text
stringlengths 184
4.48M
|
---|
<?php
/**
* Copyright © Magento, Inc. All rights reserved.
* See COPYING.txt for license details.
*/
declare(strict_types=1);
namespace Magento\Setup\Test\Unit\Controller;
use Laminas\View\Model\JsonModel;
use Laminas\View\Model\ViewModel;
use Magento\Setup\Controller\Navigation;
use Magento\Setup\Model\Cron\Status;
use Magento\Setup\Model\Navigation as NavModel;
use Magento\Setup\Model\ObjectManagerProvider;
use PHPUnit\Framework\MockObject\MockObject;
use PHPUnit\Framework\TestCase;
class NavigationTest extends TestCase
{
/**
* @var MockObject|\Magento\Setup\Model\Navigation
*/
private $navigationModel;
/**
* @var \Magento\Setup\Controller\Navigation
*/
private $controller;
/**
* @var Status|MockObject
*/
private $status;
/**
* @var ObjectManagerProvider|MockObject
*/
private $objectManagerProvider;
protected function setUp(): void
{
$this->navigationModel = $this->createMock(\Magento\Setup\Model\Navigation::class);
$this->status = $this->createMock(Status::class);
$this->objectManagerProvider =
$this->createMock(ObjectManagerProvider::class);
$this->controller = new Navigation($this->navigationModel, $this->status, $this->objectManagerProvider);
}
public function testIndexAction()
{
$this->navigationModel->expects($this->once())->method('getData')->willReturn('some data');
$viewModel = $this->controller->indexAction();
$this->assertInstanceOf(JsonModel::class, $viewModel);
$this->assertArrayHasKey('nav', $viewModel->getVariables());
}
public function testMenuActionUpdater()
{
$viewModel = $this->controller->menuAction();
$this->assertInstanceOf(ViewModel::class, $viewModel);
$variables = $viewModel->getVariables();
$this->assertArrayHasKey('menu', $variables);
$this->assertArrayHasKey('main', $variables);
$this->assertTrue($viewModel->terminate());
$this->assertSame('/magento/setup/navigation/menu.phtml', $viewModel->getTemplate());
}
public function testMenuActionInstaller()
{
$viewModel = $this->controller->menuAction();
$this->assertInstanceOf(ViewModel::class, $viewModel);
$variables = $viewModel->getVariables();
$this->assertArrayHasKey('menu', $variables);
$this->assertArrayHasKey('main', $variables);
$this->assertTrue($viewModel->terminate());
$this->assertSame('/magento/setup/navigation/menu.phtml', $viewModel->getTemplate());
}
public function testHeaderBarInstaller()
{
$this->navigationModel->expects($this->once())->method('getType')->willReturn(NavModel::NAV_INSTALLER);
$viewModel = $this->controller->headerBarAction();
$this->assertInstanceOf(ViewModel::class, $viewModel);
$variables = $viewModel->getVariables();
$this->assertArrayHasKey('menu', $variables);
$this->assertArrayHasKey('main', $variables);
$this->assertTrue($viewModel->terminate());
$this->assertSame('/magento/setup/navigation/header-bar.phtml', $viewModel->getTemplate());
}
public function testHeaderBarUpdater()
{
$this->navigationModel->expects($this->once())->method('getType')->willReturn(NavModel::NAV_UPDATER);
$viewModel = $this->controller->headerBarAction();
$this->assertInstanceOf(ViewModel::class, $viewModel);
$variables = $viewModel->getVariables();
$this->assertArrayHasKey('menu', $variables);
$this->assertArrayHasKey('main', $variables);
$this->assertTrue($viewModel->terminate());
$this->assertSame('/magento/setup/navigation/header-bar.phtml', $viewModel->getTemplate());
}
} |
import 'dart:math';
import 'package:flutter/material.dart';
class AnimatedContainerPage extends StatefulWidget {
@override
_AnimatedContainerPageState createState() => _AnimatedContainerPageState();
}
class _AnimatedContainerPageState extends State<AnimatedContainerPage> {
double _width = 50.0;
double _height = 50.0;
Color _color = Colors.pink;
BorderRadiusGeometry _borderRadius = BorderRadius.circular(8.0);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Animated Container'),
),
body: Center(
child: AnimatedContainer(
width: _width,
height: _height,
decoration: BoxDecoration(borderRadius: _borderRadius, color: _color),
duration: Duration(milliseconds: 500),
curve: Curves.easeInOutCubic,
),
),
floatingActionButton: _crearBotones(),
);
}
_crearBotones() {
return Row(
mainAxisAlignment: MainAxisAlignment.end,
children: <Widget>[
FloatingActionButton(
heroTag: null,
onPressed: () => _reset(),
child: Icon(Icons.restore),
),
SizedBox(
width: 5.0,
),
FloatingActionButton(
heroTag: null,
onPressed: () => _randomContainer(),
child: Icon(Icons.play_arrow),
),
],
);
}
void _randomContainer() {
setState(() {
final random = Random();
_width = random.nextInt(300).toDouble();
_height = random.nextInt(300).toDouble();
_color = Color.fromRGBO(
random.nextInt(255), random.nextInt(255), random.nextInt(255), 1);
_borderRadius = BorderRadius.circular(random.nextInt(100).toDouble());
});
}
void _reset() {
setState(() {
_width = 50;
_height = 50;
_color = Colors.pink;
_borderRadius = BorderRadius.circular(10);
});
}
} |
package com.example.shopapp.controller;
import com.example.shopapp.dtos.OrderDetailDTO;
import com.example.shopapp.exceptions.DataNotFoundException;
import com.example.shopapp.models.Order;
import com.example.shopapp.models.OrderDetail;
import com.example.shopapp.responses.OrderDetailResponse;
import com.example.shopapp.service.Inp.OrderDetailServiceInp;
import jakarta.validation.Valid;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("${api.prefix}/order_details")
public class OrderDetailController {
@Autowired
OrderDetailServiceInp orderDetailServiceInp;
@PostMapping()
public ResponseEntity<?> createOrderDetail(@Valid @RequestBody OrderDetailDTO orderDetailDTO) throws DataNotFoundException {
orderDetailServiceInp.createOrderDetail(orderDetailDTO);
return new ResponseEntity<>(orderDetailDTO, HttpStatus.OK);
}
@GetMapping("/{id}")
public ResponseEntity<?> getOrderDetail(@PathVariable int id) {
OrderDetail existingOrderDetail = orderDetailServiceInp.getOrderDetail(id);
OrderDetailResponse orderDetailResponse = OrderDetailResponse.fromOrderDetail(existingOrderDetail);
return new ResponseEntity<>(orderDetailResponse, HttpStatus.OK);
}
@GetMapping("/order/{order_id}")
public ResponseEntity<?> getAllOrderDetail(@PathVariable int order_id) {
//List<OrderDetail> orderDetailList = orderDetailServiceInp.getOrderDetails(order_id);
return new ResponseEntity<>(orderDetailServiceInp.getOrderDetails(order_id), HttpStatus.OK);
}
// fix
@PutMapping("/{id}")
public ResponseEntity<?> updateOrderDetail(@PathVariable int id,
@Valid @RequestBody OrderDetailDTO orderDetailDTO) {
orderDetailServiceInp.updateOrderDetail(id, orderDetailDTO);
return new ResponseEntity<>("Update order detail with id = " + id + " successfully", HttpStatus.OK);
}
//fix
@DeleteMapping("/{id}")
public ResponseEntity<?> deleteOrderDetail(@PathVariable int id) {
orderDetailServiceInp.deleteOrderDetail(id);
return new ResponseEntity<>("Delete order with id = " + id + " successfully", HttpStatus.OK);
}
} |
# Nano Stores Logger
<img align="right" width="92" height="92" title="Nano Stores logo"
src="https://nanostores.github.io/nanostores/logo.svg">
Logger of lifecycles, changes and actions for **[Nano Stores]**,
a tiny state manager with many atomic tree-shakable stores.
* **Clean.** All messages are stacked in compact, collapsible nested groups.
* **Descriptive.** Detailed descriptions with a clear comparison of the old and new values.
* **Pretty designed.** Compact logo and color badges for quick reading.
* **Flexible.** Ability to disable and filter certain types of messages.
* **Supports all types of stores**: Atom, Map and Deep Map.
[Nano Stores]: https://github.com/nanostores/nanostores/
<p align="center">
<picture>
<source media="(prefers-color-scheme: dark)" srcset="./img/dark.png">
<source media="(prefers-color-scheme: light)" srcset="./img/light.png">
<img alt="Nano Stores Logger" src="./img/light.png">
</picture>
</p>
## Install
```sh
npm install @nanostores/logger
```
## Usage
```js
import { logger } from '@nanostores/logger'
import { $profile, $users } from './stores/index.js'
let destroy = logger({
'Profile': $profile,
'Users': $users
})
```
### Filter messages
#### Disable specific types of logs
Using `messages` option you can disable
**mount**, **unmount**, **change** or **action** log messages.
```js
import { logger } from '@nanostores/logger'
import { $users } from './stores/index.js'
let destroy = logger({ $users }, {
messages: {
mount: false,
unmount: false
}
})
```
#### Disable logs of actions with a specific name
Using the `ignoreActions` option, you can specify the names of actions
that will not be logged.
```js
import { logger } from '@nanostores/logger'
import { $users } from './stores/index.js'
let destroy = logger({ $users }, {
ignoreActions: [
'Change Username',
'Fetch User Profile'
]
})
```
### Custom messages
You can create custom log messages and collapsible nested groups of messages
with your own name and badge color or with any predefined types.
Available types: `action`, `arguments`, `build`, `change`, `error`, `mount`,
`new`, `old`, `unmount`, `value`.
```js
import { group, groupEnd, log } from '@nanostores/logger'
log({
logo: true,
type: {
color: '#510080',
name: 'Fetch'
},
message: [
['bold', 'Profile'],
['regular', 'store is trying to get new values']
]
})
```
## Advanced usage
### Logging map creators
With `creatorLogger` you can log map creators such as
[Logux’s SyncMapTemplate](https://logux.io/web-api/#globals-syncmaptemplate).
```js
import { creatorLogger } from '@nanostores/logger'
let destroy = creatorLogger({ $users }, {
nameGetter: (creatorName, store) => {
return `${creatorName}:${store.value.id}`
}
})
```
### Building devtools
If you need to create you own devtools or an extension for you devtools
we have `buildLogger` and `buildCreatorLogger` methods
with complex logging logic inside.
```js
import { buildLogger } from '@nanostores/logger'
import { $profile } from './stores/index.js'
let destroy = buildLogger($profile, 'Profile', {
mount: ({ storeName }) => {
console.log(`${storeName} was mounted`)
},
unmount: ({ storeName }) => {
console.log(`${storeName} was unmounted`)
},
change: ({ actionName, changed, newValue, oldValue, valueMessage }) => {
let message = `${storeName} was changed`
if (changed) message += `in the ${changed} key`
if (oldValue) message += `from ${oldValue}`
message += `to ${newValue}`
if (actionName) message += `by action ${actionName}`
console.log(message, valueMessage)
},
action: {
start: ({ actionName, args }) => {
let message = `${actionName} was started`
if (args.length) message += 'with arguments'
console.log(message, args)
},
error: ({ actionName, error }) => {
console.log(`${actionName} was failed`, error)
},
end: ({ actionName }) => {
console.log(`${actionName} was ended`)
}
}
})
``` |
from abc import ABC, abstractmethod
from typing import Dict, Optional
from redis import Redis
class DataStore(ABC):
@abstractmethod
def add_redirect(self, src: str, target: str) -> None:
raise NotImplementedError
@abstractmethod
def get_target(self, src: str) -> Optional[str]:
raise NotImplementedError
@abstractmethod
def has_redirect(self, src: str) -> bool:
raise NotImplementedError
@abstractmethod
def delete_redirect(self, src: str) -> bool:
raise NotImplementedError
class MemoryDataStore(DataStore):
def __init__(self):
self.redirects: Dict[str, str] = {}
def add_redirect(self, src: str, target: str) -> None:
self.redirects[src] = target
def get_target(self, src: str) -> Optional[str]:
return self.redirects.get(src)
def has_redirect(self, src: str) -> bool:
return src in self.redirects
def delete_redirect(self, src: str) -> bool:
target = self.redirects.pop(src, None)
return target is not None
class RedisDataStore(DataStore):
def __init__(self, **kwargs):
self.r = Redis(**kwargs)
def add_redirect(self, src: str, target: str) -> None:
self.r.set(src, target)
def get_target(self, src: str) -> Optional[str]:
return self.r.get(src)
def has_redirect(self, src: str) -> bool:
return self.r.exists(src)
def delete_redirect(self, src: str) -> bool:
return self.r.delete(src) |
import {Component, OnInit} from '@angular/core';
import {UserModel} from '../../models/UserModel';
import {UserService} from '../../services/user.service';
import {FormBuilder, FormGroup, Validators} from '@angular/forms';
import {AlertHelper} from '../helpers/alert.helper';
import {AuthService} from '../../services/auth.service';
import {CityModel} from '../../models/CityModel';
import {AppData} from '../../app.data';
import {LoginType} from '../helpers/enums';
import {LocationService} from '../../services/location.service';
@Component({
selector: 'app-subscribe',
templateUrl: './subscribe.page.html',
styleUrls: ['./subscribe.page.scss'],
})
export class SubscribePage implements OnInit {
public userModel: UserModel = new UserModel();
public newUser: FormGroup;
public cities = Array<CityModel>();
public districts: any[];
// tslint:disable-next-line:max-line-length
constructor(public userService: UserService, public formBuilder: FormBuilder, public alertHelper: AlertHelper, public authService: AuthService, public locationService: LocationService) {
this.newUser = this.formBuilder.group({
name: ['', Validators.compose([Validators.required])],
email: ['', Validators.compose([Validators.required, Validators.email])],
password: ['', Validators.compose([Validators.required, Validators.minLength(6)])],
city: ['', Validators.compose([Validators.required])],
district: ['', Validators.compose([Validators.required])]
});
this.cities = AppData.cities;
}
ngOnInit() {
}
async subscribe() {
try {
console.log(this.userModel);
// Check if email exist
const emailExist: boolean = await this.userService.isAuthUserExist(this.userModel);
// Alert this is email is in used
if (emailExist) {
await this.alertHelper.toastMessage('Girdiğiniz eposta kullanımdadır. Lütfen başka bir eposta deneyin.');
} else {
// Insert in auth users
await this.authService.createUser(this.userModel);
// Insert in auth database
this.userModel.loginType = LoginType.Native;
const location = await this.locationService.getCurrentPosition();
console.log(JSON.parse(JSON.stringify(location)));
/*this.userModel.city = location.city;
this.userModel.district = location.district;*/
this.userModel.latitude = location.latitude;
this.userModel.longitude = location.longitude;
await this.userService.insert(this.userModel);
// Alert the final
await this.alertHelper.toastMessage('Üyelik işlemleri başarıyla gerçekleşti.');
}
} catch (e) {
console.log(e);
}
}
async setDistricts(name: any) {
console.log(name);
for (const city of this.cities) {
if (city.name === name) {
this.districts = city.districts;
break;
}
}
}
} |
%%
%% This file is part of the `xindy'-project at the
%% Technical University Darmstadt, Computer Science Department
%% WG System Programming, Germany.
%%
%% This source is entirely written in the `noweb' literate programming
%% system.
%%
%% History at end
%%
%%
\RCS $Id$%
\RCS $Author$%
\RCS $Revision$%
\RCS $RCSfile$%
\RCS $State$%
\RCS $Date$%
%
\chapter{The \textsf{xindy}-project}
\ModuleTitle{}
\section{Introduction}
How to introduce a piece of software that is intended to process
indexes?
%\subsection{History of index processing systems}
%\subsubsection{The original {\normalfont\makeidx}-system}
%\subsubsection{How to index non-english documents?}
%\subsubsection{Who wants to index the Bible?}
%\subsection{The xindy-approach}
\section{Project realization}
In the next few sections we will give a short introduction to the
project and its realization. We will concentrate on the structure of
the project and how the system is arranged in a hierarchical
structure. We do not describe details of the implementation or give
any theoretical background here. Each module itself describes this
background, how it works and what kind of data it manipulates.
We continue with a description of the document structure and the
modularization of the \xindy-system. Thereafter follows a description
of topics that concern \Lisp as the implementation language and a
short description of the Literate Programming System (LPS) \noweb and
how to read a \noweb-source.
\subsection{Document structure and Modularization}
In this section we concentrate on the structure of the source parts of
the project. Information about other parts of the project may be found
elsewhere.
The source itself is separated into several \term{modules}. A module
is an entity of the source that contains all data descriptions and
functions that operate on those data and is intended to serve as a
separate unit with a fixed and well-documented interface and its
description. Currently the project is divided into the following
modules (higher-level modules first).
%%
\begin{deflist}{locclass}
\item[\module{xindy}] This module is the top module of the project.
\item[\module{index}] Performs building the processing of an index.
\item[\module{idxstyle}] Manages the indexstyle.
\item[\module{locref}] Deals with the low-level primitives of the
system such as basetypes, location-references, category-attributes
and location-classes.
\item[\module{base}] This module defines basic services.
\end{deflist}
%%
Each module depends only on modules that have a lower level in the
hierarchy.
\CL provides a mechanism to define units with a separate name space
called \term{packages}. For each of the above defined modules we
create a package with its own name space and explicitly \term{export}
objects from the package to make them accessible in other modules.
Each module is stored in a separate subdirectory of the top-level
directory. Every module may consist of several submodules which are
stored in separate files. One of those submodules is the \term{main}
submodule in which a description of the interface of the module can be
found. The convention is, that the name of the main submodule equals
the name of the module.
\subsection{The \noweb-system and automatic file generation}
Each part is written in the \noweb-LPS. The programming paradigm of
\term{Literate Programming} was first introduced by D.E.Knuth\cite{}.
Its idea is to combine program source and its documentiation in a
single file which helps to keep program and documentation consistent
and to generate a pretty-printed document of the source. We try to use
this paradigm to give all the interested people an idea of how this
system was programmed and to help ourselves in decreasing debugging
time and not ruining our nerves.
Our so far gained experience in programming with a LPS showed us that
we concentrated more on the description of our problem (\eg writing an
new function) and that the additional amount of time spend in thinking
about the problem and writing down the ideas helped us to write more
or less error-free code sections. This decreases debugging time and
overall time spend into implementation.
Every \noweb-file is written in \LaTeX{} with intermixed portions of so
called \term{code-chunks}. A code-chunk is introduced by a identifier
and a piece of code written plainly into the document. A code-chink
itself may contain the identifier of another code-chunk which is
expanded later during the tangling process. With the ability to
describe a program by a series of refinements all chunks form a tree
with one \term{root chunk}.
The process to generate a source-file from a given \noweb-file is
called \term{tangling}. A tool named \cmd{notangle} processes the
input file and expands all code chunks starting from the only root
chunk. The generated source is not intended to be read by a reader.
A program called \cmd{noweave} processes a \noweb-source (with
extension and \term{weaves} a \LaTeX-file. This file may now be
\TeX{}ed and the resulting \cmd{dvi}-file is ready for reading or
printing or whatever you like to do with. In the top-level Makefile
we define a target that typesets the whole source as an article.
\subsection{Naming conventions}
We have to define conventions for the names of different kind of
objects to be consistent throughout the whole project. A short
description of the conventions used in the project follows.
\subsubsection{File naming}
File naming is mainly a definition of the filename extensions. The
following extensions may accour:
\begin{deflist}{XXXX}
\item[\cmd{.nw}] the \noweb source files.
\item[\cmd{.tex}] the weaved \LaTeX{} files.
\item[\cmd{.dvi}] the \TeX{}ed documents.
\item[\cmd{.lsp}] the tangled \CL files.
\item[\cmd{.fas}] the compiled \CL sources.
\item[\cmd{.lib}] the compiled \CL libraries.
\item[\cmd{.exp}] \texttt{expect}-scripts.
\end{deflist}
We did not exceed filename length beyond 8 + 3~characters to be able
to create an easy port to MS-DOS filesystems.
\subsubsection{\Lisp naming conventions}
\subsubsection*{Identifiers}
\subsubsection*{Functions}
\subsubsection*{Slot methods}
All methods that allow access to a slot are named in a simple scheme.
[[:reader]]- and [[:accessor]]-methods are always named
[[get-]]\emph{slotname}. [[:writer]]-methods are always named
[[set-]]\emph{slotname}.
\subsubsection*{Type specifiers in the documentation}
We use the following abbreviated type-names in the \noweb-source:
%%
\begin{deflist}{XX\tstrlist}
\item [\tint] is the type of integer numbers.
\item [\tchar] is the type of ISO-Latin characters.
\item [\tstring] is the type of strings composed of ISO-Latin
characters.
\item [\tlist] is the type of a list.
\item [\tintlist] is the type of a list of integer numbers.
\item [\tcharlist] is the type of a list of characters.
\item [\tstrlist] is the type of lists containing strings.
\item [\tclass] is the type of classes.
\item [\tgenfunc] is the type of generic functions.
\item [\terror] is the type of an error.
\end{deflist}
%%
Generally, if a type name has the form \textit{type}\type{-list} we
talk about a type of a list that only contains elements of type
\textit{type}.
\subsubsection{\noweb}
\subsubsection*{\noweb chunk naming}
As mentioned earlier \noweb uses \term{chunks} to name a piece of
code. Chunks may be enlarged by additionally defining a chunk that
already appeared earlier in the source. The real source is generated
by expanding the so-called \term{root chunk}. This expansion to the
real source makes it easy so define a portion of code everywhere in
the document. We use the following naming scheme for chunks of code:
\begin{deflistit}{export interface}
\item[$*$] Root chunk of a module.
\item[Export-list of (sub-)module \emph{name}]\mbox{}\\This chunk is used to
define the (sub-)modules interface. interface.
\item[Submodule xxx] A submodules root-chunk.
\item[Class \emph{name}] Definition of a class.
\item[Pretty-printing] Everything that belongs to pretty-printing. It
allows us to disable PP in a distributable environment.
\item[RCS-Identifier] Each submodule defines this identifier which is
accessible later in the system code.
\end{deflistit}
\subsection{Regression tests with DejaGNU}
One of the major goals is to describe regression tests as part of the
source development. One can easily set up tests during development and
verify changes made to the program later by running the testsuite.
After looking around I decided to use DejaGNU because it is nearly
optimal in combination with \clisp because DejaGNU is able to connect
stdin and stdout with clisp and run the tests interactively. This
enables one to write tests that inspect internal details easily.
The regression tests are based on the \cmd{expect}-language which is a
TCL-extension. It is based on a \cmd{send}/\cmd{expect}-mechanism
which allows sending of strings to a spawned subprocess and defining
expect patterns. In our case \clisp is invoked as the subprocess and
we send \Lisp-expressions for evaluation and describe pattern that
\clisp should answer. This is a very flexible and powerful way to
achieve full control over the whole application.
In the subdirectory \file{testsuite} we find for every module a
subdirectory containing \cmd{expect}-scripts that are invoked by the
\cmd{runtool}-command. It is part of DejaGNU and invokes \cmd{expect}
on the scripts it finds in the appropriate subdirectory.
The basic configuration of \cmd{runtool} is stored in the files
\file{site.exp} and \file{config/default.exp}. After running the
regression tests the files \file{modulname.sum} and
\file{modulename.log} contain the summaries and detailed logs of the
test.
%% Local Variables:
%% mode: latex
%% TeX-master: t
%% End:
%%
%% $Log$
%% Revision 1.4 1996/01/09 14:30:32 kehr
%% Minor modifications.
%%
%% Revision 1.3 1995/12/05 18:53:16 kehr
%% Forgot to check-in some files...
%%
%% Revision 1.2 1995/11/08 16:18:46 kehr
%% Minor modifications.
%%
%% Revision 1.1 1995/11/06 16:46:09 kehr
%% Initial checkin.
%%
%% |
<?php
namespace App\Controllers;
use App\Models\CrudModel;
use App\Models\DataModel;
class Crud extends BaseController
{
function index()
{
$crudModel = new CrudModel();
$data['tbl_data'] = $crudModel->orderBy('id', 'DESC')->paginate(10);
$data['pagination_link'] = $crudModel->pager;
return view('crud_view', $data);
}
function add()
{
return view('add_data');
}
function add_validation()
{
helper(['form', 'url']);
$error = $this->validate([
'lastname' => 'required|min_length[3]',
'firstname' => 'required',
'middlename'=> 'required',
'age'=> 'required',
'birthdate'=> 'required',
'status'=> 'required',
]);
if(!$error)
{
echo view('add_data', [
'error' => $this->validator
]);
}
else
{
$crudModel = new CrudModel();
$crudModel->save([
'lastname' => $this->request->getVar('lastname'),
'firstname' => $this->request->getVar('firstname'),
'middlename'=> $this->request->getVar('middlename'),
'age'=> $this->request->getVar('age'),
'birthdate'=> $this->request->getVar('birthdate'),
'status'=> $this->request->getVar('status'),
]);
$session = \Config\Services::session();
$session->setFlashdata('success', 'Data Added');
return $this->response->redirect(base_url("test-project/public/data"));
}
}
function generateXML()
{
$dataModel = new DataModel();
$data['td'] = $dataModel->findAll();
$xml = new \SimpleXMLElement('<data></data>');
foreach ($data['td'] as $record) {
$xmlRecord = $xml->addChild('record');
foreach ($record as $key => $value) {
$xmlRecord->addChild($key, htmlspecialchars($value));
}
}
$passedData['xml'] = $xml->asXML();
return view('xml_view', $passedData);
}
function readerXML()
{
return view('xml_read');
}
function readXML()
{
//Load SimpleXML library
helper(['form', 'url']);
$error = $this->validate([
'xml_reader' => 'required'
]);
if(!$error)
{
echo view('xml_read', [
'error' => $this->validator
]);
}
else
{
$xmlData = simplexml_load_string( $this->request->getVar('xml_reader') );
$data['table_html'] = $this->generateTable($xmlData);
return view('xml_reader', $data);
}
}
private function generateTable($xml_data) {
$table_html = '<table border="1"><thead><tr>';
// Assuming the first child contains column names
foreach ($xml_data->children()->children() as $column) {
$table_html .= '<th>' . $column->getName() . '</th>';
}
$table_html .= '</tr></thead><tbody>';
foreach ($xml_data->children() as $row) {
$table_html .= '<tr>';
foreach ($row->children() as $column) {
$table_html .= '<td>' . $column . '</td>';
}
$table_html .= '</tr>';
}
$table_html .= '</tbody></table>';
return $table_html;
}
}
?> |
package vn.ehealth.web.auth;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
@EnableWebSecurity
public class WebSecurityConfig {
@Autowired
private UserDetailsService userDetailsService;
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(bCryptPasswordEncoder());
}
@Bean
public BCryptPasswordEncoder bCryptPasswordEncoder() {
return new BCryptPasswordEncoder();
}
@Configuration
public class FormLoginWebSecurityConfigurerAdapter extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/registration", "/css/**", "/js/**", "/images/**", "/STU3/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login").permitAll()
.and()
.logout().permitAll();
}
@Bean
public AuthenticationManager customAuthenticationManager() throws Exception {
return authenticationManager();
}
}
} |
import React, { useState } from "react";
import { TextField, Button, Box } from "@mui/material";
import { styled } from "@mui/material/styles";
interface FormData {
awardName: string;
}
const InputField = styled(TextField)({
marginBottom: "1rem",
});
const InsertAward: React.FC = () => {
const [formData, setFormData] = useState<FormData>({
awardName: "",
});
const [successMessage, setSuccessMessage] = useState("");
const [errorMessage, setErrorMessage] = useState("");
const handleChange = (e: React.ChangeEvent<HTMLInputElement>) => {
setFormData({ ...formData, [e.target.name]: e.target.value });
};
const handleSubmit = async (e: React.FormEvent<HTMLFormElement>) => {
e.preventDefault();
try {
const response = await fetch(
"https://functions.yandexcloud.net/d4esum4t3768sp096apb",
{
method: "POST",
headers: { "Content-Type": "application/json" },
body: JSON.stringify({
operation: "insert",
awardName: formData.awardName,
}),
}
);
const data = await response.json();
console.log(data);
setSuccessMessage("Award added successfully");
setFormData({ awardName: "" });
} catch (error) {
console.error(error);
setErrorMessage("Error adding award");
}
};
return (
<Box sx={{ p: 2 }}>
<h2>Insert Award</h2>
{successMessage && <p>{successMessage}</p>}
{errorMessage && <p>{errorMessage}</p>}
<form onSubmit={handleSubmit}>
<InputField
fullWidth
label="Award Name"
id="awardName"
name="awardName"
value={formData.awardName}
onChange={handleChange}
/>
<Box sx={{ display: "block", mt: 2 }}>
<Button variant="contained" type="submit">
Add Award
</Button>
</Box>
</form>
</Box>
);
};
export default InsertAward; |
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'package:ngewibu/app/constants/color.dart';
import '../../../data/models/detail_complate.dart' as complate;
import '../../../routes/app_pages.dart';
import '../controllers/anime_complate_controller.dart';
class AnimeComplateView extends GetView<AnimeComplateController> {
const AnimeComplateView({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Anime Complate'),
centerTitle: true,
),
body: FutureBuilder(
future: controller.getAllOngoing(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.done) {
return Obx(
() => SingleChildScrollView(
child: Padding(
padding: const EdgeInsets.all(10),
child: Column(
children: [
GridView.builder(
shrinkWrap: true,
physics: const NeverScrollableScrollPhysics(),
scrollDirection: Axis.vertical,
itemCount: controller.allAnime.length,
gridDelegate:
const SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 2,
crossAxisSpacing: 10,
mainAxisExtent: 250,
mainAxisSpacing: 10,
childAspectRatio: 3 / 4),
itemBuilder: (context, index) {
final complate.Datum animeComplate =
controller.allAnime[index];
return InkWell(
onTap: () {
Get.toNamed(Routes.ANIME_DETAIL, arguments: {
'title': animeComplate.title,
'slug': animeComplate.slug
});
},
child: Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.white54)),
child: Stack(
children: [
SizedBox(
height: 350,
width: 300,
child: Image.network(
'${animeComplate.poster}',
fit: BoxFit.cover,
),
),
Container(
decoration: BoxDecoration(
borderRadius: const BorderRadius.only(
bottomRight: Radius.circular(10),
),
color: colorSatu.withOpacity(0.9)),
child: Padding(
padding: const EdgeInsets.all(5),
child: Text(
'${animeComplate.episodeCount} Episode',
),
),
),
Positioned(
right: 0,
top: 0,
child: Container(
decoration: BoxDecoration(
borderRadius: const BorderRadius.only(
bottomLeft: Radius.circular(10),
),
color: colorSatu.withOpacity(0.9)),
child: Padding(
padding: const EdgeInsets.all(5),
child: Text(
'${animeComplate.rating}',
style: TextStyle(
color: Colors.amber[400]),
),
),
),
),
Positioned(
bottom: 0,
child: Container(
width: Get.width,
color: colorSatu.withOpacity(0.9),
child: Padding(
padding: const EdgeInsets.all(5),
child: Text(
animeComplate.title!.length >= 20
? '${animeComplate.title!.substring(0, 20)}...'
: '${animeComplate.title}',
maxLines: 1,
style: const TextStyle(fontSize: 13),
),
),
),
)
],
),
),
);
},
),
const SizedBox(
height: 10,
),
Obx(() => Text(
controller.selesai.value ? 'Halaman Terakhir' : '')),
controller.selesai.value
? const SizedBox()
: Obx(
() => controller.isLoading.value
? const SizedBox(
height: 30,
width: 30,
child: CircularProgressIndicator(),
)
: ElevatedButton(
onPressed: () {
controller.tambahAnime();
},
child: const Text('Tambah Anime'),
),
),
const SizedBox(
height: 10,
)
],
),
),
),
);
} else {
return const Center(
child: CircularProgressIndicator(),
);
}
},
),
);
}
} |
// framework
import { useEffect, useMemo, useState } from "react";
import { v4 } from "uuid";
// util
import { shouldRightSideCollapse } from "../../util/mechanics/shouldRightSideCollapse";
import { AllPlayersProps } from "../../util/propTypes";
import { useCurrentPlayer } from "../../hooks/useCurrentPlayer";
import { defaultUIState } from "../../util/setup/defaultUIState";
import { UIState } from "../../util/types";
// components
import AvailableChips from "../Resources/AvailableChips";
import SelectionView from "../Resources/SelectionView";
import Player from "./Player";
import "./AllPlayers.scss"
export default function AllPlayers({ state, setState, liftSelection, UICollapse, setUICollapse, liftCollapsed }: AllPlayersProps) {
const [playerView, setPlayerView] = useState<JSX.Element>();
const [collapseClass, setCollapseClass] = useState("all-players");
const collapseAll = () => {
liftCollapsed(true);
liftCollapsed(true, 3);
liftCollapsed(true, 2);
liftCollapsed(true, 1);
// let value = UICollapse[each as keyof UIState];
// if (each === "playerUICollapsed") {
// continue;
// } else if (each === "noblesCollapsed") {
// console.log(each);
// liftCollapsed(value);
// } else {
// console.log(each, value);
// switch (each) {
// case "tierThreeCollapsed":
// liftCollapsed(value, 3);
// break;
// case "tierTwoCollapsed":
// liftCollapsed(value, 2);
// break;
// case "tierOneCollapsed":
// liftCollapsed(value, 1);
// break;
// default: break;
// }
// }
}
const allowCollapseAll = useMemo(() => {
for (let each of Object.keys(UICollapse)) {
if (each === "playerUICollapsed") continue;
if (!UICollapse[each as keyof UIState]) return true;
}
return false;
}, [UICollapse]);
useEffect(() => {
const currentPlayer = useCurrentPlayer(state);
if (!currentPlayer) return;
setPlayerView(<Player key={v4()} player={currentPlayer} state={state} setState={setState} />);
}, [state]);
useEffect(() => {
setCollapseClass( shouldRightSideCollapse(UICollapse) ? "all-players-mini" : "all-players" );
}, [UICollapse]);
return (
<div className={collapseClass}>
{ allowCollapseAll && <button onClick={collapseAll}>Collapse All</button> }
<SelectionView state={state} setState={setState} UICollapse={UICollapse} />
<AvailableChips state={state} setState={setState} liftSelection={liftSelection} />
{ playerView }
</div>
)
} |
import { handler } from "./app";
import * as dynamoDBUtils from "../../db/dynamoDB/utils";
import { APIGatewayProxyEvent } from "aws-lambda";
const mockEvent200: APIGatewayProxyEvent = {
pathParameters: null,
body: `{"description": "Mock description","price": 1,"title": "Mock title","count": 1}`,
headers: {},
multiValueHeaders: {},
httpMethod: "POST",
isBase64Encoded: false,
path: '/products',
queryStringParameters: null,
multiValueQueryStringParameters: null,
stageVariables: null,
requestContext: {} as any,
resource: "mock-resource",
}
const mockEvent400: APIGatewayProxyEvent = {
pathParameters: null,
body: `{"description": "Mock description 2"}`,
headers: {},
multiValueHeaders: {},
httpMethod: "POST",
isBase64Encoded: false,
path: '/products',
queryStringParameters: null,
multiValueQueryStringParameters: null,
stageVariables: null,
requestContext: {} as any,
resource: "mock-resource",
}
jest.mock("../../db/dynamoDB/utils");
describe("createProduct", () => {
let createProductSpy: jest.SpyInstance;
beforeAll(() => {
createProductSpy = jest.spyOn(dynamoDBUtils, "createProduct");
});
afterEach(() => {
createProductSpy.mockClear();
});
afterAll(() => {
createProductSpy.mockRestore();
});
test("should create a product with a valid body", async () => {
const response = await handler(mockEvent200);
expect(response.statusCode).toBe(200);
expect(createProductSpy).toHaveBeenCalled();
});
test("should return 404 error with an invalid body", async () => {
const response = await handler(mockEvent400);
expect(response.statusCode).toBe(400);
expect(createProductSpy).not.toHaveBeenCalled();
});
}); |
/* ************************************************************************** */
/* */
/* ::: :::::::: */
/* Brain.hpp :+: :+: :+: */
/* +:+ +:+ +:+ */
/* By: gychoi <[email protected]> +#+ +:+ +#+ */
/* +#+#+#+#+#+ +#+ */
/* Created: 2023/08/16 19:34:27 by gychoi #+# #+# */
/* Updated: 2023/08/17 20:41:41 by gychoi ### ########.fr */
/* */
/* ************************************************************************** */
#pragma once
#ifndef __BRAIN_HPP__
# define __BRAIN_HPP__
# include <string>
class Brain
{
private:
std::string ideas[100];
public:
Brain(void);
~Brain(void);
Brain(Brain const& target);
Brain& operator=(Brain const& target);
std::string getIdea(std::size_t idx);
void setIdea(std::size_t idx, std::string const& idea);
};
#endif /* __BRAIN_HPP__ */ |
export const blueprintOpenAP = [
{
name: "Open AP Template",
slug: "open_ap/Open_AP_Template",
access: ["*"],
fields: [
{
key: "tranId",
type: "string",
label: "Tran Id",
readonly: false,
constraints: [
{
type: "required",
},
{
type: "unique",
},
],
description: "Enter Invoice number. This field should be unique.",
},
{
key: "company_name",
type: "reference",
label: "Company Name",
config: {
key: "companyName",
ref: "open_ap/Vendor",
relationship: "has-many",
},
readonly: false,
constraints: [
{
type: "required",
},
],
description:
"This is a reference to the company name of the record that must exist in your account prior to import.",
},
{
key: "subsidiary",
type: "reference",
label: "Subsidiary",
config: {
key: "Name",
ref: "open_ap/Subsidiary_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [
{
type: "required",
},
],
description:
"This is a reference to the subsidiary of the record that match the selected subsidiary on the entity record.",
},
{
key: "currency",
type: "reference",
label: "Currency",
config: {
key: "Name",
ref: "open_ap/Currency_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [
{
type: "required",
},
],
description:
"This is a reference to a currency that must exist in your account prior to import. The currency used must match the currency selected on the customer’s record.",
},
{
key: "exchangeRate",
type: "number",
label: "Exchange Rate",
readonly: false,
constraints: [],
description:
"Enter the currency exchange rate as of cutover date for the transaction. Ask your lead consultant for details.",
},
{
key: "postingPeriod",
type: "string",
label: "Posting Period",
readonly: false,
constraints: [],
description:
"This is the cutover period. Formula driven. Do not override.",
},
{
key: "tranDate",
type: "string",
label: "Tran Date",
readonly: false,
constraints: [
{
type: "required",
},
],
description: "Enter the original invoice date.",
},
{
key: "dueDate",
type: "string",
label: "Due Date",
readonly: false,
constraints: [],
description: "Enter the due date of the invoice.",
},
{
key: "referenceno",
type: "string",
label: "Reference No",
readonly: false,
constraints: [],
description: "Enter PO/reference number.",
},
{
key: "memo",
type: "string",
label: "Memo",
readonly: false,
constraints: [],
description:
"You can retain the default memo driven by the formula or enter the actual description of the invoice. ",
},
{
key: "itemLine_Item",
type: "string",
label: "Item Line Item",
readonly: false,
constraints: [],
description:
"This is the dummy item to be used in importing the open transactions. Formula driven. Do not override.",
},
{
key: "itemLine_description",
type: "string",
label: "Item Line Description",
readonly: false,
constraints: [],
description:
"You can retain the default memo driven by the formula or enter the actual description of the invoice. ",
},
{
key: "itemLine_Quantity",
type: "number",
label: "Item Line Quantity",
readonly: false,
constraints: [
{
type: "required",
},
],
description: "Enter item Quantity",
},
{
key: "itemLine_Rate",
type: "number",
label: "Item Line Rate",
readonly: false,
constraints: [
{
type: "required",
},
],
description: "Enter the rate for the item (price per quantity).",
},
{
key: "Transaction_Amount",
type: "number",
label: "Transaction Amount",
readonly: false,
constraints: [],
description:
"This is the column is calculated by multiplying the rate to the quantity. Formula driven do not override.",
},
{
key: "Base_Currency_Amount",
type: "number",
label: "Base Currency Amount",
readonly: false,
constraints: [],
description:
"This is the column is calculated by multiplying the Transaction Amount to the Exchange Rate to calculate the amount that is going to be posted to the general ledger. Formula driven. Do not override.",
},
{
key: "itemLine_department",
type: "string",
label: "Item Line Department",
readonly: false,
constraints: [],
description:
"This should be the #N/A value of the segment. Formula driven. Do not override.",
},
{
key: "itemLine_class",
type: "string",
label: "Item Line Class",
readonly: false,
constraints: [],
description:
"This should be the #N/A value of the segment. Formula driven. Do not override.",
},
{
key: "itemLine_location",
type: "string",
label: "Item Line Location",
readonly: false,
constraints: [],
description:
"This should be the #N/A value of the segment. Formula driven. Do not override.",
},
],
readonly: false,
},
{
name: "Vendor",
slug: "open_ap/Vendor",
access: ["*"],
fields: [
{
key: "externalID",
type: "string",
label: "External ID",
readonly: false,
constraints: [
{
type: "unique",
},
],
description:
"This is the Unique backend Identifier for a Vendor Record. Should be Unique for all the Vendor Records. This can be used to create a Parent-Child Relationship and to link other record sets with these Vendors.",
},
{
key: "entityid",
type: "string",
label: "Vendor ID",
readonly: false,
constraints: [
{
type: "unique",
},
],
description:
"This is the Front-End Vendor ID. Should be unique for all the Vendors. This field is not required if you use Auto-Generated Numbers.",
},
{
key: "isPerson",
type: "boolean",
label: "Is Person",
readonly: false,
constraints: [],
description:
"Choose the type of Vendor record you are creating by selecting an Individual. If set to TRUE, the First Name and Last Name will be a mandatory field to populate.",
},
{
key: "companyName",
type: "string",
label: "Company Name",
readonly: false,
constraints: [],
description: "Enter the Vendor's company name.",
},
{
key: "firstName",
type: "string",
label: "First Name",
readonly: false,
constraints: [],
description:
"Required field if the Vendor is an Individual. Leave blank for Companies.",
},
{
key: "lastName",
type: "string",
label: "Last Name",
readonly: false,
constraints: [],
description:
"Required field if the Vendor is an Individual. Leave blank for Companies.",
},
{
key: "subsidiary",
type: "reference",
label: "Subsidiary",
config: {
key: "Name",
ref: "open_ap/Subsidiary_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [
{
type: "required",
},
],
description:
"This is a reference to the subsidiary which must be created in your account prior to import. Select from the drop down field.",
},
{
key: "email",
type: "string",
label: "Email",
readonly: false,
constraints: [],
description:
"This field should contain the main E-mail Address of the Vendor. The Information entered for this field must conform to the standard e-mail Address format. [email protected]",
},
{
key: "email_payment_notif",
type: "string",
label: "Email Payment Notif",
readonly: false,
constraints: [],
description:
"This field should contain the main E-mail Address of the Vendor that will receive the remittance advice for electronic payments. For multiple e-mail addresses, use a semi-colon (;) as a delimiter (ie., [email protected];[email protected]). The Information entered for this field must conform to the standard e-mail Address format. [email protected]",
},
{
key: "phone",
type: "string",
label: "Phone",
readonly: false,
constraints: [],
description:
"The Information entered for this field can be in one of the following formats: 999-999-9999 (999) 999-9999 1-999-999-9999 1 (999) 999-9999 999-999-9999 ext 999 +44 (0) 1234-4567-568",
},
{
key: "currency",
type: "reference",
label: "Currency",
config: {
key: "Name",
ref: "open_ap/Currency_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description:
"This is mandatory if you use Multiple Currencies. It is a reference to a currency record that must exist in Lists > Accounting > Currencies prior to importing.",
},
{
key: "terms",
type: "reference",
label: "Terms",
config: {
key: "name",
ref: "open_ap/Payment_Term_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description:
"This field should have the reference to default terms that you have with this Vendor. These records must exist in Setup > Accounting > Accounting Lists > Terms prior to importing.",
},
{
key: "address1_Label",
type: "string",
label: "Address1 Label",
readonly: false,
constraints: [],
description:
"It maps to the Label of an Address and indicates the beginning of an individual Address. The Label must be unique for all the different Addresses for this Vendor.",
},
{
key: "Address_Attention",
type: "string",
label: "Address Attention",
readonly: false,
constraints: [],
description: "Enter the name of the Individual in this field.",
},
{
key: "Address1_Addressee",
type: "string",
label: "Address1 Addressee",
readonly: false,
constraints: [],
description: "Enter the Addressee or the Company Name here.",
},
{
key: "Address1_phone",
type: "string",
label: "Address1 Phone",
readonly: false,
constraints: [],
description: "Enter a phone number for your Vendor. ",
},
{
key: "Address1_line1",
type: "string",
label: "Address1 Line1",
readonly: false,
constraints: [],
description: "Enter the Address Line 1 in this field.",
},
{
key: "address1_line2",
type: "string",
label: "Address1 Line2",
readonly: false,
constraints: [],
description: "Enter the Address Line 2 in this field.",
},
{
key: "Address1_City",
type: "string",
label: "Address1 City",
readonly: false,
constraints: [],
description: "Enter the City of the Address in this field.",
},
{
key: "address1_state",
type: "reference",
label: "Address1 State",
config: {
key: "State",
ref: "open_ap/States_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description:
"Enter the State in this field. You may enter the standard abbreviation or the full state or province name.",
},
{
key: "Address1_zipCode",
type: "string",
label: "Address1 Zip Code",
readonly: false,
constraints: [],
description: "Enter the Zip Code of the Address in this field.",
},
{
key: "Address1_Country",
type: "reference",
label: "Address1 Country",
config: {
key: "Countries",
ref: "open_ap/Countries_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description:
"This is the Reference to the Country of this Address. It must match the List of the Countries in NetSuite.",
},
{
key: "Address1_defaultBilling",
type: "boolean",
label: "Address1 Default Billing",
readonly: false,
constraints: [],
description:
"If this Address is to be marked as a Default Billing Address, please put TRUE. Otherwise, enter FALSE if this is NOT a Default Billing Address.",
},
{
key: "Address1_defaultShipping",
type: "boolean",
label: "Address1 Default Shipping",
readonly: false,
constraints: [],
description:
"If this Address is to be marked as a Default Shipping Address, please put TRUE. Otherwise, enter FALSE if this is it NOT a Default Shipping Address.",
},
{
key: "emailtransactions",
type: "boolean",
label: "Email Transactions",
readonly: false,
constraints: [],
description:
"If marked as TRUE, new transactions created for the Vendor will automatically gets sent out to the third party. Make sure to set this to FALSE for the initial import. If required to be enabled, Vendor lists needs to be updated after cutover.",
},
{
key: "category",
type: "reference",
label: "Category",
config: {
key: "name",
ref: "open_ap/Vendor_Category_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description:
"Provide the Category reference for this Vendor. It must exist in Setup > Accounting > Accounting Lists > New > Vendor Category prior to importing.",
},
{
key: "isInactive",
type: "boolean",
label: "Is Inactive",
readonly: false,
constraints: [],
description:
"This is used to mark the Vendor as Inactive at the time of Import",
},
{
key: "payablesAccount",
type: "reference",
label: "Payables Account",
config: {
key: "Account Name",
ref: "open_ap/Chart_of_Accounts_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description: "",
},
{
key: "priceLevel",
type: "reference",
label: "Price Level",
config: {
key: "name",
ref: "open_ap/Price_Level_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description:
"This is a reference to a default price level at which you sell your items to this Vendor. The Price Levels must exist in Setup > Accounting > Accounting List > Price Level prior to importing.",
},
{
key: "creditLimit",
type: "number",
label: "Credit Limit",
readonly: false,
constraints: [],
description:
"This is the Credit Limit you would want to set for the Sales transactions with this Vendor.",
},
{
key: "taxItem",
type: "reference",
label: "Tax Item",
config: {
key: "name",
ref: "open_ap/Tax_Item_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description:
"This is a reference to a default Sales tax item that applies to this Vendor. The Sales Tax item must exist in Setup > Accounting > Tax Codes prior to importing.",
},
{
key: "taxItemInternalId",
type: "string",
label: "Tax Item Internal ID",
readonly: true,
constraints: [
{
type: "computed",
},
],
description: "",
},
{
key: "vatregnumber",
type: "string",
label: "Vendor's tax registration number",
readonly: false,
constraints: [],
description: "Enter this Vendor's tax registration number.",
},
{
key: "additionalCurrencies",
type: "reference",
label: "Additional Currencies",
config: {
key: "Name",
ref: "open_ap/Currency_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description:
"Add additional currencies outside of the primary currency that the Vendor uses.",
},
{
key: "Address2_attention",
type: "string",
label: "Address2 Attention",
readonly: false,
constraints: [],
description: "Enter the name of the Individual in this field.",
},
{
key: "Address2_Addressee",
type: "string",
label: "Address2 Addressee",
readonly: false,
constraints: [],
description: "Enter the Addressee or the Company Name here.",
},
{
key: "Address2_phone",
type: "string",
label: "Address2 Phone",
readonly: false,
constraints: [],
description: "Enter a phone number for your Vendor.",
},
{
key: "Address2_line1",
type: "string",
label: "Address2 Line1",
readonly: false,
constraints: [],
description: "Enter the Address Line 1 in this field.",
},
{
key: "Address2_line2",
type: "string",
label: "Address2 Line2",
readonly: false,
constraints: [],
description: "Enter the Address Line 2 in this field.",
},
{
key: "Address2_city",
type: "string",
label: "Address2 City",
readonly: false,
constraints: [],
description: "Enter the City of the Address in this field.",
},
{
key: "Address2_state",
type: "reference",
label: "Address2 State",
config: {
key: "State",
ref: "open_ap/States_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description:
"Enter the State in this field. You may enter the standard abbreviation or the full state or province name.",
},
{
key: "Address2_zipCode",
type: "string",
label: "Address2 Zip Code",
readonly: false,
constraints: [],
description: "Enter the Zip Code of the Address in this field.",
},
{
key: "Address2_country",
type: "reference",
label: "Address2 Country",
config: {
key: "Countries",
ref: "open_ap/Countries_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description:
"This is the Reference to the Country of this Address. It must match the List of the Countries in NetSuite.",
},
{
key: "Address2_defaultBilling",
type: "boolean",
label: "Address2 Default Billing",
readonly: false,
constraints: [],
description:
"If this Address is to be marked as a Default Billing Address, please put TRUE. Otherwise, enter FALSE if this is NOT a Default Billing Address.",
},
{
key: "Address2_defaultShipping",
type: "boolean",
label: "Address2 Default Shipping",
readonly: false,
constraints: [],
description:
"If this Address is to be marked as a Default Shipping Address, please put TRUE. Otherwise, enter FALSE if this is it NOT a Default Shipping Address.",
},
],
readonly: false,
},
{
name: "Subsidiary (NetSuite Extract)",
slug: "open_ap/Subsidiary_NetSuite_Extract",
access: ["*"],
fields: [
{
key: "Internal_Id",
type: "string",
label: "Internal Id",
readonly: false,
constraints: [
{
type: "unique",
},
],
description: "",
},
{
key: "External_Id",
type: "string",
label: "External Id",
readonly: false,
constraints: [],
description: "",
},
{
key: "Name",
type: "string",
label: "Name",
readonly: false,
constraints: [
{
type: "unique",
},
],
description: "",
},
{
key: "currency",
type: "reference",
label: "Currency",
config: {
key: "Name",
ref: "open_ap/Currency_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description: "",
},
{
key: "inactive",
type: "boolean",
label: "Inactive",
readonly: false,
constraints: [
{
type: "required",
},
],
description: "",
},
{
key: "IsElimination",
type: "boolean",
label: "Is Elimination",
readonly: false,
constraints: [],
description: "",
},
],
readonly: true,
},
{
name: "Currency (NetSuite Extract)",
slug: "open_ap/Currency_NetSuite_Extract",
access: ["*"],
fields: [
{
key: "Name",
type: "string",
label: "Name",
readonly: false,
constraints: [
{
type: "required",
},
{
type: "unique",
},
],
description: "",
},
],
readonly: true,
},
{
name: "Payment Term (NetSuite Extract)",
slug: "open_ap/Payment_Term_NetSuite_Extract",
access: ["*"],
fields: [
{
key: "internalId",
type: "string",
label: "Internal Id",
readonly: false,
constraints: [
{
type: "unique",
},
],
description: "",
},
{
key: "name",
type: "string",
label: "Name",
readonly: false,
constraints: [
{
type: "unique",
},
],
description: "",
},
{
key: "daysUntilNetDue",
type: "number",
label: "Days Until Net Due",
readonly: false,
constraints: [],
description: "",
},
],
readonly: false,
},
{
name: "States (NetSuite Extract)",
slug: "open_ap/States_NetSuite_Extract",
access: ["*"],
fields: [
{
key: "State",
type: "string",
label: "State",
readonly: false,
constraints: [
{
type: "unique",
},
],
description: "",
},
{
key: "abbrev.",
type: "string",
label: "State Abbreviation",
readonly: false,
constraints: [],
description: "",
},
{
key: "Country",
type: "string",
label: "Country",
readonly: false,
constraints: [],
description: "",
},
],
readonly: true,
},
{
name: "Countries (NetSuite Extract)",
slug: "open_ap/Countries_NetSuite_Extract",
access: ["*"],
fields: [
{
key: "Countries",
type: "string",
label: "Countries",
readonly: false,
constraints: [
{
type: "required",
},
{
type: "unique",
},
],
description: "",
},
],
readonly: true,
},
{
name: "Vendor Category (NetSuite Extract)",
slug: "open_ap/Vendor_Category_NetSuite_Extract",
access: ["*"],
fields: [
{
key: "internalID",
type: "string",
label: "Internal ID",
readonly: false,
constraints: [
{
type: "unique",
},
],
description: "",
},
{
key: "name",
type: "string",
label: "Name",
readonly: false,
constraints: [
{
type: "unique",
},
],
description: "",
},
],
readonly: false,
},
{
name: "Chart of Accounts (NetSuite Extract)",
slug: "open_ap/Chart_of_Accounts_NetSuite_Extract",
access: ["*"],
fields: [
{
key: "internalID",
type: "string",
label: "Internal ID",
readonly: false,
constraints: [],
description: "",
},
{
key: "externalID",
type: "string",
label: "External ID",
readonly: false,
constraints: [
{
type: "unique",
},
],
description: "",
},
{
key: "Account Number",
type: "string",
label: "Account Number",
readonly: false,
constraints: [],
description: "",
},
{
key: "Account Name",
type: "string",
label: "Account Name",
readonly: false,
constraints: [],
description: "",
},
{
key: "parent",
type: "string",
label: "Parent",
readonly: false,
constraints: [],
description: "",
},
{
key: "Account Type ",
type: "string",
label: "Account Type",
readonly: false,
constraints: [],
description: "",
},
{
key: "currency",
type: "reference",
label: "Currency",
config: {
key: "Name",
ref: "open_ap/Currency_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description: "",
},
{
key: "subsidiary",
type: "reference",
label: "Subsidiary",
config: {
key: "Name",
ref: "open_ap/Subsidiary_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [
{
type: "required",
},
],
description:
"This is a reference to the subsidiary which must be created in your account prior to import. Select from the drop down field.",
},
{
key: "includeChildren",
type: "boolean",
label: "Include Children",
readonly: false,
constraints: [],
description: "",
},
{
key: "isInactive",
type: "boolean",
label: "Is Inactive?",
readonly: false,
constraints: [],
description: "",
},
{
key: "SummaryAccount",
type: "boolean",
label: "Summary Account",
readonly: false,
constraints: [],
description: "",
},
],
readonly: false,
},
{
name: "Price Level (NetSuite Extract)",
slug: "open_ap/Price_Level_NetSuite_Extract",
access: ["*"],
fields: [
{
key: "name",
type: "string",
label: "Name",
readonly: false,
constraints: [
{
type: "unique",
},
],
description: "",
},
],
readonly: false,
},
{
name: "Tax Item (NetSuite Extract)",
slug: "open_ap/Tax_Item_NetSuite_Extract",
access: ["*"],
fields: [
{
key: "internalID",
type: "string",
label: "Internal ID",
readonly: false,
constraints: [
{
type: "unique",
},
],
description: "",
},
{
key: "name",
type: "string",
label: "Name",
readonly: false,
constraints: [
{
type: "unique",
},
],
description: "",
},
{
key: "vatRate",
type: "string",
label: "VAT Rate",
readonly: false,
constraints: [],
description: "",
},
{
key: "country",
type: "reference",
label: "Country",
config: {
key: "Countries",
ref: "open_ap/Countries_NetSuite_Extract",
relationship: "has-many",
},
readonly: false,
constraints: [],
description: "",
},
],
readonly: false,
}
]; |
import * as React from "react";
import * as ReactDom from "react-dom";
import { Version } from "@microsoft/sp-core-library";
import {
type IPropertyPaneConfiguration,
PropertyPaneDropdown,
} from "@microsoft/sp-property-pane";
import { BaseClientSideWebPart } from "@microsoft/sp-webpart-base";
import { IReadonlyTheme } from "@microsoft/sp-component-base";
import * as strings from "IncomingEmployeesWebPartStrings";
import IncomingEmployees from "./components/IncomingEmployees";
import { IIncomingEmployeesProps } from "./components/IIncomingEmployeesProps";
import {
CustomCollectionFieldType,
PropertyFieldCollectionData,
} from "@pnp/spfx-property-controls/lib/PropertyFieldCollectionData";
import { DateConvention, DateTimePicker } from "@pnp/spfx-controls-react";
import {
PeoplePicker,
PrincipalType,
} from "@pnp/spfx-controls-react/lib/PeoplePicker";
export interface IIncomingEmployeesWebPartProps {
position: string;
items: any[];
}
export default class IncomingEmployeesWebPart extends BaseClientSideWebPart<IIncomingEmployeesWebPartProps> {
public render(): void {
const element: React.ReactElement<IIncomingEmployeesProps> =
React.createElement(IncomingEmployees, {
position: this.properties.position,
items: this.properties.items || [],
displayMode: this.displayMode,
onConfigurePropPane: this._onConfigure,
});
ReactDom.render(element, this.domElement);
}
_onConfigure = (): void => {
this.context.propertyPane.open();
};
protected onInit(): Promise<void> {
return this._getEnvironmentMessage().then((message) => {
// this._environmentMessage = message;
});
}
private _getEnvironmentMessage(): Promise<string> {
if (!!this.context.sdks.microsoftTeams) {
// running in Teams, office.com or Outlook
return this.context.sdks.microsoftTeams.teamsJs.app
.getContext()
.then((context) => {
let environmentMessage: string = "";
switch (context.app.host.name) {
case "Office": // running in Office
environmentMessage = this.context.isServedFromLocalhost
? strings.AppLocalEnvironmentOffice
: strings.AppOfficeEnvironment;
break;
case "Outlook": // running in Outlook
environmentMessage = this.context.isServedFromLocalhost
? strings.AppLocalEnvironmentOutlook
: strings.AppOutlookEnvironment;
break;
case "Teams": // running in Teams
case "TeamsModern":
environmentMessage = this.context.isServedFromLocalhost
? strings.AppLocalEnvironmentTeams
: strings.AppTeamsTabEnvironment;
break;
default:
environmentMessage = strings.UnknownEnvironment;
}
return environmentMessage;
});
}
return Promise.resolve(
this.context.isServedFromLocalhost
? strings.AppLocalEnvironmentSharePoint
: strings.AppSharePointEnvironment
);
}
protected onThemeChanged(currentTheme: IReadonlyTheme | undefined): void {
if (!currentTheme) {
return;
}
// this._isDarkTheme = !!currentTheme.isInverted;
const { semanticColors } = currentTheme;
if (semanticColors) {
this.domElement.style.setProperty(
"--bodyText",
semanticColors.bodyText || null
);
this.domElement.style.setProperty("--link", semanticColors.link || null);
this.domElement.style.setProperty(
"--linkHovered",
semanticColors.linkHovered || null
);
}
}
protected onDispose(): void {
ReactDom.unmountComponentAtNode(this.domElement);
}
protected get dataVersion(): Version {
return Version.parse("1.0");
}
protected getPropertyPaneConfiguration(): IPropertyPaneConfiguration {
return {
pages: [
{
header: {
description: "Incoming Employees Web Part Settings",
},
groups: [
//Incoming employees
{
groupName: "Manage Incoming employees",
groupFields: [
PropertyFieldCollectionData("items", {
key: "items",
label: "",
panelHeader: "Manage items",
manageBtnLabel: "Manage items",
value: this.properties.items,
fields: [
{
id: "user",
title: "User",
type: CustomCollectionFieldType.custom,
required: true,
onCustomRender: (
field,
value,
onUpdate,
item,
itemId,
onError
) => {
return React.createElement(PeoplePicker, {
context: this.context as any,
personSelectionLimit: 1,
showtooltip: true,
key: itemId,
defaultSelectedUsers: [item.email],
onChange: (items: any[]) => {
item.email = items[0].secondaryText;
onUpdate(field.id, items[0]);
},
showHiddenInUI: false,
principalTypes: [PrincipalType.User],
});
},
},
{
id: "jobTitle",
title: "Job",
type: CustomCollectionFieldType.string,
required: true,
},
{
id: "description",
title: "Description",
type: CustomCollectionFieldType.string,
required: true,
},
{
id: "arrivalDate",
title: "Arrival Date",
type: CustomCollectionFieldType.custom,
required: true,
onCustomRender: (
field,
value,
onUpdate,
item,
itemId
) => {
return React.createElement(DateTimePicker, {
key: itemId,
showLabels: false,
dateConvention: DateConvention.Date,
showGoToToday: true,
value: value ? new Date(value) : undefined,
onChange: (date: Date) => {
onUpdate(field.id, date);
},
});
},
},
{
id: "hobbies",
title: "Hobbies",
type: CustomCollectionFieldType.string,
required: true,
},
],
disabled: false,
}),
],
},
{
groupName: "Configuration layout",
groupFields: [
PropertyPaneDropdown("position", {
label: "Position",
selectedKey: this.properties.position,
options: [
{ key: "justify-content-start", text: "Start" },
{ key: "justify-content-center", text: "Center" },
{ key: "justify-content-end", text: "End" },
],
}),
],
},
],
},
],
};
}
} |
60. Permutation Sequence
Solved
Hard
Topics
Companies
The set [1, 2, 3, ..., n] contains a total of n! unique permutations.
By listing and labeling all of the permutations in order, we get the following sequence for n = 3:
"123"
"132"
"213"
"231"
"312"
"321"
Given n and k, return the kth permutation sequence.
Example 1:
Input: n = 3, k = 3
Output: "213"
Example 2:
Input: n = 4, k = 9
Output: "2314"
Example 3:
Input: n = 3, k = 1
Output: "123"
Constraints:
1 <= n <= 9
1 <= k <= n!
Solution 1:
class Solution:
def getPermutation(self, n: int, k: int) -> str:
numbers = list(range(1, n + 1))
result = []
factorials = [1]
for i in range(1, n):
factorials.append(factorials[-1] * i)
k -= 1
for i in range(n, 0, -1):
index = k // factorials[i - 1]
result.append(str(numbers.pop(index)))
k %= factorials[i - 1]
return ''.join(result) |
from django.shortcuts import render, redirect
from django.contrib import messages
# Restrict User/////
from django.contrib.auth.decorators import login_required
# imported for if user is not the right user //
from django.http import HttpResponse
# imported for the user registration
# from django.contrib.auth.forms import UserCreationForm
# ..................................
# from django.contrib.auth.models import User
# and there wasn't User after Message initially
from django.contrib.auth import authenticate, login, logout
from django.db.models import Q
from .models import Room, Topic, Message, User
from .forms import RoomForm, UserForm, MyUserCreationForm
# Create your views here.
# rooms = [
# {"id" : 1, "name" : "Lets learn python!"},
# {"id" : 2, "name" : "Design with me"},
# {"id" : 3, "name" : "Frontend developers"},
# ]
def loginPage(request):
# for the register
page = 'login'
if request.user.is_authenticated:
return redirect('home')
if request.method == 'POST':
email = request.POST.get('email').lower()
password = request.POST.get('password')
#use try: to make sure the user actually exists ///
try:
#import models user at the top ///
user = User.objects.get(email=email)
# user = User.objects.get(username="tina")
except:
#import messages at top ////
messages.error(request,'User does not exist')
#if the user exists ///
#import authenticate, login and login out method at the top ///
user = authenticate(request, email=email, password=password)
# user = authenticate(request, username="tina", password="12345678qwertyui")
if user is not None:
login(request, user)
return redirect('home')
else:
messages.error(request,'User OR Password does not exist')
# for the register
context = {'page':page}
return render(request, "home/login_register.html", context)
def logoutUser(request):
logout(request)
return redirect('home')
# for register
def registerPage(request):
form = MyUserCreationForm()
if request.method == 'POST':
# the request.POST is for the password or credentials the user submitted //
form = MyUserCreationForm(request.POST)
if form.is_valid():
# Let's customize user submission //
user = form.save(commit=False)
# the commit=False is so that we can actually access the user right away,
# this is because incase the user added in some UPPERCASE, we want to make sure it's LowerCase automatically or clean it with lowercase
user.username = user.username.lower()
user.save()
# Log the user in and send them to home page ............
login(request, user)
return redirect('home')
else:
messages.error(request, 'An error occured during registration')
return render(request,"home/login_register.html", {'form': form})
# //////////
def homePage(request):
q = request.GET.get("q") if request.GET.get("q") != None else ""
# rooms = Room.objects.filter(topic__name__icontains = q)
rooms = Room.objects.filter(
Q(topic__name__icontains=q) |
Q(name__icontains=q) |
Q(description__icontains=q)
)
# the [0:5] is to limit the topics to the top 5
topics = Topic.objects.all()[0:5]
room_count = rooms.count()
# for recent activities
# room_messages =Message.objects.all()
room_messages =Message.objects.filter(Q(room__topic__name__icontains=q))
# the filter is because, if u click on javascript, only javascript with its recent activaties on it will be
# displayed, instead of with the activities on other Topics
# ...................
context = {'rooms': rooms, 'topics':topics, 'room_count': room_count, 'room_messages' : room_messages}
return render(request, 'home/index.html', context)
def roomPage(request, pk):
room = Room.objects.get(id=pk)
# for the comments in a room
room_messages = room.message_set.all()
# .order_by("-created") means newer messages will come first
# message_set.all() means, give us all the messages that are related to this room,
# the message is from the modal name Message, but now not in capital letter
# ........................
# participants.all() is due to ManyToMany relationships
participants = room.participants.all()
if request.method == "POST":
message = Message.objects.create(
user = request.user,
room = room,
body = request.POST.get("body")
# this "body" is from the input tag with the name "body" in room.html
)
# this is to automatically .add() 0r .remove() a user as a participants when the user comments in a room
room.participants.add(request.user)
return redirect('room', pk = room.id)
context = {'room' : room, 'room_messages' : room_messages, 'participants' : participants}
return render(request, 'home/room.html', context)
# def roomPage(request, pk):
# room = None
# for i in rooms:
# if i ['id'] == int(pk):
# room = i
# context = {'room': room}
# return render(request, 'home/room.html', context)
def userProfile(request, pk):
user = User.objects.get(id = pk)
# get all the users room
rooms = user.room_set.all()
# ,,,,,...........//
topics = Topic.objects.all()
room_messages = user.message_set.all()
context = {"user" : user, "rooms": rooms, "room_messages" : room_messages, "topics": topics}
return render(request, 'home/profile.html', context)
# we want this room to be restricted to certain user
@login_required(login_url = 'login')
def createRoom(request):
form = RoomForm()
topics = Topic.objects.all()
if request.method == "POST":
topic_name = request.POST.get('topic')
# get_or_create enables a user to create a new topic that was not initially created in the database .../
topic, created = Topic.objects.get_or_create(name = topic_name)
Room.objects.create(
host = request.user,
topic = topic,
name = request.POST.get('name'),
description = request.POST.get('description'),
)
# form = RoomForm(request.POST)
# if form.is_valid():
# room = form.save(commit= False)
# room.host = request.user
# room.save()
# form.save()
#dont forget to redirect at the top/////////
return redirect("home")
context = {"form":form, "topics" : topics}
return render(request, "home/room_form.html", context)
# Only authenticated user can update room
@login_required(login_url = 'login')
def updateRoom(request, pk):
room = Room.objects.get(id=pk)
form = RoomForm(instance=room)
topics = Topic.objects.all()
# if user is not the right user or owner here
if request.user != room.host:
return HttpResponse('You are not allowed here!!')
# ////////
if request.method == "POST":
topic_name = request.POST.get('topic')
# get_or_create enables a user to create a new topic that was not initially created in the database .../
topic, created = Topic.objects.get_or_create(name = topic_name)
room.name = request.POST.get('name')
# room.topic = topic is because a user can create a new topic .......//
room.topic = topic
room.description = request.POST.get('description')
room.save()
# form = RoomForm(request.POST, instance=room)
# if form.is_valid():
# form.save()
# dont forget to redirect at the top
return redirect("home")
context = {"form":form, "topics" : topics, "room" : room}
return render(request, "home/room_form.html", context)
# Only authenticated user can delete room
@login_required(login_url = 'login')
def deleteRoom(request, pk):
room = Room.objects.get(id=pk)
# if user is not the right user or owner here
if request.user != room.host:
return HttpResponse('You are not allowed here!!')
# ////////
if request.method == "POST":
room.delete()
return redirect("home")
return render(request, "home/delete.html", {"obj":room})
# Only authenticated user can delete his/her message
@login_required(login_url = 'login')
def deleteMessage(request, pk):
message = Message.objects.get(id=pk)
if request.user != message.user:
return HttpResponse('You are not allowed here!!')
if request.method == "POST":
message.delete()
return redirect("home")
return render(request, "home/delete.html", {"obj":message})
@login_required(login_url = 'login')
def updateUser(request):
user = request.user
form = UserForm(instance=user)
if request.method == 'POST':
# request.FILES is because of the enctype
form = UserForm(request.POST, request.FILES ,instance=user)
if form.is_valid():
form.save()
return redirect('user-profile', pk = user.id)
return render(request, 'home/update-user.html', {'form':form})
def topicsPage(request):
q = request.GET.get("q") if request.GET.get("q") != None else ""
topics = Topic.objects.filter(name__icontains = q)
return render(request, 'home/topics.html', {"topics":topics})
def activityPage(request):
room_messages = Message.objects.all()
return render(request, 'home/activity.html', {'room_messages':room_messages}) |
package com.kubikdata.controller;
import com.kubikdata.domain.Username;
import com.kubikdata.domain.JwtToken;
import com.kubikdata.controller.request.UserSessionRequest;
import com.kubikdata.infrastructure.UserSessionRepository;
import com.kubikdata.service.JwtBuilderGeneratorService;
import org.junit.Assert;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import org.mockito.junit.jupiter.MockitoExtension;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import static org.mockito.Mockito.verify;
import static org.mockito.Mockito.when;
@ExtendWith(MockitoExtension.class)
public class UserSessionControllerShould {
@Mock
JwtBuilderGeneratorService jwtBuilderGeneratorService;
@Mock
UserSessionRepository userSessionRepository;
@InjectMocks
UserSessionController userSessionController;
JwtToken jwtToken = new JwtToken("eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyIjp7ImlkIjoiNWVhYjJkMmUwNDgwNGU3YzgxNmE2YWRlIn0sImlhdCI6MTU4ODY4NjA4NSwiZXhwIjoxNTg4Njg5Njg1fQ.52x2bUKX9Je-4M4TXkZL-OfNOPHdwlfOdIO6km5YkZQ");
@Test
public void create_an_user_session_correctly(){
MockitoAnnotations.initMocks(this);
when(userSessionRepository.find(new Username("Username"))).thenReturn(null);
when(jwtBuilderGeneratorService.generateToken("Username")).thenReturn("eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyIjp7ImlkIjoiNWVhYjJkMmUwNDgwNGU3YzgxNmE2YWRlIn0sImlhdCI6MTU4ODY4NjA4NSwiZXhwIjoxNTg4Njg5Njg1fQ.52x2bUKX9Je-4M4TXkZL-OfNOPHdwlfOdIO6km5YkZQ");
UserSessionRequest userSessionRequest = new UserSessionRequest();
userSessionRequest.setUsername("Username");
ResponseEntity<String> response = userSessionController.addSession(userSessionRequest);
verify(userSessionRepository).save(new Username("Username"), jwtToken);
Assertions.assertEquals(jwtToken.getToken(), response.getBody());
Assertions.assertEquals(HttpStatus.OK, response.getStatusCode());
}
@Test
public void not_allow_create_an_user_session_with_empty_username(){
UserSessionRequest userSessionRequest = new UserSessionRequest();
userSessionRequest.setUsername("");
ResponseEntity<String> response = userSessionController.addSession(userSessionRequest);
Assertions.assertEquals(HttpStatus.BAD_REQUEST, response.getStatusCode());
}
} |
import { Movies } from './components/Movies'
import { useMovies } from './hooks/useMovies'
import { useSearch } from './hooks/useSearch'
import './App.css'
import { useState } from 'react'
import debounce from 'just-debounce-it'
import { useCallback } from 'react'
function App() {
const [sort, setSort] = useState(false)
const { search, setSearch, error } = useSearch()
const { movies, getMovies, loading } = useMovies({ search, sort })
const debouncedGetMovies = useCallback(
debounce(search => {
getMovies({ search })
}, 300)
, [getMovies]
)
const handleSubmit = (event) => {
event.preventDefault()
getMovies({ search })
}
const handleSort = () => {
setSort(!sort)
}
const handleChange = (event) => {
const newSearch = event.target.value
setSearch(newSearch)
debouncedGetMovies(newSearch)
}
return (
<>
<h1 style={{ alignSelf: "center"}}>Movie Finder</h1>
<header >
<form className='form' onSubmit={handleSubmit}>
<label name="movie">Movie:</label>
<input onChange={handleChange} value={search} name="movie" placeholder='Matrix, avengers...'/>
<input type="checkbox" checked={sort} onChange={handleSort}/>
<button type='submit'>Search</button>
</form>
{error && <p style={{ color: 'red'}}>{error}</p> }
</header>
<main>
{ loading ? <p>loading...</p> :
<Movies movies={movies} />}
</main>
</>
)
}
export default App |
package org.example;
import com.opencsv.CSVWriter;
import org.example.database.ConnectDatabaseMySQL;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class Main {
private static final Scanner sc = new Scanner(System.in);
static ConnectDatabaseMySQL CD = new ConnectDatabaseMySQL();
public static void main(String[] args) {
CD.connectTodatabase();
ChooseWhatToDo();
}
private static void saveToCsvFile(){
String fileFormat = ".csv";
System.out.println("The file name: ");
String fileName = sc.next() + fileFormat;
String filePath = "CsvFiles/" + fileName;
try(CSVWriter writer = new CSVWriter(new FileWriter(filePath))) {
System.out.println("How many persons do you want to add?");
Integer addANewPerson = sc.nextInt();
String[] header = {"Name", "Age"};
writer.writeNext(header);
for (int i = 0; i < addANewPerson; i++) {
System.out.println("What name do you what to add?");
String name = sc.next();
System.out.println("What age do you what to add?");
String age = sc.next();
String[] data1 = {name, age};
writer.writeNext(data1);
}
System.out.println("CSV file created successfully.");
}catch (IOException e){
e.printStackTrace();
}
}
private static void saveLoginToCsvFile(){
String fileFormat = ".csv";
System.out.println("The file name: ");
String fileName = sc.next() + fileFormat;
String filePath = "CsvFiles/" + fileName;
try(CSVWriter writer = new CSVWriter(new FileWriter(filePath))) {
String[] header = {"Name", "Age", "Role"};
writer.writeNext(header);
System.out.println("Make a new user: ");
String name = sc.next();
System.out.println("Make a new password: ");
String age = sc.next();
System.out.println("What role does the user have: ");
String role = sc.next();
String[] data1 = {name, age, role};
writer.writeNext(data1);
System.out.println("CSV file created successfully.");
}catch (IOException e){
e.printStackTrace();
}
}
private static void listAllfiles(){
String filePath = "CsvFiles/";
List<String> csvFiles = new ArrayList<>();
File directory = new File(filePath);
if (directory.exists() && directory.isDirectory()){
File[] files = directory.listFiles();
if (files != null){
for (File file : files){
if (file.isFile() && file.getName().endsWith(".csv")){
csvFiles.add(file.getName());
}
}
}
System.out.println("List of CSV files:");
for (String fileName : csvFiles){
System.out.println(fileName);
}
} else {
System.out.println("Directory does not exist or is not a directory.");
}
}
private static void deleteCsvFile(){
System.out.println("The files name: ");
String fileName = sc.next();
String filePath = "CsvFiles/" + fileName +".csv";
File file = new File(filePath);
if (file.exists()){
if (file.delete()){
System.out.println("CSV file deleted successfully.");
}else {
System.out.println("Failed to delete CSV file.");
}
}else {
System.out.println("CSV file does not exist.");
}
}
private static void saveToPersonsTable(){
System.out.println("What file do you what to save into the persons table?");
String fileName = sc.next();
CD.saveCSVFileIntoTheDatabase(fileName);
}
private static void saveToUserTable(){
System.out.println("What file do you what to save into the users table?");
String fileName = sc.next();
CD.saveUserCSVFileIntoTheDatabase(fileName);
}
private static void printMenu(){
String menuText = """
1. Save to CSV file.
2. Save new user to CSV file.
3. Print a list of all csv files.
4. Delete a CSV file.
5. Save CSV into a database.
8. exit the program.
""";
System.out.println(menuText);
}
private static void ChooseWhatToDo(){
printMenu();
while (true){
String choice = sc.next();
switch (choice){
case "1" -> {
saveToCsvFile();
printMenu();
}
case "2" ->{
saveLoginToCsvFile();
printMenu();
}
case "3" ->{
listAllfiles();
printMenu();
}
case "4" ->{
deleteCsvFile();
printMenu();
}
case "5" ->{
ChooseWhatToSave();
printMenu();
}
case "8" -> System.exit(0);
}
}
}
private static void printSaveMenu(){
String menuText = """
1. Save to Person.
2. Save to User.
3. Go Back to Menu.
""";
System.out.println(menuText);
}
private static void ChooseWhatToSave(){
int x = 0;
printSaveMenu();
while (x == 0){
String choice = sc.next();
switch (choice){
case "1" -> {
saveToPersonsTable();
printSaveMenu();
}
case "2" ->{
saveToUserTable();
printSaveMenu();
}
case "3" -> {
printMenu();
x += 1;
}
}
}
}
} |
// @ts-nocheck
import { StatusCodes } from 'http-status-codes'
import * as express from 'express'
import type { Request, Response, NextFunction, Application } from 'express'
import { createClient } from 'redis'
import * as helmet from 'helmet'
import * as session from 'express-session'
import type { SessionOptions } from 'express-session'
import { router as tokens } from './routes/tokens'
import { router as spotifyActions } from './routes/spotify-actions'
import { router as userActions } from './routes/user-actions'
import * as RedisStore from 'connect-redis'
import * as crypto from 'crypto'
import { v4 as uuidv4 } from 'uuid'
import User from './user'
import * as path from 'path'
import * as functions from 'firebase-functions'
declare module 'express-session' {
interface SessionData {
user: User
}
}
require('dotenv').config({ path: path.join(__dirname, '/.env') })
// express protects api from being called on other sites, also known as CORS and helmet secures from other problems
// more info: https://stackoverflow.com/questions/31378997/express-js-limit-api-access-to-only-pages-from-the-same-website
const app: Application = express()
const { REDIS_PORT, REDIS_HOST, REDIS_PASSWORD, SESH_SECRET, NODE_ENV } = process.env
const RedisStorage = RedisStore(session)
if (REDIS_PORT === undefined) {
throw new Error('Redis port is undefined in .env')
}
// Configure redis client
const redisClient = createClient({
host: REDIS_HOST,
port: parseInt(REDIS_PORT),
password: REDIS_PASSWORD
})
redisClient.on('error', function (err) {
console.log('Could not establish a connection with redis. ' + err)
})
redisClient.on('connect', function () {
console.log('Connected to redis successfully')
})
let sesh: SessionOptions
if (process.env.SESH_SECRET) {
sesh = {
store: new RedisStorage({ client: redisClient }),
secret: [SESH_SECRET!],
genid: function () {
return uuidv4() + crypto.randomBytes(48) // use UUIDs for session IDs
},
resave: false,
saveUninitialized: false,
name: '__sess__',
cookie: {
signed: true,
maxAge: 8.64e7 // 1 day to ms
}
}
} else {
throw new Error('NO session secret found on .env')
}
// NODE_ENV is conventionally either 'production' or 'development'
if (NODE_ENV === 'production') {
console.log('Production')
app.set('trust proxy', 1) // trust first proxy
if (sesh.cookie) {
sesh.cookie.secure = true // serve secure cookies
sesh.cookie.httpOnly = true
sesh.cookie.sameSite = true
}
}
// middleware error handling functions need all 4 parameters to notify express that it is error handling
function logErrors (err: { response: { data: any } }, _req: Request, _res: Response, next: NextFunction) {
console.log(err.response.data)
next(err)
}
// the app.use middleware run top down so we log errors at the end
app.use(
helmet({
// don't set CSP (content security policy middle ware) as this will be set manually
contentSecurityPolicy: false
})
)
app.use(
// manually override some attributes of the content security policy
helmet.contentSecurityPolicy({
useDefaults: true,
directives: {
'img-src': [
"'self'",
'data:',
// trust these src's to obtain images, as they are spotify owned
'https://i.scdn.co/',
'https://mosaic.scdn.co/',
'https://lineup-images.scdn.co/'
],
// allow these src's to be used in scripts.
'script-src': [
"'self'",
'https://cdnjs.cloudflare.com',
'https://sdk.scdn.co'
],
'frame-src': ["'self'", 'https://sdk.scdn.co']
}
})
)
app.use(session(sesh))
app.use(express.urlencoded({ extended: true }))
app.use(express.json())
app.use('/tokens', tokens)
app.use('/spotify', spotifyActions)
app.use('/user', userActions)
app.use(logErrors)
app.use(express.static(path.join(__dirname, '/public')))
// '/' represents the home page which will render index.html from express server
app.get('/', function (_req: Request, res: Response) {
res.status(StatusCodes.OK).sendFile(path.join(__dirname, '/public/index.html'))
}) // '/' represents the home page which will render index.html from express server
app.get('/playlists', function (_req: Request, res: Response) {
res
.status(StatusCodes.OK)
.sendFile(path.join(__dirname, '/public/pages/playlists-page/playlists.html'))
})
app.get('/top-tracks', function (_req: Request, res: Response) {
res
.status(StatusCodes.OK)
.sendFile(path.join(__dirname, '/public/pages/top-tracks-page/top-tracks.html'))
})
app.get('/top-artists', function (_req: Request, res: Response) {
res
.status(StatusCodes.OK)
.sendFile(path.join(__dirname, '/public/pages/top-artists-page/top-artists.html'))
})
app.get('/profile', function (_req: Request, res: Response) {
res
.status(StatusCodes.OK)
.sendFile(path.join(__dirname, '/public/pages/profile-page/profile.html'))
})
// clear session data
app.put('/clear-session', function (req: Request, res: Response, next: NextFunction) {
req.session.destroy((err) => next(err))
res.sendStatus(StatusCodes.OK)
})
app.on('listening', function () {
console.log('listening at localhost:' + process.env.EXPRESS_PORT)
// set interval to update secret every minute
setInterval(function () {
crypto.randomBytes(66, function (_err, buffer: Buffer) {
const secret = buffer.toString('hex');
(sesh.secret as Array<string>).unshift(secret)
})
}, 60000)
})
export const server = functions.https.onRequest(app) |
> 本文由 [简悦 SimpRead](http://ksria.com/simpread/) 转码, 原文地址 [juejin.cn](https://juejin.cn/post/7204389419700518967)
1 Gradle 的一些概念
--------------
Android Studio 默认的构建工具为 Gradle。在使用 Gradle 的过程中会碰到一些概念,理清他们的含义关系对我们使用和深入理解 Gradle 至关重要。
### 1.1 Gradle 、AGP(Android Gradle Plugin)、 buildTools 分别是什么,他们之间什么关系?
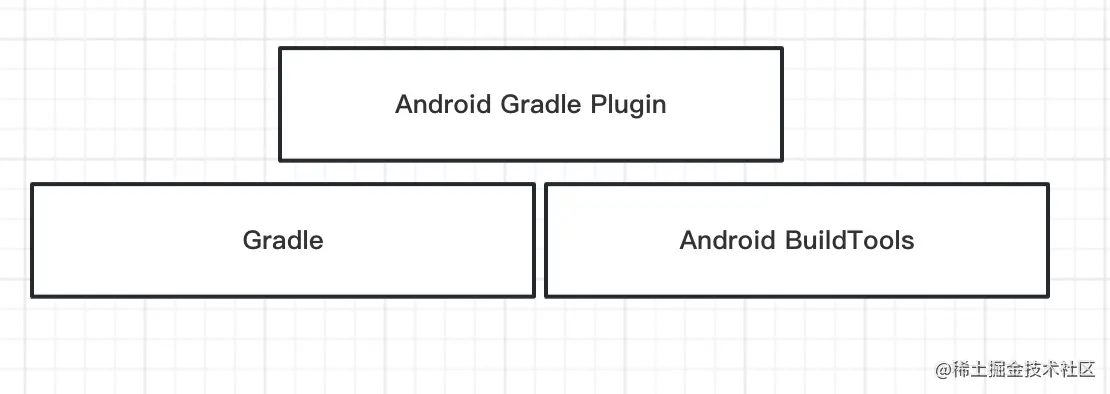
#### 1.1.1 Gradle
Gradle 是基于 JVM 的构建工具。他本身使用 jave 写的,gradle 的脚本也就是 build.gradle 通常是用 groovy 语言。
#### 1.1.2 Android BuildTools
Android SDK Build-Tools 是构建 Android apk、AAR 文件等 Android 平台产物所需的一个 Android SDK 组件,安装在 `<sdk>/build-tools/` 目录下。
```
//build-tools/
├── ⋮
├── aapt2
├── d8
├── apksigner
├── zipalign
├── ⋮
```
* AAPT2:Android 资源打包工具)是一种构建工具,Android Studio 和 Android Gradle 插件使用它来编译和打包应用的。AAPT2 会解析资源、为资源编制索引,并将资源编译为针对 Android 平台进行过优化的二进制格式
* apksigner: 工具为 APK 签名,并确保 APK 的签名将在该 APK 支持的所有版本 Android 平台上成功通过验证
* d8:Android Gradle 插件使用该工具来将项目的 Java 字节码编译为在 Android 设备上运行的 DEX 字节码
* Zipalign: 在将 APK 文件分发给最终用户之前,先使用 `zipalign` 进行优化
#### 1.1.3 Android Gradle Plugin
是基于 Gradle 的插件,Android Gradle 主要就是调用 [Android BuildTools] 这个包里的工具实现编译打包 Apk 等功能。
注: AGP3.0.0 或更高版本,插件会使用默认版本的 build Tools 无需指定。
```
android { buildToolsVersion "33.0.1"}
```
### 1.2 gradleWrapper、gradle-user-home、GradleHome
#### 1.2.1 GradleWrapper
项目的根目录的 gradle/wrapper 下
```
/gradle/wrapper/
├── gradle-wrapper.jar
└── gradle-wrapper.properties
```
负责下载管理特定版本的 gradle,确保不同环境中项目运行在相同个 gradle 版本里,还能达到复用效果。
* gradlewraper 目录下的 gradle.jar 负载下载 gradle
* gradle-wrapper.properties 内指定 gradle 的版本,下载地址,本地位置等配置信息。
#### 1.2.1 GRADLE-USER-HOME
```
gradle-user-home
├── caches // modules-2和jars-3缓存依赖和产物,且各个版本各个项目中共用
│ ├── jars-3
| ├── transforms-3
│ └── modules-2
├── wrapper //存放不同版本的gradle
│ └── dists
│ ├── ⋮
│ └── gradle-7.4-bin
| └── xxxxxxxx
| └──gradle-7.3.3 //其实这是gradle home路径
└── gradle.properties
```
默认在位置位于 /.gradle。这个目录下包含用户自定义的设置、maven 本地仓库、gradle wrapper 版本、gradle 插件和一些缓存。其中该路径下 caches 文件夹有 repo 缓存也就是项目的依赖缓存,这个缓存各个项目是公用的,提升编译速度。
#### 1.2.3 Gradle Home
```
gradle-home
├── bin
│ ├── gradle.bat
│ └── gradle
├── init.d
│ ├──..
│ └── xx.gradle
└── lib
├──...
├── gradle-wrapper-7.3.3.jar
├── gradle-tooling-api-7.3.3.jar
├──...
└── plugins
├── ...
├── gradle-publish-7.3.3.jar
├── commons-codec-1.15.jar
└──...
```
其实就是 gradle 的安装目录,里面主要包括 Gradle 的可执行文件、库文件、插件等,gradle 执行时会自动加载使用安装目录内的文件插件。
2 Gradle 生命周期
-------------
Gradle 执行构建是有生命周期的,分三个阶段初始化阶段(Initialization Phases),配置阶段 (Configuration Phases) 和执行阶段(Execution Phases)。
#### 2.1 初始化阶段
* 执行 init.gradle 脚本: init.gradel 脚本可以放置在 gradle-user-home 目录下、gradle-user-home/init.d / 和 gradle-home/init.d / 目录下,其中后两者下的脚本名称只要一. gralde 结尾即可。他们的执行顺序为依次执行。在脚本里我们可以设置一些个性化的配置,如配置 repo 源、鉴权等。
* 执行 setting 脚本: gradle 会嗅探项目里的 settings 文件,settings 文件中定义哪些项目参与到构建中。在 settings.gradle 文件中可以设置一些对所有参与的构建的项目做配置,也可以有针对性的做配置。
* 创建 project 实例:为参与到构建中的项目创建 project 实例。我们在项目的 build.gradle 脚本里使用的 project 对象就是在这个时机创建的。
#### 2.2 配置阶段
* 应用插件:下载应用声明的插件,脚本里有两种写法一种是 apply plugin 和 plugins{}。他们的区别暂不作展开。
* 配置属性:给插件做参数配置,我们最熟悉的 android{} 闭包其实就是对 AGP 里的 com.android.application 插件做参数配置。
* 应用依赖:gradle 脚本中声明使用的依赖也会在配置阶段下载应用。
* 构建有向无环 task 任务图。
* _注意_:* 执行任何 task 都会走初始化阶段和配置阶段
#### 2.3 执行阶段
执行上一个阶段也就是配置阶段构造好的有向无环图任务。
```
tasks.register('test') {
println '该语句会在配置阶段执行'
doLast {
println '该语句会在执行阶段运行'
}
}
```
3 监听 Gradle 生命周期回调
------------------
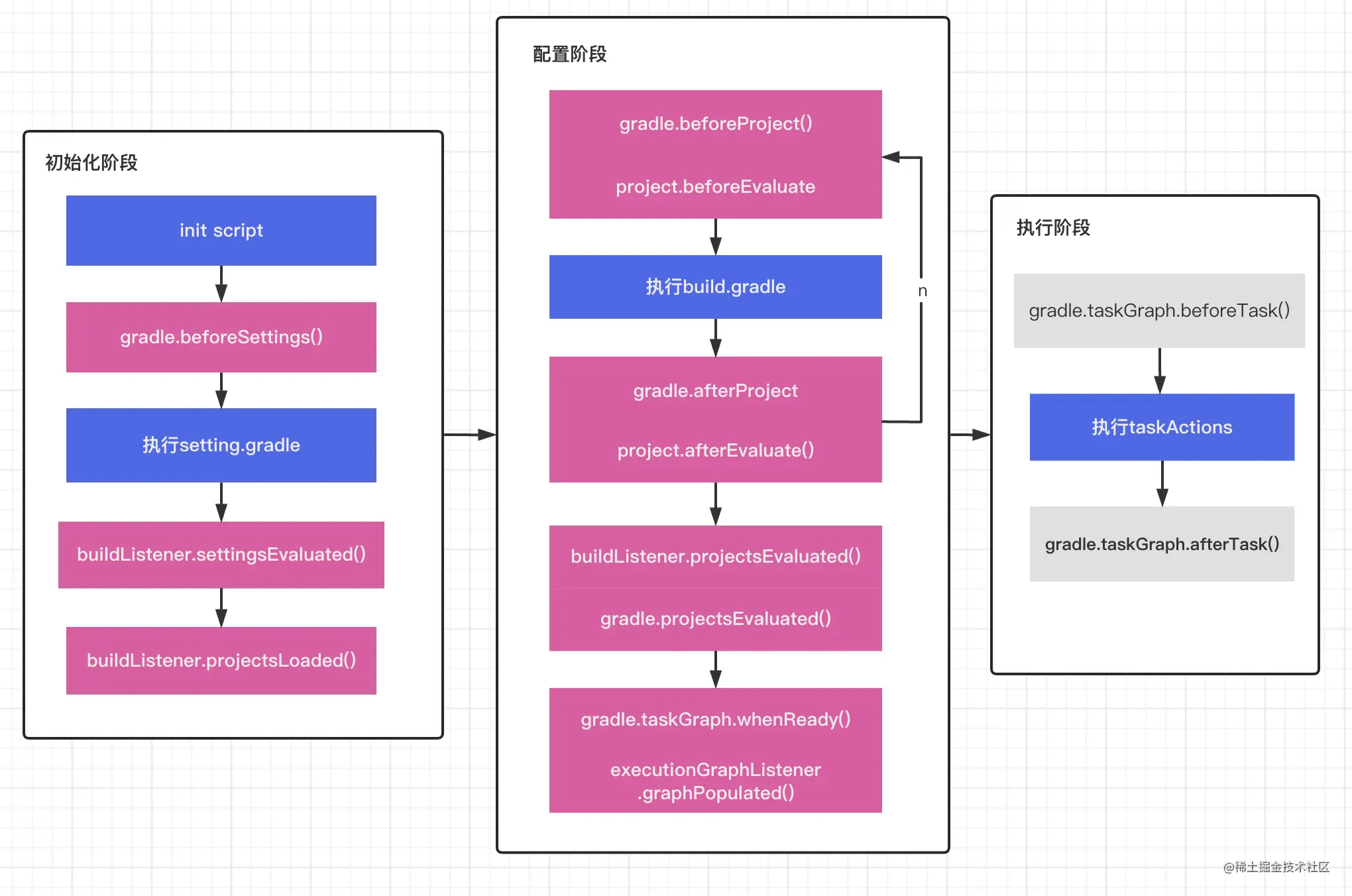
#### 3.1 监听初始化阶段回调
初始化阶段主要有两个能接收回调的地方 settingEvaluated{} 和 gradle.projectsLoaded {}
```
//settings.gradle配置执行完成
gradle.settingsEvaluated {
...
}
```
settingEvaluated{}setting 创建加载成功。在此之前有几项 gradle 有几项重要的工作已经完成。
* gradle.properties 加载完成
* init 脚本执行完成
* Settings 对象创建成功,并且从 gradle.properties 加载的值也注入到了 settings 中
```
// 所有 Project 对象创建(注意:此时 build.gradle 中的配置代码还未执行)
gradle.projectsLoaded {
...
}
```
初始化阶段还有扫描项目下的所有包含位于根 project 和子 project 中的 build.gradle 并对应生成 Project 对象,对象创建完成之后就会调用 gradle.projectsLoaded{},**但注意此时 build.gradle 中的配置代码还未执行。**
#### 3.2 配置阶段的回调
配置阶段会对项目下的每个 build.gradle 进行配置,如上图,beforeProject{}/project.beforeEvaluated{} 和 afterProject{}/after.beforeEvaluate 会执行多次,这取决于构建项目下有几个 project。
* beforeProject{}/project.beforeEvaluated{}:会在每个 project 配置之前调用.
* afterProject{}/after.beforeEvaluate:会在 build.gradle 脚本 "从头跑到尾" 之后调用。
* 当所有的 gradle.build 都执行完毕后,会调用 projectEvaluted{}
* 最后,task 图构建完成后,回调 gradle.taskGraph.whenReady {} 和 gradle.taskGraph.taskExecutionGraphListener.graphPopulated()
#### 3.3 hook 执行阶段
每个 task 执行前后,我们也有方式去监听:主要是使用 gradle.taskGraph.beforeTask{} 和 gradle.taskGraph.afterTask {},如下:
```
gradle.taskGraph.beforeTask { Task task ->
println "executing $task ..."
}
gradle.taskGraph.afterTask { Task task, TaskState state ->
if (state.failure) {
println "FAILED"
}
else {
println "done"
}
}
```
* **!!! 注意:** 这些方法在 gradle8.0 已经直接被移除掉了统统不能用,原因简单的说就是影响 cofigurationCache 具体看这里: [task_execution_events](https://link.juejin.cn?target=https%3A%2F%2Fdocs.gradle.org%2Fcurrent%2Fuserguide%2Fupgrading_version_7.html%23task_execution_events "https://docs.gradle.org/current/userguide/upgrading_version_7.html#task_execution_events")
* 替代的方式:使用 [build_service](https://link.juejin.cn?target=https%3A%2F%2Fdocs.gradle.org%2Fcurrent%2Fuserguide%2Fbuild_services.html%23concurrent_access_to_the_service "https://docs.gradle.org/current/userguide/build_services.html#concurrent_access_to_the_service")
4 Gradle 项目结构
-------------
```
root-project
├── buildSrc
| ├── ...
│ └── build.gradle
├── sub-project
│ └── build.gradle
├── build.gradle
├── settings.gradle
├── gradle.properties
├── gradle
│ └── wrapper
│ ├── gradle-wrapper.jar
│ └── gradle-wrapper.properties
├── gradlew(Unix Shell script)
└── gradlew.bat(Windows batch file)
```
### 4.1 buildSrc
* buildSrc 是位于 gradle 项目根目录的特殊目录,我们可以在这编写 Gradle 的插件和任务。
* 这里面的代码会在 gardle 的配置阶段执行。
### 4.2 gradle.Properties
* 在 gradle-user-home 目录下,项目根目录下和子项目目录下(和 build.gradle 同级)下都可以存在。
* gradle.properties 定义的值有些时配置 gradle 环境属性,也可以添加自己自定义的属性,在我们的构建脚本中可以直接访问这些值。
```
//gradle.properties中
mVersionName=1.12.1
//build.gradle
println("mVersion name in gradle.properties ${mVersionName}")
```
### 4.3 build.gradle 和 settings.gradle 文件
Gradle 世界里经过初始化阶段后,build.gradle 文件会对应生成 Poject 对象,settings.gradle 文件会生成 Settings 对象,其实还有 Gradle 对象,每次构建启动时就会创建。
5 plugin 与 task
---------------
### 5.1 Gradle Plugin
Gradle 是一个构建框架,本身不具备特别强的构建能力,具体构建能力依托于 Gradle Plugin ,比如要打包 APK 就要依托 AGP。AGP 内部通过调用 Android Build Tool 去具体执行诸如编译 class,打包资源等任务。
#### 5.1.1 Apply plugin 方式引入
Gradle Plugin 的是使用首先是引入,就跟我们在项目里需要某个第三方库需要引入一样。在跟目录的 build.gradle 中就可声明这些依赖,然后在构建脚本里声明应用,然后做配置。
```
//in rootproject/build.gradle
buildscript {
...
dependencies {
classpath:'com.android.tools.build:gradle:x.x.x'
}
}
//in subProject/build.gradle
apply plugin: 'com.android.application'//用于打包生成Apk
android {//构建Apk的配置
}
//in subProject2/build.gradle
apply plugin: 'com.android.library'//用于打包产出AAR
android{ //构建产生aar配置
}
```
如果在脚本 gradle 脚本中想使用一个第三方库,也需要通过 classpath 引入,然后就可以在脚本中使用了,示例如下。
```
//in root project build.gradle
buildscript {
dependencies {
classpath: 'com.squareup.okhttp3:okhttp:3.14.9'
}
}
import okhttp3.OkHttpClient
task("checkClasspath").doFirst {
//在脚本中使用引入了的okhttp
def client = new OkHttpClient()
..,
}
```
#### 5.1.2 plugin{} 脚本块引入
以上这种使用 plugin 的方式是分两步【引入 + 应用】,gradle 还有引入和应用一步搞定的方式。
```
plugin{ id 'com.android.library' version 'x.x.x' }
```
默认的这种不需要引入就能应用插件的方式,插件来源只能是 gradle 插件官网 [plugins.gradle.org/](https://link.juejin.cn?target=https%3A%2F%2Fplugins.gradle.org%2F "https://plugins.gradle.org/"),如果想使用自己的插件需要在 pluginManagement 中配置源如下
```
//settings.gradle
pluginManagement {
repositories {
maven {
url './maven-repo'
}
}
}
```
#### 5.1.3 其他
Gradel 插件分两种:二进制插件和脚本插件; 二进制插件就是被打包成了 jar 包的插件。脚本插件. gradle 文件可以被作为插件使用。这种插件的使用只能通过 apply plugin 方式不能使用 plugin{} 的方式。
//config.gradle 中
```
ext {
mVersion = '1.1.0'
}
```
//build.gradle
```
apply from: "../config.gradle"
println("mVersion${ext.mVersion}")
```
### 5.2 Task
#### 5.2.1 执行动作
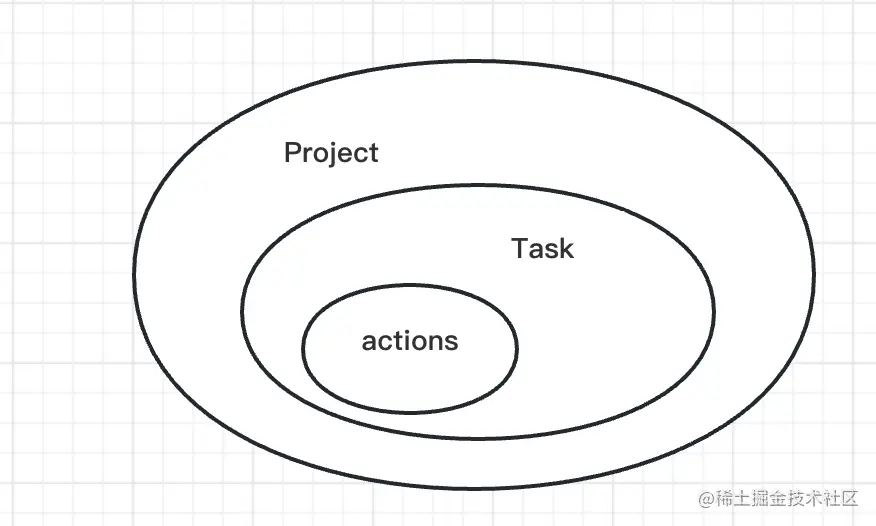
* 上文也提到过,gradle 的一次构建,就是一系列 task 的执行,task 就是 gradle 的一个执行单元。
* 一个 gradle 项目中包含若干个 project,每个 project 中包含若干个 task。
* 一个 gradle task 里面会包含若干个 action。
#### 5.2.2 创建 task
task 一般都定义在 plugin 中,我们也可以自己定义 task,给其配置名字,描述,分组,action,依赖关系等。
```
//build.gradle
project.tasks.register("aTask") {
println 'in configuration phase'
it.description("this is a sample task")
it.dependsOn("hello")
group("build")//IDE右边的Gradle快捷工具栏中,会看到该task被归类到build分组内,方便查找
description "this is a gradle hello sample" //描述
it.doLast {
println("do action >>1") //向当前task的action链路后追加action
}
it.doFirst {
println("do aciton >>2")//向当前task的action链路前查action
}
}
project.tasks.register("hello") {
it.doLast {
println("hello task is excuted-")
}
}
```
以上只是一个简单的例子,task 的创建使用可以单开一个章节。
6 结
---
本文主要是在一个可能比较高的视角,谈了谈 gradle 相关的概念,生命周期特性,插件 task 等。掌握了这些之后,还有一些更深入更细致的话题值得探索。比如
* 自定义插件,细致的 Task 详解
* gradle tansformer
* 构建性能如何提升
* AGP 常见的配置
* Gradle 项目实战
今后会写系列文章去一一探索,**关注作者获取更新**。
**写文不易,错误不足之处望不吝赐教,点赞鼓励** |
/*
* Copyright (c) 2021, UC Innovation, Inc. All Rights Reserved.
* http://www.ucinnovation.com
*
* This source code is licensed, not sold, and is subject to a written
* license agreement. Among other things, no portion of this source
* code may be copied, transmitted, disclosed, displayed, distributed,
* translated, used as the basis for a derivative work, or used, in
* whole or in part, for any program or purpose other than its intended
* use in compliance with the license agreement as part of UC Innovation's
* software. This source code and certain of the algorithms contained
* within it are confidential trade secrets of UC Innovation, Inc.
* and may not be used as the basis for any other
* software, hardware, product or service.
*/
/**
* trigger for the job object
*
* @author: Ashley Tran
* @version: 1.0
* @since: 1.0
*/
global without sharing class PORTAL_TRIG_JobHandler extends ucinn_ascendv2.ascend_TDTM_Runnable {
public static final String JOB_API_NAME = 'ucinn_portal_Job__c';
public static final Map<String, String> JOB_FIELD_TO_ADDRESS_WRAPPER_FIELD_MAP = new Map<String, String>{
'Address_Line_1__c' => PORTAL_AddressWrapper.STREET_LINE_1,
'Address_Line_2__c' => PORTAL_AddressWrapper.STREET_LINE_2,
'Address_Line_3__c' => PORTAL_AddressWrapper.STREET_LINE_3,
'City__c' => PORTAL_AddressWrapper.CITY_CONST,
'State__c' => PORTAL_AddressWrapper.STATE_CONST,
'Country__c' => PORTAL_AddressWrapper.COUNTRY_CONST,
'Postal_Code__c' => PORTAL_AddressWrapper.POSTAL_CODE
};
/**
* Method occurs on every trigger run.
*
* @param newList List of the new up to date version of the records from the triggers
* @param oldList List of the old version of the records from the trigger
* @param triggerAction Contains information about the trigger
* @param objResult Information about the obect in the trigger
* @return a new ucinn_ascendv2.ascend_TDTM_Runnable.DmlWrapper
* @since 1.0
*/
global override ucinn_ascendv2.ascend_TDTM_Runnable.DmlWrapper run(List<SObject> newList, List<SObject> oldList, ucinn_ascendv2.ascend_TDTM_Runnable.Action triggerAction, Schema.DescribeSObjectResult objResult) {
if (System.isBatch() == true || System.isFuture() == true) {
return new ucinn_ascendv2.ascend_TDTM_Runnable.DmlWrapper();
}
if (triggerAction == ucinn_ascendv2.ascend_TDTM_Runnable.Action.AfterInsert) {
geolocateJobs((List<ucinn_portal_Job__c>) newList);
} else if (triggerAction == ucinn_ascendv2.ascend_TDTM_Runnable.Action.AfterUpdate) {
geolocateJobs((List<ucinn_portal_Job__c>) oldList, (List<ucinn_portal_Job__c>) newList);
}
return new ucinn_ascendv2.ascend_TDTM_Runnable.DmlWrapper();
}
/**
* method that checks to see if the newly inserted jobs need to be
* geolocated
*
*
* @author: Ashley Tran
* @since: 1.0
*
*
* @param jobList the newly inserted jobs
*
*
*/
public static void geolocateJobs(List<ucinn_portal_Job__c> jobList) {
Map<String, String> jobIdToAddressWrapperMap = new Map<String, String>();
for (ucinn_portal_Job__c eachNewJob : jobList) {
PORTAL_AddressWrapper addressWrapper = getAddressWrapper(eachNewJob);
if (addressWrapper != null) {
jobIdToAddressWrapperMap.put(eachNewJob.Id, JSON.serialize(addressWrapper, true));
}
}
if (!jobIdToAddressWrapperMap.isEmpty()) {
geolocateAddresses(jobIdToAddressWrapperMap);
}
}
/**
* method that compares the address fields of the old job against the new
* job in order to determine if the job needs to be geolocated
*
* @author: Ashley Tran
* @since: 1.0
*
*
* @param oldJobList the old values for the jobs
* @param jobList the newly updated jobs
*
*
*/
public static void geolocateJobs(List<ucinn_portal_Job__c> oldJobList, List<ucinn_portal_Job__c> jobList) {
Map<String, String> jobIdToAddressWrapperMap = new Map<String, String>();
for (Integer index = 0; index < jobList.size(); index++) {
ucinn_portal_Job__c oldJob = oldJobList.get(index);
ucinn_portal_Job__c newJob = jobList.get(index);
PORTAL_AddressWrapper addressWrapper = getUpdatedJobAddressWrapper(oldJob, newJob);
if (addressWrapper != null) {
jobIdToAddressWrapperMap.put(newJob.Id, JSON.serialize(addressWrapper, true));
}
}
if (!jobIdToAddressWrapperMap.isEmpty()) {
geolocateAddresses(jobIdToAddressWrapperMap);
}
}
/**
* Compare two jobs and see if their address fields are different
*
* @author Jacob Huang
* @since 1.0
*
* @param oldJob ucinn_portal_Job__c: original/old job
* @param newJob ucinn_portal_Job__c: updated/new job
*
* @return PORTAL_AddressWrapper of the newJob only if the new job has different address fields than old job
*/
private static PORTAL_AddressWrapper getUpdatedJobAddressWrapper(ucinn_portal_Job__c oldJob, ucinn_portal_Job__c newJob) {
for (String eachCurrentField : JOB_FIELD_TO_ADDRESS_WRAPPER_FIELD_MAP.keySet()) {
if (oldJob?.get(eachCurrentField) != newJob?.get(eachCurrentField)) {
return getAddressWrapper(newJob);
}
}
return null;
}
/**
* Use Job address fields to create an Address Wrapper
*
* @since 1.0
*
* @param jobRecord ucinn_portal_Job__c: Any job object with street, city, state, country, and/or postal code fields
*
* @return PORTAL_AddressWrapper representing the job address
*/
private static PORTAL_AddressWrapper getAddressWrapper(ucinn_portal_Job__c jobRecord) {
Map<Object, Object> paramMap = new Map<Object, Object>();
for (String eachJobField : JOB_FIELD_TO_ADDRESS_WRAPPER_FIELD_MAP.keySet()) {
String fieldValue = (String) jobRecord.get(eachJobField);
if (String.isNotBlank(fieldValue)) {
paramMap.put(JOB_FIELD_TO_ADDRESS_WRAPPER_FIELD_MAP.get(eachJobField), fieldValue);
}
}
return paramMap.isEmpty() ? null : new PORTAL_AddressWrapper(paramMap);
}
/**
* method that takes a map of job id to addresses and makes the geolocation
* calls to the api. once the geoloation is returned, it will update the job
*
* @author: Ashley Tran
* @since: 1.0
*
*
* @param jobIdToAddressWrapperMap map of job id to deserialized address wrapper
*/
@future(callout = true)
private static void geolocateAddresses(Map<String, String> jobIdToAddressWrapperMap) {
List<ucinn_portal_Job__c> jobsToUpdate = new List<ucinn_portal_Job__c>();
for (String eachJobId : jobIdToAddressWrapperMap.keySet()) {
PORTAL_AddressWrapper address = (PORTAL_AddressWrapper) JSON.deserialize(jobIdToAddressWrapperMap.get(eachJobId), PORTAL_AddressWrapper.class);
Double latitude = address.getLatitude();
Double longitude = address.getLongitude();
if (latitude != null && longitude != null) {
jobsToUpdate.add(new ucinn_portal_Job__c(Id = eachJobId, Geolocation__latitude__s = latitude, Geolocation__longitude__s = longitude));
}
}
ucinn_ascendv2.ascend_UTIL_TDTMTrigger.toggleTDTMTrigger(JOB_API_NAME, false);
update jobsToUpdate;
ucinn_ascendv2.ascend_UTIL_TDTMTrigger.toggleTDTMTrigger(JOB_API_NAME, true);
}
} |
import React,{useState} from 'react'
import 'codemirror/lib/codemirror.css'
import 'codemirror/theme/material.css'
import 'codemirror/mode/xml/xml'
import 'codemirror/mode/javascript/javascript'
import 'codemirror/mode/css/css'
import {Controlled as ControlledEditor} from 'react-codemirror2'
import {FontAwesomeIcon} from '@fortawesome/react-fontawesome'
import {faCompressAlt , faExpandAlt} from '@fortawesome/free-solid-svg-icons'
const Editor = (props) => {
const {
displayName,
value,
language,
onChange
}= props
const handleChange= (editor,data,value) =>{
onChange(value)
}
const [open,setOpen]=useState(true)
return (
<div className={`editor-container ${open ? '':`collapsed`}`}>
<div className="editor-title">
{displayName}
<button
type="button"
className="expand-collapse-btn"
onClick={()=> setOpen(prevOpen => !prevOpen)}
>
<FontAwesomeIcon icon={open ? faCompressAlt : faExpandAlt
}/>
</button>
</div>
<ControlledEditor
onBeforeChange={handleChange}
value={value}
className="code-mirror-wrapper"
options={{
lineWrapping: true,
lint:true,
mode:language,
lineNumbers:true,
theme:'material'
}}
/>
</div>
)
}
export default Editor |
<template>
<v-card max-width="60em" class="mx-auto">
<v-toolbar flat>
<v-row align="center">
<v-col cols="auto">
<v-toolbar-title v-if="user">
<a v-if="user_profile_url" :href="user_profile_url">{{
user.display_name
}}</a>
<span v-else>{{ user.display_name }}</span>
</v-toolbar-title>
<v-toolbar-title v-else>
<v-progress-circular indeterminate />
</v-toolbar-title>
</v-col>
<v-spacer />
<v-col cols="auto">
<v-btn exact :to="{ name: 'CreateGroup' }">
<v-icon left>mdi-account-multiple-plus</v-icon>
<span>{{ $t("Create group") }}</span>
</v-btn>
</v-col>
</v-row>
<template v-slot:extension>
<v-tabs v-model="relation_tab">
<v-tab>{{ $t("As member") }}</v-tab>
<v-tab>{{ $t("As administrator") }}</v-tab>
</v-tabs>
<v-spacer />
<v-switch v-model="shallow" label="Direct" />
</template>
</v-toolbar>
<v-divider />
<v-card-text>
<v-tabs-items v-model="relation_tab">
<v-tab-item
v-for="relationship in ['member', 'administrator']"
:key="`as_${relationship}`"
>
<v-card outlined>
<v-toolbar flat>
<v-tabs v-model="officiality_tab">
<v-tab>{{ $t("Official") }}</v-tab>
<v-tab>{{ $t("Non-official") }}</v-tab>
</v-tabs>
</v-toolbar>
<v-divider />
<v-card-text>
<v-tabs-items v-model="officiality_tab">
<v-tab-item
v-for="officiality in ['official', 'nonofficial']"
:key="`as_${relationship}_${officiality}`"
>
<GroupsOfUser
:official="officiality === 'official'"
:nonofficial="officiality === 'nonofficial'"
:shallow="shallow"
:as="relationship"
/>
</v-tab-item>
</v-tabs-items>
</v-card-text>
</v-card>
</v-tab-item>
</v-tabs-items>
</v-card-text>
</v-card>
</template>
<script>
import GroupsOfUser from "@/components/GroupsOfUser.vue"
const { VUE_APP_USER_MANAGER_FRONT_URL } = process.env
export default {
name: "UserGroups",
components: {
GroupsOfUser,
},
data() {
return {
user: null,
loading: false,
relation_tab: null,
officiality_tab: null,
shallow: false,
}
},
mounted() {
this.get_user()
},
watch: {
user_id() {
this.get_user()
},
},
methods: {
get_user() {
this.loading = true
const url = `/v3/users/${this.user_id}`
this.axios
.get(url)
.then(({ data }) => {
this.user = data
})
.catch((error) => {
console.error(error)
})
.finally(() => {
this.loading = false
})
},
},
computed: {
user_id() {
return this.$route.params.user_id
},
user_profile_url() {
if (!VUE_APP_USER_MANAGER_FRONT_URL) return
return `${VUE_APP_USER_MANAGER_FRONT_URL}/users/${this.user_id}`
},
},
}
</script> |
import { DatePicker, TimePicker, message } from "antd";
import axios from "axios";
// import moment from "moment";
import dayjs from "dayjs";
import React, { useEffect, useState } from "react";
import { useParams } from "react-router-dom";
import { Store } from "../../components/Online-shopping-components/Store";
import { useContext } from "react";
// import "./BookAppointment.css";
// import Img from "../../assets/images/home-banner-image.png";
function BookAppointment() {
// const { user } = useSelector((state) => state.user);
const params = useParams();
const [doctors, setDoctors] = useState();
const [date, setDate] = useState(null);
const [time, setTime] = useState(null);
const [isAvailable, setIsAvailable] = useState(false);
const {state , dispatch : ctxDispatch} = useContext(Store);
const {userInfo } = state
const getDoctorData = async () => {
try {
const res = await axios.get(`/api/doctor/getDoctorInfoById/${params.doctorId}`,
{ doctorId: params.doctorId }
// {
// headers: {
// Authorization: "Bearer " + localStorage.getItem("token"),
// },
// }
);
if (res.data.success) {
setDoctors(res.data.data);
}
} catch (error) {
console.log(error);
}
};
const handleBooking = async () => {
setIsAvailable(false); //--
try {
// setIsAvailable(true);
// if (!date && !time) {
// return alert("Date & Time Required");
// }
const res = await axios.post(
"/api/doctor/bookAppointmentByUser", //change to user
{
doctorId: params.doctorId,
userId: userInfo._id,
doctorInfo: doctors,
userDes: userInfo,
date: date,
time: time,
}
// {
// headers: {
// Authorization: `Bearer ${localStorage.getItem("token")}`,
// },
// }
);
if (res.data.success) {
message.success(res.data.message);
}
} catch (error) {
console.log(error);
}
};
const handleAvailability = async () => {
try {
const res = await axios.post(
"/api/doctor/checkBookingAvailability",
{ doctorId: params.doctorId, date: date, time: time },
{
// headers: {
// Authorization: `Bearer ${localStorage.getItem("token")}`,
// },
}
);
if (res.data.success) {
message.success(res.data.message);
setIsAvailable(true);
} else {
message.error(res.data.message);
}
} catch (error) {
console.log(error);
}
};
useEffect(() => {
getDoctorData();
console.log("getDoctorData()");
//eslint-disable-next-line
}, []);
return (
<div className="container m-2 p-4 border shadow rounded">
{doctors && (
<div className="d-flex">
<div className="p-4">
<h5 className="mb-5">
Dr.{doctors.name} ({doctors.designation}){" "}
</h5>
<img
src="https://thumbs.dreamstime.com/b/finger-press-book-now-button-booking-reservation-icon-online-149789867.jpg"
width="50%"
height="20%"
/>
</div>
<div className="mt-5 p-3 ">
<h5>Specialization : {doctors.specialization}</h5>
<h5>Email : {doctors.email}</h5>
<h5>Phone : {doctors.phone}</h5>
<h5>
Timings : {doctors.timings && doctors.timings[0]} -{" "}
{doctors.timings && doctors.timings[1]}{" "}
</h5>
<div className="d-flex flex-column ">
<DatePicker
aria-required={"true"}
className="my-2"
style={{ width: "100%" }}
format="DD-MM-YYYY"
onChange={(value) => {
setDate(dayjs(value).format("DD-MM-YYYY"));
setIsAvailable(false);
}}
size="large"
/>
<TimePicker
aria-required={"true"}
format="HH:mm"
className="mb-2"
style={{ width: "100%" }}
onChange={(value) => {
setTime(dayjs(value).format("HH:mm"));
setIsAvailable(false);
}}
size="large"
/>
{!isAvailable && <button
className="btn btn-primary mt-2"
onClick={handleAvailability}
>
Check Availability
</button>}
{isAvailable && <button className="btn btn-dark mt-2" onClick={handleBooking}>
Book Now
</button>}
</div>
</div>
</div>
)}
</div>
);
}
export default BookAppointment; |
import { Vector3 } from "three";
import { generateUUID } from "three/src/math/MathUtils";
import {
SimpleDimensionLine,
LengthMeasurement,
} from "../../measurement/LengthMeasurement";
import { Components } from "../../core/Components";
import {
UI,
Component,
Event,
FragmentIdMap,
UIElement,
} from "../../base-types";
import { Button, SimpleUICard, FloatingWindow } from "../../ui";
import { DrawManager } from "../../annotation";
import { OrthoPerspectiveCamera } from "../OrthoPerspectiveCamera";
import { CameraProjection } from "../OrthoPerspectiveCamera/src/types";
import { FragmentHighlighter } from "../../fragments";
// TODO: Update and implement memory disposal
export interface IViewpointsManagerConfig {
selectionHighlighter: string;
drawManager?: DrawManager;
}
export interface IViewpoint {
guid: string;
title: string;
description: string | null;
position: Vector3;
target: Vector3;
selection: FragmentIdMap;
projection: CameraProjection;
dimensions: { start: Vector3; end: Vector3 }[];
filter?: { [groupSystem: string]: string };
annotations?: SVGGElement;
}
export class ViewpointsManager extends Component<string> implements UI {
// private _fragmentManager: FragmentManager;
// private _fragmentGrouper: FragmentGrouper;
private _drawManager?: DrawManager;
name: string = "ViewpointsManager";
uiElement = new UIElement<{
main: Button;
newButton: Button;
window: FloatingWindow;
}>();
enabled: boolean = true;
list: IViewpoint[] = [];
selectionHighlighter = "";
readonly onViewpointViewed = new Event<string>();
readonly onViewpointAdded = new Event<string>();
constructor(components: Components) {
super(components);
this.components = components;
}
initialize(config: IViewpointsManagerConfig) {
this.selectionHighlighter = config.selectionHighlighter;
// this._fragmentGrouper = config.fragmentGrouper;
// this._fragmentManager = config.fragmentManager;
this._drawManager = config.drawManager;
if (this.components.ui.enabled) {
this.setUI();
}
}
private setUI() {
const viewerContainer = this.components.renderer.get().domElement
.parentElement as HTMLElement;
const window = new FloatingWindow(this.components);
window.title = "Viewpoints";
viewerContainer.append(window.get());
window.visible = false;
const main = new Button(this.components, {
materialIconName: "photo_camera",
});
const newButton = new Button(this.components, {
materialIconName: "add",
name: "New viewpoint",
});
const listButton = new Button(this.components, {
materialIconName: "format_list_bulleted",
name: "Viewpoints list",
});
listButton.onClick.add(() => {
window.visible = !window.visible;
});
main.addChild(listButton, newButton);
this.uiElement.set({ main, newButton, window });
}
get(): string {
throw new Error("Method not implemented.");
}
async add(data: { title: string; description: string | null }) {
const { title, description } = data;
if (!title) {
return undefined;
}
const guid = generateUUID().toLowerCase();
// #region Store dimensions
const dimensions: { start: Vector3; end: Vector3 }[] = [];
const dimensionsComp = await this.components.tools.get(LengthMeasurement);
const allDimensions = dimensionsComp.get();
for (const dimension of allDimensions) {
dimensions.push({ start: dimension.start, end: dimension.end });
}
// #endregion
// #redgion Store selection
const highlighter = await this.components.tools.get(FragmentHighlighter);
const selection = highlighter.selection[this.selectionHighlighter];
// #endregion
// #region Store filter (WIP)
// const filter = {entities: "IFCBEAM", storeys: "N07"}
// #endregion
// #region Store camera position and target
const camera = this.components.camera as OrthoPerspectiveCamera;
const controls = camera.controls;
const target = new Vector3();
const position = new Vector3();
controls.getTarget(target);
controls.getPosition(position);
const projection = camera.getProjection();
// #endregion
// #region Store annotations
const annotations = this._drawManager?.saveDrawing(guid);
// #endregion
const viewpoint: IViewpoint = {
guid,
title,
target,
position,
selection,
// filter,
description,
dimensions,
annotations,
projection,
};
// #region UI representation
const card = new SimpleUICard(this.components, viewpoint.guid);
card.title = title;
card.description = description;
card.domElement.onclick = () => this.view(viewpoint.guid);
this.uiElement.get("window").addChild(card);
// #endregion
this.list.push(viewpoint);
await this.onViewpointAdded.trigger(guid);
return viewpoint;
}
retrieve(guid: string) {
return this.list.find((v) => v.guid === guid);
}
async view(guid: string) {
const viewpoint = this.retrieve(guid);
if (!viewpoint) {
return;
}
// #region Recover annotations
if (this._drawManager && viewpoint.annotations) {
this._drawManager.viewport.clear();
this._drawManager.enabled = true;
this._drawManager.viewport.get().append(viewpoint.annotations);
}
// #endregion
// #region Recover dimensions
const dimensionsComponent = this.components.tools.get(LengthMeasurement);
viewpoint.dimensions.forEach((data) => {
const dimension = new SimpleDimensionLine(this.components, {
start: data.start,
end: data.end,
// @ts-ignore
lineMaterial: dimensionsComponent._lineMaterial,
// @ts-ignore
endpoint: dimensionsComponent._endpointMesh,
});
dimension.createBoundingBox();
// @ts-ignore
dimensionsComponent._dimensions.push(dimension);
});
// #endregion
// #region Recover filtered elements
// if (viewpoint.filter) {
// const filterData = fragments.groups.get(viewpoint.filter)
// for (const fragmentID in fragments.list) {
// const fragment = fragments.list[fragmentID]
// fragment.setVisibility(fragment.items, false)
// }
// for (const fragmentID in filterData) {
// const ids = filterData[fragmentID]
// fragments.list[fragmentID]?.setVisibility(ids, true)
// }
// }
// #endregion
// Select elements in the viewpoint
const selection: { [fragmentID: string]: Set<string> } = {};
for (const fragmentID in viewpoint.selection) {
selection[fragmentID] = viewpoint.selection[fragmentID];
}
const highlighter = await this.components.tools.get(FragmentHighlighter);
await highlighter.highlightByID(this.selectionHighlighter, selection, true);
// #region Recover camera position & target
const camera = this.components.camera as OrthoPerspectiveCamera;
const controls = camera.controls;
controls.setLookAt(
viewpoint.position.x,
viewpoint.position.y,
viewpoint.position.z,
viewpoint.target.x,
viewpoint.target.y,
viewpoint.target.z,
true
);
await this.onViewpointViewed.trigger(guid);
// #endregion
}
} |
@startuml
Title <size:20>\nPattern: Factory Method\n
interface LoggerFactory {
+ createLogger(): Logger
}
class FileLoggerFactory implements LoggerFactory {
- filePath
+ __construct()
+ createLogger(): Logger
}
class StdoutLoggerFactory implements LoggerFactory{
+ createLogger(): Logger
}
interface Logger {
+ log()
}
class FileLogger implements Logger {
- filePath
+ __construct()
+ log(): void
}
class StdoutLogger implements Logger {
+ log(): string
}
LoggerFactory <-left- Logger: returns
@enduml |
import axios from 'axios'
import React, { useEffect, useState } from 'react'
import { Link } from 'react-router-dom'
import {Swiper , SwiperSlide} from 'swiper/react'
import {Navigation} from 'swiper/modules'
import SwiperCore from 'swiper'
import 'swiper/css/bundle'
import ListingItem from '../components/ListingItem'
export default function Home() {
const [offerListings,setOfferListings] = useState([])
const [saleListings,setSaleListings] = useState([])
const [rentListings,setRentListings] = useState([])
SwiperCore.use([Navigation])
// console.log(saleListings);
useEffect(() => {
const fetchOfferListings = async () =>{
try {
await axios.get('/api/getListings?offer=true&limit=4').then((res) =>{
setOfferListings(res.data)
fetchRentListings()
})
} catch (error) {
console.log(error);
}
}
const fetchRentListings = async () => {
try {
await axios.get('/api/getListings?type=rent&limit=4').then((res) =>{
setRentListings(res.data)
fetchSaleListings()
})
} catch (error) {
console.log(error);
}
}
const fetchSaleListings = async () => {
try {
await axios.get('/api/getListings?type=sale&limit=4').then((res) =>{
setSaleListings(res.data)
})
} catch (error) {
console.log(error);
}
}
fetchOfferListings()
},[])
return (
<div>
{/* top */}
<div className="flex flex-col gap-6 p-28 px-3 max-w-6xl mx-auto">
<h1 className='text-slate-700 font-bold text-3xl lg:text-6xl'>
Find your next <span className='text-slate-500'>perfect</span> <br /> place with ease
</h1>
<div className="text-gray-400 text-xs sm:text-sm">
Adii Estate will help you find your home fast, easy and comfortable. <br />
Our expect support are always available.
</div>
<Link to={'/search'} className='text-xs sm:text-sm text-blue-800 font-bold hover:underline'>
Let's Start now...
</Link>
</div>
{/* swiper */}
<Swiper navigation>
{
offerListings && offerListings.length > 0 &&
offerListings.map((listing) =>(
<SwiperSlide>
<div style={{background:`url(${listing.imageUrls[0]}) center no-repeat`, backgroundSize:'cover'}} className="h-[500px]" key={listing._id}></div>
</SwiperSlide>
))
}
</Swiper>
{/* listing results for offer, sale and rent */}
<div className="max-w-6xl mx-auto p-3 flex flex-col gap-8 my-10">
{
offerListings && offerListings.length > 0 && (
<div className="">
<div className="my-3">
<h2 className='text-2xl font-semibold text-slate-600'>Recent Offers</h2>
<Link className='text-sm text-blue-800 hover:underline' to={'/search?offer=true'}>
Show more offers
</Link>
</div>
<div className="flex flex-wrap gap-4">
{
offerListings.map((listing) => (
<ListingItem listing={listing} key={listing._id}></ListingItem>
))
}
</div>
</div>
)
}
{
rentListings && rentListings.length > 0 && (
<div className="">
<div className="my-3">
<h2 className='text-2xl font-semibold text-slate-600'>Recent places for rent</h2>
<Link className='text-sm text-blue-800 hover:underline' to={'/search?type=rent'}>
Show more places for rent
</Link>
</div>
<div className="flex flex-wrap gap-4">
{
rentListings.map((listing) => (
<ListingItem listing={listing} key={listing._id}></ListingItem>
))
}
</div>
</div>
)
}
{
saleListings && saleListings.length > 0 && (
<div className="">
<div className="my-3">
<h2 className='text-2xl font-semibold text-slate-600'>Recent places for sale</h2>
<Link className='text-sm text-blue-800 hover:underline' to={'/search?type=sale'}>
Show more places for sale
</Link>
</div>
<div className="flex flex-wrap gap-4">
{
saleListings.map((listing) => (
<ListingItem listing={listing} key={listing._id}></ListingItem>
))
}
</div>
</div>
)
}
</div>
</div>
)
} |
from typing import Literal
import os
import numpy as np
import scipy
import polars as pl
import torch
import elsa
import faiss
import sentence_transformers
import adm.files
import adm.params
import adm.settings
def make_sparse_interactions_matrix(*, train_test: Literal['train', 'test'] | None) -> scipy.sparse.csr_matrix:
t = adm.files.read_train(train_test).drop('Query')
max_user = t[['User']].max().item() + 1
max_item = t[['Item']].max().item() + 1
t = t.sort(['User', 'Item'])
csr = scipy.sparse.csr_matrix(
(
np.ones(t.shape[0], dtype=np.float32),
(t['User'], t['Item'])
),
shape=(max_user, max_item),
dtype=np.float32
)
return csr
def make_sparse_interactions_matrix_coo(*, train_test: Literal['train', 'test'] | None) -> scipy.sparse.coo_matrix:
t = adm.files.read_train(train_test).drop('Query')
max_user = t[['User']].max().item() + 1
max_item = t[['Item']].max().item() + 1
t = t.sort(['User', 'Item'])
coo = scipy.sparse.coo_matrix(
(
np.ones(t.shape[0], dtype=np.float32),
(t['User'], t['Item'])
),
shape=(max_user, max_item),
dtype=np.float32
)
return coo
def train_elsa(*, params: adm.params.ElsaParams) -> str:
save_path = f'{adm.settings.MODELS_FOLDER}/{adm.params.params_to_name("elsa", params)}.pt'
print(save_path)
if os.path.exists(save_path):
print('- ELSA model already exists')
return save_path
n_dims, batch_size, epochs = params['n_dims'], params['batch_size'], params['epochs']
m = make_sparse_interactions_matrix(train_test='train' if adm.settings.TRAIN_TEST else None)
_, items_cnt = m.shape
model = elsa.ELSA(n_items=items_cnt, device=adm.settings.DEVICE_TORCH, n_dims=n_dims)
model.compile()
model.fit(m, batch_size=batch_size, epochs=epochs)
torch.save(model.state_dict(), save_path)
return save_path
def load_elsa(*, params: adm.params.ElsaParams, train_test: Literal['train', 'test'] | None) -> elsa.ELSA:
_, items_cnt = make_sparse_interactions_matrix(train_test=train_test).shape
model = elsa.ELSA(n_items=items_cnt, device=adm.settings.DEVICE_TORCH, n_dims=params['n_dims'])
model.compile()
model.load_state_dict(torch.load(f'{adm.settings.MODELS_FOLDER}/{adm.params.params_to_name("elsa", params)}.pt'))
return model
def encode_sentences_train(*, params: adm.params.EncoderParams, train_test: Literal['train', 'test'] | None) -> str:
save_path = f'{adm.settings.MODELS_FOLDER}/{adm.params.params_to_name("encode_sentences_train" + ((train_test.upper() + str(adm.settings.TRAIN_RATIO)) if type(train_test) == str else ""), params)}.npy'
if os.path.exists(save_path):
print(' - Encoded sentences train already exists')
return save_path
model, batch_size = params['model'], params['batch_size']
q = adm.files.read_train(train_test)['Query'].unique(maintain_order=True).to_numpy()
model = sentence_transformers.SentenceTransformer(model, device=adm.settings.DEVICE_TORCH, trust_remote_code=True)
encoded = model.encode(q, device=adm.settings.DEVICE_TORCH, show_progress_bar=True, batch_size=batch_size)
torch.save(encoded, save_path)
return save_path
def load_encoded_sentences_train(
*,
params: adm.params.EncoderParams,
train_test: Literal['train', 'test'] | None,
) -> tuple[pl.DataFrame, torch.Tensor]:
df_train = adm.files.read_train(train_test)
queries = df_train['Query'].unique(maintain_order=True)
query_to_id = {q: i for i, q in enumerate(queries)}
df = df_train.group_by(
(
('Query', 'User') if train_test == 'test' else 'Query'
),
maintain_order=True
).agg(
pl.col('Item').first().alias('_item')
if train_test == 'test' else
pl.col('Item').value_counts(sort=True).struct[0].alias('_items')
).with_columns(
pl.col('Query').replace(query_to_id, return_dtype=pl.Int32).alias('_q_index')
).drop('Query')
encoded = torch.load(
f'{adm.settings.MODELS_FOLDER}/{adm.params.params_to_name("encode_sentences_train" + ((train_test.upper() + str(adm.settings.TRAIN_RATIO)) if type(train_test) == str else ""), params)}.npy'
)
return df, encoded
def encode_sentences_batch(*, params: adm.params.EncoderParams, batch_id: int) -> str:
save_path = f'{adm.settings.MODELS_FOLDER}/{adm.params.params_to_name("encode_sentences_batch_" + str(batch_id), params)}.npy'
if os.path.exists(save_path):
print(f'- Encoded sentences test {batch_id} already exists')
return save_path
model, batch_size = params['model'], params['batch_size']
q: pl.DataFrame
match batch_id:
case 1:
q = adm.files.read_b1()
case 2:
q = adm.files.read_b2()
case 3:
q = adm.files.read_bfinal()
case _:
raise ValueError(f'Got unexpected value batch_id: {batch_id}')
model = sentence_transformers.SentenceTransformer(model, device=adm.settings.DEVICE_TORCH, trust_remote_code=True)
encoded = model.encode(q['Query'].to_numpy(), device=adm.settings.DEVICE_TORCH, show_progress_bar=True, batch_size=batch_size)
torch.save(encoded, save_path)
return save_path
def load_encoded_sentences_batch(*, params: adm.params.EncoderParams, batch_id: int) -> tuple[pl.Series, torch.Tensor]:
match batch_id:
case 1:
q = adm.files.read_b1()
case 2:
q = adm.files.read_b2()
case 3:
q = adm.files.read_bfinal()
case _:
raise ValueError(f'Got unexpected value batch_id: {batch_id}')
encoded = torch.load(f'{adm.settings.MODELS_FOLDER}/{adm.params.params_to_name("encode_sentences_batch_" + str(batch_id), params)}.npy')
return q['User'], encoded
def build_faiss_index(*, df_embeddings: tuple[pl.DataFrame, torch.Tensor], encoder_params: adm.params.EncoderParams) -> str:
save_path = f'{adm.settings.MODELS_FOLDER}/{adm.params.params_to_name("faiss", encoder_params)}.npy'
if os.path.exists(save_path):
print('- Faiss index already exists')
return save_path
res = faiss.StandardGpuResources()
_, embeddings = df_embeddings
index = faiss.IndexIDMap(faiss.IndexFlatIP(embeddings.shape[1]))
gpu_index = faiss.index_cpu_to_gpu(res, 0, index)
faiss.normalize_L2(embeddings)
gpu_index.add_with_ids(embeddings, np.arange(embeddings.shape[0]))
faiss.write_index(faiss.index_gpu_to_cpu(gpu_index), save_path)
return save_path
def load_faiss_index(*, encoder_params: adm.params.EncoderParams) -> faiss.Index:
res = faiss.StandardGpuResources()
index = faiss.read_index(f'{adm.settings.MODELS_FOLDER}/{adm.params.params_to_name("faiss", encoder_params)}.npy')
return faiss.index_cpu_to_gpu(res, 0, index)
def scipy_sparse_to_torch_sparse(scipy_csr_matrix: scipy.sparse.csr_matrix) -> torch.cuda.FloatTensor:
data = scipy_csr_matrix.data
indices = scipy_csr_matrix.indices
indptr = scipy_csr_matrix.indptr
torch_data = torch.tensor(data, dtype=torch.float32)
torch_indices = torch.tensor(indices, dtype=torch.int32)
torch_indptr = torch.tensor(indptr, dtype=torch.int32)
torch_sparse_csr_matrix = torch.sparse_csr_tensor(
torch_indptr,
torch_indices,
torch_data,
size=scipy_csr_matrix.shape,
device=adm.settings.DEVICE_TORCH,
)
return torch_sparse_csr_matrix |
import React from 'react';
import { render } from '@utils/TestHelper';
import Button from '@components/common/Button/index';
import DefaultColor from '@themes/DefaultColor';
import { Platform, View } from 'react-native';
import _ from 'lodash';
import Touchable, { containerStyleKeys } from '@components/common/Button/Touchable';
import Color from 'color';
import TouchableOpacity from '@components/common/Button/TouchableOpacity';
import TouchableWithoutFeedback from '@components/common/Button/TouchableWithoutFeedback';
import AppView from '@utils/AppView';
let mockTheme = DefaultColor;
jest.mock('@react-navigation/native', () => ({
...jest.requireActual('@react-navigation/native'),
useTheme: jest.fn().mockImplementation(() => mockTheme),
}));
beforeEach(() => {
mockTheme = DefaultColor;
Platform.OS = 'ios';
});
test('should render correctly', () => {
const { toJSON, getByTestId } = render(<Button />);
expect(toJSON()).toMatchSnapshot();
expect(getByTestId('TouchableView')).toBeTruthy();
});
test('should have working props', () => {
const RootComponent = (
<Button className="flex-1 flex-row self-center justify-between items-center rounded border mx-1 my-1 p-1 border-sky-700 bg-sky-500" />
);
const { getByTestId, rerender } = render(RootComponent);
const style = {
flexGrow: 1,
flexShrink: 1,
flexDirection: 'row',
alignSelf: 'center',
justifyContent: 'space-between',
alignItems: 'center',
borderBottomLeftRadius: 4,
borderBottomRightRadius: 4,
borderTopLeftRadius: 4,
borderTopRightRadius: 4,
borderLeftWidth: 1,
borderRightWidth: 1,
borderTopWidth: 1,
borderBottomWidth: 1,
borderLeftColor: 'rgba(3, 105, 161, 1)',
borderRightColor: 'rgba(3, 105, 161, 1)',
borderTopColor: 'rgba(3, 105, 161, 1)',
borderBottomColor: 'rgba(3, 105, 161, 1)',
backgroundColor: 'rgba(14, 165, 233, 1)',
marginLeft: 4,
marginRight: 4,
marginTop: 4,
marginBottom: 4,
paddingLeft: 4,
paddingRight: 4,
paddingTop: 4,
paddingBottom: 4,
};
let containerStyle = _.pick(style, containerStyleKeys[Platform.OS]);
let otherStyle = _.omit(style, containerStyleKeys[Platform.OS]);
expect(getByTestId('ContainerView')).toHaveStyle(containerStyle);
expect(getByTestId('TouchableView')).toHaveStyle(otherStyle);
/**
* Shadow Button
*/
const { getByTestId: getByTestIdShadow } = render(<Button style={AppView.shadow(5)} />);
expect(getByTestIdShadow('ContainerView')).toHaveStyle({ elevation: 5 });
expect(getByTestIdShadow('TouchableView')).toHaveStyle({
shadowColor: '#000',
// shadowOffset: { height: 2, width: 0 },
shadowOpacity: 0.25,
shadowRadius: 3.84,
});
/**
* Outline Button
*/
const { getByTestId: getByTestIdOutline } = render(<Button outline />);
expect(getByTestIdOutline('ContainerView')).toHaveStyle({ elevation: 0 });
expect(getByTestIdOutline('TouchableView')).toHaveStyle({
backgroundColor: 'transparent',
borderWidth: 1,
});
/**
* Clear Button
*/
const { getByTestId: getByTestIdClear } = render(<Button clear />);
expect(getByTestIdClear('ContainerView')).toHaveStyle({ elevation: 0 });
expect(getByTestIdClear('TouchableView')).toHaveStyle({
backgroundColor: 'transparent',
borderWidth: undefined,
});
/**
* Icon Button
*/
rerender(
<Button
leftIconProps={{
type: 'material-community',
name: 'arrow-left',
}}
rightIconProps={{
type: 'material-community',
name: 'arrow-right',
}}
/>,
);
expect(getByTestId('LeftIcon')).toBeTruthy();
expect(getByTestId('RightIcon')).toBeTruthy();
/**
* Loading Button
*/
rerender(<Button loading title="Loading Button" />);
expect(getByTestId('Loading')).toBeTruthy();
expect(getByTestId('ButtonTitleText')).toHaveStyle({ opacity: 0 });
/**
* Disabled Button
*/
rerender(<Button disabled title="Disabled Button" />);
expect(getByTestId('ButtonTitleText')).toHaveStyle({
color: Color(mockTheme.colors.grey3).lighten(0.2).hex(),
});
expect(getByTestId('TouchableView')).toHaveStyle({
backgroundColor: mockTheme.colors.grey5,
});
/**
* Android
*/
Platform.OS = 'android';
const { getByTestId: getByTestIdAndroid } = render(RootComponent);
containerStyle = _.pick(style, containerStyleKeys[Platform.OS]);
otherStyle = _.omit(style, containerStyleKeys[Platform.OS]);
expect(getByTestIdAndroid('ContainerView')).toHaveStyle(containerStyle);
expect(getByTestIdAndroid('TouchableView')).toHaveStyle(otherStyle);
});
test('should have working props [Touchable]', () => {
const RootComponent = (
<Touchable className="flex-1 flex-row self-center justify-between items-center rounded border mx-1 my-1 p-1 border-sky-700 bg-sky-500" />
);
const { getByTestId } = render(RootComponent);
const style = {
flexGrow: 1,
flexShrink: 1,
flexDirection: 'row',
alignSelf: 'center',
justifyContent: 'space-between',
alignItems: 'center',
borderBottomLeftRadius: 4,
borderBottomRightRadius: 4,
borderTopLeftRadius: 4,
borderTopRightRadius: 4,
borderLeftWidth: 1,
borderRightWidth: 1,
borderTopWidth: 1,
borderBottomWidth: 1,
borderLeftColor: 'rgba(3, 105, 161, 1)',
borderRightColor: 'rgba(3, 105, 161, 1)',
borderTopColor: 'rgba(3, 105, 161, 1)',
borderBottomColor: 'rgba(3, 105, 161, 1)',
backgroundColor: 'rgba(14, 165, 233, 1)',
marginLeft: 4,
marginRight: 4,
marginTop: 4,
marginBottom: 4,
paddingLeft: 4,
paddingRight: 4,
paddingTop: 4,
paddingBottom: 4,
};
const containerStyle = _.pick(style, containerStyleKeys[Platform.OS]);
const otherStyle = _.omit(style, containerStyleKeys[Platform.OS]);
expect(getByTestId('ContainerView')).toHaveStyle(containerStyle);
expect(getByTestId('TouchableView')).toHaveStyle(otherStyle);
/**
* Android
*/
Platform.OS = 'android';
Platform.Version = 31;
const { getByTestId: getByTestIdAndroid } = render(<Touchable className="w-1 h-1 border" />);
expect(getByTestIdAndroid('ContainerView')).toHaveStyle({ width: 5, height: 5 });
});
test('should have working props [TouchableOpacity]', () => {
const RootComponent = (
<TouchableOpacity className="flex-1 flex-row self-center justify-between items-center rounded border mx-1 my-1 p-1 border-sky-700 bg-sky-500" />
);
const { getByTestId } = render(RootComponent);
expect(getByTestId('TouchableOpacity')).toHaveStyle({
flexGrow: 1,
flexShrink: 1,
flexDirection: 'row',
alignSelf: 'center',
justifyContent: 'space-between',
alignItems: 'center',
borderBottomLeftRadius: 4,
borderBottomRightRadius: 4,
borderTopLeftRadius: 4,
borderTopRightRadius: 4,
borderLeftWidth: 1,
borderRightWidth: 1,
borderTopWidth: 1,
borderBottomWidth: 1,
borderLeftColor: 'rgba(3, 105, 161, 1)',
borderRightColor: 'rgba(3, 105, 161, 1)',
borderTopColor: 'rgba(3, 105, 161, 1)',
borderBottomColor: 'rgba(3, 105, 161, 1)',
backgroundColor: 'rgba(14, 165, 233, 1)',
marginLeft: 4,
marginRight: 4,
marginTop: 4,
marginBottom: 4,
paddingLeft: 4,
paddingRight: 4,
paddingTop: 4,
paddingBottom: 4,
});
});
test('should have working props [TouchableWithoutFeedback]', () => {
const RootComponent = (
<TouchableWithoutFeedback className="flex-1 flex-row self-center justify-between items-center rounded border mx-1 my-1 p-1 border-sky-700 bg-sky-500">
<View />
</TouchableWithoutFeedback>
);
const { queryByTestId } = render(RootComponent);
expect(queryByTestId('TouchableWithoutFeedback')?.props.style).toBeFalsy();
}); |
import { useState } from 'react';
import {useNavigate} from "react-router-dom";
function RegistrationPage(){
var navigate = useNavigate()
const [data,setData] = useState({
username:"",
password:"",
firstname:"",
lastname:"",
re_password : "",
});
const {username, password, firstname, lastname, re_password} = data;
const changeHandler = e =>{
setData({...data,[e.target.name]:e.target.value});
}
const sendRegistrationInfo = (event)=>{
event.preventDefault()
fetch('http://localhost:8000/register', {
method: 'POST',
mode: 'cors',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
"username" : username,
"password" : password,
"firstname" : firstname,
"lastname" : lastname
})
})
.then(response => {
response.json().then((response_body) => {
navigate("/login")
})
})
.catch(error => {
console.error('There was an error!', error);
});
}
return (
<div>
<form className = "registerform" onSubmit={sendRegistrationInfo}>
<label>
User Name: <br />
<input type="text" placeholder="Enter your email" name="username" value = {username} onChange={changeHandler} /><br />
</label>
<label>
First Name: <br />
<input type="text" placeholder="First Name" name="firstname" value = {firstname} onChange={changeHandler} /><br />
</label>
<label>
Last Name: <br />
<input type="text" placeholder="Last Name" name="lastname" value = {lastname} onChange={changeHandler} /><br />
</label>
<label>
Password: <br />
<input type="password" placeholder="Password" name="password" value = {password} onChange={changeHandler} /><br />
</label>
<label>
Re Enter Password: <br />
<input type="password" placeholder="Re Enter Password" name="re_password" value = {re_password} onChange={changeHandler} />
</label>
<br />
<br />
<input type="submit"/>
</form>
</div>
)
}
export default RegistrationPage |
#include "board.h"
#include "piece.h"
#include "player.h"
#include <string>
#include <fstream>
#include <iostream>
#include <QMouseEvent>
#include <QPainter>
#include <QPointF>
#include <QImage>
#include <QPainterPath>
#include <QColor>
#include <QList>
#include <QFileDialog>
#include <QDebug>
#include <locale>
Board::Board(QWidget *parent)
: QWidget{parent}, boardSize(QSize(500,500)), imageOffset(QPoint(0,0)), movingPiece(nullptr)
{
std::setlocale(LC_NUMERIC, "en_US.UTF-8");
//QString fileName = QFileDialog::getOpenFileName(this, tr("Open File"), QDir::currentPath());
QString fileName = "../Board_Config/Classic_Nine_Men_Morris_corrected.txt";
get_pieces_nodes_edges_from_file(fileName.toStdString());
spacePieces = QList<Piece*>(relativeSpaces.size(), nullptr);
setMinimumSize(boardSize);
player[0] = new Player(num_black_pieces, QColor(0, 0, 0), this);
player[1] = new Player(num_white_pieces, QColor(255, 255, 255), this);
connect(player[0], &Player::endTurn, player[1], &Player::startTurn);
connect(player[1], &Player::endTurn, player[0], &Player::startTurn);
connect(player[0], &Player::eliminationStarts, player[1], &Player::becomesAttacked);
connect(player[1], &Player::eliminationStarts, player[0], &Player::becomesAttacked);
connect(player[0], &Player::pieceRemoved, player[1], &Player::finishElimination);
connect(player[1], &Player::pieceRemoved, player[0], &Player::finishElimination);
player[0]->startTurn();
}
void Board::mousePressEvent(QMouseEvent* event)
{
qDebug() << "Board";
}
void Board::drawBoard()
{
image.fill(qRgb(200, 200, 120));
QPainter painter(&image);
QList<QPoint>::iterator space;
QPainterPath circles;
QColor myPenColor = Qt::blue;
int myPenWidth = 4;
int spaceRadius = 10;
painter.setPen(QPen(myPenColor, myPenWidth, Qt::SolidLine, Qt::RoundCap, Qt::RoundJoin));
for (space = pixelSpaces.begin(); space != pixelSpaces.end(); space++){
circles.addEllipse(*space, spaceRadius, spaceRadius);
}
painter.fillPath(circles, Qt::blue);
for (int edge = 0; edge < edges.size(); edge++){
painter.drawLine(pixelSpaces[edges[edge][0]], pixelSpaces[edges[edge][1]]);
}
}
void Board::resizeNodes(int factor_x, int factor_y)
{
if (pixelSpaces.isEmpty()){
for (size_t ind = 0; ind < relativeSpaces.size(); ind++){
pixelSpaces.append(QPoint(0,0));
}
}
for (size_t ind = 0 ; ind < relativeSpaces.size(); ind++){
pixelSpaces[ind].setX( static_cast<int>(relativeSpaces[ind].x()*factor_x + imageOffset.x()));
pixelSpaces[ind].setY( static_cast<int>(relativeSpaces[ind].y()*factor_y + imageOffset.y()));
}
}
void Board::paintEvent(QPaintEvent *event)
{
QPainter painter(this);
QRect dirtyRect = event->rect();
painter.drawImage(dirtyRect, image, dirtyRect);
}
void Board::resizeEvent(QResizeEvent *event)
{
const QSize newSize = event->size();
image = QImage(newSize, QImage::Format_RGB32);
const int scaleFactor = qMin(newSize.width(), newSize.height());
boardSize.setWidth(scaleFactor);
boardSize.setHeight(scaleFactor);
imageOffset = QPoint((newSize.width() - boardSize.width())/2,
(newSize.height() - boardSize.height())/2);
resizeNodes(scaleFactor, scaleFactor);
drawBoard();
QWidget::resizeEvent(event);
emit isResized(scaleFactor, scaleFactor);
}
void Board::get_pieces_nodes_edges_from_file(const std::string file_dir)
{
std::ifstream stream(file_dir);
if (!stream) {return; } // To complete in case of file error
std::string line;
std::string key;
std::size_t index;
bool node_list = false;
bool edge_list = false;
while (std::getline(stream, line))
{
if (edge_list){
edges.append(extract_edges(line));
}
if (node_list){
if (line.find("EDGES") != std::string::npos){
node_list = false;
edge_list = true;
continue;
}
relativeSpaces.append(extract_coordinates(line));
}
if (extract_player_pieces(line, "BLACK_PIECES", num_black_pieces)) continue;
if (extract_player_pieces(line, "WHITE_PIECES", num_white_pieces)) continue;
if (line.find("SPACES") != std::string::npos){
node_list = true;
continue;
}
}
}
QPointF Board::extract_coordinates(const std::string line)
{
const std::size_t index1 = line.find(',');
const std::size_t index2 = line.find(',', index1 + 1);
const float x = std::stof(line.substr(index1+1, index2-index1-1));
const float y = std::stof(line.substr(index2+1));
return QPointF(x, y);
}
QList<int> Board::extract_edges(const std::string line)
{
const std::size_t index1 = line.find(',');
const std::size_t index2 = line.find(',', index1 + 1);
QList<int> node_list;
node_list.append(std::stoi(line.substr(index1+1, index2-index1-1)));
node_list.append(std::stoi(line.substr(index2+1)));
return node_list;
}
bool Board::extract_player_pieces(const std::string& line, const std::string& key, int& num_pieces)
{
std::size_t index;
char const* digits = "0123456789";
index = line.find(key);
if (index != std::string::npos){
index = line.find_first_of(digits);
num_pieces = std::stoi(line.substr(index));
return true;
}
return false;
}
void Board::pickUpPiece(Piece* pickedPiece)
{
movingPiece = pickedPiece;
}
void Board::fillSpace(Piece* piece, const size_t spaceInd)
{
const qsizetype oldInd = spacePieces.indexOf(piece);
if (oldInd != -1)
{
spacePieces[oldInd] = nullptr;
}
spacePieces[spaceInd] = piece;
releasePiece();
}
Piece* Board::pieceInSpace(size_t ind) const
{
if ((ind < 0) || (ind >= spacePieces.size())) {return nullptr;}
return spacePieces.at(ind);
}
void Board::mouseMoveEvent(QMouseEvent *event)
{
if (movingPiece)
movingPiece->movePiece(event->pos());
}
void Board::mouseReleaseEvent(QMouseEvent *event)
{
}
QPoint Board::getSpacePoint(size_t spaceInd) const
{
if ((spaceInd < 0) || spaceInd >= pixelSpaces.size()) return QPoint(0,0);
return pixelSpaces[spaceInd];
}
void Board::releasePiece()
{
movingPiece = nullptr;
}
void Board::removePiece(Piece* removedPiece, size_t spaceInd)
{
qDebug() << "Start Piece removal in Board";
if (spacePieces[spaceInd] == removedPiece)
spacePieces[spaceInd] = nullptr;
qDebug() << "Ended Piece removal in Board";
} |
import { ImageList, ImageListItem } from '@mui/material';
import React from 'react';
import { RootStateOrAny, useSelector, useDispatch } from 'react-redux';
import { Link } from 'react-router-dom';
import SearchForm from '../../component/searchComp/SearchForm';
import { searchQuery } from '../../redux/slice';
type ResultGif = {
id: string;
url: string;
title: string;
images: {
original: {
url: string;
};
};
};
type ResultGifs = ResultGif[];
function Search() {
const { REACT_APP_GIPHY_API_KEY } = process.env;
const [searchResults, setSearchResults] = React.useState<ResultGifs>([]);
const query = useSelector((state: RootStateOrAny) => state.search.value);
const dispatch = useDispatch();
const getGif = (event: React.FormEvent<HTMLFormElement>) => {
event.preventDefault();
fetch(
`https://api.giphy.com/v1/gifs/search?api_key=${REACT_APP_GIPHY_API_KEY}&q=${query}&limit=20`
)
.then((response) => response.json())
.then((result) => {
setSearchResults(result.data);
});
};
return (
<>
<Link to="/trending">Trending Gif</Link>
<h1>Giphy Search</h1>
<SearchForm
data-testid="search-form"
onChange={(e: React.ChangeEvent<HTMLInputElement>) =>
dispatch(searchQuery(e.target.value))
}
onSubmit={getGif}
/>
<div className="container">
<ImageList
sx={{ width: '80%', height: '100%' }}
variant="woven"
cols={3}
gap={8}
>
{searchResults.map((gif) => (
<ImageListItem key={gif.id}>
<img
src={`${gif.images.original.url}?w=161&fit=crop&auto=format`}
srcSet={`${gif.images.original.url}?w=161&fit=crop&auto=format&dpr=2 2x`}
alt={gif.title}
loading="lazy"
/>
</ImageListItem>
))}
</ImageList>
</div>
</>
);
}
export default Search; |
import React, { useState, useEffect } from "react";
import { Col, Row, Radio, Space, Collapse } from "antd";
import "../..//style/pay-form-contact.scss";
import { useContext } from "react";
import { CustomerContext } from "../../../../providers/CustomerContext";
import { creditCards } from "./credit-cards.js";
import FormCreditCard from "./form-credit-card/FormCreditCard";
import { useDispatch, useSelector } from "react-redux";
import { DownOutlined } from "@ant-design/icons";
import { postBookingAction } from "../../../../stores/slices/postBooking.slice";
const { Panel } = Collapse;
function PayMethodSection() {
const bookingInfoState = useSelector(
(state) => state.bookingInfo.bookingInfoState
);
const dispatch = useDispatch();
const { orderInfo, setOrderInfo, currentPay, setCurrentPay } =
useContext(CustomerContext);
const [payMethod, setPayMethod] = useState("Credit/Debit Card");
const [ellipsisCancel, setEllipsisCancel] = useState(false);
useEffect(() => {
let newPayMethod = {
method: payMethod,
payInfo: {
cardNumber: "",
nameOnCard: "",
},
};
setOrderInfo({ ...orderInfo, payment: newPayMethod });
localStorage.setItem(
"ORDER_INFO",
JSON.stringify({ ...orderInfo, payment: newPayMethod })
);
}, [payMethod]);
const onAddUserInfoPay = () => {
dispatch(postBookingAction(orderInfo));
setCurrentPay(currentPay + 1);
};
return (
<div className="pay-form-contact">
<h2>Pay with?</h2>
<Radio.Group
onChange={(e) => setPayMethod(e.target.value)}
value={payMethod}
>
<Space direction="vertical">
<Radio value="Cash">
<h6>Cash</h6>
{payMethod === "Cash" ? "Pay directly at the hotel" : null}
</Radio>
<Radio value="Credit/Debit Card">
<Row>
<Col span={12}>
<h6>Credit/Debit Card </h6>
</Col>
<Col span={12}>
<div className="pay-cards">
{creditCards.map((card) => (
<div key={card.name}>
<img src={card.imageUrl} alt="" />
</div>
))}
</div>
</Col>
</Row>
{payMethod === "Credit/Debit Card" ? <FormCreditCard /> : null}
</Radio>
</Space>
</Radio.Group>
<Collapse
defaultActiveKey={["1"]}
bordered={false}
expandIcon={({ isActive }) => (
<DownOutlined rotate={isActive ? 180 : 0} />
)}
expandIconPosition="end"
className="pay-method"
>
<Panel header="Cancellation policy" key="1">
<div className="">
<div
className={ellipsisCancel ? "" : "ellipsis-text"}
onClick={() => {
setEllipsisCancel(!ellipsisCancel);
}}
>
<p>
Room rate book on standard rate: Any cancellation received
within 7 days prior to arrival date will incur the full period
charge. Failure to arrive at your hotel or property will be
treated as a No-Show and no refund will be given
</p>
<p>
Room rate book under promotion: Any cancellation received within
14 days prior to arrival date will incur the full period charge.
Failure to arrive at your hotel or property will be treated as a
No-Show and no refund will be given
</p>
<p>
No-showed booking will be full charged for whole booking for
full length stay
</p>
</div>
<div
style={{
marginTop: "10px",
cursor: "pointer",
color: "#005e84",
}}
onClick={() => {
setEllipsisCancel(!ellipsisCancel);
}}
>
{ellipsisCancel ? "Read less" : "Read more"}
</div>
</div>
</Panel>
</Collapse>
<p style={{ color: "#999" }}>
By proceeding with this booking, I agree to{" "}
<span
style={{
color: "#066a92",
cursor: "pointer",
textDecoration: "underline",
}}
>
{" "}
Terms of Use
</span>{" "}
and{" "}
<span
style={{
color: "#066a92",
cursor: "pointer",
textDecoration: "underline",
}}
>
Privacy Policy
</span>
.
</p>
<div className="pay-submit-contact">
<button
type="submit"
onClick={onAddUserInfoPay}
className="booking-btn"
>
Book
</button>
</div>
</div>
);
}
export default PayMethodSection; |
<!DOCTYPE html>
<html>
<head>
<title>Bakery</title>
<link rel="icon" type="image/x-icon" href="assets/images/thebakery.png">
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name="format-detection" content="telephone=no">
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="author" content="">
<meta name="keywords" content="">
<meta name="description" content="">
<link rel="stylesheet" type="text/css"
href="assets/fonts/icomoon/icomoon.css">
<link rel="stylesheet" type="text/css" href="assets/css/slick.css" />
<link rel="stylesheet" type="text/css" href="assets/css/slick-theme.css" />
<link rel="stylesheet" type="text/css"
href="assets/css/magnific-popup.css" />
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm" crossorigin="anonymous">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com">
<link
href="https://fonts.googleapis.com/css2?family=Merriweather:ital,wght@0,400;0,700;1,400;1,700&display=swap"
rel="stylesheet">
<link rel="stylesheet" type="text/css"
href="assets/css/bootstrap.min.css" />
<link rel="stylesheet" type="text/css" href="assets/css/style.css">
<script src="//code.jquery.com/jquery-1.11.1.min.js"></script>
</head>
<body>
<div class="header-top-menu bg-black color-secondary">
<div class="container">
<div class="row">
<div class="wrap flex-container padding-small">
<div class="header-top-right">
<ul class="list-unstyled d-flex color-secondary">
<li class="pr-50"><a href="/login-page">Профил</a></li>
<li id="create" class="pr-50"><a href="/create-product">Създай продукт</a></li>
<li id="categories" class="pr-50"><a href="/categories-page">Категории</a></li>
<li class="pr-50"><a href="/admin">Виж продукти</a></li>
</ul>
</div>
</div>
<!--flex-container-->
</div>
</div>
</div>
<!----top-menu---->
<section class="our-team bg-sand padding-large">
<div class="container">
<div class="input-group mb-3">
<input type="text" class="form-control" id="search" onkeyup="filterProducts()" placeholder="Search..." aria-label="Search..." aria-describedby="basic-addon2">
<div class="input-group-append">
<span class="input-group-text" id="basic-addon2">Search</span>
</div>
</div>
<label for="name" >Търси по критерии:</label>
<div class="input-group mb-3">
<label for="name" >Име:</label>
<input type="text" id="name" class="form-control ml-3" aria-label="Text input with segmented dropdown button">
</div>
<!-- <div class="input-group mb-3">
<select name="categoryId" class="custom-select">
<option selected value="0">Избери...</option>
</select>
<div class="input-group-append">
<label class="input-group-text" for="inputGroupSelect02">Категория</label>
</div>
</div>
-->
<div class="input-group mb-3">
<label for="description" >Описание:</label>
<input type="text" id="description" class="form-control ml-3" aria-label="Text input with segmented dropdown button">
<div class="input-group-append">
<button type="button" class="input-group-text m-2" onclick="getFilteredProducts()">Търси</button>
</div>
</div>
<div class="row">
<div id="products-list"></div>
</div>
</div>
</section>
<!-- product-item col-md-3 pb-4 -->
<li class="list-group-item" id="cloneMe" style="display:none;">
<a href="update-product.html" src="images/cake-item3.jpg" class="product-image"><img></a>
<div class="text-comtent text-center">
<h5 class="pt-4">
<a href="/update-product">Black Forest Pastry</a>
</h5>
<p>Cake - Pastries</p>
<span class="price colored" id="price">$12</span>
</div>
<div class="col-sm-3 col-xs-3">
<button type="button" class="btn btn-danger pull-right remove-post">
<span class="glyphicon glyphicon-remove"></span><span
class="hidden-xs"> Изтрий</span>
</button>
</div>
</li>
</body>
<script type="text/javascript">
getAllProducts();
getAllCategories();
function getAllProducts() {
$.ajax({
url : "/products",
method : "GET",
success : function(data) {
console.log(JSON.parse(JSON.stringify(data)));
data.forEach(function(product) {
console.log(JSON.parse(JSON.stringify(product)));
addProductToUI(product.description, product.name,
product.price, product.quantity,
product.photosImagePath, product.id);
});
}
});
}
function addProductToUI(description, name, price, quantity, image, id) {
var product = $('#cloneMe').clone();
product.find('h5').text(name);
product.find('p').text(description);
product.find('#price').text(price + "лв.");
product.find('img').attr('src', image);
product.find('h5').click(function() {
localStorage.setItem('productId', id);
window.location.href = "update-product.html";
});
product.find('button').click(function() {
if(confirm("Искате ли да изтриете продукта?")) {
deleteProduct(id, product);
}
});
$('#products-list').prepend(product);
product.show();
}
function deleteProduct(id, element) {
$.ajax({
url : "/products/" + id,
method : "DELETE",
data : {
id : id
},
dataType : "json",
complete : function(data) {
switch (data.status) {
case 200:
element.remove();
break;
case 401:
windows.location.href = "index.html";
break;
case 404:
alert("The product was not found.");
break;
}
}
});
}
function filterProducts() {
var input, filter, ul, singleProductView, a, i, txtValue;
input = document.getElementById('search');
filter = input.value.toUpperCase();
list = $('#products-list')[0];
singleProductView = list.getElementsByTagName("li");
// Loop through all list items, and hide those who don't match the search query
for (i = 0; i < singleProductView.length; i++) {
a = singleProductView[i];
txtValue = a.textContent || a.innerText;
if (txtValue.toUpperCase().indexOf(filter) > -1) {
singleProductView[i].style.display = "";
} else {
singleProductView[i].style.display = "none";
}
}
}
function getFilteredProducts() {
var a;
list = $('#products-list')[0];
singleProductView = list.getElementsByTagName("li");
for (i = 0; i < singleProductView.length; i++) {
singleProductView[i].style.display = "none";
}
$.ajax({
url : "/products/filter",
method : "GET",
data: {
name: $('#name').val(),
description: $('#description').val(),
categoryId: document.querySelector("[name=categoryId]").value
},
success : function(data) {
data.forEach(function(product) {
console.log(JSON.parse(JSON.stringify(product)));
addProductToUI(product.description, product.name,
product.price, product.quantity,
product.photosImagePath, product.id);
});
}
});
}
/* function getAllCategories() {
$.ajax({
url : "/categories",
method : "GET",
success : function(data) {
data.forEach(function(category) {
const select = document.querySelector("[name=categoryId]"); // or give it an ID and use document.getElementById("req_one")
select.options[select.options.length] = new Option(
category.name, category.id);
});
}
});
};
*/
</script>
</html> |
import DeleteIcon from "@mui/icons-material/Delete";
import {
Box,
Button,
Container,
IconButton,
List,
ListItem,
ListItemText,
Typography,
} from "@mui/material";
import { useCallback, useEffect, useState } from "react";
import { useAlert } from "../../hooks/useAlert";
import axios from "../../services/axios";
import { Error as MoveError } from "../../types";
import MoveErrorCreate from "./MoveErrorCreate";
const MoveErrorList = () => {
const [moveErrors, setMoveErrors] = useState<MoveError[]>(null!);
const { showError, showSuccess } = useAlert();
const [open, setOpen] = useState(false);
const url = "/dashboard/error";
const handleOpen = () => {
setOpen(true);
};
const handleClose = () => {
setOpen(false);
};
const handleDelete = async (id: number) => {
try {
await axios.delete(`${url}/${id}`);
showSuccess("Movimiento de entrenamiento eliminado correctamente");
fetchMoveErrors();
} catch (error) {
console.error(error);
showError("Hubo un error al eliminar el movimiento de entrenamiento");
}
};
const fetchMoveErrors = useCallback(async () => {
const { data } = await axios.get(url);
setMoveErrors(data.data);
}, []);
useEffect(() => {
fetchMoveErrors().catch((error) => {
console.error(error);
showError("Hubo un error al cargar los movimientos de entrenamiento");
});
}, []);
return (
<Container component="main" maxWidth="sm">
<Box py={{ xs: 2, lg: 4 }}>
<Box
sx={{
display: "flex",
flexDirection: "row",
justifyContent: "space-between",
flexWrap: "wrap",
}}
>
<Typography variant="h1" alignSelf="start">
Movimiento de entrenamiento
</Typography>
<Button variant="contained" onClick={handleOpen}>
Crear nuevo
</Button>
</Box>
<List sx={{ mt: 1 }}>
{moveErrors?.map((moveError) => (
<ListItem
key={moveError.errorID}
disablePadding
secondaryAction={
<IconButton
aria-label="delete"
onClick={() => handleDelete(moveError.errorID)}
>
<DeleteIcon />
</IconButton>
}
>
<ListItemText primary={`${moveError.name}`} />
</ListItem>
))}
</List>
{/* TODO: Add pagination */}
</Box>
<MoveErrorCreate
open={open}
handleClose={handleClose}
fetchMoveErrors={fetchMoveErrors}
/>
</Container>
);
};
export default MoveErrorList; |
/* ************************************************************************** */
/* */
/* ::: :::::::: */
/* ft_substr.c :+: :+: :+: */
/* +:+ +:+ +:+ */
/* By: asoledad <[email protected]> +#+ +:+ +#+ */
/* +#+#+#+#+#+ +#+ */
/* Created: 2021/10/25 17:26:11 by asoledad #+# #+# */
/* Updated: 2021/11/13 19:16:31 by asoledad ### ########.fr */
/* */
/* ************************************************************************** */
#include "libft.h"
static size_t s_lenght(const char *s)
{
size_t n;
n = 0;
while (s[n])
n++;
return (n);
}
char *ft_substr(char const *s, size_t start, size_t len)
{
size_t n;
char *sub;
n = 0;
if (!s)
return ((void *) 0);
sub = (char *)malloc(len + 1);
if (!sub)
return ((void *) 0);
if (start >= s_lenght(s))
{
while (n < len)
sub[n++] = '\0';
}
else
{
while (n < len)
sub[n++] = s[start++];
}
sub[n] = '\0';
return (sub);
} |
import SwiftUI
// MARK: -
/// SplitView の Detail View
///
/// Detail View 側のナビゲーションスタックの管理を行う
struct ContentDetailView: View {
/// 詳細画面のNavigationPath
@State private var detailPath = NavigationPath()
/// 表示する値
let markdown: Markdown
/// 水平サイズクラス
let horizontal: UserInterfaceSizeClass?
/// アクセントカラー( ナビゲーションスタックにスタックされるごとに変更する )
@Binding var accentColor: Color?
var body: some View {
NavigationStack(path: $detailPath) {
// Root
detail(markdown: markdown)
}
.navigationDestination(for: Markdown.self) { markdown in
// Destination
detail(markdown: markdown)
}
}
func detail(markdown: Markdown) -> some View {
ProposalDetailView(path: $detailPath, markdown: markdown)
.onChange(of: accentColor(markdown), initial: true) { _, color in
accentColor = color
}
}
func accentColor(_ markdown: Markdown) -> Color {
markdown.proposal.state?.color ?? .darkText
}
}
#if DEBUG
#Preview {
PreviewContainer {
ContentDetailView(
markdown: .fake0418,
horizontal: .compact,
accentColor: .constant(.green)
)
}
}
#endif |
import { IsNumber, IsNotEmpty, IsString } from "class-validator";
export class CreateSaleDto {
@IsNotEmpty()
sales_id: number;
@IsNumber()
@IsNotEmpty()
customer_id: number;
@IsString()
@IsNotEmpty()
code: string;
@IsNumber()
shipping_cost: number;
@IsString()
shipping_info: string;
@IsNumber()
other_cost: number;
@IsString()
other_info: string;
@IsString()
payment_method: string;
@IsString()
status: string;
@IsNumber()
total: number;
@IsNotEmpty()
@IsNumber()
product_id: number;
@IsNotEmpty()
@IsNumber()
product_quantity: number;
} |
import PropTypes from 'prop-types'
import React from 'react'
import { connect } from 'react-redux'
import { fetchAllLists, reorderList, previewListOrder } from '../../actions/list'
import { moveListItem } from '../../actions/list-item'
import { getActiveListID } from '../../reducers/activeList'
import { getSortedLists, getAllListsFetchStatus, getallListsErrorStatus, getListInitialOrders } from '../../reducers/allLists'
import ListLink from './ListSwitcher/ListLink.jsx'
import AddList from './ListSwitcher/AddList.jsx'
import { ErrorPanel } from './Shared/ErrorPanel.jsx'
import { LoadingIndicator } from './Shared/LoadingIndicator.jsx'
class ListSwitcher extends React.Component {
constructor(props) {
super(props)
this.setNewOrder = this.setNewOrder.bind(this)
this.setListID = this.setListID.bind(this)
this.previewNewOrder = this.previewNewOrder.bind(this)
}
componentDidMount() {
// Load initial data from backend once components mounts.
const { fetchAllLists } = this.props
fetchAllLists()
}
render() {
const { isFetching, sortedLists, activeListID, allListsError, initialOrders } = this.props
if (allListsError.isError) {
return (
<ErrorPanel show={allListsError.isError}>
The list of lists could not be retrieved. Error message: <em>{allListsError.errorMessage}</em>
</ErrorPanel>
)
}
if (isFetching) {
return (
<LoadingIndicator
isFetching={true}
type='bars'
height='10%'
width='10%'
className='mx-auto d-block pt-5'
/>
)
} else {
return (
<div>
<AddList />
{sortedLists.map(item =>
<ListLink
key={item.id}
initialOrder={initialOrders[item.id]}
{...item}
activeList={item.id == activeListID ? true : false}
activeListID={activeListID}
setNewOrder={this.setNewOrder}
previewNewOrder={this.previewNewOrder}
setListID={this.setListID}
/>
)}
</div>
)
}
}
setNewOrder(id, order, initialOrder) {
const { reorderList } = this.props
reorderList(id, order, initialOrder)
}
setListID(id, listID, initialOrder) {
const { moveListItem } = this.props
moveListItem(id, listID, initialOrder)
}
previewNewOrder(dragID, dropOrder) {
const { previewListOrder } = this.props
previewListOrder(dragID, dropOrder)
}
}
const mapStateToProps = (state) => ({
initialOrders: getListInitialOrders(state),
isFetching: getAllListsFetchStatus(state),
sortedLists: getSortedLists(state),
allListsError: getallListsErrorStatus(state),
activeListID: getActiveListID(state)
})
ListSwitcher.propTypes = {
initialOrders: PropTypes.object.isRequired,
isFetching: PropTypes.bool.isRequired,
sortedLists: PropTypes.array.isRequired,
activeListID: PropTypes.number,
fetchAllLists: PropTypes.func.isRequired,
allListsError: PropTypes.object.isRequired,
reorderList: PropTypes.func.isRequired,
moveListItem: PropTypes.func.isRequired,
previewListOrder: PropTypes.func.isRequired
}
ListSwitcher = connect(
mapStateToProps,
{ fetchAllLists, reorderList, moveListItem, previewListOrder }
)(ListSwitcher)
export default ListSwitcher |
import React, { useEffect, useState } from "react";
import RateComponent from "./RateComponent/Rate";
import InputField from "./InputFieldComponent/InputField";
import CommentButton from "./Button/CommentButton";
import TextArea from "./TextArea/TextArea";
import axios from "axios";
import "./styles/AddComment.css"
const EditComment = () => {
const [email, setEmail] = useState("");
const [comment, setComment] = useState("");
const [stars, setStars] = useState(0);
const [likes, setLikes] = useState(0);
const [emailError, setEmailError] = useState({ state: false, message: "" });
const [commentError, setCommentError] = useState({
state: false,
message: "",
});
const getReview = (id) => {
axios
.get(`http://localhost:5000/api/comments/${id}`)
.then((res) => {
console.log(res.data);
setEmail(res.data.userEmail);
setStars(res.data.noOfStars);
setComment(res.data.comment);
setLikes(res.data.likes);
})
.catch((e) => {
console.error(e.message);
});
}
useEffect(() => {
getReview(window.location.pathname.split("/")[4]);
console.log(stars)
}, [])
const handleEditComment = (id) => {
if (comment === "") {
setCommentError({ state: true, message: "You must fill this field" });
}
if (email === "") {
setEmailError({ state: true, message: "You must fill this field" });
} else {
if (
!!!email.match(
/^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9-]+(?:\.[a-zA-Z0-9-]+)*$/
)
) {
setEmailError({ state: true, message: "Please enter a valid email" });
}
}
if (
comment !== "" &&
email !== "" &&
!!email.match(
/^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9-]+(?:\.[a-zA-Z0-9-]+)*$/
)
) {
setCommentError({ message: "", state: false });
setEmailError({ message: "", state: false });
const payload = {
noOfStars: stars,
comment: comment,
userEmail: email,
userPNumber: "000003",
userImage: "",
likes: likes,
roomID: window.location.pathname.split("/")[3]
};
axios
.put(`http://localhost:5000/api/comments/${id}`, payload)
.then((_res) => {
alert("Updated!");
})
.catch((e) => console.error(e.message));
}
};
return (
<div className="ac-background">
<div>
<RateComponent initValue={stars} onClick={(value) => setStars(value)} size="large" />
</div>
<div className="ac-form">
<InputField
error={emailError.state}
value = {email}
helperText={emailError.state && emailError.message}
onChange={(text) => setEmail(text)}
label="e-mail address"
/>
<TextArea
error={commentError.state}
value={comment}
helperText={commentError.state && commentError.message}
onChange={(text) => setComment(text)}
/>
<CommentButton
onClick={() => handleEditComment(window.location.pathname.split("/")[4])}
size="small"
variant="contained"
label="Edit Comment"
/>
</div>
</div>
);
};
export default EditComment; |
class Editor {
// Websocket
private _ws = null
private _wsActive = false
private _localStorageConfigs = 'OpenVRNotificationPipe.BOLL7708.Config'
private _localStorageConfigNames = 'OpenVRNotificationPipe.BOLL7708.ConfigNames'
private _localStorageLastConfigName = 'OpenVRNotificationPipe.BOLL7708.LastConfigName'
// Construct forms
private _formSubmit = document.querySelector<HTMLFormElement>('#formSubmit')
private _formConfig = document.querySelector<HTMLFormElement>('#formConfig')
private _formImage = document.querySelector<HTMLFormElement>('#formImage')
private _formProperties = document.querySelector<HTMLFormElement>('#formProperties')
private _formFollow = document.querySelector<HTMLFormElement>('#formFollow')
private _templateAnimation = document.querySelector<HTMLTemplateElement>('#templateAnimation')
private _formAnimation1 = document.querySelector<HTMLFormElement>('#formAnimation1')
private _formAnimation2 = document.querySelector<HTMLFormElement>('#formAnimation2')
private _formAnimation3 = document.querySelector<HTMLFormElement>('#formAnimation3')
private _templateTransition = document.querySelector<HTMLTemplateElement>('#templateTransition')
private _formTransitionIn = document.querySelector<HTMLFormElement>('#formTransitionIn')
private _formTransitionOut = document.querySelector<HTMLFormElement>('#formTransitionOut')
private _formTextarea = document.querySelector<HTMLFormElement>('#formTextarea')
private _templateTween = document.querySelector<HTMLTemplateElement>('#templateTween')
// General elements
private _submit = document.querySelector<HTMLButtonElement>('#submit')
private _configName: HTMLInputElement
private _configList: HTMLSelectElement
private _loadConfig = document.querySelector<HTMLButtonElement>('#loadConfig')
private _saveConfig = document.querySelector<HTMLButtonElement>('#saveConfig')
private _deleteConfig = document.querySelector<HTMLButtonElement>('#deleteConfig')
// File elements
private _imgData = null;
private _image = document.querySelector<HTMLImageElement>('#image')
private _file = document.querySelector<HTMLInputElement>('#file')
// Config elements
private _config = document.querySelector<HTMLTextAreaElement>('#config')
private _copyJSON = document.querySelector<HTMLButtonElement>('#copyJSON')
private _downloadJSON = document.querySelector<HTMLButtonElement>('#downloadJSON')
private _copyJS = document.querySelector<HTMLButtonElement>('#copyJS')
private _downloadJS = document.querySelector<HTMLButtonElement>('#downloadJS')
// Program loop
init()
{
// Key listener
document.onkeyup = function (e: KeyboardEvent) {
switch(e.code) {
case 'NumpadEnter':
case 'Enter':
this.sendNotification.call(this, e)
break
default:
// console.log(e.code)
break
}
}.bind(this)
// Clone forms from templates
this._formFollow.appendChild(this._templateTween.content.cloneNode(true))
this._formAnimation1.appendChild(this._templateAnimation.content.cloneNode(true))
this._formAnimation2.appendChild(this._templateAnimation.content.cloneNode(true))
this._formAnimation3.appendChild(this._templateAnimation.content.cloneNode(true))
this._formTransitionIn.appendChild(this._templateTransition.content.cloneNode(true))
this._formTransitionIn.appendChild(this._templateTween.content.cloneNode(true))
this._formTransitionOut.appendChild(this._templateTransition.content.cloneNode(true))
this._formTransitionOut.appendChild(this._templateTween.content.cloneNode(true))
// Modify the DOM by adding labels and renaming IDs
document.querySelectorAll('input, select, canvas').forEach(el => {
// ID
const form = el.parentElement
const name = (<HTMLFormElement> el).name ?? el.id
const id = `${form.id}-${name}`
el.id = id
// Wrapping + label
const wrapper = document.createElement('p')
el.parentNode.insertBefore(wrapper, el)
const label = document.createElement('label')
label.setAttribute('for', id)
label.innerHTML = name.toLowerCase() == 'tweentype'
? `<a href="https://easings.net/" target="_blank">${name}</a>`
: `${name}:`
wrapper.appendChild(label)
wrapper.appendChild(el)
})
// Add event listeners
this._submit.addEventListener('click', this.sendNotification.bind(this))
this._loadConfig.addEventListener('click', this.loadConfig.bind(this))
this._saveConfig.addEventListener('click', this.saveConfig.bind(this))
this._deleteConfig.addEventListener('click', this.deleteConfig.bind(this))
this._file.addEventListener('change', this.readImage.bind(this))
this._copyJSON.addEventListener('click', this.copyConfigJSON.bind(this))
this._downloadJSON.addEventListener('click', this.downloadConfigJSON.bind(this))
this._copyJS.addEventListener('click', this.copyConfigJS.bind(this))
this._downloadJS.addEventListener('click', this.downloadConfigJS.bind(this))
this._configName = document.querySelector<HTMLInputElement>('#formConfig-configName')
this._configList = document.querySelector<HTMLSelectElement>('#formConfig-configList')
this._configList.addEventListener('change', this.setCurrentConfigName.bind(this))
this._configList.addEventListener('focus', (e: Event)=> { this._configList.selectedIndex = -1 })
this.loadConfigNames()
this.connectLoop()
}
connectLoop()
{
if(!this._wsActive) {
const url = new URL(window.location.href)
const params = new URLSearchParams(url.search)
const port = params.get('port') ?? 8077
// TODO: Save port in local data
var wsUri = `ws://localhost:${port}`
this._wsActive = true
if(this._ws != null) this._ws.close()
try {
this._ws = new WebSocket(wsUri)
this._ws.onopen = function(evt) {
this._wsActive = true
document.title = 'Pipe Editor - CONNECTED'
this._submit.disabled = false
}.bind(this)
this._ws.onclose = function(evt) {
this._wsActive = false
document.title = 'Pipe Editor - DISCONNECTED'
this._submit.disabled = true
}.bind(this)
this._ws.onmessage = function(evt) {
const data = JSON.parse(evt.data)
console.log(data)
}.bind(this)
this._ws.onerror = function(evt) {
this._wsActive = false
console.error(`WebSocket Connection Error`)
document.title = `Pipe Editor - ERROR`
}.bind(this)
} catch (error) {
console.error(`WebSocket Init Error: ${error}`)
this._wsActive = false
document.title = `Pipe Editor - ERROR`
}
}
setTimeout(this.connectLoop.bind(this), 5000);
}
// Image functions
readImage() {
this._formImage.classList.remove('unset')
const files = this._file.files
if(files && files[0]) {
var FR = new FileReader()
FR.onload = function(e: ProgressEvent) {
this._image.src = FR.result
this._imgData = FR.result.toString().split(',')[1]
}.bind(this)
FR.readAsDataURL( files[0] )
}
}
getImageData() {
return this._imgData
}
getImagePath() {
return 'C:/replace/with/path/on/disk/'+(this._file.files[0]?.name ?? 'image.png')
}
// Data
sendNotification(e: Event) {
e?.preventDefault()
const data = this.getData()
// Send data
this._ws.send(JSON.stringify(data))
}
getData() {
const data = {
// General
imageData: this.getImageData(),
imagePath: this.getImagePath(),
// Standard
basicTitle: "",
basicMessage: "",
// Custom
customProperties: {}
}
// Get all form data
const propertiesData = new FormData(this._formProperties)
const propertiesJSON = Object.fromEntries(propertiesData.entries())
propertiesJSON.anchorType = propertiesData.getAll('anchorType').pop()
const followData = new FormData(this._formFollow)
const followJSON = Object.fromEntries(followData.entries())
followJSON.tweenType = followData.getAll('tweenType').pop()
const animationData1 = new FormData(this._formAnimation1)
const animationJSON1 = Object.fromEntries(animationData1.entries())
animationJSON1.property = animationData1.getAll('property').pop()
animationJSON1.phase = animationData1.getAll('phase').pop()
animationJSON1.waveform = animationData1.getAll('waveform').pop()
const animationData2 = new FormData(this._formAnimation2)
const animationJSON2 = Object.fromEntries(animationData2.entries())
animationJSON2.property = animationData2.getAll('property').pop()
animationJSON2.phase = animationData2.getAll('phase').pop()
animationJSON2.waveform = animationData2.getAll('waveform').pop()
const animationData3 = new FormData(this._formAnimation3)
const animationJSON3 = Object.fromEntries(animationData3.entries())
animationJSON3.property = animationData3.getAll('property').pop()
animationJSON3.phase = animationData3.getAll('phase').pop()
animationJSON3.waveform = animationData3.getAll('waveform').pop()
const transitionsInData = new FormData(this._formTransitionIn)
const transitionsInJSON = Object.fromEntries(transitionsInData.entries())
transitionsInJSON.tweenType = transitionsInData.getAll('tweenType').pop()
const transitionsOutData = new FormData(this._formTransitionOut)
const transitionsOutJSON = Object.fromEntries(transitionsOutData.entries())
transitionsOutJSON.tweenType = transitionsOutData.getAll('tweenType').pop()
const textareaData = new FormData(this._formTextarea)
const textareaJSON = Object.fromEntries(textareaData.entries())
textareaJSON.horizontalAlignment = textareaData.getAll('horizontalAlignment').pop()
textareaJSON.verticalAlignment = textareaData.getAll('verticalAlignment').pop()
// Fill data
data.customProperties = propertiesJSON
data.customProperties['follow'] = {}
if(followJSON.enabled) data.customProperties['follow'] = followJSON
data.customProperties['animations'] = []
if(animationJSON1.property != '0') data.customProperties['animations'].push(animationJSON1)
if(animationJSON2.property != '0') data.customProperties['animations'].push(animationJSON2)
if(animationJSON3.property != '0') data.customProperties['animations'].push(animationJSON3)
data.customProperties['transitions'] = []
data.customProperties['transitions'].push(transitionsInJSON)
data.customProperties['transitions'].push(transitionsOutJSON)
data.customProperties['textAreas'] = []
if(textareaJSON.text.toString().length > 0) data.customProperties['textAreas'].push(textareaJSON)
return data
}
copyConfigJSON(e: Event) {
e?.preventDefault()
const data = this.getData()
data.imageData = ''
this._config.innerHTML = JSON.stringify(data, null, 4)
this._config.select()
document.execCommand('copy')
}
downloadConfigJSON(e: Event) {
e?.preventDefault()
const data = this.getData()
data.imageData = ''
const json = JSON.stringify(data, null, 4)
this.download(json, 'pipe-config.json', 'text/plain')
}
copyConfigJS(e: Event) {
e?.preventDefault()
const data = this.getData()
data.imageData = ''
this._config.innerHTML = this.renderJS(data, null, 0)
this._config.select()
document.execCommand('copy')
}
downloadConfigJS(e: Event) {
e?.preventDefault()
const data = this.getData()
data.imageData = ''
const json = this.renderJS(data, null, 0)
this.download(json, 'pipe-config.js', 'text/plain')
}
// Function to download data to a file https://stackoverflow.com/a/30832210
download(data: string, filename: string, type: string) {
const file = new Blob([data], {type: type});
const a = document.createElement("a")
const url = URL.createObjectURL(file)
a.href = url
a.download = filename
document.body.appendChild(a)
a.click()
setTimeout(function() {
document.body.removeChild(a)
window.URL.revokeObjectURL(url)
}, 0)
}
loadConfig(e: Event) {
e?.preventDefault()
const configName = this._configName.value ?? ''
if(configName.length > 0) {
const json = window.localStorage.getItem(this.getConfigKey(configName))
window.localStorage.setItem(this._localStorageLastConfigName, configName)
const data = JSON.parse(json)
if(data == null) return alert(`Config "${configName}" not found`)
const properties = data.customProperties ?? {}
const follow = data.customProperties.follow ?? {}
const animation1 = data.customProperties.animations[0] ?? {}
const animation2 = data.customProperties.animations[1] ?? {}
const animation3 = data.customProperties.animations[2] ?? {}
const transitionIn = data.customProperties.transitions[0] ?? {}
const transitionOut = data.customProperties.transitions[1] ?? {}
const textarea = data.customProperties.textAreas[0] ?? {}
applyDataToForm(this._formProperties, properties)
applyDataToForm(this._formFollow, follow)
applyDataToForm(this._formAnimation1, animation1)
applyDataToForm(this._formAnimation2, animation2)
applyDataToForm(this._formAnimation3, animation3)
applyDataToForm(this._formTransitionIn, transitionIn)
applyDataToForm(this._formTransitionOut, transitionOut)
applyDataToForm(this._formTextarea, textarea)
function applyDataToForm(form, data) {
for(const key in data) {
const id = `${form.id}-${key}`
const el = document.querySelector(`#${id}`)
if(typeof data[key] != 'object') {
if(el['type'] == 'checkbox') {
el['checked'] = data[key] ?? ''
} else {
el['value'] = data[key]
}
}
}
}
alert(`Config "${configName} loaded"`)
} else {
alert('Please enter a name for your config')
}
}
saveConfig(e: Event) {
e?.preventDefault()
const configName = this._configName.value
if(configName.length > 0) {
const data = JSON.stringify(this.getData())
window.localStorage.setItem(this.getConfigKey(configName), data)
window.localStorage.setItem(this._localStorageLastConfigName, configName)
this.saveConfigName(configName)
alert(`Config "${configName}" saved`)
} else {
alert('Please enter a name for your config')
}
}
deleteConfig(e: Event) {
e?.preventDefault()
const configName = this._configName.value
if(configName.length > 0) {
const doIt = confirm(`Are you sure you want to delete config "${configName}"?`)
if(doIt) {
window.localStorage.removeItem(this.getConfigKey(configName))
window.localStorage.setItem(this._localStorageLastConfigName, '')
this.deleteConfigName(configName)
alert(`Config "${configName}" deleted`)
}
} else {
alert('Please enter a name for your config')
}
}
setCurrentConfigName(e: Event) {
e?.preventDefault()
const name = this._configList.value
this._configName.value = name
window.localStorage.setItem(this._localStorageLastConfigName, name)
}
loadConfigNames() {
const configNames = window.localStorage.getItem(this._localStorageConfigNames)
const lastConfigName = window.localStorage.getItem(this._localStorageLastConfigName)
const names = JSON.parse(configNames) ?? ['']
this._configName.value = lastConfigName ?? ''
this.applyConfigNamesToSelect(names, lastConfigName)
}
saveConfigName(name: string) {
const namesData = window.localStorage.getItem(this._localStorageConfigNames)
const names = JSON.parse(namesData) ?? []
if(!names.includes(name)) names.push(name)
window.localStorage.setItem(this._localStorageConfigNames, JSON.stringify(names))
this.applyConfigNamesToSelect(names, name)
}
deleteConfigName(name: string) {
const namesData = window.localStorage.getItem(this._localStorageConfigNames)
let names = JSON.parse(namesData) ?? []
if(names.includes(name)) delete names[names.indexOf(name)]
names = names.filter(n => n != null)
window.localStorage.setItem(this._localStorageConfigNames, JSON.stringify(names))
this.applyConfigNamesToSelect(names, name)
this._configName.value = ''
}
applyConfigNamesToSelect(names: string[], selected: string = null) {
this._configList.innerHTML = ''
for(const name of names) {
const option = document.createElement('option')
option.value = name
option.innerText = name
if(name == selected) option.selected = true
this._configList.appendChild(option)
}
}
getConfigKey(name: string): string {
return `${this._localStorageConfigs}-${name}`
}
getIndentationString(count: number, size: number) {
return count == 0
? ''
: new Array(count+1).join(
size == 0
? ''
: new Array(size+1).join(' ')
)
}
renderJS(value: any, key: string, indentCount: number) {
const indentStr = this.getIndentationString(indentCount, 4)
const keyStr = key == null
? ''
: key.includes('-')
? `'${key}': `
: `${key}: `
let result = ""
if(value === null || typeof value == 'undefined') {
result += `${indentStr}${keyStr}null`
} else if(Array.isArray(value)) {
result += `${indentStr}${keyStr}[`
const resultArr = []
for(const v of value) {
resultArr.push(this.renderJS(v, null, indentCount+1))
}
result += resultArr.length == 0
? ']'
: `\n${resultArr.join(',\n')}${indentStr}\n${indentStr}]`
} else {
const floatValue = parseFloat(value)
const boolValue = value == 'true'
? true
: value == 'false'
? false
: null
if(!isNaN(floatValue)) {
result += `${indentStr}${keyStr}${parseFloat(value)}`
} else if (boolValue !== null) {
result += `${indentStr}${keyStr}${value ? 'true' : 'false'}`
} else if(typeof value == 'string') {
result += `${indentStr}${keyStr}'${value}'`
} else if(typeof value == 'object') {
result += `${indentStr}${keyStr}{`
const resultArr = []
for (const [p, val] of Object.entries(value)) {
resultArr.push(this.renderJS(val, p, indentCount+1))
}
result += resultArr.length == 0
? '}'
: `\n${resultArr.join(',\n')}${indentStr}\n${indentStr}}`
} else {
result += `${indentStr}${keyStr}undefined`
}
}
return result
}
} |
import axios from 'axios'
import React, { useEffect, useState } from 'react'
import Navbar from '../commonComponent/Navbar'
import Scroll from '../commonComponent/Scroll'
export default function Get_list() {
const url = "https://jsonplaceholder.typicode.com/users"
const [list, setList] = useState([])
console.log('list', list)
useEffect(() => {
userList()
}, [])
const userList = () => {
axios.get(url).then((res) => {
setList(res?.data)
})
}
const [search, setsearch] = useState("")
const searchBox = (event) => {
setsearch(event.target.value)
}
return (
<>
<Navbar />
<div class="row">
<div class="col-lg-3">
<Scroll />
</div>
<div class="col-lg-9">
<input type="text" id='search-box' onChange={searchBox} placeholder="SEARCH HERE" />
<div className='view-table'>
<table className='table table-success table-striped-columns'>
<thead>
<tr>
<th>ID</th>
<th>name </th>
<th>usename </th>
<th>email</th>
<th>phone</th>
<th>Website</th>
<th>Address</th>
</tr>
</thead>
<tbody>
{
list.filter((val) => {
if(search===""){
return val
}
else if (val.name.toLowerCase().includes(search.toLocaleLowerCase())) {
return val;
}
}).map((data, index) => {
console.log("datatattttt", data)
return (
<tr>
<td>{data?.id}</td>
<td>{data?.name}</td>
<td>{data?.username}</td>
<td>{data?.email}</td>
<td>{data?.phone}</td>
<td>{data?.website}</td>
<td> {data?.address?.street}{" "}{data?.address?.zipcode}{""}{data?.address?.city}</td>
</tr>
)
})}
</tbody>
</table>
</div>
</div>
</div>
</>
)
} |
from dotenv import load_dotenv
load_dotenv()
import streamlit as st
import os
import sqlite3
import google.generativeai as genai
## Configuring my Genai Key
genai.configure(api_key=os.getenv("GOOGLE_API_KEY"))
## This is the function To Load Google Gemini Model and provide queries as response
def get_gemini_response(question,prompt):
model=genai.GenerativeModel('gemini-pro')
response=model.generate_content([prompt[0],question])
return response.text
##This is the fucntion To retrieve query from the database
def read_sql_query(sql,db):
conn=sqlite3.connect(db)
cur=conn.cursor()
cur.execute(sql)
rows=cur.fetchall()
conn.commit()
conn.close()
for row in rows:
print(row)
return rows
prompt=[
"""
You are an expert in converting English questions to SQL query!
The SQL database has the name food and has the following tables inside it
named "Users", "Restaurant", "Orders", "OrderItems","DeliveryDriver","Delivery"
with their respected columns
SECTION \n\nFor example,\nExample 1 - How many entries of records are present in Orders?,
the SQL command will be something like this SELECT COUNT(*) FROM Orders ;
\nExample 2 -Names of customers who have placed orders at restaurants that
serveItalian cuisine , the SQL command will be something like this SELECT Name
FROM Users
WHERE UserID IN (
SELECT DISTINCT UserID
FROM Orders
WHERE RestaurantID IN (
SELECT RestaurantID
FROM Restaurant
WHERE CuisineType = 'Italian'
)
);
like this you need to obtain sql queries for some of the nested,Corelated nested,groupby,having and joins type of Queries also
also the sql code should not have ``` in beginning or end and sql word in output
"""
]
#This is the UI part which was done using Streamlit
st.set_page_config(page_title="I can Retrieve Any SQL query")
st.header("SQL Database Data Retriever")
st.markdown("""
<style>
body {
color: black;
background-color: white;
}
.sidebar .sidebar-content {
background-color: #f8f9fa;
color: black;
}
</style>
""", unsafe_allow_html=True)
question=st.text_input("Input: ",key="input")
submit=st.button("Ask the question")
# if submit button is clicked
if submit:
response=get_gemini_response(question,prompt)
print(response)
response=read_sql_query(response,"food.db")
st.subheader("The Response is")
for row in response:
print(row)
st.header(row) |
#include "Form.hpp"
Form::Form() : Name(""), GradeSign(0), GradeExec(0){
this->SignedStatus = false;
}
Form::Form(std::string Name, int sign, int exec) : Name(Name), GradeSign(sign), GradeExec(exec){
if(GradeSign < 1 || GradeExec < 1)
throw GradeTooHighException();
if(GradeSign > 150 || GradeExec > 150)
throw GradeTooLowException();
this->SignedStatus = false;
}
Form::Form(Form const & f) : Name(f.Name), GradeSign(f.GradeSign), GradeExec(f.GradeExec){
*this = f;
}
Form & Form::operator=(Form const & f){
if(this != & f){
this->SignedStatus = f.SignedStatus;
}
return *this;
}
Form::~Form(){}
std::string Form::get_name() const{
return this->Name;
}
bool Form::get_sign_status() const{
return this->SignedStatus;
}
int Form::get_grade_sign() const{
return this->GradeSign;
}
int Form::get_grade_exec() const{
return this->GradeExec;
}
void Form::beSigned(Bureaucrat & b){
if(b.getGrade() <= this->get_grade_sign())
this->SignedStatus = true;
else{
throw GradeTooLowException();
}
}
const char * Form::GradeTooHighException::what() const throw(){
return "grade too high";
}
const char * Form::GradeTooLowException::what() const throw(){
return "grade too low";
}
std::ostream & operator<< (std::ostream & out, Form const & f){
if(f.get_grade_sign()){
out << " Name : " << f.get_name() << " SigneGrade : " << f.get_grade_sign() << " ExecuteGrade : " << f.get_grade_exec() << " Form is signed\n";
}
else{
out << " Name : " << f.get_name() << " SigneGrade : " << f.get_grade_sign() << " ExecuteGrade : " << f.get_grade_exec() << " Form is not signed\n";
}
return out;
} |
Please answer the below core-java Questions:
What is programing language?
A. A programming language is a formal language, which comprises a set of instructions used to produce various kinds of output. Programming languages are used in computer programming to create programs that implement specific algorithms.
What jdk contains or composed of and define each components?
A. The JDK is a software package that contains a variety of tools and utilities that make it possible to develop, package, monitor and deploy applications that build for any standard Java platform, including Java Platform, Standard Edition (Java SE); Java Platform, Micro Edition (Java ME); and Java Platform, Enterprise Edition
javac: This utility is used to compile Java source code into Java bytecode.
rmic: This utility creates skeletons and stubs for use in Remote Method Invocation (RMI).
jar: This compression utility aggregates a multitude of files into a single Java ARchive (JAR) file. The jar utility uses a standard compression algorithm used by all of the most common zip utilities.
javadoc: This utility can examine the names of classes and the methods contained within a class, as well as consume special annotations in order to create application programming interface (API) documentation for Java code.
wsgen: This generates various artifacts required by Java API for XML Web Services (JAX-WS).
javap: This utility disassembles class files, generating information about the methods, properties and attributes of a given compiled component.
What is IDE?
A. An integrated development environment (IDE) is a software suite that consolidates basic tools required to write and test software.
What are the IDEs available in the market to support java? Net beans, intelli j, Eclipse, BlueJ,
Explain the entire java life cycle. Using text editor like IntelliJ we create source files (extension .java) which is then compiled using the java compiler
to create bytecode or class file (extension .class). The class file then loads into the JVM using java loader. Finally,
JVM reads the bytecode
in memory and translate them into machine code for us to see the output.
what is class? Class is a blueprint of an object.
What is object? An object is an instance of a class.
What is the entry point of an application? An application entry point identifies a resource that is an access point to an application. Application entry points are used to control users' access to different versions of an application that is deployed on a platform.
Why main is static? This is neccessary because main() is called by the JVM before any objects are made. Since it is static it can be directly invoked via the class.
Which class is the superclass of all classes? java.lang.Object class is superclass of all classes.
What is difference between path and classpath variables?
Path refers to the system while classpath refers to the Developing Environment.
What is the difference between an Interface and Abstract class?
- Interface: In interface we cannot declare a body. All methods in Interface are abstract.
We can have multiple methods in abstract.
- Abstract class: In abstract class we have to implement the body.
Abstract class can have non-abstract methods. It can also have abstract method which is not implemented.
Can you make an Object from an Interface and Abstract class ? if not how do you use it ?
No we have to implement the interface or abstract class in a concrete class.
what is Access Specifier?
The visibility of classes, methods or variables.
What is OOP ? Define each feature of java OOP.
OOP is Object Oriented Programming. Main concepts are encapsulation, polymorphism, inheritance, and abstraction.
Encapsulation is data hiding.
Polymorphism is the ability of an object to behave differently in different situation. The two types of polymorphism are
overloading and overriding.
Inheritance is the ability of a class to acquire or inherit properties of other classes.
Abstraction is implementation hiding
.
What is Java Package and which package is imported by default?
A package organizes a set of related classes and interfaces. java.lang package is imported by default, no need to
explicitly import it. Classes in the java.lang package do not need to be imported (the compiler acts like they are
always imported).
What is API? Name list of API that you have used so far.
API stands for Application Programming Interface. Java application programming interface (API) is a list of all classes
that are part of the Java development kit (JDK). It includes all Java packages, classes, and interfaces, along with
their methods, fields, and constructors. These prewritten classes provide a tremendous amount of functionality to a
programmer.
Does java support multiple inheritance, explain why or why not?
No.
What is method overloading and when it happens?
Method overloading is when you have the same method name but with multiple arguments inside the parameters. It happens
during compile time.
Explain exceptions in java and how to handle it.
When an error occurs within a method, the method creates an object and hands it off to the runtime system.
The object,
called an exception object, contains information about the error,
including its type and the state of the
program when the error occurred.
Creating an exception object and handing it to the runtime system is called throwing an exception.
What is static keyword in java? How it has been used in variables and methods ?
Static keyword that are used for sharing the same variable or method of a given class.
What is final and how it has been used variables and methods?
What is final, finally and finalize?
Final - Final is used to apply restrictions on class, method and variable. Final class can't be inherited,
final method can't be overridden and final variable value can't be changed. (Final is a keyword)
Finally - Finally is used to place important code, it will be executed whether exception is handled or not.
(Finally is a block)
Finalize - Finalize is used to perform clean up processing just before object is garbage collected.
(Finally is a method)
What is a constructor ?
Constructor is used to initialize an object. A class can have multiple constructor.
Can we have multiple constructors in a class?
Yes as long as the parameter are different.
If we don't have a constructor declared, what is called during the object creation?
Default constructors
What is "this" keyword in java ?
this keyword refers to current object
What is "super" keyword in java? How many possible way can you use?
"Super" keyword is used to call the constructor, methods and properties of a parent class.
super can be used to refer immediate parent class instance variable.
super can be used to invoke immediate parent class method.
super() can be used to invoke immediate parent class constructor.
What is JVM stand for ?
Java Virtual Machine
Is JVM platform independent?
Yes
What version of java are you using?
1.8
What is JAR stand for ?
Java Archive is a package file format typically used to aggregate many Java class files
and associated metadata and
resources (text, images, etc.) into one file for distribution.
What is the difference between JDK and JVM?
JDK is Java Development Kit which include JVM,JRE
JVM (Java Virtual Machine) is an abstract machine.
What is the difference between JVM and JRE?
JRE is an acronym for Java Runtime Environment.It is used to provide runtime environment.It is the
implementation of JVM.It physically exists.It contains set of libraries + other files that JVM uses at runtime.
JDK is an acronym for Java Development Kit.It physically exists.It contains JRE + development tools.
What is compile time and run time?
When an application is running, it is called runtime.
The source code must be compiled into machine code in order to become an executable program, this is referred to as compile time.
What is heap?
Heap is the runtime data area from which memory for all class instances and arrays is allocated.
How java manage it's memory?
Java objects reside in an area called the heap. The heap is created when the JVM starts up and may increase
or decrease
in size while the application runs.
What is the difference between String, StringBuffer and StringBuilder?
Once a String object is created it cannot be changed
.
StringBuffer is mutable means one can change the value of the object.
StringBuilder allows manipulating character sequences by appending, deleting and inserting characters and strings
What is Singleton class?
Singleton class is to control object creation by keeping private constructor
What is Serialization and Deserialization?
Serialization is a process of converting an object into a sequence of bytes
which can be persisted to a disk or
database or can be sent through streams.
The reverse process of creating object from sequence of bytes is called deserialization
when to use transient variable in java?
Java transient keyword is used in serialization. If you define any data member as transient, it will not be serialized.
Difference between while and do..while loop?
In "while" loops, test expression is checked at first but
in "do...while" loop code is executed at first then the condition is checked.
So, the code are executed at least once in do...while loops.
What is Enum?
Enum is a set of fixed variables.
What is Iterator?
An iterator is an object that enables a programmer to traverse a container.
Which one will take more memory, an int or Integer?
int and Integer both represents same number of values.
Why is String Immutable in Java?
Because java uses the concept of string literal. For example there are 5 reference variables,
all refers to one object "arif”. If one reference variable changes the value of the object,
it will be affected to all the reference variables. That is why string objects are immutable in java.
What is constructor chaining in Java?
Constructor chaining is the process of calling one constructor from another constructor with respect to current object.
The difference between Serial and Parallel Garbage Collector?
Serial Collector is mostly designed for single-threaded environments.
Parallel Garbage Collector is used for multi-threaded environments
What is JIT stands for?
Just in time
Explain Java Heap space and Garbage collection?
Java objects reside in an area called the heap. The heap is created when the JVM starts up and may increase or
decrease in size while the application runs.
-Garbage collection is the process of looking at heap memory,
identifying which objects are in use and which are not and deleting the unused objects.
Can you guarantee the garbage collection process?
no
What is the difference between stack and heap in Java?
Java objects reside in an area called the heap. The heap is created when the JVM starts up and may increase or
decrease in size while the application runs.
-Garbage collection is the process of looking at heap memory,
identifying which objects are in use and which are not and deleting the unused objects.
What is reflection in java and why is it useful?
Java Reflection is quite powerful and can be very useful. Java Reflection makes it possible to inspect
classes, interfaces, fields and methods at runtime, without knowing the names of the classes, methods etc.
what is multithreading in java?
Multithreading in java is a process of executing multiple threads simultaneously. A thread is a lightweight
sub-process, the smallest unit of processing. Multiprocessing and multithreading, both are used to
achieve multitasking.
What is the use of synchronization in Java?
Synchronized keyword in Java is used to provide mutually exclusive access to a shared resource with
multiple threads in Java. Synchronization in Java guarantees that no two threads can execute a synchronized
method which requires the same lock simultaneously or concurrently.
What is Framework?
Frameworks generally refer to broad software development platforms
What are the testing Framework available in java?
junit and testng
Difference between jUnit and testNG?
In juniit methods have to be declared as static. TestNG does not have this constraint.
What are the dependencies for this project?
gson, java-json, mongo-java-driver, mysql-connector and testng
what is dependency injection in java?
The general concept behind dependency injection is called Inversion of Control. According to this concept a
class should not configure its dependencies statically but should be configured from the outside.
What is static binding and dynamic binding?
Static binding in Java occurs during compile time while dynamic binding occurs during runtime. |
import type { Metadata } from "next";
import { Toaster } from "sonner";
import { Inter } from "next/font/google";
import "./globals.css";
import { ThemeProvider } from "@/components/providers/theme-provider";
import { ConvexClientProvider } from "@/components/providers/convex-provider";
import { ModalProvider } from "@/components/providers/modal-provider";
const inter = Inter({ subsets: ["latin"] });
export const metadata: Metadata = {
title: "Notion",
description: "Notion Clone App",
icons: {
icon: [
{
media: "(prefers-color-scheme:light)",
url: "/logo.svg",
href: "/logo.svg",
},
{
media: "(prefers-color-scheme:dark)",
url: "/logo-dark.svg",
href: "/logo-dark.svg",
},
],
},
};
export default function RootLayout({
children,
}: {
children: React.ReactNode;
}) {
return (
<html lang="en" suppressContentEditableWarning>
<ConvexClientProvider>
<ThemeProvider
attribute="class"
defaultTheme="system"
enableSystem
disableTransitionOnChange
>
<body className={inter.className}>
<Toaster position="bottom-center" />
<ModalProvider />
{children}
</body>
</ThemeProvider>
</ConvexClientProvider>
</html>
);
} |
package day30_a_arraylist;
import java.util.ArrayList;
import java.util.Arrays;
/*
Remove Long Strings
Create a method that will accept an ArrayList of Strings and an int.
Remove any String elements that have less characters than the given number.
Return the modified ArrayList of Strings
@param list - Given ArrayList of Strings @param int - max number of characters @return - ArrayList of Strings
Ex: {“one”, “two”, “three, “four”, “five”, “six”} Max number: 4
Output: {“three, “four”, “five”
*/
public class RemoveLongString {
public static ArrayList <String> removeLongString (ArrayList <String> list, int maxLength ) {
ArrayList <String> updateList = new ArrayList<>(list);
updateList.removeIf( each -> (each.length() > maxLength) );
return updateList;
}
public static void main(String[] args) {
ArrayList <String> list = new ArrayList<>(Arrays.asList( "one", "two", "three", "four", "five", "six"));
System.out.println("Original: " + list);
System.out.println( "After update: " + removeLongString(list, 4)) ;
}
} |
import { waitFor } from '@testing-library/react';
import type Vi from 'vitest';
import fakeAddress from '__mocks__/models/address';
import { poolData } from '__mocks__/models/pools';
import { renderComponent } from 'testUtils/render';
import { useGetPools } from 'clients/api';
import useGetPool, { type UseGetPoolOutput } from '.';
describe('api/queries/useGetPool', () => {
beforeEach(() => {
(useGetPools as Vi.Mock).mockImplementation(() => ({
data: {
pools: poolData,
},
isLoading: false,
}));
});
it('returns the correct asset', async () => {
let data: Partial<UseGetPoolOutput['data']> = {};
const CallMarketContext = () => {
({ data } = useGetPool({
accountAddress: fakeAddress,
poolComptrollerAddress: poolData[0].comptrollerAddress,
}));
return <div />;
};
renderComponent(<CallMarketContext />);
await waitFor(() => expect(!!data).toBe(true));
expect(data).toMatchSnapshot();
});
it('returns undefined when no matching pool is found', async () => {
let data: Partial<UseGetPoolOutput['data']> = {};
const CallMarketContext = () => {
({ data } = useGetPool({
accountAddress: fakeAddress,
poolComptrollerAddress: 'fake-comptroller-address',
}));
return <div />;
};
renderComponent(<CallMarketContext />);
await waitFor(() => expect(data).toBe(undefined));
});
}); |
### KAPLAN-MEIER
### variaveis pensadas previamente
# DIRETO
# maternal_age
# newborn_weight
# num_prenatal_appointments
# tp_maternal_rece
# num_live_births
# tp_labor
# tp_marital_status
###
# tempo e status
y <- Surv(df$tempo_dias, df$status)
y
# analise km para tempo e status
KM <- survfit(y ~ 1, data = df)
KM
summary(KM)
# s(t)
fig15 <- autoplot(KM, conf.int = T) +
labs(x = "Tempo (dias)",
y = "Probabilidade de sobrevivência",
title = "A")
# f(t)
fig16 <- autoplot(KM, conf.int = T, fun = 'cumhaz') +
labs(x = "Tempo (dias)",
y = "Distribuição do tempo de vida")
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig16.png',
plot = fig16,
width = 8,
height = 6)
# 28 dias
df28 <- df[df$tempo_dias < 28, ] # [r, c]
z <- Surv(df28$tempo_dias, df28$status)
KM2 <- survfit(z ~ 1, data = df28)
KM2
summary(KM2)
# grafico
fig17 <- autoplot(KM2) +
labs(x = "Tempo < 28 dias",
y = "Probabilidade de sobrevivência",
title = "B")
grid.arrange(fig15, fig17, ncol = 2) -> fig15_17
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig15_17.png',
plot = fig15_17,
width = 8,
height = 6)
# estimador estimador nelson aalen
sobrev.na <- survfit(coxph(Surv(tempo_dias,status) ~ 1, data = df28)) # aqui uma variação para estimar NA (uma função dentro da outra)
sobrev.na
summary(sobrev.na)
# ambos
# comparando aalen e km
# s(t) sobrevida
fig18 <- autoplot(KM2, conf.int = T) +
labs(x = "Tempo (dias)",
y = "Probabilidade de sobrevivência",
title = "Kaplan-Meier")
fig19 <- autoplot(sobrev.na, conf.int = T) +
labs(x = "Tempo (dias)",
y = "Probabilidade de sobrevivência",
title = "Nelson-Aalen")
grid.arrange(fig18, fig19, nrow = 1) -> fig18_19
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig18_19.png',
plot = fig18_19,
width = 8,
height = 6)
# f(t) risco
fig20 <- autoplot(KM2,
conf.int = T,
fun = "cumhaz") +
labs(x = "Tempo (dias)",
y = "Distribuição do tempo de vida",
title = "Kaplan-Meier") +
coord_cartesian(ylim = c(0, 8))
fig21 <- autoplot(sobrev.na,
conf.int = T,
fun = "cumhaz") +
labs(x = "Tempo (dias)",
y = "Distribuição do tempo de vida",
title = "Nelson-Aalen") +
coord_cartesian(ylim = c(0, 8))
grid.arrange(fig20, fig21, nrow = 1) -> fig20_21
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig20_21.png',
plot = fig20_21,
width = 8,
height = 6)
summary(KM2)
summary(sobrev.na)
### ESTRATIFICACAO
# idade materna
# categorias idade materna: 12 - 22, 23 - 33, 34 - 44, 45 - 55
# criando das categorias
df_cat_maternal_age <- df |>
mutate(age_category = cut(maternal_age,
breaks = c(12, 22, 33, 44, 55),
labels = c("12-22", "23-33", "34-44", "45-55"),
include.lowest = TRUE))
# visualizando resultados
ggplot(df_cat_maternal_age, aes(x = age_category)) +
geom_bar() +
labs(x = "",
y = "")
# km estratificado pelas categorias de idade
m <- survfit(Surv(tempo_dias, status) ~ age_category, data = df_cat_maternal_age)
m # identifica categorias com n = 0
summary(m)
# rm n = 0
df_cat_maternal_age <- df_cat_maternal_age[df_cat_maternal_age$age_category != '45-55', ]
# km banco limpo
m <- survfit(Surv(tempo_dias, status) ~ age_category, data = df_cat_maternal_age)
# visualizando resultados (apos rm n = 0)
ggplot(df_cat_maternal_age, aes(x = age_category)) +
geom_bar() +
labs(x = "",
y = "")
# ajustes para o grafico
# ajustar o modelo de sobrevivencia
m <- survfit(Surv(tempo_dias, status) ~ age_category, data = df_cat_maternal_age)
# grafico
fig22 <- autoplot(m, conf.int = TRUE) +
labs(x = "Tempo (dias)",
y = "Probabilidade de sobrevivência") +
guides(fill = guide_legend(title = "Categorias de idade materna (anos)"),
color = guide_legend(title = "Categorias de idade materna (anos)")) +
theme(legend.position = "top")
# f(t)
fig23 <- autoplot(m, conf.int = T, fun = 'cumhaz') +
labs(x = "Tempo (dias)",
y = "Distribuição do tempo de vida") +
guides(fill = guide_legend(title = "Categorias de idade materna (anos)"),
color = guide_legend(title = "Categorias de idade materna (anos)")) +
theme(legend.position = "top")
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig22.png',
plot = fig22,
width = 8,
height = 6)
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig23.png',
plot = fig23,
width = 8,
height = 6)
### ESTRATIFICACAO
# pesos
# categorias de peso: 0 - 1500, 1501 - 3000, 3001 - 4500, 4501 - 6000
summary(df$newborn_weight) # visualizando max e min
# criando categorias
df_cat_newborn_wight <- df |>
mutate(weight_category = cut(newborn_weight,
breaks = c(0, 1501, 3001, 4501, 6000),
labels = c("0 a 1500", "1501 a 3000", "3001 a 4500", "4501 a 6000"),
include.lowest = TRUE))
# visualizando resultados
ggplot(df_cat_newborn_wight, aes(x = weight_category)) +
geom_bar() +
labs(x = "",
y = "")
m2 <- survfit(Surv(tempo_dias, status) ~ weight_category, data = df_cat_newborn_wight)
m2
summary(m2)
# rm n = 0
df_cat_newborn_wight <- df_cat_newborn_wight[df_cat_newborn_wight$weight_category != '4501 a 6000', ]
# km banco limpo
m2 <- survfit(Surv(tempo_dias, status) ~ weight_category, data = df_cat_newborn_wight)
# apos rm n = 0
ggplot(df_cat_newborn_wight, aes(x = weight_category)) +
geom_bar() +
labs(x = "",
y = "")
# grafico
fig24 <- autoplot(m2, conf.int = T) +
labs(x = "Tempo (dias)",
y = "Probabilidade de sobrevivência") +
guides(fill = guide_legend(title = "Categorias de peso do recém nascido (kg)"),
color = guide_legend(title = "Categorias de peso do recém nascido (kg)")) +
theme(legend.position = "top")
# f(t)
fig25 <- autoplot(m2, conf.int = T, fun = 'cumhaz') +
labs(x = "Tempo (dias)",
y = "Distribuição do tempo de vida") +
guides(fill = guide_legend(title = "Categorias de peso do recém nascido (kg)"),
color = guide_legend(title = "Categorias de peso do recém nascido (kg)")) +
theme(legend.position = "top")
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig24.png',
plot = fig24,
width = 8,
height = 6)
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig25.png',
plot = fig25,
width = 8,
height = 6)
### ESTRATIFICACAO
# numero de consultas pre natais
# categorias de consultas: 1 - 'nenhuma', 2 - 1 a 3 consultas, 3 - 4 a 6 consultas,
# 4 - 7 ou mais consultas
summary(df$num_prenatal_appointments) # visualizando max e min
# criando categorias
df_cat_prenatal_appointments <- df |>
mutate(appointments_category = cut(num_prenatal_appointments,
breaks = c(0, 1, 4, 7, 10, Inf),
labels = c("Nenhuma", "1 a 3", "4 a 6", "7 a 9", "10 ou mais"),
include.lowest = TRUE))
# visualizando resultados
ggplot(df_cat_prenatal_appointments, aes(x = appointments_category)) +
geom_bar() +
labs(x = "",
y = "")
# numero de consultas
m3 <- survfit(Surv(tempo_dias, status) ~ appointments_category, data = df_cat_prenatal_appointments)
m3
summary(m3)
# ajustar o modelo de sobrevivencia
m3 <- survfit(Surv(tempo_dias, status) ~ appointments_category, data = df_cat_prenatal_appointments)
# grafico
fig26 <- autoplot(m3, conf.int = T) +
labs(x = "Tempo (dias)",
y = "Probabilidade de sobrevivência") +
guides(fill = guide_legend(title = "Número de consultas pré-natais"),
color = guide_legend(title = "Número de consultas pré-natais")) +
theme(legend.position = "top")
# f(t)
fig27 <- autoplot(m3, conf.int = T, fun = 'cumhaz') +
labs(x = "Tempo (dias)",
y = "Distribuição do tempo de vida") +
guides(fill = guide_legend(title = "Número de consultas pré-natais"),
color = guide_legend(title = "Número de consultas pré-natais")) +
theme(legend.position = "top")
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig26.png',
plot = fig26,
width = 8,
height = 6)
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig27.png',
plot = fig27,
width = 8,
height = 6)
### ESTRATIFICACAO
# raca
# categorias de raca: 1 - branco, 2 - preto, 3 - amarelo, 4 - pardo, 5 - indigena, 9 - ignorado
df$tp_maternal_race
summary(df$tp_maternal_race)
df_cat_maternal_race <- df[df$tp_maternal_race != 9 & df$tp_maternal_race != 3, ]
# removendo as categorias 9
# removendo 3, pq nao sofreu eventos
df_cat_maternal_race <- df_cat_maternal_race %>%
mutate(tp_maternal_race = case_when(
tp_maternal_race == 1 ~ "branco",
tp_maternal_race == 2 ~ "preto",
tp_maternal_race == 3 ~ "amarelo",
tp_maternal_race == 4 ~ "pardo",
tp_maternal_race == 5 ~ "indígena",
tp_maternal_race == 9 ~ "ignorado",
TRUE ~ NA_character_ # se nao corresponder a nenhuma categoria, atribui NA
))
m4 <- survfit(Surv(tempo_dias, status) ~ tp_maternal_race, data = df_cat_maternal_race)
summary(m4)
fig28 <- autoplot(m4, conf.int = F) +
labs(x = "Tempo (dias)",
y = "Probabilidade de sobrevivência") +
guides(fill = guide_legend(title = "Raça materna"),
color = guide_legend(title = "Raça materna")) +
theme(legend.position = "top")
# f(t)
fig29 <- autoplot(m4, conf.int = F, fun = 'cumhaz') +
labs(x = "Tempo (dias)",
y = "Distribuição do tempo de vida") +
guides(fill = guide_legend(title = "Raça materna"),
color = guide_legend(title = "Raça materna")) +
theme(legend.position = "top")
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig28.png',
plot = fig28,
width = 8,
height = 6)
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig29.png',
plot = fig29,
width = 8,
height = 6)
#### ESTRATIFICACAO
# num. filhos tivos vidos
# categorias de filhos tidos vivos: 1 - 0 a 2, 2 - 3 a 4, 4 - 7 a 8, 5 - 9 a 10,
df$num_live_births
summary(df$num_live_births) # max e min
# criando categorias
df_cat_live_births <- df |>
mutate(num_liveb_category = cut(num_live_births,
breaks = c(0, 3, 5, 7, 9, Inf),
labels = c("0 a 2", "3 a 4", "5 a 6", "7 a 8", "9 a 10"),
include.lowest = TRUE))
# visualizando resultados
ggplot(df_cat_live_births, aes(x = num_liveb_category)) +
geom_bar() +
labs(x = "Número de filhos tidos",
y = "Quantidade")
# peso do filhos tidos vivos
m5 <- survfit(Surv(tempo_dias, status) ~ num_liveb_category, data = df_cat_live_births)
m5
summary(m5)
# removendo n = 0
df_cat_live_births <- df_cat_live_births[df_cat_live_births$num_liveb_category != "7 a 8" & df_cat_live_births$num_liveb_category != "9 a 10", ]
# visualizando resultados (apos rm n = 0)
ggplot(df_cat_live_births, aes(x = num_liveb_category)) +
geom_bar() +
labs(x = "Número de filhos tidos",
y = "Quantidade")
# apos rm n = 0
m5 <- survfit(Surv(tempo_dias, status) ~ num_liveb_category, data = df_cat_live_births)
# plotar o grafico de sobrevivencia usando autoplot
fig30 <- autoplot(m5, conf.int = F) +
labs(x = "Tempo (dias)",
y = "Probabilidade de sobrevivência") +
guides(fill = guide_legend(title = "Número de filhos tidos vivos"),
color = guide_legend(title = "Número de filhos tidos vivos")) +
theme(legend.position = "top")
# f(t)
fig31 <- autoplot(m5, conf.int = F, fun = 'cumhaz') +
labs(x = "Tempo (dias)",
y = "Distribuição do tempo de vida") +
guides(fill = guide_legend(title = "Número de filhos tidos vivos"),
color = guide_legend(title = "Número de filhos tidos vivos")) +
theme(legend.position = "top")
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig30.png',
plot = fig30,
width = 8,
height = 6)
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig31.png',
plot = fig31,
width = 8,
height = 6)
#### ESTRATIFICACAO
# num. tipo de parto
# categorias de parto: 1 - vaginal, 2 - cesariana e 9 ignorado
df$tp_labor
df_cat_labor <- df[df$tp_labor != 9, ] # removendo as categorias 9
df_cat_labor <- df_cat_labor %>%
mutate(tp_labor = case_when(
tp_labor == 1 ~ "vaginal",
tp_labor == 2 ~ "cesariana",
TRUE ~ NA_character_))
# tipo de parto
m6 <- survfit(Surv(tempo_dias, status) ~ tp_labor, data = df_cat_labor)
m6
summary(m6)
# plotar o grafico de sobrevivencia usando autoplot
fig32 <- autoplot(m6, conf.int = T) +
labs(x = "Tempo (dias)",
y = "Probabilidade de sobrevivência") +
guides(fill = guide_legend(title = "Tipo de parto"),
color = guide_legend(title = "Tipo de parto")) +
theme(legend.position = "top")
# f(t)
fig33 <- autoplot(m6, conf.int = T, fun = 'cumhaz') +
labs(x = "Tempo (dias)",
y = "Distribuição do tempo de vida") +
guides(fill = guide_legend(title = "Tipo de parto"),
color = guide_legend(title = "Tipo de parto")) +
theme(legend.position = "top")
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig32.png',
plot = fig32,
width = 8,
height = 6)
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig33.png',
plot = fig33,
width = 8,
height = 6)
#### ESTRATIFICACAO
# educacao
# categorias de educacao: 1 - nenhuma, 2 - 1 a 3 anos, 3 - 4 a 7 anos, 4 - 8 a 11 anos, 5 - 12 e mais, 9 - NA
df$tp_maternal_schooling
# removendo 9 e 0 (PERGUNTAR PRO CARLOS SOBRE A CATEGORIA 0)
df_cat_maternal_schooling <- df[df$tp_maternal_schooling != 9 & df$tp_maternal_schooling != 0, ]
df_cat_maternal_schooling <- df_cat_maternal_schooling %>%
mutate(tp_maternal_schooling = case_when(
tp_maternal_schooling == 1 ~ "nenhuma",
tp_maternal_schooling == 2 ~ "1 a 3",
tp_maternal_schooling == 3 ~ "4 a 7",
tp_maternal_schooling == 4 ~ "8 a 11",
tp_maternal_schooling == 5 ~ "12 e mais",
TRUE ~ NA_character_))
df_cat_maternal_schooling$tp_maternal_schooling <- factor(df_cat_maternal_schooling$tp_maternal_schooling,
levels = c("nenhuma",
"1 a 3",
"4 a 7",
"8 a 11",
"12 e mais"))
m7 <- survfit(Surv(tempo_dias, status) ~ tp_maternal_schooling, data = df_cat_maternal_schooling)
m7
summary(m7)
# plotar o grafico de sobrevivencia usando autoplot
fig34 <- autoplot(m7, conf.int = F) +
labs(x = "Tempo (dias)",
y = "Probabilidade de sobrevivência") +
guides(fill = guide_legend(title = "Escolaridade materna (anos)"),
color = guide_legend(title = "Escolaridade materna (anos)")) +
theme(legend.position = "top")
# f(t)
fig35 <- autoplot(m7, conf.int = F, fun = 'cumhaz') +
labs(x = "Tempo (dias)",
y = "Distribuição do tempo de vida") +
guides(fill = guide_legend(title = "Escolaridade materna (anos)"),
color = guide_legend(title = "Escolaridade materna (anos)")) +
theme(legend.position = "top")
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig34.png',
plot = fig34,
width = 8,
height = 6)
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig35.png',
plot = fig35,
width = 8,
height = 6)
#### ESTRATIFICACAO
# status marital
# categorias de status marital: 1 - solteiro, 2 - casado, 3 - viúvo, 4 separadado/divorciado judicialmente, 5 - uniao estavel, 9 - ignorado
df$tp_marital_status
df_cat_marital_status <- df[df$tp_marital_status != 9 & df$tp_marital_status != 3, ]
df_cat_marital_status <- df_cat_marital_status %>%
mutate(tp_marital_status = case_when(
tp_marital_status == 1 ~ "solteiro",
tp_marital_status == 2 ~ "casado",
tp_marital_status == 3 ~ "viúvo",
tp_marital_status == 4 ~ "separado ou divorciado",
tp_marital_status == 5 ~ "união estável",
TRUE ~ NA_character_))
df_cat_marital_status$tp_marital_status <- factor(df_cat_marital_status$tp_marital_status,
levels = c("solteiro",
"casado",
"viúvo",
"separado ou divorciado",
"união estável"))
# status marital
m8 <- survfit(Surv(tempo_dias, status) ~ tp_marital_status, data = df_cat_marital_status)
m8
summary(m8)
# plotar o grafico de sobrevivencia usando autoplot
fig36 <- autoplot(m8, conf.int = F) +
labs(x = "Tempo (dias)",
y = "Probabilidade de sobrevivência") +
guides(fill = guide_legend(title = "Status marital"),
color = guide_legend(title = "Status marital")) +
theme(legend.position = "top")
# f(t)
fig37 <- autoplot(m8, conf.int = F, fun = 'cumhaz') +
labs(x = "Tempo (dias)",
y = "Distribuição do tempo de vida") +
guides(fill = guide_legend(title = "Status marital"),
color = guide_legend(title = "Status marital")) +
theme(legend.position = "top")
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig36.png',
plot = fig36,
width = 8,
height = 6)
ggsave(filename = 'C:\\Users\\User\\Desktop\\dm016\\fig37.png',
plot = fig37,
width = 8,
height = 6) |
<!DOCTYPE html>
<html>
<head>
<title>Mini Facebook API v0.1</title>
{# <link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet"> #}
<link type="text/css" rel="stylesheet" href="static/css/materialize.min.css">
<link rel="shortcut icon" href="/static/favicon.ico">
{# <script type="text/javascript" src="static/js/materialize.min.js"></script> #}
{# <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> #}
<meta name="viewport" content="width=device-width, initial-scale=1.0"/>
</head>
<body class="blue darken-3">
<h5 class="center">Mini Facebook API v0.1</h5>
<div class="container">
<div class="green-text" style="margin-top: 20px">
<div class="black" style="padding: 10px">
<p class="center" style="font-size: 30px">Menghasilkan Cookies</p>
<p>API ini menggunakan cookies untuk login akun facebook anda. Cookies yg di input ke API ini harus di encrypt menggunakan base64 alasanya bukan karena keamanan tetapi agar cookies dapat dikirim menggunakan metode GET. berikut adalah bagaimana menghasilkan cookies API ini:</p>
<b>Metode:</b> POST<br>
<b>Url: </b>{{url}}/generate_cookies<br>
<b>Parameter: </b>user, pass <br><br>
<i>Contoh penggunaan:</i><br><br>
<i>import requests as r</i><br>
<i>data = {'user':'username kamu', 'pass':'password kamu'}</i><br>
<i>page = r.post("{{url}}/generate_cookies", data=data).json()</i>
</div>
</div>
<div style="padding: 5px"></div>
<div class="green-text" style="margin-top: 20px">
<div class="black" style="padding: 10px">
<p class="center" style="font-size: 30px">Melihat Informasi Saya</p>
<b>Metode:</b> GET<br>
<b>Url: </b>{{url}}/me?kuki=cookies_ente<br>
<b>Parameter: </b>kuki <br><br>
<i>Contoh penggunaan:</i><br><br>
<i>import requests as r</i><br>
<i>page = r.get("{{url}}/me?kuki=c2FsaXNnYW5zc2FuZ2FkZGQ=").json()</i>
</div>
</div>
<div style="padding: 5px"></div>
<div class="green-text" style="margin-top: 20px">
<div class="black" style="padding: 10px">
<p class="center" style="font-size: 30px">Melihat Informasi Teman</p>
<b>Metode:</b> GET<br>
<b>Url: </b>{{url}}/people/id_teman?kuki=cookies_ente<br>
<b>Parameter: </b>kuki <br><br>
<i>Contoh penggunaan:</i><br><br>
<i>import requests as r</i><br>
<i>page = r.get("{{url}}/people/100041106940465?kuki=c2FsaXNnYW5zc2FuZ2FkZGQ=").json()</i>
</div>
</div>
<div style="padding: 5px"></div>
<div class="green-text" style="margin-top: 20px">
<div class="black" style="padding: 10px">
<p class="center" style="font-size: 30px">Melihat Grup Saya</p>
<b>Metode:</b> GET<br>
<b>Url: </b>{{url}}/me/groups?kuki=cookies_ente<br>
<b>Parameter: </b>kuki <br><br>
<i>Contoh penggunaan:</i><br><br>
<i>import requests as r</i><br>
<i>page = r.get("{{url}}/me/groups?kuki=c2FsaXNnYW5zc2FuZ2FkZGQ=").json()</i>
</div>
</div>
<div style="padding: 5px"></div>
<div class="green-text" style="margin-top: 20px">
<div class="black" style="padding: 10px">
<p class="center" style="font-size: 30px">Melihat Teman Saya</p>
<b>Metode:</b> GET<br>
<b>Url: </b>{{url}}/me/friends?kuki=cookies_ente<br>
<b>Parameter: </b>kuki <br><br>
<i>Contoh penggunaan:</i><br><br>
<i>import requests as r</i><br>
<i>page = r.get("{{url}}/me/friends?kuki=c2FsaXNnYW5zc2FuZ2FkZGQ=").json()</i>
</div>
</div>
<div style="padding: 5px"></div>
<div class="green-text" style="margin-top: 20px">
<div class="black" style="padding: 10px">
<p class="center" style="font-size: 30px">Melihat Info Grup</p>
<b>Metode:</b> GET<br>
<b>Url: </b>{{url}}/group/id_grup?kuki=cookies_ente<br>
<b>Parameter: </b>kuki <br><br>
<i>Contoh penggunaan:</i><br><br>
<i>import requests as r</i><br>
<i>page = r.get("{{url}}/group/1922836934621172?kuki=c2FsaXNnYW5zc2FuZ2FkZGQ=").json()</i>
</div>
</div>
<div style="padding: 5px"></div>
<div class="green-text" style="margin-top: 20px">
<div class="black" style="padding: 10px">
<p class="center" style="font-size: 30px">Melihat Member Grup</p>
<b>Metode:</b> GET<br>
<b>Url: </b>{{url}}/group/id_grup/members?kuki=cookies_ente<br>
<b>Parameter: </b>kuki <br><br>
<i>Contoh penggunaan:</i><br><br>
<i>import requests as r</i><br>
<i>page = r.get("{{url}}/group/1922836934621172/members?kuki=c2FsaXNnYW5zc2FuZ2FkZGQ=").json()</i>
</div>
</div>
<div style="padding: 5px"></div>
<div class="green-text" style="margin-top: 20px">
<div class="black" style="padding: 10px">
<p class="center" style="font-size: 30px">Melihat Liker Postingan</p>
<b>Metode:</b> GET<br>
<b>Url: </b>{{url}}/post/id_post/likes?kuki=cookies_ente<br>
<b>Parameter: </b>kuki <br><br>
<i>Contoh penggunaan:</i><br><br>
<i>import requests as r</i><br>
<i>page = r.get("{{url}}/post/id_post/likes?kuki=c2FsaXNnYW5zc2FuZ2FkZGQ=").json()</i>
<br><br>
<span>Jika mengeksekusi perintah diatas secara default sistem akan memilih liker bertype suka. Tambahkan parameter "type" untuk melihat liker yang bertype react, untuk valuenya anda bisa lihat dibawah.</span>
<ul>
<ol>- mantapmantap = love</ol>
<ol>- sadna = sad</ol>
<ol>- ngamuk = angry</ol>
<ol>- ngakak = haha</ol>
<ol>- keren = wow</ol>
</ul><br>
<i>Contoh penggunaan:</i><br><br>
<b>Metode:</b> GET<br>
<b>Url: </b>{{url}}/post/id_post/likes?kuki=cookies_ente&type=type_na<br>
<b>Parameter: </b>kuki, type <br><br>
<i>import requests as r</i><br>
<i>page = r.get("{{url}}/post/id_post/likes?kuki=c2FsaXNnYW5zc2FuZ2FkZGQ=&type=mantapmantap").json()</i>
</div>
</div>
<div class="green-text" style="margin-top: 20px">
<div class="black" style="padding: 10px">
<p class="center" style="font-size: 30px">Menyukai Postingan</p>
<b>Metode:</b> POST<br>
<b>Url: </b>{{url}}/post/id_post/likes<br>
<b>Parameter: </b>kuki <br><br>
<i>Contoh penggunaan:</i><br><br>
<i>import requests as r</i><br>
<i>data = {'kuki:'c2FsaXNnYW5zc2FuZ2FkZGQ='}</i><br>
<i>page = r.post("{{url}}/post/166694224710808&/likes", data=data).json()</i>
<br><br><i>Atau</i><br><br>
<b>Metode:</b> GET<br>
<b>Url: </b>{{url}}/post/id_post/likes?kuki=c2FsaXNnYW5zc2FuZ2FkZGQ=&method=post<br>
<b>Parameter: </b>kuki, method <br><br>
<i>Contoh penggunaan:</i><br><br>
<i>import requests as r</i><br>
<i>page = r.post("{{url}}/post/166694224710808&/likes?kuki=c2FsaXNnYW5zc2FuZ2FkZGQ=&method=post").json()</i>
</div>
</div>
<div class="center white-text" style="padding: 25px"><strong>© 2020 salis mazaya from xiuz.sec</strong></div>
</div>
</body>
</html> |
/*
* Copyright (C) 2013 OpenJST Project
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
package org.openjst.protocols.basic.pdu.packets;
import org.openjst.commons.io.buffer.ArrayDataInputBuffer;
import org.openjst.commons.io.buffer.ArrayDataOutputBuffer;
import org.openjst.commons.io.buffer.DataBufferException;
import org.openjst.protocols.basic.pdu.Packets;
import org.openjst.protocols.basic.pdu.beans.Parameter;
import org.openjst.protocols.basic.utils.PacketUtils;
import javax.annotation.Nullable;
import java.util.Set;
/**
* @author Sergey Grachev
*/
public final class AuthResponsePacket extends AbstractAuthPacket {
private int responseStatus;
AuthResponsePacket() {
}
AuthResponsePacket(final long packetId, final int responseStatus, @Nullable final Set<Parameter> parameters) {
this.packetId = packetId;
this.parameters = parameters;
this.responseStatus = responseStatus;
}
AuthResponsePacket(final long uuid, final int responseStatus) {
this(uuid, responseStatus, null);
}
public byte[] encode() throws DataBufferException {
final ArrayDataOutputBuffer buf = new ArrayDataOutputBuffer();
buf.writeVLQInt64(packetId);
buf.writeVLQInt32(responseStatus);
PacketUtils.writeParameters(buf, parameters);
return buf.toByteArray();
}
public void decode(final byte[] data) throws DataBufferException {
final ArrayDataInputBuffer buf = new ArrayDataInputBuffer(data);
this.packetId = buf.readVLQInt64();
this.responseStatus = buf.readVLQInt32();
parameters = PacketUtils.readParameters(buf);
}
public short getType() {
return Packets.TYPE_AUTHENTICATION_RESPONSE;
}
public int getResponseStatus() {
return responseStatus;
}
@Override
public String toString() {
return "AuthResponsePacket{" +
"responseStatus=" + responseStatus +
"} " + super.toString();
}
} |
<template>
<div>
<el-table style="width: 100%" border :data="records" v-loading="loading">
<el-table-column type="index" label="序号" width="80" align="center">
</el-table-column>
<el-table-column prop="skuName" label="名称" width="width">
</el-table-column>
<el-table-column prop="skuDesc" label="描述" width="width">
</el-table-column>
<el-table-column prop="prop" label="默认图片" width="110">
<template slot-scope="{ row }">
<img
:src="row.skuDefaultImg"
alt=""
style="width: 80px; height: 80px"
/>
</template>
</el-table-column>
<el-table-column prop="weight" label="重量(KG)" width="80">
</el-table-column>
<el-table-column prop="price" label="价格(元)" width="80">
</el-table-column>
<el-table-column prop="prop" label="操作" width="width" align="center">
<template slot-scope="{ row }">
<!-- 该图标显示的是商品正在处于的状态 -->
<el-button
type="success"
icon="el-icon-bottom"
size="mini"
v-if="row.isSale == 0"
@click="onSale(row)"
></el-button>
<el-button
type="success"
icon="el-icon-top"
size="mini"
v-else
@click="cancelSale(row)"
></el-button>
<el-button
type="primary"
icon="el-icon-edit"
size="mini"
@click="$message('正在开发中...')"
></el-button>
<el-button
type="info"
icon="el-icon-info"
size="mini"
@click="getSkuInfo(row)"
></el-button>
<el-button
type="danger"
icon="el-icon-delete"
size="mini"
@click="deleteSku(row)"
></el-button>
</template>
</el-table-column>
</el-table>
<!-- 分页器 -->
<el-pagination
@size-change="sizeChange"
@current-change="getSkuList"
:current-page="page"
:page-sizes="[5, 10, 20]"
:page-size="100"
layout="prev, pager, next, jumper,->,total,sizes"
:total="total"
style="text-align: center"
>
</el-pagination>
<!-- 抽屉 -->
<el-drawer
:visible.sync="drawer"
:direction="direction"
size="50%"
:show-close="false"
>
<el-row>
<el-col :span="5">名称</el-col>
<el-col :span="16">{{ skuInfo.skuName }}</el-col>
</el-row>
<el-row>
<el-col :span="5">描述</el-col>
<el-col :span="16">{{ skuInfo.skuDesc }}</el-col>
</el-row>
<el-row>
<el-col :span="5">价格</el-col>
<el-col :span="16">{{ skuInfo.price }}</el-col>
</el-row>
<el-row>
<el-col :span="5">平台属性</el-col>
<el-col :span="16"
><el-tag
type="success"
v-for="attr in skuInfo.skuAttrValueList"
:key="attr.id"
style="margin-right: 10px; margin-bottom: 10px"
>{{ attr.attrName }}-{{ attr.valueName }}</el-tag
></el-col
>
</el-row>
<el-row>
<el-col :span="5">商品图片</el-col>
<el-col :span="16">
<el-carousel trigger="click" height="400px" style="width: 400px">
<el-carousel-item
v-for="item in skuInfo.skuImageList"
:key="item.id"
>
<img :src="item.imgUrl" width="400px" height="400px" />
</el-carousel-item>
</el-carousel>
</el-col>
</el-row>
</el-drawer>
</div>
</template>
<script>
export default {
name: 'Sku',
data() {
return {
page: 1,
limit: 5,
total: 0,
records: [],
loading: true,
drawer: false,
direction: 'rtl',
skuInfo: {}
}
},
methods: {
async getSkuList(pages = 1) {
this.page = pages
let { page, limit } = this
const result = await this.$API.sku.reqSkuList(page, limit)
if (result.code == 200) {
this.records = result.data.records
this.total = result.data.total
this.loading = false
}
},
sizeChange(limit) {
this.loading = true
this.limit = limit
this.getSkuList()
},
// 上架
async onSale(row) {
try {
this.loading = true
const result = await this.$API.sku.reqOnSale(row.id)
row.isSale = 1
this.$message({
type: 'success',
message: '上架成功'
})
this.loading = false
} catch (error) {
this.loading = false
}
},
// 下架
async cancelSale(row) {
try {
this.loading = true
const result = await this.$API.sku.reqCancelSale(row.id)
row.isSale = 0
this.$message({
type: 'success',
message: '下架成功'
})
this.loading = false
} catch (error) {
this.loading = false
}
},
// 查看当前sku信息
async getSkuInfo(row) {
this.drawer = true
const result = await this.$API.sku.reqSkuInfo(row.id)
if (result.code == 200) {
this.skuInfo = result.data
}
},
async deleteSku(row) {
this.loading = true
const result = await this.$API.sku.reqDeleteSku(row.id)
if (result.code == 200) {
this.loading = false
this.$message({
type: 'success',
message: '删除成功'
})
this.getSkuList(this.records.length > 1 ? this.page : this.page - 1)
}
}
},
mounted() {
this.getSkuList()
}
}
</script>
<style>
.el-carousel__item h3 {
color: #475669;
font-size: 14px;
opacity: 0.75;
line-height: 150px;
margin: 0;
}
.el-carousel__item:nth-child(2n) {
background-color: #99a9bf;
}
.el-carousel__item:nth-child(2n + 1) {
background-color: #d3dce6;
}
</style>
<style scoped>
.el-row .el-col-5 {
font-size: 18px;
text-align: right;
font-weight: bold;
}
.el-col {
margin: 10px;
}
/* 深度选择器 */
>>> .el-carousel__button {
width: 10px;
height: 10px;
border-radius: 50%;
background-color: #99a9bf;
}
</style> |
command SPECTROGRAM <trc> [<lo-t> <hi-t>] <spgwith> <spgstep> <outfile>
key: Spectrogram
Computes spectrogram of a trace and writes the result to a text file. The
result may be viewed with 'mapspec':
setenv UIDPATH $SH_SOURCE/img/mapspec.uid
$SH_SOURCE/img/mapspec <datafile> 30 # 30 is number of colours used
The spectrogram is computed as follows: a subwindow of N samples of the
selected trace window is transformed to frequency domain using the FFT
algorithm (see Numerical Recipes, Press et al., Cambridge University Press,
1988). N is determined by the configuration parameter spectrogram_width
and must be a power of 2. Reasonable values are e.g. 512 or 1024. Before
FFT the input trace is tapered in time domain using a cosine taper
(2*cos(x)-1, -pi < x < pi). The squared frequency amplitudes are displayed
in a colour-coded vertical bar/line in the output window. The next subwindow
for FFT is found by shifting the last one by K samples to the right. K is
determined by the configuration parameter spectrogram_step. The first
subwindow starts with the first sample of the selected trace part, the last
subwindow ends between 0 and K-1 samples before the end of the selected
trace part. The output matrix contains frequency over time. The header
info of the output file contains DELTA (sample distance in time, s), START
(absolute start time of trace), LENGTH (number of frequency samples on each
time step), LINES (number of time steps), DF (frequency sample distance in Hz).
Following are LENGTH*LINES numbers giving frequency amplitudes over the time
steps. The first LENGTH samples refer to time sample 1, the second LENGTH
samples refer to time sample 2 and so on.
parameters
----------
<trc> --- parameter type: trace
Trace to compute spectrogram.
<lo-t>, <hi-t> --- parameter type: real
Time window on input trace. If not specified the current
display window is used. If <lo-t> is an astrisk ("*")
you can select the window by graphic cursor.
<spgwidth> --- parameter type: integer
Width of an FFT window in samples. Must be a power of 2. Is number N in
above description.
<spgstep> --- parameter type: integer
Step size in samples. Is number K in above description.
example
-------
spectrogram 3 ;;; 512 5 SPEC.DAT
! computes spectrogram of trace number 3 on the total available
! time window and writes the output to file SPEC.DAT. |
<?php
/*
* $Id: TouchTask.php 144 2007-02-05 15:19:00Z hans $
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
* OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
* This software consists of voluntary contributions made by many individuals
* and is licensed under the LGPL. For more information please see
* <http://phing.info>.
*/
require_once 'phing/Task.php';
include_once 'phing/util/DirectoryScanner.php';
include_once 'phing/types/FileSet.php';
include_once 'phing/util/FileUtils.php';
include_once 'phing/system/io/PhingFile.php';
include_once 'phing/system/io/IOException.php';
/**
* Touch a file and/or fileset(s); corresponds to the Unix touch command.
*
* If the file to touch doesn't exist, an empty one is created.
*
* @version $Revision: 1.12 $
* @package phing.tasks.system
*/
class TouchTask extends Task {
private $file;
private $millis = -1;
private $dateTime;
private $filesets = array();
private $fileUtils;
function __construct() {
$this->fileUtils = new FileUtils();
}
/**
* Sets a single source file to touch. If the file does not exist
* an empty file will be created.
*/
function setFile(PhingFile $file) {
$this->file = $file;
}
/**
* the new modification time of the file
* in milliseconds since midnight Jan 1 1970.
* Optional, default=now
*/
function setMillis($millis) {
$this->millis = (int) $millis;
}
/**
* the new modification time of the file
* in the format MM/DD/YYYY HH:MM AM or PM;
* Optional, default=now
*/
function setDatetime($dateTime) {
$this->dateTime = (string) $dateTime;
}
/**
* Nested creator, adds a set of files (nested fileset attribute).
* @return FileSet
*/
function createFileSet() {
$num = array_push($this->filesets, new FileSet());
return $this->filesets[$num-1];
}
/**
* Execute the touch operation.
*/
function main() {
$savedMillis = $this->millis;
if ($this->file === null && count($this->filesets) === 0) {
throw new BuildException("Specify at least one source - a file or a fileset.");
}
if ($this->file !== null && $this->file->exists() && $this->file->isDirectory()) {
throw new BuildException("Use a fileset to touch directories.");
}
try { // try to touch file
if ($this->dateTime !== null) {
$this->setMillis(strtotime($this->dateTime));
if ($this->millis < 0) {
throw new BuildException("Date of {$this->dateTime} results in negative milliseconds value relative to epoch (January 1, 1970, 00:00:00 GMT).");
}
}
$this->_touch();
} catch (Exception $ex) {
throw new BuildException("Error touch()ing file", $ex, $this->location);
}
$this->millis = $savedMillis;
}
/**
* Does the actual work.
*/
function _touch() {
if ($this->file !== null) {
if (!$this->file->exists()) {
$this->log("Creating " . $this->file->__toString(), Project::MSG_INFO);
try { // try to create file
$this->file->createNewFile();
} catch(IOException $ioe) {
throw new BuildException("Error creating new file " . $this->file->__toString(), $ioe, $this->location);
}
}
}
$resetMillis = false;
if ($this->millis < 0) {
$resetMillis = true;
$this->millis = Phing::currentTimeMillis();
}
if ($this->file !== null) {
$this->touchFile($this->file);
}
// deal with the filesets
foreach($this->filesets as $fs) {
$ds = $fs->getDirectoryScanner($this->getProject());
$fromDir = $fs->getDir($this->getProject());
$srcFiles = $ds->getIncludedFiles();
$srcDirs = $ds->getIncludedDirectories();
for ($j=0,$_j=count($srcFiles); $j < $_j; $j++) {
$this->touchFile(new PhingFile($fromDir, (string) $srcFiles[$j]));
}
for ($j=0,$_j=count($srcDirs); $j < $_j ; $j++) {
$this->touchFile(new PhingFile($fromDir, (string) $srcDirs[$j]));
}
}
if ($resetMillis) {
$this->millis = -1;
}
}
private function touchFile($file) {
if ( !$file->canWrite() ) {
throw new BuildException("Can not change modification date of read-only file " . $file->__toString());
}
$file->setLastModified($this->millis);
}
} |
package Pages;
import org.openqa.selenium.By;
import io.appium.java_client.MobileElement;
import io.appium.java_client.android.AndroidDriver;
import io.appium.java_client.android.AndroidElement;
import testCommons.Errors;
public class Options extends Page {
/********************/
/* *** Elements *** */
/********************/
private static String optionsDoneButtonId = Page.connectId + "option_done_btn";
private static String loggedInEmailTextViewId = Page.connectId + "loggedIn_email";
private static String signUpOrLogInItemId = Page.connectId + "signup_or_login_item";
public enum OptionItem {
SIGN_UP_OR_LOG_IN(signUpOrLogInItemId),
LOGGED_IN_AS(signUpOrLogInItemId),
UPDATE_PASSWORD(Page.connectId + "update_password_item"),
FACEBOOK(Page.connectId + "facebook_login_item"),
GOOGLE_PLUS(Page.connectId + "google_plus_login_item"),
MY_LOCATION(Page.connectId + "reset_location_item"),
MY_GENRE(Page.connectId + "my_genres_item"),
AUTOPLAY_ON_OPEN(Page.connectId + "play_last_station_item"),
EXPLICIT_CONTENT(Page.connectId + "explicit_content_item"),
CAR_CONNECTIONS(Page.connectId + "bluetooth_connections_item"),
TERMS_OF_USE(Page.connectId + "terms_and_conditions_item"),
PRIVACY_POLICY(Page.connectId + "private_policy_item"),
ABOUT(Page.connectId + "about_item"),
HELP(Page.connectId + "help_item");
public final String mId;
private OptionItem (String id) {
mId = id;
}
public String toString () {
return name().replace('_', ' ').replaceAll("AND", "&");
}
}
/*******************/
/* *** Getters *** */
/*******************/
public static AndroidElement getDoneButton (AndroidDriver<MobileElement> d) {
return waitForVisible(d, By.id(optionsDoneButtonId), 7);
}
public static AndroidElement getLoggedInEmailTextView (AndroidDriver<MobileElement> d) {
return scrollUntil(d, DOWN, By.id(loggedInEmailTextViewId));
}
public static AndroidElement scrollToGetOptionItem (AndroidDriver<MobileElement> d, int direction, OptionItem option) {
return scrollUntil(d, direction, By.id(option.mId));
}
/***************************************/
/* *** Individual Element Behavior *** */
/***************************************/
public static Errors tapDoneButton (AndroidDriver<MobileElement> d) {
return click(d, getDoneButton(d), "Cannot click on done button!", "tapDoneButton");
}
public static Errors scrollAndTapOptionItem (AndroidDriver<MobileElement> d, int direction, OptionItem option) {
String errorMessage = String.format("Cannot tap option item: %s.", option.toString());
return click(d, scrollToGetOptionItem(d, direction, option), errorMessage, "scrollAndTapOptionItem");
}
/*******************/
/* *** Utility *** */
/*******************/
public static String getLoggedInEmail (AndroidDriver<MobileElement> d) {
return getText(d, getLoggedInEmailTextView(d));
}
public static Errors logOut (AndroidDriver<MobileElement> d) {
Errors err = new Errors();
err.add(d, scrollAndTapOptionItem(d, DOWN, OptionItem.LOGGED_IN_AS));
err.add(d, tapRedDialogButton(d)); // Log out button
err.add(d, Page.tapWhiteDialogButton(d)); // Okay button
return err;
}
} |
package BasicExercises.Collection.TreeSet;
import java.util.TreeSet;
import java.util.Iterator;
public class TreeSet6_10 {
public static void main(String[] args) {
System.out.println("Exercise 6");
/*
Напишите программу на Java для клонирования списка наборов деревьев в другой набор деревьев.
*/
//Мой вариант
// TreeSet <String> tset = new TreeSet <String>();
//
// tset.add("Red");
// tset.add("Green");
// tset.add("Black");
// tset.add("White");
// tset.add("Pink");
//
// System.out.println("The TreeSet:\n" + tset);
//
// TreeSet cloned_set = new TreeSet();
// cloned_set = (TreeSet)tset.clone();
// System.out.println("The cloned TreeSet:\n" + cloned_set);
//Вариант 2
// // create an empty tree set
// TreeSet<String> t_set = new TreeSet<String>();
// // use add() method to add values in the tree set
// t_set.add("Red");
// t_set.add("Green");
// t_set.add("Black");
// t_set.add("Pink");
// t_set.add("orange");
//
// System.out.println("Original tree set:" + t_set);
// TreeSet<String> new_t_set = (TreeSet<String>)t_set.clone();
// System.out.println("Cloned tree list: " + t_set);
System.out.println("Exercise 7");
/*
Напишите программу на Java, чтобы получить количество элементов в наборе деревьев.
*/
//Мой вариант
// TreeSet <String> tset = new TreeSet <String>();
//
// tset.add("Red");
// tset.add("Green");
// tset.add("Black");
// tset.add("White");
// tset.add("Pink");
//
// System.out.println("The TreeSet:\n" + tset);
//
// System.out.println("The size of the set is: " + tset.size());
//Вариант 2
// // create an empty tree set
// TreeSet<String> t_set = new TreeSet<String>();
// // use add() method to add values in the tree set
// t_set.add("Red");
// t_set.add("Green");
// t_set.add("Black");
// t_set.add("Pink");
// t_set.add("orange");
// System.out.println("Original tree set: " + t_set);
// System.out.println("Size of the tree set: " + t_set.size());
System.out.println("Exercise 8");
/*
Напишите программу на Java для сравнения двух наборов деревьев.
*/
//Мой вариант
// TreeSet <String> tset = new TreeSet <String>();
//
// tset.add("Red");
// tset.add("Green");
// tset.add("Black");
// tset.add("White");
// tset.add("Pink");
//
// System.out.println("The TreeSet:\n" + tset);
//
// TreeSet <String> tset2 = new TreeSet <String>();
//
// tset2.add("Red");
// tset2.add("Green");
// tset2.add("Black");
// tset2.add("White");
//
// System.out.println("The TreeSet 2:\n" + tset2);
//
// // compare TreeSet with TreeSet2
// if (tset.equals(tset2) == true) {
// System.out.println("TreeSet are equal");
// } else {
// // else block execute when
// // ArrayList are not equal
// System.out.println();
// System.out.println("TreeSet are not equal");
// }
//Вариант 2
// // Create a empty tree set
// TreeSet<String> t_set1 = new TreeSet<String>();
// // use add() method to add values in the tree set
// t_set1.add("Red");
// t_set1.add("Green");
// t_set1.add("Black");
// t_set1.add("White");
// System.out.println("Free Tree set: "+t_set1);
//
// TreeSet<String> t_set2 = new TreeSet<String>();
// t_set2.add("Red");
// t_set2.add("Pink");
// t_set2.add("Black");
// t_set2.add("Orange");
// System.out.println("Second Tree set: "+t_set2);
// //comparison output in tree set
// TreeSet<String> result_set = new TreeSet<String>();
// for (String element : t_set1){
// System.out.println(t_set2.contains(element) ? "Yes" : "No");
// }
System.out.println("Exercise 9");
/*
Напишите программу на Java для поиска чисел меньше 7 в древовидном наборе.
*/
// // creating TreeSet
// TreeSet <Integer>tree_num = new TreeSet<Integer>();
// TreeSet <Integer>treeheadset = new TreeSet<Integer>();
//
// // Add numbers in the tree
// tree_num.add(1);
// tree_num.add(2);
// tree_num.add(3);
// tree_num.add(5);
// tree_num.add(6);
// tree_num.add(7);
// tree_num.add(8);
// tree_num.add(9);
// tree_num.add(10);
//
// // Find numbers less than 7
// treeheadset = (TreeSet)tree_num.headSet(7);
//
// // create an iterator
// Iterator iterator;
// iterator = treeheadset.iterator();
//
// //Displaying the tree set data
// System.out.println("Tree set data: ");
// while (iterator.hasNext()){
// System.out.println(iterator.next() + " ");
// }
System.out.println("Exercise 10");
/*
Напишите программу на Java, чтобы получить элемент в наборе деревьев, который больше или равен заданному элементу.
*/
//Мой вариант
// TreeSet <Integer> tset = new TreeSet <Integer>();
//
// tset.add(10);
// tset.add(20);
// tset.add(30);
// tset.add(40);
// tset.add(50);
//
// System.out.println("The TreeSet:\n" + tset);
//
// int value = tset.ceiling(25);
//
// System.out.println("Ceiling value for 25: " + value);
//Вариант 2
// // creating TreeSet
// TreeSet <Integer>tree_num = new TreeSet<Integer>();
// TreeSet <Integer>treeheadset = new TreeSet<Integer>();
//
// // Add numbers in the tree
// tree_num.add(10);
// tree_num.add(22);
// tree_num.add(36);
// tree_num.add(25);
// tree_num.add(16);
// tree_num.add(70);
// tree_num.add(82);
// tree_num.add(89);
// tree_num.add(14);
//
// System.out.println("Greater than or equal to 86 : "+tree_num.ceiling(86));
// System.out.println("Greater than or equal to 29 : "+tree_num.ceiling(29));
}
} |
#include <SoftwareSerial.h>
#include <ESP8266WiFi.h>
#include <WiFiClientSecure.h>
#include <UniversalTelegramBot.h>
#include <ArduinoJson.h>
SoftwareSerial mega(4,5);
// Wifi network station credentials
#define WIFI_SSID "Ray"
#define WIFI_PASSWORD "12345678"
// Telegram BOT Token (Get from Botfather)
#define CHAT_ID "1379411172"
#define BOT_TOKEN "5191068410:AAHe_Uj236bwSj9cY7q80UTlQIJdPm1FnsU"
const unsigned long BOT_MTBS = 1000; // mean time between scan messages
X509List cert(TELEGRAM_CERTIFICATE_ROOT);
WiFiClientSecure secured_client;
UniversalTelegramBot bot(BOT_TOKEN, secured_client);
unsigned long bot_lasttime; // last time messages' scan has been done
String perintah = "";
String data;
char c;
String dataMega = "";
String arrMega[4];
int indexMega = 0;
bool dataTampil = false;
int led1 = 12;
int led2 = 14;
void setup() {
pinMode(led1, OUTPUT);
pinMode(led2, OUTPUT);
Serial.begin(9600);
mega.begin(9600);
Serial.println();
// attempt to connect to Wifi network:
Serial.print("Connecting to Wifi SSID ");
Serial.print(WIFI_SSID);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
secured_client.setTrustAnchors(&cert); // Add root certificate for api.telegram.org
while (WiFi.status() != WL_CONNECTED)
{
Serial.print(".");
delay(500);
digitalWrite(led1, LOW);
digitalWrite(led2, HIGH);
}
Serial.print("\nWiFi connected. IP address: ");
Serial.println(WiFi.localIP());
Serial.print("Retrieving time: ");
configTime(0, 0, "pool.ntp.org"); // get UTC time via NTP
time_t now = time(nullptr);
while (now < 24 * 3600)
{
Serial.print(".");
delay(100);
now = time(nullptr);
}
Serial.println(now);
bot.sendMessage(CHAT_ID, "Bot started up", "");
}
void handleNewMessages(int numNewMessages)
{
Serial.print("handleNewMessages ");
Serial.println(numNewMessages);
for (int i = 0; i < numNewMessages; i++)
{
String chat_id = bot.messages[i].chat_id;
String text = bot.messages[i].text;
String from_name = bot.messages[i].from_name;
if (from_name == "")
from_name = "Guest";
if (text == "/motor1on"){
perintah = "motor1on";
mega.println(perintah);
bot.sendMessage(chat_id, "Motor 1 ON", "");
}else if (text == "/motor1off"){
perintah = "motor1off";
mega.println(perintah);
bot.sendMessage(chat_id, "Motor 1 OFF", "");
}else if (text == "/motor2on"){
perintah = "motor2on";
mega.println(perintah);
bot.sendMessage(chat_id, "Motor 2 ON", "");
}else if (text == "/motor2off"){
perintah = "motor2off";
mega.println(perintah);
bot.sendMessage(chat_id, "Motor 2 OFF", "");
}else if (text == "/motor3on"){
perintah = "motor3on";
mega.println(perintah);
bot.sendMessage(chat_id, "Motor 3 ON", "");
}else if (text == "/motor3off"){
perintah = "motor3off";
mega.println(perintah);
bot.sendMessage(chat_id, "Motor 3 OFF", "");
}else if (text == "/lampuon"){
perintah = "lampuon";
mega.println(perintah);
bot.sendMessage(chat_id, "Lampu ON", "");
}else if (text == "/lampuoff"){
perintah = "lampuoff";
mega.println(perintah);
bot.sendMessage(chat_id, "Lampu OFF", "");
}else if (text == "/monitor"){
perintah = "monitor";
mega.println(perintah);
dataTampil = true;
}else if (text == "/start"){
String welcome = "Selamat Datang.\n";
welcome += "Ini Adalah Sistem Kami.\n\n";
welcome += "/motor1on : hidup Motor 1\n";
welcome += "/motor1off : mati Motor 1\n";
welcome += "/motor2on : hidup Motor 2\n";
welcome += "/motor2off : mati Motor 2\n";
welcome += "/motor3on : hidup Motor 3\n";
welcome += "/motor3off : mati Motor 3\n";
welcome += "/lampuon : hidup Lampu\n";
welcome += "/lampuoff : mati Lampu\n";
welcome += "/monitor : Ambil Data\n";
bot.sendMessage(chat_id, welcome, "Markdown");
}
}
}
void loop() {
digitalWrite(led1, HIGH);
digitalWrite(led2, LOW);
if (millis() - bot_lasttime > BOT_MTBS)
{
int numNewMessages = bot.getUpdates(bot.last_message_received + 1);
while (numNewMessages)
{
Serial.println("got response");
handleNewMessages(numNewMessages);
numNewMessages = bot.getUpdates(bot.last_message_received + 1);
}
bot_lasttime = millis();
}
while(mega.available()>0){
c = char(mega.read());
data += c;
}
data.trim();
if(data != ""){
bot.sendMessage(CHAT_ID, String(data), "Markdown");
}
if(dataTampil == true){
bot.sendMessage(CHAT_ID, String(data), "Markdown");
}
// delay(500);
data = "";
dataTampil = false;
} |
// Function to format the date and time
function formatTime(date) {
var utcDate = date + 'Z';
var dateTime = new Date(utcDate);
var formattedTime = dateTime.toLocaleTimeString('da-DK', {
hour: '2-digit',
minute: '2-digit',
hour12: false,
timeZone: 'Europe/Copenhagen'
});
return formattedTime;
}
// Function to format the date only
function formatDate(dateTimeString) {
var dateTime = new Date(dateTimeString);
var year = dateTime.getFullYear();
var month = ('0' + (dateTime.getMonth() + 1)).slice(-2);
var day = ('0' + dateTime.getDate()).slice(-2);
return year + '/' + month + '/' + day;
}
// Event delegation for book-btn click events
$(document).on('click', '.book-btn', function () {
var selectedTime = $(this).data('time');
var hiddenDate = $(this).closest('.list-group-item').find('.hidden-date').text();
var fullDateTimeValue = $(this).closest('.list-group-item').data('datetime');
// Add the selected appointment to the "Selected Appointments" list
$('#selected-appointments').append(
'<li class="list-group-item" data-datetime="' + fullDateTimeValue + '">' + hiddenDate + ' - ' + selectedTime +
' <button class="btn btn-danger btn-sm cancel-btn"><i class="fas fa-times"></i> Fjern</button></li>'
);
// Check the number of selected appointments
var selectedAppointmentsCount = $('#selected-appointments li').length;
// Enable or disable the confirm booking button based on the count
if (selectedAppointmentsCount > 0) {
$('.confirm-booking-btn').prop('disabled', false);
} else {
$('.confirm-booking-btn').prop('disabled', true);
}
});
// Event handler for cancel buttons
$('#selected-appointments').on('click', '.cancel-btn', function () {
// Remove the canceled appointment from the list
$(this).closest('li').remove();
// Check the number of selected appointments after removal
var selectedAppointmentsCount = $('#selected-appointments li').length;
// Enable or disable the confirm booking button based on the count
if (selectedAppointmentsCount > 0) {
$('.confirm-booking-btn').prop('disabled', false);
} else {
$('.confirm-booking-btn').prop('disabled', true);
}
});
// Loop through API response and add times to list for display
function processApiResponse(apiResponse) {
$('#available-times').empty();
if (apiResponse.error !== undefined) {
var errorItem = $('<li>', {
class: 'list-group-item d-flex justify-content-between align-items-center',
html: '<div class="">' + "Ugyldig dato eller dato er før i dag" + '</div>'
});
$('#available-times').append(errorItem);
return;
}
// Iterate through the apiResponse array and append each element to the ul as li items
$.each(apiResponse, function (index, item) {
var startTime = formatTime(item.timeStart);
var endTime = formatTime(item.timeEnd);
var date = formatDate(item.timeStart);
var availableStubs = item.availableStubIds.length;
var todaysDate = new Date();
var [hours, minutes] = startTime.split('.');
var [year, month, day] = date.split('/');
var specificDate = new Date(year, month - 1, day);
specificDate.setHours(parseInt(hours), parseInt(minutes), 0, 0);
var buttonClass = 'btn btn-primary btn-sm book-btn';
var buttonText = 'Book';
// Check if availableStubs is 0, then disable the button and change its color to danger
if (availableStubs === 0 || specificDate < todaysDate) {
buttonClass = 'btn btn-danger btn-sm book-btn disabled';
buttonText = 'Booket';
}
var listItem = $('<li>', {
class: 'list-group-item d-flex justify-content-between align-items-center',
html: '<div class="hidden-date" style="display:none;">' + date + '</div>' +
startTime + ' - ' + endTime +
'<div class="">' +
'<small class="text-muted">' + availableStubs + ' tider ledige</small>' +
'</div>' +
'<button class="' + buttonClass + '" data-time="' + startTime + ' - ' + endTime + '">' +
'<i class="fas fa-plus"></i> ' + buttonText +
'</button>',
'data-datetime': item.timeStart
});
$('#available-times').append(listItem);
});
}
// Saves reserved timeslots to localstorage
function saveAppointmentsToLocalStorage() {
var appointments = [];
// Loop through each selected appointment in the list
$('#selected-appointments li').each(function () {
var appointmentText = $(this).text().trim();
// Trim the last 6 characters
appointmentText = appointmentText.slice(0, -6);
// Find the index of the first hyphen ("-")
var firstHyphenIndex = appointmentText.indexOf('-');
// Extract date and time based on the first hyphen index
var hiddenDate = appointmentText.substr(0, firstHyphenIndex).trim();
var selectedTime = appointmentText.substr(firstHyphenIndex + 1).trim();
var dateTime = $(this).data('datetime');
// Create an object for each appointment
var appointment = {
date: hiddenDate,
time: selectedTime,
datetime: dateTime
};
// Push the appointment object to the array
appointments.push(appointment);
});
// Convert the array to a JSON string and save it to localStorage
localStorage.setItem('selectedAppointments', JSON.stringify(appointments));
}
// Initialize datepicker
$('.datepicker').datepicker({
"format": 'dd/mm/yyyy',
"todayHighlight": true,
"weekStart": 1,
"autoclose": true,
"calendarWeeks": true
}).datepicker("setDate", 'now'); |
//
// UserController.swift
// HypeCloudKit
//
// Created by Maxwell Poffenbarger on 2/6/20.
// Copyright © 2020 Maxwell Poffenbarger. All rights reserved.
//
import Foundation
import CloudKit
class UserController {
//MARK: - Properties
let publicDB = CKContainer.default().publicCloudDatabase
static let sharedInstance = UserController()
var currentUser: User?
//MARK: - CRUD Functions
func createUserWith(username: String, completion: @escaping (_ result: Result<User?, UserError>) -> Void) {
fetchAppleUserReference { (reference) in
guard let reference = reference else {return completion(.failure(.noUserLoggedIn))}
let newUser = User(username: username, appleUserRef: reference)
let record = CKRecord(user: newUser)
self.publicDB.save(record) { (record, error) in
if let error = error {
print(error, error.localizedDescription)
completion(.failure(.ckError(error)))
}
guard let record = record,
let savedUser = User(ckRecord: record)
else {return completion(.failure(.couldNotUnwrap))}
self.currentUser = savedUser
print("created user: \(record.recordID.recordName) successfully")
completion(.success(savedUser))
}
}
//_191733211b868f89a77a006f267d7024
}
func fetchUser(completion: @escaping (Result<User?, UserError>) -> Void) {
fetchAppleUserReference { (reference) in
guard let reference = reference else {return completion(.failure(.noUserLoggedIn))}
let predicate = NSPredicate(format: "%K == %@", argumentArray:[UserStrings.appleUserRefKey, reference])
let query = CKQuery(recordType: UserStrings.userRecordTypeKey, predicate: predicate)
self.publicDB.perform(query, inZoneWith: nil) { (records, error) in
if let error = error {
print(error, error.localizedDescription)
completion(.failure(.ckError(error)))
}
guard let record = records?.first,
let foundUser = User(ckRecord: record) else {return completion(.failure(.couldNotUnwrap))}
print("fetched user successfully")
completion(.success(foundUser))
}
}
}
private func fetchAppleUserReference(completion: @escaping (_ reference: CKRecord.Reference?) -> Void) {
CKContainer.default().fetchUserRecordID { (recordID, error) in
if let error = error {
print(error, error.localizedDescription)
completion(nil)
}
if let recordID = recordID {
let reference = CKRecord.Reference(recordID: recordID, action: .deleteSelf)
completion(reference)
}
}
}
func updateUser() {
}
func deleteUser() {
}
}//End of class |
/* ************************************************************************** */
/* */
/* ::: :::::::: */
/* ft_substr.c :+: :+: :+: */
/* +:+ +:+ +:+ */
/* By: truby <[email protected]> +#+ +:+ +#+ */
/* +#+#+#+#+#+ +#+ */
/* Created: 2020/11/13 00:16:41 by truby #+# #+# */
/* Updated: 2021/04/27 01:08:44 by truby ### ########.fr */
/* */
/* ************************************************************************** */
#include "libft.h"
char *ft_substr(char const *s, unsigned int start, size_t len)
{
char *b;
size_t z;
z = 0;
if (s == 0)
return (NULL);
if (start >= ft_strlen(s))
len = 0;
b = (char *)malloc(sizeof(char) * (len + 1));
if (b == NULL)
return (NULL);
while (z < len)
{
b[z] = s[start];
z++;
start++;
}
b[z] = '\0';
return (b);
} |
import React, { useEffect, useState } from 'react'
import useAuth from '../../hooks/useAuth'
import { FaArrowLeft } from "react-icons/fa6";
import axios from "axios"
import toast, { Toaster } from 'react-hot-toast';
import useDownloadPdf from '../../hooks/useDownloadPdf';
function ViewReport({ reportId, onHandleViewNote }) {
const [report, setReport] = useState([])
const handleDownload = (pdfUrl) => {
useDownloadPdf(pdfUrl)
}
useEffect(() => {
axios.get(`/v1/report/${reportId}`)
.then((res) => {
console.log(res.data.data)
setReport(res.data.data)
})
.catch((err) => {
console.log(err);
})
}, [])
return (
<div className="justify-center items-center flex overflow-x-hidden overflow-y-auto fixed inset-0 z-50 outline-none focus:outline-none bg-gray-600 bg-opacity-60 backdrop-filter backdrop-blur-lg">
<div className="relative w-[80%] bg-white rounded-md " >
<div className='flex justify-between items-center p-2'>
<FaArrowLeft size={40} className="hover:bg-[#f5f5f5] cursor-pointer p-2 rounded-full" onClick={() => onHandleViewNote()} />
</div>
<div className='grid sm:grid-cols-2 grid-cols-1 gap-2 bg-white p-5 pt-0 rounded-lg w-full '>
<div className='flex-1 rounded-lg sm:w-[500px] sm:h-[500px] bg-[#f5f5f5] overflow-hidden'>
{/* <img src={`${report?.coverImage?.url}`} alt="" className='sm:hidden block' /> */}
<embed src={`${report?.pdfFile?.url}#toolbar=0`} className="sm:block hidden overflow-auto " type="application/pdf" width="103%" height="103%" />
</div>
<div className='bg-[#f5f5f5] rounded-lg p-5 flex flex-col gap-5 justify-between'>
<div className='flex flex-col gap-3'>
<h1 className='font-semibold text-md '><span className='text-lg font-semibold text-black'>Title : </span>{report?.title}</h1>
<h1> <span className='text-lg font-semibold text-black'>Description :</span>{report?.description}</h1>
<h1> <span className='text-lg font-semibold text-black'>Report Date :</span> {`${report?.reportType}`}</h1>
</div>
<div className='mx-auto'>
<button className='bg-blue-500 text-white px-3 py-2 rounded-md ' onClick={() => handleDownload(report?.pdfFile?.url)}>Download</button>
</div>
</div>
</div>
</div>
</div>
)
}
export default ViewReport |
using Microsoft.AspNetCore.Authentication.JwtBearer;
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Mvc;
using Microsoft.EntityFrameworkCore;
using Microsoft.IdentityModel.Tokens;
using MongoDB.Driver;
using System.Text;
using wellbeing_of_mind.Infastructure;
using wellbeing_of_mind.Domain;
var builder = WebApplication.CreateBuilder(args);
// CORS configuration
builder.Services.AddCors(options =>
{
options.AddPolicy("AllowOrigin", builder =>
{
builder.WithOrigins("http://localhost:3000")
.AllowAnyHeader()
.AllowAnyMethod();
});
});
// MongoDB configuration
builder.Services.AddSingleton<IMongoClient>(_ =>
{
string connectionString = Environment.GetEnvironmentVariable("MongoDB");
var settings = MongoClientSettings.FromConnectionString(connectionString);
settings.ServerApi = new ServerApi(ServerApiVersion.V1);
return new MongoClient(settings);
});
builder.Services.AddScoped<IMongoDatabase>(_ =>
{
var client = _.GetService<IMongoClient>();
if (client == null)
{
Console.WriteLine("MongoClient is null");
throw new Exception("Null");
}
return client.GetDatabase("articles");
});
// AutoMapper
builder.Services.AddAutoMapper(typeof(Program));
// Cache configuration
builder.Services.AddMemoryCache();
// Configure caching profiles
builder.Services.AddControllers(configure =>
configure.CacheProfiles.Add("Any-60",
new CacheProfile
{
Location = ResponseCacheLocation.Any,
Duration = 60
}));
// JWT authentication configuration
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
string jwtKey = Environment.GetEnvironmentVariable("JWT_KEY");
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = builder.Configuration["Jwt:Issuer"],
ValidAudience = builder.Configuration["Jwt:Audience"],
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(jwtKey))
};
});
builder.Services.AddAuthorization(options =>
{
options.AddPolicy("RequireAdminRole", policy =>
policy.RequireRole("Admin"));
options.AddPolicy("RequireModeratorRole", policy =>
policy.RequireRole("Moderator"));
options.AddPolicy("RequireRole", policy =>
policy.RequireRole("User", "Moderator", "Admin"));
});
// SQL Server
builder.Services.AddDbContext<UsersDbContext>((serviceProvider, options) =>
{
var connectionString = builder.Configuration.GetConnectionString("DefaultConnection");
options.UseSqlServer(connectionString);
});
builder.Services.AddIdentity<User, IdentityRole>(options =>
{
options.Password.RequireDigit = true;
options.Password.RequireLowercase = true;
options.Password.RequireUppercase = true;
options.Password.RequireNonAlphanumeric = true;
options.Password.RequiredLength = 8;
options.Lockout.DefaultLockoutTimeSpan = TimeSpan.FromMinutes(15);
options.Lockout.MaxFailedAccessAttempts = 5;
options.User.RequireUniqueEmail = true;
options.SignIn.RequireConfirmedAccount = false;
})
.AddEntityFrameworkStores<UsersDbContext>()
.AddDefaultTokenProviders()
.AddRoles<IdentityRole>();
async Task SeedRoles(RoleManager<IdentityRole> roleManager)
{
var roles = new List<string> { "Admin", "Moderator", "User" };
foreach (var role in roles)
{
if (!await roleManager.RoleExistsAsync(role))
{
await roleManager.CreateAsync(new IdentityRole(role));
}
}
}
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
using var scope = app.Services.CreateScope();
var roleManager = scope.ServiceProvider.GetRequiredService<RoleManager<IdentityRole>>();
await SeedRoles(roleManager);
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler();
}
app.UseHttpsRedirection();
app.UseRouting();
app.UseCors("AllowOrigin");
app.UseAuthentication();
app.UseAuthorization();
app.MapControllers();
app.UseResponseCaching();
app.Run(); |
import { Container, Image, Table } from "react-bootstrap";
import NavbarComponent from "../components/Navbar";
import { exIngredients } from "../examplemeals";
import { ButtonComponent } from "../components/ButtonComponent";
import IngredientFormModal from "../components/IngredientFormModal";
import { useState } from "react";
export const isExpired = (expiredate: Date) => {
const today = new Date();
if (expiredate < today) {
return true;
}
return false;
};
export const IngredientsListPage = () => {
const [show, setShow] = useState(false);
const handleClose = () => setShow(false);
const handleShow = () => setShow(true);
return (
<>
<Container>
<NavbarComponent />
<Container className="ingredients-picture">
<Image
fluid={true}
alt=""
src="/src/assets/ingredients.jpg"
width="920px"
height="auto"
/>
</Container>
<Container>
<Container className="ingredient-header">
<Container className="meal-info-text">
<h1 className="ingredients-h1"> Ingredients</h1>
<p className="ingredients-text">
Here you can find all the ingredients you have in your pantry.
</p>
</Container>
<Container className="ingredient-button">
<ButtonComponent onClick={handleShow}>
{" "}
Update Ingredients
</ButtonComponent>
</Container>
</Container>
<Container className="meal-info">
<IngredientFormModal show={show} handleClose={handleClose} />
<Table striped bordered hover size="sm">
<thead className="table-headings">
<tr>
<th>Ingredient</th>
<th>Quantity</th>
<th>Expiry date</th>
</tr>
</thead>
<tbody className="ingredients-text">
{exIngredients.map((ingredient) => (
<tr>
<td>{ingredient.name}</td>
<td>
{ingredient.quantity} {ingredient.unit}
</td>
<td
className={
isExpired(ingredient.expiryDate)
? "expired-text"
: "ingredients-text"
}
>
{ingredient.expiryDate.toLocaleDateString()}
</td>
</tr>
))}
</tbody>
</Table>
</Container>
</Container>
</Container>
</>
);
};
export default IngredientsListPage; |
// Copyright 2021-2024 The Connect Authors
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package connect
import (
"net/http"
"net/http/httptest"
"testing"
"connectrpc.com/connect/internal/assert"
)
func TestErrorWriter(t *testing.T) {
t.Parallel()
t.Run("RequireConnectProtocolHeader", func(t *testing.T) {
t.Parallel()
writer := NewErrorWriter(WithRequireConnectProtocolHeader())
t.Run("Unary", func(t *testing.T) {
req := httptest.NewRequest(http.MethodPost, "http://localhost", nil)
req.Header.Set("Content-Type", connectUnaryContentTypePrefix+codecNameJSON)
assert.False(t, writer.IsSupported(req))
req.Header.Set(connectHeaderProtocolVersion, connectProtocolVersion)
assert.True(t, writer.IsSupported(req))
})
t.Run("UnaryGET", func(t *testing.T) {
req := httptest.NewRequest(http.MethodGet, "http://localhost", nil)
assert.False(t, writer.IsSupported(req))
query := req.URL.Query()
query.Set(connectUnaryConnectQueryParameter, connectUnaryConnectQueryValue)
req.URL.RawQuery = query.Encode()
assert.True(t, writer.IsSupported(req))
})
t.Run("Stream", func(t *testing.T) {
req := httptest.NewRequest(http.MethodPost, "http://localhost", nil)
req.Header.Set("Content-Type", connectStreamingContentTypePrefix+codecNameJSON)
assert.True(t, writer.IsSupported(req)) // ignores WithRequireConnectProtocolHeader
req.Header.Set(connectHeaderProtocolVersion, connectProtocolVersion)
assert.True(t, writer.IsSupported(req))
})
})
t.Run("Protocols", func(t *testing.T) {
t.Parallel()
writer := NewErrorWriter() // All supported by default
t.Run("ConnectUnary", func(t *testing.T) {
req := httptest.NewRequest(http.MethodPost, "http://localhost", nil)
req.Header.Set("Content-Type", connectUnaryContentTypePrefix+codecNameJSON)
assert.True(t, writer.IsSupported(req))
})
t.Run("ConnectUnaryGET", func(t *testing.T) {
req := httptest.NewRequest(http.MethodGet, "http://localhost", nil)
assert.True(t, writer.IsSupported(req))
})
t.Run("ConnectStream", func(t *testing.T) {
req := httptest.NewRequest(http.MethodPost, "http://localhost", nil)
req.Header.Set("Content-Type", connectStreamingContentTypePrefix+codecNameJSON)
assert.True(t, writer.IsSupported(req))
})
t.Run("GRPC", func(t *testing.T) {
req := httptest.NewRequest(http.MethodPost, "http://localhost", nil)
req.Header.Set("Content-Type", grpcContentTypeDefault)
assert.True(t, writer.IsSupported(req))
req.Header.Set("Content-Type", grpcContentTypePrefix+"json")
assert.True(t, writer.IsSupported(req))
})
t.Run("GRPCWeb", func(t *testing.T) {
req := httptest.NewRequest(http.MethodPost, "http://localhost", nil)
req.Header.Set("Content-Type", grpcWebContentTypeDefault)
assert.True(t, writer.IsSupported(req))
req.Header.Set("Content-Type", grpcWebContentTypePrefix+"json")
assert.True(t, writer.IsSupported(req))
})
})
t.Run("UnknownCodec", func(t *testing.T) {
// An Unknown codec should return supported as the protocol is known and
// the error codec is agnostic to the codec used. The server can respond
// with a protocol error for the unknown codec.
t.Parallel()
writer := NewErrorWriter()
unknownCodec := "invalid"
t.Run("ConnectUnary", func(t *testing.T) {
req := httptest.NewRequest(http.MethodPost, "http://localhost", nil)
req.Header.Set("Content-Type", connectUnaryContentTypePrefix+unknownCodec)
assert.True(t, writer.IsSupported(req))
})
t.Run("ConnectStream", func(t *testing.T) {
req := httptest.NewRequest(http.MethodPost, "http://localhost", nil)
req.Header.Set("Content-Type", connectStreamingContentTypePrefix+unknownCodec)
assert.True(t, writer.IsSupported(req))
})
t.Run("GRPC", func(t *testing.T) {
req := httptest.NewRequest(http.MethodPost, "http://localhost", nil)
req.Header.Set("Content-Type", grpcContentTypePrefix+unknownCodec)
assert.True(t, writer.IsSupported(req))
})
t.Run("GRPCWeb", func(t *testing.T) {
req := httptest.NewRequest(http.MethodPost, "http://localhost", nil)
req.Header.Set("Content-Type", grpcWebContentTypePrefix+unknownCodec)
assert.True(t, writer.IsSupported(req))
})
})
} |
import PySimpleGUI as sg
import tkinter as tk
import pandas as pd
def registro(gastos):
border_style = {
"border_width": 2,
"relief": tk.SOLID
}
categorias = ('TRANSPORTE', 'COMIDAS Y BEBIDAS', 'INVERSION', 'VIAJES', 'ELECTRONICA', 'MEDICINA', 'OTROS')
sg.theme('LightBlue')
titulos_df = ['id', 'fecha', 'categoria', 'descripcion', 'valor']
titulos = ['-ID-','-FECHA-', '-CATEGORIA-', '-DESCRIPCION-', '-VALOR-']
if len(gastos['id']) < 1:
id = 1
else:
id = len(gastos['id']) + 1
layout = [
[sg.Text('NUEVO REGISTRO', justification='center', size=(300,1), font=('Courier', 18, 'bold', 'italic'), **border_style)],
[sg.Text('REGISTRO No', size=(36,1), justification='right', font=('Helvetica', 10, 'bold'), expand_x=True), sg.Input(default_text=id ,key='-ID-', size=(100,1), justification='left', expand_x=True, readonly=True)],
[sg.Text('FECHA '), sg.InputText(key='-FECHA-', expand_x=True), sg.CalendarButton("Calendario", target="-FECHA-", format="%d-%m-%Y")],
[sg.Text('CATEGORIA '), sg.Combo(values=categorias, expand_x=True, key='-CATEGORIA-', readonly=True)],
[sg.Text('DESCRIPCION'), sg.Input(key='-DESCRIPCION-', expand_x=True)],
[sg.Text('VALOR '), sg.Input(key='-VALOR-', expand_x=True)],
[sg.Button('GUARDAR',expand_x=True), sg.Button('LIMPIAR', expand_x=True), sg.Button('SALIR', expand_x=True)]
]
window = sg.Window('Nuevo registro', layout, size=(550,220))
while True:
event, values = window.read()
if event == sg.WIN_CLOSED or event == 'SALIR':
guardar(gastos)
break
if event == 'GUARDAR':
dict_values = dict(values)
dict_values = {clave: valor.upper() for clave, valor in dict_values.items() if clave != 'Calendario'}
valores = pd.Series(dict_values.values(), titulos_df)
gastos = pd.concat([gastos, valores.to_frame().T], ignore_index=True)
for i in titulos:
if i != '-ID-':
window[i].Update('')
id += 1
window['-ID-'].update(id)
window['-FECHA-'].set_focus()
sg.popup('El registro se ha guardado correctamente', title='Confirmacion')
if event == 'LIMPIAR':
for i in titulos:
if i != '-ID-':
window[i].Update('')
window['-FECHA-'].set_focus()
window.close()
return gastos
def guardar(data):
df = pd.DataFrame(data)
df.set_index('id', inplace=True)
df.to_csv('registros_gastos.csv', index=True) |
jest.mock('@ng-bootstrap/ng-bootstrap');
import { ComponentFixture, TestBed, inject, fakeAsync, tick } from '@angular/core/testing';
import { HttpResponse } from '@angular/common/http';
import { HttpClientTestingModule } from '@angular/common/http/testing';
import { of } from 'rxjs';
import { NgbActiveModal } from '@ng-bootstrap/ng-bootstrap';
import { MemberService } from '../service/member.service';
import MemberDeleteDialogComponent from './member-delete-dialog.component';
describe('Member Management Delete Component', () => {
let service: MemberService;
let mockActiveModal: NgbActiveModal;
let fixture: ComponentFixture<MemberDeleteDialogComponent>;
let component: MemberDeleteDialogComponent;
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientTestingModule, MemberDeleteDialogComponent],
providers: [NgbActiveModal],
})
.overrideTemplate(MemberDeleteDialogComponent, '')
.compileComponents();
service = TestBed.inject(MemberService);
mockActiveModal = TestBed.inject(NgbActiveModal);
fixture = TestBed.createComponent(MemberDeleteDialogComponent);
component = fixture.componentInstance;
});
describe('confirmDelete', () => {
it('Should call delete service on confirmDelete', inject(
[],
fakeAsync(() => {
jest.spyOn(service, 'delete').mockReturnValue(of(new HttpResponse({ body: {} })));
component.confirmDelete(123);
tick();
expect(service.delete).toHaveBeenCalledWith(123);
expect(mockActiveModal.close).toHaveBeenCalledWith('deleted');
}),
));
it('Should not call delete service on clear', () => {
jest.spyOn(service, 'delete');
component.cancel();
expect(service.delete).not.toHaveBeenCalled();
expect(mockActiveModal.close).not.toHaveBeenCalled();
expect(mockActiveModal.dismiss).toHaveBeenCalled();
});
});
}); |
import React, {useEffect, useState} from 'react';
import {ErrorMessage, Field, Form, Formik} from "formik";
import * as Yup from "yup";
import {Link, useNavigate, useParams} from "react-router-dom";
import * as homeService from "../../service/HomeService.jsx"
import {toast} from "react-toastify";
function ContractEdit() {
const headingStyle = {
fontSize: '1.5rem', // Font size for the heading
margin: '0', // Remove margin for the heading
letterSpacing: '1px', // Add slight letter-spacing for style
textTransform: 'uppercase', // Uppercase the heading text
fontWeight: 'bold', // Make the heading text bold
color: '#a6682d', // Adjust the text color to complement the background
textAlign: 'center'
};
const formCenter = {
marginTop: '100px', // Add margin-top
}
const [contract, setContract] = useState(null);
const {id} = useParams()
const navigate = useNavigate();
const findContractById = async (idContract) => {
const res = await homeService.findById(idContract, 2);
setContract(res);
}
const editContract = async (contract) => {
const res = await homeService.edit(contract, 2);
if (res.status === 200) {
navigate("/contract")
toast("Edit successfully")
} else {
toast.error("Edit Fail")
}
}
useEffect(() => {
findContractById(id)
}, [id]);
const validationSchema = {
contractNumber: Yup.string()
.required("Not Empty"),
startDate: Yup.string()
.required("Not Empty"),
endDate: Yup.string()
.required("Not Empty"),
totalPayment: Yup.number()
.required("Not Empty")
.min(1, "Must be > 0"),
depositAmount: Yup.number()
.required("Not Empty")
.min(1, "Must be > 0")
}
const initialValues = {
...contract
}
return (
contract && (
<>
<h3 style={headingStyle}>Edit Contract</h3>
<Formik initialValues={initialValues} onSubmit={(values) => {
editContract(values)
}} validationSchema={Yup.object(validationSchema)}
>
<Form className="container" style={formCenter}>
<div className="row">
<div className="col-md-6">
<div className="mb-3">
<label htmlFor="contractNumber" className="form-label">
Contract Number
</label>
<Field type="text" name="contractNumber" id="contractNumber"
className="form-control"/>
<ErrorMessage name="contractNumber" component="div" className="text-danger"/>
</div>
<div className="mb-3">
<label htmlFor="startDate" className="form-label">
Start Day
</label>
<Field type="date" name="startDate" id="startDate" className="form-control"/>
<ErrorMessage name="startDate" component="div" className="text-danger"/>
</div>
<div className="mb-3">
<label htmlFor="endDate" className="form-label">
End Day
</label>
<Field type="date" name="endDate" id="endDate" className="form-control"/>
<ErrorMessage name="endDate" component="div" className="text-danger"/>
</div>
</div>
<div className="col-md-6">
<div className="mb-3">
<label htmlFor="depositAmount" className="form-label">
Deposit Amount
</label>
<Field type="number" name="depositAmount" id="depositAmount"
className="form-control"/>
<ErrorMessage name="depositAmount" component="div" className="text-danger"/>
</div>
<div className="mb-3">
<label htmlFor="totalPayment" className="form-label">
Total Payment
</label>
<Field type="number" name="totalPayment" id="totalPayment"
className="form-control"/>
<ErrorMessage name="totalPayment" component="div" className="text-danger"/>
</div>
</div>
</div>
<button type="submit" className="btn btn-primary">
Submit
</button>
<Link to="/contract">
<button className="btn btn-primary">
Back to contract list
</button>
</Link>
</Form>
</Formik>
</>
)
);
}
export default ContractEdit; |
// react and next
import React, { useState } from 'react';
import getConfig from 'next/config';
import Link from 'next/link';
// material ui
import { DropzoneArea } from 'mui-file-dropzone';
import Typography from '@mui/material/Typography';
import Box from '@mui/material/Box';
import Stack from '@mui/material/Stack';
import { object } from 'yup';
import styles from '../../bulk-upload/index.module.scss';
// get public runtime settings
const {
publicRuntimeConfig: { maxFileSizeUpload }
} = getConfig();
// default dropzone text
const defaultDropzoneText = 'Drag & drop your Excel file here or click';
const UploadSpreadsheet = ({ onChange }) => {
// hooks
const [dropzoneText, setDropzoneText] = useState(defaultDropzoneText);
// this is to handle the file uploads
const handleFileUpload = (e) => {
if (e.length > 0) {
setDropzoneText(
`Your data spreadsheet is ready to be uploaded. Click on NEXT to validate your PDF files against data.`
);
onChange(e[0], 'spreadsheet');
}
};
// on file upload cancel
const onFileUploadCancel = () => {
setDropzoneText(defaultDropzoneText);
onChange(undefined, 'spreadsheet');
};
return (
<React.Fragment>
<div className={styles['form-step']}>
<Stack spacing={4} direction="column">
<Box component="div" whiteSpace="normal">
<Typography variant="body2" gutterBottom>
Please select the data spreadsheet (Microsoft Excel spreadsheet) that you want to upload to the system.
Make sure the files has the .xlsx extension and all data is correct and matches the PDF files selected in
the previous step. You can download a template in the following link,
<Link legacyBehavior href="/Soom-eIFU-Bulk-Upload-Template.xlsx" passHref>
<a target={'_blank'}>
<strong> Soom eIFU Bulk Upload Template.</strong>
</a>
</Link>
</Typography>
</Box>
<DropzoneArea
data-test-id="dropzoneSpreadSheet"
maxFileSize={maxFileSizeUpload}
filesLimit={1}
acceptedFiles={['.xlsx']}
alertSnackbarProps={{ anchorOrigin: { vertical: 'top', horizontal: 'right' } }}
useChipsForPreview
onChange={handleFileUpload}
onDelete={onFileUploadCancel}
dropzoneText={dropzoneText}
dropzoneClass={styles[`soom-dashboard-dropzone`]}
dropzoneParagraphClass={styles['dropzone-text']}
/>
</Stack>
</div>
</React.Fragment>
);
};
// override of props to support formik
UploadSpreadsheet.label = 'Data Spreadsheet';
UploadSpreadsheet.initialValues = {};
UploadSpreadsheet.validationSchema = object().shape({});
export default UploadSpreadsheet; |
/***************************************************************************
# Copyright (c) 2015-21, NVIDIA CORPORATION. All rights reserved.
#
# NVIDIA CORPORATION and its licensors retain all intellectual property
# and proprietary rights in and to this software, related documentation
# and any modifications thereto. Any use, reproduction, disclosure or
# distribution of this software and related documentation without an express
# license agreement from NVIDIA CORPORATION is strictly prohibited.
**************************************************************************/
import Utils.Math.PackedFormats;
struct PackedSurfaceData
{
uint3 pos;
uint depth;
uint normal;
uint faceNormal;
uint weightsAndLobes;
uint _pad;
}
/** Per-pixel surface data.
*/
struct SurfaceData
{
float3 pos; ///< Position in world-space.
float depth; ///< Depth from camera (-1 if invalid data).
float3 normal; ///< Shading normal in world-space.
float3 faceNormal; ///< Face normal in world-space.
float diffuseWeight; ///< Diffuse lobe weight.
float specularWeight; ///< Specular lobe weight.
float specularRoughness; ///< Specular lobe roughness (linear).
uint lobes; ///< BSDF lobes
static SurfaceData createFromPacked(const PackedSurfaceData packed)
{
SurfaceData surfaceData = {};
surfaceData.unpack(packed);
return surfaceData;
}
static SurfaceData createInvalid()
{
SurfaceData surfaceData = {};
surfaceData.depth = -1.f;
return surfaceData;
}
bool isValid()
{
return depth >= 0.f;
}
[mutating] void unpack(const PackedSurfaceData packed)
{
pos = asfloat(packed.pos);
depth = asfloat(packed.depth);
normal = decodeNormal2x16(packed.normal);
faceNormal = decodeNormal2x16(packed.faceNormal);
diffuseWeight = (packed.weightsAndLobes & 0xff) / float(0xff);
specularWeight = ((packed.weightsAndLobes >> 8) & 0xff) / float(0xff);
specularRoughness = ((packed.weightsAndLobes >> 16) & 0xff) / float(0xff);
lobes = ((packed.weightsAndLobes >> 24) & 0xff);
}
PackedSurfaceData pack()
{
PackedSurfaceData packed = {};
packed.pos = asuint(pos);
packed.depth = asuint(depth);
packed.normal = encodeNormal2x16(normal);
packed.faceNormal = encodeNormal2x16(faceNormal);
packed.weightsAndLobes = saturate(diffuseWeight) * 0xff;
packed.weightsAndLobes |= uint((saturate(specularWeight) * 0xff)) << 8;
packed.weightsAndLobes |= uint((saturate(specularRoughness) * 0xff)) << 16;
packed.weightsAndLobes |= (lobes << 24);
return packed;
}
};
uint packNormalDepth(float4 normalDepth)
{
return (encodeNormal2x8(normalDepth.xyz) << 16) | (f32tof16(normalDepth.w) & 0xffff);
}
float4 unpackNormalDepth(uint packed)
{
return float4(decodeNormal2x8(packed >> 16), f16tof32(packed));
}
/***************************************************************************
# Copyright (c) 2015-22, NVIDIA CORPORATION. All rights reserved.
#
# Redistribution and use in source and binary forms, with or without
# modification, are permitted provided that the following conditions
# are met:
# * Redistributions of source code must retain the above copyright
# notice, this list of conditions and the following disclaimer.
# * Redistributions in binary form must reproduce the above copyright
# notice, this list of conditions and the following disclaimer in the
# documentation and/or other materials provided with the distribution.
# * Neither the name of NVIDIA CORPORATION nor the names of its
# contributors may be used to endorse or promote products derived
# from this software without specific prior written permission.
#
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS "AS IS" AND ANY
# EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
# IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
# PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
# EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
# PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
# PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY
# OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
# (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
# OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
**************************************************************************/
//import Utils.Math.PackedFormats;
//import Utils.Math.FormatConversion;
//__exported import PackedTypes;
/** Stores data about primary hit points.
To reduce register pressure, some of the fields are stored in packed format
and decoded as being accessed through Slang properties.
*/
//struct SurfaceData
//{
// // The following fields are stored in PackedSurfaceData.
//
// float3 position; ///< Position in world-space.
// float viewDepth; ///< Distance from viewer to surface (negative for invalid surface).
// uint packedNormal; ///< Packed surface normal in world-space (octahedral mapping).
// uint packedWeights; ///< Packed diffuse/specular reflectance and roughness.
//
// // The following fields are *NOT* stored in PackedSurfaceData.
//
// float3 viewDir; ///< Direction to the viewer in world-space.
//
// // True if surface data is valid.
// property bool valid
// {
// get { return viewDepth >= 0.f; }
// }
//
// // Shading normal.
// property float3 normal
// {
// get { return decodeNormal2x16(packedNormal); }
// set { packedNormal = encodeNormal2x16(newValue); }
// }
//
// // Diffuse reflectance.
// property float diffuse
// {
// get { return unpackUnorm8(packedWeights); }
// set { packedWeights = (packedWeights & ~0xff) | packUnorm8(newValue); }
// }
//
// // Specular reflectance.
// property float specular
// {
// get { return unpackUnorm8(packedWeights >> 8); }
// set { packedWeights = (packedWeights & ~0xff00) | (packUnorm8(newValue) << 8); }
// }
//
// // Specular roughness.
// property float roughness
// {
// get { return unpackUnorm8(packedWeights >> 16); }
// set { packedWeights = (packedWeights & ~0xff0000) | (packUnorm8(newValue) << 16); }
// }
//
// // Diffuse lobe probability.
// property float diffuseProb
// {
// get { return unpackUnorm8(packedWeights >> 24); }
// set { packedWeights = (packedWeights & ~0xff000000) | (packUnorm8(newValue) << 24); }
// }
//
// /** Initialize an invalid surface.
// */
// __init()
// {
// this = {};
// viewDepth = -1.f;
// }
//
// /** Initialize a surface.
// \param[in] packed Packed surface data.
// \param[in] viewDir View direction.
// */
// __init(const PackedSurfaceData packed, const float3 viewDir)
// {
// position = asfloat(packed.position);
// viewDepth = asfloat(packed.viewDepth);
// packedNormal = packed.normal;
// packedWeights = packed.weights;
// this.viewDir = viewDir;
// }
//
// /** Pack surface data.
// */
// PackedSurfaceData pack()
// {
// PackedSurfaceData packed = {};
// packed.position = asuint(position);
// packed.viewDepth = asuint(viewDepth);
// packed.normal = packedNormal;
// packed.weights = packedWeights;
// return packed;
// }
//}; |
/**
* Product controllers for handler request
* @module controllers/orderControllers
*/
import { Request, Response, NextFunction } from "express";
import { IProduct } from "../index.type";
import ProductService from "../services/product.service";
/**
* product services for all crud operation in db
* @const
*/
const productService = new ProductService();
/**
* create product controller
* @async
* @param req
* @param res
* @param next
*/
export async function createProductController(
req: Request,
res: Response,
next: NextFunction
) {
try {
const { id: userId } = req.params;
const data = req.body;
const product = await productService.create(userId, data);
res.status(200).json(product);
} catch (error) {
next(error);
}
}
/**
* get all products controller
* @async
* @param _req
* @param res
* @param next
*/
export async function getProductsController(
_req: Request,
res: Response,
next: NextFunction
) {
try {
const products = await productService.findAll();
res.status(200).json(products);
} catch (error) {
next(error);
}
}
/**
* get products controller
* @async
* @param req
* @param res
* @param next
*/
export async function getProductController(
req: Request,
res: Response,
next: NextFunction
) {
try {
const { id } = req.params;
const product = await productService.findOne(id);
res.status(200).json(product);
} catch (error) {
next(error);
}
}
/**
* product update controller
* @async
* @param req
* @param res
* @param next
*/
export async function updateProductController(
req: Request,
res: Response,
next: NextFunction
) {
try {
const { id } = req.params;
const data: Partial<IProduct> = req.body;
const productUpdated = await productService.update(id, data);
res.status(201).json(productUpdated);
} catch (error) {
next(error);
}
}
/**
* delete product controller
* @async
* @param req
* @param res
* @param next
*/
export async function productDeleteController(
req: Request,
res: Response,
next: NextFunction
) {
try {
const { id } = req.params;
const rta = await productService.delete(id);
res.status(201).json(rta);
} catch (error) {
next(error);
}
}
/**
* add category to product
* @param req
* @param res
* @param next
*/
export async function addCategoryToProductController(
req: Request,
res: Response,
next: NextFunction
) {
try {
const { id } = req.params;
const { id: categoryId } = req.body;
const rta = await productService.addCategory(id, categoryId);
res.status(201).json(rta);
} catch (error) {
next(error);
}
}
/**
* remove category from product
* @param req
* @param res
* @param next
*/
export async function removeCategoryToProductController(
req: Request,
res: Response,
next: NextFunction
) {
try {
const { id } = req.params;
const { id: categoryId } = req.body;
const rta = await productService.removeCategory(id, categoryId);
res.status(201).json(rta);
} catch (error) {
next(error);
}
} |
package com.raven.database.entity
import androidx.room.ColumnInfo
import androidx.room.Embedded
import androidx.room.Entity
import androidx.room.PrimaryKey
@Entity(tableName = "articles")
data class ArticleDB(
@PrimaryKey
@ColumnInfo("id")
val id: Long,
@ColumnInfo("url")
val url: String,
@ColumnInfo("section")
val section: String,
@ColumnInfo("title")
val title: String,
@ColumnInfo("published_date")
val publishedDate: String,
// @Embedded(prefix = "media_")
// val media: ArticleMediaDB,
@ColumnInfo("caption")
val caption: String,
@ColumnInfo("images", typeAffinity = ColumnInfo.BLOB)
val images: ByteArray
) {
override fun equals(other: Any?): Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as ArticleDB
if (id != other.id) return false
if (url != other.url) return false
if (section != other.section) return false
if (title != other.title) return false
if (publishedDate != other.publishedDate) return false
if (caption != other.caption) return false
if (!images.contentEquals(other.images)) return false
return true
}
override fun hashCode(): Int {
var result = id.hashCode()
result = 31 * result + url.hashCode()
result = 31 * result + section.hashCode()
result = 31 * result + title.hashCode()
result = 31 * result + publishedDate.hashCode()
result = 31 * result + caption.hashCode()
result = 31 * result + images.contentHashCode()
return result
}
}
data class ArticleMediaDB(
@ColumnInfo("caption")
val caption: String,
@ColumnInfo("images", typeAffinity = ColumnInfo.BLOB)
val images: ByteArray,
@ColumnInfo("url")
val url: String
) {
override fun equals(other: Any?): Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as ArticleMediaDB
if (caption != other.caption) return false
if (!images.contentEquals(other.images)) return false
return true
}
override fun hashCode(): Int {
var result = caption.hashCode()
result = 31 * result + images.contentHashCode()
return result
}
} |
package com.example.nitro;
import static android.content.ContentValues.TAG;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.content.pm.ActivityInfo;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.view.Window;
import android.widget.Button;
import android.widget.Toast;
import com.google.android.gms.auth.api.signin.GoogleSignIn;
import com.google.android.gms.auth.api.signin.GoogleSignInAccount;
import com.google.android.gms.auth.api.signin.GoogleSignInClient;
import com.google.android.gms.auth.api.signin.GoogleSignInOptions;
import com.google.android.gms.common.api.ApiException;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import com.google.firebase.auth.AuthCredential;
import com.google.firebase.auth.AuthResult;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.auth.FirebaseUser;
import com.google.firebase.auth.GoogleAuthProvider;
import com.google.firebase.database.DataSnapshot;
import com.google.firebase.database.DatabaseError;
import com.google.firebase.database.FirebaseDatabase;
import com.google.firebase.database.Query;
import com.google.firebase.database.ValueEventListener;
import java.util.HashMap;
public class SignInActivity extends AppCompatActivity {
Button googleAuthBtn;
// FirebaseAuth auth;
FirebaseDatabase database;
GoogleSignInClient mGoogleSignInClient;
int RC_SIGN_IN = 20;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
this.setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
this.requestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_sign);
//sign out
if(UserInformation.auth != null){
UserInformation.auth.signOut();
if(UserInformation.userId != "none"){
Toast.makeText(SignInActivity.this, "Signed out", Toast.LENGTH_SHORT).show();
}
}
UserInformation.reset();
//auth setup
googleAuthBtn = findViewById(R.id.btnAuth);
UserInformation.auth = FirebaseAuth.getInstance();
database = FirebaseDatabase.getInstance();
GoogleSignInOptions gso = new GoogleSignInOptions.Builder(GoogleSignInOptions.DEFAULT_SIGN_IN)
.requestIdToken("1064019779857-4oflalcqbl1uab2ohfk757lf6d8slotp.apps.googleusercontent.com")
.requestEmail().build();
mGoogleSignInClient = GoogleSignIn.getClient(this, gso);
googleAuthBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
googleSignIn();
}
});
if(database == null){
Log.d("database", "Something is really wrong with the database handling...");
}
}
private void googleSignIn(){
Intent intent = mGoogleSignInClient.getSignInIntent();
startActivityForResult(intent, RC_SIGN_IN);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if(requestCode == RC_SIGN_IN){
Task<GoogleSignInAccount> task = GoogleSignIn.getSignedInAccountFromIntent(data);
try{
GoogleSignInAccount account = task.getResult(ApiException.class);
firebaseAuth(account.getIdToken());
}
catch (Exception e){
Toast.makeText(this, e.getMessage(), Toast.LENGTH_SHORT).show();
}
}
}
private void firebaseAuth(String idToken) {
AuthCredential credential = GoogleAuthProvider.getCredential(idToken, null);
UserInformation.auth.signInWithCredential(credential)
.addOnCompleteListener(new OnCompleteListener<AuthResult>() {
@Override
public void onComplete(@NonNull Task<AuthResult> task) {
if (task.isSuccessful()){
FirebaseUser user = UserInformation.auth.getCurrentUser();
HashMap<String, Object> map = new HashMap<>();
map.put("id", user.getUid());
map.put("name", user.getDisplayName());
map.put("profile", user.getPhotoUrl().toString());
map.put("leaderboardValue", 0);
map.put("themeId", 0);
boolean isNew = false;
if(database.getReference().child("users").child(user.getUid()) == null){
database.getReference().child("users").child(user.getUid()).setValue(map);
UserInformation.leaderboardValue = 0;
isNew = true;
}
UserInformation.userId = user.getUid();
UserInformation.fullName = user.getDisplayName();
UserInformation.photoUrl = user.getPhotoUrl().toString();
if(!isNew){
Query query = database.getReference().child("users").child(UserInformation.userId);
query.addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
if (dataSnapshot.exists()) {
UserData data;
try{
data = dataSnapshot.getValue(UserData.class);
UserInformation.leaderboardValue = data.leaderboardValue;
UserInformation.themeId = data.themeId;
}
catch(Exception e){
Log.d("userValue", "can't read user data correctly");
}
}
}
@Override
public void onCancelled(DatabaseError databaseError) {
}
});
}
Intent intent = new Intent(SignInActivity.this, Game.class);
startActivity(intent);
}
else{
Toast.makeText(SignInActivity.this, "Something went wrong...", Toast.LENGTH_SHORT).show();
}
}
});
}
public void asGuest(View view){
UserInformation.userId = "none";
UserInformation.fullName = "Guest";
UserInformation.photoUrl = "https://github.com/TimLaszlo004/szoftech-elm-let/blob/main/default.jpg";
Intent i = new Intent(this, Game.class);
//finish();
startActivity(i);
}
} |
from claseEmpleado import Empleado
class Contratado(Empleado):
__fechaInicio: str
__fechaFinalizacion: str
__cantHrsTrabajadas: float
__valorXhr: float
def __init__(self, dni=None, nombre=None, direccion=None, telefono=None, fechaInicio=None,
fechaFinalizacion = None,cantHrsTrabajadas=None, valorXhr=None):
super().__init__(dni, nombre, direccion, telefono)
self.__fechaInicio = fechaInicio
self.__fechaFinalizacion = fechaFinalizacion
self.__cantHrsTrabajadas = float(cantHrsTrabajadas)
self.__valorXhr = float(valorXhr)
def mostrarContratado(self):
print(f"{self.getNombre()} {self.getDNI()} {self.getDireccion()} {self.getTelefono()} {self.__fechaInicio} {self.__fechaFinalizacion} {self.__cantHrsTrabajadas} {self.__valorXhr}")
def modificarHoras(self,cantHoras):
self.__cantHrsTrabajadas += cantHoras
def sueldo(self):
return(self.__cantHrsTrabajadas*self.__valorXhr) |
<main class="form-signin w-100 m-auto">
<form [formGroup]="registerForm">
<img class="mb-1" src="/assets/img/logo.png" alt="Logo" width="150">
<h2 class="h2 mb-2 fw-normal">EtecBook</h2>
<h5 class="mb-3 fw-normal">Criar sua Conta!!!</h5>
<div class="form-floating mb-2">
<input formControlName="name" type="text" class="form-control" id="name"
placeholder="Nome" [class.is-invalid]="checkName()">
<label for="name"><i class='bx bxs-user'></i> Nome</label>
<span *ngIf="checkName()" class="text-danger text-start d-block mt-1">*Campo Obrigatório</span>
</div>
<div class="form-floating mb-2">
<input formControlName="email" type="email" class="form-control" id="email"
placeholder="Email" [class.is-invalid]="checkEmail() || checkEmailValid()">
<label for="email"><i class='bx bxs-envelope'></i> Email</label>
<span *ngIf="checkEmail()" class="text-danger text-start d-block mt-1">*Campo Obrigatório</span>
<span *ngIf="checkEmailValid()" class="text-danger text-start d-block mt-1">*Informe um Email Válido</span>
</div>
<div class="form-floating">
<input formControlName="password" type="password" class="form-control" id="password"
placeholder="Senha" [class.is-invalid]="checkPassword() || checkPasswordLength()">
<label for="password"><i class='bx bxs-lock'></i> Senha</label>
<span *ngIf="checkPassword()" class="text-danger text-start d-block mt-1">*Campo Obrigatório</span>
<span *ngIf="checkPasswordLength()" class="text-danger text-start d-block mt-1">*A Senha deve possuir no mínimo 6 caracteres</span>
</div>
<button (click)="onSubmit()" class="w-100 btn btn-lg btn-primary my-2" type="submit">Criar Conta</button>
<div class="border-text"></div>
<a routerLink="/login" class="w-100 btn btn-lg btn-success">Entrar</a>
<p class="mt-4 mb-3 text-body-secondary">© 2023</p>
</form>
</main> |
import { Box, Button, Flex, VisuallyHidden } from '@chakra-ui/react';
import { useMemo } from 'react';
import { PaginationInfo } from '../modules/shared/common.types';
type PaginationProps = PaginationInfo & {
onPrev: () => void;
onNext: () => void;
};
export function Pagination({ onPrev, onNext, page, pageCount }: PaginationProps) {
const shouldShowPrevious = useMemo(() => pageCount > 1 && page > 1, [pageCount, page]);
const shouldShowNext = useMemo(() => pageCount > 1 && page < pageCount, [pageCount, page]);
return (
<Flex>
<Box w="10">
{shouldShowPrevious && (
<Button onClick={onPrev} background={'white'}>
{'<'}
<VisuallyHidden>Previous page</VisuallyHidden>
</Button>
)}
</Box>
<Flex w="10" justifyContent="center" alignItems="center" fontWeight="bold">
{page}
</Flex>
<Box w="10">
{shouldShowNext && (
<Button onClick={onNext} background={'white'}>
<VisuallyHidden>Next page</VisuallyHidden>
{'>'}
</Button>
)}
</Box>
</Flex>
);
} |
/**
* @file Parking.h
* @author Jamie Brown/Luis Howell
* @brief
* @version 0.1
* @date 2019-02-17
*
* @copyright Copyright (c) 2019
*
*/
#ifndef PARKING_H
#define PARKING_H
#include <QWidget>
#include <QPushButton>
#include <QLabel>
#include <QTimer>
#include <QElapsedTimer>
#include <QSoundEffect>
#include <QObject>
#include "types.h"
#include <vector>
/**
* @brief Top level widget for parking screen. Displays parking sensors and reversing camera.
*
*/
class Parking : public QWidget
{
Q_OBJECT
public:
/**
* @brief Construct a new Parking object
*
* @param parent
*/
explicit Parking(QWidget *parent = 0);
public slots:
/**
* @brief
*
*/
void StateChangeMainMenu();
/**
* @brief recieves the sensor distance signals and updates the sensor visualisation
*
* @param msg Struct of sensor distances
*/
void SensorRx(sensorDist_t* msg);
private slots:
void parking_beep();
signals:
/**
* @brief
*
* @param req_state
* @param currentView
*/
void DisplayChange(state_t req_state, QWidget* currentView);
private:
/**
* @brief Create a Layout object
*
*/
void CreateLayout ();
QPushButton *m_homeButton;
QLabel *viz_label;
QSoundEffect *beep_effect;
QTimer* m_timer;
QElapsedTimer *beep_timer;
int beep_delay=0;
double smallest_dist=0;
bool rearLeftConnected=false;
bool rearRightConnected=false;
bool rearCentreConnected=false;
bool frontLeftConnected=false;
bool frontRightConnected=false;
bool frontCentreConnected=false;
double rearLeft=0;
double rearRight=0;
double rearCentre=0;
double frontLeft=0;
double frontRight=0;
double frontCentre=0;
/**
* @brief Stores the current smallest ultrasonic distance
*
*/
void calc_smallest_dist();
/**
* @brief Calculates the delay between beeps from the smallest ultrasonic distance
*
*/
void calc_beep_delay();
/**
* @brief Round a float up or down to the nearest integer
*
* @param input Float value to round
* @return int Rounded integer value
*/
int round_float(float input);
/**
* @brief Maps an ultrasonic distance to a pixel value
*
* @param input_dist A float containing a sensor distance between 0-2.0m
* @return int A value between 0-80 pixels
*/
int dist2pix(float input_dist);
/**
* @brief Converts degrees to radians
*
* @param deg A float value in degrees
* @return float The value in radians
*/
float deg2rad(float deg);
/**
* @brief Calculates the pixel coordinates of a line
*
* @param centre_x X pixel coordinate of centre of line
* @param centre_y Y pixel coordinate of centre of line
* @param bar_width Length of the line in pixels
* @param angle Angle of line
* @return vector<int> Four element vector of line points in order: x1, y1, x2, y2
*/
std::vector<int> calc_line_coor(int centre_x, int centre_y, int bar_width, int angle);
protected:
void paintEvent(QPaintEvent *event);
/**
* @brief Draws ultrasonic sensor visulisation
*
* @param qp QPainter object
*/
void draw_sensor_lines(QPainter *qp);
};
#endif // PARKING_H |
/*
Albums vs Individual Tracks
The Chinook store is setup in a way that allows customer to make purchases in one of the two ways:
purchase a whole album
purchase a collection of one or more individual tracks.
The store does not let customers purchase a whole album, and then add individual tracks to that same purchase (unless they do that by choosing each track manually).
When customers purchase albums they are charged the same price as if they had purchased each of those tracks separately.
Management are currently considering changing their purchasing strategy to save money. The strategy they are considering is to purchase only the most popular tracks from each album from record companies, instead of purchasing every track from an album.
We have been asked to find out what percentage of purchases are individual tracks vs whole albums, so that management can use this data to understand the effect this decision might have on overall revenue.
It is very common when you are performing an analysis to have 'edge cases' which prevent you from getting a 100% accurate answer to your question. In this instance, we have two edge cases to consider:
Albums that have only one or two tracks are likely to be purchased by customers as part of a collection of individual tracks.
Customers may decide to manually select every track from an album, and then add a few individual tracks from other albums to their purchase.
In the first case, since our analysis is concerned with maximizing revenue we can safely ignore albums consisting of only a few tracks. The company has previously done analysis to confirm that the second case does not happen often, so we can ignore this case also.
In order to answer the question, we're going to have to identify whether each invoice has all the tracks from an album. We can do this by getting the list of tracks from an invoice and comparing it to the list of tracks from an album.
We can find the album to compare the purchase to by looking up the album that one of the purchased tracks belongs to.
It doesn't matter which track we pick, since if it's an album purchase, that album will be the same for all tracks.
Community discussion
Write a query that categorizes each invoice as either an album purchase or not, and calculates the following summary statistics:
Number of invoices
Percentage of invoices
Write one to two sentences explaining your findings, and making a prospective recommendation on whether the Chinook store should continue to buy full albums from record companies.
*/
/* We generalize the problem by allowing invoices with both albums and individual tracks. */
/*
Each invoice may or may not include any complete albums, and we would like to find out.
1. For each invoice, figure out all the tracks in it and their associated albums
2. For each album, count the number of tracks in it
2. Compare the tracks in the invoice with the tracks in the associated albums by counting */
/* count the number of tracks in each album */
CREATE TABLE Album_Track AS
SELECT *, count(track.track_id) AS AlbumTrackCount
FROM track
LEFT JOIN album
ON track.album_id = album.album_id
GROUP BY album.album_id;
/* Count the number of tracks in each invoice for each album */
Create Table Invoice_Album AS
SELECT i.invoice_id as InvoiceID,
a.album_id as AlbumID,
count(t.track_id) as InvoiceTrackCount,
AlbumTrackCount
FROM invoice i
LEFT JOIN invoice_line il
ON i.invoice_id = il.invoice_id
LEFT JOIN track t
ON il.track_id = t.track_id
LEFT JOIN Album_Track a
ON t.album_id = a.album_id
GROUP BY InvoiceID, AlbumID;
/* Check if each invoice contains at least one complete album */
CREATE TABLE Album_Purchases AS
SELECT InvoiceID, sum(Complete_Album_Or_Not) AS Albums_Purchased
FROM (
SELECT *,
CASE
WHEN AlbumTrackCount > InvoiceTrackCount THEN 0
ELSE 1
END AS Complete_Album_Or_Not
FROM Invoice_Album
)
GROUP BY InvoiceID;
WITH Total_Number_Of_Invoices AS
(
SELECT count(*)*1.0 AS num_invoices
FROM invoice
)
/* Calculate the Percentage of Invoices with Complete Albums */
SELECT
sum(IF_Albums_Purchased) / (SELECT num_invoices FROM Total_Number_Of_Invoices) * 100
As Percentage_Of_Invoices_With_Album_Purchases
FROM
/* an invoice contains a complete album (regardless of how many) is marked 1 otherwise 0 */
(SELECT *,
CASE
WHEN Albums_Purchased > 0 Then 1
ELSE 0
END AS IF_Albums_Purchased
FROM Album_Purchases); |
# SpaceshipTitanic_TensorFlow_RandomForest
This notebook walks you through how to train a baseline Random Forest model using TensorFlow Decision Forests on the Spaceship Titanic dataset made available for Kaggle competition.
The code will look as follows:
```
import tensorflow_decision_forests as tfdf
import pandas as pd
dataset = pd.read_csv("project/dataset.csv")
tf_dataset = tfdf.keras.pd_dataframe_to_tf_dataset(dataset, label="my_label")
model = tfdf.keras.RandomForestModel()
model.fit(tf_dataset)
print(model.summary())
```
Decision Forests are a family of tree-based models including Random Forests and Gradient Boosted Trees. They are the best place to start when working with tabular data, and will often outperform (or provide a strong baseline) before you begin experimenting with neural networks. |
# Claw Full Stack Developer Intern Interview Task
## Task Submission 23 May 2024
Provided a links to postman API used, Live project links and my GitHub repository containing the source code for both the front-end and back-end. Additionally, include instructions on how to set up and run the application locally.
- Frontend Link: https://b00kflow.netlify.app/
- Backend Link: https://book-management-system-silk.vercel.app/
- GitHub Link: https://github.com/nandk4552/book-management-system
- POSTMAN API's: https://www.postman.com/grey-station-125149-1/workspace/claw-full-stack-internship-nandk4552-gmail-com/collection/21207467-008baac2-f8ff-497b-b6ac-4a9354f182ba?action=share&creator=21207467
## Contact Details
```
Name: DEVARLA NAND KISHORE
Email: [email protected]
LinkedIn: https://www.linkedin.com/in/nandk4552/
Twitter: https://x.com/nandk_1
```
## Project Overview
This project is a full-stack web application that allows users to manage a collection of books. It includes both front-end and back-end components, built using React.js, Node.js, MongoDB, and REST APIs.
### Features
1. **Book Management User Interface (Front-End):**
- All Books are visible in the home page
- Each user can add, view, edit, and delete books on their books only.
- Each book has the following fields: title, author, genre, and year published.
- The application is responsive and works well on different screen sizes.
2. **Integration with Back-End (Node.js, Express):**
- API calls are made to the back-end to fetch, add, update, and delete book data.
- Axios or the Fetch API is used to handle HTTP requests.
3. **API Endpoints (Back-End):**
- RESTful API endpoints are set up:
_Authentication Routes_
- REGISTER USER || POST || `api/v1/auth/register`
- LOGIN USER|| POST || `api/v1/auth/login`
_User Routes_
- GET USER || POST || api/v1/user/get-user
- GET ALL USERS || POST || api/v1/user/all-users
- UPDATE USER || POST || api/v1/user/update-user
- UPDATE PASSWORD || POST || api/v1/user/update-user
- RESET USER PASSWORD || POST || api/v1/user/reset-password
- DELETE USER || POST || `api/v1/user/delete-user`
_Book Routes_
- GET ALL BOOKS || GET || `/api/v1/books`
- GET ALL BOOKS BY LOGGED IN USER ID || GET || `/api/v1/books/get-by-user`
- GET BOOK BY ID || GET || `/api/v1/books/:id`
- CREATE A NEW BOOK || POST || `/api/v1/books`
- UPDATE A BOOK BY ID || PUT || `/api/v1/books/:id`
- DELETE A BOOK BY ID || DELETE || `/api/v1/books/:id`
- FILTER FOR BOOK GENRES || GET || `/api/v1/books/filter/genres`
- FILTER FOR BOOK AUTHORS || GET ||` /api/v1/books/filter/authors`
- FILTER FOR BOOK TITLES || GET ||` /api/v1/books/filter/titles`
4. **Data Validation and Error Handling (Back-End):**
- Incoming data for book fields is validated.
- Errors are handled gracefully, returning appropriate HTTP status codes and messages.
5. **Database Integration (MongoDB):**
- MongoDB is used to store book data and user data.
- Mongoose models define data schema and interaction.
## Getting Started
1. Clone this repository to your local machine.
2. Install dependencies for both the front-end and back-end:
- Front-End (React.js): Navigate to the `client` directory and run `npm install`.
- Back-End (Node.js): Navigate to the `server` directory and run `npm install`.
3. Set up your MongoDB database and configure the connection in the back-end.
4. Run the application:
- Front-End: In the `client` directory, run `npm run dev`.
- Back-End: In the root directory i.e book-management-system, run `npm run server`.
- Or else to run both applications run `npm run dev` in the root directory i.e book-management-system.
## Bonus Task
implemented the following features for additional points:
- User authentication using JWT to protect certain API endpoints.
- Search and filter functionality for books.
- Pagination for the list of books using ant design Table component. |
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet"
href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<link rel="stylesheet" href="/resources/css/userMng/userMngList.css" />
<!-- notoSans -->
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link
href="https://fonts.googleapis.com/css2?family=Noto+Sans&display=swap"
rel="stylesheet">
<link
href="https://fonts.googleapis.com/css2?family=Noto+Sans&family=Noto+Sans+KR&display=swap"
rel="stylesheet">
<script src="https://code.jquery.com/jquery-3.4.1.js"></script>
<meta charset="UTF-8">
<title>점포수정</title>
</head>
<style>
input{
width: auto;
}
#notice{
margin: 40px;}
.form-btn {
display: unset;}
.category{
font-size: 1.2em;
}
.register-msg>li {
height: 10px;
width: 283px;
margin: auto;
}
.register-msg {
margin: 0px;
height: 8px;
padding: 0;
list-style:none;
color: red;
}
</style>
<body>
<section id="notice" class="notice">
<div class="container2">
<div class="category">점포수정</div>
<hr style="border: solid 1.2px; width: 97%;">
<form action="/store/update" method="post" id="storeForm"
onsubmit="return submitForm()" style="margin-top:20px">
<table id="search-box">
<tr>
<th>지점명</th>
<td><input type="text" id="storeNm" name="storeNm"
value="${store.storeNm}" required>
<input type="hidden" value="${store.storeNm}"
id="storeNmHidden"></input> <!-- 숨겨진 필드 추가 --> <input
type="button" name="strnm" value="중복확인" class="check"
id="name-check-btn" required></td>
<th>점포연락처</th>
<td><input type="text" id="storeTelNo" name="storeTelNo"
oninput="hypenTel(this)" maxlength="13"
value="${store.storeTelNo}" placeholder="하이픈(-)은 자동입력 됩니다.."></td>
</tr>
<tr>
<th>좌표</th>
<td><input class="field" id="lat" name="latitude"
value="${store.latitude}" size="12" />, <input class="field" id="lng"
name="longitude" value="${store.longitude}" size="12"></td>
<th>사용여부</th>
<td><select name="useYn">
<option value="Y">Y</option>
<option value="N">N</option>
</select></td>
</tr>
<tr>
<th colspan="1">주소</th>
<td colspan="3"><input type="text" id="address_kakao" name="storeAddr"
placeholder="클릭해주세요" value="${store.storeAddr}" size="40" readonly />, <input
type="text" name="storeAddrDtl" id="storeAddrDtl"
value="${store.storeAddrDtl}" required /></td>
</tr>
<tr>
<th colspan="1">지역분류</th>
<td colspan="3">
<input type="hidden" id="dtl" value="${store.locationCd}"><select id="location" name="locationCd" size="1" onchange="changeLocationCd()">
<option value="">지역선택</option>
<c:forEach var="list" items="${list}">
<option value="${list.locationCd}"
${store.locationCd == list.locationCd ? 'selected' : ''}>${list.locationNm}</option>
</c:forEach>
</select> <select id="locationSeoul" name="locationCdSeoul" size="1" >
<option value="">지역선택 상세</option>
</select></td>
</tr>
</table>
<div id="staticMap" style="width: 700px; height: 400px; margin: 20px auto;"></div>
<div class="updatecancle" style="text-align: center;">
<ul class="register-msg" id="msg" style="display:none;">
<li id="userPwdConfirmMsg">중복확인이 필요합니다.</li>
</ul>
<input type="hidden" name="storeSeq" value="${store.storeSeq}" style="">
<button type="submit" name="save" class="form-btn" value="저장"
id="submitBtn" style="display: inline-block; margin-left: 5px; margin-right: 5px; margin-top:20px">저장</button>
<button type="button" class="form-btn"
value="취소" onclick="history.back()" style="display: inline-block; margin-left: 5px; margin-right: 5px; margin-top:20px">취소</button>
</div>
</form>
</div>
</section>
<!-- Google Maps API -->
<script
src="https://maps.googleapis.com/maps/api/js?key=AIzaSyAY5-GHYNs9VdzbExAcRYn5NPHIsu727XI&libraries=places&callback=initAutocomplete"
async defer></script>
<!-- Daum Postcode API -->
<script
src="//t1.daumcdn.net/mapjsapi/bundle/postcode/prod/postcode.v2.js"></script>
<script>
window.onload = function() {
document.getElementById("address_kakao").addEventListener("click", function() {
new daum.Postcode({
oncomplete: function(data) {
document.getElementById("address_kakao").value = data.address;
document.querySelector("input[name=storeAddrDtl]").focus();
updateLatLng();
}
}).open();
});
var dtlCd = $("#dtl").val();
console.log(dtlCd);
// 정규 표현식 패턴
var pattern = /\d+/;
// 정규 표현식과 일치하는 부분 찾기
var matches = dtlCd.match(pattern);
if (matches) {
// 정규 표현식과 일치하는 첫 번째 숫자 얻기
var locCd = matches[0];
/* $("#dtl").val()=locCd; */
console.log(locCd);
var selectElement = document.getElementById('location');
// select 요소의 option 요소를 모두 가져옵니다.
var options = selectElement.getElementsByTagName('option');
// 각 option 요소를 확인하며 선택 여부를 결정합니다.
for (var i = 0; i < options.length; i++) {
var option = options[i];
var locationCd = option.value;
// store.locationCd와 hiddenValue를 비교하여 선택 여부를 결정합니다.
if (locationCd == locCd) {
option.selected = true;
} else {
option.selected = false;
}
}} else{
var locCd = $("#location").val();
}
$.ajax({
type : "GET", // 타입 (get, post, put 등등)
url : "/search-location-code", // 요청할 서버url
dataType : "json", // 데이터 타입 (html, xml, json, text 등등)
data : {
'locCd' : locCd,
},
success : function(data) { // 결과 성공 콜백함수
changeLosctionDtlCd(data);
}
});
}
function changeLosctionDtlCd(data){
var dtlLocation= $("#dtl").val();
var locOption=$("select[name=locationCdSeoul]");
var content='<option value="">지역 상세 선택</option>';
for(var i=0;i<data.length;i++){
content +='<option value="' + data[i].grpCdDtlId + '" ' + (dtlLocation == data[i].grpCdDtlId ? 'selected' : '') + '>' + data[i].grpCdDtlNm + '</option>';
}
locOption.html(content);
}
//대분류 지역 선택한 값에 맞춰서 소분류 지역 값 조회
function changeLocationCd(){
var locCd = $("#location").val();
console.log(locCd);
$.ajax({
type : "GET", // 타입 (get, post, put 등등)
url : "/search-location-code", // 요청할 서버url
dataType : "json", // 데이터 타입 (html, xml, json, text 등등)
data : {
'locCd' : locCd,
},
success : function(data) { // 결과 성공 콜백함수
changeLosctionDtlCd(data);
}
});
}
function updateLatLng() {
var address = document.getElementById("address_kakao").value;
var geocoder = new google.maps.Geocoder();
geocoder.geocode({
'address': address
}, function(results, status) {
if (status === google.maps.GeocoderStatus.OK) {
var lat = results[0].geometry.location.lat();
var lng = results[0].geometry.location.lng();
document.getElementById("lat").value = lat;
document.getElementById("lng").value = lng;
} else {
console.log('Geocode was not successful for the following reason: ' + status);
}
});
}
const hypenTel = function(target) {
if (!target) {
return "";
}
const value = target.value.replace(/[^0-9]/g, ""); // 숫자 이외의 모든 문자를 제거
let result = [];
let restNumber = "";
// 지역번호와 나머지 번호로 나누기
if (value.startsWith("02")) {
// 서울 02 지역번호
result.push(value.substr(0, 2));
restNumber = value.substring(2);
if (restNumber.length == 8) {
// 4자리 이상인 경우
result.push(restNumber.substring(0, 4));
result.push(restNumber.substring(4));
} else if (7 >= restNumber.length) {
// 4자리 이상인 경우
result.push(restNumber.substring(0, 3));
result.push(restNumber.substring(3));
} else {
// 4자리 미만인 경우
result.push(restNumber.substring(0, 4));
result.push(restNumber.substring(4));
}
} else if (value.startsWith("1")) {
// 지역 번호가 없는 경우
// 1xxx-yyyy
restNumber = value;
if (restNumber.length >= 4) {
// 4자리 이상인 경우
result.push(restNumber.substring(0, 4));
result.push(restNumber.substring(4));
} else {
// 4자리 미만인 경우
result.push(restNumber);
}
} else {
// 나머지 3자리 지역번호
// 0xx-yyyy-zzzz
result.push(value.substr(0, 3));
restNumber = value.substring(3);
if (restNumber.length == 8) {
// 4자리 이상인 경우
result.push(restNumber.substring(0, 4));
result.push(restNumber.substring(4));
} else if (7 >= restNumber.length) {
// 4자리 이상인 경우
result.push(restNumber.substring(0, 3));
result.push(restNumber.substring(3));
} else {
// 4자리 미만인 경우
result.push(restNumber.substring(0, 4));
result.push(restNumber.substring(4));
}
}
const hyphenatedValue = result.filter(function(val) {
return val;
}).join("-");
// 입력 필드에 결과 설정
target.value = hyphenatedValue;
}
var con = document.getElementById("msg");
const storeNmHidden = document.querySelector("#storeNmHidden");
const storeNm = document.querySelector("#storeNm");
const submitBtn = document.querySelector("#submitBtn");
storeNm.addEventListener("input", function() {
if (storeNm.value === storeNmHidden.value) {
submitBtn.disabled = false; // Enable the submit button
msg.style.display="none";
} else {
submitBtn.disabled = true; // Disable the submit button
msg.style.display="block";
}
});
const nameCheckBtn = document.querySelector("#name-check-btn");
nameCheckBtn.addEventListener("click", function() {
const storeNm = document.getElementById("storeNm").value;
if (!storeNm) {
alert("점포명을 입력해주세요.");
return;
}
$.ajax({
async: true,
type: 'POST',
url: "${pageContext.request.contextPath}/user/namecheck",
data: JSON.stringify(storeNm),
dataType: "json",
contentType: "application/json; charset=UTF-8",
success: function (check) {
if (check == "1") {
alert("사용 가능한 점포명입니다.");
// Update the hidden field value
document.getElementById("storeNmHidden").value = storeNm;
// Check if storeNm and storeNmHidden are equal
if (storeNm === storeNmHidden.value) {
submitBtn.disabled = false;
msg.style.display="none";// Enable the submit button
} else {
submitBtn.disabled = true; // Disable the submit button
msg.style.display="block";
}
} else {
alert("이미 존재하는 점포명입니다.");
document.getElementById("storeNmHidden").value = storeNm;
// Check if storeNm and storeNmHidden are equal
if (storeNm === storeNmHidden.value) {
submitBtn.disabled = false;
msg.style.display="none";// Enable the submit button
} else {
submitBtn.disabled = true; // Disable the submit button
msg.style.display="block";
}
}
},
error: function (error) {
console.error("에러 발생:", error);
alert("서버와의 통신 중 오류가 발생했습니다.");
msg.style.display="block";
}
});
});
function submitForm() {
var form = document.getElementById("storeForm");
storeNm.value = storeNm.value.replace(/,/g, '');
console.log(storeNm);
$.ajax({
type: "POST",
url: form.getAttribute("action"),
data: $(form).serialize(),
contentType: "application/x-www-form-urlencoded; charset=UTF-8",
success: function (response) {
var Close = confirm("저장이 완료되었습니다. 창을 닫으시겠습니까?");
console.log(storeNm);
if (Close) {
window.opener.location.href = "/store";
window.close();
}
},
error: function (error) {
console.error("AJAX 요청 실패:", error);
alert("빈칸을 모두 입력해주세요.");
}
});
return false;
}
</script>
<script type="text/javascript" src="//dapi.kakao.com/v2/maps/sdk.js?appkey=8ebfc94bdd15e21c9b4d64159a004634"></script>
<script type="text/javascript">
function initializeKakaoMap() {
kakao.maps.load(function () {
// Kakao 지도 초기화 코드
var markerPosition = new kakao.maps.LatLng(${store.latitude}, ${store.longitude});
var marker = {
position: markerPosition
};
var staticMapContainer = document.getElementById('staticMap');
var staticMapOption = {
center: new kakao.maps.LatLng(${store.latitude}, ${store.longitude}),
level: 3,
marker: marker
};
var staticMap = new kakao.maps.StaticMap(staticMapContainer, staticMapOption);
});
}
// Kakao 지도 스크립트 로드 후 호출되도록 이벤트 핸들러 추가
window.addEventListener('load', initializeKakaoMap);
</script>
</body>
</html> |
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'package:rss_interactive_v2/constants/color_constants.dart';
import 'package:rss_interactive_v2/models/coin_model.dart';
class CoinCard extends StatelessWidget {
final CoinModel coinModel;
const CoinCard({super.key, required this.coinModel});
@override
Widget build(BuildContext context) {
return SizedBox(
height: 80,
width: Get.width,
child: Row(
children: [
SizedBox(
width: 80,
child: Container(
padding: const EdgeInsets.all(15),
child: Image.network(coinModel.image!)),
),
SizedBox(
width: (Get.width * 3) / 4,
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container(
width: Get.width / 4,
alignment: Alignment.centerLeft,
child: Text(
"${coinModel.symbol!.toUpperCase()}/USDT",
style: const TextStyle(
color: ColorConstants.white,
fontWeight: FontWeight.bold,
fontSize: 15),
),
),
Container(
alignment: Alignment.centerLeft,
width: Get.width / 4,
child: Text(coinModel.name!,
style: const TextStyle(
color: ColorConstants.unselectedItemColor,
fontSize: 13)),
),
],
),
const SizedBox(
width: 10,
),
Container(
width: Get.width / 5,
alignment: Alignment.center,
child: Text(
coinModel.currentPrice.toStringAsFixed(2),
style: const TextStyle(
color: ColorConstants.white,
fontWeight: FontWeight.bold,
fontSize: 15),
),
),
const SizedBox(
width: 10,
),
Container(
alignment: Alignment.centerRight,
width: Get.width / 5,
child: Container(
height: 40,
width: 75,
decoration: BoxDecoration(
color: coinModel.priceChangePercentage24H >= 0
? Colors.green
: Colors.red,
borderRadius: BorderRadius.circular(10)),
padding: const EdgeInsets.all(10),
alignment: Alignment.center,
child: Text(
"% ${coinModel.priceChangePercentage24H.toStringAsFixed(2)}",
style: const TextStyle(color: ColorConstants.white,fontWeight: FontWeight.w500)),
),
)
],
),
),
)
],
),
);
}
} |
import React, {useEffect} from 'react';
import {AppDispatch, RootState} from '../../store/types';
import {connect} from 'react-redux';
import {FlatList, StyleSheet, View} from 'react-native';
import {ISetsDispatchProps, ISetsProps, ISetsStateProps} from './types';
import {
getAllSets,
getIsSetsRecordsLoaded,
} from '../../store/slices/pokemonSets/selector';
import {getAllSetsThunk} from '../../store/slices/pokemonSets/thunks';
import {SetListItem} from '../../components/setListItem/setListItem';
import {colors} from '../../constants/colors';
import {HeaderBar} from '../../components/headerBar/headerBar';
import {Loader} from '../../components/loader/loader';
const Sets = (props: ISetsProps) => {
useEffect(() => {
props.onLoadData();
// eslint-disable-next-line react-hooks/exhaustive-deps
}, []);
return props.isSetsLoaded ? (
<View style={styles.container}>
<HeaderBar title="Sets" />
<FlatList
data={props.sets}
renderItem={({item}) => <SetListItem set={item} />}
keyExtractor={item => item.id}
extraData={props.sets}
horizontal={false}
numColumns={2}
/>
</View>
) : (
<Loader />
);
};
const styles = StyleSheet.create({
container: {
backgroundColor: colors.WHITE,
alignItems: 'center',
},
});
const mapStateToProps = (state: RootState): ISetsStateProps => ({
sets: getAllSets(state),
isSetsLoaded: getIsSetsRecordsLoaded(state),
});
const mapDispatchToProps = (dispatch: AppDispatch): ISetsDispatchProps => ({
onLoadData: () => {
dispatch(getAllSetsThunk());
},
});
export default connect(mapStateToProps, mapDispatchToProps)(Sets); |
import {
ActionFunctionArgs,
redirect,
useActionData,
useLoaderData,
} from "react-router-dom";
import { getUsers } from "../helpers/api/getUsers";
import { createPost } from "../helpers/api/createPost";
import { NewPostType } from "../types/newPostType";
import { FormErrorsType } from "../types/formErrorsType";
import PostForm from "../components/PostForm";
import { PostType } from "../types/postType";
import { UserType } from "../types/userType";
import postFormValidator from "../helpers/validation/postFormValidator";
async function loader({
request: { signal },
}: {
request: { signal: AbortSignal };
}) {
const users = getUsers({ signal });
return { users: await users };
}
async function action(args: ActionFunctionArgs) {
const { signal } = args.request;
const formData = await args.request.formData();
const userId = formData.get("userId") as string;
const title = formData.get("title") as string;
const body = formData.get("body") as string;
const formErrors = postFormValidator({ userId, title, body });
// if any of error fields are not epty, return all errors
if (Object.keys(formErrors).length) {
return formErrors;
}
const dataToPost: NewPostType = {
userId: Number(userId),
title: title,
body: body,
};
const post: PostType = await createPost(dataToPost, { signal });
return redirect(`/posts/${post.id}`);
}
function NewPost() {
const { users } = useLoaderData() as {
users: UserType[];
};
const errorMessages = useActionData() as FormErrorsType;
return (
<>
<h1 className="page-title">New Post</h1>
<PostForm users={users} errorMessages={errorMessages} />
</>
);
}
export const newPostRoute = {
loader,
action,
element: <NewPost />,
}; |
import PostHeader from "./post-header";
import classes from "./post-content.module.css";
import ReactMarkdown from "react-markdown";
import Image from "next/image";
import { Prism as SyntaxHighlighter } from "react-syntax-highlighter";
import { atomDark } from "react-syntax-highlighter/dist/cjs/styles/prism";
// const POST_DATA = {
// slug: "react-js-blog",
// title: "Getting started with react",
// image: "react.jpg",
// content: "# this is the content",
// date: "2022-08-03",
// };
function PostContent({ post }) {
const imagePath = `/images/posts/${post.image}`;
const customRenderers = {
p(paragraph) {
const { node } = paragraph;
if (node.children[0].tagName === "img") {
const image = node.children[0];
return (
<div className={classes.image}>
<Image
src={`/images/posts/${image.properties.src}`}
alt={image.alt}
width={600}
height={300}
/>
</div>
);
}
return <p>{paragraph.children}</p>;
},
code(code) {
const { className, children } = code;
const language = className.split("-")[1]; // className is something like language-js => We need the "js" part here
return (
<SyntaxHighlighter style={atomDark} language={language}>
{children}
</SyntaxHighlighter>
);
},
};
return (
<article className={classes.content}>
<PostHeader title={post.title} image={imagePath} />
<ReactMarkdown components={customRenderers}>{post.content}</ReactMarkdown>
</article>
);
}
export default PostContent; |
#include "lists.h"
/**
* add_nodeint_end- creates node
* @head: pointer to head
* @n: int
* Return: pointer
*/
listint_t *add_nodeint_end(listint_t **head, const int n)
{
listint_t *new_node = (listint_t *) malloc(sizeof(listint_t));
listint_t *last = *head;
if (new_node == NULL)
{
free(new_node);
return (NULL);
}
new_node->n = n;
new_node->next = NULL;
if (*head == NULL)
{
*head = new_node;
return (*head);
}
while (last->next != NULL)
last = last->next;
last->next = new_node;
return (*head);
} |
import {Component} from 'react'
import './index.css'
class Speedometer extends Component {
state = {count: 0}
accelerate = () => {
const {count} = this.state
if (count < 200) {
this.setState(prevState => ({count: prevState.count + 10}))
}
}
brake = () => {
const {count} = this.state
if (count > 0) {
this.setState(prevState => ({count: prevState.count - 10}))
}
}
render() {
const {count} = this.state
return (
<div className="c1">
<h1 className="h1">SPEEDOMETER</h1>
<img
src="https://assets.ccbp.in/frontend/react-js/speedometer-img.png"
className="img1"
alt="speedometer"
/>
<h1 className="p1">Speed is {count}mph</h1>
<p className="p2">Min Limit is 0mph, Max Limit is 200mph</p>
<div className="c">
<button type="button" className="b1" onClick={this.accelerate}>
Accelerate
</button>
<button type="button" className="b2" onClick={this.brake}>
Apply Brake
</button>
</div>
</div>
)
}
}
export default Speedometer |
import { Component, OnInit, NgModule, signal } from '@angular/core';
import { CommonModule } from '@angular/common';
import { GeneralModule } from 'src/app/general/general.module';
import { NgbModal } from '@ng-bootstrap/ng-bootstrap';
import { HelperService, Messages, MessageType } from 'src/app/admin/helper.service';
import { UsuariosPaswordFormComponent } from '../usuarios-pasword-form/usuarios-pasword-form.component'
import { GeneralParameterService } from '../../../../../generic/general.service';
import { DatatableParameter } from '../../../../../admin/datatable.parameters';
import { Usuario } from '../usuarios.module';
import { LANGUAGE_DATATABLE } from 'src/app/admin/datatable.language';
@Component({
selector: 'app-usuarios-index',
standalone: false,
templateUrl: './usuarios-index.component.html',
styleUrl: './usuarios-index.component.css'
})
export class UsuariosIndexComponent implements OnInit {
API_URL: any;
title = "Listado de Usuarios";
breadcrumb!: any[];
botones: String[] = ['btn-nuevo'];
listUsuarios = signal<Usuario[]>([]);
constructor(
private service: GeneralParameterService,
private modalService: NgbModal,
private helperService: HelperService
) {
this.breadcrumb = [{ name: `Inicio`, icon: `fa-duotone fa-house` }, { name: "Seguridad", icon: "fa-duotone fa-lock" }, { name: "Usuarios", icon: "fa-duotone fa-user-gear" }];
}
ngOnInit(): void {
this.cargarLista();
}
cargarLista() {
this.getData()
.then((datos) => {
datos.data.forEach((item: any) => {
this.listUsuarios.update(listUsuarios => {
const Usuario: Usuario = {
id: item.id,
activo: item.activo,
userName: item.userName,
password: item.password,
personaId: item.personaId,
persona: item.persona,
};
return [...listUsuarios, Usuario];
});
});
setTimeout(() => {
$("#datatable").DataTable({
dom: 'Blfrtip',
destroy: true,
language: LANGUAGE_DATATABLE,
processing: true
});
}, 200);
})
.catch((error) => {
console.error('Error al obtener los datos:', error);
});
}
getData(): Promise<any> {
var data = new DatatableParameter(); data.pageNumber = ""; data.pageSize = ""; data.filter = ""; data.columnOrder = ""; data.directionOrder = "";
return new Promise((resolve, reject) => {
this.service.datatable("Usuario", data).subscribe(
(datos) => {
resolve(datos);
},
(error) => {
reject(error);
}
)
});
}
refrescarTabla() {
$("#datatable").DataTable().destroy();
this.listUsuarios = signal<Usuario[]>([]);
this.cargarLista();
}
nuevo() {
this.helperService.redirectApp("dashboard/seguridad/usuarios/crear");
}
update(id: any) {
this.helperService.redirectApp(`dashboard/seguridad/usuarios/editar/${id}`);
}
deleteGeneric(id: any) {
this.helperService.confirmDelete(() => {
this.service.delete("Usuario", id).subscribe(
(response) => {
if (response.status) {
this.helperService.showMessage(MessageType.SUCCESS, Messages.DELETESUCCESS);
this.refrescarTabla();
}
},
(error) => {
this.helperService.showMessage(MessageType.ERROR, error.error.message);
}
)
});
}
changePassword(id: any) {
let modal = this.modalService.open(UsuariosPaswordFormComponent, { size: 'lg', keyboard: false, backdrop: false, centered: true });
modal.componentInstance.titleData = "Usuario";
modal.componentInstance.serviceName = "Usuario";
modal.componentInstance.id = id;
modal.componentInstance.key = "Persona";
modal.result.then(res => {
if (res) {
this.refrescarTabla();
}
})
}
}
@NgModule({
declarations: [
UsuariosIndexComponent,
],
imports: [
CommonModule,
GeneralModule,
]
})
export class UsuariosIndexModule { } |
// SPDX-FileCopyrightText: 2024 SAP SE or an SAP affiliate company and Gardener contributors
//
// SPDX-License-Identifier: Apache-2.0
package ttl_test
import (
"context"
"time"
"github.com/go-logr/logr"
. "github.com/onsi/ginkgo/v2"
. "github.com/onsi/gomega"
corev1 "k8s.io/api/core/v1"
apierrors "k8s.io/apimachinery/pkg/api/errors"
metav1 "k8s.io/apimachinery/pkg/apis/meta/v1"
"k8s.io/utils/ptr"
"sigs.k8s.io/controller-runtime/pkg/client"
"sigs.k8s.io/controller-runtime/pkg/reconcile"
tmv1beta1 "github.com/gardener/test-infra/pkg/apis/testmachinery/v1beta1"
"github.com/gardener/test-infra/pkg/testmachinery"
"github.com/gardener/test-infra/pkg/testmachinery/controller/ttl"
)
var _ = Describe("Reconcile", func() {
var (
ctx context.Context
namespace string
controller reconcile.Reconciler
)
BeforeEach(func() {
ctx = context.Background()
ns := &corev1.Namespace{}
ns.GenerateName = "test-"
if err := fakeClient.Create(ctx, ns); err != nil {
if !apierrors.IsAlreadyExists(err) {
Expect(err).ToNot(HaveOccurred())
}
}
namespace = ns.Name
controller = ttl.New(logr.Discard(), fakeClient, testmachinery.TestMachineryScheme)
})
AfterEach(func() {
defer ctx.Done()
ns := &corev1.Namespace{}
ns.Name = namespace
Expect(fakeClient.Delete(ctx, ns)).To(Succeed())
})
It("should not delete a testrun when no ttl is set", func() {
tr := &tmv1beta1.Testrun{}
tr.Name = "no-ttl"
tr.Namespace = namespace
Expect(fakeClient.Create(ctx, tr)).To(Succeed())
res, err := controller.Reconcile(ctx, reconcile.Request{NamespacedName: client.ObjectKeyFromObject(tr)})
Expect(err).ToNot(HaveOccurred())
Expect(res.RequeueAfter.Seconds()).To(Equal(0.0))
Expect(fakeClient.Get(ctx, client.ObjectKeyFromObject(tr), tr)).To(Succeed())
Expect(tr.DeletionTimestamp.IsZero()).To(BeTrue())
})
It("should not delete a testrun and requeue when its ttl is no yet exceeded", func() {
tr := &tmv1beta1.Testrun{}
tr.Name = "not-exceeded"
tr.Namespace = namespace
tr.Spec.TTLSecondsAfterFinished = ptr.To(int32(10))
Expect(fakeClient.Create(ctx, tr)).To(Succeed())
tr.Status.Phase = tmv1beta1.RunPhaseSuccess
t := metav1.Now()
tr.Status.CompletionTime = &t
Expect(fakeClient.Status().Update(ctx, tr)).To(Succeed())
res, err := controller.Reconcile(ctx, reconcile.Request{NamespacedName: client.ObjectKeyFromObject(tr)})
Expect(err).ToNot(HaveOccurred())
Expect(res.RequeueAfter.Seconds()).To(BeNumerically("~", 10.0, 1.2))
Expect(fakeClient.Get(ctx, client.ObjectKeyFromObject(tr), tr)).To(Succeed())
Expect(tr.DeletionTimestamp.IsZero()).To(BeTrue())
})
It("should delete a testrun when its ttl is exceeded", func() {
tr := &tmv1beta1.Testrun{}
tr.Name = "exceeded"
tr.Namespace = namespace
tr.Spec.TTLSecondsAfterFinished = ptr.To(int32(10))
Expect(fakeClient.Create(ctx, tr)).To(Succeed())
tr.Status.Phase = tmv1beta1.RunPhaseSuccess
tr.Status.CompletionTime = &metav1.Time{Time: metav1.Now().Add(-20 * time.Second)}
Expect(fakeClient.Status().Update(ctx, tr)).To(Succeed())
res, err := controller.Reconcile(ctx, reconcile.Request{NamespacedName: client.ObjectKeyFromObject(tr)})
Expect(err).ToNot(HaveOccurred())
Expect(res.RequeueAfter.Seconds()).To(Equal(0.0))
err = fakeClient.Get(ctx, client.ObjectKeyFromObject(tr), tr)
if !apierrors.IsNotFound(err) {
Expect(err).ToNot(HaveOccurred())
}
if err == nil {
Expect(tr.DeletionTimestamp.IsZero()).To(BeFalse())
}
})
It("should use the creation timestamp if no completion timestamp exists", func() {
tr := &tmv1beta1.Testrun{}
tr.Name = "no-ts"
tr.Namespace = namespace
tr.Spec.TTLSecondsAfterFinished = ptr.To(int32(10))
Expect(fakeClient.Create(ctx, tr)).To(Succeed())
tr.Status.Phase = tmv1beta1.RunPhaseError
Expect(fakeClient.Status().Update(ctx, tr)).To(Succeed())
res, err := controller.Reconcile(ctx, reconcile.Request{NamespacedName: client.ObjectKeyFromObject(tr)})
Expect(err).ToNot(HaveOccurred())
Expect(res.RequeueAfter.Seconds()).To(BeNumerically("~", 10.0, 1))
Expect(fakeClient.Get(ctx, client.ObjectKeyFromObject(tr), tr)).To(Succeed())
Expect(tr.DeletionTimestamp.IsZero()).To(BeTrue())
})
}) |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Grid Layout</title>
<style>
body {
display: grid;
grid-template-columns: 1fr 300px;
grid-template-rows: 300px 50px 1fr 50px;
grid-template-areas: "baner baner""menu menu""blog column ""stopka stopka";
max-width: 1200px;
min-height: 100vh;
font-size: 40px;
color: white;
font-family: sans-serif;
margin: 0 auto;
}
header {
background-color: red;
grid-area: baner;
}
nav {
background-color: #45e;
grid-area: menu;
}
main {
background-color: #a55;
grid-area: blog;
}
aside {
background-color: #6a1;
grid-area: column;
}
footer {
background-color: purple;
grid-area: stopka;
}
@media (max-width: 1024px) {
body {
grid-template-columns: 1fr;
grid-template-rows: 50px 300px 1fr 200px 50px;
grid-template-areas: "menu""baner""blog""column""stopka";
}
}
</style>
</head>
<body>
<header>Header</header>
<nav>Nav</nav>
<main>Main</main>
<aside>Aside</aside>
<footer>Stopka</footer>
</body>
</html> |
//
// Created by ned on 08/12/22.
//
import SwiftUI
import NukeUI
struct SettingsView: View {
@StateObject private var viewModel = SettingsViewModel()
@State private var point: CGPoint = .zero
@State private var degrees: Double = 0
@State private var imageHeight: CGFloat = 160
var body: some View {
List {
Section {
VStack {
imageView
titleView
madeByView
}
.frame(maxWidth: .infinity)
}
.listRowBackground(Color.clear)
.listRowInsets(.none)
Section("Links") {
LabeledContent("Website") {
Link("edoardo.fyi", destination: URL(string: "https://edoardo.fyi")!)
}
LabeledContent("GitHub") {
Link("n3d1117/stats-ios", destination: URL(string: "https://github.com/n3d1117/stats-ios")!)
}
LabeledContent("Donate") {
Link("Buy me a Coffee", destination: URL(string: "https://buymeacoff.ee/ne_do")!)
}
}
.listRowBackground(Color(UIColor.darkGray).opacity(0.25))
Section("Third party libraries") {
Link("kean/Nuke", destination: URL(string: "https://github.com/kean/Nuke.git")!)
Link("mergesort/Boutique", destination: URL(string: "https://github.com/mergesort/Boutique")!)
Link("malcommac/SwiftDate", destination: URL(string: "https://github.com/malcommac/SwiftDate")!)
Link("indragiek/DominantColor", destination: URL(string: "https://github.com/indragiek/DominantColor")!)
}
.listRowBackground(Color(UIColor.darkGray).opacity(0.25))
}
.scrollContentBackground(.hidden)
.overlay(alignment: .topTrailing) {
CloseButton()
.padding()
}
}
private var imageView: some View {
GeometryReader { geometry in
LazyImage(url: URL(string: "https://edoardo.fyi/me.jpeg")) { state in
if let image = state.image {
image
.clipShape(Circle())
} else {
Image(systemName: "photo")
.resizable()
.clipShape(Circle())
.redacted(reason: .placeholder)
}
}
.scaledToFill()
.padding(5)
.frame(width: imageHeight, height: imageHeight)
.contentShape(Rectangle())
.rotation3DEffect(.degrees(degrees), axis: (x: point.x, y: point.y, z: 0))
.simultaneousGesture(
DragGesture(minimumDistance: 0)
.onChanged { gesture in
let centerX = geometry.size.width / 2
let centerY = geometry.size.height / 2
let x = 0 - (gesture.location.y / centerY - 1)
let y = (gesture.location.x / centerX - 1)
let x1 = gesture.location.x - centerX
let y1 = gesture.location.y - centerY
let range = sqrt(x1 * x1 + y1 * y1)
let degreesFactor = range / sqrt(2 * centerX * centerX)
withAnimation {
point = CGPoint(x: x, y: y)
degrees = Double(15 * degreesFactor.clamped(0, 1))
}
}
.onEnded { _ in
withAnimation {
point = .zero
degrees = 0
}
}
)
}
.frame(width: imageHeight, height: imageHeight)
}
private var titleView: some View {
HStack(alignment: .lastTextBaseline) {
Text("Stats")
.font(.title)
.fontWeight(.semibold)
if let appVersion = viewModel.appVersion {
Text("v" + appVersion)
.font(.body)
.foregroundColor(.secondary)
}
}
.padding(.bottom, 1)
}
private var madeByView: some View {
Group {
Text("Made with ") + Text(Image(systemName: "heart")).font(.caption).baselineOffset(2) + Text(" by ned")
}
.foregroundColor(.secondary)
}
}
struct SettingsView_Preview: PreviewProvider {
static var previews: some View {
SettingsView()
}
} |
package com.candroid.candroidrcc026
import android.os.Bundle
import android.view.View
import androidx.appcompat.app.AppCompatActivity
import com.candroid.candroidrcc026.databinding.ActivityMainBinding
import com.google.android.gms.ads.AdRequest
import retrofit2.Call
import retrofit2.Callback
import retrofit2.Response
import retrofit2.Retrofit
import retrofit2.converter.gson.GsonConverterFactory
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
loadNews()
binding.refresh.setOnRefreshListener { loadNews() }
val adRequest = AdRequest.Builder().build()
binding.adView.loadAd(adRequest)
}
private fun loadNews(){
val retrofit = Retrofit
.Builder()
.baseUrl("https://newsapi.org")
.addConverterFactory(GsonConverterFactory.create())
.build()
val cat = intent.getStringExtra("cat") //sports
val prefs = getSharedPreferences("settings", MODE_PRIVATE)
val code = prefs.getString("code", "us")
val callable = retrofit.create(Callable::class.java)
callable.getNews(cat!!, code!!).enqueue(object : Callback<NewsModel>{
override fun onResponse(call: Call<NewsModel>, response: Response<NewsModel>) {
val news = response.body()
val articles = news?.articles
//Log.d("trace", "Link: ${articles?.get(0)?.urlToImage}")
val adapter = NewsAdapter(this@MainActivity, articles!!)
binding.newsRv.adapter = adapter
binding.refresh.isRefreshing = false
binding.progress.visibility = View.GONE
}
override fun onFailure(call: Call<NewsModel>, t: Throwable) {
}
})
}
} |
// Load the data from the CSV file
d3.csv("datasets/merged_dataset.csv").then(function(data) {
// Parse the data and convert strings to appropriate types
data.forEach(function(d) {
d.date = d3.timeParse("%Y-%m-%d")(d.date);
d.approve_estimate = +d.approve_estimate;
d.frequency = +d.frequency;
});
const containerWidth = d3.select("#approval_chart5").node().getBoundingClientRect().width;
// Set up the SVG and scales
const margin = {top: 20, right: 50, bottom: 30, left: 50},
width = containerWidth - margin.left - margin.right,
height = 500 - margin.top - margin.bottom;
const x = d3.scaleTime()
.range([0, width])
.domain(d3.extent(data, d => d.date));
const y0 = d3.scaleLinear()
.range([height, 0])
.domain([0, d3.max(data, d => d.approve_estimate)]);
const y1 = d3.scaleLinear()
.range([height, 0])
.domain([d3.min(data, d => d.frequency), d3.max(data, d => d.frequency)]);
const svg = d3.select("#approval_chart5").append("svg")
.attr("width", width + margin.left + margin.right)
.attr("height", height + margin.top + margin.bottom)
.append("g")
.attr("transform", "translate(" + margin.left + "," + margin.top + ")");
// Add a title to the graph
// svg.append("text")
// .attr("x", width/2) // x-coordinate of the text
// .attr("y", margin.top+50) // y-coordinate of the text
// .attr("text-anchor", "middle") // center the text horizontally
// .text("Timeseries Comparison of Trump Frequency (red) vs. Voter Popularity (blue)");
// Define the line for approval estimate
const line1 = d3.line()
.x(d => x(d.date))
.y(d => y0(d.approve_estimate));
// Define the line for frequency
const line2 = d3.line()
.defined(function(d) { return d.value !== null; })
.x(d => x(d.date))
.y(d => y1(d.frequency))
.curve(d3.curveMonotoneX);;
// Create a tooltip
const tooltip = d3.select('body').append('div')
.attr('class', 'tooltip')
.style('position', 'absolute')
.style('background-color', 'white')
.style('border', 'solid')
.style('border-width', '1px')
.style('border-radius', '5px')
.style('font-size', '12px')
.style('padding', '5px')
.style('visibility', 'hidden'); // Hidden by default
// Draw the lines
svg.append("path")
.datum(data)
.attr("class", "line")
.attr("d", line1)
.attr("stroke", "#6b486b")
.attr("fill", "none")
.on('mouseover', function(event, d) {
tooltip.style('visibility', 'visible')
.html(`${d3.timeFormat("%m/%d/%Y")(x.invert(event.pageX - margin.left))}`)
.style('left', `${event.pageX + 10}px`)
.style('top', `${event.pageY + 10}px`);
})
.on('mouseout', function(event, d) {
tooltip.style('visibility', 'hidden');
});
svg.append("path")
.datum(data)
.attr("class", "line")
.attr("d", line2)
.attr("stroke", "#d0743c")
.attr("fill", "none")
.on('mouseover', function(event, d) {
tooltip.style('visibility', 'visible')
.html(`${d3.timeFormat("%m/%d/%Y")(x.invert(event.pageX - margin.left))}`)
.style('left', `${event.pageX + 10}px`)
.style('top', `${event.pageY + 10}px`);
})
.on('mouseout', function(event, d) {
tooltip.style('visibility', 'hidden');
});
// Add the X Axis
svg.append("g")
.attr("transform", "translate(0," + height + ")")
.call(d3.axisBottom(x));
// Add the Y0 Axis
svg.append("g")
.call(d3.axisLeft(y0));
// Add the Y1 Axis
svg.append("g")
.attr("transform", "translate(" + width + " ,0)")
.call(d3.axisRight(y1));
// Add legend
const legend = svg.append("g")
.attr("transform", `translate(0,${margin.top})`);
// outletNames.forEach((outlet, index) => {
legend.append("rect")
.attr("x", 100)
.attr("width", 18)
.attr("height", 18)
.style("fill", "#6b486b");
legend.append("text")
.attr("x", 100 + 24)
.attr("y", 9)
.attr("dy", ".35em")
.style("text-anchor", "start")
.text("Approval Estimate");
legend.append("rect")
.attr("x", 400)
.attr("width", 18)
.attr("height", 18)
.style("fill", "#d0743c");
legend.append("text")
.attr("x", 400 + 24)
.attr("y", 9)
.attr("dy", ".35em")
.style("text-anchor", "start")
.text("Frequency");
// });
}); |
import React, { useState } from "react";
import "./CreateProduct.css";
import { useAlert } from "react-alert";
import { Button } from "@material-ui/core";
import MetaData from "../layout/MetaData";
import AccountTreeIcon from "@material-ui/icons/AccountTree";
import DescriptionIcon from "@material-ui/icons/Description";
import StorageIcon from "@material-ui/icons/Storage";
import SpellcheckIcon from "@material-ui/icons/Spellcheck";
import AttachMoneyIcon from "@material-ui/icons/AttachMoney";
import SideBar from "./Sidebar";
const CreateProduct = ({history}) => {
const alert = useAlert();
const [name,setName] = useState("");
const [price,setPrice] = useState("");
const [description,setDescription] = useState("");
const [category,setCategory] = useState("");
const [stock,setStock] = useState("");
const [images,setImages] = useState([]);
const [imagesPreview,setImagesPreview] = useState([]);
const [loading,setLoading] = useState(false);
const categories = [
"Laptop",
"Footwear",
"Bottom",
"Tops",
"Attire",
"Camera",
"SmartPhones",
];
const createProductSubmitHandler = async (e) => {
e.preventDefault();
const myForm = new FormData();
myForm.set("name", name);
myForm.set("price", price);
myForm.set("description", description);
myForm.set("category", category);
myForm.set("Stock", stock);
images.forEach((image) => {
myForm.append("pro_image", image);
});
try{
setLoading(true);
const response = await fetch('/api/v1/admin/product/create',{
method: "POST",
body: myForm
});
const data = await response.json(response);
setLoading(false);
if(data.status === 200)
{
alert.success(data.message);
history.push('/admin/all-products');
}
else
{
alert.error(data.message);
}
}
catch(error)
{
alert.error(error.data.message);
}
}
const createProductImagesChange = async (e) => {
const files = Array.from(e.target.files);
setImages([]);
setImagesPreview([]);
files.forEach((file) => {
const reader = new FileReader();
reader.onload = () => {
if (reader.readyState === 2) {
setImagesPreview((old) => [...old, reader.result]);
setImages((old) => [...old, reader.result]);
}
};
reader.readAsDataURL(file);
});
}
return(
<>
<MetaData title="Create Product" />
<div className="dashboard">
<SideBar />
<div className="newProductContainer">
<form
className="createProductForm"
encType="multipart/form-data"
onSubmit={createProductSubmitHandler}
>
<h1>Create Product</h1>
<div>
<SpellcheckIcon />
<input
type="text"
placeholder="Product Name"
required
value={name}
onChange={(e) => setName(e.target.value)}
/>
</div>
<div>
<AttachMoneyIcon />
<input
type="number"
placeholder="Price"
required
onChange={(e) => setPrice(e.target.value)}
/>
</div>
<div>
<DescriptionIcon />
<textarea
placeholder="Product Description"
value={description}
onChange={(e) => setDescription(e.target.value)}
cols="30"
rows="1"
></textarea>
</div>
<div>
<AccountTreeIcon />
<select onChange={(e) => setCategory(e.target.value)}>
<option value="">Choose Category</option>
{categories.map((cate) => (
<option key={cate} value={cate}>
{cate}
</option>
))}
</select>
</div>
<div>
<StorageIcon />
<input
type="number"
placeholder="Stock"
required
onChange={(e) => setStock(e.target.value)}
/>
</div>
<div id="createProductFormFile">
<input
type="file"
name="avatar"
accept="image/*"
onChange={createProductImagesChange}
multiple
/>
</div>
<div id="createProductFormImage">
{imagesPreview.map((image, index) => (
<img key={index} src={image} alt="Product Preview" />
))}
</div>
<Button
id="createProductBtn"
type="submit"
disabled={loading ? true : false}
>
Create
</Button>
</form>
</div>
</div>
</>
)
}
export default CreateProduct |
import { Module } from '@nestjs/common';
import { AppController } from './app.controller';
import { AppService } from './app.service';
import { BoardsModule } from './boards/boards.module';
import { ConfigModule } from '@nestjs/config';
import { TypeOrmModule } from '@nestjs/typeorm';
import { BoardsEntity } from './boards/entities/boards.entity';
import { UsersModule } from './users/users.module';
import { UsersEntity } from './users/entities/users.entity';
import { AuthModule } from './auth/auth.module';
@Module({
imports: [
ConfigModule.forRoot({ isGlobal: true }),
TypeOrmModule.forRoot({
type: 'mysql',
host: '127.0.0.1',
port: 3306,
username: process.env.DB_USERNAME,
password: process.env.DB_PASSWORD,
database: process.env.DB_DATABASE,
autoLoadEntities: true,
synchronize: true,
logging: true,
keepConnectionAlive: true,
}),
TypeOrmModule.forFeature([BoardsEntity, UsersEntity]),
BoardsModule,
UsersModule,
AuthModule,
],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {} |
import {
SnackbarContent,
Button,
List,
Checkbox,
ListSubheader,
ListItemText,
ListItemIcon,
Card,
CardContent,
CardActions,
} from '@material-ui/core'
import ListItemButton from '@material-ui/core/ListItemButton'
import { useMap } from 'react-use'
import type { ExternalPluginLoadDetails } from '../types'
export function UnknownPluginLoadRequestUI({ plugins, onConfirm }: UnknownPluginLoadRequestProps) {
const [_selected, { get, set }] = useMap({} as Record<string, boolean>)
const confirmAll = () => onConfirm(plugins)
const selected = plugins.filter((x) => _selected[x.url])
const confirmSelected = () => onConfirm(selected)
if (plugins.length === 0) return null
if (plugins.length === 1)
return (
<SnackbarContent
message={`Do you want to load an external plugin from ${plugins[0].url}?`}
action={<Button onClick={confirmAll}>Load</Button>}
/>
)
return (
<Card variant="outlined">
<CardContent sx={{ paddingBottom: 0 }}>
<List
dense
subheader={
<ListSubheader>New unknown Mask plugins found. Do you want to load them?</ListSubheader>
}>
{plugins.map((x) => (
<ListItemButton dense onClick={() => set(x.url, !get(x.url))} key={x.url}>
<ListItemIcon>
<Checkbox disableRipple edge="start" tabIndex={-1} checked={!!get(x.url)} />
</ListItemIcon>
<ListItemText primary={x.url} />
</ListItemButton>
))}
</List>
</CardContent>
<CardActions disableSpacing sx={{ flexDirection: 'row-reverse' }}>
<Button disabled={selected.length === 0} onClick={confirmSelected}>
Load
</Button>
<Button onClick={confirmAll}>Load All</Button>
</CardActions>
</Card>
)
}
export interface UnknownPluginLoadRequestProps {
plugins: ExternalPluginLoadDetails[]
onConfirm(list: ExternalPluginLoadDetails[]): void
} |
use std::io::Write;
use std::path::{Path, PathBuf};
use futures_util::{Future, StreamExt, TryFutureExt};
use once_cell::sync::Lazy;
use reqwest::{Body, Client, ClientBuilder, IntoUrl, Response, Url};
use serde::de::DeserializeOwned;
use tokio::io::AsyncWriteExt;
use tokio::{fs, io};
use crate::prelude::*;
use crate::reporter::Case;
use crate::reporter::Reporter;
/// Default retry count for operations.
pub const RETRY_COUNT: u8 = 3;
/// Initializes the network client.
///
/// Uses `CARGO_PKG_NAME/CARGO_PKG_VERSION` as user agent.
static CLIENT: Lazy<Client> = Lazy::new(|| {
ClientBuilder::new()
.user_agent(concat!(
env!("CARGO_PKG_NAME"),
"/",
env!("CARGO_PKG_VERSION")
))
.build()
.expect("Couldn't create the network client.")
});
/// Sends a GET request to the given URL.
///
/// Retries the request if it fails with the given amount of retries (default = 3).
pub async fn get(url: impl IntoUrl) -> Result<Response> {
Ok(retry(|| CLIENT.get(url.as_str()).send(), reqwest::Result::is_ok).await?)
}
/// Sends a POST request to the given URL with payload.
///
pub async fn post<P : DeserializeOwned + ToString>(url: impl IntoUrl, payload : P) -> Result<Response>{
let payload = Body::from(payload.to_string());
Ok(CLIENT.post(url.as_str()).body(payload).send().await?)
}
/// Sends a GET request to the given URL and returns JSON value.
///
/// Retries the request if it fails with the given amount of retries (default = 3).
pub async fn get_json<F: DeserializeOwned>(url: impl IntoUrl) -> Result<F> {
Ok(get(url).await?.json::<F>().await?)
}
pub async fn download_retry<R: Reporter>(
url: impl IntoUrl,
path: &impl AsRef<Path>,
reporter: &Option<R>,
) -> Result<()> {
retry(|| download(url.as_str(), path, &reporter), Result::is_ok).await
}
/// Download file from the given URL to the destination path.
///
/// Retries the download if it fails with the given amount of retries (default = 3).
pub async fn download<R: Reporter>(
url: impl IntoUrl,
path: impl AsRef<Path>,
reporter: &Option<R>,
) -> Result<()> {
let path = path.as_ref();
let response = get(url).await?;
let total_size = response.content_length().unwrap_or(0);
if let Some(parent) = path.parent() {
fs::create_dir_all(parent).await?;
}
// This is not necessary for now.
// But necessary for parallelism that will be implemented in the future.
let temp_path = format!("{}.tmp", path.display());
let mut temp_file = fs::OpenOptions::new()
.create(true)
.write(true)
.truncate(true)
.open(&temp_path)
.await?;
let mut progress: f64 = 0f64;
let mut stream = response.bytes_stream();
reporter.send(Case::SetMaxSubProgress(total_size as f64));
while let Some(item) = stream.next().await {
let chunk = item?;
temp_file.write_all(&chunk).await?;
progress += chunk.len() as f64;
reporter.send(Case::SetSubProgress(progress));
}
fs::rename(temp_path, path).await?;
Ok(())
}
/// Method that allows us to retry to call functions without code repeating.
async fn retry<A, B: std::future::Future<Output = A>>(
f: impl Fn() -> B,
handler: impl Fn(&A) -> bool,
) -> A {
let mut retries = 0;
loop {
retries += 1;
let f = f();
let r: A = f.await;
if handler(&r) || retries >= RETRY_COUNT {
return r;
}
}
} |
---
date: 2024-02-03 19:03:19.668689-07:00
description: "How to: Lua does not support regular expressions natively in the same\
\ way as languages like Perl or Python. Instead, it offers pattern matching\u2026"
lastmod: '2024-03-13T22:45:00.196217-06:00'
model: gpt-4-0125-preview
summary: Lua does not support regular expressions natively in the same way as languages
like Perl or Python.
title: Using regular expressions
weight: 11
---
## How to:
Lua does not support regular expressions natively in the same way as languages like Perl or Python. Instead, it offers pattern matching capabilities that cover many common use cases of regular expressions. However, for full-fledged regular expression support, one can use a third-party library such as `lrexlib`.
### Basic Pattern Matching in Lua:
Lua provides a powerful pattern matching system that you can use for simple substitutions and searches:
```lua
-- Simple search
local str = "Hello, World!"
if string.find(str, "World") then
print("Match found!")
end
-- Output: Match found!
-- Simple substitution
local s = string.gsub("Lua is great!", "great", "awesome")
print(s)
-- Output: Lua is awesome!
```
### Capturing Substrings:
You can capture parts of the string that match patterns:
```lua
local date = "Today is 17/05/2023."
local d, m, y = string.match(date, "(%d+)/(%d+)/(%d+)")
print("Day:", d, "Month:", m, "Year:", y)
-- Output: Day: 17 Month: 05 Year: 2023
```
### Using `lrexlib` for Regular Expressions:
To use actual regular expressions, you can install and use `lrexlib`. Assuming you have it installed (`luarocks install lrexlib-pcre`), you can do more complex pattern matching:
```lua
local rex = require 'rex_pcre'
local text = "The rain in Spain stays mainly in the plain."
local regex = "\\bS\\w+"
local count, err = rex.gsub(text, regex, function(w)
return w:upper()
end)
if err then
print("Error:", err)
else
print("Modified text:", text)
print("Substitutions made:", count)
end
-- Example output: Modified text: The RAIN in SPAIN stays MAINLY in the plain.
-- Substitutions made: 3
```
The above examples illustrate basic usage within Lua's own pattern matching system and how to harness the power of regular expressions via `lrexlib`. Whether you're performing simple string manipulations or require the full versatility of regular expressions, Lua, coupled with powerful libraries, can accommodate your needs. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.