos
% moved implementation of keepmom and keepcsd over to beamformer subfunction
%
% Revision 1.74 2005/05/17 17:56:38 roboos
% changed all "if" occurences of & and | into && and ||
% this makes the code more compatible with Octave and also seems to be in closer correspondence with Matlab documentation on shortcircuited evaluation of sequential boolean constructs
% implemented support for projecting the single trial fft matrix through the filters of beamformer_pcc
%
% Revision 1.73 2005/04/25 16:05:11 roboos
% includedupdated options for cortex segmentation based grid in the documentation (not in main documentation)
%
% Revision 1.72 2005/03/03 13:57:29 roboos
% implemented pseudovalue resampling, i.e. a combination of jackknife and the complete average
%
% Revision 1.71 2005/03/03 10:57:43 roboos
% Renamed the source analysis methods, the submethods for dics are now
% recognized by their additional required fields (refchan/refdip). Added
% support for experimental pcc method (not yet implemented in a clean way),
% pcc is now a re3cognised submethod of the standard beamformer function.
% Included mne method in the documentation.
%
% Revision 1.70 2005/02/14 21:53:14 roboos
% changed assignment of empty struct to dip into 'clear dip' (for mentat-style parallelization)
%
% Revision 1.69 2005/01/26 08:38:50 roboos
% extended and fixed the experimental partcanoncorr code
%
% Revision 1.68 2005/01/11 12:43:03 roboos
% added temporary hack to support partcanoncorr as a beamformer method
%
% Revision 1.67 2005/01/10 17:26:46 roboos
% added support for 2nd method of parallelizing the code, separated
% two methods by the name of the backend toolbox (beowulf or mentat)
%
% Revision 1.66 2004/12/08 18:00:13 roboos
% implemented consistent method of selecting a subset of channels for
% forward and inverse computations using cfg.channel and updated the
% ducumentation
%
% Revision 1.65 2004/10/21 09:01:34 roboos
% added numcondition=2 for averaging two conditions while resampling
%
% Revision 1.64 2004/09/28 08:01:03 roboos
% added residual variance scanning with single dipole (cfg.method=rv)
% added 'yes' as allowed value for cfg.parallel
%
% Revision 1.63 2004/09/21 14:30:07 roboos
% fixed bug in DICS/power for two conditions (Nbaseline~=Ntrials)
%
% Revision 1.62 2004/09/21 13:22:15 roboos
% in the previous revision (1.61) initial support for MNE was added but accidentally not logged in CVS
% this revision only adds a single comment line with the undocumented method=mne option
%
% Revision 1.61 2004/09/21 13:15:32 roboos
% *** empty log message ***
%
% Revision 1.60 2004/09/21 12:49:00 roboos
% fixed a bug in averaging of 2 input conditions using prepare_resampled_data (using cfg2)
%
% Revision 1.59 2004/09/14 16:28:49 roboos
% incorporated the changes on the "exp-resampling-parallel" branch
% new implementation of resampling/randomization
% new implementation of parallellization
%
% Revision 1.58.4.5 2004/09/08 16:36:00 roboos
% fixed many bugs that stopped execution through rigorous testing
% correctness of the output still has to be evaluated
% non-proportional trial weighing is not implemented yet
%
% Revision 1.58.4.4 2004/09/08 12:25:13 roboos
% auto-indented code using matlab editor, fixed one incorrect "end"
%
% Revision 1.58.4.3 2004/09/08 09:35:49 roboos
% implemented prepare_resampled_data and new evalwulf for DICS, some related changes to LCMV
%
% Revision 1.58.4.2 2004/09/06 16:32:28 roboos
% implemented new prepare_resampled_data for a few resampling strategies and lcmv only, untested
%
% Revision 1.58.4.1 2004/09/06 16:10:26 roboos
% disabled parallelization over grid (still in code)
% moved documentation for paralellization to hidden location
% fixed bug in jackknife+lcmv
%
% Revision 1.58 2004/08/16 12:14:33 roboos
% fixed small bug that was introduced in the previous update
%
% Revision 1.57 2004/08/16 10:53:09 roboos
% fixed bug for lcmv together with randomization (trial assignment was oubviously wrong)
%
% Revision 1.56 2004/08/16 10:01:23 roboos
% updated help, fixed bug in output assignment with permutation, changed handling of keepleadfield and precomputed leadfields
%
% Revision 1.55 2004/08/06 12:23:04 roboos
% fixed small bug in default setting for cfg.permutation
%
% Revision 1.54 2004/08/05 07:14:25 roboos
% implemented permutation analogous to randomization, updated and restructured help
%
% Revision 1.53 2004/08/05 06:37:29 roboos
% extra removal of leadfield in output source and cfg, removed old revision comments
%
% Revision 1.52 2004/08/04 11:53:03 roboos
% updated the help
%
% Revision 1.51 2004/06/09 10:07:19 roberto
% added version information and input data configuration to output cfg
%
% Revision 1.50 2004/03/01 11:01:55 roberto
% added precomputed filters to grid and using these, implemented singletrial for dics
%
% Revision 1.49 2004/02/05 12:19:36 roberto
% moved the code that was common with dipolefitting into separate prepare_grid and prepare_sens_vol functions
% also renamed internal subfunction freq_to_matrices to prepare_freq_matrices and moved into separate function
%
% Revision 1.48 2004/02/02 10:48:11 roberto
% fixed two bugs in randomization for lcmv & timelocked data
%
% Revision 1.47 2004/01/26 09:04:54 roberto
% fixed serious bug in selection of spheres for multi-sphere volume model
% selection was based on wrong indices, causing reference magnetometer spheres
% and gradiometer spheres to be mixed up
%
% Revision 1.46 2004/01/22 10:50:55 roberto
% fixed bug in cfg.keepmom
% improved handling of pre-computation of leadfield without scanning
%
% Revision 1.45 2004/01/21 13:07:52 roberto
% added a fprintf-line to indicate the progress in randomization (which takes quite some time)
%
% Revision 1.44 2004/01/20 09:09:45 roberto
% added computation of leadfield in case not provided and multiple trials have to
% be scanned
%
% Revision 1.43 2004/01/14 14:29:43 roberto
% changed handling of sgncmbindx etc inside freq_to_matrices subfunction
% mainly cosmetical changes, but also fixed bug in refindx for pow_refchan
%
% Revision 1.42 2004/01/14 08:50:05 roberto
% changed the name of output variables for randomization
%
% Revision 1.41 2004/01/08 09:25:35 roberto
% canged cfg.label into freq.sgn inside freq_to_matrices subfunction to fix bug
%
% Revision 1.40 2004/01/08 09:14:49 roberto
% added option keepmom to save memory, default is 'yes'
fieldtripdefs
% set a timer to determine how long the sourceanalysis takes in total
tic;
cfg = checkconfig(cfg, 'trackconfig', 'on');
% check if the input data is valid for this function
data = checkdata(data, 'datatype', {'timelock', 'freq', 'comp'}, 'feedback', 'yes');
if nargin>2
baseline = checkdata(baseline, 'datatype', {'timelock', 'freq', 'comp'}, 'feedback', 'yes');
end
% check if the input cfg is valid for this function
cfg = checkconfig(cfg, 'renamed', {'jacknife', 'jackknife'});
cfg = checkconfig(cfg, 'renamed', {'refchannel', 'refchan'});
cfg = checkconfig(cfg, 'renamedval', {'method', 'power', 'dics'});
cfg = checkconfig(cfg, 'renamedval', {'method', 'coh_refchan', 'dics'});
cfg = checkconfig(cfg, 'renamedval', {'method', 'coh_refdip', 'dics'});
cfg = checkconfig(cfg, 'renamedval', {'method', 'dics_cohrefchan', 'dics'});
cfg = checkconfig(cfg, 'renamedval', {'method', 'dics_cohrefdip', 'dics'});
cfg = checkconfig(cfg, 'forbidden', {'parallel'});
% determine the type of input data
if isfield(data, 'freq')
isfreq = 1;
iscomp = 0;
istimelock = 0;
elseif isfield(data, 'topo')
isfreq = 0;
iscomp = 1;
istimelock = 0;
elseif isfield(data, 'time')
iscomp = 0;
isfreq = 0;
istimelock = 1;
else
error('input data is not recognized');
end
% set the defaults
if ~isfield(cfg, 'method') && istimelock, cfg.method = 'lcmv'; end
if ~isfield(cfg, 'method') && isfreq, cfg.method = 'dics'; end
if ~isfield(cfg, 'keeptrials') cfg.keeptrials = 'no'; end
if ~isfield(cfg, 'keepfilter') cfg.keepfilter = 'no'; end
if ~isfield(cfg, 'keepleadfield') cfg.keepleadfield = 'no'; end
if ~isfield(cfg, 'keepcsd') cfg.keepcsd = 'no'; end
if ~isfield(cfg, 'keepmom') cfg.keepmom = 'yes'; end
if ~isfield(cfg, 'projectnoise') cfg.projectnoise = 'no'; end
if ~isfield(cfg, 'trialweight') cfg.trialweight = 'equal'; end
if ~isfield(cfg, 'jackknife'), cfg.jackknife = 'no'; end
if ~isfield(cfg, 'pseudovalue'), cfg.pseudovalue = 'no'; end
if ~isfield(cfg, 'bootstrap'), cfg.bootstrap = 'no'; end
if ~isfield(cfg, 'singletrial'), cfg.singletrial = 'no'; end
if ~isfield(cfg, 'rawtrial'), cfg.rawtrial = 'no'; end
if ~isfield(cfg, 'randomization'), cfg.randomization = 'no'; end
if ~isfield(cfg, 'numrandomization'), cfg.numrandomization = 100; end
if ~isfield(cfg, 'permutation'), cfg.permutation = 'no'; end
if ~isfield(cfg, 'numpermutation'), cfg.numpermutation = 100; end
if ~isfield(cfg, 'wakewulf'), cfg.wakewulf = 'yes'; end
if ~isfield(cfg, 'killwulf'), cfg.killwulf = 'yes'; end
if ~isfield(cfg, 'feedback'), cfg.feedback = 'text'; end
if ~isfield(cfg, 'supdip'), cfg.supdip = []; end
if ~isfield(cfg, 'lambda'), cfg.lambda = []; end
if ~isfield(cfg, 'powmethod'), cfg.powmethod = []; end
if ~isfield(cfg, 'channel'), cfg.channel = 'all'; end
if ~isfield(cfg, 'normalize'), cfg.normalize = 'no'; end
if ~isfield(cfg, 'prewhiten'), cfg.prewhiten = 'no'; end
% if ~isfield(cfg, 'reducerank'), cfg.reducerank = 'no'; end % the default for this depends on EEG/MEG and is set below
% put the low-level options pertaining to the source reconstruction method in their own field
% put the low-level options pertaining to the dipole grid in their own field
cfg = checkconfig(cfg, 'createsubcfg', {cfg.method, 'grid'});
convertfreq = 0;
convertcomp = 0;
if ~istimelock && (strcmp(cfg.method, 'mne') || strcmp(cfg.method, 'loreta') || strcmp(cfg.method, 'rv') || strcmp(cfg.method, 'music'))
% these timelock methods are also supported for frequency or component data
if isfreq
[data, cfg] = freq2timelock(cfg, data);
convertfreq = 1; % flag indicating that the data was converted
elseif iscomp
[data, cfg] = comp2timelock(cfg, data);
convertcomp = 1; % flag indicating that the data was converted
end
istimelock = 1; % from now on the data can be treated as timelocked
isfreq = 0;
iscomp = 0;
end
% select only those channels that are present in the data
cfg.channel = channelselection(cfg.channel, data.label);
if nargin>2 && (strcmp(cfg.randomization, 'no') && strcmp(cfg.permutation, 'no') && strcmp(cfg.prewhiten, 'no'))
error('input of two conditions only makes sense if you want to randomize or permute, or if you want to prewhiten');
elseif nargin<3 && (strcmp(cfg.randomization, 'yes') || strcmp(cfg.permutation, 'yes'))
error('randomization or permutation requires that you give two conditions as input');
end
if isfield(cfg, 'latency') && istimelock
error('specification of cfg.latency is only required for time-frequency data');
end
if sum([strcmp(cfg.jackknife, 'yes'), strcmp(cfg.bootstrap, 'yes'), strcmp(cfg.pseudovalue, 'yes'), strcmp(cfg.singletrial, 'yes'), strcmp(cfg.rawtrial, 'yes'), strcmp(cfg.randomization, 'yes'), strcmp(cfg.permutation, 'yes')])>1
error('jackknife, bootstrap, pseudovalue, singletrial, rawtrial, randomization and permutation are mutually exclusive');
end
if isfreq
if ~strcmp(data.dimord, 'chan_freq') && ...
~strcmp(data.dimord, 'chan_freq_time') && ...
~strcmp(data.dimord, 'rpt_chan_freq') && ...
~strcmp(data.dimord, 'rpt_chan_freq_time') && ...
~strcmp(data.dimord, 'rpttap_chan_freq') && ...
~strcmp(data.dimord, 'rpttap_chan_freq_time')
error('dimord of input frequency data is not recognized');
end
end
% collect and preprocess the electrodes/gradiometer and head model
[vol, sens, cfg] = prepare_headmodel(cfg, data);
% It might be that the number of channels in the data, the number of
% channels in the electrode/gradiometer definition and the number of
% channels in the multisphere volume conduction model are different.
% Hence a subset of the data channels will be used.
Nchans = length(cfg.channel);
% set the default for reducing the rank of the leadfields, this is an
% option to the specific method and will be passed on to the low-level
% function
if ~isfield(cfg.(cfg.method), 'reducerank')
if senstype(sens, 'meg')
cfg.(cfg.method).reducerank = 2;
else
cfg.(cfg.method).reducerank = 3;
end
end
if strcmp(cfg.keepleadfield, 'yes') && (~isfield(cfg, 'grid') || ~isfield(cfg.grid, 'leadfield'))
% precompute the leadfields upon the users request
fprintf('precomputing leadfields\n');
[grid, cfg] = prepare_leadfield(cfg, data);
elseif (strcmp(cfg.permutation, 'yes') || ...
strcmp(cfg.randomization, 'yes') || ...
strcmp(cfg.bootstrap, 'yes') || ...
strcmp(cfg.jackknife, 'yes') || ...
strcmp(cfg.pseudovalue, 'yes') || ...
strcmp(cfg.singletrial, 'yes') || ...
strcmp(cfg.rawtrial, 'yes')) && (~isfield(cfg, 'grid') || ~isfield(cfg.grid, 'leadfield'))
% also precompute the leadfields if multiple trials have to be processed
fprintf('precomputing leadfields for efficient handling of multiple trials\n');
[grid, cfg] = prepare_leadfield(cfg, data);
else
% only prepare the grid positions, the leadfield will be computed on the fly if not present
[grid, cfg] = prepare_dipole_grid(cfg, vol, sens);
end
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% do frequency domain source reconstruction
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
if isfreq && any(strcmp(cfg.method, {'dics', 'pcc'}))
if strcmp(cfg.method, 'pcc')
% HACK: requires some extra defaults
if ~isfield(cfg, 'refdip'), cfg.refdip = []; end
if ~isfield(cfg, 'supdip'), cfg.supdip = []; end
if ~isfield(cfg, 'refchan'), cfg.refchan = []; end
if ~isfield(cfg, 'supchan'), cfg.supchan = []; end
cfg.refchan = channelselection(cfg.refchan, data.label);
cfg.supchan = channelselection(cfg.supchan, data.label);
% HACK: use some experimental code
if nargin>2 && strcmp(cfg.prewhiten, 'no'),
error('not supported')
end
tmpcfg = cfg;
tmpcfg.refchan = ''; % prepare_freq_matrices should not know explicitly about the refchan
tmpcfg.channel = cfg.channel(:)';
if isfield(cfg, 'refchan')
% add the refchan implicitely
tmpcfg.channel = [tmpcfg.channel cfg.refchan(:)'];
end
if isfield(cfg, 'supchan')
% add the supchan implicitely
tmpcfg.channel = [tmpcfg.channel cfg.supchan(:)'];
end
% select the data in the channels and the frequency of interest
[Cf, Cr, Pr, Ntrials, tmpcfg] = prepare_freq_matrices(tmpcfg, data);
if strcmp(cfg.prewhiten, 'yes'),
[Cfb, Crb, Prb, Ntrialsb, tmpcfgb] = prepare_freq_matrices(tmpcfg, baseline);
Cf = prewhitening_filter2(squeeze(mean(Cf,1)), squeeze(mean(Cfb,1)));
Ntrials = 1;
end
if isfield(cfg, 'refchan') && ~isempty(cfg.refchan)
[dum, refchanindx] = match_str(cfg.refchan, tmpcfg.channel);
else
refchanindx = [];
end
if isfield(cfg, 'supchan') && ~isempty(cfg.supchan)
[dum, supchanindx] = match_str(cfg.supchan,tmpcfg.channel);
else
supchanindx = [];
end
Nchans = length(tmpcfg.channel); % update the number of channels
% if the input data has a complete fourier spectrum, project it through the filters
% FIXME it was incorrect , since the
% ' leads to a conjugate transposition check this in beamformer_pcc
if isfield(data, 'fourierspctrm')
[dum, datchanindx] = match_str(tmpcfg.channel, data.label);
fbin = nearest(data.freq, cfg.frequency);
if strcmp(data.dimord, 'chan_freq')
avg = data.fourierspctrm(datchanindx, fbin);
elseif strcmp(data.dimord, 'rpt_chan_freq') || strcmp(data.dimord, 'rpttap_chan_freq'),
%avg = data.fourierspctrm(:, datchanindx, fbin)';
avg = transpose(data.fourierspctrm(:, datchanindx, fbin));
elseif strcmp(data.dimord, 'chan_freq_time')
tbin = nearest(data.time, cfg.latency);
avg = data.fourierspctrm(datchanindx, fbin, tbin);
elseif strcmp(data.dimord, 'rpt_chan_freq_time') || strcmp(data.dimord, 'rpttap_chan_freq_time'),
tbin = nearest(data.time, cfg.latency);
%avg = data.fourierspctrm(:, datchanindx, fbin, tbin)';
avg = transpose(data.fourierspctrm(:, datchanindx, fbin, tbin));
end
else
avg = [];
end
else
% HACK: use the default code
% convert the input data, so that Cf, Cr and Pr contain either the average over all trials (if Ntrials==1)
% or the individual cross-spectral-densities/powers of each individual trial (if Ntrials>1)
[Cf, Cr, Pr, Ntrials, cfg] = prepare_freq_matrices(cfg, data);
end
if strcmp(cfg.method, 'dics')
% assign a descriptive name to each of the dics sub-methods, the default is power only
if strcmp(cfg.method, 'dics') && isfield(cfg, 'refdip') && ~isempty(cfg.refdip);
submethod = 'dics_refdip';
elseif strcmp(cfg.method, 'dics') && isfield(cfg, 'refchan') && ~isempty(cfg.refchan);
submethod = 'dics_refchan';
else
submethod = 'dics_power';
end
end
% fill these with NaNs, so that I dont have to treat them separately
if isempty(Cr), Cr = nan*zeros(Ntrials, Nchans, 1); end
if isempty(Pr), Pr = nan*zeros(Ntrials, 1, 1); end
if nargin>2
% repeat the conversion for the baseline condition
[bCf, bCr, bPr, Nbaseline, cfg] = prepare_freq_matrices(cfg, baseline);
% fill these with NaNs, so that I dont have to treat them separately
if isempty(bCr), bCr = nan*zeros(Nbaseline, Nchans, 1); end
if isempty(bPr), bPr = nan*zeros(Nbaseline, 1, 1); end
% rename the active condition for convenience
aCf = Cf;
aCr = Cr;
aPr = Pr;
% this is required for averaging 2 conditions using prepare_resampled_data
cfg2 = [];
cfg2.numcondition = 2;
% this is required for randomizing/permuting 2 conditions using prepare_resampled_data
cfg.numcondition = 2;
end
% prepare the resampling of the trials, or average the data if multiple trials are present and no resampling is neccessary
if (Ntrials<=1) && (strcmp(cfg.jackknife, 'yes') || strcmp(cfg.bootstrap, 'yes') || strcmp(cfg.pseudovalue, 'yes') || strcmp(cfg.singletrial, 'yes') || strcmp(cfg.rawtrial, 'yes') || strcmp(cfg.randomization, 'yes') || strcmp(cfg.permutation, 'yes'))
error('multiple trials required in the data\n');
elseif strcmp(cfg.permutation, 'yes')
% compute the cross-spectral density matrix without resampling
[dum, avg_aCf, avg_aCr, avg_aPr, avg_bCf, avg_bCr, avg_bPr] = prepare_resampled_data(cfg2 , aCf, aCr, aPr, bCf, bCr, bPr);
% compute the cross-spectral density matrix with random permutation
[dum, rnd_aCf, rnd_aCr, rnd_aPr, rnd_bCf, rnd_bCr, rnd_bPr] = prepare_resampled_data(cfg, aCf, aCr, aPr, bCf, bCr, bPr);
% concatenate the different resamplings
Cf = cat(1, reshape(avg_aCf, [1 Nchans Nchans]), reshape(avg_bCf, [1 Nchans Nchans]), rnd_aCf, rnd_bCf);
Cr = cat(1, reshape(avg_aCr, [1 Nchans 1 ]), reshape(avg_bCr, [1 Nchans 1 ]), rnd_aCr, rnd_bCr);
Pr = cat(1, reshape(avg_aPr, [1 1 1 ]), reshape(avg_bPr, [1 1 1 ]), rnd_aPr, rnd_bPr);
% clear temporary working copies
clear avg_aCf avg_aCr avg_aPr avg_bCf avg_bCr avg_bPr
clear rnd_aCf rnd_aCr rnd_aPr rnd_bCf rnd_bCr rnd_bPr
% the order of the resamplings should be [avgA avgB rndA rndB rndA rndB rndA rndB ....]
Nrepetitions = 2*cfg.numpermutation + 2;
order = [1 2 3:2:Nrepetitions 4:2:Nrepetitions];
Cf = Cf(order,:,:);
Cr = Cr(order,:,:);
Pr = Pr(order,:,:);
elseif strcmp(cfg.randomization, 'yes')
% compute the cross-spectral density matrix without resampling
[dum, avg_aCf, avg_aCr, avg_aPr, avg_bCf, avg_bCr, avg_bPr] = prepare_resampled_data(cfg2 , aCf, aCr, aPr, bCf, bCr, bPr);
% compute the cross-spectral density matrix with random resampling
[dum, rnd_aCf, rnd_aCr, rnd_aPr, rnd_bCf, rnd_bCr, rnd_bPr] = prepare_resampled_data(cfg, aCf, aCr, aPr, bCf, bCr, bPr);
% concatenate the different resamplings
Cf = cat(1, reshape(avg_aCf, [1 Nchans Nchans]), reshape(avg_bCf, [1 Nchans Nchans]), rnd_aCf, rnd_bCf);
Cr = cat(1, reshape(avg_aCr, [1 Nchans 1 ]), reshape(avg_bCr, [1 Nchans 1 ]), rnd_aCr, rnd_bCr);
Pr = cat(1, reshape(avg_aPr, [1 1 1 ]), reshape(avg_bPr, [1 1 1 ]), rnd_aPr, rnd_bPr);
% clear temporary working copies
clear avg_aCf avg_aCr avg_aPr avg_bCf avg_bCr avg_bPr
clear rnd_aCf rnd_aCr rnd_aPr rnd_bCf rnd_bCr rnd_bPr
% the order of the resamplings should be [avgA avgB rndA rndB rndA rndB rndA rndB ....]
Nrepetitions = 2*cfg.numrandomization + 2;
order = [1 2 3:2:Nrepetitions 4:2:Nrepetitions];
Cf = Cf(order,:,:);
Cr = Cr(order,:,:);
Pr = Pr(order,:,:);
elseif strcmp(cfg.jackknife, 'yes')
% compute the cross-spectral density matrix with jackknife resampling
[cfg, Cf, Cr, Pr] = prepare_resampled_data(cfg, Cf, Cr, Pr);
Nrepetitions = Ntrials;
elseif strcmp(cfg.bootstrap, 'yes')
% compute the cross-spectral density matrix with bootstrap resampling
[cfg, Cf, Cr, Pr] = prepare_resampled_data(cfg, Cf, Cr, Pr);
Nrepetitions = cfg.numbootstrap;
elseif strcmp(cfg.pseudovalue, 'yes')
% compute the cross-spectral density matrix with pseudovalue resampling
[cfg, Cf, Cr, Pr] = prepare_resampled_data(cfg, Cf, Cr, Pr);
Nrepetitions = Ntrials+1;
elseif strcmp(cfg.singletrial, 'yes')
% The idea is that beamformer uses the average covariance to construct the
% filter and applies it to the single trial covariance/csd. The problem
% is that beamformer will use the averaged covariance/csd to estimate the
% power and not the single trial covariance/csd
error('this option contains a bug, and is therefore not supported at the moment');
Cf = Cf; % FIXME, should be averaged and repeated for each trial
Cr = Cr; % FIXME, should be averaged and repeated for each trial
Pr = Pr; % FIXME, should be averaged and repeated for each trial
Nrepetitions = Ntrials;
elseif strcmp(cfg.rawtrial, 'yes')
% keep all the individual trials, do not average them
Cf = Cf;
Cr = Cr;
Pr = Pr;
Nrepetitions = Ntrials;
elseif Ntrials>1
% compute the average from the individual trials
Cf = reshape(sum(Cf, 1) / Ntrials, [Nchans Nchans]);
Cr = reshape(sum(Cr, 1) / Ntrials, [Nchans 1]);
Pr = reshape(sum(Pr, 1) / Ntrials, [1 1]);
Nrepetitions = 1;
elseif Ntrials==1
% no rearrangement of trials is neccesary, the data already represents the average
Cf = Cf;
Cr = Cr;
Pr = Pr;
Nrepetitions = 1;
end
% reshape so that it also looks like one trial (out of many)
if Nrepetitions==1
Cf = reshape(Cf , [1 Nchans Nchans]);
Cr = reshape(Cr , [1 Nchans 1]);
Pr = reshape(Pr , [1 1 1]);
end
% get the relevant low level options from the cfg and convert into key-value pairs
optarg = cfg2keyval(getfield(cfg, cfg.method));
for i=1:Nrepetitions
fprintf('scanning repetition %d\n', i);
if strcmp(cfg.method, 'dics') && strcmp(submethod, 'dics_power')
dip(i) = beamformer_dics(grid, sens, vol, [], squeeze(Cf(i,:,:)), optarg{:});
elseif strcmp(cfg.method, 'dics') && strcmp(submethod, 'dics_refchan')
dip(i) = beamformer_dics(grid, sens, vol, [], squeeze(Cf(i,:,:)), optarg{:}, 'Cr', Cr(i,:), 'Pr', Pr(i));
elseif strcmp(cfg.method, 'dics') && strcmp(submethod, 'dics_refdip')
dip(i) = beamformer_dics(grid, sens, vol, [], squeeze(Cf(i,:,:)), optarg{:}, 'refdip', cfg.refdip);
elseif strcmp(cfg.method, 'pcc')
dip(i) = beamformer_pcc(grid, sens, vol, avg, squeeze(Cf(i,:,:)), optarg{:}, 'refdip', cfg.refdip, 'refchan', refchanindx, 'supdip', cfg.supdip, 'supchan', supchanindx);
else
error(sprintf('method ''%s'' is unsupported for source reconstruction in the frequency domain', cfg.method));
end
end
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% do time domain source reconstruction
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
elseif istimelock && any(strcmp(cfg.method, {'lcmv', 'sam', 'mne', 'loreta', 'rv', 'music', 'pcc', 'mvl'}))
% determine the size of the data
Nsamples = size(data.avg,2);
Nchans = length(data.label);
if isfield(data, 'cov') && length(size(data.cov))==3
Ntrials = size(data.cov,1);
elseif isfield(data, 'trial') && length(size(data.trial))==3
Ntrials = size(data.trial,1);
else
Ntrials = 1;
end
if isfield(data, 'cov')
% use the estimated data covariance matrix
hascovariance = 1;
else
% add a identity covariance matrix, this simplifies the handling of the different source reconstruction methods
% since the covariance is only used by some reconstruction methods and might not allways be present in the data
if Ntrials==1
data.cov = eye(Nchans);
else
data.cov = zeros(Ntrials,Nchans,Nchans);
for i=1:Ntrials
data.cov(i,:,:) = eye(Nchans);
end
end
hascovariance = 0;
end
if strcmp(cfg.method, 'pcc')
% HACK: requires some extra defaults
if ~isfield(cfg, 'refdip'), cfg.refdip = []; end
if ~isfield(cfg, 'supdip'), cfg.supdip = []; end
% HACK: experimental code
if nargin>2
error('not supported')
end
tmpcfg = [];
tmpcfg.channel = cfg.channel(:)';
if isfield(cfg, 'refchan')
tmpcfg.channel = [tmpcfg.channel cfg.refchan(:)'];
end
if isfield(cfg, 'supchan')
tmpcfg.channel = [tmpcfg.channel cfg.supchan(:)'];
end
% select the data in the channels of interest
[dum, datchanindx] = match_str(tmpcfg.channel, data.label);
if Ntrials==1
data.avg = data.avg(datchanindx,:);
data.cov = data.cov(datchanindx,datchanindx);
else
data.avg = data.avg(datchanindx,:);
data.cov = data.cov(:,datchanindx,datchanindx);
data.trial = data.trial(:,datchanindx,:);
end
data.label = data.label(datchanindx);
if isfield(cfg, 'refchan') && ~isempty(cfg.refchan)
[dum, refchanindx] = match_str(cfg.refchan, data.label);
else
refchanindx = [];
end
if isfield(cfg, 'supchan') && ~isempty(cfg.supchan)
[dum, supchanindx] = match_str(cfg.supchan, data.label);
else
supchanindx = [];
end
Nchans = length(tmpcfg.channel); % update the number of channels
else
% HACK: use the default code
% select the channels of interest
[dum, datchanindx] = match_str(cfg.channel, data.label);
if strcmp(data.dimord, 'chan_time')
% It is in principle possible to have timelockanalysis with
% keeptrial=yes and only a single trial in the raw data.
% In that case the covariance should be represented as Nchan*Nchan
data.avg = data.avg(datchanindx,:);
data.cov = reshape(data.cov, length(datchanindx), length(datchanindx));
data.cov = data.cov(datchanindx,datchanindx);
else
data.avg = data.avg(datchanindx,:);
data.cov = data.cov(:,datchanindx,datchanindx);
data.trial = data.trial(:,datchanindx,:);
end
data.label = data.label(datchanindx);
Nchans = length(data.label);
end
if nargin>2
% baseline and active are only available together for resampling purposes,
% hence I assume here that there are multiple trials in both
baseline.avg = baseline.avg(datchanindx,:);
baseline.cov = baseline.cov(:,datchanindx,datchanindx);
baseline.trial = baseline.trial(:,datchanindx,:);
% this is required for averaging 2 conditions using prepare_resampled_data
cfg2 = [];
cfg2.numcondition = 2;
end
% prepare the resampling of the trials, or average the data if multiple trials are present and no resampling is neccessary
if (strcmp(cfg.jackknife, 'yes') || strcmp(cfg.bootstrap, 'yes') || strcmp(cfg.pseudovalue, 'yes') || strcmp(cfg.singletrial, 'yes') || strcmp(cfg.rawtrial, 'yes') || strcmp(cfg.randomization, 'yes')) && ~strcmp(data.dimord, 'rpt_chan_time')
error('multiple trials required in the data\n');
elseif strcmp(cfg.permutation, 'yes')
% compute the average and covariance without resampling
[dum, avgA, covA, avgB, covB] = prepare_resampled_data(cfg2 , data.trial, data.cov, baseline.trial, baseline.cov);
% compute the average and covariance with random permutation
[cfg, avRA, coRA, avRB, coRB] = prepare_resampled_data(cfg, data.trial, data.cov, baseline.trial, baseline.cov);
% concatenate the different resamplings
avg = cat(1, reshape(avgA, [1 Nchans Nsamples]), reshape(avgB, [1 Nchans Nsamples]), avRA, avRB);
Cy = cat(1, reshape(covA, [1 Nchans Nchans ]), reshape(covB, [1 Nchans Nchans ]), coRA, coRB);
% clear temporary working copies
clear avgA avgB covA covB
clear avRA avRB coRA coRB
% the order of the resamplings should be [avgA avgB randA randB randA randB randA randB ....]
Nrepetitions = 2*cfg.numpermutation + 2;
order = [1 2 3:2:Nrepetitions 4:2:Nrepetitions];
avg = avg(order,:,:);
Cy = Cy (order,:,:);
elseif strcmp(cfg.randomization, 'yes')
% compute the average and covariance without resampling
[dum, avgA, covA, avgB, covB] = prepare_resampled_data(cfg2 , data.trial, data.cov, baseline.trial, baseline.cov);
% compute the average and covariance with random resampling
[cfg, avRA, coRA, avRB, coRB] = prepare_resampled_data(cfg, data.trial, data.cov, baseline.trial, baseline.cov);
% concatenate the different resamplings
avg = cat(1, reshape(avgA, [1 Nchans Nsamples]), reshape(avgB, [1 Nchans Nsamples]), avRA, avRB);
Cy = cat(1, reshape(covA, [1 Nchans Nchans ]), reshape(covB, [1 Nchans Nchans ]), coRA, coRB);
% clear temporary working copies
clear avgA avgB covA covB
clear avRA avRB coRA coRB
% the order of the resamplings should be [avgA avgB randA randB randA randB randA randB ....]
Nrepetitions = 2*cfg.numrandomization + 2;
order = [1 2 3:2:Nrepetitions 4:2:Nrepetitions];
avg = avg(order,:,:);
Cy = Cy (order,:,:);
elseif strcmp(cfg.jackknife, 'yes')
% compute the jackknife repetitions for the average and covariance
[cfg, avg, Cy] = prepare_resampled_data(cfg, data.trial, data.cov);
Nrepetitions = Ntrials;
elseif strcmp(cfg.bootstrap, 'yes')
% compute the bootstrap repetitions for the average and covariance
[cfg, avg, Cy] = prepare_resampled_data(cfg, data.trial, data.cov);
Nrepetitions = cfg.numbootstrap;
elseif strcmp(cfg.pseudovalue, 'yes')
% compute the pseudovalue repetitions for the average and covariance
[cfg, avg, Cy] = prepare_resampled_data(cfg, data.trial, data.cov);
Nrepetitions = Ntrials+1;
elseif strcmp(cfg.singletrial, 'yes')
% The idea is that beamformer uses the average covariance to construct the
% filter and applies it to the single trial covariance/csd. The problem
% is that beamformer will use the averaged covariance/csd to estimate the
% power and not the single trial covariance/csd
error('this option contains a bug, and is therefore not supported at the moment');
% average the single-trial covariance matrices
Cy = mean(data.cov,1);
% copy the average covariance matrix for every individual trial
Cy = repmat(Cy, [Ntrials 1 1]);
% keep the single-trial ERFs, rename them to avg for convenience
avg = data.trial;
Nrepetitions = Ntrials;
elseif strcmp(cfg.rawtrial, 'yes')
% do not do any resampling, keep the single-trial covariances
Cy = data.cov;
% do not do any resampling, keep the single-trial ERFs (rename them to avg for convenience)
avg = data.trial;
Nrepetitions = Ntrials;
elseif Ntrials>1
% average the single-trial covariance matrices
Cy = reshape(mean(data.cov,1), [Nchans Nchans]);
% select the average ERF
avg = data.avg;
Nrepetitions = 1;
elseif Ntrials==1
% select the average covariance matrix
Cy = data.cov;
% select the average ERF
avg = data.avg;
Nrepetitions = 1;
end
% reshape so that it also looks like one trial (out of many)
if Nrepetitions==1
Cy = reshape(Cy , [1 Nchans Nchans]);
avg = reshape(avg, [1 Nchans Nsamples]);
end
% get the relevant low level options from the cfg and convert into key-value pairs
optarg = cfg2keyval(getfield(cfg, cfg.method));
if strcmp(cfg.method, 'lcmv')
for i=1:Nrepetitions
fprintf('scanning repetition %d\n', i);
dip(i) = beamformer_lcmv(grid, sens, vol, squeeze(avg(i,:,:)), squeeze(Cy(i,:,:)), optarg{:});
end
elseif strcmp(cfg.method, 'sam')
for i=1:Nrepetitions
fprintf('scanning repetition %d\n', i);
dip(i) = beamformer_sam(grid, sens, vol, squeeze(avg(i,:,:)), squeeze(Cy(i,:,:)), optarg{:});
end
elseif strcmp(cfg.method, 'pcc')
for i=1:Nrepetitions
fprintf('scanning repetition %d\n', i);
dip(i) = beamformer_pcc(grid, sens, vol, squeeze(avg(i,:,:)), squeeze(Cy(i,:,:)), optarg{:}, 'refdip', cfg.refdip, 'refchan', refchanindx, 'supchan', supchanindx);
end
elseif strcmp(cfg.method, 'mne')
for i=1:Nrepetitions
fprintf('estimating current density distribution for repetition %d\n', i);
dip(i) = minimumnormestimate(grid, sens, vol, squeeze(avg(i,:,:)), optarg{:});
end
elseif strcmp(cfg.method, 'loreta')
for i=1:Nrepetitions
fprintf('estimating LORETA current density distribution for repetition %d\n', i);
dip(i) = loreta( grid, sens, vol, squeeze(avg(i,:,:)), optarg{:});
end
elseif strcmp(cfg.method, 'rv')
for i=1:Nrepetitions
fprintf('estimating residual variance at each grid point for repetition %d\n', i);
dip(i) = residualvariance( grid, sens, vol, squeeze(avg(i,:,:)), optarg{:});
end
elseif strcmp(cfg.method, 'music')
for i=1:Nrepetitions
fprintf('computing multiple signal classification for repetition %d\n', i);
if hascovariance
dip(i) = music(grid, sens, vol, squeeze(avg(i,:,:)), 'cov', squeeze(Cy(i,:,:)), optarg{:});
else
dip(i) = music(grid, sens, vol, squeeze(avg(i,:,:)), optarg{:});
end
end
elseif strcmp(cfg.method, 'mvl')
for i=1:Nrepetitions
fprintf('estimating current density distribution for repetition %d\n', i);
fns = fieldnames(cfg);
optarg = cell(1,length(fns));
n=1;
for c=1:length(fns)
optarg{n} = fns{c};
optarg{n+1} = cfg.(fns{c});
n=n+2;
end
dip(i) = mvlestimate(grid, sens, vol, squeeze(avg(i,:,:)), optarg{:});
end
else
error(sprintf('method ''%s'' is unsupported for source reconstruction in the time domain', cfg.method));
end
end % if freq or timelock data
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% clean up and collect the results
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
if isfield(grid, 'xgrid')
% the dipoles are placed on a regular grid that is aligned with the carthesian axes
% copy the description of the grid axes
source.xgrid = grid.xgrid;
source.ygrid = grid.ygrid;
source.zgrid = grid.zgrid;
source.dim = [length(source.xgrid) length(source.ygrid) length(source.zgrid)];
end
source.vol = vol;
if exist('grad', 'var')
source.grad = grad;
elseif exist('elec', 'var')
source.elec = elec;
end
if istimelock
% add the time axis to the output
source.time = data.time;
elseif iscomp
% FIXME, add the component numbers to the output
elseif isfreq
% add the frequency axis to the output
cfg.frequency = data.freq(nearest(data.freq, cfg.frequency));
source.frequency = cfg.frequency;
if isfield(data, 'time') && isfield(cfg, 'latency')
cfg.latency = data.time(nearest(data.time, cfg.latency));
source.latency = cfg.latency;
end
if isfield(data, 'cumtapcnt'),
source.cumtapcnt = data.cumtapcnt;
end
end
if exist('dip', 'var')
% do some cleaning up, keep the dipole positions etc. in the global structure and not in each trial
source.pos = dip(1).pos;
source.inside = dip(1).inside;
source.outside = dip(1).outside;
dip = rmfield(dip, 'pos');
dip = rmfield(dip, 'inside');
dip = rmfield(dip, 'outside');
if isfield(dip(1), 'leadfield')
source.leadfield = dip(1).leadfield;
dip = rmfield(dip, 'leadfield');
end
elseif exist('grid', 'var')
% no scanning has been done, probably only the leadfield has been computed
try, source.pos = grid.pos; end
try, source.inside = grid.inside; end
try, source.outside = grid.outside; end
try, source.leadfield = grid.leadfield; end
end
if strcmp(cfg.keepleadfield, 'yes') && ~isfield(source, 'leadfield')
% add the precomputed leadfields to the output source
source.leadfield = grid.leadfield;
elseif strcmp(cfg.keepleadfield, 'no') && isfield(source, 'leadfield')
% remove the precomputed leadfields from the output source
source = rmfield(source, 'leadfield');
end
% remove the precomputed leadfields from the cfg regardless of what keepleadfield is saying
% it should not be kept in cfg, since there it takes up too much space
if isfield(cfg, 'grid') && isfield(cfg.grid, 'leadfield')
cfg.grid = rmfield(cfg.grid, 'leadfield');
end
if convertfreq
% FIXME, convert the source reconstruction back to a frequency representation
elseif convertcomp
% FIXME, convert the source reconstruction back to a component representation
end
if strcmp(cfg.jackknife, 'yes')
source.method = 'jackknife';
source.trial = dip;
source.df = Ntrials;
elseif strcmp(cfg.bootstrap, 'yes')
source.method = 'bootstrap';
source.trial = dip;
source.df = Ntrials;
elseif strcmp(cfg.pseudovalue, 'yes')
source.method = 'pseudovalue';
source.trial = dip;
elseif strcmp(cfg.singletrial, 'yes')
source.method = 'singletrial';
source.trial = dip;
source.df = Ntrials; % is this correct?
elseif strcmp(cfg.rawtrial, 'yes')
source.method = 'rawtrial';
source.trial = dip;
source.df = Ntrials; % is this correct?
elseif strcmp(cfg.randomization, 'yes')
% assign the randomized resamplings to the output, keeping the special meaning of trial 1 and 2 in mind
source.method = 'randomization';
source.avgA = dip(1);
source.avgB = dip(2);
source.trialA = dip(1+2*(1:cfg.numrandomization));
source.trialB = dip(2+2*(1:cfg.numrandomization));
elseif strcmp(cfg.permutation, 'yes')
% assign the randomized resamplings to the output, keeping the special meaning of trial 1 and 2 in mind
source.method = 'permutation';
source.avgA = dip(1);
source.avgB = dip(2);
source.trialA = dip(1+2*(1:cfg.numpermutation));
source.trialB = dip(2+2*(1:cfg.numpermutation));
elseif exist('dip', 'var')
% it looks like beamformer analysis was done on an average input, keep the average source reconstruction
source.method = 'average';
source.avg = dip;
else
% apparently no computations were performed
end
if (strcmp(cfg.jackknife, 'yes') || strcmp(cfg.bootstrap, 'yes') || strcmp(cfg.pseudovalue, 'yes') || strcmp(cfg.singletrial, 'yes') || strcmp(cfg.rawtrial, 'yes')) && strcmp(cfg.keeptrials, 'yes')
% keep the source reconstruction for each repeated or resampled trial
source.trial = dip;
end
% get the output cfg
cfg = checkconfig(cfg, 'trackconfig', 'off', 'checksize', 'yes');
% add version information to the configuration
try
% get the full name of the function
cfg.version.name = mfilename('fullpath');
catch
% required for compatibility with Matlab versions prior to release 13 (6.5)
[st, i] = dbstack;
cfg.version.name = st(i);
end
cfg.version.id = '$Id: sourceanalysis.m,v 1.1 2009-07-07 02:23:16 arno Exp $';
% remember the configuration details of the input data
if nargin==2
try, cfg.previous = data.cfg; end
elseif nargin==3
cfg.previous = [];
try, cfg.previous{1} = data.cfg; end
try, cfg.previous{2} = baseline.cfg; end
end
% remember the exact configuration details in the output
source.cfg = cfg;
fprintf('total time in sourceanalysis %.1f seconds\n', toc); |
/*
* Copyright (C) 2020 European Spallation Source ERIC.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
package org.phoebus.olog.security;
import org.junit.jupiter.api.BeforeAll;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
import org.mockito.Mockito;
import org.phoebus.olog.WebSecurityConfig;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.authentication.BadCredentialsException;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.session.FindByIndexNameSessionRepository;
import org.springframework.session.MapSession;
import org.springframework.session.Session;
import org.springframework.test.context.junit.jupiter.SpringExtension;
import javax.servlet.FilterChain;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.List;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertNotNull;
import static org.junit.jupiter.api.Assertions.assertNull;
import static org.mockito.Mockito.any;
import static org.mockito.Mockito.doThrow;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.reset;
import static org.mockito.Mockito.when;
@ExtendWith(SpringExtension.class)
@SuppressWarnings("unused")
public class SessionFilterTest {
private static AuthenticationManager authenticationManager =
Mockito.mock(AuthenticationManager.class);
private static FindByIndexNameSessionRepository sessionRepository =
Mockito.mock(FindByIndexNameSessionRepository.class);
private static SessionFilter sessionFilter;
private static HttpServletResponse httpServletResponse;
private static FilterChain filterChain;
private static Cookie[] cookies;
@BeforeAll
public static void init() {
sessionFilter = new SessionFilter(authenticationManager, sessionRepository);
httpServletResponse = Mockito.mock(HttpServletResponse.class);
filterChain = Mockito.mock(FilterChain.class);
cookies = new Cookie[]{new Cookie("SESSION", "abc")};
}
@Test
public void testGetAuthorizationFromCookie() {
HttpServletRequest httpServletRequest = Mockito.mock(HttpServletRequest.class);
when(httpServletRequest.getCookies()).thenReturn(null);
Authentication authentication = sessionFilter.getAuthenticationFromCookie(httpServletRequest);
assertNull(authentication);
reset(httpServletRequest);
when(httpServletRequest.getCookies()).thenReturn(new Cookie[]{});
authentication = sessionFilter.getAuthenticationFromCookie(httpServletRequest);
assertNull(authentication);
reset(httpServletRequest);
Cookie[] cookies = new Cookie[]{new Cookie(WebSecurityConfig.SESSION_COOKIE_NAME, "b"),
new Cookie(WebSecurityConfig.SESSION_COOKIE_NAME, "a")};
when(httpServletRequest.getCookies()).thenReturn(cookies);
when(sessionRepository.findById("a")).thenReturn(null);
authentication = sessionFilter.getAuthenticationFromCookie(httpServletRequest);
assertNull(authentication);
reset(httpServletRequest);
when(httpServletRequest.getCookies()).thenReturn(cookies);
Session session = new MapSession();
session.setAttribute(WebSecurityConfig.ROLES_ATTRIBUTE_NAME, List.of("role1"));
session.setAttribute(FindByIndexNameSessionRepository.PRINCIPAL_NAME_INDEX_NAME, "user");
when(sessionRepository.findById("a")).thenReturn(session);
authentication = sessionFilter.getAuthenticationFromCookie(httpServletRequest);
assertNotNull(authentication);
}
@Test
public void testGetUsernameAndPasswordFromAuthorizationHeader() {
assertNull(sessionFilter.getUsernameAndPassword(null));
assertNull(sessionFilter.getUsernameAndPassword("Does not start with Basic"));
String[] usernameAndPassword = sessionFilter.getUsernameAndPassword("Basic YWRtaW46YWRtaW5QYXNz");
assertEquals("admin", usernameAndPassword[0]);
assertEquals("adminPass", usernameAndPassword[1]);
}
@Test
public void testFilterWithoutCookieOrAuthenticationHeader() throws Exception {
HttpServletRequest httpServletRequest = Mockito.mock(HttpServletRequest.class);
when(httpServletRequest.getCookies()).thenReturn(null);
when(httpServletRequest.getHeader("Authorization")).thenReturn(null);
sessionFilter.doFilter(httpServletRequest, httpServletResponse, filterChain);
assertNull(SecurityContextHolder.getContext().getAuthentication());
when(httpServletRequest.getCookies()).thenReturn(new Cookie[]{});
when(httpServletRequest.getHeader("Authorization")).thenReturn(null);
sessionFilter.doFilter(httpServletRequest, httpServletResponse, filterChain);
assertNull(SecurityContextHolder.getContext().getAuthentication());
}
@Test
public void testFilterWithNullSession() throws Exception {
HttpServletRequest httpServletRequest = Mockito.mock(HttpServletRequest.class);
when(httpServletRequest.getCookies()).thenReturn(cookies);
when(sessionRepository.findById("abc")).thenReturn(null);
sessionFilter.doFilter(httpServletRequest, httpServletResponse, filterChain);
assertNull(SecurityContextHolder.getContext().getAuthentication());
}
@Test
public void testFilterWithValidSession() throws Exception {
HttpServletRequest httpServletRequest = Mockito.mock(HttpServletRequest.class);
when(httpServletRequest.getCookies()).thenReturn(cookies);
Session session = mock(Session.class);
when(session.isExpired()).thenReturn(false);
when(session.getAttribute(WebSecurityConfig.ROLES_ATTRIBUTE_NAME)).thenReturn(List.of("ROLE_ADMIN"));
when(session.getAttribute(FindByIndexNameSessionRepository.PRINCIPAL_NAME_INDEX_NAME)).thenReturn("ADMIN");
when(sessionRepository.findById("abc")).thenReturn(session);
sessionFilter.doFilter(httpServletRequest, httpServletResponse, filterChain);
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
assertNotNull(authentication);
assertEquals("ADMIN", authentication.getName());
assertEquals("ROLE_ADMIN", authentication.getAuthorities().iterator().next().getAuthority());
}
@Test
public void testFilterWithWrongAuthorizationHeader() throws Exception {
HttpServletRequest httpServletRequest = Mockito.mock(HttpServletRequest.class);
when(httpServletRequest.getCookies()).thenReturn(null);
when(httpServletRequest.getHeader("Authorization")).thenReturn("wrong header value");
sessionFilter.doFilter(httpServletRequest, httpServletResponse, filterChain);
assertNull(SecurityContextHolder.getContext().getAuthentication());
}
@Test
public void testFilterWithInvalidAuthorizationHeader() throws Exception {
HttpServletRequest httpServletRequest = Mockito.mock(HttpServletRequest.class);
when(httpServletRequest.getCookies()).thenReturn(null);
when(httpServletRequest.getHeader("Authorization")).thenReturn("Basic YWRtaW46YWRtaW5QYXNz");
doThrow(new BadCredentialsException("bad")).when(authenticationManager).authenticate(any(Authentication.class));
sessionFilter.doFilter(httpServletRequest, httpServletResponse, filterChain);
assertNull(SecurityContextHolder.getContext().getAuthentication());
}
@Test
public void testFilterWithValidAuthorizationHeader() throws Exception {
HttpServletRequest httpServletRequest = Mockito.mock(HttpServletRequest.class);
when(httpServletRequest.getCookies()).thenReturn(null);
when(httpServletRequest.getHeader("Authorization")).thenReturn("Basic YWRtaW46YWRtaW5QYXNz");
Authentication authentication = new UsernamePasswordAuthenticationToken("ADMIN",
"ADMIN_PASS");
when(authenticationManager.authenticate(any(Authentication.class))).thenReturn(authentication);
sessionFilter.doFilter(httpServletRequest, httpServletResponse, filterChain);
authentication = SecurityContextHolder.getContext().getAuthentication();
assertNotNull(authentication);
assertEquals("ADMIN", authentication.getName());
}
} |
/* Q2. Prime Sum
Problem Description
Given an even number A ( greater than 2 ), return two prime numbers whose sum will be equal to the given number.
If there is more than one solution possible, return the lexicographically smaller solution.
If [a, b] is one solution with a <= b, and [c,d] is another solution with c <= d, then
[a, b] < [c, d], If a < c OR a==c AND b < d.
NOTE: A solution will always exist. Read Goldbach's conjecture.
Problem Constraints
4 <= A <= 2*107
Input Format
First and only argument of input is an even number A.
Output Format
Return a integer array of size 2 containing primes whose sum will be equal to given number.
Example Input
4
Example Output
[2, 2]
Example Explanation
There is only 1 solution for A = 4. */
public class Solution {
public int[] primesum(int A) {
// use sieve to create the isPrime array
Boolean[] isPrime = new Boolean[A+1];
Arrays.fill(isPrime,true);
isPrime[0]=false;
isPrime[1]=false;
for(int i=2;i*i<=A;i++)
{
if(isPrime[i]==true)
{
for(int j=i*i;j<=A;j=j+i)
{
isPrime[j]=false;
}
}
}
// use isPrime to get the resultant primes
// Goldbach's conjecture states that every even natural number greater than 2 is the sum of two prime numbers
int[] sumPrimes = new int[2];
for(int i=2;i<A;i++)
{
if(isPrime[i]) // i is the 1st number which will always be less
{
int otherNum = A-i;
if(isPrime[otherNum])
{
sumPrimes[0] = i;
sumPrimes[1] = otherNum;
return sumPrimes;
}
}
}
return sumPrimes;
}
} |
import React, { useState } from 'react';
import { useForm } from 'react-hook-form';
import Swal from 'sweetalert2';
const Validation = () => {
const {
register,
handleSubmit,
formState: { errors },
reset,
} = useForm();
const [submittedData, setSubmittedData] = useState([]);
const handleDeleteClick = (index) => {
Swal.fire({
title: 'Are you sure?',
text: 'You will not be able to recover this data!',
icon: 'warning',
showCancelButton: true,
confirmButtonColor: '#3085d6',
cancelButtonColor: '#d33',
confirmButtonText: 'Yes, delete it!',
}).then((result) => {
if (result.isConfirmed) {
setSubmittedData((prevData) => {
const newData = [...prevData];
newData.splice(index, 1);
return newData;
});
Swal.fire('Deleted!', 'Your data has been deleted.', 'success');
}
});
};
const onSubmit = (data) => {
setSubmittedData((prevData) => [...prevData, data]);
reset(); // Reset the form state after successful submission
};
return (
<div className="max-w-md mx-auto p-4">
<h1 className="text-2xl font-bold mb-4">Form Validation Example</h1>
<form onSubmit={handleSubmit(onSubmit)}>
<div className="mb-4">
<label htmlFor="fullName" className="block mb-2">
Full Name:
</label>
<input
id="fullName"
className="border border-gray-300 p-2"
{...register('fullName', { required: 'Full Name is required' })}
/>
{errors.fullName && (
<p className="text-red-500 mt-2">{errors.fullName.message}</p>
)}
</div>
<div className="mb-4">
<label htmlFor="email" className="block mb-2">
Email:
</label>
<input
id="email"
className="border border-gray-300 p-2"
{...register('email', {
required: 'Email is required',
pattern: {
value: /^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}$/i,
message: 'Invalid email address',
},
})}
/>
{errors.email && (
<p className="text-red-500 mt-2">{errors.email.message}</p>
)}
</div>
<div className="mb-4">
<label htmlFor="phone" className="block mb-2">
Phone Number:
</label>
<input
id="phone"
className="border border-gray-300 p-2"
{...register('phone', {
required: 'Phone Number is required',
})}
/>
{errors.phone && (
<p className="text-red-500 mt-2">{errors.phone.message}</p>
)}
</div>
<button type="submit" className="bg-blue-500 text-white px-4 py-2 rounded">
Submit
</button>
</form>
{submittedData.length > 0 && (
<div className="mt-4">
<h2 className="text-lg font-bold mb-2">Submitted Data</h2>
<table className="border-collapse border border-gray-500">
<thead>
<tr>
<th className="border border-gray-500 p-2">Full Name</th>
<th className="border border-gray-500 p-2">Email</th>
<th className="border border-gray-500 p-2">Phone Number</th>
<th className="border border-gray-500 p-2"></th>
</tr>
</thead>
<tbody>
{submittedData.map((data, index) => (
<tr key={index}>
<td className="border border-gray-500 p-2">{data.fullName}</td>
<td className="border border-gray-500 p-2">{data.email}</td>
<td className="border border-gray-500 p-2">{data.phone}</td>
<td className="border border-gray-500 p-2 bg-red-400">
<button
className="text-red-500"
onClick={() => handleDeleteClick(index)}
>
Delete
</button>
</td>
</tr>
))}
</tbody>
</table>
</div>
)}
</div>
);
};
export default Validation; |
import { Injector, NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { ProductListComponent } from './components/product-list/product-list.component';
import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http'
import { ProductService } from './services/product.service';
import { RouterModule, Routes } from '@angular/router';
import { ProductCategoryMenuComponent } from './components/product-category-menu/product-category-menu.component';
import { SearchComponent } from './components/search/search.component';
import { ProductDetailsComponent } from './components/product-details/product-details.component';
import { NgbModule } from '@ng-bootstrap/ng-bootstrap';
import { CartStatusComponent } from './components/cart-status/cart-status.component';
import { CartDetailsComponent } from './components/cart-details/cart-details.component';
import { CheckoutComponent } from './components/checkout/checkout.component';
import { ReactiveFormsModule } from '@angular/forms';
import { LoginStatusComponent } from './components/login-status/login-status.component';
import { LoginComponent } from './components/login/login.component';
import {
OktaAuthGuard,
OktaAuthModule,
OktaCallbackComponent,
OKTA_CONFIG
} from '@okta/okta-angular';
import { OktaAuth } from '@okta/okta-auth-js';
import myAppConfig from './config/my-app-config';
import { MembersPageComponent } from './components/members-page/members-page.component';
import { Router } from '@angular/router';
import { OrderHistoryComponent } from './components/order-history/order-history.component';
import { AuthInterceptorService } from './services/auth-interceptor.service';
const oktaConfig = myAppConfig.oidc;
const oktaAuth = new OktaAuth(oktaConfig);
function sendToLoginPage(oktaAuth: OktaAuth, injector: Injector){
//Use injector to access any service available within your application
const router = injector.get(Router);
//Redirect the user to your custom Login Page
router.navigate(['/login']);
}
//NOTE - ORDER MATTERS - WORKS FROM TOP DOWN - MOST SPECIFIC TO LEAST SPECIFIC
const routes: Routes = [
{path: 'order-history', component: OrderHistoryComponent, canActivate: [OktaAuthGuard],
data: {onAuthRequired: sendToLoginPage}},
{path: 'members', component: MembersPageComponent, canActivate: [OktaAuthGuard],
data: {onAuthRequired: sendToLoginPage}},
{path: 'login/callback', component: OktaCallbackComponent},
{path: 'login', component: LoginComponent},
{path: 'checkout', component: CheckoutComponent},
{path: 'cart-details', component: CartDetailsComponent},
{path: 'products/:id', component: ProductDetailsComponent},
{path: 'search/:keyword', component: ProductListComponent},
{path: 'category/:id/:name', component: ProductListComponent},
{path: 'category', component: ProductListComponent},
{path: 'products', component: ProductListComponent},
{path: '', redirectTo: '/products', pathMatch: 'full'},
{path: '**', redirectTo: '/products', pathMatch: 'full'} //** == WILDCARD - ANTHING THAT DOES NOT MATCH THE ABOVE PATHS IS ROUTED TO '/products' IN THIS CASE
];
@NgModule({
declarations: [
AppComponent,
ProductListComponent,
SearchComponent,
ProductCategoryMenuComponent,
ProductDetailsComponent,
CartStatusComponent,
CartDetailsComponent,
CheckoutComponent,
LoginStatusComponent,
LoginComponent,
MembersPageComponent,
OrderHistoryComponent,
],
imports: [
RouterModule.forRoot(routes),
BrowserModule,
HttpClientModule,
NgbModule,
ReactiveFormsModule,
OktaAuthModule
],
providers: [ProductService, { provide: OKTA_CONFIG, useValue: { oktaAuth }},
{provide: HTTP_INTERCEPTORS, useClass: AuthInterceptorService, multi: true}],
bootstrap: [AppComponent]
})
export class AppModule { } |
/*
* Copyright Lealone Database Group.
* Licensed under the Server Side Public License, v 1.
* Initial Developer: zhh
*/
package org.lealone.docdb.server.command;
import java.util.ArrayList;
import java.util.Map;
import java.util.Map.Entry;
import org.bson.BsonBoolean;
import org.bson.BsonDocument;
import org.bson.BsonElement;
import org.bson.BsonInt32;
import org.bson.BsonInt64;
import org.bson.BsonString;
import org.bson.BsonValue;
import org.lealone.common.logging.Logger;
import org.lealone.common.logging.LoggerFactory;
import org.lealone.db.Constants;
import org.lealone.db.Database;
import org.lealone.db.LealoneDatabase;
import org.lealone.db.auth.User;
import org.lealone.db.schema.Schema;
import org.lealone.db.session.ServerSession;
import org.lealone.db.table.Table;
import org.lealone.db.value.Value;
import org.lealone.db.value.ValueInt;
import org.lealone.db.value.ValueLong;
import org.lealone.db.value.ValueMap;
import org.lealone.db.value.ValueString;
import org.lealone.docdb.server.DocDBServer;
import org.lealone.docdb.server.DocDBServerConnection;
public abstract class BsonCommand {
public static final Logger logger = LoggerFactory.getLogger(BsonCommand.class);
public static final boolean DEBUG = false;
public static BsonDocument newOkBsonDocument() {
BsonDocument document = new BsonDocument();
setOk(document);
return document;
}
public static void setOk(BsonDocument doc) {
append(doc, "ok", 1);
}
public static void setN(BsonDocument doc, int n) {
append(doc, "n", n);
}
public static void setWireVersion(BsonDocument doc) {
append(doc, "minWireVersion", 0);
append(doc, "maxWireVersion", 17);
}
public static Database getDatabase(BsonDocument doc) {
String dbName = doc.getString("$db").getValue();
if (dbName == null)
dbName = DocDBServer.DATABASE_NAME;
Database db = LealoneDatabase.getInstance().findDatabase(dbName);
if (db == null) {
String sql = "CREATE DATABASE IF NOT EXISTS " + dbName;
LealoneDatabase.getInstance().getSystemSession().prepareStatementLocal(sql).executeUpdate();
db = LealoneDatabase.getInstance().getDatabase(dbName);
}
if (!db.isInitialized())
db.init();
return db;
}
public static Table getTable(BsonDocument doc, String key, DocDBServerConnection conn) {
Database db = getDatabase(doc);
Schema schema = db.getSchema(null, Constants.SCHEMA_MAIN);
String tableName = doc.getString(key).getValue();
Table table = schema.findTableOrView(null, tableName);
if (table == null) {
try (ServerSession session = getSession(db, conn)) {
String sql = "CREATE TABLE IF NOT EXISTS " + Constants.SCHEMA_MAIN + "." + tableName
+ "(_json_ map<varchar, object>)";
session.prepareStatementLocal(sql).executeUpdate();
}
}
return schema.getTableOrView(null, tableName);
}
public static ServerSession createSession(Database db) {
return new ServerSession(db, getUser(db), 0);
// return db.createSession(getUser(db));
}
public static ServerSession getSession(Database db, DocDBServerConnection conn) {
return conn.getPooledSession(db);
}
public static User getUser(Database db) {
for (User user : db.getAllUsers()) {
if (user.isAdmin())
return user;
}
return db.getAllUsers().get(0);
}
public static void append(BsonDocument doc, String key, boolean value) {
doc.append(key, new BsonBoolean(value));
}
public static void append(BsonDocument doc, String key, int value) {
doc.append(key, new BsonInt32(value));
}
public static void append(BsonDocument doc, String key, long value) {
doc.append(key, new BsonInt64(value));
}
public static void append(BsonDocument doc, String key, String value) {
doc.append(key, new BsonString(value));
}
public static ValueMap toValueMap(BsonDocument doc) {
Value[] values = new Value[doc.size() * 2];
int index = 0;
for (Entry<String, BsonValue> e : doc.entrySet()) {
values[index++] = ValueString.get(e.getKey());
BsonValue v = e.getValue();
switch (v.getBsonType()) {
case INT32:
values[index++] = ValueInt.get(v.asInt32().getValue());
break;
case INT64:
values[index++] = ValueLong.get(v.asInt64().getValue());
break;
// case STRING:
default:
values[index++] = ValueString.get(v.asString().getValue());
}
}
ValueMap vMap = ValueMap.get(String.class, Object.class, values);
return vMap;
}
public static BsonDocument toBsonDocument(ValueMap vMap) {
BsonDocument doc = (BsonDocument) vMap.getDocument();
if (doc != null)
return doc;
synchronized (vMap) {
doc = (BsonDocument) vMap.getDocument();
if (doc != null)
return doc;
Map<Value, Value> map = vMap.getMap();
ArrayList<BsonElement> bsonElements = new ArrayList<>(map.size());
for (Entry<Value, Value> e : map.entrySet()) {
Value v = e.getValue();
BsonValue bv;
switch (v.getType()) {
case Value.INT:
bv = new BsonInt32(v.getInt());
break;
case Value.LONG:
bv = new BsonInt64(v.getLong());
break;
default:
bv = new BsonString(v.getString());
}
bsonElements.add(new BsonElement(e.getKey().getString(), bv));
}
doc = new BsonDocument(bsonElements);
vMap.setDocument(doc);
return doc;
}
}
public static Long getId(BsonDocument doc) {
BsonValue id = doc.get("_id", null);
if (id != null) {
if (id.isInt32())
return Long.valueOf(id.asInt32().getValue());
else if (id.isInt64())
return Long.valueOf(id.asInt64().getValue());
}
return null;
}
} |
// Copyright (C) 2004 Aldratech Ltd. See the LICENSE file for licensing information.
/*
This file is part of sslpp.
sslpp is free software; you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation; either version 2.1 of the License, or
(at your option) any later version.
sslpp is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License
along with sslpp; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
#ifndef SSLPP_ABSTRACT_CONNECTION_H
#define SSLPP_ABSTRACT_CONNECTION_H
#include <string>
using namespace std;
namespace sslpp {
///Abstract connection.
class abstract_connection {
public:
/// Destructor.
virtual ~abstract_connection() {
;
}
/// Output operator.
virtual abstract_connection& operator<<(const string& buf) = 0;
/// Input operator.
virtual abstract_connection& operator>>(string& buf) = 0;
/// Read max_size bytes into buf.
/**
* @param max_size Number of bytes to read into buf.
* @param buf Pre-allocated buffer to write into.
* @return >0 success, number of bytes read; <=0 error
*/
virtual int read(int max_size, char* buf) = 0;
/// Write a string to the current connection.
/**
* @param what The string to write.
* @return >0 success, number of bytes read; <=0 error
*/
virtual int write(const string& what) = 0;
/// Test status.
virtual bool valid() const = 0;
/// Return an error trace.
virtual string errors() = 0;
/// Return the endpoint.
virtual string endpoint() const = 0;
};
}
#endif |
/*
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
* or more contributor license agreements. Licensed under the Elastic License
* 2.0 and the Server Side Public License, v 1; you may not use this file except
* in compliance with, at your election, the Elastic License 2.0 or the Server
* Side Public License, v 1.
*/
package org.elasticsearch.benchmark.index.mapper;
import org.elasticsearch.common.UUIDs;
import org.elasticsearch.common.bytes.BytesArray;
import org.elasticsearch.common.compress.CompressedXContent;
import org.elasticsearch.common.xcontent.XContentHelper;
import org.elasticsearch.index.mapper.DocumentMapper;
import org.elasticsearch.index.mapper.LuceneDocument;
import org.elasticsearch.index.mapper.MapperService;
import org.elasticsearch.index.mapper.Mapping;
import org.elasticsearch.index.mapper.ParsedDocument;
import org.elasticsearch.index.mapper.SourceToParse;
import org.elasticsearch.xcontent.XContentType;
import org.openjdk.jmh.annotations.Benchmark;
import org.openjdk.jmh.annotations.BenchmarkMode;
import org.openjdk.jmh.annotations.Fork;
import org.openjdk.jmh.annotations.Measurement;
import org.openjdk.jmh.annotations.Mode;
import org.openjdk.jmh.annotations.OutputTimeUnit;
import org.openjdk.jmh.annotations.Param;
import org.openjdk.jmh.annotations.Scope;
import org.openjdk.jmh.annotations.Setup;
import org.openjdk.jmh.annotations.State;
import org.openjdk.jmh.annotations.Warmup;
import java.util.Arrays;
import java.util.List;
import java.util.Random;
import java.util.concurrent.TimeUnit;
import java.util.stream.Collectors;
import java.util.stream.DoubleStream;
import java.util.stream.IntStream;
import java.util.stream.Stream;
@Fork(value = 3)
@Warmup(iterations = 3)
@Measurement(iterations = 5)
@BenchmarkMode(Mode.AverageTime)
@OutputTimeUnit(TimeUnit.MILLISECONDS)
@State(Scope.Benchmark)
public class DynamicMapperBenchmark {
@Param({ "1600172297" })
private long seed;
private Random random;
private SourceToParse[] sources;
@Setup
public void setUp() {
this.random = new Random(seed);
this.sources = generateRandomDocuments(500);
}
private SourceToParse[] generateRandomDocuments(int count) {
var docs = new SourceToParse[count];
for (int i = 0; i < count; i++) {
docs[i] = generateRandomDocument();
}
return docs;
}
private SourceToParse generateRandomDocument() {
int textFields = 50;
int intFields = 50;
int floatFields = 50;
int objFields = 10;
int objFieldDepth = 10;
int fieldValueCountMax = 25;
StringBuilder builder = new StringBuilder();
builder.append("{");
for (int i = 0; i < textFields; i++) {
if (random.nextBoolean()) {
StringBuilder fieldValueBuilder = generateTextField(fieldValueCountMax);
builder.append("\"text_field_").append(i).append("\":").append(fieldValueBuilder).append(",");
}
}
for (int i = 0; i < intFields; i++) {
if (random.nextBoolean()) {
int fieldValueCount = random.nextInt(fieldValueCountMax);
builder.append("\"int_field_")
.append(i)
.append("\":")
.append(Arrays.toString(IntStream.generate(() -> random.nextInt()).limit(fieldValueCount).toArray()))
.append(",");
}
}
for (int i = 0; i < floatFields; i++) {
if (random.nextBoolean()) {
int fieldValueCount = random.nextInt(fieldValueCountMax);
builder.append("\"float_field_")
.append(i)
.append("\":")
.append(Arrays.toString(DoubleStream.generate(() -> random.nextFloat()).limit(fieldValueCount).toArray()))
.append(",");
}
}
for (int i = 0; i < objFields; i++) {
final int idx = i;
if (random.nextBoolean()) {
continue;
}
int objFieldDepthActual = random.nextInt(1, objFieldDepth);
String objFieldPrefix = Stream.generate(() -> "obj_field_" + idx).limit(objFieldDepthActual).collect(Collectors.joining("."));
for (int j = 0; j < textFields; j++) {
if (random.nextBoolean()) {
StringBuilder fieldValueBuilder = generateTextField(fieldValueCountMax);
builder.append("\"")
.append(objFieldPrefix)
.append(".text_field_")
.append(j)
.append("\":")
.append(fieldValueBuilder)
.append(",");
}
}
for (int j = 0; j < intFields; j++) {
if (random.nextBoolean()) {
int fieldValueCount = random.nextInt(fieldValueCountMax);
builder.append("\"")
.append(objFieldPrefix)
.append(".int_field_")
.append(j)
.append("\":")
.append(Arrays.toString(IntStream.generate(() -> random.nextInt()).limit(fieldValueCount).toArray()))
.append(",");
}
}
for (int j = 0; j < floatFields; j++) {
if (random.nextBoolean()) {
int fieldValueCount = random.nextInt(fieldValueCountMax);
builder.append("\"")
.append(objFieldPrefix)
.append(".float_field_")
.append(j)
.append("\":")
.append(Arrays.toString(DoubleStream.generate(() -> random.nextFloat()).limit(fieldValueCount).toArray()))
.append(",");
}
}
}
if (builder.charAt(builder.length() - 1) == ',') {
builder.deleteCharAt(builder.length() - 1);
}
builder.append("}");
return new SourceToParse(UUIDs.randomBase64UUID(), new BytesArray(builder.toString()), XContentType.JSON);
}
private StringBuilder generateTextField(int fieldValueCountMax) {
int fieldValueCount = random.nextInt(fieldValueCountMax);
StringBuilder fieldValueBuilder = new StringBuilder();
fieldValueBuilder.append("[");
for (int j = 0; j < fieldValueCount - 1; j++) {
fieldValueBuilder.append("\"").append(randomString(6)).append("\"").append(",");
}
return fieldValueBuilder.append("\"").append(randomString(6)).append("\"").append("]");
}
private String randomString(int maxLength) {
var length = random.nextInt(maxLength);
var builder = new StringBuilder(length);
for (int i = 0; i < length; i++) {
builder.append((byte) (32 + random.nextInt(94)));
}
return builder.toString();
}
@SafeVarargs
@SuppressWarnings("varargs")
private <T> T randomFrom(T... items) {
return items[random.nextInt(items.length)];
}
@Benchmark
public List<LuceneDocument> benchmarkDynamicallyCreatedFields() throws Exception {
MapperService mapperService = MapperServiceFactory.create("{}");
for (int i = 0; i < 25; i++) {
DocumentMapper documentMapper = mapperService.documentMapper();
Mapping mapping = null;
if (documentMapper == null) {
documentMapper = DocumentMapper.createEmpty(mapperService);
mapping = documentMapper.mapping();
}
ParsedDocument doc = documentMapper.parse(randomFrom(sources));
if (mapping != null) {
doc.addDynamicMappingsUpdate(mapping);
}
if (doc.dynamicMappingsUpdate() != null) {
mapperService.merge(
"_doc",
new CompressedXContent(XContentHelper.toXContent(doc.dynamicMappingsUpdate(), XContentType.JSON, false)),
MapperService.MergeReason.MAPPING_UPDATE
);
}
}
return mapperService.documentMapper().parse(randomFrom(sources)).docs();
}
} |
<!DOCTYPE html>
<html lang="pt-br">
<head>
<meta charset="utf-8">
</head>
<body>
<script>
/**
* * REPLACE JAVASCRIPT REGEX - SUBSTITUIR
*
* Resumo : neste tutorial, você aprenderá como usar o método string replace() para retornar uma nova string com algumas ou todas as correspondências de uma expressão regular substituída por uma string de substituição.
*
* * INTRODUÇÃO AO MÉTODO JS replace()
*
* O String.prototype.replace() método funciona com strings e expressões regulares . Este tutorial se concentra apenas em expressões regulares.
*
* Veja a seguir a sintaxe do replace() método:
replace(regexp, newSubstr);
*
* Nesta sintaxe:
- O regexp é uma expressão regular para corresponder.
- O newSubstr é uma string para substituir as correspondências. Se newSubstr estiver vazio, o replace() método remove as correspondências.
* O replace() retorna uma nova string com as correspondências substituídas pelo newSubstr. Observe que o replace() método não altera a string original, mas retorna uma nova string.
*
* Por padrão, o replace() método substitui a primeira correspondência se regexp não usar o sinalizador global ( g). Para substituir todas as correspondências, você usa o sinalizador global ( g) no arquivo regexp.
*/
</script>
</body>
</html> |
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Providers\RouteServiceProvider;
use Illuminate\Foundation\Auth\RegistersUsers;
use Illuminate\Support\Facades\Hash;
use Illuminate\Support\Facades\Validator;
use Illuminate\Support\Facades\DB;
use App\Models\User;
use Spatie\Permission\Models\Role;
class RegistroUsuarioController extends Controller
{
use RegistersUsers;
/**
* Where to redirect users after registration.
*
* @var string
*/
protected $redirectTo = RouteServiceProvider::HOME;
/**
* Create a new controller instance.
*
* @return void
*/
public function __construct()
{
$this->middleware('guest');
}
/**
* Show the registration form.
*
* @return \Illuminate\View\View
*/
public function showRegistrationForm()
{
$roles = Role::pluck('name');
return view('register_user', compact('roles'));
}
/**
* Handle a registration request for the application.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
protected function register(Request $request)
{
$this->validator($request->all())->validate();
$user = $this->create($request->all());
$this->guard()->login($user);
return $this->registered($request, $user)
?: redirect($this->redirectPath());
}
/**
* Get a validator for an incoming registration request.
*
* @param array $data
* @return \Illuminate\Contracts\Validation\Validator
*/
protected function validator(array $data)
{
return Validator::make($data, [
'name' => 'required|string|max:255',
'username' => 'required|string|max:255|unique:users',
'password' => 'required|string|min:8|confirmed',
]);
}
/**
* Create a new user instance after a valid registration.
*
* @param array $data
* @return \App\Models\User
*/
protected function create(array $data)
{
User::create([
'name' => $request->input('name'),
'username' => $request->input('username'),
'password' => bcrypt($request->input('password')),
'role' => $request->input('role'),
]);
}
} |
package studentmanager;
import DBconnection.dbconnection;
import DBmanager.StudentDbManager;
import model.Department;
import model.Student;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.List;
import java.util.Scanner;
import java.util.logging.Level;
import java.util.logging.Logger;
public class StudentOperations {
private final String CHECK_DEPARTMENT_NAME = "SELECT `dept_id` FROM `department` WHERE `dept_name` = ?";
private final StudentDbManager studentdbobj = new StudentDbManager();
private final Scanner input = new Scanner(System.in);
public void addStudentOperation() {
try (Connection connect = dbconnection.getconnection();
PreparedStatement ps = connect.prepareStatement(CHECK_DEPARTMENT_NAME)) {
Student std = new Student();
System.out.print("Enter the Student Name: ");
String studentname = input.nextLine();
System.out.print("Enter the Student Email: ");
String studentemail = input.nextLine();
System.out.print("Enter the Student Phone: ");
String studentphone = input.nextLine();
System.out.print("Enter the Student Department Name: ");
String studentdeptmentname = input.nextLine();
ps.setString(1, studentdeptmentname);
ResultSet deptResult = ps.executeQuery();
int getdeptid = 0; // Initialize getdeptid
boolean departmentExists = false;
if (deptResult.next()) {
departmentExists = true;
getdeptid = deptResult.getInt("dept_id");
}
if (departmentExists) {
// Department exists, proceed to add the student
Student s = new Student();
s.setName(studentname);
s.setEmail(studentemail);
s.setPhone(studentphone);
Department d = new Department();
d.setId(getdeptid); // Use the retrieved department ID
s.setDepartment(d);
studentdbobj.addStudent(s);
System.out.print("Record Added Successfully!");
} else {
System.out.println("The department does not exist.");
}
} catch (SQLException ex) {
Logger.getLogger(StudentOperations.class.getName()).log(Level.SEVERE, null, ex);
}
}
public void showAllStudentsOperation(){
List<Student> list = studentdbobj.getAllStudent();
for(Student student : list){
System.out.println("Student Name : " + student.getName());
System.out.println("Student Email : " + student.getEmail());
System.out.println("Student Phone : " + student.getPhone());
System.out.println("Student Department Name : " + student.getDepartment().getName());
System.out.println("Student Department Code : " + student.getDepartment().getCode());
}
}
public void updateStudentOperation(){
try {
Student stu = studentdbobj.getStudentById(1);
Connection connect = dbconnection.getconnection();
PreparedStatement ps = connect.prepareStatement(CHECK_DEPARTMENT_NAME);
System.out.print("Enter the Updated Name : ");
String updatename = input.nextLine();
System.out.print("Enter the Update Email : ");
String updateemail = input.next();
System.out.print("Enter the Updated Phone : ");
String updatephone = input.next();
System.out.print("Enter the Department Name : ");
String departname = input.next();
ps.setString(1, departname);
ResultSet deptResult = ps.executeQuery();
int getdeptid = 0; // Initialize getdeptid
boolean departmentExists = false;
while(deptResult.next()){
departmentExists = true;
getdeptid = deptResult.getInt("dept_id");
}
if(departmentExists){
stu.setName(updatename);
stu.setEmail(updateemail);
stu.setPhone(updatephone);
Department d = new Department();
d.setId(getdeptid);
stu.setDepartment(d);
studentdbobj.updateStudent(stu);
System.out.print("Record Updated Successfully!");
}else {
System.out.println("The department does not exist.");
}
} catch (SQLException ex) {
Logger.getLogger(StudentOperations.class.getName()).log(Level.SEVERE, null, ex);
}
}
public void deleteStudentOperation(){
studentdbobj.deleteStudent(1);
System.out.print("Record Deleted Successfully!");
}
} |
# payload-plugin-scheduler
Payload plugin that enables scheduled publishing for draft-enabled collections, inspired by wordpress post scheduler.

## Installation
```sh
npm i payload-plugin-scheduler
```
## Usage
```ts
// payload.config.ts
import { buildConfig } from 'payload/config'
import { ScheduledPostPlugin } from 'payload-plugin-scheduler'
import Pages from './collections/Pages'
import Posts from './collections/Posts'
export default buildConfig({
collections: [Pages, Posts],
plugins: [
ScheduledPostPlugin({
collections: ['pages', 'posts'],
interval: 10,
})
]
// ...more config
})
```
## Options
### `collections: string[]`
An array of collection slugs. All collections must have drafts enabled.
### `interval?: number`
Specify how frequently to check for scheduled posts (in minutes).
This value will also be passed to the `DatePicker` component. Defaults to 5 mins.
### `scheduledPosts?: Partial<CollectionConfig>`
Custom configuration for the scheduled posts collection that gets merged with the defaults.
## Approach
In a nutshell, the plugin creates a `publish_date` field that it uses to determine whether a pending draft update needs to be scheduled.
### `publish_date`
Custom Datetime field added to documents in enabled collections.
Includes custom `Field` and `Cell` components that include schedule status in the client-side UI.
### `scheduled_posts`
Collection added by the plugin to store pending schedule updates. Can be customized via `scheduledPosts` option.
### Cron
A configurable timer checks for any posts to be scheduled in the upcoming interval window. For each hit, it creates a separate job that's fired at that document's `publish_date` (via [node-schedule](https://github.com/node-schedule/node-schedule)). The idea here is that you can configure your interval window to avoid super long running tasks that are more prone to flaking.
## Notes
Since the plugin uses cron under the hood, it depends on a long-running server and is incompatible with short-lived/serverless environments like ECS, or Vercel if you're using Payload 3.0 beta.
I developed this plugin for a project that hasn't gone live yet. It has good test coverage but not in the wild yet -- there's your disclaimer. |
package com.taobao.arthas.core.util;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.taobao.arthas.common.IOUtils;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.HttpURLConnection;
import java.net.Socket;
import java.net.URL;
/**
* @author ralf0131 on 2015-11-11 15:39.
*/
public class NetUtils {
private static final String QOS_HOST = "localhost";
private static final int QOS_PORT = 12201;
private static final String QOS_RESPONSE_START_LINE = "pandora>[QOS Response]";
private static final int INTERNAL_SERVER_ERROR = 500;
private static final int CONNECT_TIMEOUT = 1000;
private static final int READ_TIMEOUT = 3000;
/**
* This implementation is based on Apache HttpClient.
* @param urlString the requested url
* @return the response string of given url
*/
public static Response request(String urlString) {
HttpURLConnection urlConnection = null;
InputStream in = null;
try {
URL url = new URL(urlString);
urlConnection = (HttpURLConnection)url.openConnection();
urlConnection.setConnectTimeout(CONNECT_TIMEOUT);
urlConnection.setReadTimeout(READ_TIMEOUT);;
// prefer json to text
urlConnection.setRequestProperty("Accept", "application/json,text/plain;q=0.2");
in = urlConnection.getInputStream();
BufferedReader br = new BufferedReader(new InputStreamReader(in));
String line = null;
StringBuilder sb = new StringBuilder();
while ((line = br.readLine()) != null) {
sb.append(line).append("\n");
}
int statusCode = urlConnection.getResponseCode();
String result = sb.toString().trim();
if (statusCode == INTERNAL_SERVER_ERROR) {
JSONObject errorObj = JSON.parseObject(result);
if (errorObj.containsKey("errorMsg")) {
return new Response(errorObj.getString("errorMsg"), false);
}
return new Response(result, false);
}
return new Response(result);
} catch (IOException e) {
return new Response(e.getMessage(), false);
} finally {
IOUtils.close(in);
if (urlConnection != null) {
urlConnection.disconnect();
}
}
}
/**
* @deprecated
* This implementation is based on HttpURLConnection,
* which can not detail with status code other than 200.
* @param url the requested url
* @return the response string of given url
*/
public static String simpleRequest(String url) {
BufferedReader br = null;
try {
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestProperty("Accept", "application/json");
int responseCode = con.getResponseCode();
br = new BufferedReader(new InputStreamReader(con.getInputStream()));
StringBuffer sb = new StringBuffer();
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line);
sb.append("\n");
}
String result = sb.toString().trim();
if (responseCode == 500) {
JSONObject errorObj = JSON.parseObject(result);
if (errorObj.containsKey("errorMsg")) {
return errorObj.getString("errorMsg");
}
return result;
} else {
return result;
}
} catch (Exception e) {
return null;
} finally {
if (br != null) {
try {
br.close();
} catch (IOException e) {
// ignore
}
}
}
}
/**
* Only use this method when tomcat monitor version <= 1.0.1
* This will send http request to pandora qos port 12201,
* and display the response.
* Note that pandora qos response is not fully HTTP compatible under version 2.1.0,
* so we filtered some of the content and only display useful content.
* @param path the path relative to http://localhost:12201
* e.g. /pandora/ls
* For commands that requires arguments, use the following format
* e.g. /pandora/find?arg0=RPCProtocolService
* Note that the parameter name is never used in pandora qos,
* so the name(e.g. arg0) is irrelevant.
* @return the qos response in string format
*/
public static Response requestViaSocket(String path) {
BufferedReader br = null;
try {
Socket s = new Socket(QOS_HOST, QOS_PORT);
PrintWriter pw = new PrintWriter(s.getOutputStream());
pw.println("GET " + path + " HTTP/1.1");
pw.println("Host: " + QOS_HOST + ":" + QOS_PORT);
pw.println("");
pw.flush();
br = new BufferedReader(new InputStreamReader(s.getInputStream()));
StringBuffer sb = new StringBuffer();
String line = null;
boolean start = false;
while ((line = br.readLine()) != null) {
if (start) {
sb.append(line).append("\n");
}
if (line.equals(QOS_RESPONSE_START_LINE)) {
start = true;
}
}
String result = sb.toString().trim();
return new Response(result);
} catch (Exception e) {
return new Response(e.getMessage(), false);
} finally {
if (br != null) {
try {
br.close();
} catch (IOException e) {
// ignore
}
}
}
}
public static class Response {
private boolean success;
private String content;
public Response(String content, boolean success) {
this.success = success;
this.content = content;
}
public Response(String content) {
this.content = content;
this.success = true;
}
public boolean isSuccess() {
return success;
}
public String getContent() {
return content;
}
}
/**
* Test if a port is open on the give host
*/
public static boolean serverListening(String host, int port) {
Socket s = null;
try {
s = new Socket(host, port);
return true;
} catch (Exception e) {
return false;
} finally {
if (s != null) {
try {
s.close();
} catch (Exception e) {
// ignore
}
}
}
}
} |
/********************************************************************************
* Copyright (c) 2006, 2009 IBM Corporation and others. All rights reserved.
* This program and the accompanying materials are made available under the terms
* of the Eclipse Public License v1.0 which accompanies this distribution, and is
* available at http://www.eclipse.org/legal/epl-v10.html
*
* Initial Contributors:
* The following IBM employees contributed to the Remote System Explorer
* component that contains this file: David McKnight, Kushal Munir,
* Michael Berger, David Dykstal, Phil Coulthard, Don Yantzi, Eric Simpson,
* Emily Bruner, Mazen Faraj, Adrian Storisteanu, Li Ding, and Kent Hawley.
*
* Contributors:
* Martin Oberhuber (Wind River) - [175262] IHost.getSystemType() should return IRSESystemType
* Martin Oberhuber (Wind River) - [186128] Move IProgressMonitor last in all API
* Martin Oberhuber (Wind River) - [186640] Add IRSESystemType.testProperty()
* David McKnight (IBM) - [191599] Need to pass in shell encoding
* David Dykstal (IBM) - [197036] refactored switch configuration
* David Dykstal (IBM) - [217556] remove service subsystem types
* David McKnight (IBM) - [220524] internalSwitchServiceSubSystemConfiguration -> internalSwitchSubSystemConfiguration
* Martin Oberhuber (Wind River) - [226301][api] IShellService should throw SystemMessageException on error
* Martin Oberhuber (Wind River) - [218304] Improve deferred adapter loading
* David McKnight (IBM) - [272882] [api] Handle exceptions in IService.initService()
********************************************************************************/
package org.eclipse.rse.subsystems.shells.core.subsystems.servicesubsystem;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.List;
import org.eclipse.core.runtime.IProgressMonitor;
import org.eclipse.rse.core.IRSESystemType;
import org.eclipse.rse.core.model.IHost;
import org.eclipse.rse.core.subsystems.IConnectorService;
import org.eclipse.rse.core.subsystems.ISubSystemConfiguration;
import org.eclipse.rse.services.clientserver.messages.SystemMessageException;
import org.eclipse.rse.services.shells.IHostShell;
import org.eclipse.rse.services.shells.IShellService;
import org.eclipse.rse.subsystems.files.core.subsystems.IRemoteFile;
import org.eclipse.rse.subsystems.shells.core.subsystems.IRemoteCmdSubSystem;
import org.eclipse.rse.subsystems.shells.core.subsystems.IRemoteCommandShell;
import org.eclipse.rse.subsystems.shells.core.subsystems.RemoteCmdSubSystem;
import org.eclipse.rse.ui.SystemBasePlugin;
public final class ShellServiceSubSystem extends RemoteCmdSubSystem implements IShellServiceSubSystem
{
protected String _userHome = null;
protected IShellService _hostService;
public ShellServiceSubSystem(IHost host, IConnectorService connectorService, IShellService hostService)
{
super(host, connectorService);
_hostService = hostService;
}
public IShellService getShellService()
{
return _hostService;
}
public void setShellService(IShellService service)
{
_hostService = service;
}
protected String getUserHome()
{
if (_userHome == null)
{
IRSESystemType type = getHost().getSystemType();
if (type.isLocal())
{
_userHome = System.getProperty("user.home"); //$NON-NLS-1$
}
else if (type.isWindows())
{
_userHome = "c:\\"; //$NON-NLS-1$
}
else
{
// Assume UNIX compatible file system
_userHome = "/home/" + getUserId(); //$NON-NLS-1$
}
}
return _userHome;
}
protected Object[] internalRunCommand(String cmd, Object context, IProgressMonitor monitor) throws InvocationTargetException, InterruptedException, SystemMessageException
{
return internalRunCommand(cmd, context, false, monitor);
}
protected Object[] internalRunCommand(String cmd, Object context, boolean interpretOutput, IProgressMonitor monitor) throws InvocationTargetException, InterruptedException, SystemMessageException
{
String cwd = ""; //$NON-NLS-1$
if (context instanceof IRemoteFile)
{
IRemoteFile file = (IRemoteFile) context;
cwd = file.getAbsolutePath();
}
else if (context instanceof String)
{
// assume the string is a remote path
cwd = (String)context;
}
if (cwd == null || cwd.equals("null")) //$NON-NLS-1$
{
cwd = getUserHome();
}
IShellService service = getShellService();
IHostShell hostShell = service.runCommand(cwd, cmd, getUserAndHostEnvVarsAsStringArray(), monitor);
IServiceCommandShell cmdShell = createRemoteCommandShell(this, hostShell);
hostShell.addOutputListener(cmdShell);
if (_cmdShells.size() == 0)
{
// if this is first shell, start listening so that on disconnect, we persist
getConnectorService().addCommunicationsListener(this);
}
_cmdShells.add(cmdShell);
return new Object[] {cmdShell};
}
protected IRemoteCommandShell internalRunShell(Object context, IProgressMonitor monitor) throws InvocationTargetException, InterruptedException, SystemMessageException
{
String cwd = ""; //$NON-NLS-1$
if (context instanceof IRemoteFile)
{
IRemoteFile file = (IRemoteFile) context;
cwd = file.getAbsolutePath();
}
else if (context instanceof String)
{
// assume the string is a remote path
cwd = (String)context;
}
if (cwd == null || cwd.equals("null")) //$NON-NLS-1$
{
cwd = getUserHome();
}
IShellService service = getShellService();
String encoding = getHost().getDefaultEncoding(true);
IHostShell hostShell = service.launchShell(cwd, encoding, getUserAndHostEnvVarsAsStringArray(), monitor);
IServiceCommandShell cmdShell = createRemoteCommandShell(this, hostShell);
if (cmdShell != null)
{
hostShell.addOutputListener(cmdShell);
if (_cmdShells.size() == 0)
{
// if this is first shell, start listening so that on disconnect, we persist
getConnectorService().addCommunicationsListener(this);
}
_cmdShells.add(cmdShell);
}
return cmdShell;
}
protected void internalCancelShell(Object command, IProgressMonitor monitor) throws InvocationTargetException, InterruptedException
{
if (command instanceof IServiceCommandShell)
{
IServiceCommandShell cmd = (IServiceCommandShell)command;
cmd.getHostShell().exit();
}
}
protected void internalSendCommandToShell(String cmd, Object command, IProgressMonitor monitor) throws InvocationTargetException, InterruptedException
{
if (command instanceof IServiceCommandShell)
{
IServiceCommandShell cmdWrapper = (IServiceCommandShell)command;
cmdWrapper.writeToShell(cmd);
cmdWrapper.updateHistory(cmd);
}
}
protected IServiceCommandShell createRemoteCommandShell(IRemoteCmdSubSystem cmdSS, IHostShell hostShell)
{
IShellServiceSubSystemConfiguration config = (IShellServiceSubSystemConfiguration)getParentRemoteCmdSubSystemConfiguration();
return config.createRemoteCommandShell(cmdSS, hostShell);
}
public String[] getHostEnvironment()
{
try {
return getShellService().getHostEnvironment();
} catch (SystemMessageException e) {
SystemBasePlugin.logError(e.getSystemMessage().getLevelOneText(), e);
}
return new String[0];
}
public List getHostEnvironmentVariables()
{
List l = new ArrayList();
String[] vars = getHostEnvironment();
for (int i = 0; i < vars.length; i++)
{
l.add(vars[i]);
}
return l;
}
/* (non-Javadoc)
* @see org.eclipse.rse.core.subsystems.SubSystem#canSwitchTo(org.eclipse.rse.core.subsystems.ISubSystemConfiguration)
*/
public boolean canSwitchTo(ISubSystemConfiguration configuration) {
return configuration instanceof IShellServiceSubSystemConfiguration;
}
/* (non-Javadoc)
* @see org.eclipse.rse.core.subsystems.SubSystem#internalServiceSubSystemConfiguration(org.eclipse.rse.core.subsystems.ISubSystemConfiguration)
*/
protected void internalSwitchSubSystemConfiguration(ISubSystemConfiguration newConfiguration) {
IShellServiceSubSystemConfiguration configuration = (IShellServiceSubSystemConfiguration) newConfiguration;
IHost host = getHost();
setShellService(configuration.getShellService(host));
}
/* (non-Javadoc)
* @see org.eclipse.rse.core.subsystems.SubSystem#getServiceType()
*/
public Class getServiceType()
{
return IShellService.class;
}
public void initializeSubSystem(IProgressMonitor monitor) throws SystemMessageException
{
super.initializeSubSystem(monitor);
getShellService().initService(monitor);
}
public void uninitializeSubSystem(IProgressMonitor monitor)
{
cancelAllShells();
getShellService().uninitService(monitor);
super.uninitializeSubSystem(monitor);
}
} |
import React from 'react'
import style from './StatCard.module.css'
export interface StatCardProps {
statName: string;
statValue: number;
StatIcon: any;
}
function StatCard({ statName, statValue, StatIcon }: StatCardProps) {
return (
<React.Fragment>
<div className={style.stat__card}>
<div className={style.left__item}>
<div className={style.stat__icon}>
{StatIcon}
</div>
</div>
<div className={style.right__item}>
<div className={style.stat__name}>
<h3>
{statName}
</h3>
</div>
<div className={style.stat__value}>
<h3>
{statValue}
</h3>
</div>
</div>
</div>
</React.Fragment>
)
}
export default StatCard |
(function () {
// Function to replace 'http://' with 'https://' in a URL
function toHttps(url) {
return url.replace(/http:/g, "https:");
}
// Convert <a> tags
document.querySelectorAll("a").forEach(function (link) {
if (link.href.startsWith("http:")) {
link.href = toHttps(link.href);
}
});
// Convert <link> tags for stylesheets
document.querySelectorAll('link[rel="stylesheet"]').forEach(function (link) {
if (link.href.startsWith("http:")) {
link.href = toHttps(link.href);
}
});
// Convert <script> tags
document.querySelectorAll("script").forEach(function (script) {
if (script.src.startsWith("http:")) {
script.src = toHttps(script.src);
}
});
// Convert images
document.querySelectorAll("img").forEach(function (img) {
if (img.src.startsWith("http:")) {
img.src = toHttps(img.src);
}
});
// Convert any other tags with a 'src' attribute (e.g., iframes, embeds)
document.querySelectorAll("[src]").forEach(function (element) {
if (element.src.startsWith("http:")) {
element.src = toHttps(element.src);
}
});
// Convert any inline styles with background images loaded over HTTP
document.querySelectorAll("[style]").forEach(function (element) {
if (element.style.backgroundImage.startsWith("url(http:")) {
element.style.backgroundImage = toHttps(element.style.backgroundImage);
}
});
// Convert objects, videos, and any other embeds
document.querySelectorAll("object, video, embed").forEach(function (embed) {
var data = embed.getAttribute("data");
if (data && data.startsWith("http:")) {
embed.setAttribute("data", toHttps(data));
}
var src = embed.getAttribute("src");
if (src && src.startsWith("http:")) {
embed.setAttribute("src", toHttps(src));
}
});
// Additional code to handle <script> tags for JavaScript files
document.querySelectorAll("script").forEach(function (script) {
// Check if the script src attribute exists and if it starts with 'http:'
if (script.src && script.src.startsWith("http:")) {
// Replace 'http:' with 'https:' in the script's src attribute
script.src = toHttps(script.src);
}
});
// Convert CSS @import in <style> elements
document.querySelectorAll("style").forEach(function (style) {
if (style.innerHTML.includes("http:")) {
style.innerHTML = toHttps(style.innerHTML);
}
});
function replaceImportWithLink() {
// Search for all <style> tags in the document
document.querySelectorAll("style").forEach(function (style) {
// Check if the style tag contains the specific @import directive
if (style.innerHTML.includes('@import "/misc/drupal.css";')) {
// Create a new link element for the stylesheet
var link = document.createElement("link");
link.type = "text/css";
link.href = "https://arta.cityplex.ro/misc/drupal.css"; // Changed to HTTPS
link.rel = "stylesheet";
// Append the new link element to the <head>
document.head.appendChild(link);
// Remove the <style> tag that contained the @import
style.parentNode.removeChild(style);
}
});
console.log("Replaced @import with <link> for drupal.css");
}
// Call the function to perform the replacement
replaceImportWithLink();
console.log("Conversion to HTTPS completed.");
var scripts = [
"https://arta.cityplex.ro/themes/alexa%20cityplex/httprequest.js",
"https://arta.cityplex.ro/themes/alexa%20cityplex/m_ajax.js",
"https://arta.cityplex.ro/themes/alexa%20cityplex/ticker.js",
"https://code.jquery.com/jquery-1.11.3.min.js",
"https://arta.cityplex.ro/themes/alexa%20cityplex/js/owl.carousel.min.js",
"https://arta.cityplex.ro/themes/alexa%20cityplex/js/fancybox/jquery.fancybox.pack.js?v=2.1.5",
"https://arta.cityplex.ro/themes/alexa%20cityplex/js/fancybox/helpers/jquery.fancybox-buttons.js?v=1.0.5",
"https://arta.cityplex.ro/themes/alexa%20cityplex/js/fancybox/helpers/jquery.fancybox-media.js?v=1.0.6",
"https://arta.cityplex.ro/themes/alexa%20cityplex/videojs/video.js",
"https://arta.cityplex.ro/themes/alexa%20cityplex/js/fancybox/helpers/jquery.fancybox-thumbs.js?v=1.0.7",
"https://arta.cityplex.ro/themes/alexa%20cityplex/js/main.js",
"https://platform.twitter.com/widgets.js",
"https://connect.facebook.net/en_US/sdk.js",
"https://arta.cityplex.ro/themes/alexa%20cityplex/js/bootstrap.min.js",
];
// Function to dynamically load a script
function loadScript(src) {
var script = document.createElement("script");
script.src = src;
script.async = false; // To ensure scripts are loaded in order
document.body.appendChild(script); // Append to body or head as needed
}
// Iterate through the scripts array and load each script
scripts.forEach(function (src) {
loadScript(src);
});
console.log("All external JS scripts have been loaded.");
})(); |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Ingreso</title>
<link rel="stylesheet" href="assets/css/normalize.css">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Roboto:ital,wght@0,100;0,300;0,400;0,500;0,700;0,900;1,100;1,300;1,400;1,500;1,700;1,900&display=swap" rel="stylesheet">
<link rel="stylesheet" href="assets/css/stylesLoginRegister.css">
<style>
.highlight {
background-color: red;
}
</style>
<script>
function validateEmail() {
var x = document.getElementById("myEmail").value;
if (x == "" || x == null || !x.includes("@")) {
document.getElementById("myEmail").className = "highlight";
} else {
document.getElementById("myEmail").className = "";
}
}
</script>
<script>
function validatePass() {
var pass1 = document.getElementById("myPass1").value;
var pass2 = document.getElementById("myPass2").value;
if (pass1 == "" || pass2 == "" || pass1 == null || pass2 == null || pass1 !== pass2) {
document.getElementById("myPass1").className = "highlight";
document.getElementById("myPass2").className = "highlight";
} else {
document.getElementById("myPass1").className = "";
document.getElementById("myPass2").className = "";
}
}
</script>
</head>
<body>
<main>
<div class="contenedor__todo">
<div class="caja__trasera">
<div class="caja__trasera-login">
<h3>¿Ya tienes una cuenta?</h3>
<p>Inicia sesión para entrar a la página</p>
<button id="btn__iniciar-sesion">Iniciar Sesión</button>
</div>
<div class="caja__trasera-register">
<h3>¿Aún no tienes cuenta?</h3>
<p>Regístrate para que puedas iniciar sesión</p>
<button id="btn__registrarse">Registrarse</button>
</div>
</div>
<!--Formulario de login y registro-->
<div class="contenedor__login-register">
<!--Login-->
<form action="" class="formulario__login">
<h2>Iniciar Sesión</h2>
<input type="text" placeholder="Usuario">
<input type="password" placeholder="Contraseña">
<button>Entrar</button>
</form>
<!--Registro-->
<form action="" class="formulario__register">
<h2>Registrarse</h2>
<input type="text" id="myEmail" placeholder="Correo Electronico" onblur="validateEmail()">
<input type="text" placeholder="Usuario">
<input type="password" id="myPass1" placeholder="Contraseña" onblur="validatePass()">
<input type="password" id="myPass2" placeholder="Verificar Contraseña" onblur="validatePass()">
<button>Registrarse</button>
</form>
</div>
</div>
</main>
<script src="assets/js/scriptLoginRegister.js"></script>
</body>
</html> |
import { useContext } from 'react';
import { ThemeContext } from '../context/ThemeContext';
const WorksCard = ({ data }) => {
const { theme } = useContext(ThemeContext);
const { name, img, live, code, tags } = data;
return (
<article className={`'works-card'`}>
<div
className={`works-card__content ${
theme === 'dark' ? 'glow-on-hover' : 'works-card--border-white'
}`}
>
<div className='works-card__img'>
<img src={img} alt={name} className='img-fluid-w' />
</div>
<h3 className='works-card__name ttc'>{name}</h3>
<div className='works-card__tags'>
{tags.map((tag) => {
return (
<span className='works-card__tag ttc' key={tag}>
{tag}
</span>
);
})}
</div>
</div>
<div className='works-card__links'>
<a
href={code}
rel='noreferrer'
className={`works-card__link btn btn--primary ${theme}`}
target='_blank'
>
source code
</a>
<a
href={live}
rel='noreferrer'
className={`works-card__link btn btn--primary ${theme}`}
target='_blank'
>
demo
</a>
</div>
</article>
);
};
export default WorksCard; |
# Effective STL [6] | 警惕C++最令人恼怒的解析
## 函数声明的几种方式
1. 声明一个函数f带有一个double而且返回一个int:
```c++
int f(double d);
```
2. 名为d的参数左右的括号是多余的,被忽略:
```c++
int f(double (d)); // 同上;d左右的括号被忽略
```
3. 省略了参数名:
```c++
int f(double); // 同上;参数名被省略
```
4. 第一个声明了一个函数g,它带有一个参数,那个参数是指向一个没有参数、返回double的函数的指针:
```c++
int g(double (*pf)()); // g带有一个指向函数的指针作为参数
```
5. 唯一的不同是pf使用非指针语法来声明(一个在C和C++中都有效的语法):
```c++
int g(double pf()); // 同上;pf其实是一个指针
```
6. 照常,参数名可以省略,所以这是g的第三种声明,去掉了pf这个名字:
```c++
int g(double ()); // 同上;参数名省略
```
注意参数名左右的括号(就像f的第二种声明中的d)和单独的括号(正如本例)之间的区别。
**参数名左右的括号被忽略,但单独的括号指出存在一个参数列表:它们声明了存在指向函数的指针的参数。**
## 问题探讨
假设有一个int的文件,想要把那些int拷贝到一个list中,可能会使用下面代码:
```c++
ifstream dataFile("ints.dat");
// 警告!这完成的并不是像你想象的那样
list<int> data(istream_iterator<int>(dataFile),
istream_iterator<int>());
```
这里的想法是传一对`istream_iterator`给`list`的区间构造函数,因此把int从文件拷贝到list中。
这段代码可以编译,但是运行时什么都不会做,不会从文件中读出任何数据,甚至不会构建1个`list`。
第二句并不声明list,也不调用构造函数。
这声明了一个函数data,它的返回类型是`list<int>`。这个函数data带有两个参数:
● 第1个参数叫做dataFile。它的类型是`istream_iterator<int>`。dataFile左右的括号是多余的而且被忽略。
● 第2个参数没有名字。它的类型是指向一个没有参数而且返回`istream_iterator<int>`的函数的指针。
就像下面具有这条规则的代码:
```c++
class Widget {...}; // 假设Widget有默认构造函数
Widget w();
```
这并没有声明一个叫做w的Widget,它声明了一个叫作w的没有参数且返回Widget的函数。
本来代码的初衷,是用一个文件的内容来初始化一个`list<int>`对象,现在并没有达到我们的期望。
## 解决办法
1. 函数调用前后增加括号
用括号包围一个实参的声明是不合法的,但用括号包围一个函数调用的观点是合法的,所以通过增加一对括号,代码变为:
```c++
list<int> data((istream_iterator<int>(dataFile)), // 注意在list构造函数的第一个实参左右的新括号
istream_iterator<int>());
```
这是可能的声明数据方法,给予`istream_iterators`的实用性和区间构造函数。
2. 命名迭代器对象
```c++
ifstream dataFile("ints.dat");
istream_iterator<int> dataBegin(dataFile);
istream_iterator<int> dataEnd;
list<int> data(dataBegin, dataEnd);
```
命名迭代器对象的使用和普通的STL编程风格相反,但是你得判断这种方法对编译器和必须使用编译器的人都模棱两可的代码是一个值得付出的代价。
---
> 作者: [Jian YE](https://github.com/jianye0428)
> URL: https://jianye0428.github.io/posts/clause_6/ |
var http = require('http')
var fs = require('fs')
//1.创建server
var server = http.createServer()
var wwwDir = 'F:/www'
server.on('request', function (req, res) {
var url = req.url
fs.readFile('./template.html', function (err, data) {
if (err) {
return res.end('404')
}
// 1.如何得到wwwDir目录列表中的文件名和目录名
// fs.readdir
//2.如何将得到的文件名和目录名替换到template.html中
// 2.1在template.html中需要替换的位置预留一个特殊标记(就像以前模板引擎的标一样)
// 2.2根据files生成需要的html内容
fs.readdir(wwwDir, function (err, files) {
if (err) {
return res.end('can not find www dir')
}
// 2.1生成需要替换的内容
var content = ''
files.forEach(function (item) {//files是一个数组[ 'a.txt', 'a.txt.bak', 'apple', 'index.html' ]
//在Ecmascript6的 ` 字符串中,可以使用${}来引用变量
content += `
<tr>
<td data-value="apple/"><a class="icon dir" href="/F:/www/apple/">${item}/</a></td>
<td class="detailsColumn" data-value="0"></td>
<td class="detailsColumn" data-value="1564754890">2019/8/2 下午10:08:10</td>
</tr>
`
})
//2.3替换
data = data.toString()
//普通的字符串解析替换,浏览器看到的结构就不一样了
// data.replace('^_^','apple')
data = data.replace('^_^', content)
//3.发送解析替换后的响应数据
res.end(data)
})
})
})
//3.绑定端口号,启动服务
server.listen(3000, function () {
console.log('running....')
}) |
<?php
namespace Modules\Doctors\Http\Controllers\Admin;
use App\Services\ApiRequestQueryBuilders\ApiDataTableService;
use App\Services\Response\ResponseService;
use Illuminate\Contracts\Support\Renderable;
use Illuminate\Http\Request;
use Illuminate\Routing\Controller;
use Modules\Content\Services\ContentService;
use Modules\Content\Services\ContentConverters\ImageContentConverter;
use Modules\Content\Services\ContentConverters\VideoContentConverter;
use Modules\Doctors\Entities\DoctorDiplom;
use Modules\Doctors\Http\Requests\Diploms\CreateRequest;
use Modules\Doctors\Http\Requests\Diploms\UpdateRequest;
use Modules\Doctors\Http\Resources\DiplomResource;
class DoctorDiplomsResourceController extends Controller
{
// private ApiDataTableService $QueryBuilderByRequest;
private ContentService $contentService;
public function __construct(
//ReviewService $reviewService,
// ApiDataTableService $apiHandler,//,
ContentService $contentService
) {
// $this->QueryBuilderByRequest = $apiHandler;
$this->contentService = $this->addPreviewServiceForContent($contentService);
}
/**
* Display a listing of the resource.
* @return Renderable
*/
public function index(Request $request)
{
$diploms = DoctorDiplom::query();
$diploms->with(['content' => function ($query) {
$query->where('type', 'original')->where('confirm', 1);
}]);
// $dbconnect = DB::connection('MODX')->getPDO();
// $dbname = DB::connection('MODX')->select('SHOW TABLES FROM east_prod');
// dd($dbname);
//necessarily models to collection must get with pagination data: collection($model->paginate())
//ReviewResource
// return response()->apiCollection( $reviews );
return ResponseService::apiCollection( DiplomResource::collection($diploms->paginate()) );
}
/**
* Store a newly created resource in storage.
* @param Request $request
* @return Renderable
*/
public function store(CreateRequest $request)
{
$requestData = $request->validated();
$diplom = DoctorDiplom::create($requestData);
if(isset($requestData['content']) && $requestData['content']) {
$this->contentService->store( $requestData['content'], DoctorDiplom::class, $diplom->id );
}
return response()->ok( (new DiplomResource($diplom))->jsonSerialize(), ['message' => 'Diplom created'], 200 );
}
/**
* Update the specified resource in storage.
* @param Request $request
* @param int $id
* @return Renderable
*/
public function update(UpdateRequest $request, $id)
{
$requestData = $request->validated();
if($diplom = DoctorDiplom::where('id', $id)->first()){
$diplom -> update($requestData);
if($requestData['content']) {
$this->contentService->store( $requestData['content'], DoctorDiplom::class, $id );
}
return response()->ok( new DiplomResource($diplom), ['message' => 'Diplom updated'], 200 );
}
ResponseService::error('Do not find doctor');
}
/**
* Remove the specified resource from storage.
* @param int $id
* @return Renderable
*/
public function destroy($id)
{
if(DoctorDiplom::where('id', $id)->first()){
DoctorDiplom::destroy($id);
return ResponseService::okMessage('Removed diplom');
}else{
return ResponseService::error('Failed to remove diplom');
}
}
protected function addPreviewServiceForContent( ContentService $contentService ):ContentService{
$contentService->addContentConverter( (new ImageContentConverter())
->withKey('300x300')
->withExtension('webp')
->withSize(300, 300)) ;
$contentService->addContentConverter( (new VideoContentConverter())
->withKey('300x300')
->withExtension('webm')
->withSize(300, 300)) ;
return $contentService;
}
} |
/*Query 1:
-- For each age(in years), how many patients have gone for treatment?
SELECT DATEDIFF(hour, dob , GETDATE())/8766 AS age, count(*) AS numTreatments
FROM Person
JOIN Patient ON Patient.patientID = Person.personID
JOIN Treatment ON Treatment.patientID = Patient.patientID
group by DATEDIFF(hour, dob , GETDATE())/8766
order by numTreatments desc;
*/
SELECT concat(round(DATEDIFF( current_date() , dob)/365.25,-1), ' - ', round(DATEDIFF( current_date() , dob)/365.25,-1)+10) AS age_group, count(*) AS numTreatments
FROM Patient
JOIN Treatment ON Treatment.patientID = Patient.patientID
group by age_group
order by numTreatments desc;
/*Query 2:
-- For each city, Find the number of registered people, number of pharmacies, and number of insurance companies.
drop table if exists T1;
drop table if exists T2;
drop table if exists T3;
select Address.city, count(Pharmacy.pharmacyID) as numPharmacy
into T1
from Pharmacy right join Address on Pharmacy.addressID = Address.addressID
group by city
order by count(Pharmacy.pharmacyID) desc;
select Address.city, count(InsuranceCompany.companyID) as numInsuranceCompany
into T2
from InsuranceCompany right join Address on InsuranceCompany.addressID = Address.addressID
group by city
order by count(InsuranceCompany.companyID) desc;
select Address.city, count(Person.personID) as numRegisteredPeople
into T3
from Person right join Address on Person.addressID = Address.addressID
group by city
order by count(Person.personID) desc;
select T1.city, T3.numRegisteredPeople, T2.numInsuranceCompany, T1.numPharmacy
from T1, T2, T3
where T1.city = T2.city and T2.city = T3.city
order by numRegisteredPeople desc;
*/
-- remove right join,
with pharmacy_count as
(select city, count(pharmacyID) as numPharmacy
from Pharmacy join Address using(addressID)
group by city
order by numPharmacy desc),
Insurance_company_Count as
(select city, count(companyID) as numInsuranceCompany
from InsuranceCompany join Address using(addressID)
group by city
order by numInsuranceCompany desc),
Registered_people_count as
(select city, count(personID) as numRegisteredPeople
from Person join Address using(addressID)
group by city
order by numRegisteredPeople desc)
select city, coalesce(numRegisteredPeople,0) as numRegisteredPeople,
coalesce(numInsuranceCompany,0) as numInsuranceCompany,
coalesce(numInsuranceCompany,0) as numInsuranceCompany
from (select distinct city from address) as city_name
left join Registered_people_count using(city)
left join pharmacy_count using (city)
left join Insurance_company_Count using(city)
order by numRegisteredPeople desc;
/* Query 3:
-- Total quantity of medicine for each prescription prescribed by Ally Scripts
-- If the total quantity of medicine is less than 20 tag it as "Low Quantity".
-- If the total quantity of medicine is from 20 to 49 (both numbers including) tag it as "Medium Quantity".
-- If the quantity is more than equal to 50 then tag it as "High quantity".
select
C.prescriptionID, sum(quantity) as totalQuantity,
CASE WHEN sum(quantity) < 20 THEN 'Low Quantity'
WHEN sum(quantity) < 50 THEN 'Medium Quantity'
ELSE 'High Quantity' END AS Tag
FROM Contain C
JOIN Prescription P
on P.prescriptionID = C.prescriptionID
JOIN Pharmacy on Pharmacy.pharmacyID = P.pharmacyID
where Pharmacy.pharmacyName = 'Ally Scripts'
group by C.prescriptionID;
*/
select prescriptionID, sum(quantity) as totalQuantity,
CASE
WHEN sum(quantity) < 20 THEN 'Low Quantity'
WHEN sum(quantity) < 50 THEN 'Medium Quantity'
ELSE 'High Quantity'
END AS Tag
FROM Contain
JOIN Prescription using(prescriptionID)
where PharmacyID = (select pharmacyID from pharmacy where pharmacyName = 'Ally Scripts')
group by prescriptionID;
/* Query 4:
-- The total quantity of medicine in a prescription is the sum of the quantity of all the medicines in the prescription.
-- Select the prescriptions for which the total quantity of medicine exceeds
-- the avg of the total quantity of medicines for all the prescriptions.
drop table if exists T1;
select Pharmacy.pharmacyID, Prescription.prescriptionID, sum(quantity) as totalQuantity
into T1
from Pharmacy
join Prescription on Pharmacy.pharmacyID = Prescription.pharmacyID
join Contain on Contain.prescriptionID = Prescription.prescriptionID
join Medicine on Medicine.medicineID = Contain.medicineID
join Treatment on Treatment.treatmentID = Prescription.treatmentID
where YEAR(date) = 2022
group by Pharmacy.pharmacyID, Prescription.prescriptionID
order by Pharmacy.pharmacyID, Prescription.prescriptionID;
select * from T1
where totalQuantity > (select avg(totalQuantity) from T1);
*/
with medicine_quantity as
(select pharmacyID, prescriptionID, sum(quantity) as totalQuantity
from Prescription
join Contain using(prescriptionID)
join treatment using(treatmentID)
where YEAR(date) = 2022
group by pharmacyID, prescriptionID
order by pharmacyID, prescriptionID)
select * from medicine_quantity
where totalQuantity > (select avg(totalQuantity) from medicine_quantity);
/* Query 5:
-- Select every disease that has 'p' in its name, and
-- the number of times an insurance claim was made for each of them.
SELECT Disease.diseaseName, COUNT(*) as numClaims
FROM Disease
JOIN Treatment ON Disease.diseaseID = Treatment.diseaseID
JOIN Claim On Treatment.claimID = Claim.claimID
WHERE diseaseName IN (SELECT diseaseName from Disease where diseaseName LIKE '%p%')
GROUP BY diseaseName;
*/
SELECT diseaseName, COUNT(distinct claimID) as numClaims
FROM Disease
JOIN Treatment using(diseaseID)
WHERE diseaseName rlike 'p'
GROUP BY diseaseName; |
import discord
from discord import NotFound, Interaction
from utility.discord import voice_chat
from utility.tools import music_tools
from discord.ext import bridge
import math
import asyncio
from utility.scraping import YouTube
class list_view(discord.ui.View):
"""
View for the music bot playlist
"""
def __init__(self, music_self, ctx: bridge.BridgeExtContext | bridge.BridgeApplicationContext):
super().__init__(timeout=None)
self.tools: music_tools.music_tools = music_self.tools
self.bot: bridge.Bot = music_self.bot
self.embed = None
self.index = 0
self.server = ctx.guild.id
self.ctx = ctx
self.update_buttons()
async def interaction_check(self, interaction) -> bool:
"""
Checks if the user can execute these commands
"""
if await self.bot.is_owner(interaction.user):
return True
if interaction.user.bot:
return False # if the user is bot
if interaction.user.voice == None:
return False # if the user is not in the same voice chat
if interaction.message.author.voice == None:
return False # if the bot is not currently in a voice channel
if interaction.user.voice.channel == interaction.message.author.voice.channel:
return True # if the bot and the user are in the same voice channel
return False
def update_buttons(self): # holy fuck
"""
Updates the buttons accordingly
"""
self.children[0].options = self.tools.create_options(self.ctx)
playlist_length = math.ceil(len(self.tools.playlist[self.server][0]) / 50)
for child in self.children:
child.disabled = False
if self.index == 0: # no more to go back
self.children[1].disabled = True # super back
self.children[2].disabled = True # back
if self.index >= playlist_length - 1: # no more to forward
self.children[4].disabled = True # for
self.children[5].disabled = True # super for
if self.tools.playlist[self.server][0] == []: # if the entire list is empty
for child in self.children:
child.disabled = True
self.children[3].disabled = False # refresh
self.index = 0
def update_embed(self):
"""
Updates the list embed to match the playlist
"""
for _ in range(0, self.index + 1):
self.embed = self.tools.create_embed(self.ctx, self.index)
if self.embed.description != '':
break
self.index -= 1
@discord.ui.select(placeholder='Choose page...', min_values=0, row=0)
async def select_callback(self, select: discord.ui.Select, interaction: Interaction):
"""
Select the page
"""
value = int(select.values[0])
self.index = value
self.update_embed()
self.update_buttons()
return await interaction.response.edit_message(embed=self.embed, view=self)
@discord.ui.button(label='FIRST PAGE', emoji='⏪', style=discord.ButtonStyle.red, row=1, disabled=True)
async def super_backward_callback(self, button, interaction: Interaction):
"""
Move back to the first page
"""
self.index = 0
self.update_embed()
self.update_buttons()
return await interaction.response.edit_message(embed=self.embed, view=self)
@discord.ui.button(label='PREVIOUS PAGE', emoji='◀️', style=discord.ButtonStyle.red, row=1, disabled=True)
async def backward_callback(self, button, interaction: Interaction):
"""
Go back one page
"""
self.index -= 1
self.update_embed()
self.update_buttons()
return await interaction.response.edit_message(embed=self.embed, view=self)
@discord.ui.button(label='REFRESH', emoji='🔄', style=discord.ButtonStyle.red, row=1)
async def refresh_callback(self, button, interaction: Interaction):
"""
Refresh the list
"""
self.update_embed()
self.update_buttons()
return await interaction.response.edit_message(embed=self.embed, view=self)
@discord.ui.button(label='NEXT PAGE', emoji='▶️', style=discord.ButtonStyle.red, row=1)
async def forward_callback(self, button, interaction: Interaction):
"""
Go forward one page
"""
self.index += 1
self.update_embed()
self.update_buttons()
return await interaction.response.edit_message(embed=self.embed, view=self)
@discord.ui.button(label='LAST PAGE', emoji='⏩', style=discord.ButtonStyle.red, row=1)
async def super_forward_callback(self, button, interaction: Interaction):
"""
Go to the last page
"""
self.index = math.ceil(len(self.tools.playlist[self.server][0]) / 50) - 1
self.update_embed()
self.update_buttons()
return await interaction.response.edit_message(embed=self.embed, view=self)
@discord.ui.button(label='SKIP', emoji='⏭️', style=discord.ButtonStyle.red, row=2)
async def skip_callback(self, button, interaction: Interaction):
"""
Skips the currently playing song
"""
voice_chat.resume(self.ctx)
voice_chat.stop(self.ctx)
await asyncio.sleep(0.5)
self.update_embed()
self.update_buttons()
return await interaction.response.edit_message(embed=self.embed, view=self)
@discord.ui.button(label='SHUFFLE', emoji='🔀', style=discord.ButtonStyle.red, row=2)
async def shuffle_callback(self, button, interaction: Interaction):
"""
Shuffles the playlist
"""
if self.tools.playlist[self.server][0] == []:
return
self.tools.shuffle_playlist(self.ctx.guild.id)
self.update_embed()
self.update_buttons()
return await interaction.response.edit_message(embed=self.embed, view=self)
@discord.ui.button(label='LOOP', emoji='🔁', style=discord.ButtonStyle.red, row=2)
async def loop_callback(self, button, interaction: Interaction):
"""
Enable / disable looping
"""
self.tools.looping[self.server] = not self.tools.looping[self.server]
await interaction.response.edit_message(view=self)
await self.tools.looping_response(self.ctx)
@discord.ui.button(label='PAUSE/RESUME', emoji='⏯️', style=discord.ButtonStyle.red, row=2)
async def pauseresume_callback(self, button, interaction: Interaction):
"""
Pause / resume playing
"""
vc = self.tools.voice_client[self.server]
if not vc.is_connected():
return
await interaction.response.edit_message(view=self)
if vc.is_paused():
return voice_chat.resume(self.ctx)
elif self.tools.playlist[self.server] != [] and not vc.is_playing():
return await self.tools.play_song(self.ctx)
voice_chat.pause(self.ctx)
class song_view(discord.ui.View):
def __init__(self, song: YouTube.Video):
super().__init__(timeout=None)
song_link = discord.ui.Button( # link to the video itself
label='Video',
style=discord.ButtonStyle.link,
url=f'https://www.youtube.com/watch?v={song.id}'
)
channel_link = discord.ui.Button( # link to the video creator's channel
label='Channel',
style=discord.ButtonStyle.link,
url=f'https://www.youtube.com/channel/{song.channel_id}'
)
self.add_item(song_link)
self.add_item(channel_link) |
# Public Repositorie Github Search Frontend
This is a simple frontend application that allows you to search for repositories using the Github API. It's built with React and designed to be easy to set up and use.
## Prerequisites
Before you can use this application, you need to obtain a GitHub API authorization token. Here's how you can get one:
1. **Sign In to GitHub:**
- If you don't already have a GitHub account, you'll need to create one. You can sign up for a free GitHub account at [GitHub Signup](https://github.com/join).
2. **Navigate to Developer Settings:**
- Once you're logged in to your GitHub account, click on your profile picture in the top right corner.
- From the dropdown menu, select "Settings."
- In the left sidebar, click on "Developer settings."
3. **Access Personal Access Tokens:**
- Under the "Access tokens" section in the Developer settings, click "Personal access tokens."
4. **Generate a New Token:**
- Click the "Generate token" button to create a new personal access token.
5. **Configure Token Permissions:**
- Give your token a descriptive name.
- Select the permissions (scopes) you need for your token. In most cases, for read-only access to public repositories, you can select "public_repo."
- If your application requires additional permissions, you can select those as well.
6. **Generate Token:**
- After configuring the settings, scroll down and click the "Generate token" button.
7. **Copy and Save Token:**
- Once the token is generated, GitHub will display it. Make sure to copy this token and save it in a secure location. You won't be able to see it again.
Now you have an authentication token that can be used with the application to access GitHub's API. Be cautious with this token and avoid sharing it publicly, as it provides access to your GitHub account.
## Setup
1. Clone this repository to your local machine.
```
git clone https://github.com/mateusdreher/o2filmes-challenge
cd o2filmes-challenge
```
2. Create a .env file in the root of the application and put your github token in it as follows:
```
REACT_APP_GITHUB_TOKEN=YOUR_GITHUB_TOKEN
```
You can use the provided .env.example file as a template.
3. Install the project dependencies.
```
npm install
```
## Usage
Once you have set up your Giphy API key and installed the dependencies, you can start the application by running:
npm start
The application will start, and you can access it in your web browser at http://localhost:3000.
Enter your search query in the input field and press Enter to see repositories related to your query. |
// Unless explicitly stated otherwise all files in this repository are licensed under the Apache-2.0 License.
// This product includes software developed at Datadog (https://www.datadoghq.com/).
// Copyright 2019-Present Datadog, Inc.
use serde::de::{Error, MapAccess, Visitor};
use serde::{Deserialize, Deserializer, Serialize};
use serde_with::skip_serializing_none;
use std::fmt::{self, Formatter};
/// Response object for an organization creation.
#[non_exhaustive]
#[skip_serializing_none]
#[derive(Clone, Debug, PartialEq, Serialize)]
pub struct OrganizationCreateResponse {
/// Datadog API key.
#[serde(rename = "api_key")]
pub api_key: Option<crate::datadogV1::model::ApiKey>,
/// An application key with its associated metadata.
#[serde(rename = "application_key")]
pub application_key: Option<crate::datadogV1::model::ApplicationKey>,
/// Create, edit, and manage organizations.
#[serde(rename = "org")]
pub org: Option<crate::datadogV1::model::Organization>,
/// Create, edit, and disable users.
#[serde(rename = "user")]
pub user: Option<crate::datadogV1::model::User>,
#[serde(skip)]
#[serde(default)]
pub(crate) _unparsed: bool,
}
impl OrganizationCreateResponse {
pub fn new() -> OrganizationCreateResponse {
OrganizationCreateResponse {
api_key: None,
application_key: None,
org: None,
user: None,
_unparsed: false,
}
}
pub fn api_key(mut self, value: crate::datadogV1::model::ApiKey) -> Self {
self.api_key = Some(value);
self
}
pub fn application_key(mut self, value: crate::datadogV1::model::ApplicationKey) -> Self {
self.application_key = Some(value);
self
}
pub fn org(mut self, value: crate::datadogV1::model::Organization) -> Self {
self.org = Some(value);
self
}
pub fn user(mut self, value: crate::datadogV1::model::User) -> Self {
self.user = Some(value);
self
}
}
impl Default for OrganizationCreateResponse {
fn default() -> Self {
Self::new()
}
}
impl<'de> Deserialize<'de> for OrganizationCreateResponse {
fn deserialize<D>(deserializer: D) -> Result<Self, D::Error>
where
D: Deserializer<'de>,
{
struct OrganizationCreateResponseVisitor;
impl<'a> Visitor<'a> for OrganizationCreateResponseVisitor {
type Value = OrganizationCreateResponse;
fn expecting(&self, f: &mut Formatter<'_>) -> fmt::Result {
f.write_str("a mapping")
}
fn visit_map<M>(self, mut map: M) -> Result<Self::Value, M::Error>
where
M: MapAccess<'a>,
{
let mut api_key: Option<crate::datadogV1::model::ApiKey> = None;
let mut application_key: Option<crate::datadogV1::model::ApplicationKey> = None;
let mut org: Option<crate::datadogV1::model::Organization> = None;
let mut user: Option<crate::datadogV1::model::User> = None;
let mut _unparsed = false;
while let Some((k, v)) = map.next_entry::<String, serde_json::Value>()? {
match k.as_str() {
"api_key" => {
if v.is_null() {
continue;
}
api_key = Some(serde_json::from_value(v).map_err(M::Error::custom)?);
}
"application_key" => {
if v.is_null() {
continue;
}
application_key =
Some(serde_json::from_value(v).map_err(M::Error::custom)?);
}
"org" => {
if v.is_null() {
continue;
}
org = Some(serde_json::from_value(v).map_err(M::Error::custom)?);
}
"user" => {
if v.is_null() {
continue;
}
user = Some(serde_json::from_value(v).map_err(M::Error::custom)?);
}
&_ => {}
}
}
let content = OrganizationCreateResponse {
api_key,
application_key,
org,
user,
_unparsed,
};
Ok(content)
}
}
deserializer.deserialize_any(OrganizationCreateResponseVisitor)
}
} |
local util = require 'utility'
local await = require 'await'
local pub = require 'pub'
local jsonrpc = require 'jsonrpc'
local define = require 'proto.define'
local json = require 'json'
local inspect = require 'inspect'
local platform = require 'bee.platform'
local fs = require 'bee.filesystem'
local net = require 'service.net'
local timer = require 'timer'
local reqCounter = util.counter()
local function logSend(buf)
if not RPCLOG then
return
end
log.info('rpc send:', buf)
end
local function logRecieve(proto)
if not RPCLOG then
return
end
log.info('rpc recieve:', json.encode(proto))
end
---@class proto
local m = {}
m.ability = {}
m.waiting = {}
m.holdon = {}
m.mode = 'stdio'
m.client = nil
function m.getMethodName(proto)
if proto.method:sub(1, 2) == '$/' then
return proto.method, true
else
return proto.method, false
end
end
---@param callback async fun()
function m.on(method, callback)
m.ability[method] = callback
end
function m.send(data)
local buf = jsonrpc.encode(data)
logSend(buf)
if m.mode == 'stdio' then
io.write(buf)
elseif m.mode == 'socket' then
m.client:write(buf)
end
end
function m.response(id, res)
if id == nil then
log.error('Response id is nil!', inspect(res))
return
end
if not m.holdon[id] then
log.error('Unknown response id!', id)
return
end
m.holdon[id] = nil
local data = {}
data.id = id
data.result = res == nil and json.null or res
m.send(data)
end
function m.responseErr(id, code, message)
if id == nil then
log.error('Response id is nil!', inspect(message))
return
end
if not m.holdon[id] then
log.error('Unknown response id!', id)
return
end
m.holdon[id] = nil
m.send {
id = id,
error = {
code = code,
message = message,
}
}
end
function m.notify(name, params)
m.send {
method = name,
params = params,
}
end
---@async
function m.awaitRequest(name, params)
local id = reqCounter()
m.send {
id = id,
method = name,
params = params,
}
local result, error = await.wait(function (resume)
m.waiting[id] = {
id = id,
method = name,
params = params,
resume = resume,
}
end)
if error then
log.warn(('Response of [%s] error [%d]: %s'):format(name, error.code, error.message))
end
return result
end
function m.request(name, params, callback)
local id = reqCounter()
m.send {
id = id,
method = name,
params = params,
}
m.waiting[id] = {
id = id,
method = name,
params = params,
resume = function (result, error)
if error then
log.warn(('Response of [%s] error [%d]: %s'):format(name, error.code, error.message))
end
if callback then
callback(result)
end
end
}
end
local secretOption = {
process = function (item, path)
if path[1] == 'params'
and path[2] == 'textDocument'
and path[3] == 'text'
and path[4] == nil then
return '"***"'
end
return item
end
}
function m.doMethod(proto)
logRecieve(proto)
local method, optional = m.getMethodName(proto)
local abil = m.ability[method]
if proto.id then
m.holdon[proto.id] = proto
end
if not abil then
if not optional then
log.warn('Recieved unknown proto: ' .. method)
end
if proto.id then
m.responseErr(proto.id, define.ErrorCodes.MethodNotFound, method)
end
return
end
await.call(function () ---@async
--log.debug('Start method:', method)
if proto.id then
await.setID('proto:' .. proto.id)
end
local clock = os.clock()
local ok = false
local res
-- 任务可能在执行过程中被中断,通过close来捕获
local response <close> = function ()
local passed = os.clock() - clock
if passed > 0.5 then
log.warn(('Method [%s] takes [%.3f]sec. %s'):format(method, passed, inspect(proto, secretOption)))
end
--log.debug('Finish method:', method)
if not proto.id then
return
end
await.close('proto:' .. proto.id)
if ok then
m.response(proto.id, res)
else
m.responseErr(proto.id, proto._closeReason or define.ErrorCodes.InternalError, proto._closeMessage or res)
end
end
ok, res = xpcall(abil, log.error, proto.params, proto.id)
await.delay()
end)
end
function m.close(id, reason, message)
local proto = m.holdon[id]
if not proto then
return
end
proto._closeReason = reason
proto._closeMessage = message
await.close('proto:' .. id)
end
function m.doResponse(proto)
logRecieve(proto)
local id = proto.id
local waiting = m.waiting[id]
if not waiting then
log.warn('Response id not found: ' .. inspect(proto))
return
end
m.waiting[id] = nil
if proto.error then
waiting.resume(nil, proto.error)
return
end
waiting.resume(proto.result)
end
function m.listen(mode, socketPort)
m.mode = mode
if mode == 'stdio' then
log.info('Listen Mode: stdio')
if platform.os == 'windows' then
local windows = require 'bee.windows'
windows.filemode(io.stdin, 'b')
windows.filemode(io.stdout, 'b')
end
io.stdin:setvbuf 'no'
io.stdout:setvbuf 'no'
pub.task('loadProtoByStdio')
elseif mode == 'socket' then
local unixFolder = LOGPATH .. '/unix'
fs.create_directories(fs.path(unixFolder))
local unixPath = unixFolder .. '/' .. tostring(socketPort)
local server = net.listen('unix', unixPath)
log.info('Listen Mode: socket')
log.info('Listen Port:', socketPort)
log.info('Listen Path:', unixPath)
assert(server)
local dummyClient = {
buf = '',
write = function (self, data)
self.buf = self.buf.. data
end,
update = function () end,
}
m.client = dummyClient
function server:on_accepted(client)
m.client = client
client:write(dummyClient.buf)
return true
end
function server:on_error(...)
log.error(...)
end
pub.task('loadProtoBySocket', {
port = socketPort,
unixPath = unixPath,
})
end
end
return m |
package com.jiushi.auth.config;
import com.jiushi.auth.service.JiushiUserDetailsService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.security.oauth2.config.annotation.configurers.ClientDetailsServiceConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configuration.AuthorizationServerConfigurerAdapter;
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableAuthorizationServer;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerEndpointsConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerSecurityConfigurer;
import org.springframework.security.oauth2.provider.ClientDetailsService;
import org.springframework.security.oauth2.provider.TokenGranter;
import org.springframework.security.oauth2.provider.client.JdbcClientDetailsService;
import org.springframework.security.oauth2.provider.code.AuthorizationCodeServices;
import org.springframework.security.oauth2.provider.code.JdbcAuthorizationCodeServices;
import org.springframework.security.oauth2.provider.token.*;
import org.springframework.security.oauth2.provider.token.store.JwtAccessTokenConverter;
import javax.sql.DataSource;
import java.util.ArrayList;
import java.util.List;
/**
* @author dengmingyang
* @version 1.0
* 授权服务配置
**/
@Configuration
@EnableAuthorizationServer
public class AuthorizationServer extends AuthorizationServerConfigurerAdapter {
@Autowired
private TokenStore tokenStore;
@Autowired
private ClientDetailsService clientDetailsService;
@Autowired
private JwtAccessTokenConverter jwtAccessTokenConverter;
@Autowired
private PasswordEncoder passwordEncoder;
@Autowired
private TokenGranter tokenGranter;
//客户端详情服务
@Override
public void configure(ClientDetailsServiceConfigurer clients)
throws Exception {
clients.withClientDetails(clientDetailsService);
}
//将客户端信息存储到数据库
@Bean
public ClientDetailsService clientDetailsService(DataSource dataSource) {
ClientDetailsService clientDetailsService = new JdbcClientDetailsService(dataSource);
((JdbcClientDetailsService) clientDetailsService).setPasswordEncoder(passwordEncoder);
return clientDetailsService;
}
/**
* 设置授权码模式的授权码如何存取
* @param dataSource
* @return
*/
@Bean
public AuthorizationCodeServices authorizationCodeServices(DataSource dataSource) {
return new JdbcAuthorizationCodeServices(dataSource);
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) {
// endpoints
// .authenticationManager(authenticationManager)//认证管理器
// .userDetailsService(userService)
// .tokenServices(tokenService())//令牌管理服务
// .authorizationCodeServices(authorizationCodeServices)//授权码服务
// .allowedTokenEndpointRequestMethods(HttpMethod.POST);
endpoints.tokenGranter(tokenGranter);
}
//令牌管理服务
@Bean
public AuthorizationServerTokenServices tokenService() {
DefaultTokenServices service = new DefaultTokenServices();
service.setClientDetailsService(clientDetailsService);//客户端详情服务
service.setSupportRefreshToken(true);//
service.setTokenStore(tokenStore);//令牌存储策略
//令牌增强
TokenEnhancerChain tokenEnhancerChain = this.getTokenEnhancerChain();
service.setTokenEnhancer(tokenEnhancerChain);
service.setAccessTokenValiditySeconds(7200); // 令牌默认有效期2小时
service.setRefreshTokenValiditySeconds(259200); // 刷新令牌默认有效期3天
return service;
}
private TokenEnhancerChain getTokenEnhancerChain() {
TokenEnhancerChain tokenEnhancerChain = new TokenEnhancerChain();
List<TokenEnhancer> tokenEnhancers = new ArrayList<>();
tokenEnhancers.add(jwtAccessTokenConverter);
tokenEnhancerChain.setTokenEnhancers(tokenEnhancers);
return tokenEnhancerChain;
}
@Override
public void configure(AuthorizationServerSecurityConfigurer security){
security.allowFormAuthenticationForClients(); //表单认证(申请令牌)
}
} |
#include <iostream>
#include <string>
#include <map>
class SubscriberBook{
private:
std::map<std::string, std::string> subscriber;
public:
void setAddSubscriber(const std::string& name, const std::string& phoneNumber){
subscriber[name] = phoneNumber;
}
const std::string& getNumberPhone(const std::string& nameSubscriber){
auto it = subscriber.find(nameSubscriber);
if (it != subscriber.end()){
return it -> second;
} else {
static const std::string emptyBook;
return emptyBook;
}
}
};
class Phone{
private:
SubscriberBook book;
public:
void addContactBook (const std::string& str1, const std::string& strNumb){
book.setAddSubscriber(str1, strNumb);
}
void callPhone(){
std::string bookEntry;
std::cout << "Enter the name or phone number to call: ";
std::cin >> bookEntry;
const std::string& phoneNumber = book.getNumberPhone(bookEntry);
if (!phoneNumber.empty()) {
std::cout << "CALL " << phoneNumber << std::endl;
} else {
std::cout << "Contact not found." << std::endl;
}
}
void smsPhone(){
std::string contact, message;
std::cout << "Enter the name or phone number to send a message: ";
std::cin >> contact;
std::cin.ignore();
std::cout << "Enter the message: ";
std::getline(std::cin, message);
const std::string& phoneNumber = book.getNumberPhone(contact);
if (!phoneNumber.empty()) {
std::cout << "Sending SMS to " << phoneNumber << ": " << message << std::endl;
} else {
std::cout << "Contact not found." << std::endl;
}
}
};
int main() {
Phone mobilePhone;
std::string command, name, number;
while (true){
std::cout << "Enter command (add, call, sms, exit): ";
std::cin >> command;
if (command == "add") {
std::cout << "Enter the name of the contact: ";
std::cin >> name;
std::cout << "Enter the phone number: ";
std::cin >> number;
mobilePhone.addContactBook(name, number);
} else if (command == "call") {
mobilePhone.callPhone();
} else if (command == "sms") {
mobilePhone.smsPhone();
} else if (command == "exit") {
break;
} else {
std::cout << "Invalid command." << std::endl;
}
}
return 0;
} |
import React, {useContext} from 'react'
import MovieCard from './MovieCard'
import { GlobalContext } from '../../context/GlobalState';
function Watched() {
const {watched} = useContext(GlobalContext);
return (
<div className="movie-page">
<div className="container">
<div className="header">
<h1 className="heading">Watched Movies</h1>
<span className="count-pill">
{watched.length} {watched.length === 1 ? "Movie" : "Movies"}
</span>
</div>
{watched.length > 0 ? (
<div className="movie-grid">
{watched.map((movie) => (
<MovieCard movie={movie} key={movie.id} type="watched" />
))}
</div>
) : (
<h2 className="no-movies">No movies in your list! Add some!</h2>
)}
</div>
</div>
)
}
export default Watched |
"""
Tortoise comes with a pydantic model creating function
which creates the pydantic models for you:
1 - User/SuperInSchema for creating new users
2 - User/SuperOutSchema, user objects for use outside the application
3 - User/SuperDatabaseSchema, user object for use within the application for most for validation
4 - UpdatePassword to update a user's password
"""
from pydantic import BaseModel
from tortoise.contrib.pydantic import pydantic_model_creator
from src.core.models import User
UserInSchema = pydantic_model_creator(
User,
name='UserIn',
exclude=['created_at', 'active'],
exclude_readonly=True
)
UserOutSchema = pydantic_model_creator(
User,
name='User',
exclude=['active', 'entries','fixeds', 'savings_goal', 'superuser'],
)
UserDatabaseSchema = pydantic_model_creator(User, name='User')
class UpdatePassword(BaseModel):
password: str | None |
import { createContext, useState } from "react";
import { useMutation, useQuery } from "react-query";
import { toast } from "react-toastify";
import { IContextProvider } from "../interfaces/Context.interfaces";
import {
IDeleteData,
IRegisterUserProps,
IUpdateUserProps,
IUserDataWithSales,
IUsersContextData,
} from "../interfaces/UsersContext.interfaces";
import jwt_decode from "jwt-decode";
import { api } from "../services/api";
export const UsersContext = createContext<IUsersContextData>(
{} as IUsersContextData
);
export const UsersProvider = ({ children }: IContextProvider) => {
const [userData, setUserData] = useState<IUserDataWithSales>();
const [userProfilData, setUserProfileData] = useState<IUserDataWithSales>();
const { data, isFetching, error, refetch } = useQuery("users", async () => {
const token = localStorage.getItem("@Parking:Token") || "";
const { isAdmin }: any = jwt_decode(token);
if (token && isAdmin) {
api.defaults.headers.common["Authorization"] = `Bearer ${token}`;
const response = await api.get("/users");
return response.data;
}
});
const { mutate: listUser } = useMutation(
async (userId: string): Promise<IUserDataWithSales> => {
const token = localStorage.getItem("@Parking:Token");
api.defaults.headers.common["Authorization"] = `Bearer ${token}`;
return await api.get(`/users/${userId}`).then((response) => {
return response.data;
});
},
{
onSuccess: (response) => {
setUserData(response);
},
onError: () => {
toast.error("Usuario não encontrado");
},
}
);
const { mutate: userProfile } = useMutation(
async (): Promise<IUserDataWithSales> => {
const token = localStorage.getItem("@Parking:Token");
api.defaults.headers.common["Authorization"] = `Bearer ${token}`;
return await api.get(`/users/profile`).then((response) => {
return response.data;
});
},
{
onSuccess: (response) => {
setUserProfileData(response);
},
onError: () => {
toast.error("Usuario não encontrado");
},
}
);
const { mutate: registerUser } = useMutation(
async ({ data, onClose }: IRegisterUserProps) => {
const token = localStorage.getItem("@Parking:Token");
api.defaults.headers.common["Authorization"] = `Bearer ${token}`;
return await api.post("/users", data).then((response) => {
onClose();
return response.data;
});
},
{
onSuccess: (_) => {
toast.success("Usuário cadastrado com sucesso");
refetch();
},
onError: () => {
toast.error("Username já registrado");
},
}
);
const { mutate: deleteUser } = useMutation(
async ({ userId, onClose }: IDeleteData) => {
const token = localStorage.getItem("@Parking:Token");
api.defaults.headers.common["Authorization"] = `Bearer ${token}`;
return await api.delete(`/users/${userId}/`).then((response) => {
onClose();
return response.data;
});
},
{
onSuccess: (_) => {
toast.success("Funcionário deletado");
refetch();
},
onError: () => {
toast.error("Usuário já deletado");
},
}
);
const { mutate: updateUser } = useMutation(
async ({ data, userId, onClose }: IUpdateUserProps) => {
const token = localStorage.getItem("@Parking:Token");
api.defaults.headers.common["Authorization"] = `Bearer ${token}`;
return await api.patch(`/users/${userId}/`, data).then((response) => {
onClose();
return response.data;
});
},
{
onSuccess: (_) => {
toast.success("Dados do Funcionário Atualizados");
refetch();
},
onError: () => {
toast.error("Funcionário não existe");
},
}
);
return (
<UsersContext.Provider
value={{
data,
isFetching,
error,
registerUser,
deleteUser,
updateUser,
listUser,
userData,
userProfile,
userProfilData,
}}
>
{children}
</UsersContext.Provider>
);
}; |
# BongoPawClicker





An auto clicker with bongo cat integrated
🔗[中文文档](./README/README-CN.md)
🔗[Download](https://github.com/Siriusq/BongoPawClicker/releases/download/v1.0/BongoPawClicker.exe)
🔗[Development Summary](https://siriusq.top/en/bongo-paw-blicker.html)
# Preview

# Features
- Random click interval
- Random click area, the program will click at random positions within the area.
- Unlimited continuous clicks
- Light/Dark theme switching
- Automatically switch between English and Chinese according to the system language settings
- Modify your own hotkeys
- Bongo cats react differently depending on the click type (cat's paw on the table)
- Catcall alert when clicking done
# How to Use
- Hotkey self-definition: Click on the hotkey preview text box in the settings panel, press the keyboard to record new hotkeys, and then click the Set button to save the recording.
- Random click interval: Turn on the **Random Delay Enable** switch to type the delay time in the following text box, for example, if the click interval is set to **200** ms and the delay time is set to **100** ms, the final click interval will be randomly valued from **200-300** ms. In addition, the double-click interval will be randomly selected from **50-300** milliseconds after enabling the random delay.
- Click position: Either manually enter the screen coordinates in the text box or click the **Select** button to go to the position selector and press the left mouse button to select. After turning on the **Random Area Enable** switch, you can hold the left mouse button in the position selector to frame the range.
# Development
If you want to modify the program’s code, please open **FodyWeavers.xml** and comment out **Costura**, otherwise VS won't be able to display the window preview correctly. Because **Fody.Costura** is used in the project to package the program into a single exe, DLL and other files will be embedded in the exe when packaging, which will cause VS to report an error due to inability to find the required file.
For example, if the path to a required DLL is `. /bin/Release/xxx.dll`, Fody.Costura will embed this file in BongoPawClicker.exe, the `xxx.dll` will not exist in the `bin/Release` directory, and VS will still look for the DLL according to the previous path, which leads to an error.
```xml
<?xml version="1.0" encoding="utf-8"?>
<Weavers xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="FodyWeavers.xsd">
<!--<Costura />-->
</Weavers>
```
# Murmur
My motivation for developing this clicker is that the plot of a certain open-world game that starts with G is getting more and more naive and the scriptwriters are bringing in their crap, and I couldn't stand it. Yet there is no option to skip the plot, so I decided to create a mouse clicker to help me automatically click to play the episode quickly without the need for me to watch it myself, and I can also learn WPF along the way. As for the bongo cat, it's just for fun! 🐱
# Package
### NuGet
- [Material Design In XAML](http://materialdesigninxaml.net/)
- [Costura](https://github.com/Fody/Costura)
- [Resource.Embedder](https://www.nuget.org/packages/Resource.Embedder/)
### Special thanks
- [ChatGPT](https://chat.openai.com/) |
import z from "zod"
export const registerFormSchema = z.object({
first_name: z.string().min(3, { message: "O nome deve ter pelo menos 3 caracteres." }),
last_name: z.string().min(3, { message: "O sobrenome deve ter pelo menos 3 caracteres." }),
email: z.string().email({ message: "Por favor, insira um email válido." }),
password: z.string().min(6, { message: "A senha deve ter pelo menos 6 caracteres." }),
password_confirmation: z.string(),
}).refine(data => data.password === data.password_confirmation, {
message: "As senhas não coincidem.",
path: ["password_confirmation"]
}); |
package cos
import (
"context"
"cloud.google.com/go/storage"
"github.com/lebauce/nikos/tarball"
"github.com/lebauce/nikos/types"
"github.com/pkg/errors"
"google.golang.org/api/option"
)
type Backend struct {
buildID string
client *storage.Client
}
func (b *Backend) GetKernelHeaders(directory string) error {
filename := "kernel-headers.tgz"
bucketHandle := b.client.Bucket("cos-tools")
objectHandle := bucketHandle.Object(b.buildID + "/" + filename)
reader, err := objectHandle.NewReader(context.Background())
if err != nil {
return errors.Wrap(err, "failed to download bucket object")
}
defer reader.Close()
tarball.ExtractTarball(reader, filename, directory)
return err
}
func NewBackend(target *types.Target) (*Backend, error) {
buildID := target.OSRelease["BUILD_ID"]
if buildID == "" {
return nil, errors.New("failed to detect COS version, missing BUILD_ID in /etc/os-release")
}
client, err := storage.NewClient(context.Background(), option.WithoutAuthentication())
if err != nil {
return nil, errors.Wrap(err, "failed to creating COS backend")
}
return &Backend{
client: client,
buildID: buildID,
}, nil
} |
# create Seurat object of melanoma patient TIL4 and PBMC7
library(dplyr)
library(ggplot2)
library(Seurat)
seurat.data <- Read10X(data.dir = paste0("./data/TCR/Pool80_4-9/"))
rownames(x = seurat.data[["Antibody Capture"]]) <- gsub(pattern = "*_CITEseq", replacement = "", rownames(seurat.data[["Antibody Capture"]]))
TIL4 <- CreateSeuratObject(counts = seurat.data$`Gene Expression`, project = "TIL4", min.cells = 3, min.features = 200)
TIL4[["ADT"]] <- CreateAssayObject(seurat.data$`Antibody Capture`[, colnames(x = TIL4)])
TIL4 <- NormalizeData(TIL4, assay = "ADT", normalization.method = "CLR")
TIL4[["percent.mt"]] <- PercentageFeatureSet(TIL4, pattern = "^MT-")
TIL4 <- subset(TIL4, subset = nFeature_RNA > 200 & nFeature_RNA < 2500 & percent.mt < 20)
TIL4 <- NormalizeData(TIL4)
TIL4 <- FindVariableFeatures(TIL4)
TIL4 <- ScaleData(TIL4)
TIL4 <- RunPCA(TIL4)
TIL4 <- RunUMAP(TIL4, dims = 1:30)
TIL4 <- RenameCells(TIL4, new.names = gsub(colnames(TIL4), pattern = "-1", replacement = ""))
saveRDS("./data/TCR/objects/20230104_TIL4.rds", object = TIL4)
seurat.data <- Read10X(data.dir = paste0("./data/TCR/Pool80_4-12/"))
rownames(x = seurat.data[["Antibody Capture"]]) <- gsub(pattern = "*_CITEseq", replacement = "", rownames(seurat.data[["Antibody Capture"]]))
PBMC7 <- CreateSeuratObject(counts = seurat.data$`Gene Expression`, project = "PBMC7", min.cells = 3, min.features = 200)
PBMC7[["ADT"]] <- CreateAssayObject(seurat.data$`Antibody Capture`[, colnames(x = PBMC7)])
PBMC7 <- NormalizeData(PBMC7, assay = "ADT", normalization.method = "CLR")
PBMC7[["percent.mt"]] <- PercentageFeatureSet(PBMC7, pattern = "^MT-")
PBMC7 <- subset(PBMC7, subset = nFeature_RNA > 200 & nFeature_RNA < 2500 & percent.mt < 20)
PBMC7 <- NormalizeData(PBMC7)
PBMC7 <- FindVariableFeatures(PBMC7)
PBMC7 <- ScaleData(PBMC7)
PBMC7 <- RunPCA(PBMC7)
PBMC7 <- RunUMAP(PBMC7, dims = 1:30)
PBMC7 <- RenameCells(PBMC7, new.names = gsub(colnames(PBMC7), pattern = "-1", replacement = ""))
saveRDS("./data/TCR/objects/20230104_PBMC7.rds", object = PBMC7) |
#include "Log.h"
#include "game/ClientGame.h"
#include "game/ClientInventory.h"
#include "entity/ClientPlayer.h"
#include "item/Item.h"
#include "packet/SetItemFiltersPacket.h"
#include "tileentity/Pipe.h"
#include "types/DirectedPlace.h"
#include "ui/gtk/UITypes.h"
#include "ui/module/ItemFilterModule.h"
namespace Game3 {
ItemFilterModule::ItemFilterModule(std::shared_ptr<ClientGame> game_, const std::any &argument):
game(std::move(game_)),
place(std::any_cast<DirectedPlace>(argument)) {
auto target = Gtk::DropTarget::create(Glib::Value<DragSource>::value_type(), Gdk::DragAction::MOVE);
target->signal_drop().connect([this](const Glib::ValueBase &base, double, double) {
if (!filter || base.gobj()->g_type != Glib::Value<DragSource>::value_type())
return false;
const DragSource source = static_cast<const Glib::Value<DragSource> &>(base).get();
auto source_lock = source.inventory->sharedLock();
ItemStack *stack = (*source.inventory)[source.slot];
if (stack) {
setFilter();
filter->addItem(*stack);
populate();
upload();
}
return true;
}, false);
fixed.add_controller(target);
fixed.set_size_request(68, 68);
fixed.set_halign(Gtk::Align::CENTER);
fixed.add_css_class("item-slot");
setFilter();
auto mode_click = Gtk::GestureClick::create();
mode_click->signal_released().connect([this](int, double, double) {
modeSwitch.set_active(!modeSwitch.get_active());
});
if (filter)
modeSwitch.set_active(filter->isAllowMode());
modeSwitch.signal_state_set().connect([this](bool value) {
setMode(value);
return false;
}, false);
modeSwitch.set_margin_start(10);
modeLabel.set_margin_start(10);
modeLabel.add_controller(mode_click);
modeHbox.set_margin_top(10);
modeHbox.append(modeSwitch);
modeHbox.append(modeLabel);
auto strict_click = Gtk::GestureClick::create();
strict_click->signal_released().connect([this](int, double, double) {
strictSwitch.set_active(!strictSwitch.get_active());
});
if (filter)
strictSwitch.set_active(filter->isStrict());
strictSwitch.signal_state_set().connect([this](bool value) {
setStrict(value);
return false;
}, false);
strictSwitch.set_margin_start(10);
strictLabel.set_margin_start(10);
strictLabel.add_controller(strict_click);
strictHbox.set_margin_top(10);
strictHbox.append(strictSwitch);
strictHbox.append(strictLabel);
modeHbox.set_hexpand(true);
strictHbox.set_hexpand(true);
switchesHbox.append(modeHbox);
switchesHbox.append(strictHbox);
populate();
}
Gtk::Widget & ItemFilterModule::getWidget() {
return vbox;
}
void ItemFilterModule::update() {
populate();
}
void ItemFilterModule::reset() {
populate();
}
std::optional<Buffer> ItemFilterModule::handleMessage(const std::shared_ptr<Agent> &, const std::string &, std::any &) {
return std::nullopt;
}
bool ItemFilterModule::handleShiftClick(std::shared_ptr<Inventory> source_inventory, Slot source_slot) {
if (ItemStack *stack = (*source_inventory)[source_slot]) {
setFilter();
filter->addItem(*stack);
populate();
upload();
}
return true;
}
void ItemFilterModule::setMode(bool allow) {
setFilter();
if (filter) {
filter->setAllowMode(allow);
upload();
}
}
void ItemFilterModule::setStrict(bool strict) {
setFilter();
if (filter) {
filter->setStrict(strict);
upload();
}
}
void ItemFilterModule::upload() {
if (!filter)
return;
if (!pipe) {
WARN("Pipe is missing in ItemFilterModule::upload");
return;
}
if (!game)
throw std::runtime_error("Game is missing in ItemFilterModule::upload");
game->player->send(SetItemFiltersPacket(pipe->getGID(), place.direction, *filter));
}
void ItemFilterModule::setFilter() {
if (!pipe)
pipe = std::dynamic_pointer_cast<Pipe>(place.getTileEntity());
if (!pipe)
return;
auto &filter_ref = pipe->itemFilters[place.direction];
if (!filter_ref)
filter_ref = std::make_shared<ItemFilter>();
if (filter != filter_ref)
filter = filter_ref;
}
void ItemFilterModule::populate() {
removeChildren(vbox);
widgets.clear();
vbox.append(fixed);
vbox.append(switchesHbox);
setFilter();
std::shared_lock<DefaultMutex> configs_lock;
auto &configs = filter->getConfigs(configs_lock);
for (const auto &[id, set]: configs)
for (const auto &config: set)
addHbox(id, config);
}
void ItemFilterModule::addHbox(const Identifier &id, const ItemFilter::Config &config) {
ItemStack stack(*game, id, 1, config.data);
auto hbox = std::make_unique<Gtk::Box>(Gtk::Orientation::HORIZONTAL);
auto image = makeImage(stack);
auto label = makeLabel(stack);
auto comparator = makeComparator(id, config);
auto threshold = makeThreshold(id, config);
auto button = makeButton(std::move(stack));
hbox->set_margin_top(10);
hbox->append(*image);
hbox->append(*label);
hbox->append(*comparator);
if (threshold) {
hbox->append(*threshold);
widgets.push_back(std::move(threshold));
} else {
auto spacer = std::make_unique<Gtk::Label>();
spacer->set_hexpand(true);
hbox->append(*spacer);
widgets.push_back(std::move(spacer));
}
hbox->append(*button);
vbox.append(*hbox);
widgets.push_back(std::move(button));
widgets.push_back(std::move(comparator));
widgets.push_back(std::move(label));
widgets.push_back(std::move(image));
widgets.push_back(std::move(hbox));
}
std::unique_ptr<Gtk::Image> ItemFilterModule::makeImage(ItemStack &stack) {
auto image = std::make_unique<Gtk::Image>(stack.getImage(*game));
image->set_margin(10);
image->set_margin_top(6);
image->set_size_request(32, 32);
return image;
}
std::unique_ptr<Gtk::Label> ItemFilterModule::makeLabel(const ItemStack &stack) {
auto label = std::make_unique<Gtk::Label>(stack.getTooltip());
label->set_halign(Gtk::Align::START);
label->set_margin_end(10);
return label;
}
std::unique_ptr<Gtk::Button> ItemFilterModule::makeComparator(const Identifier &id, const ItemFilter::Config &config) {
auto button = std::make_unique<Gtk::Button>();
button->set_expand(false);
button->set_has_frame(false);
if (config.comparator == ItemFilter::Comparator::Less)
button->set_label("<");
else if (config.comparator == ItemFilter::Comparator::Greater)
button->set_label(">");
else
button->set_label("~");
button->add_css_class("comparator-button");
button->signal_clicked().connect([this, id = id, config = config] {
setFilter();
{
std::unique_lock<DefaultMutex> lock;
auto &configs = filter->getConfigs(lock);
auto &set = configs[id];
ItemFilter::Config new_config = std::move(set.extract(config).value());
if (new_config.comparator == ItemFilter::Comparator::Less) {
new_config.comparator = ItemFilter::Comparator::None;
} else if (new_config.comparator == ItemFilter::Comparator::Greater) {
new_config.comparator = ItemFilter::Comparator::Less;
} else {
new_config.comparator = ItemFilter::Comparator::Greater;
}
set.insert(std::move(new_config));
}
populate();
upload();
});
return button;
}
std::unique_ptr<Gtk::SpinButton> ItemFilterModule::makeThreshold(const Identifier &id, const ItemFilter::Config &config) {
if (config.comparator == ItemFilter::Comparator::None)
return {};
auto spin = std::make_unique<Gtk::SpinButton>();
spin->set_adjustment(Gtk::Adjustment::create(0., 0., 1e9));
spin->set_digits(0);
spin->set_value(config.count);
spin->set_hexpand(true);
spin->signal_value_changed().connect([this, id = id, config = config, spin = spin.get()]() mutable {
setFilter();
{
std::unique_lock<DefaultMutex> lock;
auto &configs = filter->getConfigs(lock);
auto &set = configs[id];
config = std::move(set.extract(config).value());
config.count = spin->get_value();
set.insert(config);
}
upload();
});
return spin;
}
std::unique_ptr<Gtk::Button> ItemFilterModule::makeButton(ItemStack stack) {
auto button = std::make_unique<Gtk::Button>();
button->set_icon_name("list-remove-symbolic");
button->set_expand(false);
button->set_has_frame(false);
button->set_margin_end(10);
button->signal_clicked().connect([this, stack = std::move(stack)] {
setFilter();
if (filter) {
filter->removeItem(stack);
populate();
upload();
}
});
return button;
}
} |
---
permalink: protect-scw/task_install_the_snapcenter_plug_in_for_windows_silently_from_the_command_line.html
sidebar: sidebar
keywords:
summary: Sie können das SnapCenter-Plug-in für Microsoft Windows lokal auf einem Windows-Host installieren, wenn Sie das Plug-in nicht Remote über die SnapCenter-Benutzeroberfläche installieren können. Sie können das SnapCenter-Plug-in für Microsoft Windows-Installationsprogramm unbeaufsichtigt, im Silent-Modus, über die Windows-Befehlszeile ausführen.
---
= Installieren Sie das SnapCenter-Plug-in für Microsoft Windows im Hintergrund über die Befehlszeile
:allow-uri-read:
:icons: font
:imagesdir: ../media/
[role="lead"]
Sie können das SnapCenter-Plug-in für Microsoft Windows lokal auf einem Windows-Host installieren, wenn Sie das Plug-in nicht Remote über die SnapCenter-Benutzeroberfläche installieren können. Sie können das SnapCenter-Plug-in für Microsoft Windows-Installationsprogramm unbeaufsichtigt, im Silent-Modus, über die Windows-Befehlszeile ausführen.
*Was Sie brauchen*
* Sie müssen Microsoft .Net 4.5.2 oder höher installiert haben.
* Sie müssen PowerShell 4.0 oder höher installiert haben.
* Sie müssen die Windows-Nachrichtenwarteschlange aktiviert haben.
* Sie müssen ein lokaler Administrator auf dem Host sein.
*Schritte*
. Laden Sie das SnapCenter-Plug-in für Microsoft Windows von Ihrem Installationsort herunter.
+
Beispielsweise lautet der Standardinstallationspfad C:\ProgramData\NetApp\SnapCenter\Package Repository.
+
Auf diesen Pfad kann von dem Host zugegriffen werden, auf dem der SnapCenter-Server installiert ist.
. Kopieren Sie die Installationsdatei auf den Host, auf dem Sie das Plug-in installieren möchten.
. Navigieren Sie in der Eingabeaufforderung zum Verzeichnis, in dem Sie die Installationsdatei heruntergeladen haben.
. Geben Sie den folgenden Befehl ein und ersetzen Sie Variablen durch Ihre Daten:
+
`"snapcenter_windows_host_plugin.exe"/silent / debuglog"" /log"" BI_SNAPCENTER_PORT= SUITE_INSTALLDIR="" BI_SERVICEACCOUNT= BI_SERVICEPWD= ISFeatureInstall=SCW`
+
Beispiel:
+
`"C:\ProgramData\NetApp\SnapCenter\Package Repository \snapcenter_windows_host_plugin.exe"/silent /debuglog"C: \HPPW_SCW_Install.log" /log"C:\" BI_SNAPCENTER_PORT=8145 SUITE_INSTALLDIR="C: \Program Files\NetApp\SnapCenter" BI_SERVICEACCOUNT=domain\administrator BI_SERVICEPWD=password ISFeatureInstall=SCW`
+
NOTE: Alle Parameter, die während der Installation von Plug-in für Windows übergeben wurden, sind Groß- und Kleinschreibung.
+
Geben Sie die Werte für die folgenden Variablen ein:
+
|===
| Variabel | Wert
a|
_/Debuglog“<Debug_Log_Path>_
a|
Geben Sie den Namen und den Speicherort der Protokolldatei für das Installationsprogramm der Suite an, wie im folgenden Beispiel: Setup.exe /debuglog"C:\PathToLog\setupexe.log".
a|
BI_SNAPCENTER_PORT
a|
Geben Sie den Port an, auf dem SnapCenter mit SMCore kommuniziert.
a|
SUITE_INSTALLDIR
a|
Geben Sie das Installationsverzeichnis für das Host-Plug-in-Paket an.
a|
BI_SERVICEACCOUNT
a|
Geben Sie das SnapCenter-Plug-in für das Web-Service-Konto von Microsoft Windows an.
a|
BI_SERVICEPWD
a|
Geben Sie das Passwort für das SnapCenter-Plug-in für das Microsoft Windows-Webservice-Konto an.
a|
ISFeatureInstall
a|
Geben Sie die Lösung an, die von SnapCenter auf dem Remote-Host implementiert werden soll.
|===
+
Der Parameter _debuglog_ enthält den Pfad der Protokolldatei für SnapCenter. Das Schreiben in diese Protokolldatei ist die bevorzugte Methode, um Informationen zur Fehlerbehebung zu erhalten, da die Datei die Ergebnisse von Prüfungen enthält, die die Installation für Plug-in-Voraussetzungen ausführt.
+
Weitere Informationen zur Fehlerbehebung finden Sie bei Bedarf in der Protokolldatei für das Paket SnapCenter für Windows. Die Protokolldateien für das Paket werden (älteste zuerst) im Ordner _%Temp%_ aufgeführt, z. B. _C:\temp\_.
+
NOTE: Die Installation des Plug-ins für Windows registriert das Plug-in auf dem Host und nicht auf dem SnapCenter-Server. Sie können das Plug-in auf dem SnapCenter Server registrieren, indem Sie den Host mithilfe der SnapCenter GUI oder PowerShell Cmdlet hinzufügen. Nach dem Hinzufügen des Hosts wird das Plug-in automatisch erkannt. |
// component elements.
import {
ButtonCategoryContainer,
OptionButton,
} from './FormSelector.styles';
// component types.
import { FormSelectorT } from './FormSelector.types';
// ** generateOptionButtons | helper function ** //
//
const generateOptionButtons = ({ options, handleClick }: FormSelectorT, category: string) => {
return options.filter((option) => option.buttonCategory === category).map(({ buttonName, isActive }) => {
return (
<OptionButton key={buttonName} onClick={() => handleClick(buttonName)} $isActive={isActive}>{buttonName}</OptionButton>
);
});
};
// ** useGenerateFormButtons | custom hook ** //
// a custom hook to generate the button list that enables the choice of various queries.
const useGenerateFormButtons = ({ options, handleClick }: FormSelectorT) => {
const operations = [
generateOptionButtons({ options, handleClick }, 'basic'),
generateOptionButtons({ options, handleClick }, 'student'),
generateOptionButtons({ options, handleClick }, 'mentor'),
];
const operationButtons = operations.map((operation, index) => {
return (
<ButtonCategoryContainer key={index}>{operation}</ButtonCategoryContainer>
);
});
return {
operationButtons,
};
};
export {
useGenerateFormButtons,
}; |
// loccheck.c -- checking to find out where variables are stored.
#include <stdio.h>
void mikado(int); //function declaration
int main(void){
int pooh = 2, bah = 5; // local variablere for main
printf("Inside main() pooh = %d and &pooh = %p\n", pooh, &pooh);
printf("Inside main() bah = %d and &bah = %p\n", bah, &bah);
mikado(pooh);
printf("\n\n\n");
return 0;
}
void mikado(int bah){ //function definition
int pooh = 10; //local variable for mikado function
printf("Inside mikado() pooh = %d and &pooh = %p\n", pooh, &pooh);
printf("Inside mikado() bah = %d and &bah = %p\n", bah, &bah);
}
// Question 1.
// My output (pointers length) consists of 12 signs after 0x hexaoctal prefix
// As the author says, each of them corresponds to four bits.
// It means these 12-signs adreses have 40 (typo in book?) bits size |
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent, HttpResponse, HttpErrorResponse } from '@angular/common/http';
import { Observable, throwError } from 'rxjs';
import { map, catchError } from 'rxjs/operators';
import { AuthenticationService } from '../services/authentication.service';
import { Router } from '@angular/router';
import { environment } from 'src/environments/environments';
@Injectable()
export class HttpConfigInterceptor implements HttpInterceptor {
constructor(
private authenticationService: AuthenticationService,
private router: Router
) { }
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
const apiBase = environment.apiBase;
if (this.authenticationService.auth && this.authenticationService.auth.token) {
if (request.url.includes(apiBase)) {
request = request.clone({
setHeaders: {
Authorization: `${this.authenticationService.auth.token}`
}
});
}
}
return next.handle(request).pipe(
catchError((error: HttpErrorResponse) => {
console.log(error);
let message = '';
if (error.error && error.error.message) {
message = error.error.message;
}
if (error.status === 401 && message === 'Token has expired') {
this.authenticationService.logout();
this.router.navigate(['/'], { queryParams: { login: 'show' } });
}
return throwError(error);
}),
map((event: HttpEvent<any>) => {
if (event instanceof HttpResponse) {
if (event.body.status_code && event.body.status_code === 401) {
this.authenticationService.logout();
}
}
return event;
})
);
}
} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.