text
stringlengths 184
4.48M
|
---|
<?php
session_start();
// Periksa apakah author sudah login
if (!isset($_SESSION['author'])) {
header("Location: ../../login/author/authorlogin.php");
exit();
}
require_once '../../includes/dbconnect.php';
// Ambil daftar mata pelajaran dari database
$stmt_subjects = $conn->query("SELECT * FROM subjects");
$subjects = $stmt_subjects->fetchAll(PDO::FETCH_ASSOC);
if ($_SERVER["REQUEST_METHOD"] == "POST") {
try {
$content = $_POST['content'];
$subject_id = $_POST['subject'];
$slug = $_POST['slug']; // Tambahkan ini untuk mendapatkan nilai slug dari form
$author_id = $_SESSION['author']['id'];
$author_type = 'author'; // Menandai bahwa penulis adalah author
$stmt = $conn->prepare("INSERT INTO articles (slug, content, subject_id, author_id, author_type) VALUES (:slug, :content, :subject_id, :author_id, :author_type)");
$stmt->bindParam(':slug', $slug);
$stmt->bindParam(':content', $content);
$stmt->bindParam(':subject_id', $subject_id);
$stmt->bindParam(':author_id', $author_id, PDO::PARAM_INT); // Menambahkan tipe parameter untuk author_id
$stmt->bindParam(':author_type', $author_type);
if ($stmt->execute()) {
header("Location: index.php");
exit();
} else {
throw new Exception("Error adding article.");
}
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
}
?>
<!DOCTYPE html>
<html>
<head>
<title>Add New Article</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<link rel="stylesheet" href="https://cdn.quilljs.com/1.3.6/quill.snow.css">
<style>
*,
body {
background-color: #202124;
color: #bdc1c6ba;
}
.form-control {
background-color: #3131318e;
color: #bdc1c6ba;
border: none;
}
input[type="text"]:focus {
border-color: #343434;
box-shadow: 0 0 5px #25252580;
background-color: #3131318e;
color: #bdc1c6ba;
}
</style>
</head>
<body>
<nav class="navbar navbar-expand-lg">
<a class="navbar-brand" href="index.php">Author Dashboard</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNav"
aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link" href="../../login/author/authorlogout.php">Logout</a>
</li>
</ul>
</div>
</nav>
<div class="container mt-5 mb-5">
<h2>Add New Article</h2>
<form id="articleForm" action="" method="post">
<div class="form-group">
<label for="slug">Slug</label>
<input type="text" class="form-control bg-dark" id="slug" name="slug" readonly>
</div>
<div class="form-group">
<label for="subject">Subject</label>
<select class="form-control bg-dark" id="subject" name="subject" required>
<?php foreach ($subjects as $subject) { ?>
<option value="<?php echo $subject['id']; ?>"><?php echo $subject['name']; ?></option>
<?php } ?>
</select>
</div>
<div class="form-group">
<label for="content">Content</label>
<div id="editor" style="height: 600px; border: #bdc1c6ba 1px solid;"></div>
<textarea id="hiddenInput" name="content" style="display: none;"></textarea>
</div>
<button type="submit" class="btn btn-primary">Add Article</button>
</form>
</div>
<script src="https://cdn.quilljs.com/1.3.6/quill.min.js"></script>
<script>
var quill = new Quill('#editor', {
theme: 'snow',
modules: {
toolbar: [
[{ 'header': [1, 2, false] }],
['bold', 'italic', 'underline'],
['link'],
[{ 'list': 'ordered' }, { 'list': 'bullet' }],
['clean']
]
}
});
quill.on('text-change', function () {
var content = quill.root.innerHTML;
var cleanedContent = content.replace(/<[^>]*>/g, ''); // Membersihkan tag HTML dari konten
var words = cleanedContent.split(' ');
var slug = words.slice(0, 100).join('-');
document.getElementById('slug').value = slug;
document.getElementById('hiddenInput').value = content;
});
</script>
</body>
</html> |
# pos control analysis - get pairwise distances for lang/score/word type
library(tidyverse)
library(data.table)
library(feather)
library(here)
POS_PATH <- here("analyses/06_syntax_control/data/SUBTLEX-US frequency list with PoS information text version.txt")
WORD_PATH <- here("analyses/02_concreteness_semantics/data/ets/target_word_cluster_assignments_ets.csv")
MODEL_PATH <- here("data/processed/models/L1_high_low_models/")
OUTPATH <- "/Volumes/wilbur_the_great/syntax_control_analysis_for_paper/syntax_control_analysis_for_paper/"
LANGS <- c('ARA', 'BEN', 'BUL', 'CHI', 'DUT', 'ENG', 'FAS', 'FRE', 'GER', 'GRE', 'GUJ',
'HIN', 'IBO', 'IND', 'ITA', 'JPN','KAN', 'KOR', 'MAL', 'MAR', 'NEP', 'PAN', 'POL',
'POR', 'RUM', 'RUS', 'SPA', 'TAM', 'TEL', 'TGL', 'THA', 'TUR', 'URD', 'VIE', 'YOR')
pos <- read_tsv(POS_PATH) %>%
janitor::clean_names() %>%
rename(pos = dom_po_s_subtlex) %>%
select(word, pos)
target_words <- read_csv(WORD_PATH) %>%
select(-cluster)
target_words_with_pos <- target_words %>%
left_join(pos) %>%
mutate_all(as.factor) %>%
mutate(pos = case_when(pos %in% c("Article", "Conjunction", "Determiner", "Pronoun",
"Preposition") ~ "grammatical",
pos %in% c("Interjection", "Adverb", "Adjective",
"Verb", "Noun") ~ "content",
TRUE ~ "other")) %>%
filter(pos != "other") %>%
group_by(pos) %>%
nest()
get_pairwise_dist_between_words <- function(wordvecs, words){
word_word_dists <- coop::cosine(t(as.matrix(wordvecs))) # this is fast
wide_word_word_dists <- word_word_dists %>%
as.data.frame(row.names = words) %>%
setNames(., words) %>%
rownames_to_column(var = "w1")
long_word_word_dists <- gather(wide_word_word_dists, "w2", "cos_dist", -w1)
long_word_word_dists
}
get_pairwise_distances <- function(arg_values, outpath, model_path, target_words){
current_group <- arg_values[1]
current_word_type <- arg_values[2]
current_lang <- arg_values[3]
# get grammatical/content word lists
target_word_set <- filter(target_words, pos == current_word_type) %>%
pull(data) %>%
pluck(1) %>%
pull(word)
current_wordvecs <- read_feather(paste0(model_path, "wordvecs_", current_lang, "-", current_group, ".model.feather") )
current_words <- read_feather(paste0(model_path, "words_", current_lang, "-",current_group, ".model.feather")) %>%
rename(word = `0`)
df <- current_words %>%
bind_cols(current_wordvecs) %>%
data.table() %>%
.[word %in% target_word_set]
these_dists <- get_pairwise_dist_between_words(df[,-c("word")], df$word)
write_feather(these_dists, paste0(outpath,
current_lang, "_",
current_word_type, "_",
current_group, "_", "word_dists.feather"))
}
### DO THE THING ##
cross(list(c("low", "high"),
c("grammatical", "content"),
LANGS)) %>%
walk(get_pairwise_distances, OUTPATH, MODEL_PATH, target_words_with_pos) |
package com.argon.order.domain;
import jakarta.persistence.*;
import lombok.*;
@AllArgsConstructor(access = AccessLevel.PRIVATE)
@NoArgsConstructor
@Builder(builderMethodName = "memberBuilder", toBuilder = true)
@Entity
@Data
@Table(name="NT_MEMBER")
public class Member {
@Id
@Column(name = "MEMBER_ID", nullable = false)
private String memberId;
@Column(name = "PASSWORD", columnDefinition = "VARCHAR(200)", nullable = false)
private String password;
@Column(name = "NAME", columnDefinition = "VARCHAR(20)", nullable = false)
private String name;
@Column(name = "GRADE", columnDefinition = "VARCHAR(5)", nullable = false)
private String grade;
@Column(name = "REGIST_DATE", columnDefinition = "CHAR(14)", updatable=false)
private String registDate;
@Column(name = "REGIST_ID", columnDefinition = "VARCHAR(20)", updatable=false)
private String registId;
@Column(name = "UPDATE_DATE", columnDefinition = "CHAR(14)", insertable=false)
private String updateDate;
@Column(name = "UPDATE_ID", columnDefinition = "VARCHAR(20)", insertable=false)
private String updateId;
public static Member.MemberBuilder builder(){
return memberBuilder();
}
} |
import random
puzzles = ["Kali", "Mint", "Debian", "Arch", "Gentoo", "Fedora", "Ubuntu"]
HANGMANPICS = ['''
+---+
| |
|
|
|
|
=========''', '''
+---+
| |
O |
|
|
|
=========''', '''
+---+
| |
O |
| |
|
|
=========''', '''
+---+
| |
O |
/| |
|
|
=========''', '''
+---+
| |
O |
/|\ |
|
|
=========''', '''
+---+
| |
O |
/|\ |
/ |
|
=========''', '''
+---+
| |
O |
/|\ |
/ \ |
|
=========''']
lives = 6
guessed_letters = []
puzzle = puzzles[random.randint(0, len(puzzles) - 1)]
display_puzzle = ' '.join(['_' if char.isalpha() else '' for char in puzzle])
print("Welcome to Hangman!")
print(HANGMANPICS[0])
print("Lives: {}".format(lives))
print("Current word: {}".format(' '.join(display_puzzle)))
while lives > 0 and '_' in display_puzzle:
guess = input("Guess a letter: ").lower()
if guess.isalpha() and len(guess) == 1:
if guess in guessed_letters:
print("You already guessed that letter.")
elif guess in puzzle.lower():
for i in range(len(puzzle)):
if puzzle[i].lower() == guess:
display_puzzle = display_puzzle[:2 * i] + puzzle[i] + display_puzzle[2 * i + 1:]
else:
lives -= 1
print("Incorrect guess. Lives remaining: {}".format(lives))
print(HANGMANPICS[6 - lives])
guessed_letters.append(guess)
print("\nLives: {}".format(lives))
print("Current word: {}".format(' '.join(display_puzzle)))
#print("Guessed letters: " + ', '.join(guessed_letters))
else:
print("Invalid input. Please enter a single letter.")
if '_' not in display_puzzle:
print("Congratulations! You guessed the word.")
else:
print("Game over. The word was: {}".format(puzzle)) |
import React,{ useContext, useState } from 'react'
//import { useForm } from '../util/hooks';
import { Button,Form } from "semantic-ui-react";
import { useMutation } from '@apollo/react-hooks';
import gql from 'graphql-tag';
import { AuthContext } from '../context/auth';
function Login(props) {
const context = useContext(AuthContext)
const [errors, setErrors] = useState({})
const [values, setValues] = useState({
username:'',
password:'',
})
const onChange = (event) => {
setValues({ ...values, [event.target.name]: event.target.value});
}
const [loginUser, { loading }] = useMutation(LOGIN_USER, {
update(_, { data: { login: userData }}){//pass this option to useMutation to update cache
context.login(userData)
props.history.push('/');//use this to redirect after successful new user registry
},
onError(err){//pass the onError option to useMutation to execute this callback in case of error.
setErrors(err.graphQLErrors[0].extensions.exception.errors);
},
variables: values
});
const onSubmit = (event) =>{
event.preventDefault();
loginUser();
}
return (
<div className="form-container">
<Form onSubmit={onSubmit} noValidate className={loading ? "loading" : ''}>
<h1>Login</h1>
<Form.Input
label="Username"
placeholder="Username.."
name="username"
type="text"
value={values.username}
error={errors.username ? true : false}
onChange={onChange}
/>
<Form.Input
label="Password"
placeholder="Password.."
name="password"
type="password"
value={values.password}
error={errors.password ? true :false}
onChange={onChange}
/>
<Button type="submit" primary>Login</Button>
</Form>
{Object.keys(errors).length > 0 && (
<div className="ui error message">
<ul className="list">
{Object.values(errors).map(value=>(
<li key={value}>{value}</li>
))}
</ul>
</div>
)}
</div>
)
}
const LOGIN_USER = gql`
mutation login(
$username:String!
$password:String!
){
login(
username:$username
password:$password
) {
id email username createdAt token
}
}
`;
export default Login; |
document.addEventListener("DOMContentLoaded", (e) => {
e.preventDefault();
const url = "http://localhost:3000/Usuarios";
async function getUser() {
try {
const response = await fetch(url);
//console.log(response);
const dados = await response.json();
//console.log(dados);
dados.forEach((ele) => {
const listaUsuarios = document.getElementById("listaUsuarios");
//console.log(series.id)
const tr = document.createElement("tr");
const div = document.createElement("div");
const editar = document.createElement("button");
const excluir = document.createElement("button");
const livros = document.createElement("button");
div.classList.add("botoes");
editar.classList.add("bi", "bi-pencil-square");
excluir.classList.add("bi", "bi-trash3");
livros.classList.add("bi", "bi-book");
excluir.addEventListener('click', async () => {
const id = ele.id
if (window.confirm(`Você deseja realmente apagar esse Usuario: ${ele.NomeUsuario}`)) {
try {
const retorno = await fetch(`${url}/${id}`, {
method: 'DELETE'
})
const retornoLivros = await fetch(`http://localhost:3000/Livros/${id}`, {
method: 'DELETE'
})
if (retorno.ok) {
alert('Usuario foi deletada com sucesso!')
} else {
alert(`Erro ao deletar série ${retorno.status}`)
}
} catch (error) {
console.log(error);
}
}
})
livros.addEventListener("click", function () {
EditarLivros(ele.id, ele);
});
editar.addEventListener("click", () => {
const id = ele.id;
if (window.confirm("Você deseja atualizar o usuario?")) {
document.getElementById("title-form").textContent = "Atualizar Usuario";
document.getElementById("btnCadastrar").textContent = "Atualizar";
document.getElementById("nomeUsuario").value = ele.NomeUsuario;
document.getElementById("idadeUsuario").value = ele.IdadeUsuario;
document.getElementById("enderecoUsuario").value = ele.EnderecoUsuario;
document.getElementById("emialUsuario").value = ele.EmailUsuario;
document.getElementById("telefoneUsuario").value = ele.TelefoneUsuario;
var botao = document.getElementById("btnCadastrar");
botao.onclick = null;
// Adiciona o novo evento de clique
document.getElementById("btnCadastrar").addEventListener("click", function(e) {
EditarUser(e, ele.id, url);
});
}
});
//realizar a criação das celulas de cada elemento
const nomeUsuario = document.createElement("td");
nomeUsuario.textContent = ele.NomeUsuario;
const idadeUsuario = document.createElement("td");
idadeUsuario.textContent = ele.IdadeUsuario;
const enderecoUsuario = document.createElement("td");
enderecoUsuario.textContent = ele.EnderecoUsuario;
const emailUsuario = document.createElement("td");
emailUsuario.textContent = ele.EmailUsuario;
const telefoneUsuario = document.createElement("td");
telefoneUsuario.textContent = ele.TelefoneUsuario;
tr.appendChild(nomeUsuario);
tr.appendChild(idadeUsuario);
tr.appendChild(enderecoUsuario);
tr.appendChild(emailUsuario);
tr.appendChild(telefoneUsuario);
tr.appendChild(div);
div.appendChild(editar);
div.appendChild(excluir);
div.appendChild(livros);
listaUsuarios.appendChild(tr);
});
document.getElementById("campoBusca").addEventListener("input", buscarUsuarios);
} catch (error) {
console.log("deu erro " + error);
}
}
getUser();
});
async function EditarUser(e, id, url) {
//alert(`ola: ${id}`)
e.preventDefault();
try {
const dadosEnviadosAtualizados = {
"NomeUsuario": document.getElementById("nomeUsuario").value,
"IdadeUsuario": document.getElementById("idadeUsuario").value,
"EnderecoUsuario": document.getElementById("enderecoUsuario").value,
"EmailUsuario": document.getElementById("emialUsuario").value,
"TelefoneUsuario":document.getElementById("telefoneUsuario").value,
};
//alert(id);
const retorno = await fetch(`${url}/${id}`,
{
method: "PUT",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify(dadosEnviadosAtualizados),
}
);
if (retorno.ok) {
alert("Usuario atualizada com sucesso!");
} else {
alert(`Usuario não pode ser atualizada ${retorno.status}`);
}
//window.location.reload();
} catch (error) {
console.log(error);
}
}
async function buscarUsuarios() {
const textoBusca = document.getElementById("campoBusca").value.toLowerCase();
const usuarios = document.querySelectorAll("#listaUsuarios tr");
usuarios.forEach(usuario => {
const nomeUsuario = usuario.querySelector("td:first-child").textContent.toLowerCase();
if (nomeUsuario.includes(textoBusca)) {
usuario.style.display = "table-row";
} else {
usuario.style.display = "none";
}
});
}
function mostrarMenu() {
let menu = document.getElementById("icone");
let mostraMenu = document.getElementById("mostraIcons");
mostraMenu.style.display =
mostraMenu.style.display === "none" || mostraMenu.style.display === ""
? "flex"
: "none";
if (mostraMenu.style.display === "flex") {
//alert('flex')
menu.classList.add("bi", "bi-x-circle");
menu.classList.remove("bi", "bi-card-list");
} else if (mostraMenu.style.display === "none") {
//alert('none')
menu.classList.add("bi", "bi-card-list");
menu.classList.remove("bi", "bi-x-circle");
}
} |
/*
* Copyright (C) 1998, 1999, 2000, 2001
* Lehrstuhl fuer Technische Informatik, RWTH-Aachen, Germany
*
* This file is part of the LTI-Computer Vision Library (LTI-Lib)
*
* The LTI-Lib is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public License (LGPL)
* as published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* The LTI-Lib is distributed in the hope that it will be
* useful, but WITHOUT ANY WARRANTY; without even the implied warranty
* of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with the LTI-Lib; see the file LICENSE. If
* not, write to the Free Software Foundation, Inc., 59 Temple Place -
* Suite 330, Boston, MA 02111-1307, USA.
*/
/*----------------------------------------------------------------
* project ....: LTI Digital Image/Signal Processing Library
* file .......: ltiCentroidClustering.h
* authors ....: Peter Doerfler
* organization: LTI, RWTH Aachen
* creation ...: 29.08.2001
* revisions ..: $Id: ltiCentroidClustering.h,v 1.7 2005/06/16 09:32:39 doerfler Exp $
*/
#include "ltiObject.h"
#include "ltiClustering.h"
#ifndef _LTI_CENTROIDCLUSTERING_H_
#define _LTI_CENTROIDCLUSTERING_H_
namespace lti {
/**
* Base class for all clustering methods that use centroids or prototypes
* for describing individual clusters. A classify(const dvector&) method
* is implemented that should work for most subclasses.
*/
class centroidClustering : public clustering {
public:
/**
* default constructor
*/
centroidClustering();
/**
* copy constructor
* @param other the %object to be copied
*/
centroidClustering(const centroidClustering& other);
/**
* destructor
*/
virtual ~centroidClustering();
/**
* returns the name of this type ("centroidClustering")
*/
virtual const char* getTypeName() const;
/**
* copy data of "other" functor.
* @param other the functor to be copied
* @return a reference to this functor %object
*/
centroidClustering& copy(const centroidClustering& other);
/**
* returns used parameters
*/
const parameters& getParameters() const;
/**
* classifies a new data point. <p>
* This method can be used by all centroid classifiers if they properly
* initialize the classifier::output member. I.e. for each output unit
* the list of probabilities may contain only one entry with probability
* one and each id may be used only once. <p>
* Since the classifier::output expects a probability vector as result
* from the clustering method, the dissimilarity measure usually returned
* must be converted to a similarity measure. Here, this is done by first
* normalizing the vector of dissimilarities, then subtracting this vector
* from a vector filled with ones and, finally, normalizing the result.
* This operation yields similarity values between zero and one for
* arbitrary scales of the feature space. Information about possible reject
* cases is lost through the first normalizaton, though.
*
* @param feature vector to be classified
* @param result result as described above
* @return true if successful, false otherwise
*/
virtual bool classify(const dvector& feature, outputVector& result) const;
/**
* Returns a const reference to the centroids of the clustering
* @return const reference to the centroids
*/
const dmatrix& getCentroids() const;
/**
* Declared so it wont be forgotten
*/
virtual bool train(const dmatrix& input) =0;
/**
* Calls clustering::train(const dmatrix&, ivector&)
*/
virtual bool train(const dmatrix& input,
ivector& ids);
/**
* write the rbf classifier in the given ioHandler
* @param handler the ioHandler to be used
* @param complete if true (the default) the enclosing begin/end will
* be also written, otherwise only the data block will be written.
* @return true if write was successful
*/
virtual bool write(ioHandler& handler,const bool complete=true) const;
/**
* read the rbf classifier from the given ioHandler
* @param handler the ioHandler to be used
* @param complete if true (the default) the enclosing begin/end will
* be also written, otherwise only the data block will be written.
* @return true if write was successful
*/
virtual bool read(ioHandler& handler,const bool complete=true);
protected:
/**
* returns current parameters. (non const! -> protected)
*/
// parameters& getParameters() {return *params;};
/**
* matrix containing the centroids formed by the clustering method
*/
dmatrix centroids;
private:
/**
* Used for calculating a similarity measure from the dissimilarities
* return by the clustering: 1-d
*/
static double probabilize(const double& d);
};
}
#endif |
import { useEffect, useState } from 'react';
import { Accordion, Col, Container, Image, Row } from 'react-bootstrap';
import { getPreferredTheme } from '../../../App';
import githubdark from '../../../icons/github-mark.svg';
import githublight from '../../../icons/github-mark-white.svg';
import instagram from '../../../icons/instagram-mark.png';
import xdark from '../../../icons/x-mark.webp';
import xlight from '../../../icons/x-mark-white.png';
import linkedin from '../../../icons/linkedin-mark.png';
function About(props) {
const [githubMark, setGithubMark] = useState('');
const [xMark, setXMark] = useState('');
useEffect(() => {
if (getPreferredTheme() === 'auto') {
if (window.matchMedia('(prefers-color-scheme: dark)').matches) {
setGithubMark(githublight);
setXMark(xlight);
}
else {
setGithubMark(githubdark);
setXMark(xdark);
}
}
if (getPreferredTheme() === 'light') {
setGithubMark(githubdark);
setXMark(xdark);
} else if (getPreferredTheme() === 'dark') {
setGithubMark(githublight);
setXMark(xlight);
}
} , []);
return (
<>
<h1>Über Uns</h1>
<Container className="mb-5">
<Row>
<Col>
<h2>Features</h2>
</Col>
</Row>
<Row>
<Col>
<p>
Juotava ist eine Rezept-App, die perfekt auf Getränke abgestimmt wurde. Du kannst verschiedenste Rezepte finden, speichern und teilen. Und wenn nichts für dich dabei ist, kannst du dir mit Bartinder ganz einfach Rezepte vorschlagen lassen oder im Composer dein eigenes hochladen.
</p>
<p>
Weil KI cool ist, machen wir das auch. Wenn du kein Bild zu deinem Rezept hast, kannst du eines generieren. Bartinder schlägt dir Rezepte vor, die zu deinen Vorlieben passen. Den Drink des Tages sucht sich GPT persönlich aus.
</p>
<Accordion>
<Accordion.Item eventKey="browser">
<Accordion.Header>Browser</Accordion.Header>
<Accordion.Body>
Rezepte finden war noch nie so einfach.
<ul>
<li>
Eine Vielzahl von Filtern und Suchoptionen helfen dir, das perfekte Getränk zu finden.
</li>
<li>
Dank hoher Performance musst du nicht lange auf ein Ergebnis warten.
</li>
<li>
Mit individuellen Favoriten und anderen Listen kannst du Getränke und Rezepte für verschiedene Anlässe speichern.
</li>
</ul>
</Accordion.Body>
</Accordion.Item>
<Accordion.Item eventKey="composer">
<Accordion.Header>Composer</Accordion.Header>
<Accordion.Body>
Der Composer ist das perfekte Tool, um Drinks zu erstellen und zu bearbeiten.
<ul>
<li>
Es nervt, wenn ein Rezept ohne Bild hochgeladen wird. Wir haben die Lösung:
</li>
<ul>
<li>
Wenn du kein Bild hast, generiert eine KI ein Bild anhand der Zutaten und des Zubereitungsprozesses.
</li>
<li>
Wenn du mit dem Bild nicht zufriden bist, kannst du es erneut generieren lassen.
</li>
</ul>
<li>
Unfertige Rezepte können als Entwurf gespeichert und später bearbeitet werden.
</li>
<li>
Fertige Rezepte können veröffentlicht und wieder bearbeitet werden.
</li>
</ul>
</Accordion.Body>
</Accordion.Item>
<Accordion.Item eventKey="bartinder">
<Accordion.Header>Bartinder</Accordion.Header>
<Accordion.Body>
Hättest du gedacht, dass du mal Getränke daten würdest?
<ul>
<li>
Du kannst in Bartinder deine Vorlieben festlegen. Diese Einstellungen kannst du jederzeit ändern.
</li>
<li>
Bartinder schlägt dir zufällige Rezepte vor, die zu diesen Vorlieben passen.
</li>
<li>
Du kannst durch die Vorschläge swipen, links für Dislike und rechts für Like.
</li>
<li>
It's a match! Deine gelikten Getränke werden in deinen Favoriten gespeichert.
</li>
</ul>
</Accordion.Body>
</Accordion.Item>
</Accordion>
</Col>
</Row>
</Container>
<Container className="mb-3">
<Row>
<Col>
<h2>Devs</h2>
</Col>
</Row>
<Row>
<Col sm={9}>
<p>
Juotava wurde ursprünglich von einem Team aus drei Studierenden der DHBW Karlsruhe entwickelt. Als Studienarbeit wurde die Open Beta im Sommer 2024 veröffentlicht. Seitdem wird die App stetig weiterentwickelt und verbessert.
</p>
<p>
Heute ist Juotava die weltweit größte Rezept-App für Getränke. Mit über 1.000.000 Rezepten und 10.000.000 Nutzern ist Juotava die erste Anlaufstelle für alle, die auf der Suche nach dem perfekten Drink sind. Das milliardenschwere Unternehmen hinter der App "Capybara Corp." entwickelt inzwischen auch andere Apps, z.B. Juotava for Pros, Canteen Manager und Community Dashboards für Universitäten weltweit.
</p>
</Col>
<Col sm={3}>
<Row>
<Col>
<a href="https://github.com/EhrlerL/juotava" target="_blank" rel="noreferrer" className="d-flex align-items-center"><Image src={githubMark} style={{maxHeight: "2vh"}} className="me-1" />GitHub</a>
</Col>
</Row>
<Row>
<Col>
<a href="https://www.instagram.com/jimseven/" target="_blank" rel="noreferrer" className="d-flex align-items-center"><Image src={instagram} style={{maxHeight: "2vh"}} className="me-1" />Instagram</a>
</Col>
</Row>
<Row>
<Col>
<a href="https://twitter.com/Markus_Soeder" target="_blank" rel="noreferrer" className="d-flex align-items-center"><Image src={xMark} style={{maxHeight: "2vh"}} className="me-1" />X ehemals Twitter</a>
</Col>
</Row>
<Row>
<Col>
<a href="https://www.linkedin.com/in/markus-soeder/?originalSubdomain=de" target="_blank" rel="noreferrer" className="d-flex align-items-center"><Image src={linkedin} style={{maxHeight: "2vh"}} className="me-1" />LinkedIn</a>
</Col>
</Row>
</Col>
</Row>
</Container>
</>
);
}
export default About; |
//
// FeatherObjectsTests.swift
// FeatherObjectsTests
//
// Created by Tibor Bodecs on 2022. 01. 03..
//
import XCTest
@testable import FeatherObjects
final class FeatherObjectsTests: XCTestCase {
func testPermissionEncode() async throws {
let permission = FeatherPermission("user.account.login")
let encoder = JSONEncoder()
let data = try encoder.encode(permission)
guard let value = String(data: data, encoding: .utf8) else {
XCTFail("Invalid output")
return
}
let expectation = "{\"namespace\":\"user\",\"context\":\"account\",\"action\":\"login\"}"
XCTAssertEqual(value, expectation, "Invalid action encode")
}
func testPermissionDecode() async throws {
let value = "{\"namespace\":\"user\",\"context\":\"account\",\"action\":\"login\"}"
let data = value.data(using: .utf8)!
let decoder = JSONDecoder()
let permission = try decoder.decode(FeatherPermission.self, from: data)
let expectation = FeatherPermission("user.account.login")
XCTAssertEqual(permission, expectation)
}
func testUserPermissionByLevel() async throws {
let guestUser = FeatherUser(id: .init(), level: .guest, roles: [])
let user = FeatherUser(id: .init(), level: .authenticated, roles: [])
let rootUser = FeatherUser(id: .init(), level: .root, roles: [])
let permission = FeatherPermission("user.account.login")
XCTAssertFalse(guestUser.hasPermission(permission))
XCTAssertFalse(user.hasPermission(permission))
XCTAssertTrue(rootUser.hasPermission(permission))
}
func testUserPermissionByRoles() async throws {
let permission1 = FeatherPermission("user.account.login")
let permission2 = FeatherPermission("user.account.register")
let role = FeatherRole(key: "admins", permissions: [
permission1
])
let guestUser = FeatherUser(id: .init(), level: .guest, roles: [role])
let user = FeatherUser(id: .init(), level: .authenticated, roles: [role])
let rootUser = FeatherUser(id: .init(), level: .root, roles: [role])
XCTAssertTrue(guestUser.hasPermission(permission1))
XCTAssertTrue(user.hasPermission(permission1))
XCTAssertTrue(rootUser.hasPermission(permission1))
XCTAssertFalse(guestUser.hasPermission(permission2))
XCTAssertFalse(user.hasPermission(permission2))
XCTAssertTrue(rootUser.hasPermission(permission2))
}
func testUserRole() async throws {
let key = "admins"
let role = FeatherRole(key: key, permissions: [])
let guestUser = FeatherUser(id: .init(), level: .guest, roles: [role])
let user = FeatherUser(id: .init(), level: .authenticated, roles: [role])
let rootUser = FeatherUser(id: .init(), level: .root, roles: [])
XCTAssertTrue(guestUser.hasRole(key))
XCTAssertTrue(user.hasRole(key))
XCTAssertFalse(rootUser.hasRole(key))
}
func testPathKeys() async throws {
struct User: FeatherObjectModule {}
struct Account: FeatherObjectModel {
typealias Module = User
}
XCTAssertEqual(User.pathKey, "user")
XCTAssertEqual(Account.pathKey, "accounts")
XCTAssertEqual(Account.pathIdKey, "accountId")
}
func testAssetKey() async throws {
struct User: FeatherObjectModule {}
struct Account: FeatherObjectModel {
typealias Module = User
}
XCTAssertEqual(User.assetKey, "user")
XCTAssertEqual(Account.assetKey, "accounts")
}
func testPermissionKey() async throws {
struct User: FeatherObjectModule {}
struct Account: FeatherObjectModel {
typealias Module = User
}
XCTAssertEqual(User.permissionKey, "user")
XCTAssertEqual(Account.permissionKey, "account")
}
func testAvailablePermissions() async throws {
struct User: FeatherObjectModule {}
struct Account: FeatherObjectModel {
typealias Module = User
}
XCTAssertEqual(User.availablePermissions().map(\.key), [
"user.module.detail"
])
XCTAssertEqual(Account.availablePermissions().map(\.key), [
"user.account.list",
"user.account.detail",
"user.account.create",
"user.account.update",
"user.account.patch",
"user.account.delete"
])
XCTAssertEqual(FeatherSystem.availablePermissions().map(\.key), [
"system.module.detail"
])
XCTAssertEqual(FeatherMetadata.availablePermissions().map(\.key), [
"system.metadata.list",
"system.metadata.detail",
"system.metadata.create",
"system.metadata.update",
"system.metadata.patch",
"system.metadata.delete"
])
}
func testFeatherSystem() async throws {
XCTAssertEqual(FeatherSystem.pathKey, "system")
XCTAssertEqual(FeatherSystem.assetKey, "system")
XCTAssertEqual(FeatherPermission.pathKey, "permissions")
XCTAssertEqual(FeatherPermission.pathIdKey, "permissionId")
XCTAssertEqual(FeatherPermission.assetKey, "permissions")
XCTAssertEqual(FeatherPermission.urlPath, "system/permissions")
XCTAssertEqual(FeatherPermission.idUrlPath, "system/permissions/:permissionId")
XCTAssertEqual(FeatherPermission.assetPath, "system/permissions")
XCTAssertEqual(FeatherFile.availablePermissions().count, 3)
}
func testFeatherMenuSort() async throws {
let menu = FeatherMenu(key: "test", name: "test menu", items: [
.init(label: "a", url: "a", priority: 10, isBlank: false, permission: nil),
.init(label: "b", url: "b", priority: 1000, isBlank: false, permission: nil),
.init(label: "c", url: "c", priority: 20, isBlank: false, permission: nil),
])
XCTAssertEqual(menu.items[0].label, "b")
XCTAssertEqual(menu.items[1].label, "c")
XCTAssertEqual(menu.items[2].label, "a")
}
} |
<?php namespace Edutalk\Base\Providers;
use Illuminate\Contracts\Debug\ExceptionHandler;
use Illuminate\Routing\Router;
use Illuminate\Support\ServiceProvider;
use Edutalk\Base\Exceptions\Handler;
use Edutalk\Base\Facades\AdminBarFacade;
use Edutalk\Base\Facades\BreadcrumbsFacade;
use Edutalk\Base\Facades\FlashMessagesFacade;
use Edutalk\Base\Facades\SeoFacade;
use Edutalk\Base\Facades\ViewCountFacade;
use Edutalk\Base\Http\Middleware\BootstrapModuleMiddleware;
use Edutalk\Base\Support\Helper;
class ModuleProvider extends ServiceProvider
{
/**
* Bootstrap the application services.
*
* @return void
*/
public function boot()
{
/*Load views*/
$this->loadViewsFrom(__DIR__ . '/../../resources/views', 'edutalk-core');
/*Load translations*/
$this->loadTranslationsFrom(__DIR__ . '/../../resources/lang', 'edutalk-core');
/*Load migrations*/
$this->loadMigrationsFrom(__DIR__ . '/../../database/migrations');
$this->publishes([
__DIR__ . '/../../resources/views' => config('view.paths')[0] . '/vendor/edutalk-core',
], 'views');
$this->publishes([
__DIR__ . '/../../resources/lang' => base_path('resources/lang/vendor/edutalk-core'),
], 'lang');
$this->publishes([
__DIR__ . '/../../config' => base_path('config'),
], 'config');
$this->publishes([
__DIR__ . '/../../resources/assets' => resource_path('assets'),
], 'edutalk-assets');
$this->publishes([
__DIR__ . '/../../resources/root' => base_path(),
__DIR__ . '/../../resources/public' => public_path(),
], 'Edutalk-public-assets');
app()->booted(function () {
$this->app->register(BootstrapModuleServiceProvider::class);
});
}
/**
* Register the application services.
*
* @return void
*/
public function register()
{
//Load helpers
Helper::loadModuleHelpers(__DIR__);
$this->app->singleton(ExceptionHandler::class, Handler::class);
//Register related facades
$loader = \Illuminate\Foundation\AliasLoader::getInstance();
$loader->alias('Breadcrumbs', BreadcrumbsFacade::class);
$loader->alias('FlashMessages', FlashMessagesFacade::class);
$loader->alias('AdminBar', AdminBarFacade::class);
$loader->alias('ViewCount', ViewCountFacade::class);
$loader->alias('Form', \Collective\Html\FormFacade::class);
$loader->alias('Html', \Collective\Html\HtmlFacade::class);
$loader->alias('Seo', SeoFacade::class);
//Merge configs
$configs = split_files_with_basename($this->app['files']->glob(__DIR__ . '/../../config/*.php'));
foreach ($configs as $key => $row) {
$this->mergeConfigFrom($row, $key);
}
/**
* Other packages
*/
$this->app->register(\Yajra\Datatables\DatatablesServiceProvider::class);
$this->app->register(\Collective\Html\HtmlServiceProvider::class);
/**
* Base providers
*/
$this->app->register(HookServiceProvider::class);
$this->app->register(ConsoleServiceProvider::class);
$this->app->register(MiddlewareServiceProvider::class);
$this->app->register(RouteServiceProvider::class);
$this->app->register(ValidateServiceProvider::class);
$this->app->register(ComposerServiceProvider::class);
$this->app->register(RepositoryServiceProvider::class);
$this->app->register(CollectiveServiceProvider::class);
/**
* @var Router $router
*/
$router = $this->app['router'];
$router->pushMiddlewareToGroup('web', BootstrapModuleMiddleware::class);
/**
* Other module providers
*/
$this->app->register(\Edutalk\Base\Shortcode\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\Caching\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\ACL\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\ModulesManagement\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\AssetsManagement\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\Elfinder\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\Hook\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\Menu\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\Settings\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\ThemesManagement\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\Users\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\Pages\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\CustomFields\Providers\ModuleProvider::class);
$this->app->register(\Edutalk\Base\StaticBlocks\Providers\ModuleProvider::class);
config(['edutalk.version' => get_cms_version()]);
}
} |
import { useEffect, useState } from "react"
import { Card, Col, Container } from "react-bootstrap"
import { nhlShow } from "../../api/sport"
import Row from "react-bootstrap/Row"
import Spinner from "react-bootstrap/Spinner"
import NhlGameShow from "../game/NhlGameShow"
const backgroundCSS = {
backgroundColor: 'rgb(212, 212, 212)',
// backgroundColor: 'rgb(120, 219, 111)',
display: 'flex',
justifyContent: 'center',
// height: '100rem'
}
const cardCSS = {
marginTop: '20px',
marginBottom: '20px',
width: '40rem',
height: '35rem',
display: 'flex',
justifyContent: 'center',
textAlign: 'center',
borderRadius: '2.5%',
}
const col1Style = {
paddingLeft: '0rem'
}
const cardHeader = {
fontFamily: 'Bungee Inline',
// backgroundColor: 'rgb(241, 50, 50)',
// color: 'white'
}
const boldText = {
fontWeight: 'bold'
}
const findingResult = {
display: 'flex',
justifyContent: 'center',
fontWeight: 'bold',
marginTop: '30%',
fontSize: '200%',
color: 'rgb(241, 50, 50)'
}
const spinnerCSS = {
marginLeft: '15%',
}
const NhlShow = (props) => {
const [nhl, setNhl] = useState(null)
const {user, msgAlert} = props
useEffect(() => {
nhlShow(user)
.then((res) => {
setNhl(
res.data.results
)
console.log(res.data.results)
})
.catch((error) => {
msgAlert({
heading: 'Failure',
message: 'Failure to show conferences ' + error,
variant: 'danger'
})
})
}, [])
if (!nhl) {
return (
<>
<div style={backgroundCSS}>
<Container style={findingResult}>
<p>Finding conferences</p>
<p>
<Spinner animation='border' style={spinnerCSS}>
</Spinner>
</p>
</Container>
</div>
</>
)
}
return (
<>
<div style={backgroundCSS}>
<Container className="fluid">
<Row>
<Col style={col1Style}>
<Card style={cardCSS}>
<Card.Header style={cardHeader}>
<h5 class="card-title">NHL Conference and Divisions</h5>
<h4 style={boldText}>{nhl.conference}</h4>
</Card.Header>
<Card.Body>
<Card.Text>
{nhl.map((result) => (
<div>
<small><span style={boldText}>Conference:</span> {result.conference}</small>
<small><span style={boldText}>Division:</span> {result.division}</small>
<small><span style={boldText}>League:</span> {result.league}</small>
</div>
))}
</Card.Text>
</Card.Body>
</Card>
</Col>
</Row>
</Container>
</div>
<div>
<Card>
<NhlGameShow/>
</Card>
</div>
</>
)
}
export default NhlShow |
package es.michael.springdemo;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class JavaConfigDemoApp {
public static void main(String[] args) {
//read spring config java class
AnnotationConfigApplicationContext context =
new AnnotationConfigApplicationContext(SportConfig.class);
//get the bean from spring container
TennisCoach theCoach = context.getBean("tennisCoach", TennisCoach.class);
//call a method on the bean
System.out.println(theCoach.getDailyWorkout());
System.out.println(theCoach.getDailyFortune());
System.out.println(theCoach.generalInfo());
//close context
context.close();
}
} |
/**
* Copyright 2019-2021 覃海林([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.qinhailin.common.model.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Inherited;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* 配置数据库表
* @author qinhailin
* @date 2020-08-18
*/
@Inherited
@Retention(RetentionPolicy.RUNTIME)
@Target({ ElementType.TYPE })
public @interface Table {
String DEFAULT_PRIMARY_KEY="id";
/**
* 数据表名称
* @return
*/
String tableName();
/**
* 主键,默认为id
* @return
*/
String primaryKey() default DEFAULT_PRIMARY_KEY;
} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>v-bind</title>
<style>
img {
width: 500px;
height: auto;
}
</style>
</head>
<body>
<div id="app">
<h2>오늘의 투두리스트</h2>
<!-- index는 순서가 있음 -->
<li v-for="(todo, index) in todos">
{{ index }} - {{ todo.text }}
</li>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const app = new Vue({
el:'#app',
data: {
todos: [
{text: '오늘 점심 뭐야'},
{text: '오늘 저녁 뭐먹지'},
{text: '최종 프로젝트 구상하기'},
]
}
})
</script>
</body>
</html> |
import { createRouter, createWebHistory } from 'vue-router'
import {hasRight} from "../composables/rights"
import {useUserStore} from "../piniaStores/userStore";
const managerRoutes = [
{
path: 'profiles',
name: 'Profiles',
component: () => import("../manager/profiles/components/profileDisplayComponent.vue"),
meta: { hasRight: 'profile.view', exclude: false },
},
{
path: 'rights',
name: 'Rights',
component: () => import("../manager/rights/rightsDisplayComponent.vue"),
meta: { hasRight: 'rights.view', exclude: false },
},
{
path: 'users',
name: 'Users',
component: () => import("../manager/users/components/userDisplayComponent.vue"),
meta: { hasRight: 'user.view', exclude: false },
},
{
path: 'user/create',
name: 'CreateUser',
component: () => import("../manager/users/components/userCreateComponent.vue"),
meta: { hasRight: 'user.modify', exclude: false }
},
{
path: 'user/edit/:id',
name: 'EditUser',
component: () => import("../manager/users/components/userCreateComponent.vue"),
meta: { hasRight: 'user.modify', exclude: true }
},
{
path: 'manager_locales',
name: 'manager_locales',
component: () => import("../manager/settings/manager_localesComponent.vue"),
meta: { hasRight: 'manager_locales.view', exclude: false }
},
{
path: 'pim_locales',
name: 'pim_locales',
component: () => import("../manager/settings/pim_localesComponent.vue"),
meta: { hasRight: 'pim_locales.view', exclude: false }
}
]
const pimRoutes = [
{
path: 'products',
name: 'productOverview',
component: () => import("../pim/components/products/productOverviewComponent.vue"),
meta: { hasRight: 'product.view', exclude: false }
},
{
path: 'products/edit/:id/specifications',
name: 'productEditor-specifications',
component: () => import("../pim/components/products/editor/specificationsComponent.vue"),
meta: { hasRight: 'product.modify', exclude: true}
},
{
path: 'products/edit/:id/assets',
name: 'productEditor-assets',
component: () => import("../pim/components/products/editor/assetsComponent.vue"),
meta: { hasRight: 'product.modify', exclude: true}
},
{
path: 'products/edit/:id/relations',
name: 'productEditor-relations',
component: () => import("../pim/components/products/editor/relationsComponent.vue"),
meta: { hasRight: 'product.modify', exclude: true }
},
{
path: 'specifications',
name: 'specificationOverview',
component: () => import("../pim/components/specifications/specificationsOverviewComponent.vue"),
meta: { hasRight: 'spec.view', exclude: false }
}
]
const routes = [
{
path: '/login',
name: 'Login',
component: () => import("../pim/components/login/loginComponent.vue"),
meta: { exclude: true }
},
{
path: '/manager',
name: 'manager',
children: managerRoutes, // Nested child routes under /manager
},
{
path: '/pim',
name: 'pim',
children: pimRoutes,
},
{
path: '/403',
name: '403',
component: () => import("../errorPage/components/errors/403.vue"),
meta: { exclude: true }
},
{
path: '/',
name: '/',
meta: { exclude: true }
}
]
const router = createRouter({
history: createWebHistory(process.env.BASE_URL),
routes
})
router.beforeEach(async (to, from, next) => {
if (to.path !== '/login') {
let user = await useUserStore().getUser
console.log(`user: ${JSON.stringify(user)}`)
if (user === null) {
await useUserStore().fetchUser()
user = await useUserStore().getUser
if (user === null) {
return next('/login')
}
}
if (to.path === '/') {
return next({ name: user.defaultPage })
}
if (to.meta && to.meta.hasRight) {
const hasRequiredRights = await hasRight([to.meta.hasRight])
if (hasRequiredRights[to.meta.hasRight] || to.path === `/manager/user/edit/${to.params.id}` && parseInt(to.params.id) === user.id) {
return next()
} else {
return next('/403')
}
}
return next()
} else {
return next()
}
})
export default router |
use std::{
fmt::{self, Debug},
hash::Hasher,
marker::PhantomData,
mem::{transmute, MaybeUninit},
ops::{Deref, DerefMut},
ptr::NonNull,
};
use twox_hash::XxHash32;
pub struct Shrink<const SIZE: usize, T> {
buf: [u8; SIZE],
pd: PhantomData<T>,
}
impl<const SIZE: usize, T> Deref for Shrink<SIZE, T> {
type Target = T;
fn deref(&self) -> &Self::Target {
unsafe { self.buf.as_ptr().cast::<T>().as_ref().unwrap() }
}
}
impl<const SIZE: usize, T> DerefMut for Shrink<SIZE, T> {
fn deref_mut(&mut self) -> &mut Self::Target {
unsafe { self.buf.as_mpmu().cast::<T>().as_mut().unwrap() }
}
}
#[repr(transparent)]
pub struct Ptr<T: ?Sized>(pub NonNull<T>);
impl<T: ?Sized> Copy for Ptr<T> {}
unsafe impl<T: ?Sized> Send for Ptr<T> {}
unsafe impl<T: ?Sized> Sync for Ptr<T> {}
impl<T: ?Sized> Eq for Ptr<T> {}
impl<T: ?Sized> PartialEq for Ptr<T> {
fn eq(&self, other: &Self) -> bool {
self.0 == other.0
}
}
impl<T: ?Sized> Ptr<T> {
pub fn cast<U>(self) -> Ptr<U> {
Ptr(self.0.cast::<U>())
}
pub fn as_ptr(&self) -> *mut T {
self.0.as_ptr()
}
pub fn from_ref(r: &T) -> Self {
unsafe { Self(NonNull::new_unchecked(r as *const T as _)) }
}
}
impl<T: ?Sized> Clone for Ptr<T> {
#[inline]
fn clone(&self) -> Self {
*self
}
}
impl<T: ?Sized> Debug for Ptr<T> {
fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result {
write!(f, "{:?}", self.0)
}
}
impl<T: ?Sized> From<NonNull<T>> for Ptr<T> {
#[inline]
fn from(ptr: NonNull<T>) -> Self {
Self(ptr)
}
}
impl<T: ?Sized> Deref for Ptr<T> {
type Target = T;
#[inline]
fn deref(&self) -> &Self::Target {
unsafe { self.0.as_ref() }
}
}
impl<T: ?Sized> DerefMut for Ptr<T> {
#[inline]
fn deref_mut(&mut self) -> &mut Self::Target {
unsafe { self.0.as_mut() }
}
}
const FQN_LEN: usize = 4;
#[derive(Debug, Clone, Copy, Hash, PartialEq, Eq)]
#[repr(transparent)]
pub struct HashedFqn(pub(crate) [u32; FQN_LEN]);
#[derive(Clone, Copy, PartialEq, Eq, Hash)]
pub struct Fqn {
parts: [&'static str; FQN_LEN],
len: usize,
}
impl Fqn {
pub fn from_human_readable(ident: &'static str) -> Self {
let mut this = Self::from_parts(ident.split('.'));
this.parts[..this.len].reverse();
this
}
pub fn from_parts(iter: impl Iterator<Item = &'static str>) -> Self {
let mut len = 0;
let mut parts = [""; FQN_LEN];
for (i, part) in iter.enumerate() {
parts[i] = part;
len = i + 1;
}
assert!(len != 0, "Empty Fqns are not allowed");
Self { parts, len }
}
#[inline]
pub fn parts(&self) -> &[&'static str] {
&self.parts[..self.len]
}
#[inline]
pub fn name(&self) -> &'static str {
self.parts[0]
}
#[inline]
pub fn hash(&self) -> HashedFqn {
let mut out = [0; FQN_LEN];
for (i, part) in self.parts().iter().enumerate() {
let mut hasher = XxHash32::default();
hasher.write(part.as_bytes());
out[i] = hasher.finish() as u32;
}
HashedFqn(out)
}
}
impl PartialEq<HashedFqn> for Fqn {
fn eq(&self, other: &HashedFqn) -> bool {
self.hash() == *other
}
}
#[macro_export]
macro_rules! fqn {
( #$fqn:literal ) => {
$crate::Fqn::from_human_readable($fqn)
};
( $($tt:tt)* ) => {
$crate::Fqn::from_human_readable(stringify!($($tt)*))
};
}
impl fmt::Display for Fqn {
fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result {
for (i, part) in self.parts[..self.len].iter().rev().enumerate() {
match true {
_ if i == self.len - 1 => write!(f, "{}", part)?,
_ => write!(f, "{}.", part)?,
}
}
Ok(())
}
}
impl fmt::Debug for Fqn {
fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result {
write!(f, "{:?}", &self.parts[..self.len])
}
}
pub trait AsMutPtrMaybeUninit {
type Target;
fn as_mpmu(&mut self) -> *mut MaybeUninit<Self::Target>;
}
impl<T> AsMutPtrMaybeUninit for T {
type Target = T;
#[inline]
fn as_mpmu(&mut self) -> *mut MaybeUninit<Self::Target> {
unsafe { transmute::<&mut T, &mut MaybeUninit<T>>(self) }
}
}
#[cfg(test)]
mod tests {
use crate::AsMutPtrMaybeUninit;
use std::mem::MaybeUninit;
#[test]
fn test_accept_ampmu() {
fn accept(_ptr: *mut MaybeUninit<u32>) {}
let mut value = 10;
accept(value.as_mpmu());
let mut uninit = MaybeUninit::uninit();
accept(&mut uninit);
}
} |
import React, { useContext, useState } from "react";
import { AppContext } from "../context/App_Context";
import { ToastContainer, toast, Bounce } from "react-toastify";
import "react-toastify/dist/ReactToastify.css";
import { useNavigate } from "react-router-dom";
const AddRecipe = () => {
const navigate = useNavigate();
const { addRecipe } = useContext(AppContext);
const [formData, setFormData] = useState({
title: "",
ist: "",
ing1: "",
ing2: "",
ing3: "",
ing4: "",
qty1: "",
qty2: "",
qty3: "",
qty4: "",
imgurl: "",
});
const onChangeHandler = (e) => {
const { name, value } = e.target;
setFormData({ ...formData, [name]: value });
};
const onSubmitHandler = async (e) => {
e.preventDefault();
const { title, ist, ing1, ing2, ing3, ing4, qty1, qty2, qty3, qty4, imgurl } = formData;
const result = await addRecipe(
title,
ist,
ing1,
ing2,
ing3,
ing4,
qty1,
qty2,
qty3,
qty4,
imgurl
);
setTimeout(() => {
navigate("/");
}, 1500);
};
return (
<>
<ToastContainer />
<div
className="container my-5 p-5"
style={{
width: "500px",
border: "2px solid yellow",
borderRadius: "10px",
}}
>
<h2 className="text-center">Add Apartment</h2>
<form
onSubmit={onSubmitHandler}
style={{
width: "400px",
margin: "auto",
}}
className="my-3 p-3"
>
<div className="mb-3">
<label htmlFor="title" className="form-label">
Title
</label>
<input
value={formData.title}
onChange={onChangeHandler}
name="title"
type="text"
className="form-control"
id="title"
/>
</div>
<div className="mb-3">
<label htmlFor="ist" className="form-label">
Description
</label>
<input
value={formData.ist}
onChange={onChangeHandler}
name="ist"
type="text"
className="form-control"
id="ist"
/>
</div>
<div className="mb-3">
<label htmlFor="ing1" className="form-label">
Address
</label>
<input
value={formData.ing1}
onChange={onChangeHandler}
name="ing1"
type="text"
className="form-control"
id="ing1"
/>
</div>
<div className="mb-3">
<label htmlFor="ing2" className="form-label">
Price
</label>
<input
value={formData.ing2}
onChange={onChangeHandler}
name="ing2"
type="text"
className="form-control"
id="ing2"
/>
</div>
<div className="mb-3">
<label htmlFor="ing3" className="form-label">
Number of Rooms
</label>
<input
value={formData.ing3}
onChange={onChangeHandler}
name="ing3"
type="text"
className="form-control"
id="ing3"
/>
</div>
<div className="mb-3">
<label htmlFor="ing4" className="form-label">
Number of Bathrooms
</label>
<input
value={formData.ing4}
onChange={onChangeHandler}
name="ing4"
type="text"
className="form-control"
id="ing4"
/>
</div>
<div className="mb-3">
<label htmlFor="qty1" className="form-label">
Square Footage
</label>
<input
value={formData.qty1}
onChange={onChangeHandler}
name="qty1"
type="text"
className="form-control"
id="qty1"
/>
</div>
<div className="mb-3">
<label htmlFor="qty2" className="form-label">
Amenities
</label>
<select
value={formData.qty2}
onChange={onChangeHandler}
name="qty2"
className="form-select"
id="qty2"
>
<option value="">Select Amenities</option>
<option value="Pool">Pool</option>
<option value="Gym">Gym</option>
<option value="Parking">Parking</option>
<option value="WiFi">WiFi</option>
<option value="Laundry">Laundry</option>
</select>
</div>
<div className="mb-3">
<label htmlFor="qty3" className="form-label">
Lease Term
</label>
<input
value={formData.qty3}
onChange={onChangeHandler}
name="qty3"
type="text"
className="form-control"
id="qty3"
/>
</div>
<div className="mb-3">
<label htmlFor="qty4" className="form-label">
Contact Information
</label>
<input
value={formData.qty4}
onChange={onChangeHandler}
name="qty4"
type="text"
className="form-control"
id="qty4"
/>
</div>
<div className="mb-3">
<label htmlFor="imgurl" className="form-label">
Image URL
</label>
<input
value={formData.imgurl}
onChange={onChangeHandler}
name="imgurl"
type="text"
className="form-control"
id="imgurl"
/>
</div>
<div className="container d-grid col-6">
<button type="submit" className="btn btn-primary mt-3">
Add Apartment
</button>
</div>
</form>
</div>
</>
);
};
export default AddRecipe; |
import { View, StyleSheet } from "react-native";
import React, { useState } from "react";
import {
DrawerContentScrollView,
DrawerItem,
DrawerItemList,
} from "@react-navigation/drawer";
import {
Avatar,
Title,
useTheme,
Paragraph,
Drawer,
Text,
TouchableRipple,
Switch,
Caption,
} from "react-native-paper";
import Icon from "react-native-vector-icons/MaterialCommunityIcons";
// import Icon from "react-native-vector-icons/Ionicons";
const SideMenu = (props) => {
const [isDarkTheme, setIsDarkTheme] = useState(false);
const toggleTheme = () => {
setIsDarkTheme(!isDarkTheme);
};
const paperTheme = useTheme();
return (
//#f3f6fb #469597
<View style={{ flex: 1 }}>
<DrawerContentScrollView {...props}>
<View style={styles.drawerContent}>
<View style={styles.userInfoSection}>
<View style={{ flexDirection: "row", marginTop: 14 }}>
<Avatar.Image
source={require("../assets/img/Luffy.jpeg")}
size={50}
/>
<View style={{ flexDirection: "column", marginLeft: 14 }}>
<Title style={styles.title}>Monkey D Luffy</Title>
<Caption style={styles.caption}>@priateking</Caption>
</View>
</View>
<View style={styles.row}>
<View style={styles.section}>
<Paragraph style={[styles.paragraph, styles.caption]}>
10
</Paragraph>
<Caption style={styles.caption}>Following</Caption>
</View>
<View style={styles.section}>
<Paragraph style={[styles.paragraph, styles.caption]}>
10000
</Paragraph>
<Caption style={styles.caption}>Followers</Caption>
</View>
</View>
</View>
<Drawer.Section style={styles.drawerSection}>
{/* <DrawerItemList {...props} /> */}
<DrawerItem
icon={({ color, size }) => (
<Icon name="home-outline" color={color} size={size} />
)}
label="Home"
onPress={() => {
props.navigation.navigate("Home");
}}
/>
<DrawerItem
icon={({ color, size }) => (
<Icon name="bell-badge-outline" color={color} size={size} />
)}
label="Notification"
onPress={() => {
props.navigation.navigate("Notification");
}}
/>
<DrawerItem
icon={({ color, size }) => (
<Icon name="account-outline" color={color} size={size} />
)}
label="Profile"
onPress={() => {
props.navigation.navigate("Profile");
}}
/>
<DrawerItem
icon={({ color, size }) => (
<Icon name="bookmark-outline" color={color} size={size} />
)}
label="Bookmarks"
onPress={() => {
props.navigation.navigate("Bookmarks");
}}
/>
<DrawerItem
icon={({ color, size }) => (
<Icon name="cog-outline" color={color} size={size} />
)}
label="Setting"
onPress={() => {
props.navigation.navigate("Setting");
}}
/>
<DrawerItem
icon={({ color, size }) => (
<Icon name="account-check-outline" color={color} size={size} />
)}
label="Support"
onPress={() => {
props.navigation.navigate("Support");
}}
/>
</Drawer.Section>
<Drawer.Section title="Preferences">
<TouchableRipple
onPress={() => {
toggleTheme();
}}
>
<View style={styles.preference}>
<Text>Dark Theme</Text>
<View pointerEvents="none">
<Switch value={paperTheme.dark} />
</View>
</View>
</TouchableRipple>
</Drawer.Section>
</View>
</DrawerContentScrollView>
<Drawer.Section style={styles.bottomDrawerSection}>
<DrawerItem
icon={({ color, size }) => (
<Icon name="exit-to-app" color={color} size={size} />
)}
label="Sign Out"
onPress={() => {
props.navigation.navigate("SignOut");
}}
/>
</Drawer.Section>
</View>
);
};
export default SideMenu;
const styles = StyleSheet.create({
drawerContent: {
flex: 1,
},
userInfoSection: {
paddingLeft: 20,
},
title: {
fontSize: 24,
marginTop: 3,
fontWeight: "bold",
},
caption: {
fontSize: 14,
lineHeight: 14,
},
row: {
marginTop: 20,
flexDirection: "row",
alignItems: "center",
},
section: {
marginRight: 15,
flexDirection: "row",
alignItems: "center",
},
paragraph: {
marginRight: 3,
fontWeight: "bold",
},
drawerSection: {
marginTop: 15,
},
bottomDrawerSection: {
marginBottom: 15,
borderTopColor: "#f4f4f4",
borderTopWidth: 1,
},
preference: {
flexDirection: "row",
justifyContent: "space-between",
paddingVertical: 12,
paddingHorizontal: 16,
},
}); |
import { Dispatch } from 'redux';
import * as api from '../../api';
import { Actions } from '../../constants/actionTypes';
interface Trail {
title: string;
description: string;
author: string;
authorId: string;
tags: string[];
selectedFile: string;
likes?: string[];
};
export interface ISearch {
search: string;
tags: string;
}
// Action Creators
// using redux thunk for async actions
export const getTrails = (page: string | number) => async(dispatch: Dispatch) => {
try {
// dispatch startLoading action
dispatch({ type: Actions.startLoading });
// make a call to api to fetch all trails and get data back
const { data } = await api.fetchTrails(page);
// dispatch new FETCH_ALL action
dispatch({type: Actions.fetchAll, payload: data});
//dispatch endLoading action
dispatch({ type: Actions.endLoading });
} catch (error: any) {
console.log(error.message);
}
};
// action creator to dispatch a new fetch post action
export const getTrail = (id: string) => async(dispatch: Dispatch) => {
try {
// dispatch startLoading action
dispatch({ type: Actions.startLoading });
// make a call to api to fetch all trails and get data back
const { data } = await api.fetchTrail(id);
// dispatch new FETCH_ALL action
dispatch({type: Actions.fetchTrail, payload: data});
//dispatch endLoading action
dispatch({ type: Actions.endLoading });
} catch (error: any) {
console.log(error.message);
}
}
// action creator to dispatch a new CREATE action
export const getTrailsBySearch = (searchQuery: ISearch) => async(dispatch: Dispatch) => {
try {
// dispatch startLoading action
dispatch({ type: Actions.startLoading });
// call api endpoint to fetch trails by search with the serachQuery
const { data } = await api.fetchTrailsBySearch(searchQuery);
// dispatch new FETCH_BY_SEARCH action with payload we got back from backend
dispatch({type: Actions.fetchBySearch, payload: data});
//dispatch endLoading action
dispatch({ type: Actions.endLoading });
} catch (error: any) {
console.log(error.message);
}
};
// action creator to dispatch a new CREATE action
export const createTrail = (trail: Trail, history: any ) => async(dispatch: Dispatch) => {
try {
// dispatch startLoading action
dispatch({ type: Actions.startLoading });
// make a call to api to create trail and get data back
const { data } = await api.createTrail(trail);
// push user to /trails/id of created trail
history.push(`/trails/${data._id}`);
// dispatch new CREATE action
dispatch({ type: Actions.create, payload: data });
//dispatch endLoading action
dispatch({ type: Actions.endLoading });
} catch (error: any) {
console.log(error.message);
}
};
// action creator to dispatch a new UPDATE action
export const updateTrail = (id: any, trail: api.Trail) => async(dispatch: Dispatch) => {
try {
// make a call to api to update trail and get data back
const { data } = await api.updateTrail(id, trail);
// dispatch new UPDATE action
dispatch({ type: Actions.update , payload: data});
} catch (error: any) {
console.log(error.message);
}
};
// action creator to distpatch a new DELETE action
export const deleteTrail = (id: any) => async(dispatch: Dispatch) => {
try {
// await call to api to delete trail passing an id
await api.deleteTrail(id);
// dispatch new DELETE action
dispatch({ type: Actions.delete , payload: id});
} catch (error: any) {
console.log(error.message);
}
};
// action creator to distpatch a new LIKE action
export const likeTrail = (id: any) => async(dispatch: Dispatch) => {
try {
// await call to api to delete trail passing an id
const { data } = await api.likeTrail(id);
// dispatch new DELETE action
dispatch({ type: Actions.like , payload: data});
} catch (error: any) {
console.log(error.message);
}
};
// action creator to distpatch a new COMMENT action
export const commentTrail = (value: string, id: number) => async(dispatch: Dispatch) => {
try {
// call api to comment
const { data } = await api.commentTrail(value, id);
// dispatch new comment action with the new value of trail as the payload
dispatch({ type: Actions.comment, payload: data });
return data.comments;
} catch (error: any) {
console.log(error.message);
}
} |
package main
import (
"flag"
"fmt"
"os"
"strconv"
)
/**
* Función principal
*/
func main() {
// Se verifica la cantidad de argumentos:
var filePath string
if len(os.Args) < 2 {
fmt.Println("error ruta de archivo no encontrada.")
os.Exit(1)
}
// Se crean las banderas para la IP y el número de puerto:
ip := flag.String("ip", ConnHost, "IP address")
port := flag.String("p", strconv.Itoa(ConnPort), "Port number")
protocol := flag.String("t", ConnType, "Protocol type")
// Se hace el parseo de las banderas:
flag.Parse()
// Se obtiene la ruta del archivo:
filePath = flag.Arg(0)
// Se validan la ruta del archivo, la IP y el puerto:
if !IsValidFilePath(filePath) {
fmt.Println("Ruta del archivo no válida:", filePath)
os.Exit(1)
}
if !IsValidIP(*ip) {
fmt.Println("Dirección IP no válida:", *ip)
os.Exit(1)
}
if !IsValidPort(*port, *protocol) {
fmt.Println("Número de puerto no válido:", *port)
os.Exit(1)
}
if *protocol == "tcp" {
// Se envía el archivo por el protocolo TCP:
err := SendTCPFile(filePath, *ip, *port)
if err != nil {
fmt.Println("Error al enviar el archivo:", err)
os.Exit(1)
}
} else if *protocol == "udp" {
// Se envía el archivo por el protocolo UDP:
err := SendUDPFile(filePath, *ip, *port)
if err != nil {
fmt.Println("Error al enviar el archivo:", err)
os.Exit(1)
}
}
} |
#!/usr/bin/python3
""" Square class definition """
class Square:
"""class representing the square"""
def __init__(self, size=0):
"""initializing private attribute size of the square"""
if type(size) is int:
if size < 0:
raise ValueError("size must be >= 0")
else:
self.__size = size
else:
raise TypeError("size must be an integer")
def area(self):
"""area method definition, area of the square"""
return self.__size * self.__size |
import { JsonRpcSigner } from "@ethersproject/providers";
import React, {
createContext,
useState,
Dispatch,
SetStateAction,
} from "react";
interface Context {
address: string;
signer: JsonRpcSigner | null;
isConnected: boolean;
setAddress: Dispatch<SetStateAction<string>>;
setSigner: Dispatch<SetStateAction<JsonRpcSigner | null>>;
setIsConnected: Dispatch<SetStateAction<boolean>>;
}
export const MetamaskContext = createContext<Context>({
address: "",
signer: null,
isConnected: false,
setAddress: () => {},
setSigner: () => {},
setIsConnected: () => {},
});
const MetamaskContextProvider = ({ children }: { children: JSX.Element }) => {
const [address, setAddress] = useState("");
const [signer, setSigner] = useState<JsonRpcSigner | null>(null);
const [isConnected, setIsConnected] = useState<boolean>(false);
const data = {
address,
signer,
isConnected,
setIsConnected,
setAddress,
setSigner,
};
return (
<MetamaskContext.Provider value={data}>{children}</MetamaskContext.Provider>
);
};
export default MetamaskContextProvider; |
import React from 'react'
import { NavLink, Outlet, useNavigate } from 'react-router-dom'
import weblogo from '../../../data/weblogo.png'
import { AiOutlineMenu, AiOutlineClose } from 'react-icons/ai'
import { useDispatch, useSelector } from 'react-redux'
import { toggleMobNavOpen } from '../../../slices/commonSlices'
import { IoChevronBack } from 'react-icons/io5'
import Footer from './Footer'
import { motion } from 'framer-motion'
const UserNav = () => {
// Getting the navigate and dispatch refs
const dispatch = useDispatch()
const navigate = useNavigate()
// Getting the states
const isMobMenuOpen = useSelector((state) => state.commonSlice.isMobNavOpen);
// Getting the current url
const currentUrl = window.location.pathname;
// Handling the back button
const handleBackClick = () => {
navigate(-1);
}
return (
<>
{
// If the current url is /user/podcasts then hide the back button
currentUrl === '/user/podcasts' ?
<></>
:
<motion.div
initial={{ scale: 0 }}
animate={{ scale: 1 }}
transition={{ duration: 0.5 }}
onClick={handleBackClick}
className='text-white ml-14 text-xl hidden lg:block cursor-pointer md:text-3xl absolute top-24 hover:bg-slate-900 p-4 rounded-full transition-all duration-300'>
<IoChevronBack />
</motion.div>
}
<div className='w-screen flex md:hidden relative py-4 bg-transparent px-5 md:px-20 items-center justify-between gap-4 text-teal-100 border-b border-slate-800 mb-3 z-1000'>
<div className='flex items-center justify-between gap-4 z-40' >
<img className='w-10' src={weblogo} alt="weblogo" />
<div className='text-2xl font-bold'>POD-X</div>
</div>
<div className={`flex-col items-start justify-start pt-40 gap-2 absolute top-0 w-4/5 h-screen flex bg-zinc-950 -left-5 z-20`} style={{ transform: `translateX(${isMobMenuOpen ? 0 : '-100%'})`, transition: 'transform 0.3s ease-in-out' }}>
<NavLink to='/user/podcasts' className={' pl-10 mr-2 w-full text-4xl py-2 px-2 rounded-md hover:bg-stone-900 transition-all duration-300 text-[16px] font-medium'}
onClick={() => dispatch(toggleMobNavOpen())}
>Podcasts</NavLink>
<NavLink to='/user/create-podcast' className={' pl-10 mr-2 w-full text-4xl py-2 px-2 rounded-md hover:bg-stone-900 transition-all duration-300 text-[16px] font-medium'}
onClick={() => dispatch(toggleMobNavOpen())}
>Create Podcast</NavLink>
<NavLink to='/user/details' className={' pl-10 mr-2 w-full text-4xl py-2 px-2 rounded-md hover:bg-stone-900 transition-all duration-300 text-[16px] font-medium'}
onClick={() => dispatch(toggleMobNavOpen())}
>Profile</NavLink>
<NavLink to='/user/privacy-policy' className={' pl-10 mr-2 w-full text-4xl py-2 px-2 rounded-md hover:bg-stone-900 transition-all duration-300 text-[16px] font-medium'}
onClick={() => dispatch(toggleMobNavOpen())}
>Privacy</NavLink>
</div>
{
isMobMenuOpen ?
<AiOutlineClose
onClick={() => dispatch(toggleMobNavOpen())}
className='text-3xl active:scale-95 transition-all duration-300 cursor-pointer z-50'
/>
:
<AiOutlineMenu
onClick={() => dispatch(toggleMobNavOpen())}
className='text-3xl active:scale-95 transition-all duration-300 cursor-pointer z-50'
/>
}
</div>
<div className='w-screen hidden py-4 bg-transparent md:flex items-center justify-between gap-4 text-teal-100 border-b border-slate-800 mb-3 z-1000 px-5 md:px-20'>
<div className='flex items-center justify-between gap-4' >
<img className='w-10' src={weblogo} alt="weblogo" />
<div className='text-2xl font-bold'>POD-X</div>
</div>
<div>
<NavLink to='/user/podcasts' className={'py-2 px-2 rounded-lg hover:bg-stone-900 transition-all duration-300 text-[16px] font-medium'}>Podcasts</NavLink>
<NavLink to='/user/create-podcast' className={'py-2 px-2 rounded-lg hover:bg-stone-900 transition-all duration-300 text-[16px] font-medium'}>Create Podcast</NavLink>
<NavLink to='/user/details' className={'py-2 px-2 rounded-lg hover:bg-stone-900 transition-all duration-300 text-[16px] font-medium'}>Profile</NavLink>
</div>
</div>
<Outlet />
<Footer />
</>
)
}
export default UserNav |
import { render, screen } from '@testing-library/react';
import { Input } from './';
describe('<Input />', () => {
it('should render Input', () => {
render(<Input placeholder="placeholder" />);
expect(screen.getByRole('textbox')).toBeInTheDocument();
});
it('should render Input with placeholder', () => {
const placeholderText = 'Digite seu email';
render(<Input placeholder={placeholderText} />);
expect(screen.getByPlaceholderText(placeholderText)).toBeInTheDocument();
});
it('should render Input with error', () => {
render(<Input placeholder="error" error />);
const inputErrorClasses =
'border-error text-error placeholder-error placeholder-opacity-50';
expect(screen.getByPlaceholderText('error')).toHaveClass(inputErrorClasses);
});
it('should render Input with helperText', () => {
render(<Input placeholder="helperText" helperText="helper text" />);
expect(screen.getByText('helper text')).toBeInTheDocument();
});
it('should render Input disabled', () => {
render(<Input placeholder="disabled" disabled />);
const input = screen.getByPlaceholderText('disabled');
input.focus();
expect(input).not.toHaveFocus();
});
it('should render Input full Width', () => {
render(<Input placeholder="full width" fullWidth />);
const input = screen.getByPlaceholderText('full width');
const fullWidthStyle = 'flex w-full';
expect(input).toHaveClass(fullWidthStyle);
});
}); |
package io.js.restclient;
import io.js.models.User;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestClient;
import java.util.List;
import static org.springframework.http.MediaType.APPLICATION_JSON;
@Service
public class JsonPlaceHolderApiClient {
private final RestClient restClient;
public JsonPlaceHolderApiClient(RestClient restClient) {
this.restClient = restClient;
}
public List<User> getAllUsers() {
return restClient.get()
.uri("/users")
.retrieve()
.body(new ParameterizedTypeReference<>() {});
}
public User getUserById(int id) {
return restClient.get()
.uri("/users/{id}", id)
.accept(APPLICATION_JSON)
.retrieve()
.body(User.class);
}
User createUser(User user) {
return restClient.post()
.uri("/users")
.contentType(APPLICATION_JSON)
.body(user)
.retrieve()
.body(User.class);
}
void deleteUser(Integer id) {
restClient.delete()
.uri("/users/{id}", id)
.retrieve()
.toBodilessEntity();
}
} |
package com.ruoyi.project.parking.service.impl;
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.ruoyi.common.exception.ServiceException;
import com.ruoyi.common.utils.SecurityUtils;
import com.ruoyi.common.utils.StringUtils;
import com.ruoyi.common.utils.bean.BeanUtils;
import com.ruoyi.project.parking.domain.BlackList;
import com.ruoyi.project.parking.domain.bo.BlackListBO;
import com.ruoyi.project.parking.domain.param.BlackListParam;
import com.ruoyi.project.parking.domain.vo.BlackListVO;
import com.ruoyi.project.parking.service.IBlackListService;
import com.ruoyi.project.parking.mapper.BlackListMapper;
import org.springframework.stereotype.Service;
import java.time.LocalDateTime;
import java.util.List;
/**
* @author 琴声何来
* @description 针对表【t_black_list(黑名单表)】的数据库操作Service实现
* @since 2023-02-27 17:21:11
*/
@Service
public class BlackListServiceImpl extends ServiceImpl<BlackListMapper, BlackList>
implements IBlackListService {
/**
* @apiNote 获取黑名单列表
*/
@Override
public List<BlackList> listByParkNoAndCarNumber(BlackList blackList) {
LambdaQueryWrapper<BlackList> qw = new LambdaQueryWrapper<>();
qw.eq(StringUtils.isNotEmpty(SecurityUtils.getParkNo()), BlackList::getParkNo, SecurityUtils.getParkNo())
.like(StringUtils.isNotEmpty(blackList.getCarNumber()), BlackList::getCarNumber, blackList.getCarNumber());
return list(qw);
}
/**
* @apiNote 获取黑名单列表
*/
@Override
public List<BlackList> listByParkNoAndCarNumberUnsafe(BlackList blackList) {
LambdaQueryWrapper<BlackList> qw = new LambdaQueryWrapper<>();
qw.eq(StringUtils.isNotEmpty(blackList.getParkNo()), BlackList::getParkNo, blackList.getParkNo())
.eq(StringUtils.isNotEmpty(blackList.getCarNumber()), BlackList::getCarNumber, blackList.getCarNumber());
return list(qw);
}
/**
* @apiNote 新增单个黑名单
*/
@Override
public boolean add(BlackListVO blackListVO) {
BlackList blackList = new BlackList();
BeanUtils.copyBeanProp(blackList, blackListVO);
blackList.setParkNo(SecurityUtils.getParkNo());
blackList.setCreateBy(SecurityUtils.getUsername());
blackList.setCreateTime(LocalDateTime.now());
return save(blackList);
}
/**
* @apiNote 编辑单个黑名单
*/
@Override
public boolean editById(BlackListParam blackListParam) {
BlackList blackList = new BlackList();
BeanUtils.copyBeanProp(blackList, blackListParam);
blackList.setUpdateBy(SecurityUtils.getUsername());
blackList.setUpdateTime(LocalDateTime.now());
return updateById(blackList);
}
/**
* @apiNote 黑名单列表导入
*/
@Override
public String importBlackList(List<BlackListBO> blackListBOList, boolean updateSupport) {
if (StringUtils.isNull(blackListBOList) || blackListBOList.size() == 0) {
throw new ServiceException("导入数据不能为空!");
}
int successNum = 0;
int failureNum = 0;
StringBuilder successMsg = new StringBuilder();
StringBuilder failureMsg = new StringBuilder();
for (BlackListBO blackListBO : blackListBOList) {
if (StringUtils.isEmpty(blackListBO.getCarNumber())) {
failureMsg.append("<br/>").append(failureNum).append("、黑名单车牌号为空");
continue;
}
LambdaQueryWrapper<BlackList> qw = new LambdaQueryWrapper<>();
// 验证是否存在这个固定车
BlackList blackList = getOne(qw.eq(BlackList::getCarNumber, blackListBO.getCarNumber())
.eq(StringUtils.isNotEmpty(SecurityUtils.getParkNo()), BlackList::getParkNo, SecurityUtils.getParkNo()));
try {
if (StringUtils.isNull(blackList)) {
// 新增黑名单
BlackList addBlackList = new BlackList();
BeanUtils.copyBeanProp(addBlackList, blackListBO);
addBlackList.setParkNo(SecurityUtils.getParkNo());
addBlackList.setCreateBy(SecurityUtils.getUsername());
addBlackList.setCreateTime(LocalDateTime.now());
save(addBlackList);
successNum++;
successMsg.append("<br/>").append(successNum).append("、黑名单 ").append(blackListBO.getCarNumber()).append(" 导入成功");
} else if (updateSupport) {
// 编辑黑名单
BlackList updateBlackList = new BlackList();
BeanUtils.copyBeanProp(updateBlackList, blackListBO);
updateBlackList.setId(blackList.getId());
updateBlackList.setUpdateBy(SecurityUtils.getUsername());
updateBlackList.setUpdateTime(LocalDateTime.now());
updateById(updateBlackList);
successNum++;
successMsg.append("<br/>").append(successNum).append("、黑名单 ").append(blackListBO.getCarNumber()).append(" 更新成功");
} else {
failureNum++;
failureMsg.append("<br/>").append(failureNum).append("、黑名单 ").append(blackListBO.getCarNumber()).append(" 已存在");
}
} catch (Exception e) {
failureNum++;
String msg = "<br/>" + failureNum + "、黑名单 " + blackListBO.getCarNumber() + " 导入失败:";
failureMsg.append(msg).append(e.getMessage());
log.error(msg, e);
}
}
if (failureNum > 0) {
failureMsg.insert(0, "很抱歉,导入失败!共 " + failureNum + " 条数据格式不正确,错误如下:");
throw new ServiceException(failureMsg.toString());
} else {
successMsg.insert(0, "恭喜您,数据已全部导入成功!共 " + successNum + " 条,数据如下:");
}
return successMsg.toString();
}
} |
#pragma once
#include <string>
#include <imgui.h>
namespace JEditor {
// NOLINTNEXTLINE(hicpp-special-member-functions, cppcoreguidelines-special-member-functions)
class IPanel
{
public:
explicit IPanel(const std::string_view& name) : m_Name(name) {}
virtual ~IPanel() = default;
void Render()
{
if (ImGui::Begin(Name().c_str(), &m_Open)) { OnImGuiRender(); }
ImGui::End();
}
[[nodiscard]] inline auto Name() const -> const std::string& { return m_Name; }
inline void Open() { m_Open = true; };
inline void Close() { m_Open = false; };
[[nodiscard]] inline auto IsOpen() const -> bool { return m_Open; }
private:
virtual void OnImGuiRender() = 0;
const std::string m_Name;
bool m_Open = true;
};
}// namespace JEditor |
<!DOCTYPE html>
<html lang="en"
xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:f="http://xmlns.jcp.org/jsf/core">
<h:head>
<meta charset="UTF-8" />
<title>Add Head of State</title>
<f:event type="preRenderView" listener="#{headOfStateController.loadCountries}" />
</h:head>
<h:body>
<h:messages globalOnly="true" styleClass="error"/>
<h:form>
<h2>Add Head of State</h2>
<section>
<fieldset>
<p>
<label for="countryCode">
<span>Country Code: </span>
</label>
<!-- Displaying a list of existing countries in a drop-down box, so there are no invalid country codes when adding heads of state -->
<h:selectOneMenu id="countryCodes" value="#{headOfStateController.headOfState._id}" >
<f:selectItems value="#{headOfStateController.countries}" var="country" itemValue="#{country.code}" itemLabel="#{country.name}" />
</h:selectOneMenu>
</p>
<p>
<label for="headOfStateName">
<span>Head of State: </span>
</label>
<h:inputText id="headOfStateName" value="#{headOfStateController.headOfState.headOfState}" required="true" />
<h:messages for="headOfStateName" styleClass="error"/>
</p>
</fieldset>
</section>
<section>
<p>
<h:commandButton value="Add" action="#{headOfStateController.add(headOfStateController.headOfState)}" />
</p>
</section>
</h:form>
<h:link value="Home" outcome="index"/> <h:link value="Heads of State" outcome="list_heads_of_state"/>
</h:body>
</html> |
import React from "react";
import { shallow } from "enzyme";
import Dialog from "./Dialog";
import type { DialogProps } from "./Dialog.props";
import { Text } from "../../../Text";
const COMPONENT_NAME = "Modal";
describe("Dialog", () => {
const defaultProps: DialogProps = {
children: <Text>Test Content</Text>,
size: "medium",
height: 300,
};
it("renders without crashing", () => {
shallow(<Dialog {...defaultProps} />);
});
it("renders the correct class name", () => {
const wrapper = shallow(<Dialog {...defaultProps} />);
expect(wrapper.hasClass(`${COMPONENT_NAME}--medium`)).toBeTruthy();
expect(wrapper.hasClass("Modal")).toBeTruthy();
});
it("sets the height style when height prop is provided", () => {
const wrapper = shallow(<Dialog {...defaultProps} />);
expect(wrapper.get(0).props.style).toHaveProperty(
"--modal-dialog-height",
"300px"
);
});
it("does not set the height style when height prop is not provided", () => {
const propsWithoutHeight = { ...defaultProps, height: undefined };
const wrapper = shallow(<Dialog {...propsWithoutHeight} />);
expect(wrapper.get(0).props.style).toBeUndefined();
});
it("renders the children prop", () => {
const wrapper = shallow(<Dialog {...defaultProps} />);
expect(wrapper.find(Text)).toHaveLength(1);
});
}); |
import styled from 'styled-components';
interface styledProps {
$error: boolean;
}
export const MintFormStyle = styled.form`
display: flex;
max-width: 248px;
flex-direction: column;
align-items: center;
gap: 16px;
margin: 0 auto;
@media screen and (min-width: 1280px) {
max-width: 397px;
gap: 24px;
}
`;
export const MintFormLabelStyle = styled.label`
position: relative;
display: flex;
width: 100%;
align-items: center;
border-radius: 8px;
background: #1e1e1e;
.icon {
display: flex;
align-items: center;
justify-content: center;
padding: 12px;
}
@media screen and (min-width: 1280px) {
.icon {
padding: 20px;
}
}
`;
export const FieldStyle = styled.input<styledProps>`
width: 100%;
padding: 17px 24px;
border-radius: 0 8px 8px 0;
outline: none;
border: 1px solid transparent;
/* background-color: #181818; */
background-color: #181818;
background-clip: padding-box;
font-family: 'Messina Sans Mono';
font-size: 12px;
line-height: 1.16;
color: var(--color-dark-theme);
transition: border var(--transition);
&::placeholder {
font-family: 'Messina Sans Mono';
color: #ffffff3d;
text-transform: uppercase;
}
&:focus {
border: 1px solid;
border-color: ${props => (props.$error ? '#DC3B5A' : '#ffffff')};
}
border-color: ${props => (props.$error ? '#DC3B5A' : 'transparent')};
@media screen and (min-width: 1280px) {
font-size: 16px;
line-height: 1.9;
}
`;
export const ErrorFieldTextStyle = styled.p`
position: absolute;
bottom: -2px;
right: 0;
transform: translateY(100%);
font-family: 'Messina Sans Mono';
font-size: 10px;
line-height: 1.2;
color: var(--color-secondary);
text-transform: uppercase;
@media screen and (min-width: 1280px) {
font-size: 12px;
line-height: 1.2;
}
`;
export const MintFormSubmitBtnStyle = styled.button`
display: block;
width: 100%;
padding-top: 10px;
padding-bottom: 12px;
border-color: transparent;
border-radius: 8px;
background: #dc3b5a;
font-family: 'Right Grotesk';
font-size: 16px;
font-weight: 700;
line-height: 1.19;
color: var(--color-dark-theme);
text-transform: uppercase;
transition: color var(--transition);
&:hover {
color: var(--color-light-theme);
}
@media screen and (min-width: 1280px) {
padding-top: 16px;
padding-bottom: 20px;
font-size: 28px;
line-height: 1.2;
}
`; |
# CASH-MINT
A lightweight and safe all-in-one docker-composer for earning passive income from your unused internet
bandwidth.
A single instance of **cash-mint** can earn up to **50$** a month or even more,
this estimation is depending on your IP location.
## Compatibility
I'm creating **cash-mint** while learning [Jenkins](https://www.jenkins.io), [Systemd](https://systemd.io/),
and [Ubuntu OS](https://ubuntu.com/) architecture, so I never designed or testing **cash-mint** on another operating
system.
Here is the current supported operating system:
```shell
Description: Ubuntu 23.10
Codename: mantic
```
## Usage
To use **cash-mint**, you can run the following commands:
- Clone **cash-mint** repository
```shell
git clone https://github.com/bearaujus/cash-mint.git cash-mint
```
- Move to **cash-mint** directory
```shell
cd cash-mint
```
- Then, you need to create a copy of `.env.example` as `.env`
```shell
cp .env.example .env
```
- Fill all `<edit-me>` at recently created `.env`.
See: [How to set up env](https://github.com/bearaujus/cash-mint/blob/master/how-to/setup-env.md)
for more detailed guides.
```shell
# you can use your favorite text editor to edit .env
nano .env
```
- _(Optional)_ If you haven't installed [Docker](https://www.docker.com/)
or [Makefile](https://www.gnu.org/software/make/manual/make.html) yet:
```shell
# install docker
sudo apt update
curl -fsSL https://raw.githubusercontent.com/docker/docker-install/master/install.sh -o install-docker.sh
chmod +x install-docker.sh
sudo ./install-docker.sh
rm install-docker.sh
```
```shell
# install makefile
sudo apt update
sudo apt install make
```
- Finally, you can run the **cash-mint**. To quit, just simply press `CTRL+C`.
```shell
make run
```
- _(Optional)_ if you want to run **cash-mint** in the background:
```shell
# start the cash-mint in the background
make start
```
```shell
# see background cash-mint logs
make status
```
```shell
# stop background cash-mint
make stop
```
***Notes**: By running this project, it means you already agreed to all [list supported sites](#list-supported-sites)
agreements.
## List Supported Sites
I'm **not using** all _cash-able_ sites containers since I need to check whether the **container is safe to run or
not**. For example, like this one: [fazalfarhan01/EarnApp-Docker](https://github.com/fazalfarhan01/EarnApp-Docker) this
container maybe has scary thing running in it [issues/46](https://github.com/fazalfarhan01/EarnApp-Docker/issues/46).
I already selected carefully with the containers that I decided to use for **cash-mint**. Also, I already scan it with
antivirus called [ClamAV](https://www.clamav.net/) to ensure **cash-mint** is safe to run! ;)
Below are the list for sites that supported by **cash-mint**:
1. [BITPING](https://bitping.com)
2. [EARNFM](https://earn.fm/ref/BEARFZQR) _(referral link)_
3. [HONEYGAIN](https://r.honeygain.me/HARYO1972B) _(referral link)_
4. [MYSTERIUM](https://mystnodes.co/?referral_code=qGKgwIJSEpxvOKhZUb1BDsa1xSdPCLjTAWaacX25) _(referral link)_
5. [PACKETSTREAM](https://packetstream.io/?psr=1RV8) _(referral link)_
6. [PAWNS](https://pawns.app/?r=3284830) _(referral link)_
7. [PROXYLITE](https://proxylite.ru/?r=8Q4XCYWF) _(referral link)_
8. [PROXYRACK](https://peer.proxyrack.com/ref/phzcnf5r7nq1idtx4rhgglt6ayrtvqgkb8nwhkic) _(referral link)_
9. [REPOCKET](https://link.repocket.co/WqgJ) _(referral link)_
10. [TRAFFMONETIZER](https://traffmonetizer.com/?aff=1612556) _(referral link)_
## Notes On Your First Run
- If you don't want to use some **cash-mint** service(s), you can comment the service on `docker-compose.yml`.
- Service: [MYSTERIUM](https://mystnodes.co/?referral_code=qGKgwIJSEpxvOKhZUb1BDsa1xSdPCLjTAWaacX25) _(referral link)_
To start earning with MYSTERIUM, you need to register your local MYSTERIUM node.
- Go to http://localhost:_<MYSTERIUM_DASHBOARD_PORT>_. (See: Your recently created .env)
- Finish the node onboard.
- Go to Settings > Account.
- And you will see small box at the top right called `Mystnodes.com Integrations`.
- Click on the `Claim on mystnodes.com` button.
Now your node should be appeared on MYSTERIUM dashboard.
- Service: [PROXYRACK](https://peer.proxyrack.com/ref/phzcnf5r7nq1idtx4rhgglt6ayrtvqgkb8nwhkic) _(referral link)_
To start earning with PROXYRACK, you need to register your local PROXYRACK node.
- Go to [PROXYRACK](https://peer.proxyrack.com/ref/phzcnf5r7nq1idtx4rhgglt6ayrtvqgkb8nwhkic) _(referral link)_.
- Go to Dashboard.
- Add your node at `Add new device` section.
- You can see your PROXYRACK device ID through `.env`. (See: Your recently created .env)
## TODO
- Support cross-platform.
- Support adaptive working directory.
- Fix bugs when BITPING credentials invalid, it keeps restarting until the CPU is soooo hot. _(this is probably caused
by refresh
rate from interactive terminal BITPING is too high)_
- Test all services containers if credentials are invalid. _(because I never tried supplied invalid credentials to all
services at once)_
## License
This project is licensed under the GNU License - see
the [LICENSE](https://github.com/bearaujus/cash-mint/blob/master/LICENSE) file for details.
---
Made with ❤️ from [bearaujus](https://www.linkedin.com/in/bearaujus/) @2024 |
package com.example.hammer_test.ui.fragments
import android.annotation.SuppressLint
import android.content.Context
import android.os.Bundle
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.ArrayAdapter
import androidx.fragment.app.Fragment
import androidx.lifecycle.ViewModelProvider
import androidx.recyclerview.widget.LinearLayoutManager
import androidx.recyclerview.widget.RecyclerView
import com.example.hammer_test.R
import com.example.hammer_test.databinding.FragmentCartBinding
import com.example.hammer_test.di.AppComponent
import com.example.hammer_test.ui.adapters.CartListAdapter
import com.example.hammer_test.ui.viewmodels.CartViewModel
import javax.inject.Inject
import kotlin.properties.Delegates
class CartFragment:Fragment(R.layout.fragment_cart) {
@Inject
lateinit var viewModelFactory: ViewModelProvider.Factory
private var binding: FragmentCartBinding by Delegates.notNull()
private var viewModel : CartViewModel by Delegates.notNull()
var num = 1
var totalPrice = 0.0
override fun onAttach(context: Context) {
super.onAttach(context)
AppComponent.get().inject(this)
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
}
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
binding = FragmentCartBinding.inflate(inflater)
return binding.root
}
@SuppressLint("NotifyDataSetChanged")
override fun onViewCreated(view: View, savedInstanceState: Bundle?) {
super.onViewCreated(view, savedInstanceState)
viewModel = ViewModelProvider(this,viewModelFactory)[CartViewModel::class.java]
val cartRV = view.findViewById<RecyclerView>(R.id.cart_rv)
cartRV.apply {
layoutManager = LinearLayoutManager(
requireContext(), LinearLayoutManager.VERTICAL,false
)
}
val cartAdapter = CartListAdapter(layoutInflater,
onMinusClicked = {
// viewModel.deleteCartItem(item = it)
if(it.itemCount>1){
it.itemCount = it.itemCount - 1
}else{
viewModel.deleteCartItem(item = it)
}
},
onAddClicked = {
viewModel.insertCartItem(item = it)
it.itemCount = it.itemCount + 1
},
)
cartAdapter.notifyDataSetChanged()
cartRV.adapter = cartAdapter
viewModel.itemsLiveData.observe(viewLifecycleOwner){
cartAdapter.setItems(it)
for (item in it.indices){
totalPrice += it[item].price * it[item].itemCount.toDouble()
}
binding.buyBtn.text = "Оплатить" + " "+ totalPrice.toString() + "₽"
}
}
} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<!-- Bootstrap -->
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-1BmE4kWBq78iYhFldvKuhfTAU6auU8tT94WrHftjDbrCEXSU1oBoqyl2QvZ6jIW3" crossorigin="anonymous">
</head>
<body>
<!-- Navbar -->
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<div class="container-fluid">
<a class="navbar-brand" href="#">Skilvul</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarSkilvul" aria-controls="navbarSkilvul" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSkilvul">
<ul class="navbar-nav me-auto mb-2 mb-lg-0">
<li class="nav-item">
<a class="nav-link" href="#">Products</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Category</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Merchants</a>
</li>
</ul>
<form class="d-flex">
<input class="form-control me-2" type="search" placeholder="Search" aria-label="Search">
<button class="btn btn-outline-success" type="submit">Search</button>
</form>
</div>
</div>
</nav>
<!-- carousel -->
<div id="carouselExampleIndicators" class="carousel slide d-lg-block d-md-none d-none" data-bs-ride="carousel">
<div class="carousel-indicators">
<button type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide-to="0" class="active" aria-current="true" aria-label="Slide 1"></button>
<button type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide-to="1" aria-label="Slide 2"></button>
<button type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide-to="2" aria-label="Slide 3"></button>
<button type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide-to="3" aria-label="Slide 4"></button>
</div>
<div class="carousel-inner">
<div class="carousel-item active">
<img src="https://images.unsplash.com/photo-1593543294918-ca3634e04cdb?crop=entropy&cs=tinysrgb&fit=crop&fm=jpg&h=450&ixid=MnwxfDB8MXxyYW5kb218MHx8Y29mZmVlfHx8fHx8MTYzMzkzOTAzNw&ixlib=rb-1.2.1&q=80&utm_campaign=api-credit&utm_medium=referral&utm_source=unsplash_source&w=1000"
alt="skilvul" class="d-block w-100 img-carousel">
</div>
<div class="carousel-item">
<img src="https://images.unsplash.com/photo-1517668808822-9ebb02f2a0e6?crop=entropy&cs=tinysrgb&fit=crop&fm=jpg&h=450&ixid=MnwxfDB8MXxyYW5kb218MHx8Y29mZmVlfHx8fHx8MTYzMzkzOTI1NA&ixlib=rb-1.2.1&q=80&utm_campaign=api-credit&utm_medium=referral&utm_source=unsplash_source&w=1000"
alt="skilvul" class="d-block w-100 img-carousel">
</div>
<div class="carousel-item">
<img src="https://images.unsplash.com/photo-1504627298434-2119d6928e93?crop=entropy&cs=tinysrgb&fit=crop&fm=jpg&h=450&ixid=MnwxfDB8MXxyYW5kb218MHx8Y29mZmVlfHx8fHx8MTYzMzkzOTEwMg&ixlib=rb-1.2.1&q=80&utm_campaign=api-credit&utm_medium=referral&utm_source=unsplash_source&w=1000"
alt="skilvul" class="d-block w-100 img-carousel">
</div>
<div class="carousel-item">
<img src="https://images.unsplash.com/photo-1567357502214-dd13f3512e71?crop=entropy&cs=tinysrgb&fit=crop&fm=jpg&h=450&ixid=MnwxfDB8MXxyYW5kb218MHx8YWZmb2dhdG98fHx8fHwxNjMzOTM5NTQ0&ixlib=rb-1.2.1&q=80&utm_campaign=api-credit&utm_medium=referral&utm_source=unsplash_source&w=1000"
alt="skilvul" class="d-block w-100 img-carousel">
</div>
</div>
<button class="carousel-control-prev" type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="visually-hidden">Previous</span>
</button>
<button class="carousel-control-next" type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="visually-hidden">Next</span>
</button>
</div>
<!-- content card -->
<div class="container">
<div class="row mt-5 ">
<div class="col-lg-9 col-sm-12">
<div class="text-center text-lg-start mb-5">
<h3>Menus</h3>
</div>
<div class="row">
<div class="col-6 col-lg-12 mb-3">
<div class="card">
<div class="row">
<div class="col-lg-4">
<img src="https://images.unsplash.com/photo-1593543294918-ca3634e04cdb?crop=entropy&cs=tinysrgb&fit=crop&fm=jpg&h=450&ixid=MnwxfDB8MXxyYW5kb218MHx8Y29mZmVlfHx8fHx8MTYzMzkzOTAzNw&ixlib=rb-1.2.1&q=80&utm_campaign=api-credit&utm_medium=referral&utm_source=unsplash_source&w=700"
class="img-fluid" alt="skilvul">
</div>
<div class="col-lg-8">
<div class="card-body">
<h5 class="card-title">Card title</h5>
<p class="card-text">This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.</p>
<p class="card-text"><small class="text-muted">Last updated 3 mins ago</small></p>
</div>
</div>
</div>
</div>
</div>
<div class="col-6 col-lg-12 mb-3">
<div class="card">
<div class="row ">
<div class="col-lg-4">
<img src="https://images.unsplash.com/photo-1593543294918-ca3634e04cdb?crop=entropy&cs=tinysrgb&fit=crop&fm=jpg&h=450&ixid=MnwxfDB8MXxyYW5kb218MHx8Y29mZmVlfHx8fHx8MTYzMzkzOTAzNw&ixlib=rb-1.2.1&q=80&utm_campaign=api-credit&utm_medium=referral&utm_source=unsplash_source&w=700"
class="img-fluid" alt="skilvul">
</div>
<div class="col-lg-8">
<div class="card-body">
<h5 class="card-title">Card title</h5>
<p class="card-text">This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.</p>
<p class="card-text"><small class="text-muted">Last updated 3 mins ago</small></p>
</div>
</div>
</div>
</div>
</div>
<div class="col-6 col-lg-12 mb-3">
<div class="card">
<div class="row ">
<div class="col-lg-4">
<img src="https://images.unsplash.com/photo-1593543294918-ca3634e04cdb?crop=entropy&cs=tinysrgb&fit=crop&fm=jpg&h=450&ixid=MnwxfDB8MXxyYW5kb218MHx8Y29mZmVlfHx8fHx8MTYzMzkzOTAzNw&ixlib=rb-1.2.1&q=80&utm_campaign=api-credit&utm_medium=referral&utm_source=unsplash_source&w=700"
class="img-fluid" alt="skilvul">
</div>
<div class="col-lg-8">
<div class="card-body">
<h5 class="card-title">Card title</h5>
<p class="card-text">This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.</p>
<p class="card-text"><small class="text-muted">Last updated 3 mins ago</small></p>
</div>
</div>
</div>
</div>
</div>
<div class="col-6 col-lg-12 mb-3">
<div class="card">
<div class="row ">
<div class="col-lg-4">
<img src="https://images.unsplash.com/photo-1593543294918-ca3634e04cdb?crop=entropy&cs=tinysrgb&fit=crop&fm=jpg&h=450&ixid=MnwxfDB8MXxyYW5kb218MHx8Y29mZmVlfHx8fHx8MTYzMzkzOTAzNw&ixlib=rb-1.2.1&q=80&utm_campaign=api-credit&utm_medium=referral&utm_source=unsplash_source&w=700"
class="img-fluid" alt="skilvul">
</div>
<div class="col-lg-8">
<div class="card-body">
<h5 class="card-title">Card title</h5>
<p class="card-text">This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.</p>
<p class="card-text"><small class="text-muted">Last updated 3 mins ago</small></p>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- List Group -->
<div class="col-lg-3 d-lg-block d-md-none d-none">
<ul class="list-group list-group-flush">
<li class="list-group-item">An item</li>
<li class="list-group-item">A second item</li>
<li class="list-group-item">A third item</li>
<li class="list-group-item">A fourth item</li>
<li class="list-group-item">And a fifth one</li>
</ul>
</div>
</div>
</div>
<!-- Bootstrap bundles -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js" integrity="sha384-ka7Sk0Gln4gmtz2MlQnikT1wXgYsOg+OMhuP+IlRH9sENBO0LRn5q+8nbTov4+1p" crossorigin="anonymous"></script>
</body>
</html> |
<?php
namespace Code;
use PHPUnit\Framework\TestCase;
class ProdutoTest extends TestCase
{
private $produto;
// Roda antes de cada teste da classe
public function setUp(): void
{
$this->produto = new Produto();
}
// // Chamado uma vez, antes dos testes serem executados, no caso pode ser usado para acessar o banco pois executa só uma vez
// public static function setUpBeforeClass(): void
// {
// print __METHOD__;
// }
// // Chamado uma vez, depois dos testes serem executados, no caso pode ser usado para acessar o banco pois executa só uma vez
// public static function tearDownAfterClass(): void
// {
// print __METHOD__;
// }
public function testSeONomeDoProdutoESetadoCorretamente()
{
$produto = $this->produto;
$produto->setName('Produto 1');
$this->assertEquals('Produto 1', $produto->getName(), 'Valores não são iguais');
}
public function testSeOPrecoDoProdutoESetadoCorretamente()
{
$produto = $this->produto;
$produto->setPrice('19.99');
$this->assertEquals('19.99', $produto->getPrice(), 'Valores não são iguais');
}
public function testSeOSlugDoProdutoESetadoCorretamente()
{
$produto = $this->produto;
$produto->setSlug('produto-1');
$this->assertEquals('produto-1', $produto->getSlug(), 'Valores não são iguais');
}
/*
* @expectedException \InvalidArgumentException
* @expectedExceptionMessage Parâmetro inválido, informe um slug
*/
public function testSeSetSlugLancaExceptionQuandoNaoInformado()
{
// Essas exception tem que ficar acima da chamada de setSlug
$this->expectException('\InvalidArgumentException');
$this->expectExceptionMessage('Parâmetro inválido, informe um slug');
$product = $this->produto;
$product->setSlug('');
}
} |
package net.danvi.dmall.admin.web.view.goods.controller;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import dmall.framework.common.constants.ExceptionConstants;
import dmall.framework.common.util.MessageUtil;
import net.danvi.dmall.biz.system.security.DmallSessionDetails;
import org.springframework.security.authentication.BadCredentialsException;
import org.springframework.stereotype.Controller;
import org.springframework.validation.BindingResult;
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.servlet.ModelAndView;
import dmall.framework.common.exception.JsonValidationException;
import dmall.framework.common.model.ResultListModel;
import dmall.framework.common.model.ResultModel;
import lombok.extern.slf4j.Slf4j;
import net.danvi.dmall.biz.app.goods.model.BrandVO;
import net.danvi.dmall.biz.app.goods.model.CategoryDisplayManagePO;
import net.danvi.dmall.biz.app.goods.model.CategoryDisplayManageSO;
import net.danvi.dmall.biz.app.goods.model.CategoryDisplayManageVO;
import net.danvi.dmall.biz.app.goods.model.CategoryPO;
import net.danvi.dmall.biz.app.goods.model.CategorySO;
import net.danvi.dmall.biz.app.goods.model.CategoryVO;
import net.danvi.dmall.biz.app.goods.model.GoodsVO;
import net.danvi.dmall.biz.app.goods.service.CategoryManageService;
import net.danvi.dmall.biz.app.goods.service.GoodsManageService;
import net.danvi.dmall.biz.common.service.BizService;
import net.danvi.dmall.biz.system.security.SessionDetailHelper;
import net.danvi.dmall.biz.system.validation.DeleteGroup;
import net.danvi.dmall.biz.system.validation.UpdateGroup;
/**
* <pre>
* 프로젝트명 : 41.admin.web
* 작성일 : 2016. 6. 22.
* 작성자 : dong
* 설명 : 상품 카테고리 정보 관리 컴포넌트의 컨트롤러 클래스
* </pre>
*/
@Slf4j
@Controller
@RequestMapping("/admin/goods")
public class CategoryManageController {
@Resource(name = "categoryManageService")
private CategoryManageService categoryManageService;
@Resource(name = "bizService")
private BizService bizService;
@Resource(name = "goodsManageService")
private GoodsManageService goodsManageService;
/**
* <pre>
* 작성일 : 2016. 6. 22.
* 작성자 : dong
* 설명 : 카테고리 관리 화면을 반환
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2016. 6. 22. dong - 최초생성
* </pre>
*
* @param
* @return
*/
@RequestMapping("/category-info")
public ModelAndView viewCategoryInfo() {
ModelAndView mv = new ModelAndView("/admin/goods/CategoryManage");
DmallSessionDetails sessionInfo = SessionDetailHelper.getDetails();
if (sessionInfo == null) {
throw new BadCredentialsException(MessageUtil.getMessage(ExceptionConstants.BIZ_EXCEPTION_LNG + ExceptionConstants.ERROR_CODE_LOGIN_SESSION));
}
mv.addObject("siteNo", sessionInfo.getSiteNo());
return mv;
}
/**
* <pre>
* 작성일 : 2016. 6. 22.
* 작성자 : dong
* 설명 : 카테고리 리스트 조회(트리목록)
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2016. 6. 22. dong - 최초생성
* </pre>
*
* @param CategorySO
* @param
* @return
*/
@RequestMapping("/category-list")
public @ResponseBody List<CategoryVO> selectCategoryList(CategorySO so, BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
throw new JsonValidationException(bindingResult);
}
List<CategoryVO> result = categoryManageService.selectCategoryList(so);
return result;
}
/**
* <pre>
* 작성일 : 2018. 12. 10.
* 작성자 : hskim
* 설명 : 카테고리 리스트 조회(배너관리 1depth 카테고리 조회용)
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2018. 12. 10. hskim - 최초생성
* </pre>
*
* @param CategorySO
* @param
* @return
*/
@RequestMapping("/category-list-1depth")
public @ResponseBody ResultListModel<CategoryVO> selectCategoryList1depth(CategorySO so, BindingResult bindingResult) {
ResultListModel<CategoryVO> result = new ResultListModel<>();
List<CategoryVO> list = categoryManageService.selectCategoryList1depth(so);
result.setResultList(list);
result.setSuccess(list.size() > 0 ? true : false);
return result;
}
/**
* <pre>
* 작성일 : 2016. 6. 22.
* 작성자 : dong
* 설명 : 카테고리에 상품, 쿠폰 존재 여부 확인
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2016. 6. 22. dong - 최초생성
* </pre>
*
* @param CategorySO
* @return
*/
@RequestMapping("/category-goods-coupon")
public @ResponseBody Map<String, Integer> selectCtgGoodsCpCnt(CategorySO so, BindingResult bindingResult)
throws Exception {
// 사이트 번호
so.setSiteNo(SessionDetailHelper.getDetails().getSiteNo());
if (bindingResult.hasErrors()) {
log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
throw new JsonValidationException(bindingResult);
}
CategoryPO po = new CategoryPO();
po.setCtgNo(so.getCtgNo());
po.setSiteNo(SessionDetailHelper.getDetails().getSiteNo());
List<Integer> ctgNoList = categoryManageService.selectChildCtgNo(po);
po.setChildCtgNoList(ctgNoList);
Integer cpCnt = categoryManageService.selectCpYn(po);
Integer ctgGoodsCnt = categoryManageService.selectCtgGoodsYn(po);
Map<String, Integer> childCtgCnt = new HashMap<String, Integer>();
childCtgCnt.put("ctgGoodsCnt", ctgGoodsCnt);
childCtgCnt.put("cpCnt", cpCnt);
return childCtgCnt;
}
/**
* <pre>
* 작성일 : 2016. 6. 21.
* 작성자 : dong
* 설명 : 카테고리 삭제
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2016. 6. 21. dong - 최초생성
* </pre>
*
* @param CategoryPO
* @return
* @throws Exception
*/
@RequestMapping("/category-delete")
public @ResponseBody ResultModel<CategoryPO> deleteCategory(@Validated(DeleteGroup.class) CategoryPO po,
BindingResult bindingResult) throws Exception {
// 삭제자 번호
po.setRegrNo(SessionDetailHelper.getDetails().getSession().getMemberNo());
// 사이트 번호
po.setSiteNo(SessionDetailHelper.getDetails().getSiteNo());
if (bindingResult.hasErrors()) {
log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
throw new JsonValidationException(bindingResult);
}
ResultModel<CategoryPO> result = categoryManageService.deleteCategory(po);
return result;
}
/**
* <pre>
* 작성일 : 2016. 6. 21.
* 작성자 : dong
* 설명 : 카테고리 정보 조회
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2016. 6. 21. dong - 최초생성
* </pre>
*
* @param CategorySO
* @return
* @throws Exception
*/
@RequestMapping("/category")
public @ResponseBody ResultModel<CategoryVO> selectCategory(CategorySO so, BindingResult bindingResult)
throws Exception {
if (bindingResult.hasErrors()) {
log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
throw new JsonValidationException(bindingResult);
}
// 사이트 번호
so.setSiteNo(SessionDetailHelper.getDetails().getSiteNo());
ResultModel<CategoryVO> result = categoryManageService.selectCategory(so);
return result;
}
/**
* <pre>
* 작성일 : 2016. 6. 21.
* 작성자 : dong
* 설명 : 카테고리 정보 수정
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2016. 6. 21. dong - 최초생성
* </pre>
*
* @param CategoryPO
* @return
* @throws Exception
*/
@RequestMapping("/category-update")
public @ResponseBody ResultModel<CategoryPO> updateCatagory(@Validated(UpdateGroup.class) CategoryPO po,
BindingResult bindingResult,
HttpServletRequest request) throws Exception {
if (bindingResult.hasErrors()) {
log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
throw new JsonValidationException(bindingResult);
}
// 등록자 번호
po.setRegrNo(SessionDetailHelper.getDetails().getSession().getMemberNo());
// 사이트 번호
po.setSiteNo(SessionDetailHelper.getDetails().getSiteNo());
ResultModel<CategoryPO> result = categoryManageService.updateCatagory(po, request);
return result;
}
/**
* <pre>
* 작성일 : 2016. 6. 30.
* 작성자 : dong
* 설명 : 카테고리 전시존 정보 수정
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2016. 6. 30. dong - 최초생성
* </pre>
*
* @param CategoryDisplayManagePO
* @return
* @throws Exception
*/
@RequestMapping("/category-display-update")
public @ResponseBody ResultModel<CategoryDisplayManagePO> updateCatagoryDisplayManage(CategoryDisplayManagePO po,
BindingResult bindingResult) throws Exception {
// po.setUseYnArray();
// 등록자 번호
po.setRegrNo(SessionDetailHelper.getDetails().getSession().getMemberNo());
// 사이트 번호
po.setSiteNo(SessionDetailHelper.getDetails().getSiteNo());
if (bindingResult.hasErrors()) {
log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
throw new JsonValidationException(bindingResult);
}
ResultModel<CategoryDisplayManagePO> result = categoryManageService.updateCatagoryDisplayManage(po);
return result;
}
/**
* <pre>
* 작성일 : 2016. 6. 28.
* 작성자 : dong
* 설명 : 카테고리 등록
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2016. 6. 28. dong - 최초생성
* </pre>
*
* @param CategoryPO
* @return
* @throws Exception
*/
@RequestMapping("/category-insert")
public @ResponseBody ResultModel<CategoryPO> insertCategory(CategoryPO po, BindingResult bindingResult)
throws Exception {
ResultModel<CategoryPO> result = new ResultModel<>();
// 등록자 번호
po.setRegrNo(SessionDetailHelper.getDetails().getSession().getMemberNo());
// 사이트 번호
po.setSiteNo(SessionDetailHelper.getDetails().getSiteNo());
if (bindingResult.hasErrors()) {
log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
throw new JsonValidationException(bindingResult);
}
String[] ctgNmArr = po.getInsCtgNm();
// 카테고리 진열 유형 코드 디폴트 값 set
if (po.getCtgExhbtionTypeCd() == null || po.getCtgExhbtionTypeCd() == "") {
po.setCtgExhbtionTypeCd("1");
}
// 카테고리 전시 유형 코드 디폴트 값 set
if (po.getCtgDispTypeCd() == null || po.getCtgDispTypeCd() == "") {
po.setCtgDispTypeCd("03");
}
// 모바일 쇼핑몰 적용 여부 디폴트 값 set
if (po.getMobileSpmallApplyYn() == null || po.getMobileSpmallApplyYn() == "") {
po.setMobileSpmallApplyYn("Y");
}
// 카테고리 메인 사용 여부 디폴트 값 set
if (po.getCtgMainUseYn() == null || po.getCtgMainUseYn() == "") {
po.setCtgMainUseYn("N");
}
for (int i = 0; i < ctgNmArr.length; i++) {
// po.setCtgSerialNo(bizService.getSequence("CTG_SERIAL_NO", po.getSiteNo()));
po.setCtgNm(ctgNmArr[i]);
if (po.getCtgNm() != null && po.getCtgNm() != "") {
result = categoryManageService.insertCategory(po);
}
}
return result;
}
/**
* <pre>
* 작성일 : 2016. 6. 29.
* 작성자 : dong
* 설명 : 카테고리 전시존 정보 조회
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2016. 6. 29. dong - 최초생성
* </pre>
*
* @param CategoryDisplayManageSO
* @return
* @throws Exception
*/
@RequestMapping("/category-displaymanage-list")
public @ResponseBody List<CategoryDisplayManageVO> selectCtgDispMngList(CategoryDisplayManageSO so,
BindingResult bindingResult) throws Exception {
// 사이트 번호
so.setSiteNo(SessionDetailHelper.getDetails().getSiteNo());
if (bindingResult.hasErrors()) {
log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
throw new JsonValidationException(bindingResult);
}
List<CategoryDisplayManageVO> result = categoryManageService.selectCtgDispMngList(so);
return result;
}
/**
* <pre>
* 작성일 : 2016. 7. 04.
* 작성자 : dong
* 설명 : 카테고리 전시존 상품 목록 조회
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2016. 7. 04. dong - 최초생성
* </pre>
*
* @param CategoryDisplayManageSO
* @return
* @throws Exception
*/
@RequestMapping("/category-display-goods")
public @ResponseBody List<GoodsVO> selectCtgDispGoodsList(CategoryDisplayManageSO so, BindingResult bindingResult)
throws Exception {
if (bindingResult.hasErrors()) {
log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
throw new JsonValidationException(bindingResult);
}
List<GoodsVO> result = categoryManageService.selectCtgDispGoodsList(so);
return result;
}
/**
* <pre>
* 작성일 : 2018. 9. 20.
* 작성자 : hskim
* 설명 : 카테고리 순서 변경
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2018. 9. 20. hskim - 최초생성
* </pre>
*
* @param CategoryPO
* @return
* @throws Exception
*/
@RequestMapping("/category-sort")
public @ResponseBody ResultModel<CategoryPO> updateCatagorySort(@Validated(UpdateGroup.class) CategoryPO po,
BindingResult bindingResult) throws Exception {
// 사이트 번호
po.setSiteNo(SessionDetailHelper.getDetails().getSiteNo());
if (bindingResult.hasErrors()) {
log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
throw new JsonValidationException(bindingResult);
}
ResultModel<CategoryPO> result = categoryManageService.updateCatagorySort(po);
return result;
}
/**
* <pre>
* 작성일 : 2016. 7. 07.
* 작성자 : dong
* 설명 : 카테고리 노출 상품 조회
*
* 수정내역(수정일 수정자 - 수정내용)
* -------------------------------------------------------------------------
* 2016. 7. 07. dong - 최초생성
* </pre>
*
* @param CategorySO
* @return
* @throws Exception
*/
// @RequestMapping("/category-rank-status")
// public @ResponseBody List<DisplayGoodsVO> selectCtgGoodsList(CategorySO so, BindingResult bindingResult)
// throws Exception {
//
// // 사이트 번호
// so.setSiteNo(SessionDetailHelper.getDetails().getSiteNo());
//
// if (bindingResult.hasErrors()) {
// log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
// throw new JsonValidationException(bindingResult);
// }
//
// List<DisplayGoodsVO> result = categoryManageService.selectCtgGoodsList(so);
//
// return result;
// }
//
// /**
// * <pre>
// * 작성일 : 2016. 7. 07.
// * 작성자 : dong
// * 설명 : 카테고리 노출 상품 관리 전시 여부 설정
// *
// * 수정내역(수정일 수정자 - 수정내용)
// * -------------------------------------------------------------------------
// * 2016. 7. 07. dong - 최초생성
// * </pre>
// *
// * @param CategoryPO
// * @return
// * @throws Exception
// */
// @RequestMapping("/goods-display-update")
// public @ResponseBody ResultModel<CategoryPO> updateCtgGoodsDispYn(CategoryPO po, BindingResult bindingResult)
// throws Exception {
//
// // 등록자 번호
// po.setRegrNo(SessionDetailHelper.getDetails().getSession().getMemberNo());
// // 사이트 번호
// po.setSiteNo(SessionDetailHelper.getDetails().getSiteNo());
//
// if (bindingResult.hasErrors()) {
// log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
// throw new JsonValidationException(bindingResult);
// }
//
// ResultModel<CategoryPO> result = categoryManageService.updateCtgGoodsDispYn(po);
//
// return result;
// }
//
// /**
// * <pre>
// * 작성일 : 2016. 7. 08.
// * 작성자 : dong
// * 설명 : 카테고리 노출 상품 관리 설정
// *
// * 수정내역(수정일 수정자 - 수정내용)
// * -------------------------------------------------------------------------
// * 2016. 7. 08. dong - 최초생성
// * </pre>
// *
// * @param CategoryPO
// * @return
// * @throws Exception
// */
// @RequestMapping("/goods-show-update")
// public @ResponseBody ResultModel<CategoryPO> updateCtgShowGoodsManage(CategoryPO po, BindingResult bindingResult)
// throws Exception {
//
// // 등록자 번호
// po.setRegrNo(SessionDetailHelper.getDetails().getSession().getMemberNo());
// // 사이트 번호
// po.setSiteNo(SessionDetailHelper.getDetails().getSiteNo());
//
// if (bindingResult.hasErrors()) {
// log.debug("ERROR : {}", bindingResult.getAllErrors().toString());
// throw new JsonValidationException(bindingResult);
// }
//
// ResultModel<CategoryPO> result = categoryManageService.updateCtgShowGoodsManage(po);
//
// return result;
// }
} |
//
// ExpressPayCreditvoidAdapter.swift
// ExpressPaySDK
//
// Created by ExpressPay(zik) on 15.02.2021.
//
import Foundation
/// The API Adapter for the CREDITVOID operation.
///
/// See *ExpressPayCreditvoidService*
///
/// See *ExpressPayCreditvoidCallback*
public final class ExpressPayCreditvoidAdapter: ExpressPayBaseAdapter<ExpressPayCreditvoidService> {
private let expressPayAmountFormatter = ExpressPayAmountFormatter()
/// Executes the *ExpressPayCreditvoidService.creditvoid* request.
/// - Parameters:
/// - transactionId: transaction ID in the Payment Platform. UUID format value.
/// - payerEmail: customer’s email. String up to 256 characters.
/// - cardNumber: the credit card number.
/// - amount: the amount for capture. Only one partial capture is allowed. Numbers in the form XXXX.XX (without leading zeros).
/// - callback: the *ExpressPayCreditvoidCallback*.
/// - Returns: the *URLSessionDataTask*
@discardableResult
public func execute(transactionId: String,
payerEmail: String,
cardNumber: String,
amount: Double?,
callback: @escaping ExpressPayCreditvoidCallback) -> URLSessionDataTask {
let hash = ExpressPayHashUtil.hash(email: payerEmail,
cardNumber: cardNumber,
transactionId: transactionId)!
return execute(transactionId: transactionId,
hash: hash,
amount: amount,
callback: callback)
}
/// Executes the *ExpressPayCreditvoidService.creditvoid* request.
/// - Parameters:
/// - transactionId: transaction ID in the Payment Platform. UUID format value.
/// - hash: special signature to validate your request to payment platform.
/// - amount: the amount for capture. Only one partial capture is allowed. Numbers in the form XXXX.XX (without leading zeros).
/// - callback: the *ExpressPayCreditvoidCallback*.
/// - Returns: the *URLSessionDataTask*
@discardableResult
public func execute(transactionId: String,
hash: String,
amount: Double?,
callback: @escaping ExpressPayCreditvoidCallback) -> URLSessionDataTask {
procesedRequest(action: .capture,
params: .init(transactionId: transactionId,
amount: expressPayAmountFormatter.amountFormat(for: amount),
hash: hash),
callback: callback)
}
} |
import { Component, OnInit } from '@angular/core';
import { Router } from '@angular/router';
import * as moment from 'moment';
import {
FormGroup,
FormControl,
Validators,
FormBuilder,
AbstractControl,
} from '@angular/forms';
import { Order } from 'src/app/model/order';
import { OrderService } from 'src/app/service/order.service';
import { User } from 'src/app/model/user';
import { UserService } from 'src/app/service/user.service';
@Component({
selector: 'app-createdit-order',
templateUrl: './createdit-order.component.html',
styleUrls: ['./createdit-order.component.css']
})
export class CreateditOrderComponent implements OnInit {
form: FormGroup = new FormGroup({});
order: Order = new Order();
mensaje: string = '';
dueDateOrder = new FormControl(new Date());
listaUsers:User[]=[]
idUserSeleccionada1:number=0
idUserSeleccionada2:number=0
constructor(
private oS: OrderService,
private uS: UserService,
private router: Router,
private formBuilder: FormBuilder
) {}
ngOnInit(): void {
this.form = this.formBuilder.group({
idOrder: [''],
shippingDate: ['', Validators.required],
arriveDate: ['', [Validators.required]],
destinationCountry: ['', Validators.required],
destinationCity: ['', Validators.required],
destinationAddress: ['', Validators.required],
originCountry: ['', Validators.required],
originCity: ['', Validators.required],
user: ['', [Validators.required]],
});
this.uS.list().subscribe((data) => {
this.listaUsers = data;
});
}
accept(): void {
if (this.form.valid) {
this.order.idOrder = this.form.value.idOrder;
this.order.shippingDate = this.form.value.shippingDate;
this.order.arriveDate = this.form.value.arriveDate;
this.order.destinationCountry = this.form.value.destinationCountry;
this.order.destinationCity = this.form.value.destinationCity;
this.order.destinationAddress = this.form.value.destinationAddress;
this.order.originCountry = this.form.value.originCountry;
this.order.originCity = this.form.value.originCity;
this.order.user.idUser = this.form.value.user;
this.oS.insert(this.order).subscribe((data) => {
this.oS.list().subscribe((data) => {
this.oS.setList(data);
});
});
this.router.navigate(['order']);
} else {
this.mensaje = 'Por favor complete todos los campos obligatorios.';
}
}
obtenerControlCampo(nombreCampo: string): AbstractControl {
const control = this.form.get(nombreCampo);
if (!control) {
throw new Error(`Control no encontrado para el campo ${nombreCampo}`);
}
return control;
}
} |
Reproducing structure-property relationships for different classes of
materials. This also enables quantification and for plots between different
variables than those provided by the source to be made. One can see that,
e.g., the density and melting point are positively correlated, even across
classes of materials. A simple explanation, that materials will generally be
more dense the stronger (and therefore more closely packed) their bonding is,
may explain this. In particular, polymers melt at much lower temperatures
because it is not atomic bonds that are lost, but intermolecular ones
(cross-linked polymers will generally decompose before melting).
One can also see that the yield stress at a given (compressive) strength is
about an order of magnitude higher for ceramics than the yield stress at a
given (tensile) strength for non-ceramics. By the note in the provided
reference, since the compressive strength is 1 order of magnitude higher than
the tensile strength for ceramics, all materials follow a nearly an equality
for tensile strength and yield stress. This implies that strain hardening is
not a significant effect in most materials, that is, the strength and yield
stress are nearly equal. Since yield stress is necessarily less than or equal
to strength, it must appear to one side of the 1:1 line, as is also shown.
Though silicon is a ceramic, it's elastic modulus is similar to metals
(similar to that of copper alloys!). Its fracture toughness is orders of
magnitude lower and so it fractures, but for the small amount of deformation
which it may undergo before crack propagation and fracturing, it does so as
easily as a metal. This is important in semiconductor processing, especially
because silicon wafers are around 700 𝜇m thick but can be 300 mm in diameter,
allowing them to deflect significantly in the direction perpendicular to the
plane of the wafer under low stress. The yield strain for silicon is higher
than that for metals, at around 2.2% compared to 1.2%---it just fractures
before it reaches the yield point, generally. A perfect single crystal of
silicon strains twice as much and with the same elastic modulus as a metal,
leading to it being a stronger material. In general, ceramics are brittle only
because their fracture toughness is small, and perfect crystals subject to
quasistatically imposed loads are stronger than metals.
Data Source: Materials Data Book, Cambridge University Engineering Department.
Both quantative data used here and qualitative correlation plots are provided
in this source. This source makes some finer categories than just metal,
ceramic, and polymer, such as polymer foam, which has significant void space
filled with air.
Temperature in deg. C.
Young's modulus in GPa
Density in g/cm^3 (= MG/m^3)
Yield stress in MPa
Tensile strength in MPa
Fracture toughness in MPa*m^(1/2) |
import { HttpException, HttpStatus, Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import {
IPaginationOptions,
paginate,
Pagination,
} from 'nestjs-typeorm-paginate';
import { NewsService } from 'src/news/news.service';
import { UserEntity } from 'src/user/entity/user.entity';
import { UserService } from 'src/user/user.service';
import { Repository } from 'typeorm';
import { CreateNewsCommentDTO, UpdateNewsCommentDTO } from './news_comment.dto';
import { NewsCommentEntity } from './news_comment.entity';
@Injectable()
export class NewsCommentService {
constructor(
@InjectRepository(NewsCommentEntity)
private newsCommentRepository: Repository<NewsCommentEntity>,
private newsService: NewsService,
private userService: UserService,
) {}
async getComments(
newsId: number,
search: string,
options: IPaginationOptions,
): Promise<Pagination<NewsCommentEntity>> {
if (!newsId) {
throw new HttpException('News comments not found!', HttpStatus.NOT_FOUND);
}
const queryBuilder = this.newsCommentRepository
.createQueryBuilder('c')
.leftJoinAndSelect('c.author', 'author')
.innerJoinAndSelect('c.news', 'news', 'news.id = :newsId', { newsId })
.where('c.title like :search', { search: `%${search}%` })
.where('c.content like :search', { search: `%${search}%` })
.orderBy('commentedAt', 'DESC');
return paginate<NewsCommentEntity>(queryBuilder, options);
}
async getCommentById(id: number): Promise<NewsCommentEntity> {
const comment = await this.newsCommentRepository.findOne({
where: { id },
relations: ['news', 'author'],
});
if (!comment) {
throw new HttpException('Comment not found!', HttpStatus.NOT_FOUND);
}
return comment;
}
async createComment(
commentPayload: CreateNewsCommentDTO,
user: UserEntity,
): Promise<NewsCommentEntity> {
const author = await this.userService.getUserById(user.id);
const news = await this.newsService.getNewsById(commentPayload.newsId);
const newComment = await this.newsCommentRepository.save({
content: commentPayload.content,
commentedAt: new Date(),
author,
news,
});
return newComment;
}
async editComment(
id: number,
commentPayload: UpdateNewsCommentDTO,
user: UserEntity,
): Promise<NewsCommentEntity> {
const author = await this.userService.getUserById(user.id);
const comment = await this.newsCommentRepository.findOneBy({
id,
author,
});
if (!comment) {
throw new HttpException('Forbidden', HttpStatus.FORBIDDEN);
}
comment.content = commentPayload.content;
const newComment = await this.newsCommentRepository.save(comment);
return newComment;
}
async deleteComment(
id: number,
user: UserEntity,
): Promise<NewsCommentEntity> {
const author = await this.userService.getUserById(user.id);
const comment = await this.newsCommentRepository.findOneBy({
id,
author,
});
if (!comment) {
throw new HttpException('Forbidden', HttpStatus.FORBIDDEN);
}
const deletedComment = await this.newsCommentRepository.remove(comment);
return deletedComment;
}
} |
import { useState, useEffect } from "react";
import logo from "../assets/logo.png";
import designer from "../assets/designer.png"
import insight from "../assets/insight.png"
import recruiter from "../assets/recruiter2.png"
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome';
import { faAngleLeft } from "@fortawesome/free-solid-svg-icons";
import {useForm} from "react-hook-form"
import { useNavigate } from "react-router-dom";
import axios from "axios";
import { profileRouter } from "../utils/apiRoutes";
function ParentComp() {
const [isDesignerChecked, setIsDesignerChecked] = useState(false);
const [isRecruiterChecked, setIsRecruiterChecked] = useState(false);
const [isInsightsChecked, setIsInsightsChecked] = useState(false);
const [formStep, setFormStep] = useState(0);
const [image, setImage] = useState(null);
const navigate = useNavigate();
useEffect(()=>{
const userData = localStorage.getItem("userData");
if(!userData)
navigate("/sign-up");
},[]);
const {
register,
handleSubmit,
formState: { errors, isValid, },
} = useForm({mode:"all"})
const handleChange = (event) => {
const selectedImage = event.target.files[0];
setImage(URL.createObjectURL(selectedImage));
};
const onSubmit = async(data) => {
const formData = new FormData();
formData.append("location", data.location);
if(data.recruiter)formData.append("recruiter", data.recruiter);
if(data.designer)formData.append("designer", data.designer);
if(data.insights)formData.append("insights", data.insights);
formData.append("profileImage", data.image[0]);
const userinfo= localStorage.getItem("userData");
const userId= JSON.parse(userinfo)._id;
const res = await axios.post(`${profileRouter}/${userId}`,
formData
,{
headers: { "Content-Type": "multipart/form-data" },
});
navigate("/verify")
}
const nextFormStep = ()=>{
setFormStep(curr=>curr+1);
}
const prevFormStep = ()=>{
setFormStep(curr=>curr-1);
}
// const revalidateForm = () => {
// trigger(); // Revalidate the form
// };
return (
<div className="p-6">
{/* <img src={logo} alt="" height={80} width={80} /> */}
<div className="flex">
<img src={logo} alt="" height={80} width={80} /> <FontAwesomeIcon className=
{`self-center mx-4 py-2 px-3 text-gray-500 cursor-pointer bg-gray-200 rounded-md ${formStep===0&& "invisible"} `}icon={faAngleLeft} onClick={formStep!==0 ? prevFormStep:undefined}/>
</div>
<form onSubmit={handleSubmit(onSubmit)} className="flex flex-col items-center justify-center ">
{formStep===0 ?
<div className="">
<div>
<h1 className="font-extrabold text-2xl md:text-3xl mb-3">
Welcome! Let's create your profile
</h1>
<div className="mb-10 text-gray-500 text-sm font-medium">
Let others get to know you better! You can do these later
</div>
</div>
<div className="font-bold mb-3">Add an avatar</div>
<div className="flex mb-10 items-center">
{/* <label> */}
<div className={`w-28 h-28 sm:w-32 sm:h-32 border-dashed border-2 ${image && "border-none"} border-gray-300 rounded-full flex justify-center items-center me-8 sm:me-10 overflow-hidden`}>
{image!==null ? (
<img src={image} alt="Uploaded" className="w-full h-full object-cover " />
) : (
<div className="w-5 m-auto top-50">
<svg
xmlns="http://www.w3.org/2000/svg"
version="1.1"
x="0px"
y="0px"
viewBox="0 0 100 125"
enableBackground="new 0 0 100 100"
>
<path
d="M81.916,22.583H68.165l-4.793-8.5H36.628l-4.793,8.5H18.084c-6.341,0-11.5,5.159-11.5,11.5v40.334c0,6.341,5.159,11.5,11.5,11.5h63.832c6.341,0,11.5-5.159,11.5-11.5V34.083C93.416,27.742,88.257,22.583,81.916,22.583z M50,76.999c-11.901,0-21.583-9.683-21.583-21.583c0-11.899,9.682-21.582,21.583-21.582s21.583,9.683,21.583,21.582C71.583,67.316,61.901,76.999,50,76.999z M53.5,40.834h-7v11h-11v7h11v11h7v-11h11v-7h-11V40.834z"
fill="#808080"
/>
</svg>
{/* <input id="upload1" type="file" className="hidden " name="image" accept="image/jpeg, image/png, image/jpg" {...register("image",{onChange:handleChange,required:"Required"})} /> */}
</div>
)}
</div>
{/* </label> */}
<div className="rounded-md border p-2 text-sm h-10 my-3">
<label
htmlFor="upload1"
className="flex flex-col items-center gap-2 cursor-pointer"
>
<span className="font-semibold">Choose image</span>
</label>
<input
id="upload1"
type="file"
className="hidden"
accept="image/jpeg, image/png, image/jpg"
{...register("image",{onChange:handleChange,required:"Required"})}
/>
</div>
</div>
<label htmlFor="location " className="block text-sm font-bold mb-3">Add your location</label>
<input type="text" id="location" className="bg-white focus:outline-none border-b-2 block w-full py-2.5 text-sm font-semibold mb-8 sm:mb-14 md:mb-16 focus:bg-white" placeholder="Enter a location" {...register("location",{required:"Location is required"})} />
<div className="w-52">
<button type="submit" className={`text-white bg-pink-500 hover:bg-pink-600 focus:ring-4 focus:outline-none focus:ring-pink-300 font-medium rounded-lg text-sm px-8 py-2 text-center w-full ${ (!image &&!isValid) && "cursor-not-allowed"}`} onClick={nextFormStep} disabled={!image && !isValid}
>Next</button>
</div>
</div>:
<div // className="m-auto " style={{ width: "50rem" }}
>
<h1 className="font-extrabold text-2xl md:text-3xl mb-3 text-center">
What brings you to Dribble?
</h1>
<div className="text-gray-500 text-xs md:text-sm font-medium text-center">
Select the option that best describes you. Don't worry, you can
explore other options later.
</div>
<div className="grid sm:grid-cols-3 mt-16 mb-6 gap-14 sm:gap-5 md:gap-7 justify-items-center ">
<label className="cursor-pointer w-60 sm:w-48 md:w-56 ">
{/* <input type="checkbox" className="peer sr-only" name="size-choice"/> */}
<div className={`rounded-lg ring-1 ring-gray-300 transition-all active:scale-95 ${isDesignerChecked && "ring-pink-500 ring-2 relative"} `}>
<img src={designer} alt="designer" className={`h-32 m-auto mb-2 ${isDesignerChecked &&"absolute -top-16 left-11"}`} />
<div className={`text-center p-3 ${isDesignerChecked&&"pt-16"}`}>
<div className="font-extrabold">I'm Designer looking to share my work</div>
<div className={`${!isDesignerChecked&&"hidden"} text-xs font-medium text-gray-500`} >Lorem ipsum dolor sit amet consectetur adipisicing elit. Officiis deserunt aspernatur voluptatibus itaque dignissimos explicabo necessitatibu</div>
<input type="checkbox" className="peer sr-only" checked={isDesignerChecked} {...register("designer",{onChange:() => setIsDesignerChecked(!isDesignerChecked)})}
/>
<span className="ring-black flex justify-center opacity-0 transition-all peer-checked:opacity-100 text-center">
<svg xmlns="http://www.w3.org/2000/svg" className="fill-pink-500 stroke-white" width="32" height="32" viewBox="0 0 24 24" strokeWidth="1.5" stroke="#2c3e50" fill="none" strokeLinecap="round" strokeLinejoin="round">
<path stroke="none" d="M0 0h24v24H0z" fill="none" />
<circle cx="12" cy="12" r="9" />
<path d="M9 12l2 2l4 -4" />
</svg>
</span>
{/* <span className="w-4 h-4 border"></span> */}
</div>
{/* <div > <span className="w-4 h-4 border"></span></div> */}
</div>
</label>
<label className="cursor-pointer w-60 sm:w-48 md:w-56 ">
{/* <input type="checkbox" className="peer sr-only" name="size-choice"/> */}
<div className={`rounded-lg ring-1 ring-gray-300 transition-all active:scale-95 ${isRecruiterChecked && "ring-pink-500 ring-2 relative"} `}>
<img src={recruiter} alt="designer" className={`h-32 m-auto mb-2 ${isRecruiterChecked &&"absolute -top-16 left-11"}`} />
<div className={`text-center p-3 ${isRecruiterChecked&&"pt-16"}`}>
<div className="font-extrabold">I'm looking to hire a designer</div>
<div className={`${!isRecruiterChecked&&"hidden"} text-xs font-medium text-gray-500`} >Lorem ipsum dolor sit amet consectetur adipisicing elit. Officiis deserunt aspernatur voluptatibus itaque dignissimos explicabo necessitatibu</div>
<input type="checkbox" className="peer sr-only" {...register("recruiter",{onChange:()=>setIsRecruiterChecked(!isRecruiterChecked)})} checked={isRecruiterChecked}/>
<span className="ring-black flex justify-center opacity-0 transition-all peer-checked:opacity-100 text-center">
<svg xmlns="http://www.w3.org/2000/svg" className="fill-pink-500 stroke-white" width="32" height="32" viewBox="0 0 24 24" strokeWidth="1.5" stroke="#2c3e50" fill="none" strokeLinecap="round" strokeLinejoin="round">
<path stroke="none" d="M0 0h24v24H0z" fill="none" />
<circle cx="12" cy="12" r="9" />
<path d="M9 12l2 2l4 -4" />
</svg>
</span>
</div>
</div>
</label>
<label className="cursor-pointer w-60 sm:w-48 md:w-56 ">
<div className={`rounded-lg ring-1 ring-gray-300 transition-all active:scale-95 ${isInsightsChecked && "ring-pink-500 ring-2 relative"} `}>
<img src={insight} alt="designer" className={`h-32 m-auto mb-2 ${isInsightsChecked &&"absolute -top-16 left-11"}`} />
<div className={`text-center p-3 ${isInsightsChecked&&"pt-16"}`}>
<div className="font-extrabold">I'm looking for design inspiration</div>
<div className={`${!isInsightsChecked&&"hidden"} text-xs font-medium text-gray-500`} >Lorem ipsum dolor sit amet consectetur adipisicing elit. Officiis deserunt aspernatur voluptatibus itaque dignissimos explicabo necessitatibu</div>
<input type="checkbox" className="peer sr-only" checked={isInsightsChecked} {...register("insights",{onChange:() => setIsInsightsChecked(!isInsightsChecked)})}
/>
<span className="ring-black flex justify-center opacity-0 transition-all peer-checked:opacity-100 text-center">
<svg xmlns="http://www.w3.org/2000/svg" className="fill-pink-500 stroke-white" width="32" height="32" viewBox="0 0 24 24" strokeWidth="1.5" stroke="#2c3e50" fill="none" strokeLinecap="round" strokeLinejoin="round">
<path stroke="none" d="M0 0h24v24H0z" fill="none" />
<circle cx="12" cy="12" r="9" />
<path d="M9 12l2 2l4 -4" />
</svg>
</span>
</div>
</div>
</label>
</div>
<div className="flex flex-col items-center">
<div className={`mb-3 font-bold ${isDesignerChecked || isInsightsChecked ||isRecruiterChecked ?'visible':'invisible'} text-sm`}>Anything else? You can select multiple </div>
{/* <Link to="/"> */}
<button
type="submit"
className={`text-white bg-pink-500 hover:bg-pink-600 focus:ring-4 focus:outline-none focus:ring-pink-300 font-medium rounded-lg text-sm px-8 py-2 w-52 ${!(isDesignerChecked || isInsightsChecked ||isRecruiterChecked) && "cursor-not-allowed"}`}
disabled={!(isDesignerChecked || isInsightsChecked ||isRecruiterChecked)}
>
Finish
</button>
{/* </Link> */}
<div className="text-xs text-gray-400 font-bold w-full m-2 text-center">
or Press RETURN
</div>
</div>
</div>
// </div>
}
</form>
</div>
)
}
export default ParentComp |
"use client";
import Header from "../header";
import { Context, } from "@/components/client";
import { useContext, useState } from "react";
import toast from "react-hot-toast";
import { redirect, useRouter } from "next/navigation";
import Image from "next/image";
import Hide from "../assets/hide.svg"
import Show from "../assets/show.svg"
import { decryptPassword, encryptPassword } from "@/utils/features";
export default function page() {
const [title, setTitle] = useState("");
const [uid, setUid] = useState("");
const [password, setPassword] = useState("");
const { user } = useContext(Context);
const router = useRouter()
const [hidepassword, setHidePassword] = useState(true);
const submitHandler = async (e) => {
e.preventDefault();
if (!uid || !password || !title ) {
return toast.error("All fields are required.");
}
const encodedPassword = encryptPassword(password,user.password)
try {
const res = await fetch("/api/newhandle", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
title,
uid,
password:encodedPassword,
}),
});
const data = await res.json();
if (!data.success) {
throw new Error(data.message); // Throw an error object with the message
}
toast.success(data.message);
setTitle("");
setUid("");
setPassword("");
router.push("/")
router.refresh()
} catch (error) {
toast.error(error.message); // Render only the error message
}
};
if (!user._id) {
router.push('/login');
return null;
}
return (
<main className="flex w-full h-full grow items-start justify-center pt-20 py-2 gap-5">
<Header />
<div className="w-full px-5 ">
<form onSubmit={submitHandler} class="bg-white shadow-md rounded px-8 pt-6 pb-8 mb-4 space-y-4 md:space-y-6 ">
<div>
<label
for="title"
className="block mb-2 text-sm font-medium text-gray-900 "
>
Enter the Platform Name
</label>
<input
onChange={(e) => setTitle(e.target.value)}
value={title}
type="title"
name="title"
id="title"
className="bg-gray-50 border border-gray-300 text-gray-900 sm:text-sm rounded-lg focus:outline-none block w-full p-2.5 "
placeholder="instagram, linkedIn, facebook"
required=""
></input>
</div>
<div>
<label
for="uid"
className="block mb-2 text-sm font-medium text-gray-900 "
>
Enter the UID for SignIn
</label>
<input
value={uid}
onChange={(e) => setUid(e.target.value)}
type="uid"
name="uid"
id="uid"
className="bg-gray-50 border border-gray-300 text-gray-900 sm:text-sm rounded-lg focus:outline-none block w-full p-2.5 "
placeholder="e.g @jerry, 88897868908"
required=""
></input>
</div>
<div>
<label
htmlFor="password"
className="block mb-2 text-sm font-medium text-gray-900 "
>
Password
</label>
<div className="bg-gray-50 border focus:border-2 border-gray-300 text-gray-900 sm:text-sm rounded-lg focus:ring-black w-full flex ">
<input
value={password}
type={hidepassword?"password":"text"}
placeholder="••••••••"
onChange={(e) => setPassword(e.target.value)}
className="w-full bg-transparent p-2.5 rounded-lg focus:outline-none "></input>
<Image alt="hide/show" onClick={()=>{
setHidePassword(
!hidepassword
)}} className="aspect-square mx-4" src={hidepassword?Hide:Show}></Image>
</div>
</div>
<span className="w-full flex justify-between">
{" "}
<button
className="bg-black text-white font-bold py-2 px-4 min-w-24 rounded "
type="submit"
>
Add
</button>
</span>
</form>
</div>
</main>
);
} |
/*
* Copyright 2017-2021 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.docksidestage.remote.petstore.user;
import java.util.function.Consumer;
import javax.annotation.Resource;
import org.dbflute.remoteapi.mock.MockHttpClient;
import org.docksidestage.remote.petstore.user.createwitharray.RemoteUserCreatewitharrayParam;
import org.docksidestage.remote.petstore.user.createwithlist.RemoteUserCreatewithlistParam;
import org.docksidestage.remote.petstore.user.index.RemoteUserGetReturn;
import org.docksidestage.remote.petstore.user.index.RemoteUserParam;
import org.docksidestage.remote.petstore.user.index.RemoteUserPutParam;
import org.docksidestage.remote.petstore.user.login.RemoteUserLoginParam;
import org.docksidestage.unit.UnitRemoteapigenTestCase;
import org.lastaflute.web.servlet.request.RequestManager;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* The behavior test for remote API of user.
* @author FreeGen
*/
public class RemotePetstoreUserBhvTest extends UnitRemoteapigenTestCase {
private static final Logger logger = LoggerFactory.getLogger(RemotePetstoreUserBhvTest.class);
@Resource
private RequestManager requestManager;
public void test_request() {
// ## Arrange ##
Consumer<RemoteUserParam> paramLambda = param -> {
param.id = 1L;
param.username = "username";
param.firstName = "firstName";
param.lastName = "lastName";
param.email = "email";
param.password = "password";
param.phone = "phone";
param.userStatus = 1;
};
// ## Act ##
createBhv(null).request(paramLambda);
}
public void test_requestCreatewitharray() {
// ## Arrange ##
Consumer<java.util.List<RemoteUserCreatewitharrayParam>> paramLambda = param -> {
RemoteUserCreatewitharrayParam rowParam = new RemoteUserCreatewitharrayParam();
rowParam.id = 1L;
rowParam.username = "username";
rowParam.firstName = "firstName";
rowParam.lastName = "lastName";
rowParam.email = "email";
rowParam.password = "password";
rowParam.phone = "phone";
rowParam.userStatus = 1;
param.add(rowParam);
};
// ## Act ##
createBhv(null).requestCreatewitharray(paramLambda);
}
public void test_requestCreatewithlist() {
// ## Arrange ##
Consumer<java.util.List<RemoteUserCreatewithlistParam>> paramLambda = param -> {
RemoteUserCreatewithlistParam rowParam = new RemoteUserCreatewithlistParam();
rowParam.id = 1L;
rowParam.username = "username";
rowParam.firstName = "firstName";
rowParam.lastName = "lastName";
rowParam.email = "email";
rowParam.password = "password";
rowParam.phone = "phone";
rowParam.userStatus = 1;
param.add(rowParam);
};
// ## Act ##
createBhv(null).requestCreatewithlist(paramLambda);
}
public void test_requestLogin() {
// ## Arrange ##
Consumer<RemoteUserLoginParam> paramLambda = param -> {
param.username = "username";
param.password = "password";
};
// ## Act ##
String returnBean = createBhv("String").requestLogin(paramLambda);
// ## Assert ##
logger.debug("returnBean={}", returnBean);
}
public void test_requestLogout() {
// ## Act ##
createBhv(null).requestLogout();
}
public void test_requestGet() {
// ## Arrange ##
String username = "username";
// ## Act ##
RemoteUserGetReturn returnBean = createBhv("{}").requestGet(username);
// ## Assert ##
logger.debug("id={}", returnBean.id);
logger.debug("username={}", returnBean.username);
logger.debug("firstName={}", returnBean.firstName);
logger.debug("lastName={}", returnBean.lastName);
logger.debug("email={}", returnBean.email);
logger.debug("password={}", returnBean.password);
logger.debug("phone={}", returnBean.phone);
logger.debug("userStatus={}", returnBean.userStatus);
}
public void test_requestPut() {
// ## Arrange ##
String username = "username";
Consumer<RemoteUserPutParam> paramLambda = param -> {
param.id = 1L;
param.username = "username";
param.firstName = "firstName";
param.lastName = "lastName";
param.email = "email";
param.password = "password";
param.phone = "phone";
param.userStatus = 1;
};
// ## Act ##
createBhv(null).requestPut(username, paramLambda);
}
public void test_requestDelete() {
// ## Arrange ##
String username = "username";
// ## Act ##
createBhv(null).requestDelete(username);
}
private RemotePetstoreUserBhv createBhv(String json) {
MockHttpClient client = MockHttpClient.create(response -> {
if (json == null) {
response.asJsonNoContent(request -> true);
} else {
response.asJsonDirectly(json, request -> true);
}
});
registerMock(client);
RemotePetstoreUserBhv bhv = new RemotePetstoreUserBhv(requestManager);
inject(bhv);
return bhv;
}
} |
<ui:composition xmlns="http://www.w3.org/1999/xhtml" xmlns:ui="http://xmlns.jcp.org/jsf/facelets"
xmlns:f="http://xmlns.jcp.org/jsf/core" xmlns:p="http://primefaces.org/ui" xmlns:h="http://xmlns.jcp.org/jsf/html" xmlns:hftl="http://hftl.org"
xmlns:hf="http://xmlns.jcp.org/jsf/composite/tags" template="/layout/template.xhtml">
<ui:define name="body">
<hftl:decorateFormPanel formId="reindexForm" label="#{messages['indexing.indexPanel']}">
<ui:define name="fields">
<h:panelGroup rendered="#{!indexingBean.enabled}">
<div class="ui-messages ui-widget">
<div class="ui-messages-info ui-corner-all">
<span class="ui-messages-info-warn"></span>
<ul>
<li><h:outputText value="#{messages['indexing.notEnabled']}" styleClass="ui-messages-warn-summary" /></li>
</ul>
</div>
</div>
</h:panelGroup>
<h:panelGroup rendered="#{indexingBean.enabled}">
<h:panelGroup id="reindexSummary">
<h:panelGroup rendered="#{indexingBean.reindexingFuture!=null}">
<p:poll interval="5" update="reindexSummary" stop="#{indexingBean.reindexingFuture!=null and indexingBean.reindexingFuture.isDone()}"
widgetVar="reindexStatusPoll" onerror="PF('reindexStatusPoll').stop()" />
<h:panelGroup rendered="#{indexingBean.reindexingFuture!=null}">
<h:panelGroup rendered="#{!indexingBean.reindexingFuture.isDone()}">
<div class="ui-messages ui-widget">
<div class="ui-messages-info ui-corner-all">
<span class="ui-messages-info-icon"></span>
<ul>
<li><h:outputText value="#{messages['indexing.reindexingInProgress']}" styleClass="ui-messages-info-summary" /></li>
</ul>
</div>
</div>
</h:panelGroup>
<h:panelGroup rendered="#{indexingBean.reindexingFuture.isDone()}">
<h:panelGroup rendered="#{indexingBean.reindexingFuture.get().exception == null}">
<div class="ui-messages ui-widget">
<div class="ui-messages-info ui-corner-all">
<span class="ui-messages-info-icon"></span>
<ul>
<li><h:outputText value="#{messages['indexing.finished']}" styleClass="ui-messages-info-summary" /></li>
</ul>
</div>
</div>
<p:dataTable lazy="false" value="#{indexingBean.reindexingFuture.get().recordsProcessed.entrySet()}" var="stats">
<p:column headerText="#{messages['indexing.stats.entityClass']}">
<h:outputText value="#{stats.key}" />
</p:column>
<p:column headerText="#{messages['indexing.stats.numberTotal']}">
<h:outputText value="#{stats.value.total}" />
</p:column>
<p:column headerText="#{messages['indexing.stats.numberOK']}">
<h:outputText value="#{stats.value.successfull}" />
</p:column>
</p:dataTable>
</h:panelGroup>
<h:panelGroup rendered="#{indexingBean.reindexingFuture.get().exception != null}">
<div class="ui-messages ui-widget">
<div class="ui-messages-error ui-corner-all">
<span class="ui-messages-error-icon"></span>
<ul>
<li><h:outputText value="#{messages['indexing.reindexingFailed']}" styleClass="ui-messages-error-summary" /></li>
<li><h:outputText value="&nbsp;" escape="false" /></li>
<li><h:outputText value="#{indexingBean.reindexingFuture.get().exception.message}" /></li>
</ul>
</div>
</div>
</h:panelGroup>
</h:panelGroup>
</h:panelGroup>
</h:panelGroup>
</h:panelGroup>
</h:panelGroup>
</ui:define>
<ui:define name="buttons">
<p:remoteCommand name="updateIndexSummary" update=":reindexForm:messages :reindexForm:reindexSummary" />
<p:commandButton action="#{indexingBean.cleanAndReindex}" value="#{messages['indexing.reindex']}" process="@this" update=":reindexForm:messages"
oncomplete="updateIndexSummary()" rendered="#{indexingBean.enabled and (indexingBean.reindexingFuture ==null or indexingBean.reindexingFuture.isDone())}">
<p:confirm header="#{messages['commons.confirmationHeader']}" message="#{messages['indexing.confirmReindex']}" icon="ui-icon-alert" />
</p:commandButton>
<p:commandButton action="#{indexingBean.cleanAndReindexAll}" value="#{messages['indexing.reindexAll']}" process="@this" update=":reindexForm:messages"
oncomplete="updateIndexSummary()" rendered="#{indexingBean.enabled and currentUser.providerCode == null and (indexingBean.reindexingFuture ==null or indexingBean.reindexingFuture.isDone())}">
<p:confirm header="#{messages['commons.confirmationHeader']}" message="#{messages['indexing.confirmReindex']}" icon="ui-icon-alert" />
</p:commandButton>
<p:commandButton value="#{messages['commons.refresh']}" update="messages reindexSummary"
rendered="#{indexingBean.reindexingFuture !=null and !indexingBean.reindexingFuture.isDone()}" />
</ui:define>
</hftl:decorateFormPanel>
</ui:define>
</ui:composition> |
<!DOCTYPE html>
<head>
<link rel="stylesheet" href="../style.css" />
<link rel="preconnect" href="https://fonts.gstatic.com">
<link href="https://fonts.googleapis.com/css2?family=Montserrat&display=swap" rel="stylesheet">
<link href="../prism.css" rel="stylesheet" />
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="description" content="Page description">
<meta property="og:title" content="Jetpack Compose: Rows - Hoinzey.dev">
<meta property="og:description" content="Post on android jetpack compose rows">
<meta property="og:locale" content="en_GB">
<meta property="og:type" content="website">
<meta property="og:url" content="https://www.hoinzey.dev/posts/compose-row.html">
<script async src="../prism.js"></script>
<script async src="../script.js"></script>
<title>
Jetpack Compose: Rows
</title>
</head>
<body>
<div class="container">
<header>
<h1>Hoinzey</h1>
<h2>Javascript. Kotlin. Android. Java</h2>
</header>
<div class="post">
<h1 class="post-h1">Jetpack Compose: Rows</h1>
<p class="post-date">10<sup>th</sup> April 2022</p>
<article class = "post-article">
<p class="post-paragraph">
Rows are one of the fundamental building blocks of compose. Think of them like a linear layout with a horizontal arrangement.
Lets take a look at what makes a row:
</p>
<br/>
<pre>
<code class="language-kotlin">
@Composable
inline fun Row(
modifier: Modifier = Modifier,
horizontalArrangement: Arrangement.Horizontal = Arrangement.Start,
verticalAlignment: Alignment.Vertical = Alignment.Top,
content: @Composable RowScope.() -> Unit)</code>
</pre>
<br/>
<p class="post-paragraph">
As rows are often used as a container for other content, we have access to a vertical alignment and horizontal arrangement properties which we can set.
There is also <code class="emph">RowScope</code> which gives the row's children access to some more values which can be used to influence their layout and display.
</p>
<br/>
<h2 class="post-h2">Vertical alignment</h2>
<h2 class="post-h2">Start, Center, Bottom</h2>
<p class="post-paragraph">
These values are pretty self-explanatory. They will stick the children vertically to the start center or end of the row
</p>
<br/>
<div class="code-block-wrapper cbw-3">
<div class="cb-header">Top</div>
<div class="cb-header">Center</div>
<div class="cb-header">Bottom</div>
<div class="code-block cb-3">
<pre>
<code class="language-kotlin">
Row(verticalAlignment = Alignment.Top) {
Text(...)
Text(...)
}</code>
</pre>
</div>
<div class="code-block cb-3">
<pre>
<code class="language-kotlin">
Row(verticalAlignment = Alignment.CenterVertically) {
Text(...)
Text(...)
}</code>
</pre>
</div>
<div class="code-block cb-3">
<pre>
<code class="language-kotlin">
Row(verticalAlignment = Alignment.Bottom) {
Text(...)
Text(...)
}</code>
</pre>
</div>
</div>
<br/>
<img src="../assets/compose-rows/vert-top-center-bottom.png" >
<h2 class="post-h2">Horizontal arrangement</h2>
<h2 class="post-h2">Start, Center, End</h2>
<p class="post-paragraph">
Again these values are pretty self-explanatory. They will stick the children horizontally to the start, center or end of the row
</p>
<br/>
<div class="code-block-wrapper cbw-3">
<div class="cb-header">Start</div>
<div class="cb-header">Center</div>
<div class="cb-header">End</div>
<div class="code-block cb-3">
<pre>
<code class="language-kotlin">
Row(horizontalArrangement = Arrangement.Start) {
Text(...)
Text(...)
}</code>
</pre>
</div>
<div class="code-block cb-3">
<pre>
<code class="language-kotlin">
Row(horizontalArrangement = Arrangement.Center) {
Text(...)
Text(...)
}</code>
</pre>
</div>
<div class="code-block cb-3">
<pre>
<code class="language-kotlin">
Row(horizontalArrangement = Arrangement.End) {
Text(...)
Text(...)
}</code>
</pre>
</div>
</div>
<br/>
<img src="../assets/compose-rows/hor-start-center-end.png" >
<h2 class="post-h2">Space between</h2>
<p class="post-paragraph">
Space between will evenly distribute the children throughout the row without adding any additional spacing before
the first of after the last child
</p>
<br/>
<pre>
<code class="language-kotlin">
Row(horizontalArrangement = Arrangement.SpaceBetween) {
Text(...)
Text(...)
}</code>
</pre>
<br/>
<img src="../assets/compose-rows/hor-space-between.png" >
<br/>
<h2 class="post-h3">Space around</h2>
<p class="post-paragraph">
Shamelessly taken from the docs: Place children such that they are spaced evenly across the main axis,
including free space before the first child and after the last child, but half the amount of space existing
otherwise between two consecutive children. Visually: #1##2##3# for LTR and #3##2##1# for RTL
</p>
<br/>
<pre>
<code class="language-kotlin">
Row(horizontalArrangement = Arrangement.SpaceAround) {
Text(...)
Text(...)
}</code>
</pre>
<br/>
<img src="../assets/compose-rows/hor-space-around.png" >
<br/>
<h2 class="post-h3">Space evenly</h2>
<p class="post-paragraph">
Place children such that they are spaced evenly across the main axis,
including free space before the first child and after the last child.
Visually: #1#2#3# for LTR and #3#2#1# for RTL
</p>
<br/>
<pre>
<code class="language-kotlin">
Row(horizontalArrangement = Arrangement.SpaceEvenly) {
Text(...)
Text(...)
}</code>
</pre>
<br/>
<img src="../assets/compose-rows/hor-space-evenly.png" >
<br/>
<h2 class="post-h2">RowScope</h2>
<p class="post-paragraph">
Any composable created within our Row has access to its RowScope. This gives access to some useful modifiers that the children can make use of.
</p>
<br/>
<h2 class="post-h2">Align</h2>
<p class="post-paragraph">
The align modifier takes in an Alignment as seen above in the vertical examples. If a child has this set through a modifier it <em>overrides</em> any that
is set on the parent. Allowing for a staggered set of views for example.
</p>
<div class="code-block-wrapper cbw-2">
<div class="cb-header">Example</div>
<div class="cb-header">Align modifier</div>
<div class="code-block cb-2">
<pre>
<code class="language-kotlin">
Row(...) {
Text(modifier = Modifier.align(Alignment.CenterVertically))
Text(...)
}</code>
</pre>
</div>
<div class="code-block cb-2">
<pre>
<code class="language-kotlin">
fun Modifier.align(alignment: Alignment.Vertical): Modifier</code>
</pre>
</div>
</div>
<br/>
<img src="../assets/compose-rows/ver-align-mid.png" >
<h2 class="post-h2">Weight</h2>
<p class="post-paragraph">
The weight modifier works much the same way as it does in HTML. It allows you to say that certain composables should take up greater portions of the screen
than others.
</p>
<br/>
<p class="post-paragraph">
For example of you have 2 Views - The first has a weight of 2 and the second has a weight of 1, the first View will take up 2/3 of the screen and leave
1/3 to the second view.
</p>
<br/>
<p class="post-paragraph">
The fill property allows us to say that if a composable should take up 2/3 but its content only currently fills 1/3 then we will only draw it
as 1/3 but the remaining space can't be taken by our other views.
</p>
<div class="code-block-wrapper cbw-2">
<div class="cb-header">Weight 1 / 1 / 1</div>
<div class="cb-header">Weight 1 / 2 / 1</div>
<div class="code-block cb-2">
<pre>
<code class="language-kotlin">
Row(...) {
Text(modifier = Modifier.weight(1f))
Text(modifier = Modifier.weight(1f))
Text(modifier = Modifier.weight(1f))
}</code>
</pre>
</div>
<div class="code-block cb-2">
<pre>
<code class="language-kotlin">
Row(...) {
Text(modifier = Modifier.weight(1f)
Text(modifier = Modifier.weight(2f))
Text(modifier = Modifier.weight(1f))
}</code>
</pre>
</div>
</div>
<br/>
<img src="../assets/compose-rows/hor-weight-even.png" >
<img src="../assets/compose-rows/hor-weight-odd.png" >
</article>
</div>
<aside class="aside">
<section class="bio">
<p>
This is not a blog, plenty of people do a better job of that.
I work in software and I forget stuff so I'm going to write it down
</p>
</section>
<section class="links">
<ul>
<li>
<a href="../index.html">Home</a>
</li>
</ul>
</section>
</aside>
</div>
<footer class="footer"></footer>
</body> |
from abc import ABC, abstractmethod
from dataclasses import dataclass
from typing import List
from .consts import ColorType, CommandOpcodes
@dataclass
class Command(ABC):
"""
Base command class
"""
@abstractmethod
def opcode(self) -> CommandOpcodes:
"""
Returns the opcode for this command
"""
@abstractmethod
def serialize_params(self) -> List[int]:
"""
Returns the serialized params for this command
"""
@dataclass
class OnOffCommand(Command):
"""
A command to turn lights on/off
"""
on: bool
def opcode(self) -> CommandOpcodes:
return CommandOpcodes.SET_ON_OFF
def serialize_params(self) -> List[int]:
return [0x01 if self.on else 0x00, 0x00, 0x00]
@dataclass
class SetBrightnessCommand(Command):
"""
A command to set brightness
"""
brightness: int
def opcode(self) -> CommandOpcodes:
return CommandOpcodes.SET_BRIGHTNESS
def serialize_params(self) -> List[int]:
return [self.brightness]
@dataclass
class SetColorCommand(Command):
"""
A command to set rgb color
"""
r: int
g: int
b: int
def opcode(self) -> CommandOpcodes:
return CommandOpcodes.SET_COLOR
def serialize_params(self) -> List[int]:
return [ColorType.RGB.value, self.r, self.g, self.b]
@dataclass
class SetTemperatureCommand(Command):
"""
A command to set white color temperature
"""
temperature: int
def opcode(self) -> CommandOpcodes:
return CommandOpcodes.SET_COLOR
def serialize_params(self) -> List[int]:
return [ColorType.TEMPERATURE.value, self.temperature] |
import { KeyValueType } from "../../types/KeyValue.type";
import { PubSubBulkPublishMessage } from "../../types/pubsub/PubSubBulkPublishMessage.type";
import { PubSubBulkPublishResponse } from "../../types/pubsub/PubSubBulkPublishResponse.type";
import { PubSubPublishOptions } from "../../types/pubsub/PubSubPublishOptions.type";
import { PubSubPublishResponseType } from "../../types/pubsub/PubSubPublishResponse.type";
export default interface IClientPubSub {
/**
* Publish data to a topic.
* If the data is a valid cloud event, it will be published with Content-Type: application/cloudevents+json.
* Otherwise, if it's a JSON object, it will be published with Content-Type: application/json.
* Otherwise, it will be published with Content-Type: text/plain.
* @param pubSubName name of the pubsub component
* @param topic name of the topic
* @param data data to publish
* @param metadata metadata for the message
*
* @returns response from the publish
*/
publish(pubSubName: string, topic: string, data?: object | string, options?: PubSubPublishOptions): Promise<PubSubPublishResponseType>;
/**
* Publish data to a topic in bulk.
* If the data is a valid cloud event, it will be published with Content-Type: application/cloudevents+json.
* Otherwise, if it's a JSON object, it will be published with Content-Type: application/json.
* Otherwise, it will be published with Content-Type: text/plain.
* @param pubSubName name of the pubsub component
* @param topic name of the topic
* @param messages array of messages to publish
* @param metadata metadata for the request
*
* @returns list of failed entries if any
*/
publishBulk(pubSubName: string, topic: string, messages: PubSubBulkPublishMessage[], metadata?: KeyValueType): Promise<PubSubBulkPublishResponse>;
} |
import React from "react";
import {View, Text, FlatList, ScrollView, TouchableOpacity} from "react-native";
import PropTypes from "prop-types";
import styles from "./style";
import MatchItem from "./MatchItem";
import {themes} from "../../constants/colors";
import ScrollableTabView from "react-native-scrollable-tab-view";
import scrollPersistTaps, {scrollProps} from "../../utils/scrollPersistTaps";
import EmptyView from "./EmptyView";
export default class CricketView extends React.Component {
static propTypes = {
navigation: PropTypes.object,
theme: PropTypes.string
};
constructor(props) {
super(props);
this.state = {
matches: [
{
id: 1,
league_name: 'MGM Odisha T20',
team_a: {
name: 'Odisha Cheetahs',
avatar: 'default',
symbol: 'ODC'
},
team_b: {
name: 'Odisha Pumas',
avatar: 'default',
symbol: 'OPU'
},
left_time: '24h',
is_live: true,
is_timing: true,
allow_notify: true,
team_count: 2,
contest_count: 2
},
{
id: 2,
league_name: 'MGM Odisha T20',
team_a: {
name: 'Odisha Cheetahs',
avatar: 'default',
symbol: 'ODC'
},
team_b: {
name: 'Odisha Pumas',
avatar: 'default',
symbol: 'OPU'
},
left_time: '24h',
is_live: true,
is_timing: true,
allow_notify: true,
team_count: 1,
contest_count: 1
}
],
}
}
onPressMatch = (match) => {
const { navigation } = this.props;
navigation.navigate('ContestView', { match: {...match} });
}
renderTabContent = (type) => {
const { navigation, theme } = this.props;
const { matches } = this.state;
if(!matches.length){
return (
<EmptyView theme={theme} onViewComing={() => navigation.navigate('HomeView')} />
)
}
return (
<View
key={`Cricket-${type}`}
style={styles.tabContent}
>
<FlatList
key={`cricket-matches`}
style={styles.matchList}
keyExtractor={item => item.id || item}
data={matches}
renderItem={({ item }) => {
return (<MatchItem data={item} onPress={ () => this.onPressMatch(item)} theme={theme}/>);
}}
initialNumToRender={10}
removeClippedSubviews
/>
</View>
);
}
goToPage = (i) => {
if(this.tabViewRef){
this.tabViewRef.goToPage(i);
}
}
isActivePage = (i) => {
return this.tabViewRef && this.tabViewRef.state.currentPage === i;
}
renderTab = () =>{
const { theme } = this.props;
let tabs = [
{ text: 'Upcoming' },
{ text: 'Live' },
{ text: 'Completed' },
];
return (
<View style={{ ...styles.tabBarContainer, backgroundColor: themes[theme].backgroundColor }}>
<ScrollView horizontal={true} showsHorizontalScrollIndicator={false} {...scrollPersistTaps} contentContainerStyle={styles.subTabsContainer}>
{ tabs.map((tab, i) => (
<TouchableOpacity
activeOpacity={0.7}
key={tab.text}
onPress={() => this.goToPage(i)}
style={styles.subTab}
testID={`Cricket-${ tab }`}
>
{ this.isActivePage(i) ?
<Text style={{ ...styles.activeTabText, color: themes[theme].activeTintColor }}>{tab.text}</Text>
:
<Text style={{ ...styles.tabText, color: themes[theme].inactiveTintColor }}>{tab.text}</Text>
}
</TouchableOpacity>
))}
</ScrollView>
</View>
);
}
render = () => {
const { navigation, theme } = this.props;
return (
<ScrollableTabView
key='Cricket'
ref={ref => this.tabViewRef = ref}
renderTabBar={ this.renderTab }
initialPage={0}
contentProps={scrollProps}
>
{ this.renderTabContent('upcoming')}
{ this.renderTabContent('live')}
{ this.renderTabContent('completed')}
</ScrollableTabView>
);
}
} |
import React from "react";
class Expressions extends React.Component {
constructor(props) {
super(props);
this.state = {lines: []};
this.headers = [
{ key: 'ID', label: 'ID'},
{ key: 'Chinese', label: 'Chinese' },
{ key: 'English', label: 'English' },
{ key: 'Comment', label: 'Comment' },
];
}
// deleteEmployees = (event) => {
// event.preventDefault();
// if(window.confirm('Are you sure, want to delete the selected employee?')) {
// alert(this.state.checkedBoxes + " Succesfully Deleted");
// }
// }
// toggleCheckbox = (e, item) => {
// if(e.target.checked) {
// let arr = this.state.checkedBoxes;
// arr.push(item.id);
// this.setState = { checkedBoxes: arr};
// } else {
// let items = this.state.checkedBoxes.splice(this.state.checkedBoxes.indexOf(item.id), 1);
// this.setState = {
// checkedBoxes: items
// }
// }
// console.log(this.state.checkedBoxes);
// }
componentDidMount() {
// Fetch returns " A Promise that resolves to a Response object."
// in the browser, you can inspect the response.
// remember the following syntax, x y is not declared.
// fetch(file)
// .then(x => x.text())
// .then(y => myDisplay(y));
fetch('http://localhost:80/myFirstSite_Back/toFetchData.php?table=Expressions').then(response => {
// response refers to the Response object returned by fetch.
// you can use X to refer to it as well.
console.log(response);
// https://developer.mozilla.org/en-US/docs/Web/API/Response/json
// response.json takes json and turn it into an object.
return response.json();
//Where is the 'result' from?
// it refers to the result of response.json().
// it doesn't need to be declared.
}).then(result => {
// Work with JSON data here
console.log(result);
this.setState({
// result is an js object returned by response.json()
lines:result
});
}).catch(err => {
// Do something for an error here
console.log("Error Reading data " + err);
});
}
render() {
// Isn't this expression redundant?
const lineFound = this.state.lines && this.state.lines.length;
if(lineFound) {
return (
<div className="container"><h1>Hard Translations Between Chinese and English</h1>
<div id="msg"></div>
{/* <button type="button" className="btn btn-danger" onClick={this.deleteEmployees}>Delete Selected Employee(s)</button> */}
{/* I think the table is styled by bootstrap */}
<table className="table table-bordered table-striped">
<thead>
<tr>
{
// What is this line doing here?
this.headers.map(function(h) {
return (
// th defines a header cell in HTML
<th key={h.key}>{h.label}</th>
)
})
}
</tr>
</thead>
<tbody>
{
// 这个item 和index分别指代的啥?
this.state.lines.map(function(item, index) {
// to put two quizzes in an array and shuffle them
// must test if it works.
// const quizOne = <td><Quiz question={item.QuestionOne} choices={[item.WordOne,item.WordTwo]} answer={item.WordOne}/></td>
// const quizTwo = <td><Quiz question={item.QuestionTwo} choices={[item.WordOne,item.WordTwo]} answer={item.WordTwo}/></td>
// const a = [quizOne,quizTwo].sort()
return (
<tr key={index}>
{/* <td><input type="checkbox" className="selectsingle" value="{item.id}" checked={this.state.checkedBoxes.find((p) => p.id === item.id)} onChange={(e) => this.toggleCheckbox(e, item)}/>
{item.id}
</td> */}
{/* 到后续要用JSON里面的内容的时候,按照下面这样就可以获取了 */}
<td>{item.ID}</td>
<td>{item.Chinese}</td>
<td>{item.English}</td>
<td>{item.Comment}</td>
</tr>
)}.bind(this))
}
</tbody>
</table>
</div>
)
} else {
return (
<div id="container">
No product found
</div>
)
}
}
}
export default Expressions; |
import { useState, useEffect } from 'react';
import PricingPlan from '../components/PricingPlan';
const MyWallet = () => {
const [showModal, setShowModal] = useState(false);
const [walletType, setWalletType] = useState('');
const [walletAddress, setWalletAddress] = useState('');
const [walletTypeError, setWalletTypeError] = useState(false);
const [walletAddressError, setWalletAddressError] = useState(false);
const [wallets, setWallets] = useState(() => {
// Load wallets from local storage on component mount
const storedWallets = localStorage.getItem('wallets');
return storedWallets ? JSON.parse(storedWallets) : [];
});
useEffect(() => {
// Save wallets to local storage whenever it changes
localStorage.setItem('wallets', JSON.stringify(wallets));
}, [wallets]);
// Function to handle "Add my Wallet" button click
const handleAddWalletClick = (e) => {
e.preventDefault();
setShowModal(true);
// Disable scrolling when modal is opened
document.body.style.overflow = 'hidden';
};
// Function to handle wallet addition
const handleWalletAdded = (e) => {
e.preventDefault();
if (walletType && walletAddress) {
// Add the new wallet to the wallets state
setWallets((prevWallets) => [
...prevWallets,
{ type: walletType, address: walletAddress },
]);
// Reset input fields and errors
setWalletType('');
setWalletAddress('');
setWalletTypeError(false);
setWalletAddressError(false);
setShowModal(false); // Close the modal after adding wallet
// Enable scrolling when modal is closed
document.body.style.overflow = 'auto';
} else {
if (!walletType) setWalletTypeError(true);
if (!walletAddress) setWalletAddressError(true);
}
};
// Function to handle cancellation of wallet addition
const handleCancel = () => {
// Reset input fields and errors
setWalletType('');
setWalletAddress('');
setWalletTypeError(false);
setWalletAddressError(false);
setShowModal(false);
// Enable scrolling when modal is closed
document.body.style.overflow = 'auto';
};
// Function to handle deletion of wallet address
const handleDeleteWallet = (index) => {
const updatedWallets = [...wallets];
updatedWallets.splice(index, 1);
setWallets(updatedWallets);
};
return (
<div className="h-screen flex flex-col justify-between">
<div className="shadow-xl py-5 px-5 max-lg:mt-10">
<p className="text-xl my-5">My Wallets</p>
{wallets.map((wallet, index) => (
<div key={index} className="flex items-center justify-between mb-4">
<p>
{wallet.type}: {wallet.address}
</p>
<button
className="text-red-500"
onClick={() => handleDeleteWallet(index)}
>
Delete
</button>
</div>
))}
{!showModal && (
<div className="flex justify-center">
<button
className="font-normal text-center transition-all duration-150 ease-in-out hover:bg-blue-700 cursor-pointer hover:text-white text-[#007bff] border-2 px-5 py-2 rounded border-[#007bff]"
onClick={handleAddWalletClick}
>
Add my Wallet
</button>
</div>
)}
</div>
{/* Display modal if showModal state is true */}
{showModal && (
<div className="fixed inset-0 flex items-center justify-center bg-black bg-opacity-50">
<div className="bg-white rounded-lg p-8 w-96">
<p className="text-center font-semibold mb-3">Add Wallet</p>
<select
name="walletType"
value={walletType}
onChange={(e) => {
setWalletType(e.target.value);
setWalletTypeError(false); // Reset error state
}}
className="w-full rounded border border-gray-300 p-2 mb-3"
>
<option value="">Select Wallet Type</option>
<option value="BTC">BTC</option>
<option value="ETH">ETH</option>
<option value="LTC">LTC</option>
<option value="XRP">XRP</option>
<option value="USDT">USDT</option>
<option value="Account Balance">Account Balance</option>
</select>
{/* Wallet address input */}
<input
type="text"
value={walletAddress}
onChange={(e) => {
setWalletAddress(e.target.value);
setWalletAddressError(false); // Reset error state
}}
className="w-full rounded border border-gray-300 p-2 mb-3"
placeholder="Enter Wallet Address"
/>
{/* Error messages */}
{walletTypeError && (
<p className="text-red-500 text-sm mb-3">
Please select a wallet type
</p>
)}
{walletAddressError && (
<p className="text-red-500 text-sm mb-3">
Please enter your wallet address
</p>
)}
{/* Buttons */}
<div className="flex justify-between">
<button
className="bg-blue-500 text-white px-4 py-2 rounded hover:bg-blue-600"
onClick={handleWalletAdded}
>
Add my Wallet
</button>
<button
className="bg-gray-300 text-gray-700 px-4 py-2 rounded hover:bg-gray-400"
onClick={handleCancel}
>
Cancel
</button>
</div>
</div>
</div>
)}
<PricingPlan />
</div>
);
};
export default MyWallet; |
package actions
import (
"context"
"encoding/hex"
"mime"
"net/http"
"github.com/lantah/go/network"
"github.com/lantah/go/protocols/orbitr"
hProblem "github.com/lantah/go/services/orbitr/internal/render/problem"
"github.com/lantah/go/services/orbitr/internal/resourceadapter"
"github.com/lantah/go/services/orbitr/internal/txsub"
"github.com/lantah/go/support/errors"
"github.com/lantah/go/support/render/hal"
"github.com/lantah/go/support/render/problem"
"github.com/lantah/go/xdr"
)
type NetworkSubmitter interface {
Submit(ctx context.Context, rawTx string, envelope xdr.TransactionEnvelope, hash string) <-chan txsub.Result
}
type SubmitTransactionHandler struct {
Submitter NetworkSubmitter
NetworkPassphrase string
DisableTxSub bool
CoreStateGetter
}
type envelopeInfo struct {
hash string
innerHash string
raw string
parsed xdr.TransactionEnvelope
}
func extractEnvelopeInfo(raw string, passphrase string) (envelopeInfo, error) {
result := envelopeInfo{raw: raw}
err := xdr.SafeUnmarshalBase64(raw, &result.parsed)
if err != nil {
return result, err
}
var hash [32]byte
hash, err = network.HashTransactionInEnvelope(result.parsed, passphrase)
if err != nil {
return result, err
}
result.hash = hex.EncodeToString(hash[:])
if result.parsed.IsFeeBump() {
hash, err = network.HashTransaction(result.parsed.FeeBump.Tx.InnerTx.V1.Tx, passphrase)
if err != nil {
return result, err
}
result.innerHash = hex.EncodeToString(hash[:])
}
return result, nil
}
func (handler SubmitTransactionHandler) validateBodyType(r *http.Request) error {
c := r.Header.Get("Content-Type")
if c == "" {
return nil
}
mt, _, err := mime.ParseMediaType(c)
if err != nil {
return errors.Wrap(err, "Could not determine mime type")
}
if mt != "application/x-www-form-urlencoded" && mt != "multipart/form-data" {
return &hProblem.UnsupportedMediaType
}
return nil
}
func (handler SubmitTransactionHandler) response(r *http.Request, info envelopeInfo, result txsub.Result) (hal.Pageable, error) {
if result.Err == nil {
var resource orbitr.Transaction
err := resourceadapter.PopulateTransaction(
r.Context(),
info.hash,
&resource,
result.Transaction,
)
return resource, err
}
if result.Err == txsub.ErrTimeout {
return nil, &hProblem.Timeout
}
if result.Err == txsub.ErrCanceled {
return nil, &hProblem.ClientDisconnected
}
switch err := result.Err.(type) {
case *txsub.FailedTransactionError:
rcr := orbitr.TransactionResultCodes{}
resourceadapter.PopulateTransactionResultCodes(
r.Context(),
info.hash,
&rcr,
err,
)
return nil, &problem.P{
Type: "transaction_failed",
Title: "Transaction Failed",
Status: http.StatusBadRequest,
Detail: "The transaction failed when submitted to the lantah network. " +
"The `extras.result_codes` field on this response contains further " +
"details. Descriptions of each code can be found at: " +
"https://developers.stellar.org/api/errors/http-status-codes/horizon-specific/transaction-failed/",
Extras: map[string]interface{}{
"envelope_xdr": info.raw,
"result_xdr": err.ResultXDR,
"result_codes": rcr,
},
}
}
return nil, result.Err
}
func (handler SubmitTransactionHandler) GetResource(w HeaderWriter, r *http.Request) (interface{}, error) {
if err := handler.validateBodyType(r); err != nil {
return nil, err
}
if handler.DisableTxSub {
return nil, &problem.P{
Type: "transaction_submission_disabled",
Title: "Transaction Submission Disabled",
Status: http.StatusMethodNotAllowed,
Detail: "Transaction submission has been disabled for OrbitR. " +
"To enable it again, remove env variable DISABLE_TX_SUB.",
Extras: map[string]interface{}{},
}
}
raw, err := getString(r, "tx")
if err != nil {
return nil, err
}
info, err := extractEnvelopeInfo(raw, handler.NetworkPassphrase)
if err != nil {
return nil, &problem.P{
Type: "transaction_malformed",
Title: "Transaction Malformed",
Status: http.StatusBadRequest,
Detail: "OrbitR could not decode the transaction envelope in this " +
"request. A transaction should be an XDR TransactionEnvelope struct " +
"encoded using base64. The envelope read from this request is " +
"echoed in the `extras.envelope_xdr` field of this response for your " +
"convenience.",
Extras: map[string]interface{}{
"envelope_xdr": raw,
},
}
}
coreState := handler.GetCoreState()
if !coreState.Synced {
return nil, hProblem.StaleHistory
}
submission := handler.Submitter.Submit(r.Context(), info.raw, info.parsed, info.hash)
select {
case result := <-submission:
return handler.response(r, info, result)
case <-r.Context().Done():
if r.Context().Err() == context.Canceled {
return nil, hProblem.ClientDisconnected
}
return nil, hProblem.Timeout
}
} |
import React from 'react';
import DashboardHeader from '../../../components/DashboardHeader/DashboardHeader';
import usePayment from '../../../hooks/usePayment';
import EmptyData from '../../../components/EmptyData/EmptyData';
import { Link } from 'react-router-dom';
import FadeInAnimation from '../../../components/FadeInAnimation/FadeInAnimation';
import { Helmet } from 'react-helmet-async';
const EnrolledClasses = () => {
const [payments, isLoading] = usePayment();
console.log(payments);
return (
<>
<div className="container mx-auto px-4 sm:px-8 py-8">
<Helmet>
<title>Enrolled Courses</title>
</Helmet>
<DashboardHeader title={"My Enrolled Courses"} />
{payments && Array.isArray(payments) && payments.length > 0 ? (
<FadeInAnimation>
<div className="overflow-x-auto mt-3">
<table className="table">
<thead className="text-base text-gray-700 dark:text-white">
<tr>
<th> SL </th>
<th> Course </th>
<th> Instructor Name </th>
<th> Instructor Email </th>
<th> Enrolled Date </th>
</tr>
</thead>
<tbody className="text-gray-600 dark:text-white">
{payments.map((payment) =>
payment.items.map((item, index) => (
<tr key={item._id} className="border-gray-300">
<th>{index + 1}</th>
<td>
<div className="flex items-center gap-3">
<div className="avatar">
<div className="mask mask-squircle w-12 h-12">
<img
src={item.itemsImage}
alt="Avatar Tailwind CSS Component"
/>
</div>
</div>
<div>
<div className="font-semibold">
{item.itemsName}
</div>
</div>
</div>
</td>
<td className="px-6 py-4">{item?.Instructor}</td>
<td className="px-6 py-4">{item?.InstructorEmail}</td>
<td className="px-6 py-4">
{payment.date.slice(0, 10)}
</td>
</tr>
))
)}
</tbody>
</table>
</div>
</FadeInAnimation>
) : (
<>
<FadeInAnimation>
<EmptyData message={"You haven't enrolled to any class"} />
<div className="text-center -mt-52 md:-mt-40">
<Link
className="btn bg-amber-500 text-white custom-btn"
to="/courses"
>
Add Course To Cart
</Link>
</div>
</FadeInAnimation>
</>
)}
</div>
</>
);
};
export default EnrolledClasses; |
part of 'product_cubit.dart';
@immutable
abstract class ProductState {
final List<ProductModel> products;
ProductState(this.products);
}
class ProductInitialState extends ProductState {
ProductInitialState() : super([]);
}
class ProductLoadingState extends ProductState{
ProductLoadingState(super.products);
}
class ProductLoadedState extends ProductState{
ProductLoadedState(super.products);
}
class ProductErrorState extends ProductState{
final String message;
ProductErrorState(this.message , super.products);
} |
# terrace area as percentage of neighbourhood total non-built area
library(sf)
library(tmap)
library(tmaptools)
library(tidyverse)
library(dbscan)
library(fs)
set.seed(1992)
# download terraces
t <- read.csv("https://opendata-ajuntament.barcelona.cat/data/dataset/9cefbfa2-bcdf-44a0-b63a-372b48f9da93/resource/291a2c18-b786-4dfd-ae92-737a5f353699/download/2022_1s_data_set_opendata_terrasses.csv")
t_sf <- st_as_sf(t, coords = c(x = "X_ETRS89", y = "Y_ETRS89"), crs = "EPSG:25831")
#
t_area_neighbourhood <- tapply(t$SUPERFICIE_OCUPADA,t$NOM_BARRI,sum)
t_area_df <- data.frame("barri" = names(t_area_neighbourhood),
"area" = unname(t_area_neighbourhood))
# download and read layer w/ neighbourhoods
temp <- tempfile()
download.file(URLencode("https://opendata-ajuntament.barcelona.cat/data/dataset/808daafa-d9ce-48c0-925a-fa5afdb1ed41/resource/cd800462-f326-429f-a67a-c69b7fc4c50a/download"), temp)
unzip(temp, exdir = "data/adm_units")
bcn <- read_sf("data/adm_units/0301100100_UNITATS_ADM_POLIGONS.json")
nbs <- bcn[bcn$CONJ_DESCR == "Barris",]
# get total built area per neighbourhood
builds <-
# join terrace area, get total area
nbs_terrace_area <- nbs |>
select(NOM, geometry) |>
mutate(NOM = case_when(NOM == "el Poble-sec" ~ "el Poble Sec",
TRUE ~ NOM)) |>
left_join(t_area_df, by = c("NOM"="barri")) |>
rename("terrace_area" = "area") |>
mutate("total_area" = as.numeric(st_area(geometry)),
"pct_terraces" = (terrace_area/total_area)*100)
palette <- c("#00843E", "#ebc246", "#dc143c","#654321","#000000")
# plot
tm_shape(nbs_terrace_area) +
tm_polygons(col = "pct_terraces",
palette = palette,
title = "% of surface",
style = "jenks",
textNA = "Sense dades") +
tm_compass() +
tm_scale_bar() +
tm_layout("Dia 03: Polígons\n% de la superfície dels barris\nocupada per terrasses a Barcelona",
fontfamily = "Roboto condensed",
bg.color = "antiquewhite2",
legend.format=list(text.separator = "-",
fun=function(x) paste0(formatC(x, digits=2, format="f"), " %"))) |
part of 'order_watcher_bloc.dart';
class OrderWatcherState extends Equatable {
const OrderWatcherState({
required this.orderTicketList,
required this.failureOption,
required this.status,
});
final List<OrderTicketData> orderTicketList;
final Option<Failure> failureOption;
final LoadStatus status;
factory OrderWatcherState.initial() => OrderWatcherState(
orderTicketList: const <OrderTicketData>[],
failureOption: none(),
status: LoadStatus.initial,
);
OrderWatcherState copyWith({
List<OrderTicketData>? orderTicketList,
Option<Failure>? failureOption,
LoadStatus? status,
}) {
return OrderWatcherState(
orderTicketList: orderTicketList ?? this.orderTicketList,
failureOption: failureOption ?? this.failureOption,
status: status ?? this.status,
);
}
@override
List<Object> get props => [
orderTicketList,
failureOption,
status,
];
} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Acent Services</title>
<meta content="width=device-width, initial-scale=1.0" name="viewport">
<meta content="" name="keywords">
<meta content="" name="description">
<!-- Favicon -->
<link href="img/favicon.ico" rel="icon">
<!-- Google Web Fonts -->
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Heebo:wght@400;500&family=Jost:wght@500;600;700&display=swap"
rel="stylesheet">
<!-- Icon Font Stylesheet -->
<link href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.10.0/css/all.min.css" rel="stylesheet">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/font/bootstrap-icons.css" rel="stylesheet">
<!-- Libraries Stylesheet -->
<link href="lib/animate/animate.min.css" rel="stylesheet">
<link href="lib/owlcarousel/assets/owl.carousel.min.css" rel="stylesheet">
<!-- Customized Bootstrap Stylesheet -->
<link href="css/bootstrap.min.css" rel="stylesheet">
<!-- Template Stylesheet -->
<link href="css/style.css" rel="stylesheet">
</head>
<body data-bs-spy="scroll" data-bs-target=".navbar" data-bs-offset="51">
<div class="container-xxl bg-white p-0">
<!-- Spinner Start -->
<div id="spinner"
class="show bg-white position-fixed translate-middle w-100 vh-100 top-50 start-50 d-flex align-items-center justify-content-center">
<div class="spinner-grow text-primary" style="width: 3rem; height: 3rem;" role="status">
<span class="sr-only">Loading...</span>
</div>
</div>
<!-- Spinner End -->
<!-- Navbar & Hero Start -->
<div class="container-xxl position-relative p-0" id="home">
<nav class="navbar navbar-expand-lg navbar-light px-4 px-lg-5 py-3 py-lg-0">
<a href="" class="navbar-brand p-0">
<h1 class="m-0">Acent Services</h1>
<!-- <img src="img/logo.png" alt="Logo"> -->
</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarCollapse">
<span class="fa fa-bars"></span>
</button>
<div class="collapse navbar-collapse" id="navbarCollapse">
<div class="navbar-nav mx-auto py-0">
<a href="#home" class="nav-item nav-link active">Home</a>
<a href="#about" class="nav-item nav-link">About</a>
<a href="#feature" class="nav-item nav-link">Services</a>
<a href="#careers" class="nav-item nav-link">Careers</a>
<a href="#pricing" class="nav-item nav-link">Pricing</a>
<a href="#review" class="nav-item nav-link">Review</a>
<a href="#contact" class="nav-item nav-link">Contact</a>
</div>
<a href="" class="btn btn-primary-gradient rounded-pill py-2 px-4 ms-3 d-none d-lg-block">Technical
Support</a>
</div>
</nav>
<div class="container-xxl bg-primary hero-header">
<div class="container px-lg-5">
<div class="row g-5">
<div class="col-lg-8 text-center text-lg-start">
<h1 class="text-white mb-4 animated slideInDown">Providing exceptional support with every
call, 24/7.</h1>
<p class="text-white pb-3 animated slideInDown">Our call center operates around the clock to
ensure that every customer receives prompt, professional, and personalized assistance,
reinforcing our commitment to exceptional support.</p>
<a href="#about"
class="btn btn-primary-gradient py-sm-3 px-4 px-sm-5 rounded-pill me-3 animated slideInLeft">Read
More</a>
<a href=""
class="btn btn-secondary-gradient py-sm-3 px-4 px-sm-5 rounded-pill animated slideInRight">Contact
Us</a>
</div>
<div class="col-lg-4 d-flex justify-content-center justify-content-lg-end wow fadeInUp"
data-wow-delay="0.3s">
<div class="owl-carousel screenshot-carousel">
<img class="img-fluid" src="./img/1.jpg" alt="">
<img class="img-fluid" src="./img/2.jpg" alt="">
<img class="img-fluid" src="./img/3.jpg" alt="">
</div>
</div>
</div>
</div>
</div>
</div>
<!-- Navbar & Hero End -->
<!-- About Start -->
<div class="container-xxl py-5" id="about">
<div class="container py-5 px-lg-5">
<div class="row g-5 align-items-center">
<div class="col-lg-6 wow fadeInUp" data-wow-delay="0.1s">
<h5 class="text-primary-gradient fw-medium">About Us</h5>
<h1 class="mb-4">#1 For Excellence in every call, day and night.</h1>
<p class="mb-4">
Lorem ipsum dolor sit, amet consectetur adipisicing elit. Repudiandae voluptate harum
numquam corporis, ipsa hic mollitia libero cum fuga maiores ipsum, reiciendis voluptates
architecto doloribus dolor modi omnis quo vitae.<br />
Lorem ipsum dolor sit, amet consectetur adipisicing elit. Repudiandae voluptate harum
numquam corporis, ipsa hic mollitia libero cum fuga maiores ipsum, reiciendis voluptates
architecto doloribus dolor modi omnis quo vitae. reiciendis voluptates
architecto doloribus dolor modi omnis quo vitae.
</p>
<div class="row g-4 mb-4">
<div class="col-sm-6 wow fadeIn" data-wow-delay="0.7s">
<div class="d-flex">
<i class="fa fa-comments fa-2x text-secondary-gradient flex-shrink-0 mt-1"></i>
<div class="ms-3">
<h2 class="mb-0" data-toggle="counter-up">1234</h2>
<p class="text-secondary-gradient mb-0">Clients Reviews</p>
</div>
</div>
</div>
</div>
<a href="" class="btn btn-primary-gradient py-sm-3 px-4 px-sm-5 rounded-pill mt-3">Read More</a>
</div>
<div class="col-lg-6">
<img class="img-fluid wow fadeInUp" data-wow-delay="0.5s"
src="https://blogimages.softwaresuggest.com/blog/wp-content/uploads/2021/03/14170730/How-To-Setup-A-Call-Center-A-Comprehensive-Guide.jpg">
</div>
</div>
</div>
</div>
<!-- About End -->
<!-- Features Start -->
<div class="container-xxl py-5" id="feature">
<div class="container py-5 px-lg-5">
<div class="text-center wow fadeInUp" data-wow-delay="0.1s">
<h5 class="text-primary-gradient fw-medium">Our Services</h5>
<h1 class="mb-5">Awesome Services</h1>
</div>
<div class="row g-4">
<div class="col-lg-4 col-md-6 wow fadeInUp" data-wow-delay="0.1s">
<div class="feature-item bg-light rounded p-4">
<div class="d-inline-flex align-items-center justify-content-center bg-primary-gradient rounded-circle mb-4"
style="width: 60px; height: 60px;">
<i class="fa fa-eye text-white fs-4"></i>
</div>
<h5 class="mb-3">Multichannel Support</h5>
<p class="m-0">Erat ipsum justo amet duo et elitr dolor, est duo duo eos lorem sed diam stet
diam sed stet lorem.</p>
</div>
</div>
<div class="col-lg-4 col-md-6 wow fadeInUp" data-wow-delay="0.3s">
<div class="feature-item bg-light rounded p-4">
<div class="d-inline-flex align-items-center justify-content-center bg-secondary-gradient rounded-circle mb-4"
style="width: 60px; height: 60px;">
<i class="fa fa-layer-group text-white fs-4"></i>
</div>
<h5 class="mb-3">Interactive Voice Response</h5>
<p class="m-0">Erat ipsum justo amet duo et elitr dolor, est duo duo eos lorem sed diam stet
diam sed stet lorem.</p>
</div>
</div>
<div class="col-lg-4 col-md-6 wow fadeInUp" data-wow-delay="0.5s">
<div class="feature-item bg-light rounded p-4">
<div class="d-inline-flex align-items-center justify-content-center bg-primary-gradient rounded-circle mb-4"
style="width: 60px; height: 60px;">
<i class="fa fa-edit text-white fs-4"></i>
</div>
<h5 class="mb-3">Automatic Call Distribution</h5>
<p class="m-0">Erat ipsum justo amet duo et elitr dolor, est duo duo eos lorem sed diam stet
diam sed stet lorem.</p>
</div>
</div>
<div class="col-lg-4 col-md-6 wow fadeInUp" data-wow-delay="0.1s">
<div class="feature-item bg-light rounded p-4">
<div class="d-inline-flex align-items-center justify-content-center bg-secondary-gradient rounded-circle mb-4"
style="width: 60px; height: 60px;">
<i class="fa fa-shield-alt text-white fs-4"></i>
</div>
<h5 class="mb-3">Call Recording and Monitoring</h5>
<p class="m-0">Erat ipsum justo amet duo et elitr dolor, est duo duo eos lorem sed diam stet
diam sed stet lorem.</p>
</div>
</div>
<div class="col-lg-4 col-md-6 wow fadeInUp" data-wow-delay="0.3s">
<div class="feature-item bg-light rounded p-4">
<div class="d-inline-flex align-items-center justify-content-center bg-primary-gradient rounded-circle mb-4"
style="width: 60px; height: 60px;">
<i class="fa fa-cloud text-white fs-4"></i>
</div>
<h5 class="mb-3">Workforce Management</h5>
<p class="m-0">Erat ipsum justo amet duo et elitr dolor, est duo duo eos lorem sed diam stet
diam sed stet lorem.</p>
</div>
</div>
<div class="col-lg-4 col-md-6 wow fadeInUp" data-wow-delay="0.5s">
<div class="feature-item bg-light rounded p-4">
<div class="d-inline-flex align-items-center justify-content-center bg-secondary-gradient rounded-circle mb-4"
style="width: 60px; height: 60px;">
<i class="fa fa-mobile-alt text-white fs-4"></i>
</div>
<h5 class="mb-3">Queue Management</h5>
<p class="m-0">Erat ipsum justo amet duo et elitr dolor, est duo duo eos lorem sed diam stet
diam sed stet lorem.</p>
</div>
</div>
</div>
</div>
</div>
<!-- Features End -->
<!-- careers Start -->
<div class="container-xxl py-5" id="careers">
<div class="container py-5 px-lg-5">
<div class="row g-5 align-items-center">
<div class="col-lg-4 d-flex justify-content-center justify-content-lg-end wow fadeInUp"
data-wow-delay="0.3s">
<div class="owl-carousel screenshot-carousel">
<img class="img-fluid" src="img/c1.jpg" alt="">
<img class="img-fluid" src="img/c2.jpg" alt="">
<img class="img-fluid" src="img/c3.jpg" alt="">
</div>
</div>
<div class="col-lg-8 wow fadeInUp" data-wow-delay="0.1s">
<h5 class="text-primary-gradient fw-medium">Careers</h5>
<h1 class="mb-4">Careers in Customer Excellence</h1>
<p class="mb-4">Embark on a rewarding career in our call center, where your communication skills
and dedication to excellence are valued and nurtured. Here, you'll have the chance to grow
professionally, tackle new challenges, and be a vital part of a team committed to delivering
exceptional customer experiences. With continuous training and development opportunities, we
empower you to achieve your full potential and advance your career in a dynamic, supportive
environment.</p>
<p><i class="fa fa-check text-primary-gradient me-3"></i>Professional Growth</p>
<p><i class="fa fa-check text-primary-gradient me-3"></i>Supportive Work Environment</p>
<p class="mb-4"><i class="fa fa-check text-primary-gradient me-3"></i>Competitive Compensation
</p>
<a href="" class="btn btn-primary-gradient py-sm-3 px-4 px-sm-5 rounded-pill mt-3">View All</a>
</div>
</div>
</div>
</div>
<!-- careers End -->
<!-- Pricing Start -->
<div class="container-xxl py-5" id="pricing">
<div class="container py-5 px-lg-5">
<div class="text-center wow fadeInUp" data-wow-delay="0.1s">
<h5 class="text-primary-gradient fw-medium">Pricing Plan</h5>
<h1 class="mb-5">Choose Your Plan</h1>
</div>
<div class="tab-class text-center pricing wow fadeInUp" data-wow-delay="0.1s">
<ul
class="nav nav-pills d-inline-flex justify-content-center bg-primary-gradient rounded-pill mb-5">
<li class="nav-item">
<button class="nav-link active" data-bs-toggle="pill" href="#tab-1">Monthly</button>
</li>
<li class="nav-item">
<button class="nav-link" data-bs-toggle="pill" href="#tab-2">Yearly</button>
</li>
</ul>
<div class="tab-content text-start">
<div id="tab-1" class="tab-pane fade show p-0 active">
<div class="row g-4">
<div class="col-lg-4">
<div class="bg-light rounded">
<div class="border-bottom p-4 mb-4">
<h4 class="text-primary-gradient mb-1">Starter Plan</h4>
<span>Powerful & Awesome Features</span>
</div>
<div class="p-4 pt-0">
<h1 class="mb-3">
<small class="align-top"
style="font-size: 22px; line-height: 45px;">$</small>14.99<small
class="align-bottom" style="font-size: 16px; line-height: 40px;">/
Month</small>
</h1>
<div class="d-flex justify-content-between mb-3"><span>Lorem ipsum dolor,
sit amet </span><i
class="fa fa-check text-primary-gradient pt-1"></i></div>
<div class="d-flex justify-content-between mb-3"><span>Corrupti eligendi
totam</span><i class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<div class="d-flex justify-content-between mb-3"><span>Corrupti eligendi
totam est</span><i
class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<div class="d-flex justify-content-between mb-2"><span>Lorem ipsum
dolor</span><i class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<a href="" class="btn btn-primary-gradient rounded-pill py-2 px-4 mt-4">Get
Started</a>
</div>
</div>
</div>
<div class="col-lg-4">
<div class="bg-light rounded border">
<div class="border-bottom p-4 mb-4">
<h4 class="text-primary-gradient mb-1">Advance Plan</h4>
<span>Powerful & Awesome Features</span>
</div>
<div class="p-4 pt-0">
<h1 class="mb-3">
<small class="align-top"
style="font-size: 22px; line-height: 45px;">$</small>24.99<small
class="align-bottom" style="font-size: 16px; line-height: 40px;">/
Month</small>
</h1>
<div class="d-flex justify-content-between mb-3"><span>Lorem ipsum dolor,
sit amet </span><i
class="fa fa-check text-primary-gradient pt-1"></i></div>
<div class="d-flex justify-content-between mb-3"><span>Corrupti eligendi
totam</span><i class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<div class="d-flex justify-content-between mb-3"><span>Corrupti eligendi
totam est</span><i
class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<div class="d-flex justify-content-between mb-2"><span>Lorem ipsum
dolor</span><i class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<a href="" class="btn btn-primary-gradient rounded-pill py-2 px-4 mt-4">Get
Started</a>
</div>
</div>
</div>
<div class="col-lg-4">
<div class="bg-light rounded">
<div class="border-bottom p-4 mb-4">
<h4 class="text-primary-gradient mb-1">Premium Plan</h4>
<span>Powerful & Awesome Features</span>
</div>
<div class="p-4 pt-0">
<h1 class="mb-3">
<small class="align-top"
style="font-size: 22px; line-height: 45px;">$</small>34.99<small
class="align-bottom" style="font-size: 16px; line-height: 40px;">/
Month</small>
</h1>
<div class="d-flex justify-content-between mb-3"><span>Lorem ipsum dolor,
sit amet </span><i
class="fa fa-check text-primary-gradient pt-1"></i></div>
<div class="d-flex justify-content-between mb-3"><span>Corrupti eligendi
totam</span><i class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<div class="d-flex justify-content-between mb-3"><span>Corrupti eligendi
totam est</span><i
class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<div class="d-flex justify-content-between mb-2"><span>Lorem ipsum
dolor</span><i class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<a href="" class="btn btn-primary-gradient rounded-pill py-2 px-4 mt-4">Get
Started</a>
</div>
</div>
</div>
</div>
</div>
<div id="tab-2" class="tab-pane fade p-0">
<div class="row g-4">
<div class="col-lg-4">
<div class="bg-light rounded">
<div class="border-bottom p-4 mb-4">
<h4 class="text-primary-gradient mb-1">Starter Plan</h4>
<span>Powerful & Awesome Features</span>
</div>
<div class="p-4 pt-0">
<h1 class="mb-3">
<small class="align-top"
style="font-size: 22px; line-height: 45px;">$</small>114.99<small
class="align-bottom" style="font-size: 16px; line-height: 40px;">/
Yearly</small>
</h1>
<div class="d-flex justify-content-between mb-3"><span>Lorem ipsum dolor,
sit amet </span><i
class="fa fa-check text-primary-gradient pt-1"></i></div>
<div class="d-flex justify-content-between mb-3"><span>Corrupti eligendi
totam</span><i class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<div class="d-flex justify-content-between mb-3"><span>Corrupti eligendi
totam est</span><i
class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<div class="d-flex justify-content-between mb-2"><span>Lorem ipsum
dolor</span><i class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<a href="" class="btn btn-primary-gradient rounded-pill py-2 px-4 mt-4">Get
Started</a>
</div>
</div>
</div>
<div class="col-lg-4">
<div class="bg-light rounded border">
<div class="border-bottom p-4 mb-4">
<h4 class="text-primary-gradient mb-1">Advance Plan</h4>
<span>Powerful & Awesome Features</span>
</div>
<div class="p-4 pt-0">
<h1 class="mb-3">
<small class="align-top"
style="font-size: 22px; line-height: 45px;">$</small>124.99<small
class="align-bottom" style="font-size: 16px; line-height: 40px;">/
Yearly</small>
</h1>
<div class="d-flex justify-content-between mb-3"><span>Lorem ipsum dolor,
sit amet </span><i
class="fa fa-check text-primary-gradient pt-1"></i></div>
<div class="d-flex justify-content-between mb-3"><span>Corrupti eligendi
totam</span><i class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<div class="d-flex justify-content-between mb-3"><span>Corrupti eligendi
totam est</span><i
class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<div class="d-flex justify-content-between mb-2"><span>Lorem ipsum
dolor</span><i class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<a href="" class="btn btn-primary-gradient rounded-pill py-2 px-4 mt-4">Get
Started</a>
</div>
</div>
</div>
<div class="col-lg-4">
<div class="bg-light rounded">
<div class="border-bottom p-4 mb-4">
<h4 class="text-primary-gradient mb-1">Premium Plan</h4>
<span>Powerful & Awesome Features</span>
</div>
<div class="p-4 pt-0">
<h1 class="mb-3">
<small class="align-top"
style="font-size: 22px; line-height: 45px;">$</small>134.99<small
class="align-bottom" style="font-size: 16px; line-height: 40px;">/
Yearly</small>
</h1>
<div class="d-flex justify-content-between mb-3"><span>Lorem ipsum dolor,
sit amet </span><i
class="fa fa-check text-primary-gradient pt-1"></i></div>
<div class="d-flex justify-content-between mb-3"><span>Corrupti eligendi
totam</span><i class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<div class="d-flex justify-content-between mb-3"><span>Corrupti eligendi
totam est</span><i
class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<div class="d-flex justify-content-between mb-2"><span>Lorem ipsum
dolor</span><i class="fa fa-check text-primary-gradient pt-1"></i>
</div>
<a href="" class="btn btn-primary-gradient rounded-pill py-2 px-4 mt-4">Get
Started</a>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- Pricing End -->
<!-- Testimonial Start -->
<div class="container-xxl py-5" id="review">
<div class="container py-5 px-lg-5">
<div class="text-center wow fadeInUp" data-wow-delay="0.1s">
<h5 class="text-primary-gradient fw-medium">Testimonial</h5>
<h1 class="mb-5">What Say Our Clients!</h1>
</div>
<div class="owl-carousel testimonial-carousel wow fadeInUp" data-wow-delay="0.1s">
<div class="testimonial-item rounded p-4">
<div class="d-flex align-items-center mb-4">
<img class="img-fluid bg-white rounded flex-shrink-0 p-1" src="img/cl.jpg"
style="width: 85px; height: 85px;">
<div class="ms-4">
<h5 class="mb-1">Client Name</h5>
<p class="mb-1">Profession</p>
<div>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
</div>
</div>
</div>
<p class="mb-0">Tempor erat elitr rebum at clita. Diam dolor diam ipsum sit diam amet diam et
eos. Clita erat ipsum et lorem et sit.</p>
</div>
<div class="testimonial-item rounded p-4">
<div class="d-flex align-items-center mb-4">
<img class="img-fluid bg-white rounded flex-shrink-0 p-1" src="img/cl.jpg"
style="width: 85px; height: 85px;">
<div class="ms-4">
<h5 class="mb-1">Client Name</h5>
<p class="mb-1">Profession</p>
<div>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
</div>
</div>
</div>
<p class="mb-0">Tempor erat elitr rebum at clita. Diam dolor diam ipsum sit diam amet diam et
eos. Clita erat ipsum et lorem et sit.</p>
</div>
<div class="testimonial-item rounded p-4">
<div class="d-flex align-items-center mb-4">
<img class="img-fluid bg-white rounded flex-shrink-0 p-1" src="img/cl.jpg"
style="width: 85px; height: 85px;">
<div class="ms-4">
<h5 class="mb-1">Client Name</h5>
<p class="mb-1">Profession</p>
<div>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
</div>
</div>
</div>
<p class="mb-0">Tempor erat elitr rebum at clita. Diam dolor diam ipsum sit diam amet diam et
eos. Clita erat ipsum et lorem et sit.</p>
</div>
<div class="testimonial-item rounded p-4">
<div class="d-flex align-items-center mb-4">
<img class="img-fluid bg-white rounded flex-shrink-0 p-1" src="img/cl.jpg"
style="width: 85px; height: 85px;">
<div class="ms-4">
<h5 class="mb-1">Client Name</h5>
<p class="mb-1">Profession</p>
<div>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
<small class="fa fa-star text-warning"></small>
</div>
</div>
</div>
<p class="mb-0">Tempor erat elitr rebum at clita. Diam dolor diam ipsum sit diam amet diam et
eos. Clita erat ipsum et lorem et sit.</p>
</div>
</div>
</div>
</div>
<!-- Testimonial End -->
<!-- Contact Start -->
<div class="container-xxl py-5" id="contact">
<div class="container py-5 px-lg-5">
<div class="text-center wow fadeInUp" data-wow-delay="0.1s">
<h5 class="text-primary-gradient fw-medium">Contact Us</h5>
<h1 class="mb-5">Get In Touch!</h1>
</div>
<div class="row justify-content-center">
<div class="col-lg-9">
<div class="wow fadeInUp" data-wow-delay="0.3s">
<p class="text-center mb-4">Get in touch with us – we're here to help, anytime you need. Our
dedicated team is ready to provide prompt and professional assistance, ensuring your
questions are answered and your needs are met.</p>
<form>
<div class="row g-3">
<div class="col-md-6">
<div class="form-floating">
<input type="text" class="form-control" id="name" placeholder="Your Name">
<label for="name">Your Name</label>
</div>
</div>
<div class="col-md-6">
<div class="form-floating">
<input type="email" class="form-control" id="email"
placeholder="Your Email">
<label for="email">Your Email</label>
</div>
</div>
<div class="col-12">
<div class="form-floating">
<input type="text" class="form-control" id="subject" placeholder="Subject">
<label for="subject">Subject</label>
</div>
</div>
<div class="col-12">
<div class="form-floating">
<textarea class="form-control" placeholder="Leave a message here"
id="message" style="height: 150px"></textarea>
<label for="message">Message</label>
</div>
</div>
<div class="col-12 text-center">
<button class="btn btn-primary-gradient rounded-pill py-3 px-5"
type="submit">Send Message</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
<!-- Contact End -->
<!-- Footer Start -->
<div class="container-fluid bg-primary text-light footer wow fadeIn" data-wow-delay="0.1s">
<div class="container py-5 px-lg-5">
<div class="row g-5">
<div class="col-md-6 col-lg-3">
<h4 class="text-white mb-4">Address</h4>
<p><i class="fa fa-map-marker-alt me-3"></i>123 Street, Colombo, Sri Lanka</p>
<p><i class="fa fa-phone-alt me-3"></i>+94 76 57 56 576</p>
<p><i class="fa fa-envelope me-3"></i>[email protected]</p>
<div class="d-flex pt-2">
<a class="btn btn-outline-light btn-social" href=""><i class="fab fa-twitter"></i></a>
<a class="btn btn-outline-light btn-social" href=""><i class="fab fa-facebook-f"></i></a>
<a class="btn btn-outline-light btn-social" href=""><i class="fab fa-instagram"></i></a>
<a class="btn btn-outline-light btn-social" href=""><i class="fab fa-linkedin-in"></i></a>
</div>
</div>
<div class="col-md-6 col-lg-3">
<h4 class="text-white mb-4">Quick Link</h4>
<a class="btn btn-link" href="">About Us</a>
<a class="btn btn-link" href="">Contact Us</a>
<a class="btn btn-link" href="">Privacy Policy</a>
<a class="btn btn-link" href="">Terms & Condition</a>
<a class="btn btn-link" href="">Career</a>
</div>
<div class="col-md-6 col-lg-3">
<h4 class="text-white mb-4">Popular Link</h4>
<a class="btn btn-link" href="">About Us</a>
<a class="btn btn-link" href="">Contact Us</a>
<a class="btn btn-link" href="">Privacy Policy</a>
<a class="btn btn-link" href="">Terms & Condition</a>
<a class="btn btn-link" href="">Career</a>
</div>
<div class="col-md-6 col-lg-3">
<h4 class="text-white mb-4">Newsletter</h4>
<p>Lorem ipsum dolor sit amet elit. Phasellus nec pretium mi. Curabitur facilisis ornare velit
non vulpu</p>
<div class="position-relative w-100 mt-3">
<input class="form-control border-0 rounded-pill w-100 ps-4 pe-5" type="text"
placeholder="Your Email" style="height: 48px;">
<button type="button" class="btn shadow-none position-absolute top-0 end-0 mt-1 me-2"><i
class="fa fa-paper-plane text-primary-gradient fs-4"></i></button>
</div>
</div>
</div>
</div>
<div class="container px-lg-5">
<div class="copyright">
<div class="row">
<div class="col-md-6 text-center text-md-start mb-3 mb-md-0">
© <a class="border-bottom" href="#">Acent Services</a>, All Right Reserved.
<!--/*** This template is free as long as you keep the footer author’s credit link/attribution link/backlink. If you'd like to use the template without the footer author’s credit link/attribution link/backlink, you can purchase the Credit Removal License from "https://htmlcodex.com/credit-removal". Thank you for your support. ***/-->
Designed By <a class="border-bottom" href="https://cipherlabz.com">Cipher Labz (Pvt) Ltd</a>
</div>
<div class="col-md-6 text-center text-md-end">
<div class="footer-menu">
<a href="">Home</a>
<a href="">Cookies</a>
<a href="">Help</a>
<a href="">FQAs</a>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- Footer End -->
<!-- Back to Top -->
<a href="#" class="btn btn-lg btn-lg-square back-to-top pt-2"><i class="bi bi-arrow-up text-white"></i></a>
</div>
<!-- JavaScript Libraries -->
<script src="https://code.jquery.com/jquery-3.4.1.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
<script src="lib/wow/wow.min.js"></script>
<script src="lib/easing/easing.min.js"></script>
<script src="lib/waypoints/waypoints.min.js"></script>
<script src="lib/counterup/counterup.min.js"></script>
<script src="lib/owlcarousel/owl.carousel.min.js"></script>
<!-- Template Javascript -->
<script src="js/main.js"></script>
</body>
</html> |
import { Box, Checkbox, FormControlLabel, Typography } from "@mui/material";
import PropTypes from "prop-types";
import React from "react";
const FilterByService = ({ filters = {}, onChange }) => {
// const [values, setValues] = useState({
// isPromotion: Boolean(filters.isPromotion),
// isFreeShip: Boolean(filters.isFreeShip),
// });
const handleChange = (e) => {
if (!onChange) return;
const { name, checked } = e.target;
// setValues((prevValues) => ({
// ...prevValues,
// [name]: [checked],
// }));
if (onChange) onChange({ [name]: checked });
};
return (
<Box
sx={{
padding: 2,
borderTop: `1px solid gray`,
}}>
<Typography variant="subtitle2">Service</Typography>
<ul>
{[
{ value: "isPromotion", label: "Sale Off" },
{ value: "isFreeShip", label: "Delivery" },
].map((service) => (
<li key={service.value}>
<FormControlLabel
control={
<Checkbox
checked={Boolean(filters[service.value])}
onChange={handleChange}
name={service.value}
color="primary"
/>
}
label={service.label}
/>
</li>
))}
</ul>
</Box>
);
};
FilterByService.propTypes = {
onChange: PropTypes.func,
filters: PropTypes.object,
};
export default FilterByService; |
require_relative '../../spec_helper'
require_relative 'fixtures/common'
require_relative 'shared/new'
describe "Exception.exception" do
it_behaves_like :exception_new, :exception
end
describe "Exception#exception" do
it "returns self when passed no argument" do
e = RuntimeError.new
e.should == e.exception
end
it "returns self when passed self as an argument" do
e = RuntimeError.new
e.should == e.exception(e)
end
it "returns an exception of the same class as self with the message given as argument" do
e = RuntimeError.new
e2 = e.exception("message")
e2.should be_an_instance_of(RuntimeError)
e2.message.should == "message"
end
it "when raised will be rescued as the new exception" do
begin
begin
raised_first = StandardError.new('first')
raise raised_first
rescue => caught_first
raised_second = raised_first.exception('second')
raise raised_second
end
rescue => caught_second
end
raised_first.should == caught_first
raised_second.should == caught_second
end
it "captures an exception into $!" do
exception = begin
raise
rescue RuntimeError
$!
end
exception.class.should == RuntimeError
exception.message.should == ""
exception.backtrace.first.should =~ /exception_spec/
end
class CustomArgumentError < StandardError
attr_reader :val
def initialize(val)
@val = val
end
end
it "returns an exception of the same class as self with the message given as argument, but without reinitializing" do
e = CustomArgumentError.new(:boom)
e2 = e.exception("message")
e2.should be_an_instance_of(CustomArgumentError)
e2.val.should == :boom
e2.message.should == "message"
end
end |
package es.upm.btb.helloworldkt
import android.location.Location
import android.os.Bundle
import android.util.Log
import android.widget.Button
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
import androidx.core.content.ContextCompat
import org.osmdroid.config.Configuration
import org.osmdroid.tileprovider.tilesource.TileSourceFactory
import org.osmdroid.util.GeoPoint
import org.osmdroid.views.MapView
import org.osmdroid.views.overlay.Marker
import org.osmdroid.views.overlay.Polyline
class OpenStreetMapActivity : AppCompatActivity() {
private val TAG = "btaOpenStreetMapActivity"
private lateinit var map: MapView
val parkingCoordinates = listOf(
GeoPoint(40.38323792632855, -3.783621006318095), // Aviación Española
GeoPoint(40.434154, -3.598831), // Estadio Metropolitano Sur
GeoPoint(40.4844428688153, -3.66450251273440), // Fuente de la Mora
GeoPoint(40.36521668890186, -3.737923481612698), // Islazul
GeoPoint( 40.4941929509737, -3.72723600089653), // Pitis
GeoPoint(40.428193768950756, -3.673778093084516), // Alcántara
GeoPoint(40.426273740022, -3.70037418702346), // Arquitecto Ribera
GeoPoint(40.4381779662151, -3.67683406459837), // Avenida de América
GeoPoint(40.4112391063366, -3.73808787568741), // Avenida de Portugal
GeoPoint(40.40709468568204, -3.7038875277131464), // Casino de la Reina
GeoPoint(40.4242207643363, -3.67342185936768), // Felipe II
GeoPoint(40.4296270729669, -3.70279096159205), //F uencarral
GeoPoint(40.41840695632423, -3.7119903936183274), // Plaza de Oriente
GeoPoint(40.4158677606471, -3.70689561392158), // Plaza Mayor
GeoPoint(40.408797321706984, -3.6763215894089827), // Reyes Magos
GeoPoint(40.4329452991722, -3.68611338542774), // Serrano I
GeoPoint(40.4303442290635, -3.68850808858792), // Serrano II
GeoPoint(40.4244268291578, -3.68965177313431), // Serrano III
GeoPoint(40.41777541448619, -3.7002800119895505), // Sevilla
GeoPoint(40.4272702030791, -3.68408892366904), // Velázquez - Ayala
GeoPoint(40.4298019274054, -3.69323812827144), // Almagro
GeoPoint(40.44429510303696, -3.6776974842015506), // Auditorio Nacional de Música
GeoPoint(40.41845756968, -3.70704603703524), // Descalzas
GeoPoint(40.407386591674,-3.71001515747525), // Glorieta Puerta de Toledo
GeoPoint(40.4442297702559,-3.67765536310739), // Museo de la Ciudad
GeoPoint(40.4254346221439,-3.6884680270103), // Plaza de Colón
GeoPoint(40.4237811996075,-3.71152321262521), // Plaza de España
GeoPoint(40.4145312089554,-3.70056009896723), // Santa Ana
GeoPoint(40.38728599111143,-3.7063081042559407) //Usera
)
val parkingNames = listOf(
"Aparcamiento disuasorio Aviación Española",
"Aparcamiento disuasorio Estadio Metropolitano Sur",
"Aparcamiento disuasorio Fuente de la Mora",
"Aparcamiento disuasorio Islazul",
"Aparcamiento disuasorio Pitis",
"Aparcamiento mixto. Alcántara",
"Aparcamiento mixto. Arquitecto Ribera",
"Aparcamiento mixto. Avenida de América (intercambiador)",
"Aparcamiento mixto. Avenida de Portugal",
"Aparcamiento mixto. Casino de la Reina",
"Aparcamiento mixto. Felipe II",
"Aparcamiento mixto. Fuencarral",
"Aparcamiento mixto. Plaza de Oriente",
"Aparcamiento mixto. Plaza Mayor",
"Aparcamiento mixto. Reyes Magos",
"Aparcamiento mixto. Serrano I",
"Aparcamiento mixto. Serrano II",
"Aparcamiento mixto. Serrano III",
"Aparcamiento mixto. Sevilla",
"Aparcamiento mixto. Velázquez - Ayala",
"Aparcamiento público. Almagro",
"Aparcamiento público. Auditorio Nacional de Música (Príncipe de Vergara)",
"Aparcamiento público. Descalzas",
"Aparcamiento público. Glorieta Puerta de Toledo",
"Aparcamiento público. Museo de la Ciudad",
"Aparcamiento público. Plaza de Colón",
"Aparcamiento público. Plaza de España",
"Aparcamiento público. Santa Ana",
"Aparcamiento público. Usera"
)
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_open_street_map)
Log.d(TAG, "onCreate: The activity is being created.");
val bundle = intent.getBundleExtra("locationBundle")
val location: Location? = bundle?.getParcelable("location")
if (location != null) {
Log.i(TAG, "onCreate: Location["+location.altitude+"]["+location.latitude+"]["+location.longitude+"][")
Configuration.getInstance().load(applicationContext, getSharedPreferences("osm", MODE_PRIVATE))
map = findViewById(R.id.map)
map.setTileSource(TileSourceFactory.MAPNIK)
map.controller.setZoom(18.0)
val startPoint = GeoPoint(location.latitude, location.longitude)
map.controller.setCenter(startPoint)
addMarker(startPoint, "My current location")
addMarkers(map,parkingCoordinates,parkingNames)
//addMarkersAndRoute(map, parkingCoordinates, parkingNames)
}
val nearestParkingButton: Button = findViewById(R.id.nearestParkingButton)
nearestParkingButton.setOnClickListener {
location?.let {
calculateDistancesToParkings(it.latitude, it.longitude)
}
}
}
private fun calculateDistancesToParkings(userLat: Double, userLon: Double) {
val distances = mutableListOf<Pair<String, Double>>()
for (i in parkingCoordinates.indices) {
val parkingLat = parkingCoordinates[i].latitude
val parkingLon = parkingCoordinates[i].longitude
val distance = calculateEuclideanDistance(userLat, userLon, parkingLat, parkingLon)
distances.add(Pair(parkingNames[i], distance))
}
distances.sortBy { it.second } // Ordenar por distancia
val nearestParking = distances.firstOrNull()
nearestParking?.let {
Log.d(TAG, "Nearest Parking: ${it.first} at distance ${it.second}")
Toast.makeText(this, "Nearest Parking: ${it.first}", Toast.LENGTH_LONG).show()
}
}
private fun calculateEuclideanDistance(lat1: Double, lon1: Double, lat2: Double, lon2: Double): Double {
return Math.sqrt(Math.pow(lat2 - lat1, 2.0) + Math.pow(lon2 - lon1, 2.0))
}
private fun addMarker(point: GeoPoint, title: String) {
val marker = Marker(map)
marker.position = point
marker.setAnchor(Marker.ANCHOR_CENTER, Marker.ANCHOR_BOTTOM)
marker.title = title
map.overlays.add(marker)
map.invalidate() // Reload map
marker.icon = ContextCompat.getDrawable(this, R.drawable.location)
map.overlays.add(marker)
}
fun addMarkers(mapView: MapView, locationsCoords: List<GeoPoint>, locationsNames: List<String>) {
for (location in locationsCoords) {
val marker = Marker(mapView)
marker.position = location
marker.setAnchor(Marker.ANCHOR_CENTER, Marker.ANCHOR_BOTTOM)
marker.title = "Marker at ${locationsNames.get(locationsCoords.indexOf(location))} ${location.latitude}, ${location.longitude}"
marker.icon = ContextCompat.getDrawable(this, R.drawable.marker)
mapView.overlays.add(marker)
}
mapView.invalidate() // Refresh the map to display the new markers
}
//define route
fun addMarkersAndRoute(mapView: MapView, locationsCoords: List<GeoPoint>, locationsNames: List<String>) {
if (locationsCoords.size != locationsNames.size) {
Log.e("addMarkersAndRoute", "Locations and names lists must have the same number of items.")
return
}
val route = Polyline()
route.setPoints(locationsCoords)
route.color = ContextCompat.getColor(this, R.color.teal_700)
mapView.overlays.add(route)
for (location in locationsCoords) {
val marker = Marker(mapView)
marker.position = location
marker.setAnchor(Marker.ANCHOR_CENTER, Marker.ANCHOR_BOTTOM)
val locationIndex = locationsCoords.indexOf(location)
marker.title = "Marker at ${locationsNames[locationIndex]} ${location.latitude}, ${location.longitude}"
marker.icon = ContextCompat.getDrawable(this,R.drawable.marker)
mapView.overlays.add(marker)
}
mapView.invalidate()
}
override fun onResume() {
super.onResume()
if (::map.isInitialized) {
map.onResume()
} else {
Log.e("OpenStreetMapActivity", "map no está inicializado")
}
}
override fun onPause() {
super.onPause()
map.onPause()
}
} |
package Tests;
import Page.AutocompletePage;
import Page.MainPage;
import org.junit.jupiter.api.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.interactions.Actions;
import java.util.List;
import java.util.concurrent.ThreadLocalRandom;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class AutocompleteTest extends TestBase {
MainPage mainPage = new MainPage();
AutocompletePage autocompletePage = new AutocompletePage();
@Test
public void autocompleteTest() {
driver.get("https://seleniumui.moderntester.pl/");
Actions action = new Actions(driver);
WebElement menuWidgets = driver.findElement(By.linkText(mainPage.menuWidgetsLinkText));
WebElement menuWidgetsAutocomplete = driver.findElement(By.id(mainPage.widgetsAutocompleteId));
action.moveToElement(menuWidgets).moveToElement(menuWidgetsAutocomplete).click().build().perform();
WebElement input = driver.findElement(By.id(autocompletePage.inputId));
input.sendKeys("a");
List<WebElement> resultsList = driver.findElements(By.cssSelector(autocompletePage.resultCssSelector));
for (WebElement result : resultsList) {
System.out.println(result.getText());
}
int randomNumber = ThreadLocalRandom.current().nextInt(0, resultsList.size());
String chosenValue = resultsList.get(randomNumber).getText();
resultsList.get(randomNumber).click();
assertEquals(chosenValue, driver.findElement(By.id(autocompletePage.inputId)).getAttribute("value"));
}
} |
import 'package:flutter/cupertino.dart';
import 'package:get/get.dart';
import 'package:proc01/controllers/progress_bar_timer_controller.dart';
import 'package:proc01/models/questions.dart';
import 'package:proc01/screens/score.dart';
import 'package:proc01/screens/welcome.dart';
class QuestionController extends GetxController
with SingleGetTickerProviderMixin {
ProgressBarTimerController timerController =
Get.put(ProgressBarTimerController());
late PageController _pageController;
List<Question> _questions = sample_data
.map((e) => Question(
id: e['id'],
question: e['question'],
answer: e['answer_index'],
options: e['options']))
.toList();
bool _isAnswered = false;
int _correctAns = 0;
int _selectedAns = 0;
RxInt _questionNumber = 1.obs;
int _numOfCorrectAns = 0;
PageController get pageController => this._pageController;
List<Question> get questions => this._questions;
bool get isAnswered => this._isAnswered;
int get correctAns => this._correctAns;
int get selectedAns => this._selectedAns;
RxInt get questionNumber => this._questionNumber;
int get numOfCorrectAns => this._numOfCorrectAns;
@override
void onInit() {
_pageController = PageController();
timerController.initListener(nextQuestion);
super.onInit();
}
@override
void onClose() {
_pageController.dispose();
super.onClose();
}
void checkAns(Question question, int selected) {
_isAnswered = true;
_correctAns = question.answer;
_selectedAns = selected;
if (_correctAns == _selectedAns) _numOfCorrectAns++;
timerController.stop();
update();
Future.delayed(Duration(seconds: 1), () {
_isAnswered = false;
nextQuestion();
});
}
void nextQuestion() {
if (_questionNumber.value != _questions.length) {
_isAnswered = false;
_pageController.nextPage(
duration: Duration(milliseconds: 200), curve: Curves.ease);
timerController.reset();
} else {
// Get package provide us simple way to naviigate another page
Get.to(ScoreScreen());
}
}
void updateTheQnNum(int index) {
_questionNumber.value = index + 1;
}
void newGame() {
_isAnswered = false;
_correctAns = 0;
_selectedAns = 0;
_questionNumber = 1.obs;
_numOfCorrectAns = 0;
_pageController = PageController();
timerController.newGame();
Get.to(WelcomeScreen());
}
} |
import { useState } from "react";
import { useHistory } from "react-router-dom";
import { newPost } from "../services";
import { TextField, Button } from "@mui/material";
const NewPost = () => {
const [title, setTitle] = useState("");
const [content, setContent] = useState("");
const history = useHistory();
const handleSubmit = async (e) => {
try {
e.preventDefault();
const post = {
title,
content,
};
await newPost(post);
history.push("/");
} catch (error) {
console.error(error.message);
}
};
return (
<section className="post-stop">
<h3 id="add-peeve-text">Add a Peeve!</h3>
<TextField
style={{
width: "500px",
["@media (max-width:480px)"]: {
width: "38px",
},
}}
id="new-title"
required
autoFocus
placeholder="Enter peeve title"
value={title}
onChange={(e) => setTitle(e.target.value)}
/>
<TextField
style={{
width: "500px",
}}
id="new-title"
required
autoFocus
placeholder="Tell us what peeves you off..."
value={content}
onChange={(e) => setContent(e.target.value)}
/>
<Button onClick={handleSubmit} variant="contained">
Post
</Button>
</section>
);
};
export default NewPost; |
import { useConfirmationDialog } from '@/contexts/ConfirmContext'
import ZenApi from '@/services/trader'
import { Box, Button, Typography } from '@material-ui/core'
import { grey } from '@material-ui/core/colors'
import { Alert } from '@material-ui/lab'
import moment from 'moment'
import React from 'react'
import { useDispatch } from 'react-redux'
import { toast } from 'react-toastify'
import FeatureUsageView from '../../../featureAndUsage/lists/FeatureUsageList'
import { usePlanDialog } from '../../../pricing/dialogs/PlanDialog'
import { getSubscriptions } from '../../slices/subscriptionSlice'
const SubscriptionManagementItem = ({ sub }) => {
const { showPlanDialog } = usePlanDialog()
const dispatch = useDispatch()
const { getConfirmation } = useConfirmationDialog()
const formatCycle = (billingPlan) => {
if (billingPlan?.cycle === 'monthly') {
return (
<>
Billed <b>₹{billingPlan?.price}</b> monthly
</>
)
}
if (billingPlan?.cycle === 'annual') {
return (
<>
Billed <b>₹{billingPlan?.price}</b> yearly
</>
)
}
}
const cancelPlan = async (subId) => {
try {
await ZenApi.Subscription.Subscription.cancel(subId)
toast.success('Your plan will cancel at the end date')
dispatch(getSubscriptions())
} catch (error) {
toast.error('There was an error cancelling your plan')
}
}
const undoCancelPlan = async (subId) => {
try {
await ZenApi.Subscription.Subscription.undoCancel(subId)
toast.success('Your cancellation has been reverted')
dispatch(getSubscriptions())
} catch (error) {
toast.error('There was an error undoing cancellation of your plan')
}
}
return (
<Box elevation={0} borderBottom={`1px solid ${grey[200]}`}>
<Box mb={0} display="flex" alignItems="flex-start" p={2}>
<Box>
<Typography variant="subtitle2">
{sub.plan.plan_group.name}
</Typography>
<Typography variant="h6" style={{ fontWeight: 700 }}>
{sub.plan.name}
</Typography>
<Typography variant="body2">
{formatCycle(sub.billing_plan)}
</Typography>
{sub.current_end && (
<Typography variant="caption">
{sub.cancel_at_period_end ? 'Cancels on' : 'Expires On'}:{' '}
{moment(sub.current_end).format('DD MMM YYYY')}
</Typography>
)}
</Box>
<Box ml="auto">
{sub.cancel_at_period_end ? (
<Button
variant="text"
size="small"
color="default"
onClick={() => {
undoCancelPlan(sub.id)
}}>
Undo Cancel
</Button>
) : (
!sub.complimentary &&
!sub.trial && (
<Button
variant="text"
size="small"
color="default"
onClick={async () => {
if (
await getConfirmation({
title: `Cancel plan?`,
message: `Are you sure you want to cancel this plan? The plan will be cancelled on ${moment(
sub.current_end
).format('DD MMM YYYY')}`,
btnConfirmText: 'Cancel'
})
) {
cancelPlan(sub.id)
}
}}>
Cancel
</Button>
)
)}
<Button
variant="text"
size="small"
color="primary"
onClick={() => {
showPlanDialog(sub.plan.plan_group.tag)
}}>
Change/Renew
</Button>
</Box>
</Box>
{sub.status === 'active' &&
(sub.trial || sub.complimentary) &&
sub.current_end && (
<Alert severity="info">
Your {sub.trial ? 'trial' : 'complimentary'} plan will expire{' '}
{moment(sub.current_end).fromNow()}
</Alert>
)}
<FeatureUsageView subscription={sub} />
</Box>
)
}
export default SubscriptionManagementItem |
function findClosestElements(arr: number[], k: number, x: number): number[] {
let left = 0;
let right = arr.length - 1;
const answer: number[] = [];
while (left <= right) {
let mid = left + Math.floor((right - left) / 2);
// ? Instead of finding the x position in the array, we can find the left boundary index of the of the k elements
// ? subarray closest to x.
// * (x - arr[mid]): for sure x element would be greater than it's left boundary since the array is sorted, so we
// * subtracted arr[mid] from x to catch the positive values.
// * (arr[mid + k] - x): and now x element would be smaller than it's right boundary since the array is sorted, so we
// * subtracted x from arr[mid + k] and added k to mid to get the right boundary.
if (x - arr[mid] > arr[mid + k] - x) {
left = mid + 1;
} else {
right = mid - 1;
}
}
// ? here we are adding the the search space to the result, and we used it like this to work with the least search space
// ? which is one element, since arr.slice(left, k) will fail in some edge cases!
for (let i = 0; i < k; i++) {
answer.push(arr[left + i]);
}
return answer;
}; |
import { BrowserModule } from '@angular/platform-browser';
import { NgModule, CUSTOM_ELEMENTS_SCHEMA } from '@angular/core';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { ServiceWorkerModule } from '@angular/service-worker';
import { environment } from '../environments/environment';
import { HttpClientModule } from '@angular/common/http';
import { JourneyService } from './core/services/history.service';
import { StoreStateModule } from './+state/store-state.module';
import { RouterModule } from '@angular/router';
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule.withServerTransition({ appId: 'serverApp' }),
AppRoutingModule,
HttpClientModule,
ServiceWorkerModule.register('ngsw-worker.js', {
enabled: environment.production
}),
StoreStateModule,
],
providers: [],
bootstrap: [AppComponent],
entryComponents: [],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
export class AppModule {
constructor(private service: JourneyService){
this.service.listenToRouter();
}
} |
import 'dart:convert';
import 'package:dio/dio.dart';
import 'package:tezda_ecom/domain/api/dio_client.dart';
import 'package:tezda_ecom/domain/api/endpoints.dart';
import '../../application/home/home_controller.dart';
import '../../domain/models/product/product.dart';
abstract class IProductRepo {
factory IProductRepo() = RepositoryImpl;
List<Product> getAllProducts();
List<String> getAllCategories();
List<Product> getCategoryProducts({required String category_ID});
Product getSingleProduct({required String product_ID});
}
class RepositoryImpl implements IProductRepo {
DioClient client = DioClient(Dio());
@override
getAllCategories() async {
var responce = await client.request(endPoint: EndPoint.getAllCategories);
List<String> categories = jsonDecode(responce.data).cast<String>();
print(categories);
HomeController.categories.value = categories;
return categories;
}
@override
getAllProducts() async {
var responce = await client.request(endPoint: EndPoint.getAllProducts);
final datas = ((jsonDecode(responce.data)) as List).map((e) {
return Product.fromJson(e);
}).toList();
print(datas[0].category.toString());
HomeController.products.value = datas;
return datas;
}
@override
getCategoryProducts({required String category_ID}) async {
var responce = await client.customrequest(
URL: "${EndPoint.getProductsInCategoryByID.url}/$category_ID",
endPoint: EndPoint.getProductsInCategoryByID);
final datas = ((jsonDecode(responce.data)) as List).map((e) {
return Product.fromJson(e);
}).toList();
HomeController.products.value = datas;
return datas;
}
@override
getSingleProduct({required String product_ID}) async {
var responce = await client.customrequest(
URL: "${EndPoint.getSingleProductByID.url}/$product_ID",
endPoint: EndPoint.getSingleProductByID);
var product = Product.fromJson(jsonDecode(responce.data));
HomeController.product.value = product;
print(product.title);
return product;
}
} |
import React from "react";
import { Modal, Button } from "react-bootstrap";
import { getIndexFromValuta, getVrijednostiFromDb } from "../Utility/DistributivnaMreza";
import { dajIndexZaVrijednost, dajNizZaValutu, dajVrijednostiZaValutu } from "../Utility/StabloUI";
export default function KasaInfo(prop) {
const { node, show, setShown, valutaVrijednosti } = prop;
const hideModal = (e) => {
setShown(false);
};
const getStringFromIndex = (index) => {
switch(index) {
case 0: return "BAM";
case 1: return "EUR";
case 2: return "USD";
case 3: return "HRK";
case 4: return "GBP";
default: return "UNKNOWN";
}
}
const infoKasa = () => {
if(node.kasatype == "Prodajna kasa" || node.kasatype == "Prodajno-primajuća kasa") {
var vrijednosti = dajVrijednostiZaValutu(node.kasavaluta, valutaVrijednosti);
var index = dajIndexZaVrijednost(vrijednosti, node.kasavrijednost);
var stanje = dajNizZaValutu(node.kasavaluta, [node.trenbam, node.treneur, node.trenusd, node.trenhrk, node.trengbp]);
return (<p>Broj kartica vrijednosti {vrijednosti[index]}{node.kasavaluta} : {stanje[index]}</p>)
}
else {
var nizoviStanja = [node.trenbam, node.treneur, node.trenusd, node.trenhrk, node.trengbp];
var nizoviVrijednosti = [valutaVrijednosti.bamvrijednosti, valutaVrijednosti.eurvrijednosti, valutaVrijednosti.usdvrijednosti, valutaVrijednosti.hrkvrijednosti, valutaVrijednosti.gbpvrijednosti]
var podaci = [];
for(var i = 0; i < 5; i++) {
for(var j = 0; j < nizoviStanja[i].length; j++) {
podaci.push(<><p>Broja kartica vrijednosti {nizoviVrijednosti[i][j]} {getStringFromIndex(i)}: {nizoviStanja[i][j]}</p></>);
}
}
return podaci;
}
/* return (
<div>{
(node.kasatype == "Prodajna kasa" || node.kasatype == "Prodajno-primajuća kasa") ? (
<>
<p> {node ? node.kasatype : ""} </p>
<p> Valuta: {node ? node.kasavaluta : ""} <br />
Vrijednost kartica: {node ? node.kasavrijednost : ""} <br />
Broj kartica: {getCardNumber()}
</p>
</>
) : (
<>
<p> {node ? node.kasatype : ""} </p>
<p> Valuta: {node ? node.kasavaluta : ""} <br />
Broj kartica vrijednosti 2: {node ? node.tren2[getIndexFromValuta(node.kasavaluta)] : ""} <br />
Broj kartica vrijednosti 5: {node ? node.tren5[getIndexFromValuta(node.kasavaluta)] : ""} <br />
Broj kartica vrijednosti 10: {node ? node.tren10[getIndexFromValuta(node.kasavaluta)] : ""} <br />
Broj kartica vrijednosti 20: {node ? node.tren20[getIndexFromValuta(node.kasavaluta)] : ""} <br />
Broj kartica vrijednosti 50: {node ? node.tren50[getIndexFromValuta(node.kasavaluta)] : ""} <br />
Broj kartica vrijednosti 100: {node ? node.tren100[getIndexFromValuta(node.kasavaluta)] : ""} <br />
</p>
</>
)
}
</div>
);*/
}
return (
<Modal show={show} onHide={hideModal}>
<Modal.Header closeButton>
<Modal.Title>{node ? node.title : ""}, id: {node ? node.id : ""}</Modal.Title>
</Modal.Header>
<Modal.Body>
<p>{node ? infoKasa() : ""}</p>
</Modal.Body>
<Modal.Footer>
<Button onClick={hideModal}>OK</Button>
</Modal.Footer>
</Modal>
);
} |
C> @file
C> @brief Test whether a character string is "missing"
C>
C> @author J. Ator @date 2012-06-07
C> Check whether a
C> character string returned from a previous call to subroutine
C> readlc() was encoded as "missing" (all bits set to 1)
C> within the actual BUFR data subset.
C>
C> @param[in] STR -- character*(*): String
C> @param[in] LSTR -- integer: Length of string, i.e. number of
C> characters within STR to be tested
C> @returns icbfms -- integer:
C> - 0 = STR is not "missing"
C> - 1 = STR is "missing"
C>
C> @remarks
C> - The use of an integer return code allows this function
C> to be called in a logical context from application programs
C> written in C as well as in Fortran.
C>
C> @author J. Ator @date 2012-06-07
RECURSIVE FUNCTION ICBFMS ( STR, LSTR ) RESULT ( IRET )
USE MODV_IM8B
CHARACTER*(*) STR
CHARACTER*8 STRZ
REAL*8 RL8Z
CHARACTER*16 ZZ
CHARACTER*16 ZM_BE
PARAMETER ( ZM_BE = '202020E076483742' )
C* 10E10 stored as hexadecimal on a big-endian system.
CHARACTER*16 ZM_LE
PARAMETER ( ZM_LE = '42374876E8000000' )
C* 10E10 stored as hexadecimal on a little-endian system.
EQUIVALENCE(STRZ,RL8Z)
C-----------------------------------------------------------------------
C Check for I8 integers.
IF ( IM8B ) THEN
IM8B = .FALSE.
CALL X84 ( LSTR, MY_LSTR, 1 )
IRET = ICBFMS ( STR, MY_LSTR )
IM8B = .TRUE.
RETURN
END IF
IRET = 0
NUMCHR = MIN(LSTR,LEN(STR))
C* Beginning with version 10.2.0 of the NCEPLIBS-bufr, "missing" strings
C* have always been explicitly encoded with all bits set to 1,
C* which is the correct encoding per WMO regulations. However,
C* prior to version 10.2.0, the NCEPLIBS-bufr stored "missing" strings by
C* encoding the REAL*8 value of 10E10 into the string, so the
C* following logic attempts to identify some of these earlier
C cases, at least for strings between 4 and 8 bytes in length.
IF ( NUMCHR.GE.4 .AND. NUMCHR.LE.8 ) THEN
DO II = 1, NUMCHR
STRZ(II:II) = STR(II:II)
END DO
WRITE (ZZ,'(Z16.16)') RL8Z
I = 2*(8-NUMCHR)+1
N = 16
IF ( ZZ(I:N).EQ.ZM_BE(I:N) .OR. ZZ(I:N).EQ.ZM_LE(I:N) ) THEN
IRET = 1
RETURN
END IF
END IF
C* Otherwise, the logic below will check for "missing" strings of
C* any length which are correctly encoded with all bits set to 1,
C* including those encoded by NCEPLIBS-bufr version 10.2.0 or later.
DO II=1,NUMCHR
STRZ(1:1) = STR(II:II)
IF ( IUPM(STRZ(1:1),8).NE.255 ) RETURN
ENDDO
IRET = 1
RETURN
END |
from abc import ABC, abstractmethod
from enum import Enum
from typing import Optional, Set, Tuple, Union
import numpy as np
from scipy.stats import gennorm
from sklearn.linear_model import lars_path_gram
from sklearn.utils.validation import check_random_state
class ProjectionVector:
beta: np.ndarray
q: int
def __init__(self, q: int):
self.q = q
def _normalize(self) -> None:
try:
self.beta = self.beta / np.linalg.norm(self.beta, ord=self.q, axis=0)
except AttributeError:
raise AttributeError("Must set attribute `beta` before normalizing.")
class ProjectionSampler(ProjectionVector):
def __init__(
self,
p: int,
q: int = 2,
sparsity: int = -1,
random_state: Optional[Union[int, np.random.RandomState]] = None,
):
"""Generates a normalized random projection vector (for initialization purposes).
Args:
p: The dimension of the vector.
q: The order of ell^q unit ball from which to sample.
sparsity: The number of non-zero coordinates; pass -1 for a dense vector.
random_state: NumPy random state.
"""
super().__init__(q=q)
_rs = check_random_state(random_state)
if sparsity > 0:
q_generalized_normal = np.zeros((p, 1))
idxs = _rs.choice(a=p, size=sparsity, replace=False)
q_generalized_normal[idxs, 0] = gennorm.rvs(beta=q, size=sparsity)
else:
q_generalized_normal = gennorm.rvs(beta=q, size=(p, 1))
self.beta = q_generalized_normal
self._normalize()
class SufficientStatisticsRegressionProjection(ABC, ProjectionVector):
def fit(self, x: np.ndarray, y: np.ndarray, w: Optional[np.ndarray] = None) -> None:
"""Fits weighted least squares (WLS) linear regression model via sufficient statistics.
Args:
x: design matrix of shape (n_samples, n_features)
y: response matrix of shape (n_samples, n_responses)
w: weight vector of shape (n_samples,)
"""
w = self._reshape_weights(x.shape[0], w)
xtx, xty = self.compute_sufficient_statistics(x, y, w)
wess = self.compute_effective_sample_size(w)
self._fit_sufficient(xtx, xty, wess)
def fit_normalize(self, x: np.ndarray, y: np.ndarray, w: Optional[np.ndarray] = None) -> None:
self.fit(x, y, w)
self._normalize()
def predict(self, x: np.ndarray) -> np.ndarray:
"""Predicts from linear model."""
return x @ self.beta
@staticmethod
def _reshape_weights(n: int, w: Optional[np.ndarray] = None) -> np.ndarray:
if w is None:
w = np.ones(n)
return w.reshape((n, 1))
@staticmethod
def compute_sufficient_statistics(
x: np.ndarray, y: np.ndarray, w: np.ndarray
) -> Tuple[np.ndarray, np.ndarray]:
"""Computes (weighted) sufficient statistics for WLS regression (Gram xtx, cross xty)."""
xtx = np.multiply(x, w).T @ x / w.sum()
xty = np.multiply(x, w).T @ y / w.sum()
return xtx, xty
@staticmethod
def compute_effective_sample_size(w: np.ndarray) -> float:
"""Computes effective sample size given sample weights."""
return w.sum() ** 2.0 / (w ** 2.0).sum()
@abstractmethod
def _fit_sufficient(self, xtx, xty, wess) -> None:
"""Fits linear model using only second-order sufficient statistics."""
class LowerUpperRegressionProjection(SufficientStatisticsRegressionProjection):
ridge: float
def __init__(self, q: int = 2, ridge: float = 0.0):
"""Instantiates a WLS linear model with ridge regularization and q-normalized beta.
This implementation computes regression coefficients by solving a system of linear equations
via the LU decomposition, which is the technique implemented by `gesv`, the LAPACK routine
called by `np.linalg.solve`.
Args:
q: The order of ell^q norm with which to normalize resultant beta.
ridge: Regularization level.
"""
super().__init__(q=q)
self.ridge = ridge
def _fit_sufficient(self, xtx, xty, wess) -> None:
self.beta = np.linalg.solve(xtx + self.ridge * np.eye(xtx.shape[0]), xty)
class LeastAngleRegressionProjection(SufficientStatisticsRegressionProjection):
max_iter: int
min_corr: float
alpha: np.ndarray
def __init__(self, q: int = 2, max_iter: int = 100, min_corr: float = 1e-4):
"""Instantiates a WLS linear regression model with sparse and q-normalized beta.
This implementation computes regression coefficients by iteratively traversing the LASSO
regularization path, which serves as an efficient way to solve the l1-regularized least
squares optimization problem (on par with, say, FISTA, but typically more efficient than
black-box quadratic programming methods).
Args:
q: The order of ell^q norm with which to normalize resultant beta.
max_iter: Maximum number of iterations.
"""
super().__init__(q=q)
self.max_iter = max_iter
self.min_corr = min_corr
def _fit_sufficient(self, xtx, xty, wess) -> None:
self.alpha, _, coefs = lars_path_gram(
Xy=xty[:, 0],
Gram=xtx,
n_samples=wess,
max_iter=self.max_iter,
alpha_min=self.min_corr,
method="lasso",
)
self.beta = coefs[:, [-1]]
class ProjectionOptimizerRegistry(Enum):
lower_upper = LowerUpperRegressionProjection
least_angle = LeastAngleRegressionProjection
@classmethod
def valid_mnemonics(cls) -> Set[str]:
return set(name for name, _ in cls.__members__.items())
@classmethod
def valid_regressors(cls) -> Set[SufficientStatisticsRegressionProjection]:
return set(value for _, value in cls.__members__.items()) |
function asyncThing(value) {
return new Promise((resolve) => {
setTimeout(() => resolve(value), 100);
});
}
async function map() {
return [1, 2, 3, 4].map(async (value) => {
const v = await asyncThing(value);
return v * 2;
});
}
map()
.then((v) => Promise.all(v))
.then((v) => console.log(v))
.catch((err) => console.error(err));
async function filter() {
return [1, 2, 3, 4].filter(async (value) => {
const v = await asyncThing(value);
return v % 2 === 0;
});
}
filter()
.then((v) => console.log(v))
.catch((err) => console.error(err));
async function reduce() {
return [1, 2, 3, 4].reduce(async (acc, value) => {
return (await acc) + (await asyncThing(value));
}, Promise.resolve(0));
}
reduce()
.then((v) => console.log(v))
.catch((err) => console.error(err)); |
import { Controller, Get, NotFoundException, Param, Res } from '@nestjs/common';
import { ApiParam, ApiResponse, ApiTags } from '@nestjs/swagger';
import { Response } from 'express';
import { FileStorage } from 'src/application/core/interfaces/storage/file-storage';
@ApiTags('Storage')
@Controller('storage')
export class ServeFileController {
constructor(private readonly fileStorage: FileStorage) {}
@ApiParam({ name: 'key', description: 'Key of the file' })
@Get(':key')
@ApiResponse({ status: 200, description: 'File retrieved successfully' })
@ApiResponse({ status: 404, description: 'File not found' })
async serveFile(@Param('key') key: string, @Res() res: Response) {
const file = await this.fileStorage.get(key);
if (!file) {
throw new NotFoundException({
code: 'FILE_NOT_FOUND',
message: 'File not found',
});
}
res.setHeader(
'Content-Disposition',
`inline; filename="${file.originalName}"`,
);
res.setHeader('Content-Type', file.mimetype);
res.send(file.content);
}
} |
取消引用 Capstone 项目
以之前的项目为基础,创建一个生动活泼的 Android 应用程序!
客观的
这是一条漫长的路,现在是你完成旅程的时候了。
使用您所学到的有关应用程序设计、Java、XML 和 Android 的所有知识来实现 Unquote。
在此项目中,您将整合之前应用程序构建项目的成就,并将其封装成一个可运行的 Android 游戏。
第 1 部分:准备您的项目
取消引用基本上已经完成了一半,但是到目前为止您生成的代码已经存在于 Codecademy 的平台上。在本部分中,您将把之前的工作合并到Unquote Android Studio 项目中,并首次启动您的应用程序。
我们鼓励您使用迄今为止构建的所有代码。但如果您愿意,您可以在此 zip 文件夹中访问MainActivity.java、Question.java和Activity_main.xml的代码
1-A。打开您的取消引用 Android 项目
如果您不再有权访问该项目,请使用以下参数创建一个新的 Android 项目:
名称:取消引号
最低 API 级别:16
语言:Java
模板:空活动
1-B。准备MainActivity.java
导航到MainActivity.java您的项目,然后删除该文件中最顶行下方的所有内容。删除以下所有内容:package com.{your-company-name}.unquote;。
暗示
1-C。粘贴到 MainActivity.java
MainActivity.java将本节中包含的文件内容复制到您的MainActivity.java项目文件中。
不要复制最上面的行:因为这会与您的实际包名称package com.example.unquote;冲突。
暗示
1-D。创建一个问题类
打开后MainActivity.java,导航至“文件”菜单。
选择“文件”,然后选择“新建”,然后单击“Java 类”。在类名称字段中输入“问题”,然后单击“确定”。
完成此任务后,请继续关注该Question.java选项卡。
1-E。粘贴到 Question.java
复制本节中包含的文件内容Question.java,并将其粘贴到QuestionAndroid Studio 中类的大括号之间。
暗示
1-F。打开并清除activity_main.xml
打开activity_main.xml布局文件。您可以在资源管理器的布局部分找到它。
打开后,如果您看不到“文本”或“代码”(XML 内容),请切换到允许您直接编辑底层 XML 内容的视图。
选择文件中的每一行activity_main.xml并将其删除。
1-G。把所有的东西都换掉
复制本节中包含的文件内容activity_main.xml并将其粘贴到项目的activity_main.xml.
如果您想使用activity_main.xml在之前的Unquote项目中创建的布局文件,请使用声明的属性面板修改现有的组件 ID 以匹配以下内容:
成分 ID
报价图片查看 iv_main_question_image
问题文本查看 tv_main_question_title
提交按钮 btn_main_submit_answer
回答 0 按钮 btn_main_answer_0
接听 1 按钮 btn_main_answer_1
接听 2 按钮 btn_main_answer_2
接听 3 按钮 btn_main_answer_3
TextView 剩余问题数(“99”) tv_main_questions_remaining_count
剩余问题 TextView(“剩余问题”) tv_main_questions_remaining
1-H。第一次运行取消引用
您需要一部真正的 Android 手机或 Android 虚拟设备才能运行Unquote。
如果您需要有关如何创建虚拟设备以及如何为个人设备启用开发模式的指导,请参阅本文。
1-I。没什么可看的……
但这都是你的!进入下一部分继续构建Unquote。
第 2 节. 导入艺术品
演示将普通应用程序与特殊应用程序区分开来,因此我们创建了一个自定义Unquote应用程序图标和 6 张手绘报价图像,供您向玩家演示。
在本节中,您将把这些资源导入到您的应用程序中,修改应用程序的启动器图标,并将该图标呈现给内部的玩家MainActivity。
2-A。下载艺术品
Unquote所需的每个文件都位于此 zip 存档内。下载文件夹并将其解压到桌面。
2-B。在 Android Studio 中,显示资源管理器
如果找不到资源管理器,请在菜单下查找“视图” > “工具窗口” > “资源管理器”。
2-C。将 unquote-drawables 文件夹拖到资源管理器面板中
这将使文件成为Unquote的一部分,并可供您的代码和布局访问。
您可以在 Windows 或 OS X 中通过单击文件夹图标并将其拖到资源管理器窗口中来执行此操作。
这将显示导入 Drawables向导,Drawables 是与应用程序捆绑在一起的图像和图形。
单击“下一步”按钮,然后在接下来的屏幕上单击“导入” 。瞧,这些图像现在是Unquote的一部分!
2-D。打开 AndroidManifest.xml
AndroidManifest.xml您可以在项目树中的app > manifests >下找到该文件AndroidManifest.xml。
2-E。使用您的自定义取消引用图标
AndroidManifest.xml除此之外,它还告诉 Android 操作系统在哪里可以找到您的应用程序图标。它通过两行提供此信息:
<!-- some attributes here -->
android:icon="@mipmap/ic_launcher"
<!-- more attributes -->
android:roundIcon="@mipmap/ic_launcher_round"
<!-- and more attributes -->
从 Android v26 开始,Google 建议您提供自适应图标,允许设备将角变圆或将图像切割成他们认为合适的任何形状。你不会那样做的。
为了简化,删除第二行并将第一行更改为android:icon="@drawable/ic_unquote_icon"。名为的图像ic_unquote_icon与您之前导入的图像捆绑在一起。
2-F。保存并重新启动
重新启动您的应用程序并查看Unquote闪亮的新图标!
暗示
2-G。炫耀一下
您可能已经看到一些时髦的应用程序将其应用程序图标放置在其界面的顶部栏(也称为 ActionBar)中。
为了确保Unquote保持时尚,您最终将开始履行所有这些小评论留下的承诺TODO。
TODO 2-G在以下位置添加以下四行MainActivity:
// TODO 2-G: Show app icon in ActionBar
getSupportActionBar().setDisplayShowHomeEnabled(true);
getSupportActionBar().setLogo(R.drawable.ic_unquote_icon);
getSupportActionBar().setDisplayUseLogoEnabled(true);
getSupportActionBar().setElevation(0);
不用担心学习这些方法,它们是小众 Android API,您无需立即掌握。
保存并重新启动“取消引用”即可在大(小)屏幕上看到精美的新图标。现在感觉很正式,不是吗?
2-H。使用“真实”的假报价
打开MainActivity,然后向下滚动到您的startNewGame()方法。
使用该对象将每个对象的R.drawable第一个参数 () 替换为适当的假报价图像。int imageIdQuestionTODO 2-H
确保Question总共有 6 个对象,并使用此 PDF中提供的问题和答案。
稍后,您将使用该成员变量来设置屏幕Question.imageId上呈现的图像。ImageView
暗示
2-I。在下一节中...
您将恢复视图并开始在屏幕上显示这些引言、他们的问题以及他们可能的答案!
“太好啦!太好啦!太好啦!”
— Isla Von Frederickson 博士
可能是髋关节置换术的发明者
第 3 节. 查找并分配视图对象
如果不首先恢复 View 对象,您将无法更新任何文本、使用图像资源或响应单击。在本节中,您将从布局中提取 View 对象并开始在代码中使用它们。
让我们开始!
3-A。声明View成员变量
您可以findViewById()在 中的任何地方MainActivity使用,但对于复杂的布局,此方法会变得昂贵 - 昂贵是因为您的用户可能会发现应用程序暂时无响应或缓慢(滞后),因为您的代码重复调用findViewById()。
要解决此限制,请将所需的所有视图声明为MainActivity成员变量。然后,MainActivity可以在任何地方访问和修改视图,而无需重新调用findViewById()。
为您的代码需要访问的每个视图声明一个成员变量 at TODO 3-A,以下是我们推荐的名称(并将在示例中使用):
questionImageView
questionTextView
questionsRemainingTextView
answer0Button
answer1Button
answer2Button
answer3Button
submitButton
暗示
3-B。分配所有 View 成员变量
用于findViewById()将每个 View 成员变量分配给位于 处的膨胀 View 对象TODO 3-B。
请记住,findViewById()找到与给定标识符(在布局中提供)相对应的视图activity_main.xml,并让您在代码中直接控制该视图。
暗示
3-C。显示剩余问题数
恢复所有视图后,您就可以displayQuestionsRemaining()在 处构建方法了TODO 3-C。
此方法不返回任何内容 ( void),并将剩余问题的数量作为整数参数。
声明方法并让它修改questionsRemainingTextView对象。
暗示
3-D。使用displayQuestionsRemaining()
此方法现在功能齐全,取消注释并在必要时调用它(TODO 3-D.i和TODO 3-D.ii)。
更新剩余计数在这两个时刻至关重要,它们是代码中我们更改剩余问题数量的唯一位置。第一个是在玩家提交答案之后,第二个是在我们创建新游戏之后。
暗示
3-E。重新运行取消引用
通过displayQuestionsRemaining()行动,您现在有了一个可以工作的问题计数器!
重新运行Unquote后,您应该看到“剩余 6 个问题”(而不是 99)。如果没有,请返回检查您的工作!
暗示
3-F。声明显示问题()
此方法不返回任何内容 ( void),并将修改Question向玩家呈现对象所需的所有 View 对象。
首先声明该方法并将主体保留为空TODO 3-F。
3-G。实现显示问题()
您应该有权访问实现此方法所需的每个视图:您的 main ImageView、您的问题TextView和您的四个答案Button视图(全部在 3-A 中声明为成员变量)。
您必须使用setText()或setImageResource()方法修改这些 View 对象。
暗示
3-H。取消注释 displayQuestion()
对于TODO 3-H.i和TODO 3-H.ii,删除这些行前面的注释(删除//)。
在 处3-H.i,我们在选择一个新问题供玩家回答后立即调用此方法。并且在3-H.ii,
暗示
3-I。重新运行取消引用
如果一切按计划进行,您应该会在屏幕上看到 6 个可用问题之一!
图像、问题和四个答案都应反映 中提供的数据startNewGame()。如果没有,请返回检查您的工作。
暗示
第 4 节:使用 onAnswerSelected() 选择答案
最后!玩家可以在屏幕上看到一个问题,这几乎完成了 80% 的工作。剩下的就是确保点击和点击屏幕会产生玩家期望的准确响应。
在本部分中,您将使玩家能够从每个问题提供的四个可能答案中选择一个。
4-A。声明 onAnswerSelected()
此方法不返回任何内容 ( void),并且需要一个整数来标识玩家的答案(0 到 3)。
该方法不仅会设置playerChoice成员变量,还会执行许多界面和逻辑更新以保持游戏继续进行。
在 处声明此方法TODO 4-A。您将在 中完成以下 3 个任务onAnswerSelected()。
4-B。恢复当前问题
您的第一个任务onAnswerSelected()是更新底层Question对象以反映玩家的选择。
首先将对当前对象的引用保存Question在名为 的变量中currentQuestion。
暗示
4-C。更改玩家的答案
在 中Question,您定义了一个名为 的成员变量playerAnswer来跟踪玩家选择的答案。
修改对象playerAnswer的成员变量currentQuestion以匹配玩家的选择(answerSelection)。
暗示
4-D。表明一个选择
当玩家做出选择时,您需要显示一些视觉指示来表明他们所做的选择。
实现此目的的一种方法是在✔给出的答案的开头添加一个符号Button。
例如,Button“Abraham Lincoln”的答案在玩家单击后会变成“✔ Abraham Lincoln”。
使用“✔”符号,在此页面上突出显示它,复制并粘贴到您的代码中!
暗示
4-E。聆听点击声
使用该View.setOnClickListener()方法将空的点击侦听器分配给Button位于 的所有四个答案视图TODO 4-E。
暗示
4-F。每个按钮,一个选择
当用户单击答案按钮时,他们没有意识到每个答案都与一个数字(0、1、2 和 3)相关联,他们只知道自己做出了哪个选择。
但是您为每个事件提供的点击处理程序Button必须onAnswerSelected()根据每个事件Button代表的底层答案标识符进行调用。
在四个点击侦听器中的每一个中,onAnswerSelected()使用适当的answerSelected参数进行调用。
暗示
4-G。运行取消引用
并做出您的选择!
如果一切看起来都正确,那么就有问题了。
4-H。你也注意到了,是吗?
如果您的逻辑正确,则每个答案在您单击后Button都会显示一个复选符号 ( )。✔
然而,即使您的“选择”发生变化,您之前的选择仍然被选择!你如何解决这个问题?
思考几分钟,然后继续进行 4-I。
暗示
4-I。冗余挽救了局面
Button要解决 4-H 中发现的问题,请在 4-E 中添加的逻辑之前重置所有四个答案视图的文本。
这意味着,通过setText()再次使用,您可以将所有按钮重置到其起始位置(无“✔”)。
暗示
4-J。尝试再尝试
再运行一次“取消引用”Button ,然后观察您的选择从一个到下一个的优雅移动!
凭借您选择正确答案的能力,您距离为玩家评分并结束游戏又近了一步。
第 5 节. 游戏结束
一场永远不会结束的游戏根本就不是游戏,而是一场噩梦。
在本部分中,您将允许玩家(无论输赢)结束他们的Unquote回合。如果他们敢于再次测试他们的知识,你就会给他们这个机会。
5-A。提交时点击
Button使用View.setOnClickListener()at将点击侦听器分配给您的提交答案TODO 5-A。
单击Button布局右下角的 时,会锁定玩家的选择并移至下一个问题(或结束游戏)。
暗示
5-B。调用 onAnswerSubmission()
您在上一个项目中就开始使用此方法,现在您可以使用它了!
从 5-A 中声明的点击处理程序调用此方法,以允许玩家提交他们的最终答案。
暗示
5-C。玩一个完整的游戏
取消引用现在可以播放了!玩游戏并在 Android Studio 中观看Logcat以获取游戏结束消息。
有点虎头蛇尾吧?让我们解决这个问题。
5-D。开始对话
以前,您的应用程序将游戏结束消息打印到Logcat,但AlertDialog可以帮助您将此消息呈现给真正会阅读它的人:玩家。
在 Android 中,您可以使用内置 API 来显示一个小型弹出窗口,其中包含自定义消息和可单击选项。该弹出窗口称为AlertDialog.
在 处TODO 5-D,删除该System.out…行,并将其替换为以下内容:
// TODO 5-D: Show a popup instead
AlertDialog.Builder gameOverDialogBuilder = new AlertDialog.Builder(MainActivity.this);
gameOverDialogBuilder.setCancelable(false);
此类Builder可帮助您生成符合播放器设备视觉主题的弹出窗口(它始终看起来正确)。
该AlertDialog.Builder.setCancelable()方法可以防止玩家通过点击弹出窗口外部意外取消弹出窗口。
5-E。给它一个标题
标题AlertDialog作为一条短消息显示在弹出窗口的顶部。
用于AlertDialog.Builder.setTitle()为紧邻 5-D 中添加的代码下方的弹出窗口设置此短消息。
暗示
5-F。传播消息
用于向玩家AlertDialog.Builder.setMessage()展示您的内容。gameOverMessage它将gameOverMessage在弹出窗口中显示为一小段文本。
暗示
5-G。再给他们一次机会……
最后,让我们再给玩家一次Unquote的机会。
AlertDialog可以呈现积极、中性和消极按钮(从左到右或从上到下指示它们的位置,具体取决于设备)。
使用以下代码创建一个肯定AlertDialog按钮,将其放置在您为 5-F 编写的代码的正下方:
// (5-F code)
gameOverDialogBuilder.setPositiveButton("Play Again!", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
startNewGame();
}
});
暗示
5-H。呈现对话框
AlertDialog当使用对象创建时AlertDialog.Builder,您必须在它出现在屏幕上之前调用另外两个方法。在上一个任务中的代码下方添加以下行:
// (5-G code)
gameOverDialogBuilder.create().show();
5-I。再玩一次,萨姆
重新启动取消报价并进行另一轮。这次,您的游戏结束消息应该出现在一个简单而有效的弹出窗口中!
按“再玩一次!”,Unquote开始新一轮的问答游戏!
第 6 节. 接受挑战
取消引用看起来不错并且运行良好。在最后一部分中,我们要求您找到(并修复)一些错误,并添加一组新的琐事问题供玩家回答。
本节的提示不会像前面的那么详细,所以请发挥你的思维能力!😉
6-A。请提交
当您在选择选项之前提交答案时会发生什么?
暗示
6-B。修复此错误
提交答案时,Unquote必须首先确保玩家选择了答案(默认的 除外-1)。
您如何检查这种情况以及您应该在哪里检查它以防止Unquote接受-1作为答案?
暗示
6-C。更多问题,更多答案
当您精心构建Unquote时,我们的图形艺术家有时间消磨时间,因此我们让他们设计更多报价供您在游戏中使用。
下载这些附加资源并将其解压缩到您的桌面。在里面,您会发现另外 7 个可在Unquote中使用的报价图像,以及一个详细说明每个图像附带的问题和答案的 PDF!
6-D。创建第二组 Question 对象
将 6-C 中的图像导入为 Drawable 后,在前 6 个对象的正下方再定义 7 个Question对象,并将它们添加到列表中。startNewGame()Questionquestions
6-E。扭转一下……
第一组报价与第二组报价有很大不同。
您将使用循环和generateRandomNumber()方法从可用的 13 个问题中选择 6 个问题 — 这些是您的玩家每轮必须回答的 6 个问题。
你会怎么做?
暗示
6-F。设置你的循环
您需要一个运行 7 次的循环,现在就设置一个。
暗示
6-G。随机选择一个
Question在循环中,您需要从questions列表中随机选择一个对象。
使用(您在游戏逻辑第一部分generateRandomNumber()中编写的方法)随机选择一个。Question
暗示
6-H。删除随机选择的 Question 对象
ArrayList.remove()允许您根据项目在列表中的位置(索引)从列表中删除项目。使用此方法删除随机选择的Quesion对象。
暗示 |
import React, { useState } from 'react'
import { useDispatch, useSelector } from 'react-redux'
import { postAdded } from './postsSlice'
export const AddPostForm = () => {
const [title, setTitle] = useState("")
const [content, setContent] = useState("")
const [userId, setUserId] = useState("")
const dispatch = useDispatch()
const users = useSelector(state => state.users)
const onUserChanged = e => setUserId(e.target.value)
const onTitleChanged = e => setTitle(e.target.value)
const onContentChanged = e => setContent(e.target.value)
const onSave = () => {
if(title && content) {
dispatch(postAdded( title, content, userId ))
setTitle("")
setContent("")
}
}
const canSave = Boolean(title) && Boolean(content) && Boolean(userId)
const userOptions = users.map(user => (
<option key={user.id} value={user.id}>
{user.name}
</option>
))
return (
<section>
<h2> Add a New Post</h2>
<form>
<label htmlFor="postTitle">Post Title:</label>
<input
type="text"
id="postTitle"
name="postTitle"
value={title}
onChange={onTitleChanged}
/>
<label htmlFor="postUser">User:</label>
<select id="postUser" value={userId} onChange={onUserChanged}>
{userOptions}
</select>
<label htmlFor="postContent">Content:</label>
<textarea
id="postContent"
name="postContent"
value={content}
onChange={onContentChanged}
/>
<button
type="button"
onClick={onSave}
disabled={!canSave}
>Save </button>
</form>
</section>
)
} |
import { useDispatch, useSelector } from "react-redux";
import { fetchContacts } from "redux/operations";
import ContactForm from "./ContactForm/ContactForm";
import Filter from './Filter/Filter';
import ContactList from "./ContactList/ContactList";
import css from './App.module.css';
import { useEffect } from "react";
import { selectIsLoading, selectError } from "redux/selectors";
export const App = () => {
const dispatch = useDispatch();
const isLoading = useSelector(selectIsLoading);
const error = useSelector(selectError);
useEffect(() => {
dispatch(fetchContacts());
}, [dispatch]);
return (
<div className={css.body}>
<h1 className={css.title}>Phonebook</h1>
<div className={css.wrapper}>
<ContactForm />
<div className={css.contacts}>
<h2 className={css.contactsTitle} >Contacts</h2>
<Filter />
<ContactList />
{isLoading && !error && <p>Loading...</p>}
</div>
</div>
</div>
);
}; |
<?php
class sw_get_in_touh extends \Elementor\Widget_Base {
public function get_name() {
return 'sw_get_in_touh';
}
public function get_title() {
return __( 'Software Get In Touch', 'prysm' );
}
public function get_icon() {
return 'eicon-table-of-contents';
}
public function get_categories() {
return ['prysm-category'];
}
protected function register_controls() {
$this->start_controls_section(
'heading__content',
[
'label' => __( 'Heading Content', 'prysm' ),
'tab' => \Elementor\Controls_Manager::TAB_CONTENT,
]
);
$this->add_control(
'sub_title', [
'label' => esc_html__( 'Sub Title', 'prysm' ),
'type' => \Elementor\Controls_Manager::TEXT,
]
);
$this->add_control(
'title', [
'label' => esc_html__( 'Title', 'prysm' ),
'type' => \Elementor\Controls_Manager::TEXTAREA,
]
);
$this->add_control(
'heading_info', [
'label' => esc_html__( 'Heading Info', 'prysm' ),
'type' => \Elementor\Controls_Manager::TEXTAREA,
]
);
$this->add_control(
'btn_text', [
'label' => esc_html__( 'Button Text', 'prysm' ),
'type' => \Elementor\Controls_Manager::TEXT,
]
);
$this->add_control(
'btn_link',
[
'label' => __( 'Button Link', 'prysm' ),
'type' => \Elementor\Controls_Manager::URL,
'placeholder' => __( 'https://your-link.com', 'prysm' ),
]
);
$this->add_control(
'shape1', [
'label' => esc_html__( 'Shape 1', 'prysm' ),
'type' => \Elementor\Controls_Manager::MEDIA,
]
);
$this->add_control(
'shape2', [
'label' => esc_html__( 'Shape 2', 'prysm' ),
'type' => \Elementor\Controls_Manager::MEDIA,
]
);
$this->add_control(
'circle_img', [
'label' => esc_html__( 'Circle Img', 'prysm' ),
'type' => \Elementor\Controls_Manager::MEDIA,
]
);
$this->add_control(
'vector_img', [
'label' => esc_html__( 'Vector Img', 'prysm' ),
'type' => \Elementor\Controls_Manager::MEDIA,
]
);
$this->end_controls_section();
$this->start_controls_section(
'section_heading_style',
[
'label' => esc_html__( 'Heading Style', 'prysm' ),
'tab' => \Elementor\Controls_Manager::TAB_STYLE,
]
);
$this->add_control(
'h_stylish_title',
[
'type' => \Elementor\Controls_Manager::HEADING,
'label' => esc_html__( 'Stylish itle Style', 'prysm' ),
'separator' => 'before',
]
);
$this->add_control(
's_title_color',
[
'label' => esc_html__( 'Title Color', 'prysm' ),
'type' => \Elementor\Controls_Manager::COLOR,
'selectors' => [
'{{WRAPPER}} .pr3-title-area span' => 'color: {{VALUE}} ',
],
]
);
$this->add_group_control(
\Elementor\Group_Control_Typography::get_type(),
[
'name' => 's_title_typography',
'label' => esc_html__( 'Typography', 'prysm' ),
'selector' => '{{WRAPPER}} .pr3-title-area span',
'fields_options' => [
'typography' => [
'default' => 'custom',
],
],
]
);
$this->add_control(
'h_title',
[
'type' => \Elementor\Controls_Manager::HEADING,
'label' => esc_html__( 'Title Style', 'prysm' ),
'separator' => 'before',
]
);
$this->add_control(
'title_color',
[
'label' => esc_html__( 'Title Color', 'prysm' ),
'type' => \Elementor\Controls_Manager::COLOR,
'selectors' => [
'{{WRAPPER}} .pr3-title-area h3' => 'color: {{VALUE}} ',
],
]
);
$this->add_group_control(
\Elementor\Group_Control_Typography::get_type(),
[
'name' => 'title_typography',
'label' => esc_html__( 'Typography', 'prysm' ),
'selector' => '{{WRAPPER}} .pr3-title-area h3',
'fields_options' => [
'typography' => [
'default' => 'custom',
],
],
]
);
$this->add_control(
'_info_content_title_',
[
'type' => \Elementor\Controls_Manager::HEADING,
'label' => esc_html__( 'Info Content', 'prysm' ),
'separator' => 'before',
]
);
$this->add_control(
'content_color',
[
'label' => esc_html__( 'Text Color', 'prysm' ),
'type' => \Elementor\Controls_Manager::COLOR,
'selectors' => [
'{{WRAPPER}} .pr3-title-area .pr3-pera-txt p' => 'color: {{VALUE}} ',
],
]
);
$this->add_group_control(
\Elementor\Group_Control_Typography::get_type(),
[
'name' => 'text_typography',
'label' => esc_html__( 'Typography', 'prysm' ),
'selector' => '{{WRAPPER}} .pr3-title-area .pr3-pera-txt p',
'fields_options' => [
'typography' => [
'default' => 'custom',
],
],
]
);
$this->end_controls_section();
$this->start_controls_section(
'btn_one_style',
[
'label' => esc_html__( 'Button One Style', 'prysm' ),
'tab' => \Elementor\Controls_Manager::TAB_STYLE,
]
);
$this->start_controls_tabs( '_banner_button_1' );
$this->start_controls_tab(
'_prysm_btn__banner_normal',
[
'label' => esc_html__( 'Normal', 'prysm-extension' ),
]
);
$this->add_control(
'btn__color',
[
'label' => esc_html__( 'Color', 'prysm-extension' ),
'type' => \Elementor\Controls_Manager::COLOR,
'selectors' => [
'{{WRAPPER}} .pr3-primary-btn a' => 'color: {{VALUE}}',
],
]
);
$this->add_group_control(
\Elementor\Group_Control_Background::get_type(),
[
'name' => 'btn_bg_color',
'label' => __( 'Background', 'prysm-extension' ),
'types' => ['classic', 'gradient'],
'exclude' => ['image'],
'selector' => '{{WRAPPER}} .pr3-primary-btn a::after',
]
);
$this->add_responsive_control(
'btn_border_radious',
[
'label' => esc_html__( 'Border Radius', 'prysm-extension' ),
'type' => \Elementor\Controls_Manager::DIMENSIONS,
'size_units' => ['px', '%'],
'selectors' => [
'{{WRAPPER}} .pr3-primary-btn a::after' => 'border-radius: {{TOP}}{{UNIT}} {{RIGHT}}{{UNIT}} {{BOTTOM}}{{UNIT}} {{LEFT}}{{UNIT}};',
],
]
);
$this->add_group_control(
\Elementor\Group_Control_Border::get_type(),
[
'name' => 'border',
'label' => __( 'Border', 'prysm-extension' ),
'selector' => '{{WRAPPER}} .pr3-primary-btn a',
]
);
$this->add_responsive_control(
'btn_padding',
[
'label' => esc_html__( 'Border Radius', 'prysm-extension' ),
'type' => \Elementor\Controls_Manager::DIMENSIONS,
'size_units' => ['px', '%'],
'selectors' => [
'{{WRAPPER}} .pr3-primary-btn a' => 'padding: {{TOP}}{{UNIT}} {{RIGHT}}{{UNIT}} {{BOTTOM}}{{UNIT}} {{LEFT}}{{UNIT}};',
],
]
);
$this->end_controls_tab();
$this->start_controls_tab(
'_prysm_btn_hover',
[
'label' => esc_html__( 'Hover', 'prysm-extension' ),
]
);
$this->add_control(
'btn__hover_color',
[
'label' => esc_html__( 'Color', 'prysm-extension' ),
'type' => \Elementor\Controls_Manager::COLOR,
'selectors' => [
'{{WRAPPER}} .pr3-primary-btn a:hover' => 'color: {{VALUE}}',
],
]
);
$this->add_group_control(
\Elementor\Group_Control_Background::get_type(),
[
'name' => 'btn_hover_bg_color',
'label' => __( 'Background', 'prysm-extension' ),
'types' => ['classic', 'gradient'],
'exclude' => ['image'],
'selector' => '{{WRAPPER}} .pr3-primary-btn a:hover, .pr3-primary-btn a::before',
]
);
$this->add_responsive_control(
'btn_hover_border_radious',
[
'label' => esc_html__( 'Border Radius', 'prysm-extension' ),
'type' => \Elementor\Controls_Manager::DIMENSIONS,
'size_units' => ['px', '%'],
'selectors' => [
'{{WRAPPER}} .pr3-primary-btn a:hover' => 'border-radius: {{TOP}}{{UNIT}} {{RIGHT}}{{UNIT}} {{BOTTOM}}{{UNIT}} {{LEFT}}{{UNIT}};',
],
]
);
$this->add_group_control(
\Elementor\Group_Control_Border::get_type(),
[
'name' => 'btn_hover_border',
'label' => __( 'Border', 'prysm-extension' ),
'selector' => '{{WRAPPER}} .pr3-primary-btn a:hover',
]
);
$this->add_responsive_control(
'btn_hover_padding',
[
'label' => esc_html__( 'Border Radius', 'prysm-extension' ),
'type' => \Elementor\Controls_Manager::DIMENSIONS,
'size_units' => ['px', '%'],
'selectors' => [
'{{WRAPPER}} .pr3-primary-btn a:hover' => 'padding: {{TOP}}{{UNIT}} {{RIGHT}}{{UNIT}} {{BOTTOM}}{{UNIT}} {{LEFT}}{{UNIT}};',
],
]
);
$this->end_controls_tab();
$this->end_controls_tabs();
$this->end_controls_section();
}
protected function render() {
$settings = $this->get_settings_for_display();
$sub_title = $settings['sub_title'];
$title = $settings['title'];
$heading_info = $settings['heading_info'];
$btn_text = $settings['btn_text'];
$btn_link = $settings['btn_link'];
$shape1 = $settings['shape1']['url'];
$shape2 = $settings['shape2']['url'];
$circle_img = $settings['circle_img']['url'];
$vector_img = $settings['vector_img']['url'];
?>
<section class="pr3-get-in-touch">
<span class="pr3-get-net-shape"><img src="<?php echo esc_url($shape1);?>" alt=""></span>
<span class="pr3-get-plus-shape"><img src="<?php echo esc_url($shape2);?>" alt=""></span>
<div class="container">
<div class="row">
<div class="col-lg-6">
<div class="pr3-title-area pr3-get-left text-left wow fadeInUp">
<div class="pr3-headline">
<span><?php echo esc_html($sub_title);?></span>
<div class="pr3-headline">
<h3><?php echo esc_html($title);?></h3>
</div>
<div class="pr3-pera-txt">
<p><?php echo __($heading_info);?></p>
</div>
</div>
<div class="pr3-primary-btn">
<a href="<?php echo esc_url($btn_link['url']);?>"><?php echo esc_html($btn_text);?></a>
</div>
</div>
</div>
<div class="col-lg-5 offset-lg-1">
<div class="pr3-get-right wow fadeInRight" data-wow-delay="0.2s">
<div class="pr3-get-circle">
<img src="<?php echo esc_url($circle_img);?>" alt="">
</div>
<div class="pr3-get-vector-img">
<img src="<?php echo esc_url($vector_img);?>" alt="">
</div>
</div>
</div>
</div>
</div>
</section>
<?php
}
} |
'use strict';
const express = require('express');
const path = require('path');
const favicon = require('serve-favicon');
const logger = require('morgan');
const cookieParser = require('cookie-parser');
const bodyParser = require('body-parser');
// Api Routes
const demoApiRouter = require('./api').demo;
// View Routes
const viewsRouter = require('./routes/index');
const app = express();
// view engine setup
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'pug');
// uncomment after placing your favicon in /public
app.use(favicon(path.join(__dirname, 'public', 'favicon.ico')));
app.use(logger('dev'));
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: false }));
app.use(cookieParser());
app.use(express.static(path.join(__dirname, './public')));
// Setting config params in the request object for usage by following request handling
app.use(function (req, res, next) {
req.config = {
env: process.env.NODE_ENV || 'production'
};
next();
});
// API Routes
app.use(demoApiRouter);
// Setting generic locals for different pug rendering usage
app.use(function (req, res, next) {
res.locals.env = req.config.env;
next();
});
// Pages Routes
app.use(viewsRouter);
// catch 404 and forward to error handler
app.use(function(req, res, next) {
const err = new Error('Not Found');
err.status = 404;
next(err);
});
// error handler
app.use(function(err, req, res) {
// set locals, only providing error in development
res.locals.message = err.message;
// render the error page
res.status(err.status || 500);
res.render('error');
});
module.exports = app; |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/submissions/
You are given an array prices where prices[i] is the price of a given stock on the ith day.
You want to maximize your profit by choosing a single day to buy one stock and choosing a different day in the future to sell that stock.
Return the maximum profit you can achieve from this transaction. If you cannot achieve any profit, return 0.
Example 1:
Input: prices = [7,1,5,3,6,4]
Output: 5
Explanation: Buy on day 2 (price = 1) and sell on day 5 (price = 6), profit = 6-1 = 5.
Note that buying on day 2 and selling on day 1 is not allowed because you must buy before you sell.
Example 2:
Input: prices = [7,6,4,3,1]
Output: 0
Explanation: In this case, no transactions are done and the max profit = 0.
// OPTIMAL
// TC : O(N)
// SC : O(1)
class Solution {
public int maxProfit(int[] prices) {
int n=prices.length;
int l=0; // buy
int r=1; // sell
int profit=0;
while(r<n){
profit=Math.max(profit, prices[r]-prices[l]);
if(prices[r]<prices[l]){
l=r;
}
r++;
}
return profit;
}
}
// BRUTE FORCE----> TLE
// TC : O(N^2)
// SC : O(1)
// class Solution {
// public int maxProfit(int[] prices) {
// int n=prices.length;
// int profit=Integer.MIN_VALUE;
// for(int i=0;i<n;i++){
// for(int j=i;j<n;j++){
// profit=Math.max(profit, prices[j]-prices[i]);
// }
// }
// return profit;
// }
// } |
import React from 'react'
import cn from 'classnames'
import Link from 'next/link'
import { useRouter } from 'next/router'
import renderComponent from './utils/render-component'
import { getFSRoute } from './utils/get-fs-route'
import useMenuContext from './utils/menu-context'
import { useConfig } from './config'
import Search from './search'
import Flexsearch from './flexsearch'
import GitHubIcon from './icons/github'
import DiscordIcon from './icons/discord'
import { Item, PageItem } from './utils/normalize-pages'
interface NavBarProps {
isRTL?: boolean | null
flatDirectories: Item[]
items: PageItem[]
}
export default function Navbar({ flatDirectories, items }: NavBarProps) {
const config = useConfig()
const { locale, asPath } = useRouter()
const activeRoute = getFSRoute(asPath, locale)
const { menu, setMenu } = useMenuContext()
const bannerKey = config.bannerKey || 'honey-banner'
return (
<>
<script
dangerouslySetInnerHTML={{
__html: `try{if(localStorage.getItem(${JSON.stringify(
bannerKey
)})==='0'){document.body.classList.add('honey-banner-hidden')}}catch(e){}`
}}
/>
{config.banner ? (
<div className="honey-banner-container text-sm h-10 sticky top-0 md:relative pl-10 flex items-center text-slate-50 bg-neutral-900 dark:text-white z-20 dark:bg-[linear-gradient(1deg,#383838,#212121)]">
<div className="max-w-[90rem] mx-auto w-full py-1 text-center font-medium pl-[max(env(safe-area-inset-left),1.5rem)] pr-[max(env(safe-area-inset-right),1.5rem)] truncate whitespace-nowrap">
{renderComponent(config.banner, {
locale
})}
</div>
<button
className="mr-2 w-8 opacity-80 hover:opacity-100"
onClick={() => {
try {
localStorage.setItem(bannerKey, '0')
} catch (e) { }
document.body.classList.add('honey-banner-hidden')
}}
>
<svg
xmlns="http://www.w3.org/2000/svg"
className="h-4 w-4 mx-auto"
viewBox="0 0 20 20"
fill="currentColor"
>
<path
fillRule="evenodd"
d="M4.293 4.293a1 1 0 011.414 0L10 8.586l4.293-4.293a1 1 0 111.414 1.414L11.414 10l4.293 4.293a1 1 0 01-1.414 1.414L10 11.414l-4.293 4.293a1 1 0 01-1.414-1.414L8.586 10 4.293 5.707a1 1 0 010-1.414z"
clipRule="evenodd"
/>
</svg>
</button>
</div>
) : null}
<div
className={
'honey-nav-container z-20 sticky bg-transparent w-full top-0'
}
>
<div className="honey-nav-container-blur absolute w-full h-full bg-white dark:bg-dark pointer-events-none" />
<nav className="flex gap-2 max-w-[90rem] mx-auto items-center left-0 right-0 h-16 pl-[max(env(safe-area-inset-left),1.5rem)] pr-[max(env(safe-area-inset-right),1.5rem)]">
<div className="flex items-center mr-2 flex-auto">
<Link href="/">
<a className="no-underline text-current inline-flex items-center hover:opacity-75">
{renderComponent(config.logo, { locale })}
</a>
</Link>
</div>
<div className="flex-1" />
{items
? items.map(page => {
if (page.hidden) return null
let href = page.href || page.route || '#'
// If it's a directory
if (page.children) {
href =
(page.withIndexPage ? page.route : page.firstChildRoute) ||
href
}
const isActive =
page.route === activeRoute ||
activeRoute.startsWith(page.route + '/')
return (
<Link href={href} key={page.route}>
<a
className={cn(
'honey-nav-link',
'no-underline whitespace-nowrap p-2 -ml-2 hidden md:inline-block',
isActive
? 'active text-current font-medium'
: 'text-gray-600 hover:text-gray-800 dark:text-gray-400 dark:hover:text-gray-200'
)}
aria-selected={isActive}
{...(page.newWindow
? { target: '_blank', rel: 'noopener noreferrer' }
: {})}
>
{page.title}
</a>
</Link>
)
})
: null}
<div>
<div className="hidden md:inline-block">
{config.customSearch ||
(config.search ? (
config.unstable_flexsearch ? (
<Flexsearch />
) : (
<Search directories={flatDirectories} />
)
) : null)}
</div>
</div>
{config.projectLink || config.github ? (
<a
className="text-current p-2"
href={config.projectLink || config.github}
target="_blank"
rel="noreferrer"
>
{config.projectLinkIcon ? (
renderComponent(config.projectLinkIcon, { locale })
) : (
<React.Fragment>
<GitHubIcon height={24} />
<span className="sr-only">GitHub</span>
</React.Fragment>
)}
</a>
) : null}
{config.projectChatLink ? (
<a
className="text-current p-2"
href={config.projectChatLink}
target="_blank"
rel="noreferrer"
>
{config.projectChatLinkIcon ? (
renderComponent(config.projectChatLinkIcon, { locale })
) : (
<React.Fragment>
<DiscordIcon height={24} />
<span className="sr-only">Discord</span>
</React.Fragment>
)}
</a>
) : null}
<button
className="honey-menu-icon block md:hidden p-2"
onClick={() => setMenu(!menu)}
>
<svg
fill="none"
width="24"
height="24"
viewBox="0 0 24 24"
stroke="currentColor"
className={cn({ open: menu })}
>
<g>
<path
strokeLinecap="round"
strokeLinejoin="round"
strokeWidth={2}
d="M4 6h16"
/>
</g>
<path
strokeLinecap="round"
strokeLinejoin="round"
strokeWidth={2}
d="M4 12h16"
/>
<g>
<path
strokeLinecap="round"
strokeLinejoin="round"
strokeWidth={2}
d="M4 18h16"
/>
</g>
</svg>
</button>
</nav>
</div>
</>
)
} |
import { useRef } from 'react';
import { ColumnDef, flexRender, getCoreRowModel, useReactTable } from '@tanstack/react-table';
import Color from 'color';
import { isOntimeBlock, isOntimeDelay, isOntimeEvent, OntimeRundown, OntimeRundownEntry } from 'ontime-types';
import useFollowComponent from '../../common/hooks/useFollowComponent';
import { useLocalStorage } from '../../common/hooks/useLocalStorage';
import { getAccessibleColour } from '../../common/utils/styleUtils';
import BlockRow from './cuesheet-table-elements/BlockRow';
import CuesheetHeader from './cuesheet-table-elements/CuesheetHeader';
import DelayRow from './cuesheet-table-elements/DelayRow';
import EventRow from './cuesheet-table-elements/EventRow';
import CuesheetTableSettings from './cuesheet-table-settings/CuesheetTableSettings';
import { useCuesheetSettings } from './store/CuesheetSettings';
import { initialColumnOrder } from './cuesheetCols';
import style from './Cuesheet.module.scss';
interface CuesheetProps {
data: OntimeRundown;
columns: ColumnDef<OntimeRundownEntry>[];
handleUpdate: (rowIndex: number, accessor: keyof OntimeRundownEntry, payload: unknown) => void;
selectedId: string | null;
}
export default function Cuesheet({ data, columns, handleUpdate, selectedId }: CuesheetProps) {
const { followSelected, showSettings, showDelayBlock, showPrevious } = useCuesheetSettings();
const [columnVisibility, setColumnVisibility] = useLocalStorage('table-hidden', {});
const [columnOrder, saveColumnOrder] = useLocalStorage<string[]>('table-order', initialColumnOrder);
const [columnSizing, setColumnSizing] = useLocalStorage('table-sizes', {});
const selectedRef = useRef<HTMLTableRowElement | null>(null);
const tableContainerRef = useRef<HTMLDivElement | null>(null);
useFollowComponent({ followRef: selectedRef, scrollRef: tableContainerRef, doFollow: followSelected });
const table = useReactTable({
data,
columns,
columnResizeMode: 'onChange',
state: {
columnOrder,
columnVisibility,
columnSizing,
},
meta: {
handleUpdate,
},
onColumnVisibilityChange: setColumnVisibility,
onColumnSizingChange: setColumnSizing,
getCoreRowModel: getCoreRowModel(),
});
const resetColumnOrder = () => {
saveColumnOrder(initialColumnOrder);
};
const setAllVisible = () => {
table.toggleAllColumnsVisible(true);
};
const resetColumnResizing = () => {
setColumnSizing({});
};
const headerGroups = table.getHeaderGroups();
const rowModel = table.getRowModel();
const allLeafColumns = table.getAllLeafColumns();
let eventIndex = 0;
let isPast = Boolean(selectedId);
return (
<>
{showSettings && (
<CuesheetTableSettings
columns={allLeafColumns}
handleResetResizing={resetColumnResizing}
handleResetReordering={resetColumnOrder}
handleClearToggles={setAllVisible}
/>
)}
<div ref={tableContainerRef} className={style.cuesheetContainer}>
<table className={style.cuesheet}>
<CuesheetHeader headerGroups={headerGroups} />
<tbody>
{rowModel.rows.map((row) => {
const key = row.original.id;
const isSelected = selectedId === key;
if (isSelected) {
isPast = false;
}
if (isOntimeBlock(row.original)) {
return <BlockRow key={key} title={row.original.title} />;
}
if (isOntimeDelay(row.original)) {
const delayVal = row.original.duration;
if (!showDelayBlock || delayVal === 0) {
return null;
}
return <DelayRow key={key} duration={delayVal} />;
}
if (isOntimeEvent(row.original)) {
eventIndex++;
const isSelected = key === selectedId;
if (isSelected) {
isPast = false;
}
if (isPast && !showPrevious) {
return null;
}
let rowBgColour: string | undefined;
if (isSelected) {
rowBgColour = 'var(--cuesheet-running-bg-override, #D20300)'; // $red-700
} else if (row.original.colour) {
try {
// the colour is user defined and might be invalid
const accessibleBackgroundColor = Color(getAccessibleColour(row.original.colour).backgroundColor);
rowBgColour = accessibleBackgroundColor.fade(0.75).hexa();
} catch (_error) {
/* we do not handle errors here */
}
}
return (
<EventRow
key={key}
eventIndex={eventIndex}
isPast={isPast}
selectedRef={isSelected ? selectedRef : undefined}
skip={row.original.skip}
colour={row.original.colour}
>
{row.getVisibleCells().map((cell) => {
return (
<td key={cell.id} style={{ width: cell.column.getSize(), backgroundColor: rowBgColour }}>
{flexRender(cell.column.columnDef.cell, cell.getContext())}
</td>
);
})}
</EventRow>
);
}
// currently there is no scenario where entryType is not handled above, either way...
return null;
})}
</tbody>
</table>
</div>
</>
);
} |
import { isFirefox, isSafari } from './browser';
import { isMacintosh, isWindows } from './platform';
export interface IMouseEvent {
readonly browserEvent: MouseEvent;
readonly leftButton: boolean;
readonly middleButton: boolean;
readonly rightButton: boolean;
readonly buttons: number;
readonly target: HTMLElement;
readonly detail: number;
readonly posx: number;
readonly posy: number;
readonly ctrlKey: boolean;
readonly shiftKey: boolean;
readonly altKey: boolean;
readonly metaKey: boolean;
readonly timestamp: number;
preventDefault(): void;
stopPropagation(): void;
}
export class StandardMouseEvent implements IMouseEvent {
public readonly browserEvent: MouseEvent;
public readonly leftButton: boolean;
public readonly middleButton: boolean;
public readonly rightButton: boolean;
public readonly buttons: number;
public readonly target: HTMLElement;
public detail: number;
public readonly posx: number;
public readonly posy: number;
public readonly ctrlKey: boolean;
public readonly shiftKey: boolean;
public readonly altKey: boolean;
public readonly metaKey: boolean;
public readonly timestamp: number;
constructor(e: MouseEvent) {
this.timestamp = Date.now();
this.browserEvent = e;
this.leftButton = e.button === 0;
this.middleButton = e.button === 1;
this.rightButton = e.button === 2;
this.buttons = e.buttons;
this.target = <HTMLElement>e.target;
this.detail = e.detail || 1;
if (e.type === 'dblclick') {
this.detail = 2;
}
this.ctrlKey = e.ctrlKey;
this.shiftKey = e.shiftKey;
this.altKey = e.altKey;
this.metaKey = e.metaKey;
if (typeof e.pageX === 'number') {
this.posx = e.pageX;
this.posy = e.pageY;
} else {
// Probably hit by MSGestureEvent
this.posx = e.clientX + this.target.ownerDocument.body.scrollLeft + this.target.ownerDocument.documentElement.scrollLeft;
this.posy = e.clientY + this.target.ownerDocument.body.scrollTop + this.target.ownerDocument.documentElement.scrollTop;
}
}
public preventDefault(): void {
this.browserEvent.preventDefault();
}
public stopPropagation(): void {
this.browserEvent.stopPropagation();
}
}
export class DragMouseEvent extends StandardMouseEvent {
public readonly dataTransfer: DataTransfer;
constructor(e: MouseEvent) {
super(e);
this.dataTransfer = (<any>e).dataTransfer;
}
}
export interface IMouseWheelEvent extends MouseEvent {
readonly wheelDelta: number;
readonly wheelDeltaX: number;
readonly wheelDeltaY: number;
readonly deltaX: number;
readonly deltaY: number;
readonly deltaZ: number;
readonly deltaMode: number;
}
interface IWebKitMouseWheelEvent {
wheelDeltaY: number;
wheelDeltaX: number;
}
interface IGeckoMouseWheelEvent {
HORIZONTAL_AXIS: number;
VERTICAL_AXIS: number;
axis: number;
detail: number;
}
export class StandardWheelEvent {
public readonly browserEvent: IMouseWheelEvent | null;
public readonly deltaY: number;
public readonly deltaX: number;
public readonly target: Node;
constructor(e: IMouseWheelEvent | null, deltaX: number = 0, deltaY: number = 0) {
this.browserEvent = e || null;
this.target = e ? (e.target || (<any>e).targetNode || e.srcElement) : null;
this.deltaY = deltaY;
this.deltaX = deltaX;
if (e) {
// Old (deprecated) wheel events
const e1 = <IWebKitMouseWheelEvent><any>e;
const e2 = <IGeckoMouseWheelEvent><any>e;
// vertical delta scroll
if (typeof e1.wheelDeltaY !== 'undefined') {
this.deltaY = e1.wheelDeltaY / 120;
} else if (typeof e2.VERTICAL_AXIS !== 'undefined' && e2.axis === e2.VERTICAL_AXIS) {
this.deltaY = -e2.detail / 3;
} else if (e.type === 'wheel') {
// Modern wheel event
// https://developer.mozilla.org/en-US/docs/Web/API/WheelEvent
const ev = <WheelEvent><unknown>e;
if (ev.deltaMode === ev.DOM_DELTA_LINE) {
// the deltas are expressed in lines
if (isFirefox && !isMacintosh) {
this.deltaY = -e.deltaY / 3;
} else {
this.deltaY = -e.deltaY;
}
} else {
this.deltaY = -e.deltaY / 40;
}
}
// horizontal delta scroll
if (typeof e1.wheelDeltaX !== 'undefined') {
if (isSafari && isWindows) {
this.deltaX = -(e1.wheelDeltaX / 120);
} else {
this.deltaX = e1.wheelDeltaX / 120;
}
} else if (typeof e2.HORIZONTAL_AXIS !== 'undefined' && e2.axis === e2.HORIZONTAL_AXIS) {
this.deltaX = -e.detail / 3;
} else if (e.type === 'wheel') {
// Modern wheel event
// https://developer.mozilla.org/en-US/docs/Web/API/WheelEvent
const ev = <WheelEvent><unknown>e;
if (ev.deltaMode === ev.DOM_DELTA_LINE) {
// the deltas are expressed in lines
if (isFirefox && !isMacintosh) {
this.deltaX = -e.deltaX / 3;
} else {
this.deltaX = -e.deltaX;
}
} else {
this.deltaX = -e.deltaX / 40;
}
}
// Assume a vertical scroll if nothing else worked
if (this.deltaY === 0 && this.deltaX === 0 && e.wheelDelta) {
this.deltaY = e.wheelDelta / 120;
}
}
}
public preventDefault(): void {
this.browserEvent?.preventDefault();
}
public stopPropagation(): void {
this.browserEvent?.stopPropagation();
}
} |
import { useState, useEffect } from 'react';
function Counter() {
// let timeIntro;
const [amplitude, setAmplitude] = useState(1);
const [timeTravel, setTimeTravel] = useState(0);
const [timeIntro, setTimeIntro] = useState('Today is ');
const oneDay = 86400000;
const now = new Date().getTime(); // => e.g. 1699567737723
const handleTimeSubtract = () => {
setTimeTravel((travel) => travel - amplitude);
};
const handleTimeAdd = () => {
setTimeTravel((travel) => travel + amplitude);
};
const handleNumberInput = (e) => {
setTimeTravel(Number(e.target.value));
};
useEffect(() => {
console.log('In UE, timeT:', timeTravel, typeof timeTravel);
const timeIntroPhrase =
timeTravel === 0
? 'Today is '
: timeTravel === 1
? ' day from today is '
: timeTravel === -1
? ' day ago was '
: timeTravel > 1
? ' days from today is '
: ' days ago was ';
setTimeIntro(timeIntroPhrase);
}, [timeTravel]);
return (
<div>
<div className='inline'>
<input type="range" value={amplitude} min="1" max="10" onChange={(e)=> setAmplitude(Number(e.target.value))} />
<span>{ amplitude}</span>
</div>
<div className='inline'>
<button onClick={handleTimeSubtract}>-</button>
<input type='number' value={timeTravel} onChange={handleNumberInput} />
<button onClick={handleTimeAdd}>+</button>
</div>
<p className='phrase'>
{timeTravel !== 0 ? Math.abs(timeTravel) : ''}
{timeIntro}
{new Date(now + oneDay * timeTravel).toDateString()}
</p>
</div>
);
}
export default Counter; |
<?php
namespace App\Http\Controllers;
use App\Http\Requests\FuncionarioRequest;
use App\Models\User;
use App\Repositories\AdminRepository;
use Illuminate\Http\Request;
class AdminController extends Controller
{
public function __construct(private AdminRepository $repository)
{
}
public function admin()
{
setlocale(LC_TIME, 'pt_BR.utf8', 'pt_BR', 'Portuguese_Brazil');
$horarios = $this->repository->admin();
return view('dashboard.index', [
'horarios' => $horarios,
]);
}
public function store(Request $request)
{
$data = $this->repository->store($request);
return redirect()->route('admin', [
'data' => $data,
]);
}
public function mesesIndex()
{
$meses = $this->repository->mesesIndex();
return view('dashboard.meses', [
'meses' => $meses
]);
}
public function fecharMes()
{
$meses = $this->repository->fecharMes();
return redirect()
->route('meses-index', [
'meses' => $meses,
]);
}
public function funcionariosIndex()
{
$users = User::all();
return view('dashboard.funcionarios', [
'users' => $users
]);
}
public function funcionarioDetalhes($id)
{
$horarios = $this->repository->funcionarioDetalhes($id);
return view('dashboard.detalhes-funcionario', [
'id' => $id,
'horarios' => $horarios,
]);
}
public function adicionarFuncionario()
{
return view('dashboard.adicionar-funcionario');
}
public function adicionarFuncionarioPost(FuncionarioRequest $request)
{
$novo_funcionario = $this->repository->adicionarFuncionarioPost($request);
return redirect()->route('funcionarios')->with('message', 'Funcionário(a) cadastrado(a) com sucesso.');
}
public function editarFuncionario($id)
{
$user = $this->repository->editarFuncionario($id);
return view('dashboard.editar-funcionario', [
'user' => $user,
]);
}
public function editarFuncionarioPost(FuncionarioRequest $request)
{
$user = $this->repository->editarFuncionarioPost($request);
return redirect()->route('funcionarios')->with('message', 'Funcionário(a) Editado(a) com sucesso!');
}
public function excluirFuncionario($id)
{
$funcionario = $this->repository->excluirFuncionario($id);
return redirect()->route('funcionarios')->with('message', 'Funcionário(a) Excluído(a) com sucesso!');
}
} |
// import React, { useContext, useState, useEffect, useCallback } from 'react';
// import { withRouter } from 'react-router-dom';
// import {
// InputBase,
// IconButton,
// Popper,
// CircularProgress,
// Grid,
// } from '@material-ui/core';
// import { ArrowBack } from '@material-ui/icons';
// import { GlobalContext } from '../GlobalState';
// import suggestSearch from '../../apis/suggestSearch';
// import AutoSearchResult from './AutoSearchResult';
// import youtubeSearch from '../../apis/youtubeSearch';
// import jsonp from 'jsonp';
// const SearchBox = ({ history, location }) => {
// let params = new URLSearchParams(location.search);
// const [{ searchState }, dispatch] = useContext(GlobalContext);
// const setSearchState = useCallback(
// (data) => {
// dispatch({ type: 'setSearchState', snippet: data });
// },
// [dispatch]
// );
// const setSearchResult = useCallback(
// (data) => {
// // console.log(data);
// dispatch({ type: 'setSearchResult', snippet: data });
// },
// [dispatch]
// );
// const [searchQuery, setSearchQuery] = useState('');
// const [autoSearchData, setAutoSearch] = useState('');
// const [ytSearchQuery, setYtSearchQuery] = useState(null);
// // toggle popper
// const [isPopperOpen, setPopper] = useState(true);
// // console.log('search box re rendered');
// // get back the selected search data
// const onSearchSelect = (result) => {
// setSearchQuery(result);
// setYtSearchQuery(result);
// setSearchState('searching');
// history.push({ pathname: '/search', search: `?q=${result}` });
// };
// // when user hits enter then also fetch the data from yt api
// const onSearchSubmit = (e) => {
// e.preventDefault();
// // console.log(e.target.lastChild);
// e.target.lastChild.lastChild.blur();
// setSearchState('searching');
// setYtSearchQuery(searchQuery);
// history.push({ pathname: '/search', search: `?q=${searchQuery}` });
// };
// const debounce = (func, delay = 150) => {
// let timeId;
// return function () {
// let context = this,
// args = arguments;
// clearTimeout(timeId);
// timeId = setTimeout(() => {
// func.apply(context, args);
// }, delay);
// };
// };
// const getQueryString = (queryParams) => {
// let queryStr = '';
// for (let param in queryParams)
// queryStr += `${param}=${queryParams[param]}&`;
// // remove the last &
// return queryStr.slice(0, -1);
// };
// // for controlled input change
// const onChange = (event) => {
// setSearchQuery(event.target.value);
// debounce((e) => {
// getAutocomplete();
// })(event);
// };
// // get autocomplete data form api
// const getAutocomplete = () => {
// suggestSearch.params.q = searchQuery;
// jsonp(
// suggestSearch.baseURL + getQueryString(suggestSearch.params),
// null,
// (err, response) => {
// setAutoSearch(response[1]);
// }
// );
// };
// // get youtube search result from api
// useEffect(() => {
// const searchYt = async (data) => {
// const res = await youtubeSearch.get('/search', {
// params: {
// q: data,
// maxResults: 15,
// },
// });
// setSearchResult(res.data.items);
// setSearchState('completed');
// };
// // only search if there is any value
// if (ytSearchQuery && ytSearchQuery !== '') {
// searchYt(ytSearchQuery);
// }
// // // console.log(ytSearchQuery);
// }, [ytSearchQuery, setSearchResult, setSearchState]);
// useEffect(() => {
// // console.log('search state', searchState);
// }, [searchState]);
// useEffect(() => {
// // Listen for changes to the current location.
// const query = params.get('q');
// if (query) {
// setYtSearchQuery(query);
// setSearchQuery(query);
// setSearchState('searching');
// // console.log('changing query to', query);
// }
// // const unlisten = history.listen(location => {
// // // setYtSearchQuery(params.get("q"));
// // // we will se the q from params
// // // location is an object like window.location
// // const path = location.pathname;
// // // if (path.slice(1, 7) === "search" || path.slice(1, 5) === "song") {
// // // setSearchState("searched");
// // // } else {
// // // setSearchState("home");
// // // }
// // });
// }, [setSearchState, setYtSearchQuery]);
// // show loading icon while we fetch the results from api
// const popperResult = () => {
// switch (searchState) {
// case 'searching':
// return (
// <Grid
// style={{ height: '100vh' }}
// container
// justify="center"
// alignItems="center"
// >
// <CircularProgress />
// </Grid>
// );
// case 'clicked':
// return (
// <AutoSearchResult
// results={autoSearchData}
// onSearchSelect={onSearchSelect}
// />
// );
// case 'completed':
// setPopper(false);
// break;
// default:
// break;
// }
// // console.log('Function ran');
// };
// return (
// <>
// <IconButton
// onClick={() => {
// setSearchState('home');
// if (history.location.pathname === '/search') {
// history.goBack();
// }
// // only go back if u have searched something
// }}
// color="inherit"
// aria-label="Menu"
// >
// <ArrowBack />
// </IconButton>
// <form style={{ width: '100%' }} onSubmit={(e) => onSearchSubmit(e)}>
// <InputBase
// fullWidth
// placeholder="Search..."
// autoFocus
// style={{ color: '#fff', paddingLeft: '16px' }}
// value={searchQuery}
// onChange={onChange}
// // on click we will show popper
// onClick={() => {
// setSearchState('clicked');
// setPopper(true);
// }}
// />
// </form>
// <Popper
// className="searchPopper"
// open={isPopperOpen}
// anchorEl={document.getElementById('navbar')}
// popperOptions={{
// modifiers: {
// preventOverflow: {
// padding: 0,
// },
// },
// }}
// placement="bottom"
// >
// {popperResult}
// </Popper>
// </>
// );
// };
// export default withRouter(SearchBox); |
import * as React from 'react';
import * as DropdownMenuPrimitive from '@radix-ui/react-dropdown-menu';
import { cn } from '@lib/helpers';
import { RxCheck, RxChevronRight, RxCircle } from 'react-icons/rx';
import { Dialog, DialogContent, DialogTrigger } from './dialog';
import { DialogProps } from '@radix-ui/react-dialog';
const DropdownMenu = DropdownMenuPrimitive.Root;
const DropdownMenuTrigger = DropdownMenuPrimitive.Trigger;
const DropdownMenuGroup = DropdownMenuPrimitive.Group;
const DropdownMenuPortal = DropdownMenuPrimitive.Portal;
const DropdownMenuSub = DropdownMenuPrimitive.Sub;
const DropdownMenuRadioGroup = DropdownMenuPrimitive.RadioGroup;
const DropdownMenuSubTrigger = React.forwardRef<
React.ElementRef<typeof DropdownMenuPrimitive.SubTrigger>,
React.ComponentPropsWithoutRef<typeof DropdownMenuPrimitive.SubTrigger> & {
inset?: boolean;
}
>(({ className, inset, children, ...props }, ref) => (
<DropdownMenuPrimitive.SubTrigger
ref={ref}
className={cn(
'flex cursor-default select-none items-center rounded-sm py-1.5 px-2 text-sm font-medium outline-none focus:bg-slate-100 data-[state=open]:bg-slate-100 dark:focus:bg-slate-700 dark:data-[state=open]:bg-slate-700',
inset && 'pl-8',
className
)}
{...props}
>
{children}
<RxChevronRight className="ml-auto h-4 w-4" />
</DropdownMenuPrimitive.SubTrigger>
));
DropdownMenuSubTrigger.displayName =
DropdownMenuPrimitive.SubTrigger.displayName;
const DropdownMenuSubContent = React.forwardRef<
React.ElementRef<typeof DropdownMenuPrimitive.SubContent>,
React.ComponentPropsWithoutRef<typeof DropdownMenuPrimitive.SubContent>
>(({ className, ...props }, ref) => (
<DropdownMenuPrimitive.SubContent
ref={ref}
className={cn(
'z-50 min-w-[8rem] overflow-hidden rounded-md border border-slate-100 bg-white p-1 text-slate-700 shadow-md animate-in slide-in-from-left-1 dark:border-slate-800 dark:bg-slate-800 dark:text-slate-400',
className
)}
{...props}
/>
));
DropdownMenuSubContent.displayName =
DropdownMenuPrimitive.SubContent.displayName;
const DropdownMenuContent = React.forwardRef<
React.ElementRef<typeof DropdownMenuPrimitive.Content>,
React.ComponentPropsWithoutRef<typeof DropdownMenuPrimitive.Content>
>(({ className, sideOffset = 4, ...props }, ref) => (
<DropdownMenuPrimitive.Portal>
<DropdownMenuPrimitive.Content
ref={ref}
sideOffset={sideOffset}
className={cn(
'z-50 min-w-[8rem] overflow-hidden rounded-md border border-slate-100 bg-white p-2 text-slate-700 shadow-lg animate-in data-[side=right]:slide-in-from-left-2 data-[side=left]:slide-in-from-right-2 data-[side=bottom]:slide-in-from-top-2 data-[side=top]:slide-in-from-bottom-2 dark:border-slate-700 dark:bg-slate-900 dark:text-slate-300',
className
)}
{...props}
/>
</DropdownMenuPrimitive.Portal>
));
DropdownMenuContent.displayName = DropdownMenuPrimitive.Content.displayName;
type DropdownMenuItemElementRef = React.ElementRef<
typeof DropdownMenuPrimitive.Item
>;
type DropdownMenuItemPropsWithoutRef = React.ComponentPropsWithoutRef<
typeof DropdownMenuPrimitive.Item
>;
const DropdownMenuItem = React.forwardRef<
DropdownMenuItemElementRef,
DropdownMenuItemPropsWithoutRef & {
inset?: boolean;
}
>(({ className, inset, ...props }, ref) => (
<DropdownMenuPrimitive.Item
ref={ref}
className={cn(
'relative flex cursor-default select-none items-center rounded-sm py-1.5 px-2 text-sm font-medium outline-none transition-colors duration-150 ease-in focus:bg-slate-100 data-[disabled]:pointer-events-none data-[disabled]:opacity-50 dark:focus:bg-slate-700',
inset && 'pl-8',
className
)}
{...props}
/>
));
DropdownMenuItem.displayName = DropdownMenuPrimitive.Item.displayName;
const DropdownMenuCheckboxItem = React.forwardRef<
React.ElementRef<typeof DropdownMenuPrimitive.CheckboxItem>,
React.ComponentPropsWithoutRef<typeof DropdownMenuPrimitive.CheckboxItem>
>(({ className, children, checked, ...props }, ref) => (
<DropdownMenuPrimitive.CheckboxItem
ref={ref}
className={cn(
'relative flex cursor-default select-none items-center rounded-sm py-1.5 pl-8 pr-2 text-sm font-medium outline-none focus:bg-slate-100 data-[disabled]:pointer-events-none data-[disabled]:opacity-50 dark:focus:bg-slate-700',
className
)}
checked={checked}
{...props}
>
<span className="absolute left-2 flex h-3.5 w-3.5 items-center justify-center">
<DropdownMenuPrimitive.ItemIndicator>
<RxCheck className="h-4 w-4" />
</DropdownMenuPrimitive.ItemIndicator>
</span>
{children}
</DropdownMenuPrimitive.CheckboxItem>
));
DropdownMenuCheckboxItem.displayName =
DropdownMenuPrimitive.CheckboxItem.displayName;
const DropdownMenuRadioItem = React.forwardRef<
React.ElementRef<typeof DropdownMenuPrimitive.RadioItem>,
React.ComponentPropsWithoutRef<typeof DropdownMenuPrimitive.RadioItem>
>(({ className, children, ...props }, ref) => (
<DropdownMenuPrimitive.RadioItem
ref={ref}
className={cn(
'relative flex cursor-default select-none items-center rounded-sm py-1.5 pl-8 pr-2 text-sm font-medium outline-none focus:bg-slate-100 data-[disabled]:pointer-events-none data-[disabled]:opacity-50 dark:focus:bg-slate-700',
className
)}
{...props}
>
<span className="absolute left-2 flex h-3.5 w-3.5 items-center justify-center">
<DropdownMenuPrimitive.ItemIndicator>
<RxCircle className="h-2 w-2 fill-current" />
</DropdownMenuPrimitive.ItemIndicator>
</span>
{children}
</DropdownMenuPrimitive.RadioItem>
));
DropdownMenuRadioItem.displayName = DropdownMenuPrimitive.RadioItem.displayName;
const DropdownMenuLabel = React.forwardRef<
React.ElementRef<typeof DropdownMenuPrimitive.Label>,
React.ComponentPropsWithoutRef<typeof DropdownMenuPrimitive.Label> & {
inset?: boolean;
}
>(({ className, inset, ...props }, ref) => (
<DropdownMenuPrimitive.Label
ref={ref}
className={cn(
'px-2 py-1.5 text-sm font-semibold text-slate-900 dark:text-slate-300',
inset && 'pl-8',
className
)}
{...props}
/>
));
DropdownMenuLabel.displayName = DropdownMenuPrimitive.Label.displayName;
const DropdownMenuSeparator = React.forwardRef<
React.ElementRef<typeof DropdownMenuPrimitive.Separator>,
React.ComponentPropsWithoutRef<typeof DropdownMenuPrimitive.Separator>
>(({ className, ...props }, ref) => (
<DropdownMenuPrimitive.Separator
ref={ref}
className={cn(
'-mx-1 my-1 h-px bg-slate-100 dark:bg-slate-700',
className
)}
{...props}
/>
));
DropdownMenuSeparator.displayName = DropdownMenuPrimitive.Separator.displayName;
const DropdownMenuShortcut = ({
className,
...props
}: React.HTMLAttributes<HTMLSpanElement>) => {
return (
<span
className={cn(
'ml-auto text-xs tracking-widest text-slate-500',
className
)}
{...props}
/>
);
};
DropdownMenuShortcut.displayName = 'DropdownMenuShortcut';
const DropdownMenuDialogItem = React.forwardRef<
DropdownMenuItemElementRef,
DropdownMenuItemPropsWithoutRef & {
trigger: React.ReactNode;
open?: boolean;
onOpenChange?: DialogProps['onOpenChange'];
onSelect?: DropdownMenuPrimitive.DropdownMenuItemProps['onSelect'];
}
>(({ trigger, children, open, onOpenChange, onSelect, ...props }, ref) => {
return (
<Dialog open={open} onOpenChange={onOpenChange}>
<DialogTrigger asChild>
<DropdownMenuItem
ref={ref}
onSelect={(event) => {
event.preventDefault();
onSelect && onSelect(event);
}}
{...props}
>
{trigger}
</DropdownMenuItem>
</DialogTrigger>
<DialogContent>{children}</DialogContent>
</Dialog>
);
});
DropdownMenuDialogItem.displayName = 'DropdownMenuDialogItem';
export {
DropdownMenu,
DropdownMenuTrigger,
DropdownMenuContent,
DropdownMenuItem,
DropdownMenuCheckboxItem,
DropdownMenuRadioItem,
DropdownMenuLabel,
DropdownMenuSeparator,
DropdownMenuShortcut,
DropdownMenuGroup,
DropdownMenuPortal,
DropdownMenuSub,
DropdownMenuSubContent,
DropdownMenuSubTrigger,
DropdownMenuRadioGroup,
DropdownMenuDialogItem,
}; |
import 'package:sixam_mart/util/dimensions.dart';
import 'package:sixam_mart/util/styles.dart';
import 'package:flutter/material.dart';
import 'package:get/get.dart';
class ProfileCard extends StatelessWidget {
final String title;
final String data;
ProfileCard({@required this.data, @required this.title});
@override
Widget build(BuildContext context) {
return Expanded(
child: Container(
height: 100,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(Dimensions.RADIUS_SMALL),
color: Theme.of(context).cardColor,
boxShadow: [
BoxShadow(
color: Colors.grey[Get.isDarkMode ? 800 : 200],
blurRadius: 5,
spreadRadius: 1)
],
),
child: Column(mainAxisAlignment: MainAxisAlignment.center, children: [
Text(data,
style: robotoMedium.copyWith(
fontSize: Dimensions.fontSizeExtraLarge,
color: Theme.of(context).primaryColor,
)),
SizedBox(height: Dimensions.PADDING_SIZE_SMALL),
Text(title,
style: robotoRegular.copyWith(
fontSize: Dimensions.fontSizeSmall,
color: Theme.of(context).disabledColor,
)),
]),
));
}
} |
//
// SettingsTableView.swift
// ClipPic
//
// Created by RayShin Lee on 2022/6/29.
//
import UIKit
protocol SettingsTableViewDelegate: AnyObject {
func showAccountSettingVC()
func signOut()
func deleteAccount()
}
class SettingsTableView: UITableView {
// MARK: - Properties
weak var interactionDelegate: SettingsTableViewDelegate?
// MARK: - View life cycle
init(frame: CGRect) {
super.init(frame: frame, style: .plain)
self.backgroundColor = .systemBackground
register(SettingTableViewCell.self, forCellReuseIdentifier: "settingCell")
dataSource = self
delegate = self
}
required init?(coder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
// MARK: - UITableView
extension SettingsTableView: UITableViewDataSource, UITableViewDelegate {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 3
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = self.dequeueReusableCell(withIdentifier: "settingCell", for: indexPath)
guard let settingCell = cell as? SettingTableViewCell else {
return cell
}
switch indexPath.row {
case 0:
settingCell.settingOptionLabel.text = "Edit Profile"
case 1:
settingCell.settingOptionLabel.text = "Sign Out"
case 2:
settingCell.settingOptionLabel.text = "Delete Account"
default:
assert(false)
}
return settingCell
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return 50
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
switch indexPath.row {
case 0:
interactionDelegate?.showAccountSettingVC()
case 1:
interactionDelegate?.signOut()
case 2:
interactionDelegate?.deleteAccount()
default:
fatalError("didSelectRowAt error")
}
}
} |
import React from "react";
import TextField from "@material-ui/core/TextField";
import useStyles from "../Styles";
interface NumberInputComponentProps {
value: number;
onChange: (value: number) => void;
label: string;
max: number;
}
const NumberInputComponent: React.FC<NumberInputComponentProps> = ({
value,
onChange,
label,
max,
}) => {
const classes = useStyles();
return (
<TextField
type="text"
label={label}
variant="outlined"
value={value}
onChange={(e) => {
const input = e.target.value;
if (/^\d*$/.test(input)) {
onChange(Number(input));
}
}}
inputProps={{ min: 1, max }}
className={`${classes.numberInput} ${classes.adultsInput}`}
/>
);
};
export default NumberInputComponent; |
import React, { useState } from "react";
import { FaTrashAlt, FaPencilAlt } from "react-icons/fa";
import { editEvent } from "/src/features/events";
import styles from "./EventEntry.module.css";
const EventEntry = ({ event, onDelete }) => {
const [isEditing, setIsEditing] = useState(false);
const [linkText, setLinkText] = useState(event.link_text || "");
const [title, setTitle] = useState(event.title || "");
const [description, setDescription] = useState(event.description || "");
const [pdfFile, setPdfFile] = useState(null);
const handleEditToggle = () => setIsEditing(!isEditing);
const handleSubmit = async (e) => {
e.preventDefault();
try {
await editEvent(event.id, title, description, pdfFile, linkText);
setIsEditing(false);
} catch (error) {
console.error("Error editing event:", error);
}
};
return (
<div className={`${styles.card} ${isEditing ? styles.editing : ""}`}>
<div className={styles.infoContainer}>
<div className={styles.actions}>
<button className={styles.actionButton} onClick={handleEditToggle}>
<FaPencilAlt className={styles.actionIcon} />
</button>
<button className={styles.actionButton} onClick={() => onDelete(event.id)}>
<FaTrashAlt className={styles.actionIcon} />
</button>
</div>
<h4 className={styles.date}>{formatDate(event.start_date)}</h4>
<p className={styles.description}>{event.title || "No description available"}</p>
</div>
<form className={styles.editForm} onSubmit={handleSubmit}>
<p className={styles.instructions}>Modify the event title and description below</p>
<label htmlFor="eventTitle" className={styles.label}>
Event Title
</label>
<input
id="eventTitle"
type="text"
value={title}
onChange={(e) => setTitle(e.target.value)}
className={styles.textField}
placeholder="Event Title"
required
/>
<label htmlFor="eventDescription" className={styles.label}>
Event Description
</label>
<textarea
id="eventDescription"
value={description}
onChange={(e) => setDescription(e.target.value)}
className={styles.textArea}
placeholder="Event Description"
required
/>
<p className={styles.instructions}>Attach pdf and provide link text (optional)</p>
<input
type="file"
accept="application/pdf"
className={styles.fileInput}
onChange={(e) => setPdfFile(e.target.files[0])}
/>
<input
type="text"
value={linkText}
onChange={(e) => setLinkText(e.target.value)}
className={styles.textField}
placeholder="Link text"
/>
<button type="submit" className={styles.submitButton}>
Submit
</button>
</form>
</div>
);
};
function formatDate(dateString) {
const options = { year: "numeric", month: "long", day: "numeric" };
return new Intl.DateTimeFormat("en-US", options).format(new Date(dateString));
}
export default EventEntry; |
import type { SharedType } from '@maybe-finance/shared'
import { useAccountContext, BrowserUtil } from '@maybe-finance/client/shared'
import { NumberUtil } from '@maybe-finance/shared'
import { DateTime } from 'luxon'
import toast from 'react-hot-toast'
import { RiAddLine, RiPencilLine } from 'react-icons/ri'
import { LoanCard } from './LoanCard'
export type LoanDetailProps = {
account: SharedType.AccountDetail
showComingSoon?: boolean
}
export function LoanDetail({ account, showComingSoon = false }: LoanDetailProps) {
const { loan } = account
const { editAccount } = useAccountContext()
return (
<div data-testid="loan-detail-cards">
<div className="flex justify-between items-center mb-4">
<h5 className="uppercase">Loan Overview</h5>
<button
className="flex items-center px-2 py-1 font-medium bg-gray-500 rounded hover:bg-gray-400"
onClick={() => {
if (!account) {
toast.error('Unable to edit loan')
return
}
editAccount(account)
}}
>
{!loan ? (
<>
<RiAddLine className="w-5 h-5 text-gray-50" />
<span className="ml-2 text-base">Add terms</span>
</>
) : (
<>
<RiPencilLine className="w-5 h-5 text-gray-50" />
<span className="ml-2 text-base">Edit terms</span>
</>
)}
</button>
</div>
<div className="relative grid grid-cols-1 gap-4 md:grid-cols-2 xl:grid-cols-3">
<LoanCard
isLoading={false}
title="Loan amount"
info="The contractual starting balance for this loan"
detail={
loan
? {
metricValue: NumberUtil.format(
loan.originationPrincipal,
'currency'
),
metricDetail: `Originated on ${DateTime.fromISO(
loan.originationDate ?? ''
).toFormat('MMM d, yyyy')}`,
}
: undefined
}
/>
<LoanCard
isLoading={false}
title="Remaining balance"
info="The remaining balance on this loan"
detail={
loan && loan.originationPrincipal && account.currentBalance
? {
metricValue: NumberUtil.format(
account.currentBalance.toNumber(),
'currency'
),
metricDetail: `${NumberUtil.format(
loan.originationPrincipal - account.currentBalance.toNumber(),
'currency'
)} principal already paid`,
}
: undefined
}
/>
<LoanCard
isLoading={false}
title="Loan terms"
info="The details of your loan contract"
detail={
loan && loan.originationDate && loan.maturityDate && loan.interestRate
? {
metricValue: BrowserUtil.formatLoanTerm(loan),
metricDetail:
loan.interestRate.type !== 'fixed'
? 'Variable rate loan'
: loan.interestRate.rate
? `Fixed rate, ${NumberUtil.format(
loan.interestRate.rate,
'percent',
{
signDisplay: 'auto',
minimumFractionDigits: 2,
maximumFractionDigits: 2,
}
)} interest annually`
: 'Fixed rate loan',
}
: undefined
}
/>
{!loan && (
<div className="absolute inset-0 flex flex-col items-center justify-center w-full h-full text-center bg-black rounded bg-opacity-90 backdrop-blur-sm">
<div className="text-gray-50 text-base max-w-[400px]">
<p>This is where your loan details will show. </p>
<p>
<button
className="text-white hover:text-opacity-90"
onClick={() => editAccount(account)}
>
Add loan terms
</button>{' '}
to see both the chart and these metrics.
</p>
</div>
</div>
)}
</div>
{showComingSoon && (
<div className="p-10 my-10">
<div className="flex flex-col items-center max-w-lg mx-auto text-center">
<img alt="Maybe" width={74} height={60} src="/assets/maybe-gray.svg" />
<h4 className="mt-3 mb-2">
This view is kinda empty, but there's a reason for that
</h4>
<p className="text-base text-gray-50">
We are constantly reviewing and prioritizing your feedback, and we know
you're itching to see some more details about your loan! Hold tight,
this feature is on our roadmap and we're getting to it as quickly as we
can!
</p>
</div>
</div>
)}
</div>
)
} |
import axios, { AxiosResponse } from 'axios';
import { queryToString } from '../../utils/binanceUtils';
const url = 'https://testnet.binancefuture.com';
export enum OrderSide {
BUY = 'BUY',
SELL = 'SELL',
BOTH = 'BOTH',
}
export enum OrderType {
LIMIT = 'LIMIT',
MARKET = 'MARKET',
TAKE_PROFIT = 'TAKE_PROFIT',
STOP_MARKET = 'STOP_MARKET',
TAKE_PROFIT_MARKET = 'TAKE_PROFIT_MARKET',
TRAILING_STOP_MARKET = 'TAKE_PROFIT_MARKET',
}
export interface Key {
apiKey: string;
apiSecret: string;
}
export interface ApiCreateOrder extends Key {
symbol: string;
side: OrderSide;
price: number;
quantity: number;
stopLoss: number;
type?: OrderType;
}
export interface ApiGetPositions extends Key {
symbol?: string;
}
export interface ApiCloseOrder extends Key {
symbol: string;
ids?: string[];
}
export interface ApiClosePosition extends Key {
symbol: string;
side: OrderSide;
price: number;
quantity: number;
}
export const GetBalance = async (key: Key) => {
const { apiKey, apiSecret } = key;
const headers = {
'X-MBX-APIKEY': apiKey,
};
const { data } = await axios.get(
`${url}/fapi/v2/balance?${queryToString(apiSecret, {}, true)}`,
{ headers }
);
console.log('balance:', data);
return data;
};
export const GetPositions = async (position: ApiGetPositions) => {
const { symbol, apiKey, apiSecret } = position;
const headers = {
'X-MBX-APIKEY': apiKey,
};
const payload = {};
if (symbol) Object.assign(payload, { symbol });
const { data } = await axios.get(
`${url}/fapi/v2/positionRisk?${queryToString(apiSecret, payload, true)}`,
{ headers }
);
return data;
};
export const CreateOrder = async (signal: ApiCreateOrder) => {
const {
symbol,
side,
price,
type = OrderType.LIMIT,
quantity,
// stopLoss,
apiKey,
apiSecret,
} = signal;
const headers = {
'X-MBX-APIKEY': apiKey,
};
const payload = {
symbol,
side,
quantity,
price,
type,
timeInForce: 'GTC',
};
try {
const { data: orderResult } = await axios.post(
`${url}/fapi/v1/order?${queryToString(apiSecret, payload, true)}`,
null,
{ headers }
);
console.log('createOrderResult:', orderResult);
return orderResult;
} catch (error) {
console.log(error);
}
};
export const CancelOrders = async (order: ApiCloseOrder) => {
const { apiKey, apiSecret, symbol, ids = [] } = order;
if (ids.length === 0) return [];
const headers = {
'X-MBX-APIKEY': apiKey,
};
let response: AxiosResponse;
if (ids.length > 1) {
const payload = {
symbol,
orderIdList: `[${ids.join(',')}]`,
};
response = await axios.delete(
`${url}/fapi/v1/batchOrders?${queryToString(apiSecret, payload, true)}`,
{ headers }
);
return response.data;
} else {
const payload = {
symbol,
orderId: ids[0],
};
response = await axios.delete(
`${url}/fapi/v1/order?${queryToString(apiSecret, payload, true)}`,
{ headers }
);
return [response.data];
}
};
export const ClosePosition = async (position: ApiClosePosition) => {
const { symbol, side, apiKey, apiSecret, quantity } = position;
if (quantity === 0) return;
const headers = {
'X-MBX-APIKEY': apiKey,
};
const payload = {
symbol,
side,
type: OrderType.MARKET,
quantity: side === OrderSide.BUY ? -quantity : quantity,
reduceOnly: true,
};
const { data: orderResult } = await axios.post(
`${url}/fapi/v1/order?${queryToString(apiSecret, payload, true)}`,
null,
{ headers }
);
console.log('closedPositionResult:', orderResult);
return orderResult;
}; |
#pragma once
#include <visibility.h>
#include <defs/MdsInterface.hpp>
#include <defs/MeasurementObjectDefs.hpp>
#include <defs/Receiver.hpp>
#include <set>
#include <mutex>
#include <functional>
#include <string>
#include <vector>
#include <defs/Log.hpp>
#ifdef _WIN32
#ifdef WRITE_API
class WRITE_API std::thread;
class WRITE_API std::mutex;
template class WRITE_API std::vector<double>;
template class WRITE_API std::vector<char>;
template class WRITE_API std::vector<std::string>;
template class WRITE_API std::allocator<std::string>;
template class WRITE_API std::allocator<char>;
template class WRITE_API std::allocator<std::basic_string<char, std::char_traits<char>, std::allocator<char> > >;
template class WRITE_API std::vector<std::basic_string<char, std::char_traits<char>, std::allocator<char> >, std::allocator<std::basic_string<char, std::char_traits<char>, std::allocator<char> > > >;
class WRITE_API std::string;
#endif
#endif
namespace visualizer
{
class WRITE_API WriteFile :
public MeasurementObject,
public DataReceiverObject,
public InterfaceAccess,
public ObjectControl,
public GuiControlIfc
{
InterfaceAccess* interfaceAccess_;
uint8_t instanceNb_;
uint64_t handle_;
std::string name_;
MeasurementObjectType type_;
LoggingInterface *logger_;
bool isProcessing_;
bool showGui_;
std::mutex mtx_;
std::ofstream arduinoFile_;
std::ofstream webcamFile_;
public:
WriteFile(InterfaceAccess* interfaceAccess, uint8_t nb, const std::string& name, std::function<uint64_t(uint8_t, MeasurementObjectType)> handle);
virtual ~WriteFile();
//! MeasurementObject interface implementation
virtual const uint8_t& getInstanceNumber();
virtual const uint64_t& getHandle();
virtual const MeasurementObjectType& getType();
virtual const std::string& getName();
//! DataReceiverObject interface implementation
virtual bool validatePackage(DataPackageCPtr package);
//! InterfaceAccess interface implementation
virtual void* getInterface(const std::string& interfaceName);
virtual void initializeObject() override;
virtual void terminateObject() override;
void show(ImGuiContext* ctx) override;
void writeArduinoPackage(DataPackageCPtr pkg);
void writeWebcamPackage(DataPackageCPtr pkg);
};
}
extern "C" WRITE_API MeasurementObjectPtr createMO(InterfaceAccess* interfaceAccess, const uint8_t instanceNb, const char* name, std::function<uint64_t(uint8_t, MeasurementObjectType)> handleFunc)
{
return new visualizer::WriteFile(interfaceAccess, instanceNb, name, handleFunc);
} |
# Java集合(下)
## Map集合体系
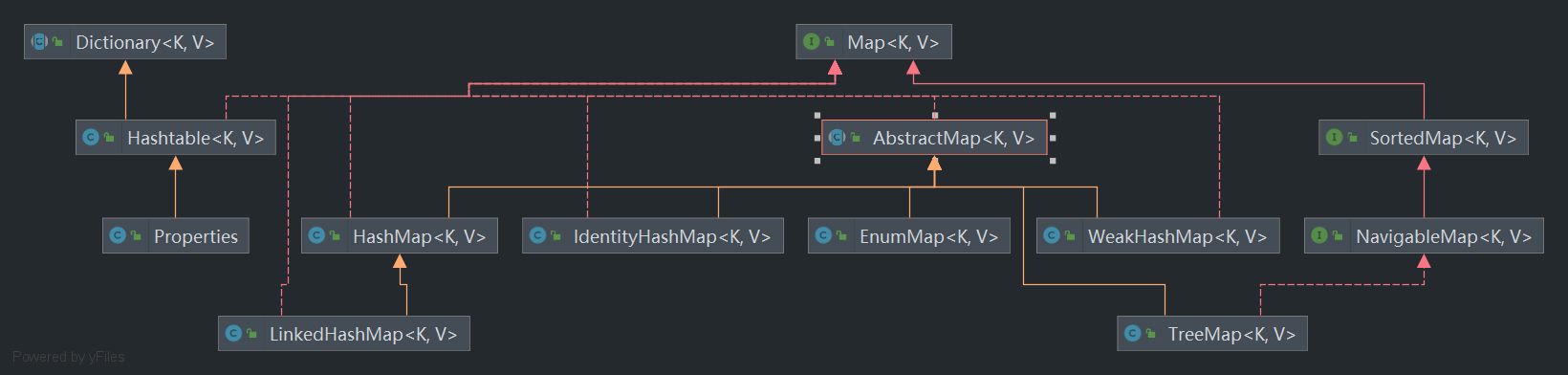
Map集合可以将对象映射到其它对象上,即映射关系(key-value),并且它和数组与Collection一样很容易扩展到多维,很容易通过容器组合来生成强大的数据结构。Map其实就相当于一个映射表(关联数组),其基本思想是它维护的键值对关联,因此我们可以通过键来查找值
**Map集合特点:**
* Map集合的特点都是由键决定的
* Map集合的键是无序,不重复的,无索引的,值不做要求(可以重复)
* Map集合后面重复的键对应的值会覆盖前面重复键的值
* Map集合的键值对都可以为null
```java
public class MapTest1 {
public static void main(String[] args) {
Map<Integer,Integer> map = new HashMap<>();
map.put(null,null);
map.forEach((k,v)->{
System.out.println(k+" "+v);//null null
});
map.put(null,666);
map.put(1,555);
map.put(0,999);
map.forEach((k,v)->{
//null 666 //覆盖了前面的值
//0 999
//1 555
System.out.println(k+" "+v);
});
}
}
```
**Map结构的理解:**
* Map中的key:无序的,不可重复的,使用set存储所有的key,key所在的类要重写equals()和hashCode(), (以hashMap为例)。
* Map中的value:无序的,可重复的,使用Collection存储所有的value, value所在的类要重写equals()
* 一个键值对key-value,构成了一个Entry对象
* Map中的Entry是无序的,不可重复的,使用set存储所有的entry
### Map的存在
```java
public interface Map<K,V>
```
Java标准类库中包含了`Map`的几种实现,包括`HashMap`,`TreeMap`,`LinkedHashMap`等,它们都拥有同样的基本接口,这表现在效率,键值对的保存及呈现次序,对象的保存周期,映射表如何在多线程中工作和判定“键”等价的策略等方面。
`Map`这个接口取代了`Dictionary`类,`Dictionary`类是一个完全抽象的类,而不是一个接口。`Map`接口提供三个集合视图,它们允许将映射的内容视为一组键、值的集合或键-值映射的集合。映射的顺序定义为映射集合视图上的迭代器返回其元素的顺序。
`Map`接口与`Collection`一样,是其一系列集合的根目录,是双列集合最深层次的抽象,制定了最初的标准
以下列举了Map中的大部分接口
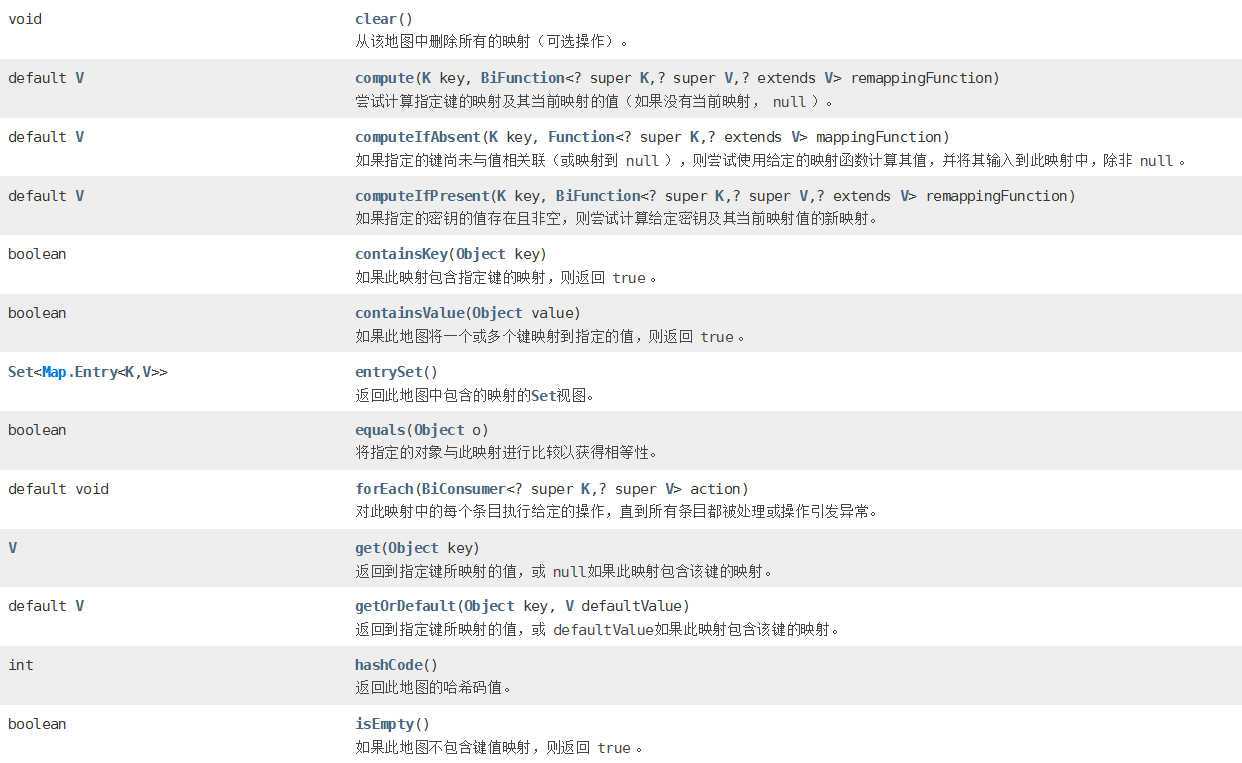
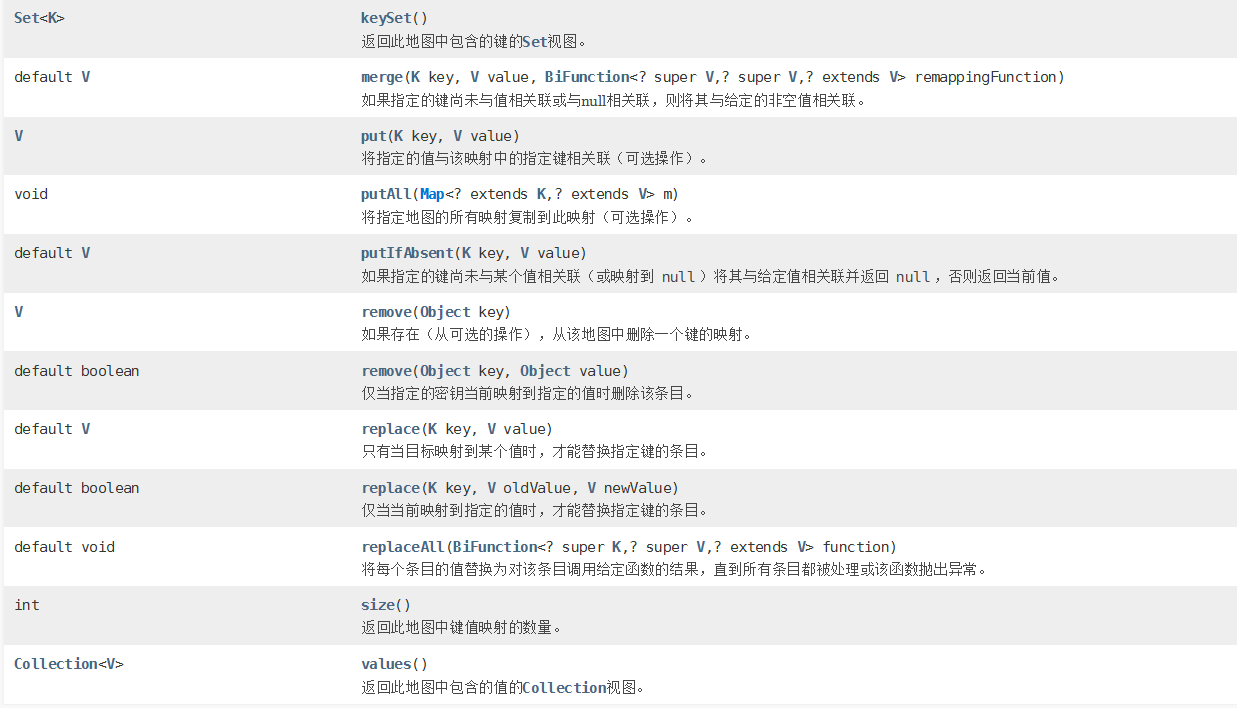
`default void forEach(BiConsumer<? super K, ? super V> action)`用于遍历Map集合,在Map接口中提供了默认实现。`BiConsumer`接受两个输入参数并且不返回结果。 这是`Consumer`的二元专业化 ,可以说是用于保存要执行代码
```java
default void forEach(BiConsumer<? super K, ? super V> action) {//遍历Map集合
Objects.requireNonNull(action);
for (Map.Entry<K, V> entry : entrySet()) {
K k;
V v;
try {
k = entry.getKey();//获取key值
v = entry.getValue();//获取value值
} catch(IllegalStateException ise) {
throw new ConcurrentModificationException(ise);
}
action.accept(k, v);//对给定的参数执行此操作
}
}
```
```java
public class MapTest2 {
public static void main(String[] args) {
Map<Integer,Integer> map = new HashMap<>();
for (int i = 0; i < 5; i++) {
map.put(i,i);
}
map.forEach((k,y)->{
System.out.println(k+" "+y);
});
}
}
```
`action.accept(k, v)`会执行`System.out.println(k+" "+y);`这条语句,当然这里用到的是`HashMap`中重写后的`forEach`方法
在Map接口下还存在一个接口
```java
interface Entry<K,V>
```
映射条目(键-值对)。entrySet方法返回映射的集合视图,其元素属于这个类。获取映射条目引用的唯一方法是从这个集合视图的迭代器中获取。条目对象仅在迭代期间有效;更正式的说法是,如果迭代器在返回映射项之后修改了备份映射,则映射项的行为是未定义的,除非通过映射项上的setValue操作。
* `public static <K extends Comparable<? super K>, V> Comparator<Map.Entry<K,V>> comparingByKey()`:返回比较Map的比较器。按键位自然顺序进入。返回的比较器是可序列化的,并且在比较条目与空键时抛出NullPointerException异常。
```java
public static <K extends Comparable<? super K>, V> Comparator<Map.Entry<K,V>> comparingByKey() {
return (Comparator<Map.Entry<K, V>> & Serializable)
(c1, c2) -> c1.getKey().compareTo(c2.getKey());
}
```
从函数名可以得知它的主要作用应该是按照 key 自然排序,并且这是一个泛型方法,主要说明 K 的上限是 Comparable (K必须实现这个接口)不过return语句中,有这么一个&符号确实出乎意料, 盲猜这里应该得同时实现 Comparator 和Serializable接口。
然后就是一个常见的 lambda 表达式了,重写了 Comparator 中的 compare 方法
```java
public class MapTest2 {
public static void main(String[] args) {
Map<String,Integer> map = new HashMap<>();
for (int i = 5; i > 0; i--) {
map.put(i+"",i);
}
map.put("a",0);
map.put("b",0);
map.put("c",0);
List<Map.Entry<String, Integer>> collect = map.entrySet().stream().sorted(Map.Entry.comparingByKey()).collect(
Collectors.toList()
);
System.out.println(map);//{1=1, a=0, 2=2, b=0, 3=3, c=0, 4=4, 5=5}
System.out.println(collect);//[1=1, 2=2, 3=3, 4=4, 5=5, a=0, b=0, c=0]
}
}
```
* `public static <K, V> Comparator<Map.Entry<K, V>> comparingByValue(Comparator<? super V> cmp)`:返回比较Map的比较器。使用给定的Comparator按值输入。如果指定的比较器也是可序列化的,则返回的比较器是可序列化的。
```java
public static <K, V> Comparator<Map.Entry<K, V>> comparingByValue(Comparator<? super V> cmp) {
Objects.requireNonNull(cmp);
return (Comparator<Map.Entry<K, V>> & Serializable)
(c1, c2) -> cmp.compare(c1.getValue(), c2.getValue());
}
```
### AbstractMap的存在
```java
public abstract class AbstractMap<K,V> implements Map<K,V>
```
该类提供`Map`接口的框架实现,以尽量减少实现该接口所需的工作。要实现一个不可修改的映射,程序员只需要扩展这个类并为entrySet方法提供一个实现,该方法返回映射的集合视图。通常,返回的集合将依次在`AbstractSet`之上实现。这个集合不应该支持添加或删除方法,它的迭代器也不应该支持删除方法。
要实现可修改的映射,必须额外覆盖该类的put方法(否则会抛出`UnsupportedOperationException`), `entrySet().iterator()`返回的迭代器必须额外实现它的`remove`方法。按照map接口规范中的建议,程序员通常应该提供一个void(无参数)和映射构造函数。
一些基本的方法与`AbstractCollection`中类似
* `public boolean containsValue(Object value)`:如果此映射将一个或多个键映射到指定的值,则返回`true`
```java
public boolean containsValue(Object value) {
Iterator<Entry<K,V>> i = entrySet().iterator();//获取set集合的迭代器,set集合存储Entry,Entry存储键值对
if (value==null) {//为空使用==比较
while (i.hasNext()) {
Entry<K,V> e = i.next();
if (e.getValue()==null)
return true;
}
} else {//不为空使用equals方法比较值(重写过)
while (i.hasNext()) {
Entry<K,V> e = i.next();
if (value.equals(e.getValue()))
return true;
}
}
return false;
}
```
* `public boolean equals(Object o)`:将指定的对象与此映射进行比较以获得相等性,这是容器间的比较
```java
public boolean equals(Object o) {
if (o == this)//同一引用
return true;
if (!(o instanceof Map))
return false;
Map<?,?> m = (Map<?,?>) o;//转型
if (m.size() != size())//键值对数不同
return false;
try {
Iterator<Entry<K,V>> i = entrySet().iterator();//获取键值对的迭代器
while (i.hasNext()) {//遍历比较
Entry<K,V> e = i.next();
K key = e.getKey();
V value = e.getValue();
//键值对需要对应相同
if (value == null) {//value为null使用==进行比较
if (!(m.get(key)==null && m.containsKey(key)))
return false;
} else {//不为null使用equals进行比较(重写后的)
if (!value.equals(m.get(key)))
return false;
}
}
} catch (ClassCastException unused) {
return false;
} catch (NullPointerException unused) {
return false;
}
return true;
}
```
* `public int hashCode()`:返回此Map的哈希码值,Map的哈希码被定义为`entrySet()`视图中每个条目的哈希码的总和。 这确保了`m1.equals(m2)`时`m1.hashCode()==m2.hashCode()`
```java
public int hashCode() {
int h = 0;
Iterator<Entry<K,V>> i = entrySet().iterator();
while (i.hasNext())
h += i.next().hashCode();
return h;
}
```
**在`AbstractMap`接口中还存在两个类**
```java
public static class SimpleEntry<K,V> implements Entry<K,V>, java.io.Serializable
```
实现类`Entry<K,V>`方法,维护键和值的条目。value值可以使用setValue方法更改。该类简化了构建自定义映射实现的过程。例如,在`Map.entrySet().toarray`方法中可以很方便返回`SimpleEntry`实例的数组。
```java
public int hashCode() {
return (key == null ? 0 : key.hashCode()) ^
(value == null ? 0 : value.hashCode());
}
```
对key和value对应的哈希值执行异或操作(相应的位相同为0,不相同为1)
```java
public static class SimpleImmutableEntry<K,V> implements Entry<K,V>, java.io.Serializable
```
一种保持不可变键和值的条目。这个类不支持方法`setValue`。这个类在返回键-值映射的线程安全快照的方法中可能很方便。
### HashMap
#### 底层数据结构
**JDK8之前的,底层使用数组+链表组成,JDK8开始后,底层采用数组+链表+红黑树组成。**
##### 数组
数组(Array)是有序的元素序列。有限个类型相同的变量的集合
```java
//在第一次使用时初始化,并根据需要调整大小。
//分配时,长度总是2的幂。允许某些操作的长度为0,以允许当前不需要的引导机制
transient Node<K,V>[] table;
```
**为什么长度总是2的幂?**
为了能让 HashMap 存取高效,尽量较少碰撞,也就是要尽量把数据分配均匀。我们上面也讲到了过了,Hash 值的范围值-2147483648 到 2147483647,前后加起来大概 40 亿的映射空间,只要哈希函数映射得比较均匀松散,一般应用是很难出现碰撞的。但是一个 40 亿长度的数组,内存是放不下的。所以这个散列值是不能直接拿来用的。用之前还要先做对数组的长度取模运算,得到的余数才能用来要存放的位置也就是对应的数组下标。
我们首先可能会想到采用`%`取余的操作来实现,`hash%length`。但是取余运算效率并不高。同时可以发现*取余(%)操作中如果除数是 2 的幂次则等价于与其除数减一的与(&)操作,即`hash%length==hash&(length-1)`的前提是 length 是 2 的 n 次方。* 并且 *采用二进制位操作 &,相对于%能够提高运算效率*。
所以最终为了提高运算效率,使用`&`操作来代替`%`(用`hash & (n - 1)`来代替`hash % n`),HashMap 的必须是 2 的幂次方(n 代表数组长度)。

**还有一点就是在`HashMap`扩容的时候可以保证同一个桶中的元素均匀的散列到新的桶中,具体一点就是同一个桶中的元素在扩容后一半留在原先的桶中,一半放到了新的桶中。(下面说)**
**取余(%)操作中如果除数是 2 的幂次则等价于与其除数减一的与(&)操作,为什么?**
2的幂次在使用二进制表示时,第一位总是1,而剩下的都为0。如 4的二进制为`100`,8的二进制为`1000`
2的幂次-1在使用二进制表示时,所有位都是1,如4-1=3对应的二进制为`11`,8-1=7对应的二进制为`111`
`&`,与操作对应位同时为1则为1,否则为0,所以对2的幂次-1进行与操作其实就是*将最高位抛弃,低位保留*,这与取余操作原理相同
##### 链表
链表是一种物理存储单元上非连续、非顺序的存储结构,数据元素的逻辑顺序是通过链表中的指针链接次序实现的。链表由一系列结点(链表中每一个元素称为结点)组成,结点可以在运行时动态生成。每个结点包括两个部分:一个是存储数据元素的数据域,另一个是存储下一个结点地址的指针域。
```java
static class Node<K,V> implements Map.Entry<K,V> {
final int hash;
final K key;
V value;
Node<K,V> next;
Node(int hash, K key, V value, Node<K,V> next) {
this.hash = hash;
this.key = key;
this.value = value;
this.next = next;
}
public final K getKey() { return key; }
public final V getValue() { return value; }
public final String toString() { return key + "=" + value; }
public final int hashCode() {
return Objects.hashCode(key) ^ Objects.hashCode(value);
}
public final V setValue(V newValue) {
V oldValue = value;
value = newValue;
return oldValue;
}
public final boolean equals(Object o) {
if (o == this)
return true;
if (o instanceof Map.Entry) {
Map.Entry<?,?> e = (Map.Entry<?,?>)o;
if (Objects.equals(key, e.getKey()) &&
Objects.equals(value, e.getValue()))
return true;
}
return false;
}
}
```
**“拉链法”** :将链表和数组相结合。也就是说创建一个链表数组,数组中每一格就是一个链表。若遇到哈希冲突,则将冲突的值加到链表中即可。



##### 红黑树
**二叉查找树**
*概述:*
二叉排序树(Binary Sort Tree),又称二叉查找树(Binary Search Tree),亦称二叉搜索树.是数据结构中的一类.在一般情况下,查询效率比链表结构要高
*定义:*
* 一棵二叉查找树是一棵二叉树,每个节点都含有一个Comparable的键(以及对应的值)
* 每个节点的键都大于左子树中任意节点的键而小于右子树中任意节点的键
* 每个节点都有两个链接,左链接、右链接,分别指向自己的左子节点和右子节点,链接也可以指向null
* 尽管链接指向的是节点,可以将每个链接看做指向了另一棵二叉树.这个思路能帮助理解二叉查找树的递归方法
*二叉查找树的特性:*
* 左子树上所有结点的值均小于或等于它的根结点的值
* 右子树上所有结点的值均大于或等于它的根结点的值
* 左、右子树也分别为二叉排序树
*二叉查找树的优点:*
二叉排序树是一种比较有用的折衷方案(采用了二分查找的思想),数组的搜索比较方便,可以直接用下标,但删除或者插入某些元素就比较麻烦;链表与之相反,删除和插入元素很快,但查找很慢。二叉排序树就既有链表的好处,也有数组的好处,在处理大批量的动态的数据是比较有用
*二叉查找树的缺点:*
顺序存储可能会浪费空间(在非完全二叉树的时候),但是读取某个指定的节点的时候效率比较高O(0)
链式存储相对二叉树比较大的时候浪费空间较少,但是读取某个指定节点的时候效率偏低O(nlogn)
在插入新节点可能会出现一些极端的情况失去平衡,查找效率接近线性查找

**红黑树**
红黑树是一种特化的AVL树平衡二叉树,都是在进行插入和删除操作时通过特定操作保持二叉查找树的平衡,从而获得较高的查找性能。
红黑树虽然很复杂,但它的最坏情况运行时间也是非常良好的,并且在实践中是高效的: 它可以在O(log n)时间内做查找,插入和删除(n 为树中元素的数目)。
红黑树每个结点上增加一个存储位表示结点的颜色,可以是Red或Black。 通过对任何一条从根到叶子的路径上各个结点着色方式的限制,**红黑树确保没有一条路径会比其他路径长出俩倍,因而是接近平衡的。**
**性质:**
1、根节点是黑色的
2、每个节点不是红色的就是黑色的
3、红色节点的子节点一定是黑色的
4、红色节点不能相连
5、从根节点到叶节点或空子节点的每条路径,必须包含相同数目的黑色节点(即相同的黑色高度)
**红黑树的具体实现后面再说吧呜呜呜**
#### 哈希表
**什么是哈希表?**
根据设定的哈希函数H(key)和处理冲突的方法将一组关键字映射到一个有限的连续的地址集(区间)上,并以关键字在地址集中的“像”作为记录在表中的位置,这种表变被称为哈希表,这一映像过程称为哈希造表或*散列*,所得存储位置称为哈希地址或散列地址。
哈希表的查找效率非常高(拿空间换时间)
**哈希函数的构造方法**
* 直接定址法
取关键字或者关键字的某个线性函数值为哈希地址。对于不同的关键字不会发生冲突,但实际中很少使用
* 数字分析法
假设关键字是以r为基的数(如以10为基的十进制数),并且哈希表中可能出现的关键字都是事先知道的,则可以取关键字的若干数位组成哈希地址
* 平方取中法
取关键字平方后的中间几位为哈希地址。取的位数由表长决定
* 折叠法
将关键字分割成位数相同的几部分(最后一部分的位数可以不同),然后取这几部分叠加和(舍去进位)作为哈希地址。关键字位数很多,而且关键字中每一位上数字分布大致均匀时,可以采用这种方法
* 除留余数法
取关键字被某个不大于哈希表表长m的数p除后所得余数为哈希地址,即`H(key) = key MOD p,p<=m`。它不仅可以对关键字直接取模,也可以在折叠,平方取中等运算之后取模。一般为了减少同义词(具有相同函数值的关键字)选取p为质数或不包含小于20的质因数的合数
* 随机数法
**处理冲突的方法**
不同关键字得到同一哈希地址,这种现象称为*冲突*
* 开放地址法
`Hi=(H(key) + di) MOD m ,i=1,2,...,k(k<=m-1)`,H(key)为哈希函数;m为哈希表表长;di为增量序列
若di=1,2,3,...,m-1,称为线性探测再散列
若di=1^2,-1^2,2^2,-2^2,3^3,...,(+|-)k^2,(k<=m/2)称为二次探测再散列
若di=伪随机数序列,称为伪随机探测再散列
* 再哈希法
`Hi = RHi(key),i=1,2,3,...,k`,RHi均是不同的哈希函数,即在同义词产生地址冲突时计算另一个哈希函数的地址,直到冲突不再发生
* 链地址法
将所有关键字为同义词的记录存储在同一线性表中。
* 建立一个公共溢出区
将哈希表分为基本表和溢出表两部分,凡是和基本表发生冲突的元素,一律填入溢出表。建立一个公共溢出区域,就是把冲突的都放在另一个地方,不在表里面。
#### HashMap集合的相关机制
我们应该知道`HashMap`中最基础的几个点:
1. `Java`中`HashMap`的实现的基础数据结构是数组,每一对`key`->`value`的键值对组成`Entity`类以双向链表的形式存放到这个数组中
2. 元素在数组中的位置由`key.hashCode()`的值决定,如果两个`key`的哈希值相等,即发生了哈希碰撞,则这两个`key`对应的`Entity`将以链表的形式存放在数组中
3. 调用`HashMap.get()`的时候会首先计算`key`的值,继而在数组中找到`key`对应的位置,然后遍历该位置上的链表找相应的值。
4. 为了提升整个`HashMap`的读取效率,当`HashMap`中存储的元素大小等于桶数组大小乘以负载因子的时候整个`HashMap`就要扩容,以减小哈希碰撞
5. 在`Java 8`中如果桶数组的同一个位置上的链表数量超过一个定值,则整个链表有一定概率会转为一棵红黑树。
整体来看,`HashMap`中最重要的点有四个:**初始化**,**数据寻址-`hash`方法**,**数据存储-`put`方法**,**扩容-`resize`方法**
##### 初始化
**其中用到了一些默认的初始值:**
```java
//默认的负载因子
static final float DEFAULT_LOAD_FACTOR = 0.75f;
```
负载因子决定着何时触发扩容机制。当`HashMap`中的数组元素个数超过阈值时便会触发扩容机制,阈值计算方式是`capacity * loadFactor`,默认`HashMap`中`loadFactor`是0.75。
**为什么是0.75呢?**
在注释中有这样一段话

大致意思就是在理想情况下,使用随机哈希码,节点出现的频率在hash桶中遵循泊松分布,同时给出了桶中元素个数和概率的对照表。从上面的表中可以看到当桶中元素到达8个的时候,概率已经变得非常小,也就是说用0.75作为加载因子,每个碰撞位置的链表长度超过8个是几乎不可能的。
所以总得来说就是负载因子为0.75的时候,不仅空间利用率比较高,而且避免了相当多的Hash冲突,使得底层的链表或者是红黑树的高度比较低,提升了空间效率。
```java
//最大容量
static final int MAXIMUM_CAPACITY = 1 << 30;
```
```java
//默认初始化的数组大小 (哈希桶数量)-必须是2的幂
static final int DEFAULT_INITIAL_CAPACITY = 1 << 4;
```
**`HashMap`的构造方法有四个:**
```java
//构造一个空的 HashMap,默认初始容量(16)和默认负载因子(0.75)
public HashMap() {
this.loadFactor = DEFAULT_LOAD_FACTOR;
}
```
```java
//构造一个空的 HashMap具有指定的初始容量和默认负载因子(0.75)
public HashMap(int initialCapacity) {
this(initialCapacity, DEFAULT_LOAD_FACTOR);
}
```
```java
//构造一个空的 HashMap具有指定的初始容量和负载因子
public HashMap(int initialCapacity, float loadFactor) {
if (initialCapacity < 0)
throw new IllegalArgumentException("Illegal initial capacity: " +
initialCapacity);
if (initialCapacity > MAXIMUM_CAPACITY)
initialCapacity = MAXIMUM_CAPACITY;
if (loadFactor <= 0 || Float.isNaN(loadFactor))
throw new IllegalArgumentException("Illegal load factor: " +
loadFactor);
this.loadFactor = loadFactor;
//要调整大小的下一个大小值(容量*负载因数)。
this.threshold = tableSizeFor(initialCapacity);
}
//返回给定目标容量的2次幂大小
static final int tableSizeFor(int cap) {
int n = cap - 1;
n |= n >>> 1;
n |= n >>> 2;
n |= n >>> 4;
n |= n >>> 8;
n |= n >>> 16;
return (n < 0) ? 1 : (n >= MAXIMUM_CAPACITY) ? MAXIMUM_CAPACITY : n + 1;
}
```
`tableSizeFor`的作用是找到大于`cap`的最小的2的整数幂,我们假设n(注意是n,不是cap哈)对应的二进制为000001xxxxxx,其中x代表的二进制位是0是1我们不关心,
`n |= n >>> 1;`执行后`n`的值为:

可以看到此时`n`的二进制最高两位已经变成了1,再接着执行第二行代码:
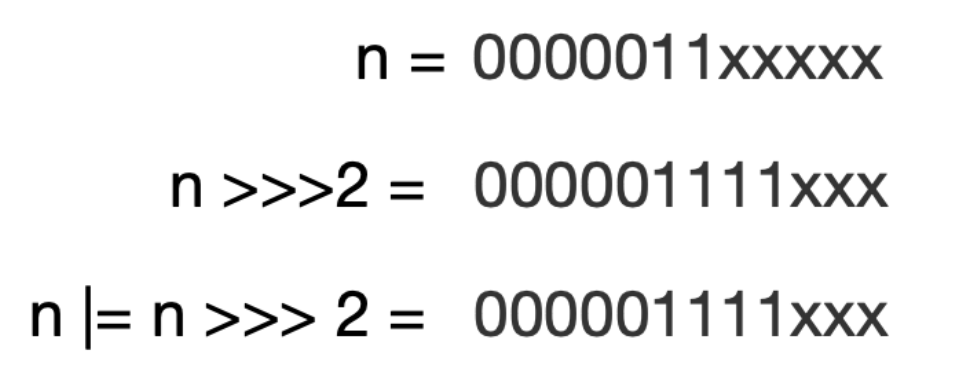
可见`n`的二进制最高四位已经变成了1,等到执行完代码`n |= n >>> 16;`之后,`n`的二进制最低位全都变成了1,也就是`n = 2^x - 1`其中x和n的值有关,如果没有超过`MAXIMUM_CAPACITY`,最后会返回一个2的正整数次幂,因此`tableSizeFor`的作用就是保证返回一个比入参大的最小的2的正整数次幂。
```java
//用与指定Map相同的映射构造一个新的HashMap
public HashMap(Map<? extends K, ? extends V> m) {
this.loadFactor = DEFAULT_LOAD_FACTOR;
putMapEntries(m, false);
}
```
我们执行构造方法后`HashMap`的空间并没有开辟(前三种),只是将一些即将用到的参数进行初始化(如负载因子),在`HashMap`第一次执行`put`的时候才会开辟数组空间
##### 数据寻址-`hash`方法
在`HashMap`这个特殊的数据结构中,`hash`函数承担着寻址定址的作用,其性能对整个`HashMap`的性能影响巨大,那什么才是一个好的`hash`函数呢?
- 计算出来的哈希值足够散列,能够有效减少哈希碰撞
- 本身能够快速计算得出,因为`HashMap`每次调用`get`和`put`的时候都会调用`hash`方法
```java
static final int hash(Object key) {
int h;
return (key == null) ? 0 : (h = key.hashCode()) ^ (h >>> 16);
}
```
最终我们需要将哈希值映射到数组下标上,即`hash & (table.length - 1)`
hash值其实是一个int类型,二进制位为32位,而HashMap的table数组初始化size为16,取余操作为`hashCode & 15 ==> hashCode & 1111` 。这将会存在一个巨大的问题,1111只会与hashCode的低四位进行与操作,也就是hashCode的高位其实并没有参与运算,会导很多hash值不同而高位有区别的数,最后算出来的索引都是一样的。
为了避免这种情况,HashMap将高16位与低16位进行异或,这样可以保证高位的数据也参与到与运算中来,以增大索引的散列程度,让数据分布得更为均匀。
*为什么用异或,不用 & 或者 | 操作,因为异或可以保证两个数值的特性,&运算使得结果向0靠近, |运算使得结果向1靠近*
##### 数据存储-`put`方法
在`Java 8`中`put`这个方法的思路分为以下几步:
1. 调用`key`的`hashCode`方法计算哈希值,并据此计算出数组下标index
2. 如果发现当前的桶数组为`null`,则调用`resize()`方法进行初始化
3. 如果没有发生哈希碰撞,则直接放到对应的桶中
4. 如果发生哈希碰撞,且节点已经存在,就替换掉相应的`value`
5. 如果发生哈希碰撞,且桶中存放的是树状结构,则挂载到树上
6. 如果碰撞后为链表,添加到链表尾,如果链表长度超过`TREEIFY_THRESHOLD`默认是8,则将链表转换为树结构
7. 数据`put`完成后,如果`HashMap`的总数超过`threshold`就要`resize`
具体代码以及注释如下:
```java
public V put(K key, V value) {
// 调用上文已经分析过的hash方法
return putVal(hash(key), key, value, false, true);
}
final V putVal(int hash, K key, V value, boolean onlyIfAbsent,
boolean evict) {
Node<K,V>[] tab; Node<K,V> p; int n, i;
if ((tab = table) == null || (n = tab.length) == 0)
// 第一次put时,会调用resize进行桶数组初始化
n = (tab = resize()).length;
// 根据数组长度和哈希值相与来寻址
if ((p = tab[i = (n - 1) & hash]) == null)
// 如果没有哈希碰撞,直接放到桶中
tab[i] = newNode(hash, key, value, null);
else {
Node<K,V> e; K k;
if (p.hash == hash &&
((k = p.key) == key || (key != null && key.equals(k))))
// 哈希碰撞,且节点已存在,直接替换
e = p;
else if (p instanceof TreeNode)
// 哈希碰撞,树结构
e = ((TreeNode<K,V>)p).putTreeVal(this, tab, hash, key, value);
else {
// 哈希碰撞,链表结构
for (int binCount = 0; ; ++binCount) {
if ((e = p.next) == null) {
p.next = newNode(hash, key, value, null);
// 链表过长,转换为树结构
if (binCount >= TREEIFY_THRESHOLD - 1) // -1 for 1st
treeifyBin(tab, hash);
break;
}
if (e.hash == hash &&
((k = e.key) == key || (key != null && key.equals(k))))
// 如果节点已存在,则跳出循环
break;
// 否则,指针后移,继续后循环
p = e;
}
}
if (e != null) {
// 对应着上文中节点已存在,跳出循环的分支
// 直接替换
V oldValue = e.value;
if (!onlyIfAbsent || oldValue == null)
e.value = value;
afterNodeAccess(e);
return oldValue;
}
}
++modCount;
if (++size > threshold)
// 如果超过阈值,还需要扩容
resize();
afterNodeInsertion(evict);
return null;
}
```
##### 扩容-`resize`方法
`resize`是整个`HashMap`中最复杂的一个模块,如果在`put`数据之后超过了`threshold`的值,则需要扩容,扩容意味着桶数组大小变化,我们在前文中分析过,`HashMap`寻址是通过`index =(table.length - 1) & key.hash();`来计算的,现在`table.length`发生了变化,势必会导致部分`key`的位置也发生了变化,`HashMap`是如何设计的呢?
这里就涉及到桶数组长度为2的正整数幂的第二个优势了:当桶数组长度为2的正整数幂时,如果桶发生扩容(长度翻倍),则桶中的元素大概只有一半需要切换到新的桶中,另一半留在原先的桶中就可以,并且这个概率可以看做是均等的。

通过这个分析可以看到如果在即将扩容的那个位上`key.hash()`的二进制值为0,则扩容后在桶中的地址不变,否则,扩容后的最高位变为了1,新的地址也可以快速计算出来`newIndex = oldCap + oldIndex;`
下面是`Java 8`中的实现:
```java
final Node<K,V>[] resize() {
Node<K,V>[] oldTab = table;
int oldCap = (oldTab == null) ? 0 : oldTab.length;
int oldThr = threshold;
int newCap, newThr = 0;
if (oldCap > 0) {
// 如果oldCap > 0则对应的是扩容而不是初始化
if (oldCap >= MAXIMUM_CAPACITY) {
threshold = Integer.MAX_VALUE;
return oldTab;
}
// 没有超过最大值,就扩大为原先的2倍
else if ((newCap = oldCap << 1) < MAXIMUM_CAPACITY &&
oldCap >= DEFAULT_INITIAL_CAPACITY)
newThr = oldThr << 1; // double threshold
}
else if (oldThr > 0) // initial capacity was placed in threshold
// 如果oldCap为0, 但是oldThr不为0,则代表的是table还未进行过初始化
newCap = oldThr;
else { // zero initial threshold signifies using defaults
newCap = DEFAULT_INITIAL_CAPACITY;
newThr = (int)(DEFAULT_LOAD_FACTOR * DEFAULT_INITIAL_CAPACITY);
}
if (newThr == 0) {
// 如果到这里newThr还未计算,比如初始化时,则根据容量计算出新的阈值
float ft = (float)newCap * loadFactor;
newThr = (newCap < MAXIMUM_CAPACITY && ft < (float)MAXIMUM_CAPACITY ?
(int)ft : Integer.MAX_VALUE);
}
threshold = newThr;
@SuppressWarnings({"rawtypes","unchecked"})
Node<K,V>[] newTab = (Node<K,V>[])new Node[newCap];
table = newTab;
if (oldTab != null) {
for (int j = 0; j < oldCap; ++j) {
// 遍历之前的桶数组,对其值重新散列
Node<K,V> e;
if ((e = oldTab[j]) != null) {
oldTab[j] = null;
if (e.next == null)
// 如果原先的桶中只有一个元素,则直接放置到新的桶中
newTab[e.hash & (newCap - 1)] = e;
else if (e instanceof TreeNode)
((TreeNode<K,V>)e).split(this, newTab, j, oldCap);
else { // preserve order
// 如果原先的桶中是链表
Node<K,V> loHead = null, loTail = null;
// hiHead和hiTail代表元素在新的桶中和旧的桶中的位置不一致
Node<K,V> hiHead = null, hiTail = null;
Node<K,V> next;
do {
next = e.next;
if ((e.hash & oldCap) == 0) {
if (loTail == null)
loHead = e;
else
loTail.next = e;
loTail = e;
}
else {
if (hiTail == null)
hiHead = e;
else
hiTail.next = e;
hiTail = e;
}
} while ((e = next) != null);
if (loTail != null) {
loTail.next = null;
// loHead和loTail代表元素在新的桶中和旧的桶中的位置一致
newTab[j] = loHead;
}
if (hiTail != null) {
hiTail.next = null;
// 新的桶中的位置 = 旧的桶中的位置 + oldCap
newTab[j + oldCap] = hiHead;
}
}
}
}
}
return newTab;
}
```
**一些常用方法的源码解析后面再说**
### LinkedHashMap
```java
public class LinkedHashMap<K,V> extends HashMap<K,V> implements Map<K,V>
```
**特点:**
* 由键决定:有序、不重复、无索引。
* 这里的有序指的是保证存储和取出的元素顺序一致
* 原理:底层数据结构是依然哈希表,只是每个键值对元素又额外的多了一个双链表的机制记录存储的顺序。
```java
//链表的头节点
transient LinkedHashMap.Entry<K,V> head;
//链表的尾节点
transient LinkedHashMap.Entry<K,V> tail;
```
HashMap和双向链表合二为一即是LinkedHashMap。所谓LinkedHashMap,其落脚点在HashMap,因此更准确地说,它是一个将所有Entry节点链入一个双向链表的HashMap。由于LinkedHashMap是HashMap的子类,所以LinkedHashMap自然会拥有HashMap的所有特性。比如,LinkedHashMap的元素存取过程基本与HashMap基本类似,只是在细节实现上稍有不同。当然,这是由LinkedHashMap本身的特性所决定的,因为它额外维护了一个双向链表用于保持迭代顺序。
不过遗憾的是,HashMap是无序的,也就是说,迭代HashMap所得到的元素顺序并不是它们最初放置到HashMap的顺序。HashMap的这一缺点往往会造成诸多不便,因为在有些场景中,我们确需要用到一个可以保持插入顺序的Map。庆幸的是,JDK为我们解决了这个问题,它为HashMap提供了一个子类 —— LinkedHashMap。虽然LinkedHashMap增加了时间和空间上的开销,但是它通过维护一个额外的双向链表保证了迭代顺序。
```java
//此链接散列映射的迭代排序方法:对于访问顺序为true,对于插入顺序为false。
final boolean accessOrder;
```
特别地,该迭代顺序可以是插入顺序,也可以是访问顺序。因此,根据链表中元素的顺序可以将LinkedHashMap分为:保持插入顺序的LinkedHashMap 和 保持访问顺序的LinkedHashMap,其中LinkedHashMap的默认实现是按插入顺序排序的。

内部节点的next指针用于维护`HashMap`各个桶中的`Entry`链,before、after用于维护`LinkedHashMap`的双向链表

### TreeMap
```java
public class TreeMap<K,V> extends AbstractMap<K,V> implements NavigableMap<K,V>, Cloneable, java.io.Serializable
```
**特点:**
* 由键决定特性:不重复、无索引、可排序
* 可排序:按照键数据的大小默认升序(有小到大)排序。只能对键排序。
* 注意:TreeMap集合是一定要排序的,可以默认排序,也可以将键按照指定的规则进行排序
* `TreeMap`跟`TreeSet`一样底层原理是一样的。
TreeMap是红黑二叉树的典型实现

root用来存储整个树的根节点
**具体分析后面再说** |
<center>
<?php
session_start();
if (isset($_SESSION["loggedin"]) && $_SESSION["loggedin"] === true) {
header("location: index.php");
exit;
}
require_once "config.php";
$username = $password = "";
$username_err = $password_err = $login_err = "";
if ($_SERVER["REQUEST_METHOD"] == "POST") {
if (empty(trim($_POST["username"]))) {
$username_err = "Skriv brugernavn";
} else {
$username = trim($_POST["username"]);
}
if (empty(trim($_POST["password"]))) {
$password_err = "Skriv kodeord";
} else {
$password = trim($_POST["password"]);
}
if (empty($username_err) && empty($password_err)) {
$sql = "SELECT id, username, password FROM users WHERE username = ?";
if ($stmt = mysqli_prepare($link, $sql)) {
mysqli_stmt_bind_param($stmt, "s", $param_username);
$param_username = $username;
if (mysqli_stmt_execute($stmt)) {
mysqli_stmt_store_result($stmt);
if (mysqli_stmt_num_rows($stmt) == 1) {
mysqli_stmt_bind_result($stmt, $id, $username, $hashed_password);
if (mysqli_stmt_fetch($stmt)) {
if (password_verify($password, $hashed_password)) {
session_start();
$_SESSION["loggedin"] = true;
$_SESSION["id"] = $id;
$_SESSION["username"] = $username;
header("location: index.php");
} else {
$login_err = "Ugyldigt brugernavn eller kodeord.";
}
}
} else {
$login_err = "Ugyldigt brugernavn eller kodeord.";
}
} else {
echo "Noget gik galt, prøv igen senere.";
}
mysqli_stmt_close($stmt);
}
}
mysqli_close($link);
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Renas Log ind</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<style>
body {
font: 14px sans-serif;
}
.wrapper {
width: 360px;
padding: 20px;
}
</style>
</head>
<body>
<div class="wrapper">
<h2>Log ind</h2>
<p>Skriv dine oplsyninger for at komme igang.</p>
<?php
if (!empty($login_err)) {
echo '<div class="alert alert-danger">' . $login_err . '</div>';
}
?>
<form action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]); ?>" method="post">
<div class="form-group">
<label>Brugernavn</label>
<input type="text" name="username" class="form-control <?php echo (!empty($username_err)) ? 'is-invalid' : ''; ?>" value="<?php echo $username; ?>">
<span class="invalid-feedback"><?php echo $username_err; ?></span>
</div>
<div class="form-group">
<label>Kodeord</label>
<input type="password" name="password" class="form-control <?php echo (!empty($password_err)) ? 'is-invalid' : ''; ?>">
<span class="invalid-feedback"><?php echo $password_err; ?></span>
</div>
<div class="form-group">
<input type="submit" class="btn btn-primary" value="Log ind">
</div>
<p>Har du ikke en bruger? <a href="register.php">Registrer dig nu</a>.</p>
</form>
</div>
</body>
</html>
</center> |
use std::{
collections::{HashMap, HashSet},
error::Error,
fs,
str::FromStr,
};
fn main() {
let raw_input = fs::read_to_string("day4a.txt").unwrap();
let drawn_numbers = DrawnNumbers::from_str(&raw_input).unwrap();
let mut boards = Boards::from_str(&raw_input).unwrap();
let mut last_bingo = None;
let mut winners = Winners::new();
for nb in drawn_numbers.0 {
for (board_idx, board) in boards.inner.iter_mut().enumerate() {
let bingo = board.scan(nb);
if let Some(rep) = bingo {
if winners.last(board_idx).is_some() {
last_bingo = Some(rep);
}
}
}
}
println!("Answer: {}", last_bingo.unwrap());
}
#[derive(Debug)]
struct DrawnNumbers(Vec<i32>);
impl FromStr for DrawnNumbers {
type Err = Box<dyn Error>;
fn from_str(input: &str) -> Result<Self, Self::Err> {
let drawn_numbers = input.lines().next().unwrap();
let drawn_numbers: Vec<&str> = drawn_numbers.split(',').collect();
let drawn_numbers: Vec<i32> = drawn_numbers
.iter()
.map(|e| e.parse::<i32>().unwrap())
.collect();
Ok(Self(drawn_numbers))
}
}
#[derive(Debug, Clone)]
enum Mark {
Marked(i32),
Unmarked(i32),
}
#[derive(Debug, Clone)]
struct Board {
board: Vec<Vec<Mark>>,
row_marks: HashMap<usize, Vec<i32>>,
col_marks: HashMap<usize, Vec<i32>>,
}
impl Board {
fn new(board: Vec<Vec<i32>>) -> Self {
let board = board
.iter()
.map(|row| row.iter().map(|&nb| Mark::Unmarked(nb)).collect())
.collect();
Self {
board,
row_marks: HashMap::default(),
col_marks: HashMap::default(),
}
}
fn scan(&mut self, drawn_nb: i32) -> Option<i32> {
for (r, row) in self.board.iter_mut().enumerate() {
let col_idx = row.iter().position(|e| match e {
Mark::Marked(nb) => nb == &drawn_nb,
Mark::Unmarked(nb) => nb == &drawn_nb,
});
if let Some(c) = col_idx {
// Marked the item
row[c] = Mark::Marked(drawn_nb);
// add row index to row mark list
let v = self.row_marks.entry(r).or_insert_with(Vec::new);
v.push(drawn_nb);
if v.len() == 5 {
let mut unmarkeds = 0;
for row in &self.board {
for col in row {
match &col {
Mark::Unmarked(c) => unmarkeds += c,
Mark::Marked(_) => (),
}
}
}
return Some(unmarkeds * drawn_nb);
}
// add col index to col mark list
let v = self.col_marks.entry(c).or_insert_with(Vec::new);
v.push(drawn_nb);
if v.len() == 5 {
let mut unmarkeds = 0;
for row in &self.board {
for col in row {
match &col {
Mark::Unmarked(c) => unmarkeds += c,
Mark::Marked(_) => (),
}
}
}
return Some(unmarkeds * drawn_nb);
}
}
}
None
}
}
struct Winners(HashSet<usize>);
impl Winners {
fn new() -> Self {
Self(HashSet::default())
}
fn last(&mut self, idx: usize) -> Option<usize> {
let prev_len = self.0.len();
self.0.insert(idx);
if self.0.len() != prev_len {
Some(idx)
} else {
None
}
}
}
struct Boards {
inner: Vec<Board>,
}
impl FromStr for Boards {
type Err = Box<dyn Error>;
fn from_str(input: &str) -> Result<Self, Self::Err> {
let boards_input = input.lines().skip(2);
let mut boards = Vec::new();
let mut raw_board = Vec::new();
for line in boards_input {
if !line.is_empty() {
let row: Vec<i32> = line
.split_terminator(' ')
.filter_map(|nb| nb.parse::<i32>().ok())
.collect();
raw_board.push(row);
} else {
let board = Board::new(raw_board.clone());
boards.push(board);
raw_board.clear();
}
}
Ok(Self { inner: boards })
}
} |
#!/usr/bin/python3
"""This module defines the HBNBCommand class for the command-line console."""
import cmd
from models.base_model import BaseModel
from models import storage
from models.place import Place
from models.city import City
from models.amenity import Amenity
from models.review import Review
from models.state import State
from models.user import User
class HBNBCommand(cmd.Cmd):
"""Define HBNBCommand class.
This class implements the command-line console for the AirBnB project.
"""
prompt = "(hbnb) "
def default(self, line):
"""Called when the command is not recognized."""
valid_class_names = [
'BaseModel',
'Place',
'City',
'Amenity',
'Review',
'State',
'User' # Add more if needed
]
parts = line.split('.')
if len(parts) == 2:
class_name = parts[0]
if class_name in valid_class_names:
if parts[1] == 'all()':
objs = [
str(obj)
for obj
in storage.all(cls=eval(class_name)).values()
]
print(objs)
elif parts[1] == 'count()':
count = len(storage.all(cls=eval(class_name)).values())
print(count)
else:
args = parts[1].split('(')
if len(args) == 2 and args[1].endswith(')'):
method_name = args[0]
if method_name == 'show':
instance_id = args[1][:-1]
self.do_show(
"{} {}".format(class_name, instance_id)
)
else:
print("*** Unknown syntax: {}".format(line))
else:
print("*** Unknown syntax: {}".format(line))
else:
print("** class doesn't exist **")
else:
print("*** Unknown syntax: {}".format(line))
def do_quit(self, line):
"""Quit the console."""
return True
def do_EOF(self, line):
"""Quit the console using EOF (Ctrl+D)."""
return True
def do_empty(self, line):
"""Do nothing when the user enters nothing + Enter."""
pass
def do_create(self, line):
"""Create a new instance of a specified class."""
args = line.split()
if len(args) == 0:
print("** class name missing **")
return
class_name = args[0]
try:
# Get the class from storage
obj_class = eval(class_name)
except NameError:
print("** class doesn't exist **")
return
new_instance = obj_class()
new_instance.save()
print(new_instance.id)
def do_all(self, line):
"""Print string representations of all instances.
based on class name or all classes.
"""
args = line.split()
if len(args) == 0:
all_objs = storage.all()
for obj in all_objs.values():
print(obj)
else:
class_name = args[0]
if not hasattr(storage, '_FileStorage__objects'):
storage.reload()
valid_class_names = [
'BaseModel',
'Place',
'City',
'Amenity',
'Review',
'State',
'User' # Add more if needed
]
if class_name not in valid_class_names:
print("** class doesn't exist **")
return
objs = [
str(obj)
for obj in storage.all(cls=eval(class_name)).values()
]
print(objs)
def do_show(self, line):
"""Show the string representation of an instance.
This is based on class name and ID.
"""
args = line.split()
if len(args) == 0:
print("** class name missing **")
return
class_name = args[0]
valid_class_names = [
'BaseModel',
'Place',
'City',
'Amenity',
'Review',
'State',
'User' # Add more if needed
]
if class_name not in valid_class_names:
print("** class doesn't exist **")
return
if len(args) < 2:
print("** instance id missing **")
return
instance_id = args[1]
key = "{}.{}".format(class_name, instance_id)
all_objs = storage.all()
if key in all_objs:
obj = all_objs[key]
print(obj)
else:
print("** no instance found **")
def do_destroy(self, line):
"""Destroy an instance based on class name and ID."""
args = line.split()
if len(args) == 0:
print("** class name missing **")
return
class_name = args[0]
valid_class_names = [
'BaseModel',
'Place',
'City',
'Amenity',
'Review',
'State'
] # Add more if needed
if class_name not in valid_class_names:
print("** class doesn't exist **")
return
if len(args) < 2:
print("** instance id missing **")
return
instance_id = args[1]
key = "{}.{}".format(class_name, instance_id)
all_objs = storage.all()
if key in all_objs:
del all_objs[key]
storage.save()
else:
print("** no instance found **")
def do_update(self, line):
"""Update an instance based on class name and ID.
by adding or updating attributes.
"""
args = line.split()
if len(args) == 0:
print("** class name missing **")
return
class_name = args[0]
valid_class_names = [
'BaseModel',
'Place',
'City',
'Amenity',
'Review',
'State'
] # Add more if needed
if class_name not in valid_class_names:
print("** class doesn't exist **")
return
if len(args) < 2:
print("** instance id missing **")
return
instance_id = args[1]
key = "{}.{}".format(class_name, instance_id)
all_objs = storage.all()
if key in all_objs:
obj = all_objs[key]
if len(args) < 3:
print("** attribute name missing **")
return
if len(args) < 4:
print("** value missing **")
return
attribute_name = args[2]
attribute_value = args[3]
setattr(obj, attribute_name, attribute_value)
obj.save()
else:
print("** no instance found **")
def do_count(self, line):
"""Count the number of instances of a class."""
args = line.split()
if len(args) == 0:
print("** class name missing **")
return
class_name = args[0]
valid_class_names = [
'BaseModel',
'Place',
'City',
'Amenity',
'Review',
'State',
'User' # Add more if needed
]
if class_name not in valid_class_names:
print("** class doesn't exist **")
return
count = len(storage.all(cls=eval(class_name)).values())
print(count)
if __name__ == '__main__':
HBNBCommand().cmdloop() |
class ThreadDemo8
{
public static void main(String arr[])
{
System.out.println("Inside main method"); //main is a parent thread
Demo obj1 = new Demo();
Demo obj2 = new Demo();
Thread t1 = new Thread(obj1); //creation of new thread
Thread t2 = new Thread(obj2);
t1.setName("First");//t1 and t2 are siblings
t2.setName("Second");
try
{
t1.start(); //start() will internally call run() when scheduler
//schedules it
t1.join(); //Because of it both works serially
t2.start();
t2.join();
}
catch(InterruptedException obj)
{}
System.out.println("End of main thread");
}
}
class Demo extends Thread
{
public void run()
{
int i = 0;
for(i = 1; i <= 10; i++)
{
try
{
System.out.println(Thread.currentThread().getName()+" : " +i);
Thread.sleep(500);//Its a ms, 1sec is 1000ms;needs to handle checked exception
}
catch(InterruptedException obj)
{}
}
}
} |
-- Exercice 1: Lister tous les employés
SELECT *
FROM employees;
-- Exercice 2: Lister le nom, prénom et titre de chaque employé
SELECT employees.lastName, employees.firstName, employees.jobTitle
FROM employees;
-- Exercice 3: Lister les Réprésentants Commerciaux ('Sales Rep') de la table employés
SELECT *
FROM employees
WHERE employees.jobTitle = 'Sales Rep';
-- Exercice 4 : Lister les différents statuts de la table 'orders', sans doublons
SELECT DISTINCT STATUS
FROM orders;
-- Exercice 5: Lister les paiements réalisés au mois de Mai
SELECT *
FROM payements
WHERE month(payements) = 5;
-- Exercice 6: Lister les paiements réalisés en mai ou en juin 2005
SELECT *
FROM payements
WHERE year(payementDate) = 2005
AND (
month(payementDate) = 5
OR
month(payementDate) = 6
);
-- Exercice 7: Lister le nom et prénom des clients(customers) en les combinant au sein d'une seule colonne nommée 'fullName'
SELECT CONCAT(contactLastName, ' ', contactFirstName) AS fullName
FROM customers;
-- Exercice 8: Lister les produits commençant par les caractères “195”
SELECT *
FROM products
WHERE products.productName
LIKE '195%'; --% au debut = fini par / % entre entouré = contient / % a la fin = commence par
-- Exercice 9: Lister le nom des produits qui ont un prix de vente (buyPrice) entre 70 et 100 $.
SELECT p.productName
FROM products p
WHERE p.buyPrice BETWEEN "70" and "100";
-- Exercice 10: Lister les clients (customers) ayant l'un des prénoms suivants : 'Alexander', 'Daniel', 'Elizabeth'
SELECT *
FROM customers
WHERE customers.contactFirstName IN ("Alexander", "Daniel", "Elizabeth");
-- Exercice 11: Lister les clients ordonnés par Nom de famille en ordre descendant
SELECT customers.contactLastName
FROM customers
ORDER BY customers.contactLastName DESC; -- DESC = ordre descendant / ASC = ordre croissant
-- Exercice 12: Certains clients ont le même prénom. Réalisez une requête qui retourne les prénoms en doublons
SELECT customers.contactFirstName
FROM `customers`
GROUP BY customers.contactFirstName
HAVING COUNT (customers.contactFirstName) > 1;
-- Exercice 13: Lister les 10 derniers enregistrements (en fonction de la colonne paymentDate) de la table payments
SELECT *
FROM payements
ORDER BY payementDate
LIMIT 10;
-- Exercice 14: Retourner le paiement le plus cher
SELECT * MAX(payements.amount)
FROM `payements`;
-- Exercice 15: Retourner la ville d'un bureau (office city) accompagné par le nombre d'employés associés à ce bureau (officeCode)
SELECT o.city, COUNT(e.officeCode) AS 'nb_employe' FROM offices o
LEFT JOIN employees e
ON e.officeCode = o.officeCode
GROUP BY o.city;
-- Exercice 16: Lettre à la DSI. Vous avez a reçu un courrier postal d'un client mais les informations sont à moitié effacées. Vous cherchez à retrouver qui celà peut bien être
-- En déchiffrant la lettre, vous arrivez uniquement à récupérer le deuxième et troisième caractère du code postal... '78'.
-- Réalisez une requête SQL permettant de lister les clients correspondants à ce code postal.**
SELECT *
FROM customers
WHERE postalCode
LIKE '_78__';
-- Exercice 17: Lister le chiffre d'affaire (somme des paiements), regroupés par année
SELECT YEAR(paymentDate) as "année", SUM(amount) as "CA"
FROM payments
GROUP BY année;
-- Exercice 18: Lister le chiffre d'affaire (somme des paiements), regroupés par année et mois. ordonnés chronologiquement
SELECT SUM(amount), YEAR(payements.paymentDate) y, EXTRACT (MONTH FROM payements.payementDate) m
FROM payements
GROUP BY y, m
ORDER BY payements.payementDate;
-- Exercice 19: Vous organisez une tombola. Faites une requête permettant de sélectionner un client aléatoirement
SELECT *, RAND() as R
FROM customers
ORDER BY R
LIMIT 1;
-- Exercice 20: Les employés ont des responsables (reportsTo). Retournez les employés n'ayant pas de supérieur
SELECT * FROM employees
WHERE employees.reportsTo IS NULL;
-- Exercice 21: Retourner les employés (employeeNumber, firstName, lastName) ayant le plus de responsabilités (le plus de reportsTo associés)
WITH cte(employeeNumber, firstName, lastName, underlings) AS (
SELECT e.employeeNumber, e.firstName, e.lastName, count(e2.employeeNumber) as 'underlings'
FROM employees e
JOIN employees e2 on e2.reportsTo = e.employeeNumber
GROUP by e.employeeNumber
)
SELECT cte.employeeNumber, cte.firstName, cte.lastName
FROM cte
WHERE cte.underlings = (SELECT MAX(underlings) from cte);
-- Exercice 22: Une erreur logicielle a eu lien, incrémentez toutes les factures de 5$.
UPDATE payments SET amount = amount + 5;
-- Exercice 23: Avez-vous remarqué que la table des paiements n'est pas liée aux orders? Comparez le montant des commandes par rapport aux paiements , regroupés par client
SELECT p.customerNumber, p.paiements, orders.ordres
FROM
(SELECT payments.customerNumber, SUM(payments.amount) as "paiements"
FROM payments GROUP BY customerNumber) p
JOIN
(SELECT o.customerNumber, SUM(od.quantityOrdered * od.priceEach) as "ordres"
FROM orderdetails od
JOIN orders o ON o.orderNumber = od.orderNumber)
GROUP BY o.customerNumber orders
ON orders.customerNumber = p.customerNumber;
-- Exercice 24: Les produits se vendent à des prix variants dans le temps. Retournez pour chaque produit le montant max et le montant min de la table orderdetails, ainsi que son prix actuel dans la table products
SELECT orderdetails.productCode, MAX(orderdetails.priceEach) as `Max`, MIN(orderdetails.priceEach) as `Min`, products.buyPrice
FROM orderdetails
JOIN products ON orderdetails.productCode = products.productCode
GROUP BY orderdetails.productCode; |
unit prop;
{$mode objfpc}{$H+}
interface
uses
{$IFDEF UNIX}
CThreads,
{$ENDIF}
Classes, SysUtils;
type
TPropItems = array[0..11] of string;
TPropDataReadyEvent = procedure of object;
TPropThread = class(TThread)
protected
procedure Execute; override;
public
call, rig: string;
OnPropDataReady: TPropDataReadyEvent;
constructor Create;
end;
var
PropThread: TPropThread;
PropItems: TPropItems;
(*
PropItems[0]: KComm latest version number
PropItems[1]: not used
PropItems[2]: WWV update date
PropItems[3]: Daily sunspot number
PropItems[4]: 10.7cm solar flux
PropItems[5]: A index (+ delta)
PropItems[6]: K index (+ delta)
PropItems[7]: LF propagation forecast
PropItems[8]: MF propagation forecast
PropItems[9]: HF propagation forecast
PropItems[10]: Solar forecast (short)
PropItems[11]: Solar forecast (long)
*)
implementation
uses
HTTPSend, SynaCode, Main, Utils;
const
propurl = 'http://www.g4ilo.com/kcomm_chk.php?call=%s&rig=%s&platform=%s&version=%s';
constructor TPropThread.Create;
begin
FreeOnTerminate := true;
OnPropDataReady := nil;
inherited Create(true);
end;
procedure TPropThread.Execute;
var
i, p: integer;
res: TStringList;
data, platform: string;
begin
res := TStringList.Create;
try
{$IFDEF WINDOWS}
platform := WinVerString[Ord(GetWinVersion)];
{$ELSE}
platform := 'Linux';
{$ENDIF}
if HttpGetText(Format(propurl,[call,EncodeURL(rig),EncodeURL(platform),sVerNo]),res) then
begin
data := res.Text;
i := 0;
repeat
p := Pos('|',data);
if p > 0 then
begin
PropItems[i] := StripTags(Copy(data,1,p-1));
Delete(data,1,p);
end;
Inc(i);
until (p=0) or (i > 11);
if Assigned(OnPropDataReady) then
Synchronize(OnPropDataReady);
end;
finally
res.Destroy;
end;
end;
end. |
[[tags: manual]]
[[toc:]]
== Cross Development
Since CHICKEN generates C code, it is relatively easy to create
programs and libraries for a different architecture than the one the
compiler is executing on, a process commonly called ''cross
compiling''. Basically you can simply compile Scheme code to C and
then invoke your target-specific cross compiler. To automate the
process of invoking the correct C compiler with the correct settings
and to simplify the use of extensions, CHICKEN can be built in a
special "cross-compilation" mode.
Note: in the following text we refer to the "target" as being the
platform on which the software is intended to run in the end. We use
the term "host" as the system that builds this software. Others use a
different nomenclature or switch the meaning of the words.
=== Preparations
Make sure you have a cross-toolchain in your {{PATH}}. In this
example, a Linux system is used to generate binaries for an ARM based
embedded system.
==== Building the target libraries
First you need a version of the runtime system ({{libchicken}}),
compiled for the target system. Obtain and unpack a tarball of the
CHICKEN sources, or check out the code from the official code
repository, then build the libraries and necessary development files:
make ARCH= \
PREFIX=/usr \
PLATFORM=linux
HOSTSYSTEM=arm-none-linux-gnueabi \
DESTDIR=$HOME/target \
TARGET_FEATURES="-no-feature x86 -feature arm" \
libs install-dev
This will build the CHICKEN libraries and install them in {{~/target}},
which we use as a temporary place to store the target files. A few things
to note:
* {{ARCH}} is empty, since we don't want the build process to detect the
architecture (since the target-architecture is likely to be different).
* {{PREFIX}} gives the prefix ''on the target system'', under which the
libraries will finally be installed. In this case it will be {{/usr/lib}}.
* {{PLATFORM}} determines the target platform. It must be one of the officially
supported platforms CHICKEN runs on.
* {{HOSTSYSTEM}} is an identifier for the target system and will be used as
the name prefix of the cross C compiler (in this case {{arm-none-linux-gnueabi-gcc}}).
If your cross compiler does not follow this convention, pass {{C_COMPILER}} and
{{LIBRARIAN}} to the {{make(1)}} invocation, with the names of the C compiler and
{{ar(1)}} tool, respectively.
* {{DESTDIR}} holds the directory where the compiled library files will temporarily
installeds into.
* {{TARGET_FEATURES}} contains extra options to be passed to the target-specific
Scheme translator; in this case we disable and enable features so that code like
the following will do the right thing when cross-compiled:
<enscript hightlight=scheme>
(cond-expand
(x86 <do this ...>)
...)
</enscript>
* If you obtained the sources from a source-code repository and not
from an official release tarball, you will need a {{chicken}}
executable to compile the Scheme sources of the runtime system. In
this case pass yet another variable to the {{make(1)}} invocation:
{{CHICKEN=<where the "chicken" executable is}}.
* You can also put all those variables into a file, say {{config.mk}}
and run {{make CONFIG=config.mk}}.
You should now have these files on {{~/target}}:
`-- usr
|-- include
| |-- chicken-config.h
| `-- chicken.h
|-- lib
| |-- chicken
| | `-- 6
| | `-- types.db
| |-- libchicken.a
| `-- libchicken.so
`-- share
You should now transfer {{libchicken.so}} to the target system, and place
it in {{/usr}}.
==== Building the "cross chicken"
Next, we will build another chicken, one that uses the cross C compiler to
generate target-specific code that uses the target-specific runtime library
we have just built.
Again, unpack a CHICKEN release tarball or a source tree and run
{{make(1)}} once again:
make PLATFORM=linux \
PREFIX=$HOME/cross-chicken \
TARGETSYSTEM=arm-none-linux-gnueabi \
PROGRAM_PREFIX=arm- \
TARGET_PREFIX=$HOME/target/usr \
TARGET_RUN_PREFIX=/usr \
install
* {{PREFIX}} gives the place where the "cross chicken" should be installed
into. It is recommended not to install into a standard location (like {{/usr/local}}
or {{$HOME}}) - some files will conflict with a normal CHICKEN installation.
* {{TARGETSYSTEM}} gives the name-prefix of the cross C compiler.
* {{PROGRAM_PREFIX}} determines the name-prefix of the CHICKEN tools to be created.
* {{TARGET_PREFIX}} specifies where the target-specific files (libraries and
headers) are located. This is the location where we installed the runtime
system into.
* {{TARGET_RUN_PREFIX}} holds the PREFIX that will be effective at runtime
(so {{libchicken.so}} will be found in {{$TARGET_RUN_PREFIX/lib}}).
* Make sure to use the same version of the CHICKEN sources for the target and
the cross build.
* If you build the cross chicken from repository sources, the same note
about the {{CHICKEN}} variable applies as given above.
In {{~/cross-chicken}}, you should find the following:
|-- bin
| |-- arm-chicken
| |-- arm-chicken-bug
| |-- arm-chicken-install
| |-- arm-chicken-profile
| |-- arm-chicken-status
| |-- arm-chicken-uninstall
| |-- arm-csc
| `-- arm-csi
|-- include
| |-- chicken-config.h
| `-- chicken.h
|-- lib
| |-- chicken
| | `-- 6
| | :
| |
| |-- libchicken.a
| |-- libchicken.so -> libchicken.so.6
| `-- libchicken.so.6
`-- share
|-- chicken
| |-- doc
: ; :
| |
| `-- setup.defaults
`-- man
`-- man1
:
To make sure that the right C compiler is used, we ask {{arm-csc}} to show
the name of the cross C compiler:
% ~/cross-chicken/arm-csc -cc-name
arm-none-linux-gnueabi-gcc
Looks good.
=== Using it
==== Compiling simple programs
% ~/cross-chicken/arm-csc -v hello.scm
/home/felix/cross-chicken/arm-cross-chicken/bin/arm-chicken hello.scm -output-file hello.c -quiet
arm-none-linux-gnueabi-gcc hello.c -o hello.o -c -fno-strict-aliasing -DHAVE_CHICKEN_CONFIG_H -g -Wall \
-Wno-unused -I /home/felix/cross-chicken/arm-chicken/include
rm hello.c
arm-none-linux-gnueabi-gcc hello.o -o hello -L/home/felix/cross-chicken/arm-chicken/lib -Wl,-R/usr/lib -lm \
-ldl -lchicken
rm hello.o
Is it an ARM binary?
% file hello
hello: ELF 32-bit LSB executable, ARM, version 1 (SYSV), for GNU/Linux 2.6.16, dynamically linked (uses shared libs), not stripped
Yes, looks good.
==== Compiling extensions
By default, the tools that CHICKEN provides to install, list and uninstall
extensions will operate on both the host and the target repository.
So running {{arm-chicken-install}} will compile and install the extension
for the host system and for the cross-target. To selectively install, uninstall
or list extensions for either the host or the target system use the
{{-host}} and {{-target}} options for the tools.
=== "Target-only" extensions
Sometimes an extension will only be compilable for the target platform
(for example libraries that use system-dependent features). In this
case you will have to work around the problem that the host-compiler
still may need compile-time information from the target-only
extension, like the import library of modules. One option is to copy
the import-library into the repository of the host compiler:
# optionally, you can compile the import library:
# ~/cross-chicken/arm-csc -O3 -d0 -s target-only-extension.import.scm
cp target-only-extension.import.scm ~/cross-chicken/lib/chicken/6
=== Final notes
Cross-development is a very tricky process - it often involves countless
manual steps and it is very easy to forget an important detail or mix
up target and host systems. Also, full 100% platform neutrality is
hard to achieve. CHICKEN tries very hard to make this transparent, but
at the price of considerable complexity in the code that manages
extensions.
----
Previous: [[Deployment]]
Next: [[Data representation]] |
#pragma once
#include <cmath>
#include "pico/stdlib.h"
enum class PIDType {
P_pole_placement_closed_loop_plant,
PI_pole_placement_1st_order_plant,
PID_pole_placement_2nd_order_plant,
P_manual_tunning,
PD_manual_tunning,
PI_manual_tunning,
PID_manual_tunning
};
class PIDController {
public: // Public attributes
PIDType PID_type;
float kp_bias; /// Bias for P-controller
float kp; /// Proportional term gain.
float ki; /// Integral term gain.
float kd; /// Derivative term gain (careful, usually is not needed because the signal from usually encoders has zero to no noise).
float N; /// Derivative filter coefficient. Use only when derivative term gain is different from zero. \todo implement PID with filter on the derivative term
bool has_derivative_filter;
const float MAX_OUTPUT; /// For output clamping and anti-windup
float sp_threshold;
bool at_sp;
private: // Private attributes
float last_error;
float last_output;
float last_integral;
float last_derivative;
public: // Public methods
// This PID implementation assumes the output variable can take values from -max_ouput to +max_output. This was designed with the application of controlling dc motors in mind.
PIDController(float max_output);
void setGains(PIDType type, float *params, float derivative_fc=(1<<32-1));
void changeSPThreshold(float threshold); // Threshold in the same unit as the process variable
/**
* @brief This function creates a new PIDController object that automatically calculates the controller
* constants based on the given first-order motor model and desired response parameters.
* The input to the model of the motor has to be Duty cycle of a PWM signal.
*
* @param type For calling this constructor the type has to be PIDType::PI_pole_placement_1st_order_plant.
* @param K Gain of the first order system representing the motor.
* @param tau [s]. Time constant of the first order system representing the motor.
* @param tss [s]. Desired settling time for the controlled system.
* @param OS [%]. Desired overshoot for the controlled system.
*/
/**
* @brief This function creates a new PIDController object that automatically calculates the controller
* constants based on the given second-order motor model and desired response parameters.
* The input to the model of the motor has to be Duty cycle of a PWM signal.
*
* @param type For calling this constructor the type has to be PIDType::PID_pole_placement_2nd_order_plant.
* @param K Gain of the second order system representing the motor.
* @param zeta Dampening ratio of the second order system representing the motor.
* @param wn Undamped natural frequency of the second order system representing the motor.
* @param tss [s]. Desired settling time for the controlled system.
* @param OS [%]. Desired overshoot for the controlled system.
*/
/**
* @brief This function should be called every sampling time of the control system
*
* @param error (sp-pv). Difference between desired motor output and actual motor output.
* @return DC [%]. Duty cycle of the PWM. The magnitude dictates strength of movement, the sign dictates the direction.
*/
float calculateControl(float error);
inline float clamp(float value);
float getError();
private: // Private methods
float pCalculateControl(float error);
float piCalculateControl(float error, float delta_time);
float pidPpCalculateControl(float error, float delta_time);
float pidMCalculateControl(float error, float delta_time);
}; |
<?php
require_once __DIR__ . '/init.php';
// Get all subscribers
$subscribers = $listMonk->subscribers()->getAll();
echo "All subscribers:\n";
foreach ($subscribers as $subscriber) {
echo $subscriber->getId() . " - " . $subscriber->getName() . "\n";
}
// Get a subscriber by id
$subscriber = $listMonk->subscribers()->get(5);
echo "Subscriber with id 1:\n";
echo $subscriber->getId() . " - " . $subscriber->getName() . "\n";
// Create a new subscriber
$newSubscriber = new \AdnanHussainTurki\ListMonk\Models\MonkSubscriber();
$newSubscriber->setName("Test Subscriber");
$newSubscriber->setEmail(random_int(23, 1000). "@gmail.com");
$newSubscriber->setStatus("enabled");
$newSubscriber->setLists([1, 2]);
$newSubscriberMonk = $listMonk->subscribers()->create($newSubscriber);
echo "New subscriber created:\n";
echo $newSubscriberMonk->getId(). " - " . $newSubscriberMonk->getName() . "\n";
// Update the created subscriber
$newSubscriberMonk->setName("Test Subscriber Updated");
$newSubscriberMonk->setEmail(random_int(23, 1000). "@email.com");
$newSubscriberMonk->setStatus("disabled");
$newSubscriberMonk->setLists([1, 2, 3]);
$updatedSubscriber = $listMonk->subscribers()->update($newSubscriberMonk);
echo "Updated subscriber:\n";
echo $updatedSubscriber->getId(). " - " . $updatedSubscriber->getName() . "\n";
// Delete the created subscriber
$deletedSubscriber = $listMonk->subscribers()->delete($updatedSubscriber->getId());
echo "Subscriber deleted:\n";
echo $deletedSubscriber->getId(). " - " . $deletedSubscriber->getName() . "\n"; |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.