text
stringlengths 184
4.48M
|
---|
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
import { setContext } from '@apollo/client/link/context'
import { getMainDefinition } from '@apollo/client/utilities'
import { relayStylePagination } from '@apollo/client/utilities';
import { createClient } from 'graphql-ws';
import { GraphQLWsLink } from '@apollo/client/link/subscriptions'
import {
ApolloClient,
ApolloProvider,
HttpLink,
InMemoryCache,
split
} from '@apollo/client'
const server = process.env.REACT_APP_SERVER || "https://small-wood-4274.fly.dev/"
const websocket = process.env.REACT_APP_WEBSOCKET || "wss://small-wood-4274.fly.dev/"
const authLink = setContext((_, { headers }) => {
const token = localStorage.getItem('socialPlatformUserToken')
return {
headers: {
...headers,
authorization: token ? `bearer ${token}` : null
}
}
})
const httpLink = new HttpLink({ uri: server })
const wsLink = new GraphQLWsLink(
createClient({ url: websocket, })
)
const splitLink = split(
({ query }) => {
const definition = getMainDefinition(query)
return (
definition.kind === 'OperationDefinition' &&
definition.operation === 'subscription'
)
},
wsLink,
authLink.concat(httpLink)
)
const client = new ApolloClient({
cache: new InMemoryCache({
typePolicies: {
Query: {
fields: {
allMessages: relayStylePagination()
}
}
}
}),
link: splitLink
})
const root = ReactDOM.createRoot(
document.getElementById('root') as HTMLElement
);
root.render(
<ApolloProvider client={client}>
<App />
</ApolloProvider>
); |
//
// MonoTlsProviderFactory.cs
//
// Author:
// Martin Baulig <[email protected]>
//
// Copyright (c) 2015 Xamarin, Inc.
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
#if SECURITY_DEP
#if MONO_SECURITY_ALIAS
extern alias MonoSecurity;
using MSI = MonoSecurity::Mono.Security.Interface;
using MX = MonoSecurity::Mono.Security.X509;
#else
using MSI = Mono.Security.Interface;
using MX = Mono.Security.X509;
#endif
using System.Security.Cryptography.X509Certificates;
#endif
using System;
using System.Net;
using System.Collections.Generic;
#if !MOBILE
using System.Reflection;
#endif
namespace Mono.Net.Security
{
/*
* Keep in sync with Mono.Security/Mono.Security.Interface/MonoTlsProvider.cs.
*
*/
static partial class MonoTlsProviderFactory
{
#region Internal API
/*
* APIs in this section are for consumption within System.dll only - do not access via
* reflection or from friend assemblies.
*
* @IMonoTlsProvider is defined as empty interface outside 'SECURITY_DEP', so we don't need
* this conditional here.
*/
internal static IMonoTlsProvider GetProviderInternal ()
{
#if SECURITY_DEP
lock (locker) {
InitializeInternal ();
return defaultProvider;
}
#else
throw new NotSupportedException ("TLS Support not available.");
#endif
}
#if SECURITY_DEP
internal static void InitializeInternal ()
{
lock (locker) {
if (initialized)
return;
MSI.MonoTlsProvider provider;
try {
provider = CreateDefaultProviderImpl ();
} catch (Exception ex) {
throw new NotSupportedException ("TLS Support not available.", ex);
}
if (provider == null)
throw new NotSupportedException ("TLS Support not available.");
defaultProvider = new Private.MonoTlsProviderWrapper (provider);
initialized = true;
}
}
internal static void InitializeInternal (string provider)
{
lock (locker) {
if (initialized)
throw new NotSupportedException ("TLS Subsystem already initialized.");
var msiProvider = LookupProvider (provider, true);
defaultProvider = new Private.MonoTlsProviderWrapper (msiProvider);
initialized = true;
}
}
#endif
static object locker = new object ();
static bool initialized;
static IMonoTlsProvider defaultProvider;
#endregion
#if SECURITY_DEP
static Dictionary<string,string> providerRegistration;
static Type LookupProviderType (string name, bool throwOnError)
{
lock (locker) {
InitializeProviderRegistration ();
string typeName;
if (!providerRegistration.TryGetValue (name, out typeName)) {
if (throwOnError)
throw new NotSupportedException (string.Format ("No such TLS Provider: `{0}'.", name));
return null;
}
var type = Type.GetType (typeName, false);
if (type == null && throwOnError)
throw new NotSupportedException (string.Format ("Could not find TLS Provider: `{0}'.", typeName));
return type;
}
}
static MSI.MonoTlsProvider LookupProvider (string name, bool throwOnError)
{
var type = LookupProviderType (name, throwOnError);
if (type == null)
return null;
try {
return (MSI.MonoTlsProvider)Activator.CreateInstance (type, true);
} catch (Exception ex) {
throw new NotSupportedException (string.Format ("Unable to instantiate TLS Provider `{0}'.", type), ex);
}
}
static void InitializeProviderRegistration ()
{
lock (locker) {
if (providerRegistration != null)
return;
providerRegistration = new Dictionary<string,string> ();
providerRegistration.Add ("legacy", "Mono.Net.Security.LegacyTlsProvider");
providerRegistration.Add ("default", "Mono.Net.Security.LegacyTlsProvider");
if (Mono.Btls.MonoBtlsProvider.IsSupported ())
providerRegistration.Add ("btls", "Mono.Btls.MonoBtlsProvider");
X509Helper2.Initialize ();
}
}
#if MOBILE_STATIC || !MOBILE
static MSI.MonoTlsProvider TryDynamicLoad ()
{
var variable = Environment.GetEnvironmentVariable ("MONO_TLS_PROVIDER");
if (string.IsNullOrEmpty (variable))
variable = "default";
return LookupProvider (variable, true);
}
static MSI.MonoTlsProvider CreateDefaultProviderImpl ()
{
var provider = TryDynamicLoad ();
if (provider != null)
return provider;
return new LegacyTlsProvider ();
}
#endif
#region Mono.Security visible API
/*
* "Public" section, intended to be consumed via reflection.
*
* Mono.Security.dll provides a public wrapper around these.
*/
internal static MSI.MonoTlsProvider GetProvider ()
{
var provider = GetProviderInternal ();
if (provider == null)
throw new NotSupportedException ("No TLS Provider available.");
return provider.Provider;
}
internal static bool IsProviderSupported (string name)
{
return LookupProvider (name, false) != null;
}
internal static MSI.MonoTlsProvider GetProvider (string name)
{
return LookupProvider (name, false);
}
internal static bool IsInitialized {
get {
lock (locker) {
return initialized;
}
}
}
internal static void Initialize ()
{
#if SECURITY_DEP
InitializeInternal ();
#else
throw new NotSupportedException ("TLS Support not available.");
#endif
}
internal static void Initialize (string provider)
{
#if SECURITY_DEP
InitializeInternal (provider);
#else
throw new NotSupportedException ("TLS Support not available.");
#endif
}
internal static HttpWebRequest CreateHttpsRequest (Uri requestUri, MSI.MonoTlsProvider provider, MSI.MonoTlsSettings settings)
{
lock (locker) {
var internalProvider = provider != null ? new Private.MonoTlsProviderWrapper (provider) : null;
return new HttpWebRequest (requestUri, internalProvider, settings);
}
}
internal static HttpListener CreateHttpListener (X509Certificate certificate, MSI.MonoTlsProvider provider, MSI.MonoTlsSettings settings)
{
lock (locker) {
var internalProvider = provider != null ? new Private.MonoTlsProviderWrapper (provider) : null;
return new HttpListener (certificate, internalProvider, settings);
}
}
#endregion
#endif
}
} |
package com.nit.jdbc;
import java.sql.Statement;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Scanner;
public class SelectTest5_TryWithResource {
public static void main(String[] args) {
try(Scanner sc = new Scanner(System.in);
Connection con = DriverManager.getConnection("jdbc:oracle:thin:@localhost:1521:xe","system","admin");
Statement st = con.createStatement();
){
//read input from enduser
String desg1=null,desg2=null,desg3=null;
if(sc!=null) {
System.out.println("Enter Desg1::");
desg1=sc.next().toUpperCase();//gives CLERK
System.out.println("Enter Desg2::");
desg2=sc.next().toUpperCase();//gives BANK
System.out.println("Enter Desg3::");
desg3=sc.next().toUpperCase();//gives Teacher
}
//convert input va;ues as the required for the SQL Query
//(' CLERK','BANK','Teacher')
String cond ="('"+desg1+"' ,'"+desg2+"' ,'"+desg3+"')";
//prepare the SQL Query
//SELECT EMPNO,ENAME,JOB,SAL FROM EMP WHERE JOB IN('CLERK','BANK','TEACHER')ORDER BY JOB;
String query="SELECT EMPNO,ENAME,JOB,SAL FROM EMP WHERE JOB IN"+cond+"ORDER BY JOB";
System.out.println(query);
//send and execute the SQL Query in Db s/w
try(ResultSet rs=st.executeQuery(query)){//nested try with resource
//process the result obj
if(rs!=null) {
boolean isRSEmpty=true;
while(rs.next()) {
isRSEmpty=false;
System.out.println(rs.getInt(1)+" "+rs.getString(2)+" "+rs.getString(3)+" "+rs.getFloat(4));
}//while
if(isRSEmpty)
System.out.println("No record found");
else
System.out.println("Record found and display");
}//if
}//try2
}//try1
catch(SQLException se) {
se.printStackTrace();
}
catch(Exception e) {
e.printStackTrace();
}
}//main
}//class |
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class UpdateApartmentsTableAddUserRelation extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('apartments', function (Blueprint $table) {
$table->unsignedBigInteger('user_id')->after('id')->nullable();
$table->foreign('user_id')
->references('id')
->on('users')
->onDelete('set null');
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('apartments', function (Blueprint $table) {
$table->dropForeign(['user_id']);
$table->dropColumn('user_id');
});
}
} |
import os
from datasets import Dataset, load_from_disk
class CodeDatasetBuilder:
"""
A utility class to build and manage code datasets.
Args:
root_dir (str): The root directory to search for code files.
Attributes:
root_dir (str): The root directory to search for code files.
Example:
code_builder = CodeDatasetBuilder("lucidrains_repositories")
code_builder.save_dataset("lucidrains_python_code_dataset", exclude_files=["setup.py"], exclude_dirs=["tests"])
code_builder.push_to_hub("lucidrains_python_code_dataset", organization="kye")
"""
def __init__(self, root_dir):
self.root_dir = root_dir
def collect_code_files(
self, file_extension=".py", exclude_files=None, exclude_dirs=None
):
"""
Collects code snippets from files in the specified directory and its subdirectories.
Args:
file_extension (str, optional): The file extension of code files to include (default is ".py").
exclude_files (list of str, optional): List of file names to exclude from collection (default is None).
exclude_dirs (list of str, optional): List of directory names to exclude from collection (default is None).
Returns:
list of str: List of code snippets.
"""
code_snippets = []
exclude_files = set(exclude_files) if exclude_files else set()
exclude_dirs = set(exclude_dirs) if exclude_dirs else set()
try:
for root, dirs, files in os.walk(self.root_dir):
# Exclude specified directories
dirs[:] = [d for d in dirs if d not in exclude_dirs]
for file in files:
if file.endswith(file_extension) and file not in exclude_files:
with open(os.path.join(root, file), "r", encoding="utf-8") as f:
code = f.read()
code_snippets.append(code)
except Exception as e:
raise RuntimeError(f"Error while collecting code files: {e}")
return code_snippets
def create_dataset(
self, file_extension=".py", exclude_files=None, exclude_dirs=None
):
"""
Creates a dataset from collected code snippets.
Args:
file_extension (str, optional): The file extension of code files to include (default is ".py").
exclude_files (list of str, optional): List of file names to exclude from collection (default is None).
exclude_dirs (list of str, optional): List of directory names to exclude from collection (default is None).
Returns:
datasets.Dataset: The code dataset.
"""
code_snippets = self.collect_code_files(
file_extension, exclude_files, exclude_dirs
)
dataset = Dataset.from_dict({"code": code_snippets})
return dataset
def save_dataset(
self, dataset_name, file_extension=".py", exclude_files=None, exclude_dirs=None
):
"""
Saves the code dataset to disk.
Args:
dataset_name (str): The name for the saved dataset.
file_extension (str, optional): The file extension of code files to include (default is ".py").
exclude_files (list of str, optional): List of file names to exclude from collection (default is None).
exclude_dirs (list of str, optional): List of directory names to exclude from collection (default is None).
Raises:
RuntimeError: If there is an error while saving the dataset.
"""
dataset = self.create_dataset(file_extension, exclude_files, exclude_dirs)
try:
dataset.save_to_disk(dataset_name)
except Exception as e:
raise RuntimeError(f"Error while saving the dataset: {e}")
def load_dataset(self, dataset_name):
"""
Loads a code dataset from disk.
Args:
dataset_name (str): The name of the saved dataset.
Returns:
datasets.Dataset: The loaded code dataset.
"""
try:
loaded_dataset = load_from_disk(dataset_name)
return loaded_dataset
except Exception as e:
raise RuntimeError(f"Error while loading the dataset: {e}")
def push_to_hub(self, dataset_name):
"""
Pushes the code dataset to the Hugging Face Model Hub.
Args:
dataset_name (str): The name of the saved dataset.
organization (str, optional): The organization on the Model Hub to push to (default is "username").
"""
try:
self.load_dataset.push_to_hub(f"{dataset_name}")
except Exception as e:
raise RuntimeError(
f"Error while pushing the dataset to the Hugging Face Model Hub: {e}"
)
# Example usage:
code_builder = CodeDatasetBuilder("huggingface")
code_builder.save_dataset(
"huggingface", exclude_files=["setup.py"], exclude_dirs=["tests"]
)
code_builder.push_to_hub("all-hf-python-2") |
using Microsoft.AspNetCore.Mvc;
using Moq;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using webapi.Controllers;
using webapi.DTO;
using webapi.Interfaces;
using webapi.Models;
using webapi.Models.DND;
using webapi.Models.DND.Enums;
using Xunit;
namespace webapiUnitTests.ControllersUnitTests
{
public class CharacterSheetControllerUT
{
[Fact]
public async Task GetDNDCardById_ShouldReturnOk_WhenIdIsValid()
{
var mockService = new Mock<ICharacterSheetService>();
var mockService2 = new Mock<IUserService>();
mockService.Setup(s => s.GetDNDCardByIdAsync(It.IsAny<int>()))
.ReturnsAsync(Helpers.Characters()[0]);
var controller = new CharacterSheetController(mockService.Object, mockService2.Object);
var result = await controller.GetDNDCardById(1);
var okResult = Assert.IsType<OkObjectResult>(result);
var returnValue = Assert.IsType<DndCharacter>(okResult.Value);
Assert.Equal(1, returnValue.ID);
}
[Fact]
public async Task GetDNDCardById_ShouldReturnNotFound_WhenIdIsInvalid()
{
var mockService = new Mock<ICharacterSheetService>();
var mockService2 = new Mock<IUserService>();
mockService.Setup(s => s.GetDNDCardByIdAsync(It.IsAny<int>()))
.ReturnsAsync((DndCharacter?) null);
var controller = new CharacterSheetController(mockService.Object, mockService2.Object);
var result = await controller.GetDNDCardById(1);
Assert.IsType<NotFoundResult>(result);
}
[Fact]
public async Task GetAllDNDCharacters_ShouldReturnOk()
{
var mockService = new Mock<ICharacterSheetService>();
var mockService2 = new Mock<IUserService>();
mockService.Setup(s => s.GetAllDNDCharactersAsync())
.ReturnsAsync(Helpers.Characters());
var controller = new CharacterSheetController(mockService.Object, mockService2.Object);
var result = await controller.GetAllDNDCharacters();
var okResult = Assert.IsType<OkObjectResult>(result);
var returnValue = Assert.IsType<List<DndCharacter>>(okResult.Value);
Assert.Equal(3, returnValue.Count);
}
[Fact]
public async Task GetAllDNDCharactersByFilters_ShouldReturnOk()
{
var mockService = new Mock<ICharacterSheetService>();
var mockService2 = new Mock<IUserService>();
mockService.Setup(s => s.GetAllDNDCharactersByFiltersAsync(It.IsAny<int>(), It.IsAny<SystemType>()))
.ReturnsAsync(Helpers.Characters());
var controller = new CharacterSheetController(mockService.Object, mockService2.Object);
var result = await controller.GetAllDNDCharactersByFilters(1, SystemType.DND);
var okResult = Assert.IsType<OkObjectResult>(result);
var returnValue = Assert.IsType<List<DndCharacter>>(okResult.Value);
Assert.Equal(3, returnValue.Count);
}
[Fact]
public async Task GetAllDNDCharacters_ShouldReturnOkWithEmptyList_WhenNoCharactersExist()
{
var mockService = new Mock<ICharacterSheetService>();
var mockService2 = new Mock<IUserService>();
mockService.Setup(s => s.GetAllDNDCharactersAsync())
.ReturnsAsync(new List<DndCharacter>());
var controller = new CharacterSheetController(mockService.Object, mockService2.Object);
var result = await controller.GetAllDNDCharacters();
var okResult = Assert.IsType<OkObjectResult>(result);
var returnValue = Assert.IsType<List<DndCharacter>>(okResult.Value);
Assert.Empty(returnValue);
}
[Fact]
public async Task GetAllDNDCharactersByFilters_ShouldReturnOkWithEmptyList_WhenNoMatches()
{
var mockService = new Mock<ICharacterSheetService>();
var mockService2 = new Mock<IUserService>();
mockService.Setup(s => s.GetAllDNDCharactersByFiltersAsync(It.IsAny<int>(), It.IsAny<SystemType?>()))
.ReturnsAsync(new List<DndCharacter>());
var controller = new CharacterSheetController(mockService.Object, mockService2.Object);
var result = await controller.GetAllDNDCharactersByFilters(1, SystemType.DND);
var okResult = Assert.IsType<OkObjectResult>(result);
var returnValue = Assert.IsType<List<DndCharacter>>(okResult.Value);
Assert.Empty(returnValue);
}
[Fact]
public async Task CreateNewDndCharacter_ReturnsNotFound_WhenUserNotFound()
{
// Arrange
var mockUserService = new Mock<IUserService>();
var mockCharacterService = new Mock<ICharacterSheetService>();
mockUserService.Setup(s => s.GetUserByTokenAsync(It.IsAny<int>()))
.ReturnsAsync((User?)null);
var controller = new CharacterSheetController(mockCharacterService.Object, mockUserService.Object);
// Act
var result = await controller.CreateNewDndCharacter(1, new DndCharacterDto());
// Assert
Assert.IsType<NotFoundResult>(result);
}
[Fact]
public async Task CreateNewDndCharacter_ReturnsBadRequest_WhenExceptionOccurs()
{
// Arrange
var mockUserService = new Mock<IUserService>();
var mockCharacterService = new Mock<ICharacterSheetService>();
mockUserService.Setup(s => s.GetUserByTokenAsync(It.IsAny<int>()))
.ThrowsAsync(new Exception("An error occurred"));
var controller = new CharacterSheetController(mockCharacterService.Object, mockUserService.Object);
// Act
var result = await controller.CreateNewDndCharacter(1, new DndCharacterDto());
// Assert
var badRequestResult = Assert.IsType<BadRequestObjectResult>(result);
Assert.Equal("An error occurred", badRequestResult.Value);
}
[Fact]
public async Task CreateNewDndCharacter_ReturnsOk_WhenSuccessful()
{
// Arrange
var mockUserService = new Mock<IUserService>();
var mockCharacterService = new Mock<ICharacterSheetService>();
var user = new User { ID = 1, UserToken = 1 };
var character = new DndCharacter { ID = 1, UserToken = 1 };
mockUserService.Setup(s => s.GetUserByTokenAsync(It.IsAny<int>()))
.ReturnsAsync(user);
mockCharacterService.Setup(s => s.CreateCharacterAsync(It.IsAny<int>(), It.IsAny<DndCharacterDto>()))
.ReturnsAsync(character);
var controller = new CharacterSheetController(mockCharacterService.Object, mockUserService.Object);
// Act
var result = await controller.CreateNewDndCharacter(1, new DndCharacterDto());
// Assert
Assert.IsType<OkResult>(result);
mockUserService.Verify(m => m.UpdateUserAndCharacterRelationship(It.IsAny<int>(), It.IsAny<int>()), Times.Once);
}
}
} |
#include <ESP8266WiFi.h>
#include <ThingSpeak.h>
#include <Wire.h>
#include <Adafruit_BMP085.h>
#include <dht.h>
#include <ESP8266HTTPClient.h>
/* Global Variables */
#ifndef STASSID
#define STASSID "EVO-Charji-2C4E"
#define STAPSK "G55BHv2G"
#endif
const char* serverName = "http://weathermonitoringstationee.000webhostapp.com//post-data.php";
String apiKeyValue = "12345";
#define seaLevelPressure 101325.0 /* Standard Atmospheric Pressure */
#define sensorDHT11 2 /* NodeMCU D4 */
#define _analog A0
#define _S0 D3
#define _S1 D7
#define _S2 D8
WiFiClient client;
Adafruit_BMP085 bmp;
dht DHT;
const char* ssid = STASSID;
const char* password = STAPSK;
long channel_ID = 1583586;
const char* write_API_Key = "P2I91BUMUB83EO7H";
long channel2_ID = 1616771;
const char* write_API2_Key = "VV7S17VFGB31GH3G";
int analogValue;
/* Setup */
void setup() {
Serial.begin(9600);
/* Wifi */
Serial.print("Connecting to: ");
Serial.println(ssid);
/* Client Mode */
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
Serial.println("REBOOT");
/* BMP180 */
if (!bmp.begin())
{
Serial.println("Error....Something wrong with BMP!");
}
/* Multiplexer */
pinMode(_S0, OUTPUT);
pinMode(_S1, OUTPUT);
pinMode(_S2, OUTPUT);
digitalWrite(_S0, LOW);
digitalWrite(_S1, LOW);
digitalWrite(_S2, LOW);
/* ThingSpeak */
ThingSpeak.begin(client);
}
/* Loop */
void loop() {
double Temperature, Altitude, RealAltitude;
int Pressure, CalcPressure;
float temperature11, humidity;
int intensityRain, intensityLight, airQuality;
/* BMP180 */
Temperature = bmp.readTemperature();
Pressure = bmp.readPressure();
Altitude = bmp.readAltitude();
CalcPressure = bmp.readSealevelPressure();
RealAltitude = bmp.readAltitude(seaLevelPressure);
/* <END> */
/* DHT11 */
DHT.read11(sensorDHT11);
temperature11 = DHT.temperature;
humidity = DHT.humidity;
/* <END> */
/* Rain Intensity */
digitalWrite(_S0, LOW);
digitalWrite(_S1, LOW);
digitalWrite(_S2, LOW);
delay(100);
intensityRain = analogRead(_analog);
/* <<END> */
/* Light Intensity */
digitalWrite(_S0, HIGH);
digitalWrite(_S1, LOW);
digitalWrite(_S2, LOW);
delay(100);
intensityLight = analogRead(_analog);
/* <<END> */
/* Air Quality */
digitalWrite(_S0, LOW);
digitalWrite(_S1, HIGH);
digitalWrite(_S2, LOW);
delay(100);
airQuality = analogRead(_analog);
/* <<END> */
/* Serial Print */
Serial.println("Temperature = " + (String) Temperature + " *C");
Serial.println("Pressure = " + (String) Pressure + " Pa"); /*Absolute or Actual Ambient Temperature. It will vary with both altitude and weather */
Serial.println("Altitude = " + (String) Altitude + " meters");
Serial.println("Pressure (sealevel) = " + (String) CalcPressure + " Pa"); /* Adjusted Pressure */
Serial.println("Real altitude = " + (String) RealAltitude + " meters");
Serial.println("Temperature11 (*C): " + (String) temperature11);
Serial.println("Humidity: " + (String) humidity + " %");
Serial.println("Rain:" + (String)intensityRain);
Serial.println("Light Intensity:" + (String) intensityLight);
Serial.println("Air Quality:" + (String) airQuality);
Serial.println();
/* <<END> */
/* ThingSpeak */
ThingSpeak.setField(1, (float) Temperature);
ThingSpeak.setField(2, (float) Pressure);
ThingSpeak.setField(3, (float) Altitude);
ThingSpeak.setField(4, (float) CalcPressure);
ThingSpeak.setField(5, humidity);
ThingSpeak.setField(6, (float) intensityRain);
ThingSpeak.setField(7, (float) intensityLight);
ThingSpeak.setField(8, (float) airQuality);
ThingSpeak.writeFields(channel_ID, write_API_Key);
/* <<END> */
delay(1100);
float maxT = ThingSpeak.readFloatField(channel2_ID, 1, write_API2_Key);
delay(1100);
float minT = ThingSpeak.readFloatField(channel2_ID, 2, write_API2_Key);
delay(1100);
float RealFeel = ThingSpeak.readFloatField(channel2_ID, 5, write_API2_Key);
delay(1100);
float dewPoint = ThingSpeak.readFloatField(channel2_ID, 4, write_API2_Key);
Serial.println("maxT:" + (String) maxT);
Serial.println("MinT:" + (String) minT);
Serial.println("RealFeel:" + (String) RealFeel);
Serial.println("DewPoint" + (String) dewPoint);
Serial.println();
if(WiFi.status()== WL_CONNECTED){
HTTPClient http;
http.begin(client,serverName);
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
// Prepare your HTTP POST request data
String httpRequestData = "api_key=" + apiKeyValue + "&Temprature=" + (float) Temperature
+ "&Humidity=" + humidity + "&Pressure=" + (float) Pressure
+ "&Altitude=" +float(Altitude) + "&Light=" + intensityLight +"&Rain=" +intensityRain + "&AirQuality=" + (float) airQuality + "&RealFeel=" + RealFeel + "&DewPoint=" + dewPoint;
Serial.print("httpRequestData: ");
Serial.println(httpRequestData);
int httpResponseCode = http.POST(httpRequestData);
if (httpResponseCode>0) {
Serial.print("HTTP Response code: ");
Serial.println(httpResponseCode);
}
else {
Serial.print("Error code: ");
Serial.println(httpResponseCode);
}
http.end();
}
else {
Serial.println("WiFi Disconnected");
}
delay(12000);
} |
import { task } from "hardhat/config";
import { UniswapV3Adapter, TestToken } from "../typechain";
import { BigNumber } from "ethers";
import { HardhatRuntimeEnvironment, TaskArguments } from "hardhat/types";
task("createPool", "Creating a new pool")
.addParam("contract", "The UniswapV3Adapter address")
.addParam("tokenA", "addres tokenA")
.addParam("tokenB", "addres tokenB")
.addParam("sqrtPrice")
.setAction(
async (
taskArgs: TaskArguments,
hre: HardhatRuntimeEnvironment
): Promise<void> => {
const v3Adapter: UniswapV3Adapter = <UniswapV3Adapter>(
await hre.ethers.getContractAt(
"UniswapV3Adapter",
taskArgs.contract as string
)
);
const tokenA: TestToken = <TestToken>(
await hre.ethers.getContractAt("TestToken", taskArgs.tokenA as string)
);
const tokenB: TestToken = <TestToken>(
await hre.ethers.getContractAt("TestToken", taskArgs.tokenB as string)
);
const sqrtPrice: BigNumber = taskArgs.sqrtPrice;
await v3Adapter.createPool(tokenA.address, tokenB.address, sqrtPrice);
const filter = v3Adapter.filters.PoolCreated();
const events = await v3Adapter.queryFilter(filter);
const poolAddress = events[0].args["pool"];
const token0 = events[0].args["token0"];
const token1 = events[0].args["token1"];
const fee = events[0].args["fee"];
console.log("Pool created!");
console.log(`Pool address: ${poolAddress}`);
console.log(`Token0: ${token0}`);
console.log(`Token1: ${token1}`);
console.log(`Fee: ${fee}`);
}
); |
using HotelManagementApi.Interfaces;
using HotelManagementApi.Models;
using HotelManagementApi.Models.DTOs;
using HotelManagementApi.Services;
using Microsoft.AspNetCore.Authorization;
using Microsoft.AspNetCore.Cors;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
namespace HotelManagementApi.Controllers
{
[EnableCors("MyCors")]
[Route("api/[controller]")]
[ApiController]
public class RoomController : ControllerBase
{
private readonly IRoomService _roomService;
public RoomController(IRoomService roomService)
{
_roomService = roomService;
}
[HttpGet]
public ActionResult Get()
{
var result = _roomService.GetAllRooms();
if (result == null)
{
return NotFound("No Rooms are available at this moment");
}
return Ok(result);
}
[Authorize]
[HttpPost]
public IActionResult AddRoom([FromBody] Room room)
{
bool isRoomAdded = _roomService.AddANewRoom(room);
if (isRoomAdded)
{
return Ok("Room added successfully!");
}
return BadRequest("Room addition failed. The room may already exist.");
}
[HttpPut]
public ActionResult Update(RoomDTO roomDTO)
{
try
{
var result = _roomService.UpdatePrice(roomDTO);
if (result == null)
return NotFound();
return Ok(result);
}
catch (Exception e)
{
return BadRequest(e.Message);
}
}
[HttpDelete]
public ActionResult DeleteHotel(int id)
{
try
{
var result = _roomService.DeleteRoom(id);
if (result == null)
return NotFound();
return Ok(result);
}
catch (Exception e)
{
return BadRequest(e.Message);
}
}
}
} |
import React, { useState } from "react";
import "./upload.css";
import Papa from "papaparse";
import {utils, writeFile } from "xlsx";
function UploadComponent() {
const [data, setData] = useState([]);
const [coldata, setColData] = useState([]);
const [valuedata, setvalueData] = useState([]);
// handleImport
const handleImport = (event) => {
Papa.parse(event.target.files[0], {
header: true,
skipEmptyLines: true,
complete: function (result) {
const colArray = [];
const valueArray = [];
result.data.map((data) => {
colArray.push(Object.keys(data));
valueArray.push(Object.values(data));
});
setData(result.data);
setColData(colArray[0]);
setvalueData(valueArray);
},
});
};
// handleExport
const handleExoprt = () => {
const headings = [["FirstName", "LastName", "DOB", "City"]];
const wb = utils.book_new();
const ws = utils.json_to_sheet([]);
utils.sheet_add_aoa(ws, headings);
utils.sheet_add_json(ws, data, { origin: "A2", skipHeader: true });
utils.book_append_sheet(wb, ws, "Report");
writeFile(wb, "Report.xlsx");
};
return (
<div className="import">
{/* download template */}
<div className="download-template">
<button onClick={handleExoprt}>Download Template</button>
</div>
{/* upload template */}
<div className="button-wrap">
<label className="button" htmlFor="upload">
Upload Template
</label>
<input id="upload" type="file" accept=".csv" onChange={handleImport} />
</div>
{data.length == 0 ? (
<h4>no data found</h4>
) : (
<div className="data" style={{ marginTop: "2rem" }}>
<table>
<thead>
<tr>
{coldata.map((d, i) => {
return <th key={i}>{d}</th>;
})}
</tr>
</thead>
<tbody>
{valuedata.map((d, i) => {
return (
<tr key={i}>
{d.map((subdata, i) => {
return <td key={i}>{subdata}</td>;
})}
</tr>
);
})}
</tbody>
</table>
</div>
)}
</div>
);
}
export default UploadComponent; |
# How to: Meld for Git diffs in Ubuntu Hardy
- published: 2008-06-02 12:10
- tags: linux, software, git
Are you using [Git](http://git.or.cz/) in Ubuntu and want to use an external visual diff viewer? It's easy! I will be using [Meld](http://meld.sourceforge.net/) for this example but most visual diff tools should be similar. If you don't already have Meld, then install it:
sudo apt-get install meld
Ok. Now let's begin by breaking it. Enter this into a terminal:
git config --global diff.external meld
Then navigate to a Git tracked directory and enter this (with an actual filename):
git diff filename
Meld will open but it will complain about bad parameters. The problem is that Git sends its external diff viewer seven parameters when Meld only needs two of them; two filenames of files to compare. One way to fix it is to write a script to format the parameters before sending them to Meld. Let's do that.
Create a new python script in your home directory (or wherever, it doesn't matter) and call it _diff.py_.
#! Python
#!/usr/bin/python
import sys
import os
os.system('meld "%s" "%s"' % (sys.argv[2], sys.argv[5]))
Now we can set Git to perform it's diff on our new script (replacing the path with yours):
git config --global diff.external /home/nathan/diff.py
git diff filename
This time when we do a diff it will launch Meld with the right parameters and we will see our visual diff. |
package testBase;
import java.io.File;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.time.Duration;
import java.util.ResourceBundle;
import org.apache.commons.io.FileUtils;
import org.apache.commons.lang3.RandomStringUtils;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.LogManager;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.edge.EdgeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.annotations.*;
import io.github.bonigarcia.wdm.WebDriverManager;
public class BaseTest{
public static WebDriver driver;
public Logger logger;
public ResourceBundle rb ;// for reading values from config file
@BeforeClass(groups={"Sanity","Master","Regression"})
@Parameters("browser")
public void setUp(String br) throws InterruptedException
{
logger = LogManager.getLogger(this.getClass());
rb = ResourceBundle.getBundle("config");
if(br.equals("chrome"))
{
ChromeOptions chromeOptions = new ChromeOptions();
chromeOptions.addArguments("--remote-allow-origins=*","ignore-certificate-errors");
WebDriverManager.chromedriver().setup();
driver =new ChromeDriver(chromeOptions);
}
else if (br.equals("firefox"))
{ WebDriverManager.firefoxdriver().setup();
driver = new FirefoxDriver();
}
else
{
WebDriverManager.edgedriver().setup();
driver =new EdgeDriver();
}
driver.manage().deleteAllCookies();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
driver.get(rb.getString("appURL"));
Thread.sleep(3000);
System.out.println("Browser Launched & nnavigated to URL");
driver.manage().window().maximize();
}
@AfterClass(groups={"Sanity","Master","Regression"})
public void tearDown()
{
driver.quit();
}
public String randomeString() {
String generatedString = RandomStringUtils.randomAlphabetic(5);
return (generatedString);
}
public String randomeNumber() {
String generatedString2 = RandomStringUtils.randomNumeric(10);
return (generatedString2);
}
public String randomAlphaNumeric() {
String st = RandomStringUtils.randomAlphabetic(4);
String num = RandomStringUtils.randomNumeric(3);
return (st+"@"+num);
}
public String captureScreen(String tname) throws IOException {
String timeStamp = new SimpleDateFormat("yyyyMMddhhmmss").format(new Date());
TakesScreenshot takesScreenshot = (TakesScreenshot) driver;
File source = takesScreenshot.getScreenshotAs(OutputType.FILE);
String destination = System.getProperty("user.dir") + "\\screenshots\\" + tname + "_" + timeStamp + ".png";
try {
FileUtils.copyFile(source, new File(destination));
} catch (Exception e) {
e.getMessage();
}
return destination;
}
} |
import { useQuery } from '@apollo/client'
import { useState } from 'react'
import { FeedDocument, UserFullDocument } from '../graphql/gql'
import moment from 'moment'
import LoadingScreen from './LoadingScreen'
import Avatar from './Avatar'
import Post from './Post'
type Props = {
current: boolean
name: string
userId: number
}
export default function Profile({ current, name, userId }: Props) {
const [section, setSection] = useState('posts')
const { data, loading, error } = useQuery(UserFullDocument, {
variables: {
userId: userId
}
})
const {
data: feedData,
loading: feedLoading,
error: feedError
} = useQuery(FeedDocument, {
variables: {
limit: 20,
offset: 0,
// TODO: set this to section to switch between posts, likes & comments
selection: 'profile',
userId: 0
},
fetchPolicy: 'cache-and-network'
})
if (!data || loading) return <LoadingScreen />
const userData = {
following: data.user.following,
followers: data.user.follower,
dateJoined: moment.unix(data.user.date).format('DD/MM/YY')
}
const editProfile = () => {
console.log('edit profile click')
}
const follow = () => {
console.log('follow')
}
return (
<div className="m-auto flex grow flex-col rounded-2xl border-2 border-white text-center sm:w-[640px] md:w-[768px]">
<header className="flex items-center justify-around px-48">
<Avatar name={name} size={128} />
<h1>{name}</h1>
</header>
<div className="profile_panel flex flex-col items-start border-2 border-gray-500 p-4 text-2xl">
{current ? (
<button className="btn mb-4" onClick={editProfile}>
edit profile
</button>
) : (
<button className="btn mb-4" onClick={follow}>
follow
</button>
)}
<div className="profile_panel_numbers flex w-full justify-between">
<span>
{userData.followers.length} followers,{' '}
{userData.following.length} following
</span>
<span>joined {userData.dateJoined}</span>
</div>
</div>
<div className="profile_panel_select grid grid-cols-3 space-x-7 border-b-2 text-2xl">
<button className="btn" onClick={() => setSection('posts')}>
posts
</button>
<button className="btn" onClick={() => setSection('likes')}>
likes
</button>
<button className="btn" onClick={() => setSection('comments')}>
comments
</button>
</div>
{section == 'posts' ? (
<div className="posts">
{!feedLoading && feedData ? (
feedData.feed.map(post => {
return (
<Post
id={post.id}
text={post.text}
date={post.date}
reactions={post.likes}
userId={userId}
key={post.id}
/>
)
})
) : (
<LoadingScreen />
)}
</div>
) : section == 'likes' ? (
<div className="likes">
{/* <Post
id={798324}
text="like me pls"
reactionsCount={{
cool: 1488,
nice: 19,
angry: 2,
shit: 0,
user_reaction: 'cool'
}}
date={Date.now()}
user={'anton'}
/>
<Post
id={798325}
text="Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum"
reactionsCount={{
cool: 322,
nice: 135,
angry: 3452,
shit: 2345,
user_reaction: 'nice'
}}
date={1665491590000}
user={'oleg'}
/> */}
likes will be here
</div>
) : section == 'comments' ? (
<div className="comments">
{/* <Post
id={798326}
text="wow"
reactionsCount={{
cool: 2354,
nice: 234,
angry: 65,
shit: 587,
user_reaction: 'shit'
}}
date={Date.now()}
user={'danya'}
/>
<Post
id={798327}
text="amazing"
reactionsCount={{
cool: 5867,
nice: 98,
angry: 40,
shit: 0,
user_reaction: 'cool'
}}
date={Date.now()}
user={'danya'}
/>
<Post
id={798328}
text="this will be dead..."
reactionsCount={{
cool: 32043,
nice: 123,
angry: 2030,
shit: 1,
user_reaction: 'angry'
}}
date={Date.now()}
user={'valera'}
/> */}
comments will be here
</div>
) : (
<div className="error">wtf</div>
)}
</div>
)
} |
// users.js
const router = require("express").Router();
const { User, validate } = require("../models/user");
const bcrypt = require("bcrypt");
const auth = require("../middleware/auth");
const admin = require("../middleware/admin");
const validObjectId = require("../middleware/validObjectId");
// POST User to create a new user
router.post("/", async (req, res) => {
const { error } = validate(req.body);
if (error) return res.status(400).send({ message: error.details[0].message });
const userToCreate = await User.findOne({ email: req.body.email });
if (userToCreate)
return res.status(403).send({ message: "User with given email already exists!!!" })
const salt = await bcrypt.genSalt(Number(process.env.SALT) || 10);
const hashPassword = await bcrypt.hash(req.body.password, salt);
let newUser = await new User({
...req.body,
password: hashPassword
}).save()
newUser.password = undefined;
newUser.__v = undefined;
res.status(200).send({ data: newUser, message: "Account Created Successfully!" })
});
// GET User to get all Users routes
router.get("/", admin, async (req, res) => {
const usersToGet = await User.find().select("-password -__v");
res.status(200).send({data: usersToGet});
});
// GET User by Id
router.get("/:id", [validObjectId, auth], async (req, res) => {
const userToGet = await User.findById(req.params.id).select("-password -__v");
res.status(200).send({ data: userToGet });
});
// UPDATE user by Id
router.put("/:id", [validObjectId, auth], async (req, res) => {
try {
const userToUpdate = await User.findByIdAndUpdate(
req.params.id,
{$set: req.body},
{ new: true })
.select("-password -__v");
res.status(200).send({ data: userToUpdate });
} catch (error) {
res.status(500).send({ message: error.message });
}
});
// DELETE user by id
router.delete("/:id", [validObjectId, admin], async (req, res) => {
try {
const userToDelete = await User.findByIdAndDelete(req.params.id);
res.status(200).send({data: userToDelete, message: "Successfully Deleted User"})
} catch (error) {
res.status(500).send(error);
}
});
module.exports = router; |
import { useEffect, useState } from "react";
import { Flex, Radio, RadioGroup, Stack, useColorMode } from "@chakra-ui/react";
const LOCAL_STORAGE_KEY = "chakra-ui-color-mode";
type ColorModeValue = "light" | "dark" | "system";
const ColorModeSelector: React.VFC = () => {
const { colorMode: chakraColorMode, setColorMode: setChakraColorMode } =
useColorMode();
const [colorMode, setColorMode] = useState<ColorModeValue>("system");
useEffect(() => {
if (localStorage.getItem(LOCAL_STORAGE_KEY) === null) {
setColorMode("system");
} else {
setColorMode(chakraColorMode);
}
}, []);
const updateColorMode = (value: ColorModeValue) => {
if (value !== colorMode) {
setColorMode(value);
if (value === "system") {
localStorage.removeItem(LOCAL_STORAGE_KEY);
location.reload();
} else {
setChakraColorMode(value);
}
}
};
return (
<Flex gap="2">
<RadioGroup
onChange={(value) => {
updateColorMode(value as ColorModeValue);
}}
value={colorMode}
>
<Stack>
<Radio value="system">デバイス設定を使用</Radio>
<Radio value="light">ライトテーマ</Radio>
<Radio value="dark">ダークテーマ</Radio>
</Stack>
</RadioGroup>
</Flex>
);
};
export default ColorModeSelector; |
package com.mz.ddd.common.persistence.eventsourcing.aggregate
import com.mz.ddd.common.api.domain.Aggregate
import com.mz.ddd.common.api.domain.DomainCommand
import com.mz.ddd.common.api.domain.DomainEvent
import com.mz.ddd.common.api.domain.Id
import reactor.core.publisher.Mono
/**
* Data class representing the effect of a command on an aggregate.
* It contains the updated aggregate and the list of domain events generated by the command.
*
* @param aggregate The updated aggregate after the command execution.
* @param events The list of domain events generated by the command.
*/
data class CommandEffect<A : Aggregate, E : DomainEvent>(val aggregate: A, val events: List<E> = listOf())
/**
* Interface for the repository of aggregates.
* It provides methods to execute commands on aggregates and to find aggregates by their ID.
*
* @param A The type of the aggregate.
* @param C The type of the command.
* @param E The type of the domain event.
*/
interface AggregateRepository<A : Aggregate, C : DomainCommand, E : DomainEvent> {
/**
* Executes a command on an aggregate.
* Optionally, a pre-execution step can be performed exclusively before the command execution.
*
* @param id The ID of the aggregate.
* @param command The command to be executed.
* @param exclusivePreExecute An optional pre-execution step to be performed exclusively before the command execution.
* @return A Mono emitting the effect of the command on the aggregate.
*/
fun execute(id: Id, command: C, exclusivePreExecute: (() -> Mono<Boolean>)? = null): Mono<CommandEffect<A, E>>
/**
* Finds an aggregate by its ID.
*
* @param id The ID of the aggregate.
* @return A Mono emitting the found aggregate.
*/
fun find(id: Id): Mono<A>
} |
#!/bin/python3
class List:
def __init__(self, value=None):
self.val = value
self.next = None
class DList(List):
def __init__(self, value=None):
List.__init__(self)
self.prev = None
self.val = value
class ListHead:
def __init__(self):
self.head = None
def add(self, val):
l = List(val)
tracer = self.head
if (tracer == None):
self.head = l
return
while (tracer.next != None):
tracer = tracer.next
tracer.next = l
def insert(self, val):
l = List(val)
l.next = self.head
self.head = l
def remove(self, val):
tracer = self.head
index = 0
if (self.head.val == val):
self.head = self.head.next
return index
index += 1
while (tracer.next != None and tracer.next.val != val):
tracer = tracer.next
index += 1
if (tracer.next == None):
print(f"Failed to find value {val} in list")
return -1
tracer.next = tracer.next.next # remove the value
return index
def print(self):
print("Printing linked list (forward)")
tracer = self.head
index = 0
if (tracer == None):
print("Empty list.")
return -1
while (tracer != None):
print(f"Index: {index}\t - {tracer.val}")
tracer = tracer.next
index += 1
class CListHead(ListHead):
def __init__(self):
ListHead.__init__(self)
self.tail = None
def add(self, val):
l = DList(val)
tracer = self.head
if (tracer == None):
self.head = l
self.tail = l
return
while (tracer.next != None):
tracer = tracer.next
l.prev = tracer
tracer.next = l
self.tail = l
def insert(self, val):
l = DList(val)
l.next = self.head
self.head.prev = l # Circular
self.head = l
if (self.tail == None):
self.tail = self.head
def remove(self, val):
tracer = self.head
index = 0
if (self.head.val == val):
self.head = self.head.next
return index
index += 1
while (tracer.next != None and tracer.next.val != val):
tracer = tracer.next
index += 1
if (tracer.next == None):
print(f"Failed to find value {val} in list")
return -1
tracer.next = tracer.next.next # remove the value
if (tracer.next == None):
self.tail = tracer
else:
tracer.next.prev = tracer
return index
def print_reverse(self): # Extend print function since we have circular pointer capabilities
print("Printing linked list (reverse)")
tracer = self.tail
index = 0
if (tracer == None):
print("Empty list.")
return -1
while (tracer != self.head.prev):
print(f"Index: {index}\t - {tracer.val}")
tracer = tracer.prev
index += 1
print("Showing examples for singly linked lists\n");
head = ListHead()
head.print(); # Empty list
head.add(", World");
head.insert("Hello");
head.add("!");
head.print(); # Hello, World!
res = head.remove("Hello");
if (res != -1):
print(f"Removed item from index {res}\n");
else:
print("Failed to find item in list\n");
res = head.remove("!");
head.print(); # , World
res = head.remove("????"); # Doesn't exist
head.print(); # , World!
## Same again but with DList/circular
print("\n\n***Showing examples for doubly and circular linked lists***\n");
head = CListHead()
head.print(); # Empty list
head.print_reverse(); # Empty list
head.add(", World");
head.insert("Hello");
head.add("!");
head.print(); # Hello, World !
head.print_reverse(); # , World ! Hello
res = head.remove("Hello");
if (res != -1):
print(f"Removed item from index {res}\n");
else:
print("Failed to find item in list\n");
res = head.remove("!");
head.print(); # , World
head.print_reverse(); #, World
res = head.remove("????"); # Doesn't exist
head.print(); # , World!
head.print_reverse(); |
/**
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.soybeanMilk.example.resolver;
import java.util.List;
import java.util.Set;
/**
* SoybeanMilk示例
* @author [email protected]
* @date 2012-5-10
*/
public class GenericResolver
{
public static abstract class BaseResolver<T>
{
public void simple(T t)
{
System.out.println();
System.out.println("T value is :"+t.toString()+", type is :"+t.getClass().getName());
System.out.println();
}
public void list(List<T> list)
{
System.out.println();
if(list != null)
{
for(int i=0; i<list.size(); i++)
{
T t=list.get(i);
System.out.println("List<T> "+i+" value is :"+t.toString()+", type is :"+t.getClass().getName());
}
}
System.out.println();
}
public void set(Set<T> set)
{
System.out.println();
if(set != null)
{
int i=0;
for(T t : set)
{
System.out.println("Set<T> "+i+" value is :"+t.toString()+", type is :"+t.getClass().getName());
i++;
}
}
System.out.println();
}
public void array(T[] array)
{
System.out.println();
if(array != null)
{
int i=0;
for(T t : array)
{
System.out.println("T[] "+i+" value is :"+t.toString()+", type is :"+t.getClass().getName());
i++;
}
}
System.out.println();
}
}
public static class MyResolver extends BaseResolver<Integer>{}
} |
//
// CommunityServiceNoteView.swift
// ReflectEarlyTesting2
//
// Created by Greydon O'Keefe on 3/4/23.
//
import Firebase
import FirebaseFirestoreSwift
import Foundation
import SwiftUI
struct CommunityServiceNoteView: View {
@StateObject var communityServiceViewModel = CommunityServiceViewModel()
@ObservedObject var studentService = StudentService.studentService
@State private var presentNewOrganizationForm = false
var body: some View {
Form {
Section(header: Text("Organization")) {
Picker("Organization", selection: $communityServiceViewModel.model.organization) {
Text("Select").tag("Select") //basically added empty tag and it solve the case
ForEach(studentService.student?.organizations ?? [], id: \.self) { organization in
Text(organization)
.font(.custom("Outfit-Light", size: 15))
}
}
Button(action: {
presentNewOrganizationForm = true
}, label: {
HStack {
Spacer()
Text("Add Organization")
.font(.custom("Outfit-Semibold", size: 15))
.foregroundColor(.mint)
Spacer()
}
})
.buttonStyle(.plain)
.alert("New Organization", isPresented: $presentNewOrganizationForm, actions: {
TextField("New Organization", text: $communityServiceViewModel.newOrganization)
Button("Create", action: {
if !(studentService.student?.organizations.contains(communityServiceViewModel.newOrganization) ?? false) {
studentService.student?.organizations.append(communityServiceViewModel.newOrganization)
}
communityServiceViewModel.newOrganization = ""
})
Button("Cancel", role: .cancel, action: {
communityServiceViewModel.newOrganization = ""
})
}, message: {
Text("Type the name of the new organization")
})
}
Section(header: Text("Note Name")) {
TextField("", text: $communityServiceViewModel.model.noteName)
}
Section(header: Text("Hours")) {
HStack {
Slider(value: $communityServiceViewModel.model.hours, in: 0...5, step: 0.25)
.tint(.mint)
Text("\(communityServiceViewModel.model.hours, specifier: "%.2f")")
}
}
Section(header: Text("Describe Your Experience")) {
TextEditor(text: $communityServiceViewModel.model.description)
}
Section(header: Text("Specific Detail")) {
TextEditor(text: $communityServiceViewModel.model.keyDetail)
}
Section(header: Text("What Does this reveal about you?")) {
TextEditor(text: $communityServiceViewModel.model.revelation)
}
Section(header: Text("Write freely")) {
TextEditor(text: $communityServiceViewModel.model.freeThought)
}
}
.font(.custom("Outfit-Light", size: 15))
.alert(communityServiceViewModel.alertMessage, isPresented: $communityServiceViewModel.showAlert) {
Button("OK", role: .cancel) { }
}
.navigationBarTitleDisplayMode(.inline)
.toolbar {
ToolbarItem {
Button(action: communityServiceViewModel.write, label: { Text("SAVE").font(.custom("Outfit-Bold", size: 17)).foregroundColor(.mint) })
.buttonStyle(.plain)
}
}
}
}
/// want it to pull users organizations so they can pick from this
class CommunityServiceViewModel: ObservableObject {
@Published var model = CommunityServiceModel()
@ObservedObject var studentService = StudentService.studentService
@Published var newOrganization = ""
@Published var showAlert = false
@Published var alertMessage = "Saved Successfully"
func write() {
do {
model.userId = Auth.auth().currentUser?.uid
_ = try Firestore.firestore().collection("communityServiceReflections").addDocument(from: model)
studentService.addPoints(
[self.model.organization,
self.model.noteName,
self.model.description,
self.model.keyDetail,
self.model.revelation,
self.model.freeThought
]
)
studentService.student?.serviceGoals[0] += Float(self.model.hours)
alertMessage = "Saved Successfully"
showAlert = true
} catch {
print("\(error.localizedDescription)")
alertMessage = "Error. Retry."
showAlert = true
}
}
}
struct CommunityServiceModel: Identifiable, Codable {
@DocumentID var id: String?
var date: Timestamp = Timestamp(date: Date())
var noteName: String = ""
var organization: String = ""
var hours: Double = 1.0
var description: String = ""
var keyDetail: String = ""
var revelation: String = ""
var freeThought: String = ""
var userId: String?
}
struct CommunityServiceNoteView_Previews: PreviewProvider {
static var previews: some View {
CommunityServiceNoteView()
}
} |
defmodule IndieWeb.CacheTest do
use IndieWeb.TestCase, async: true
alias IndieWeb.Cache, as: Subject
doctest Subject
describe ".adapter/0" do
test "defaults to using Cachex" do
assert Subject.adapter() == IndieWeb.Cache.Adapters.Cachex
end
end
describe ".get/2" do
@describetag skip: true
test "defaults to provided value" do
assert "default_value" =
IndieWeb.Cache.get("foo_not_found", "default_value")
end
test "finds value in cache" do
IndieWeb.Cache.set("foo", "in_cache", [])
assert "in_cache" = IndieWeb.Cache.get("foo")
end
test "nils out if value not found" do
refute IndieWeb.Cache.get("foo_not_found")
end
end
describe ".set/2" do
test "adds value to cache" do
assert IndieWeb.Cache.set("foo", "bar")
assert "bar" = IndieWeb.Cache.get("foo")
end
end
describe ".delete/1" do
test "removes value from cache" do
assert IndieWeb.Cache.set("foo", "in_cache", [])
assert IndieWeb.Cache.delete("foo")
assert is_nil(IndieWeb.Cache.get("foo"))
end
end
end |
import { Injectable } from '@angular/core';
import { ToastController } from '@ionic/angular';
@Injectable({
providedIn: 'root'
})
export class ToastsService {
constructor(
public toastController: ToastController
) { }
async error(error: string) {
const toast = await this.toastController.create({
message: error,
color: 'warning',
duration: 4000
});
toast.present();
}
async info(info: string) {
const toast = await this.toastController.create({
message: info,
duration: 4000
});
toast.present();
}
} |
import 'package:flutter/material.dart';
import 'package:flutter_rating_bar/flutter_rating_bar.dart';
import 'package:medical_clinic_team13/core/theming/consts/app_fonts.dart';
import '../../../../core/theming/consts/app_colors.dart';
import '../../../../core/theming/consts/size_config.dart';
class VerticalDoctorCard extends StatefulWidget {
VerticalDoctorCard({
super.key,
required this.image,
required this.doctorName,
this.yearsExperience,
this.typeDisease,
this.ratingWidget,
});
final String doctorName;
final String? yearsExperience;
final String? typeDisease;
final Widget? ratingWidget;
final String image;
@override
State<VerticalDoctorCard> createState() => _VerticalDoctorCardState();
}
class _VerticalDoctorCardState extends State<VerticalDoctorCard> {
double? _ratingValue;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.only(top: 2, right: 6, left: 6),
child: Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(12),
color: AppColors.primary,
),
width: SizeConfig.screenWidth * 0.49,
//height: SizeConfig.screenHeight * 6,
child: Column(
children: [
SizedBox(height: 10),
Container(
width: SizeConfig.screenWidth * 0.27,
height: SizeConfig.screenHeight * 0.15,
decoration: BoxDecoration(
border: Border.all(
color: AppColors.secondary,
width: 2.0,
),
),
child: Image.asset(widget.image),
),
Padding(
padding: EdgeInsets.only(top: 5),
child: Text(
textAlign: TextAlign.center,
widget.doctorName,
style: AppFonts.font18W600deepBlue.copyWith(
color: AppColors.secondary,
),
),
),
Padding(
padding: EdgeInsets.only(top: 1),
child: Text(
textAlign: TextAlign.center,
widget.yearsExperience!,
style: AppFonts.font16W400WhiteNormal.copyWith(
fontSize: 12,
),
),
),
Padding(
padding: EdgeInsets.only(top: 5),
child: RatingBar(
initialRating: 0,
direction: Axis.horizontal,
allowHalfRating: true,
itemCount: 5,
itemSize: 20,
ratingWidget: RatingWidget(
full: const Icon(
Icons.star,
color: AppColors.star,
),
half: const Icon(
Icons.star_half,
color: AppColors.star,
),
empty: const Icon(
Icons.star_outline,
color: AppColors.secondary,
)),
onRatingUpdate: (value) {
setState(() {
_ratingValue = value;
});
}),
),
],
),
),
);
}
} |
---
title: "[SPRING] 검증 - BindingResult & FieldError/ObjectError "
categories:
- Spring
tags:
- [Framework, Spring, Java]
toc: true
toc_sticky: true
date: 2022-06-30 18:08:10
last_modified_at: 2022-06-30 18:08:13
---
# 검증 요구사항
상품 관리 시스템에 새로운 요구사항이 추가되었다.
- 타입 검증<br>- 가격, 수량에 문자가 들어가면 검증 오류 처리
- 필드 검증<br>- 상품명: 필수, 공백X<br>- 가격: 1000원 이상, 1백만원 이하 <br>- 수량: 최대 9999
- 특정 필드의 범위를 넘어서는 검증<br>- 가격 * 수량의 합은 10,000원 이상
지금까지 만든 웹 애플리케이션은 폼 입력시 숫자를 문자로 작성하거나해서 검증 오류가 발생하면 오류 화면으로 바로 이동한다. <br>
웹 서비스는 폼 입력시 오류가 발생하면, 고객이 입력한 데이터를 유지한 상태로 어떤 오류가 발생했는지 친절하게 알려주어야 한다.<br><br>
컨트롤러의 중요한 역할중 하나는 HTTP 요청이 정상인지 검증하는 것이다. <br>
그리고 정상 로직보다 이런 검증 로직을 잘 개발하는 것이 어쩌면 더 어려울 수 있다.
> 참고: 클라이언트 검증, 서버 검증<br>
- 클라이언트 검증은 조작할 수 있으므로 보안에 취약하다.
- 서버만으로 검증하면, 즉각적인 고객 사용성이 부족해진다.
- 둘을 적절히 섞어서 사용하되, 최종적으로 서버 검증은 필수
- API 방식을 사용하면 API 스펙을 잘 정의해서 검증 오류를 API 응답 결과에 잘 남겨주어야 함
먼저 검증을 직접 구현해보고, 뒤에서 스프링과 타임리프가 제공하는 검증 기능을 활용해보자.
# V1 - 검증 직접 처리
이전 프로젝트에 이어서 검증(Validation) 기능을 학습해보자.
## 소개
### 상품 저장 성공
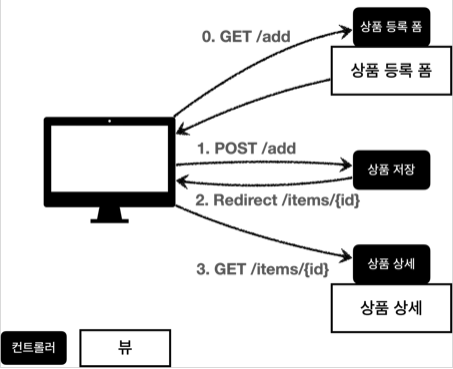<br>
사용자가 상품 등록 폼에서 정상 범위의 데이터를 입력하면, 서버에서는 검증 로직이 통과하고, 상품을 저장하고, 상품 상세 화면으로 redirect한다.
### 상품 저장 실패
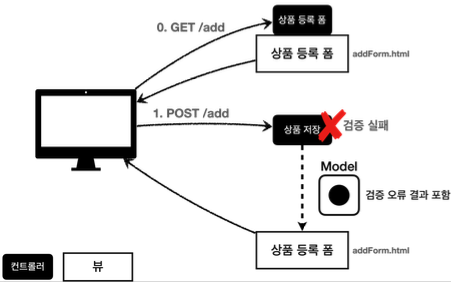<br>
고객이 상품 등록 폼에서 상품명을 입력하지 않거나, 가격, 수량 등이 너무 작거나 커서 검증 범위를 넘어서면, 서버 검증 로직이 실패해야 한다. <br>
이렇게 검증에 실패한 경우 고객에게 다시 상품 등록 폼을 보여주고, 어떤 값을 잘못 입력했는지 친절하게 알려주어야 한다.<br><br>
이제 요구사항에 맞추어 검증 로직을 직접 개발해보자.
## 개발
### ☂️ 상품 등록 검증
#### 컨트롤러 - `ValidationItemControllerV1`
```java
@Slf4j
@Controller
@RequestMapping("/validation/v1/items")
@RequiredArgsConstructor
public class ValidationItemControllerV1 {
...
@PostMapping("/add")
public String addItem(@ModelAttribute Item item, RedirectAttributes redirectAttributes, Model model) {
// 1) 검증 오류 결과를 보관
Map<String, String> errors = new HashMap<>();
// 2) 검증 로직
if (!StringUtils.hasText(item.getItemName())){
errors.put("itemName", "상품 이름은 필수입니다.");
}
if (item.getPrice()==null || item.getPrice()<1000 || item.getPrice()>1000000){
errors.put("price", "가격은 1,000 ~ 1,000,000 까지 허용합니다.");
}
if (item.getQuantity()==null || item.getQuantity()>=9999){
errors.put("quantity", "수량은 최대 9,999 까지 허용합니다.");
}
// 3) 특정 필드가 아닌 복합 룰 검증
if (item.getPrice()!=null && item.getQuantity()!=null){
int resultPrice = item.getPrice() * item.getQuantity();
if (resultPrice<10000){
errors.put("globalError", "가격 * 수량의 합은 10,000원 이상이어야 합니다. 현재 값 = " + resultPrice);
}
}
// 4) 검증에 실패하면 다시 입력 폼으로
if (!errors.isEmpty()){
log.info("errors = {}", errors);
model.addAttribute("errors", errors);
return "validation/v1/addForm";
}
// 성공 로직
Item savedItem = itemRepository.save(item);
redirectAttributes.addAttribute("itemId", savedItem.getId());
redirectAttributes.addAttribute("status", true);
return "redirect:/validation/v1/items/{itemId}";
}
...
}
```
##### 1) 검증 오류 보관
`Map<String, String> errors = new HashMap<>();`<br>
만약 검증시 오류가 발생하면 어떤 검증에서 오류가 발생했는지 정보를 담아둔다.
##### 2) 검증 로직
```java
if (!StringUtils.hasText(item.getItemName())) {
errors.put("itemName", "상품 이름은 필수입니다.");
}
```
📍 `import org.springframework.util.StringUtils;` 추가 필요<br><br>
검증시 오류가 발생하면 `errors` 에 담아둔다. <br>
이때 어떤 필드에서 오류가 발생했는지 구분하기 위해 오류가 발생한 필드명을 `key` 로 사용한다. <br>
이후 뷰에서 이 데이터를 사용해서 고객에게 친절한 오류 메시지를 출력할 수 있다.
##### 3) 특정 필드의 범위를 넘어서는 검증 로직
특정 필드를 넘어서는 오류를 처리해야 할 수도 있다. <br>
이때는 필드 이름을 넣을 수 없으므로 `globalError` 라는 `key` 를 사용하였다.
##### 4) 검증에 실패하면 다시 입력 폼으로
만약 검증에서 오류 메시지가 하나라도 있으면 오류 메시지를 출력하기 위해 `model`에 `errors` 를 담고, 입력 폼이 있는 뷰 템플릿으로 보낸다.
#### 뷰 - `addForm.html`
`validation/v1/addForm.html` 수정
```html
...
<style>
.container {
max-width: 560px;
}
.field-error {
border-color: #dc3545;
color: #dc3545;
}
</style>
...
<form action="item.html" th:action th:object="${item}" method="post">
<div th:if="${errors?.containsKey('globalError')}">
<p class="field-error" th:text="${errors['globalError']}">전체 오류 메시지</p>
</div>
<div>
<label for="itemName" th:text="#{label.item.itemName}">상품명</label>
<input type="text" id="itemName" th:field="*{itemName}"
th:class="${errors?.containsKey('itemName')} ? 'form-control field-error' : 'form-control'"
class="form-control"
placeholder="이름을 입력하세요">
<div class="field-error" th:if="${errors?.containsKey('itemName')}" th:text="${errors['itemName']}">
상품명 오류
</div>
</div>
<div>
<label for="price" th:text="#{label.item.price}">가격</label>
<input type="text" id="price" th:field="*{price}"
th:classappend="${errors?.containsKey('price')} ? 'field-error' : _"
class="form-control"
placeholder="가격을 입력하세요">
<div class="field-error" th:if="${errors?.containsKey('price')}" th:text="${errors['price']}">
가격 오류
</div>
</div>
<div>
<label for="quantity" th:text="#{label.item.quantity}">수량</label>
<input type="text" id="quantity" th:field="*{quantity}" class="form-control"
th:classappend="${errors?.containsKey('quantity')} ? 'field-error' : _"
placeholder="수량을 입력하세요">
<div class="field-error" th:if="${errors?.containsKey('quantity')}" th:text="${errors['quantity']}">
수량 오류
</div>
</div>
<hr class="my-4">
...
```
##### css 추가
이 부분은 오류 메시지를 빨간색으로 강조하기 위해 추가했다.
##### 전체 오류 메시지
오류 메시지는 `errors` 에 내용이 있을 때만 출력하면 된다. <br>
타임리프의 `th:if` 를 사용하면 조건에 만족할 때만 해당 HTML 태그를 출력할 수 있다.
> 💡 참고 - Safe Navigation Operator (`?.`)<br>
만약 여기에서 `errors` 가 `null` 이라면 어떻게 될까? 생각해보면 등록폼에 진입한 시점에는 `errors` 가 없다.<br>
따라서 `errors.containsKey()` 를 호출하는 순간 `NullPointerException` 이 발생한다.<br><br>
`errors?.` 은 `errors` 가 `null` 일때 `NullPointerException` 이 발생하는 대신, `null` 을 반환하는 문법이다.<br>
`th:if` 에서 `null` 은 실패로 처리되므로 오류 메시지가 출력되지 않는다.<br>
이것은 스프링의 SpringEL이 제공하는 문법이다.
##### 필드 오류 처리 - `th:classappend`
- `th:class="${errors?.containsKey('itemName')} ? 'form-control field-error' : 'form-control'"`
- `th:classappend="${errors?.containsKey('itemName')} ? 'field-error' : _"` 🌟
위의 두 코드는 같은 의미이다. `th:class`를 사용할 때보다 `th:classappend` 를 사용하면 더 깔끔하게 코드를 구성할 수 있다.<br><br>
`classappend` 를 사용해서 해당 필드에 오류가 있으면 `field-error` 라는 클래스 정보를 더해서 폼의 색깔을 빨간색으로 강조한다. <br>
만약 값이 없으면 `_` (No-Operation)을 사용해서 아무것도 하지 않는다.
#### 정리
- 만약 검증 오류가 발생하면 입력 폼을 다시 보여준다.
- 검증 오류들을 고객에게 친절하게 안내해서 다시 입력할 수 있게 한다.
- 검증 오류가 발생해도 고객이 입력한 데이터가 유지된다.
지금까지는 상품 등록의 검증을 살펴봤다.<br>
상품 수정의 검증은 더 효율적인 검증 처리 방법을 학습한 다음에 진행한다.
##### 남은 문제점
- 뷰 템플릿에서 중복 처리가 많다. 뭔가 비슷하다.
- 타입 오류 처리가 안된다. <br>
`Item` 의 `price` , `quantity` 같은 숫자 필드는 타입이 `Integer` 이므로 문자 타입으로 설정하는 것이 불가능하다. <br>
숫자 타입에 문자가 들어오면 오류가 발생한다. 그런데 이러한 오류는 스프링MVC에서 컨트롤러에 진입하기도 전에 예외가 발생하기 때문에, 컨트롤러가 호출되지도 않고, 400 예외가 발생하면서 오류 페이지를 띄워준다.
- `Item`의 `price`에 문자를 입력하는 것처럼 타입 오류가 발생해도 고객이 입력한 문자를 화면에 남겨야 한다. <br>
만약 컨트롤러가 호출된다고 가정해도 `Item` 의 `price` 는 `Integer` 이므로 문자를 보관할 수가 없다. <br>
결국 문자는 바인딩이 불가능하므로 고객이 입력한 문자가 사라지게 되고, 고객은 본인이 어떤 내용을 입력해서 오류가 발생했는지 이해하기 어렵다.
- 결국 고객이 입력한 값도 어딘가에 별도로 관리가 되어야 한다.
# V2 - 스프링 제공
지금부터 스프링이 제공하는 검증 오류 처리 방법을 하나씩 알아보자. 여기서 핵심은 BindingResult이다. 우선 코드로 확인해보자.
## 1. BindingResult 1
### 컨트롤러 - `ValidationItemControllerV2`
#### `addItemV1` - `FieldError`&`ObjectError`
```java
@Slf4j
@Controller
@RequestMapping("/validation/v2/items")
@RequiredArgsConstructor
public class ValidationItemControllerV2 {
...
@PostMapping("/add")
public String addItemV1(@ModelAttribute Item item, BindingResult bindingResult, RedirectAttributes redirectAttributes, Model model) {
// 검증 로직
if (!StringUtils.hasText(item.getItemName())){
bindingResult.addError(new FieldError("item","itemName", "상품 이름은 필수입니다."));
}
if (item.getPrice()==null || item.getPrice()<1000 || item.getPrice()>1000000){
bindingResult.addError(new FieldError("item","price", "가격은 1,000 ~ 1,000,000 까지 허용합니다."));
}
if (item.getQuantity()==null || item.getQuantity()>=9999){
bindingResult.addError(new FieldError("item","quantity", "수량은 최대 9,999 까지 허용합니다."));
}
// 특정 필드가 아닌 복합 룰 검증
if (item.getPrice()!=null && item.getQuantity()!=null){
int resultPrice = item.getPrice() * item.getQuantity();
if (resultPrice<10000){
bindingResult.addError(new ObjectError("item", "가격 * 수량의 합은 10,000원 이상이어야 합니다. 현재 값 = " + resultPrice));
}
}
// 검증에 실패하면 다시 입력 폼으로
if (bindingResult.hasErrors()){
log.info("errors={}", bindingResult);
return "validation/v2/addForm";
}
// 성공 로직
Item savedItem = itemRepository.save(item);
redirectAttributes.addAttribute("itemId", savedItem.getId());
redirectAttributes.addAttribute("status", true);
return "redirect:/validation/v2/items/{itemId}";
}
...
}
```
> 주의 🚨<br>
`BindingResult bindingResult` 파라미터의 위치는 `@ModelAttribute Item item` 다음에 와야 한다.
- 이전 코드와의 차이점
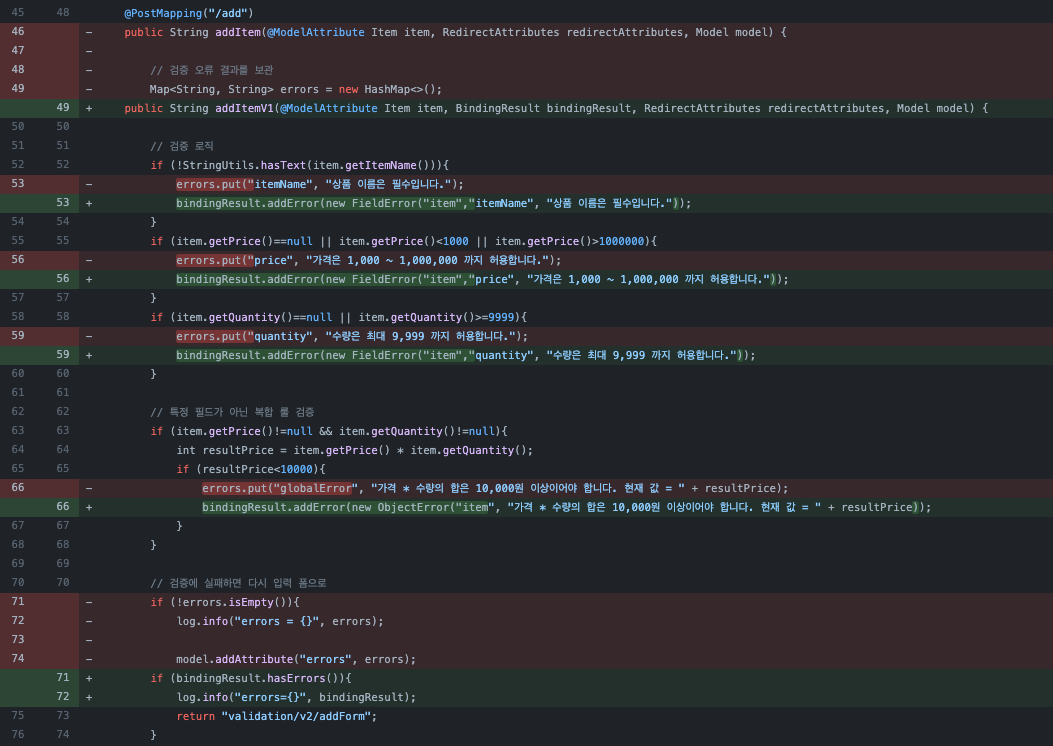
#### 필드 오류 - `FieldError`
```java
if (!StringUtils.hasText(item.getItemName())){
bindingResult.addError(new FieldError("item","itemName", "상품 이름은 필수입니다."));
}
```
- `FieldError` 생성자 - `public FieldError(String objectName, String field, String defaultMessage) {}`<br>
• `objectName` : `@ModelAttribute` 이름<br>
• `field` : 오류가 발생한 필드 이름<br>
• `defaultMessage` : 오류 기본 메시지
필드에 오류가 있으면 `FieldError` 객체를 생성해서 `bindingResult` 에 담아두면 된다.
#### 글로벌 오류 - `ObjectError`
```java
if (item.getPrice()!=null && item.getQuantity()!=null){
int resultPrice = item.getPrice() * item.getQuantity();
if (resultPrice<10000){
bindingResult.addError(new ObjectError("item", "가격 * 수량의 합은 10,000원 이상이어야 합니다. 현재 값 = " + resultPrice));
}
}
```
- `ObjectError` 생성자 - `public ObjectError(String objectName, String defaultMessage) {}`<br>
• `objectName` : `@ModelAttribute` 의 이름<br>
• `defaultMessage` : 오류 기본 메시지
특정 필드를 넘어서는 오류가 있으면 `ObjectError` 객체를 생성해서 `bindingResult` 에 담아두면 된다.
### 뷰 - `addForm.html`
`validation/v2/addForm.html` 수정
```html
...
<div class="py-5 text-center">
<h2 th:text="#{page.addItem}">상품 등록</h2>
</div>
<form action="item.html" th:action th:object="${item}" method="post">
<div th:if="${#fields.hasGlobalErrors()}">
<p class="field-error" th:each="err : ${#fields.globalErrors()}" th:text="${err}">글로벌 오류 메시지</p>
</div>
<div>
<label for="itemName" th:text="#{label.item.itemName}">상품명</label>
<input type="text" id="itemName" th:field="*{itemName}"
th:errorclass="field-error" class="form-control"
placeholder="이름을 입력하세요">
<div class="field-error" th:errors="*{itemName}">
상품명 오류
</div>
</div>
<div>
<label for="price" th:text="#{label.item.price}">가격</label>
<input type="text" id="price" th:field="*{price}"
th:errorclass="field-error" class="form-control"
placeholder="가격을 입력하세요">
<div class="field-error" th:errors="*{price}">
가격 오류
</div>
</div>
<div>
<label for="quantity" th:text="#{label.item.quantity}">수량</label>
<input type="text" id="quantity" th:field="*{quantity}"
th:errorclass="field-error" class="form-control"
placeholder="수량을 입력하세요">
<div class="field-error" th:errors="*{quantity}">
수량 오류
</div>
</div>
<hr class="my-4">
...
```
- 이전 코드와의 차이점
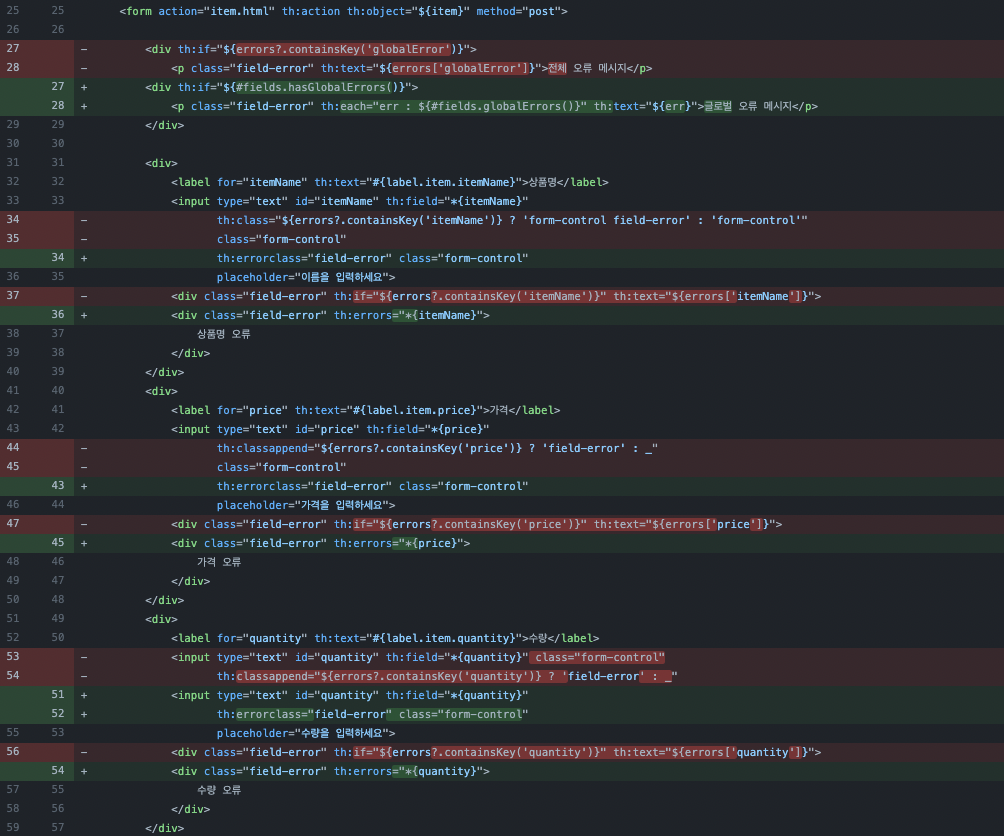
#### 필드 오류
```html
<div>
<label for="itemName" th:text="#{label.item.itemName}">상품명</label>
<input type="text" id="itemName" th:field="*{itemName}"
th:errorclass="field-error" class="form-control"
placeholder="이름을 입력하세요">
<div class="field-error" th:errors="*{itemName}">
상품명 오류
</div>
</div>
```
- `th:errors` : 해당 필드에 오류가 있는 경우에 태그를 출력한다. `th:if` 의 편의 버전이다.
- `th:errorclass` : `th:field` 에서 지정한 필드에 오류가 있으면 `class` 정보를 추가한다.
#### 글로벌 오류
```html
<div th:if="${#fields.hasGlobalErrors()}">
<p class="field-error" th:each="err : ${#fields.globalErrors()}" th:text="${err}">글로벌 오류 메시지</p>
</div>
```
- `#fields` :` #fields` 로 `BindingResult` 가 제공하는 검증 오류에 접근할 수 있다.
### 검증과 오류 메시지 공식 메뉴얼
[https://www.thymeleaf.org/doc/tutorials/3.0/thymeleafspring.html#validation-and-error-messages](https://www.thymeleaf.org/doc/tutorials/3.0/thymeleafspring.html#validation-and-error-messages)
## 2. BindingResult 2
- 스프링이 제공하는 검증 오류를 보관하는 객체이다. 검증 오류가 발생하면 여기에 보관하면 된다.
- `BindingResult` 가 있으면 `@ModelAttribute` 에 데이터 바인딩 시 오류가 발생해도 컨트롤러가 호출된다!
- 예) `@ModelAttribute`에 바인딩 시 타입 오류가 발생하면?<br>
• `BindingResult` 가 없으면 400 오류가 발생하면서 컨트롤러가 호출되지 않고, 오류 페이지로 이동한다.<br>
• `BindingResult` 가 있으면 오류 정보( `FieldError` )를 `BindingResult` 에 담아서 컨트롤러를 정상 호출한다.
> 주의 🚨
- `BindingResult` 는 검증할 대상 바로 다음에 와야한다. 순서가 중요하다. <br>
예를 들어서 `@ModelAttribute Item item` , 바로 다음에 `BindingResult` 가 와야 한다.
- `BindingResult` 는 `Model에` 자동으로 포함된다.
### BindingResult에 검증 오류를 적용하는 3가지 방법
1. `@ModelAttribute` 의 객체에 타입 오류 등으로 바인딩이 실패하는 경우 스프링이 `FieldError` 생성해서 `BindingResult` 에 넣어준다.
2. 개발자가 직접 넣어준다.
3. `Validator` 사용 -> 이것은 뒤에서 설명
### 정리
`BindingResult` , `FieldError` , `ObjectError` 를 사용해서 오류 메시지를 처리하는 방법을 알아보았다. <br>
그런데 오류가 발생하는 경우 고객이 입력한 내용이 모두 사라진다. 이 문제를 해결해보자.
## 3. FieldError, ObjectError
- 목표<br>
• 사용자 입력 오류 메시지가 화면에 남도록 하자.<br>예) 가격을 1000원 미만으로 설정시 입력한 값이 남아있어야 한다.<br>
• `FieldError` , `ObjectError` 에 대해서 더 자세히 알아보자.
### 컨트롤러 - `ValidationItemControllerV2`
#### `addItemV2` - `rejectedValue` 파라미터 사용
```java
@Slf4j
@Controller
@RequestMapping("/validation/v2/items")
@RequiredArgsConstructor
public class ValidationItemControllerV2 {
...
@PostMapping("/add")
public String addItemV2(@ModelAttribute Item item, BindingResult bindingResult, RedirectAttributes redirectAttributes, Model model) {
// 검증 로직
if (!StringUtils.hasText(item.getItemName())){
bindingResult.addError(new FieldError("item","itemName", item.getItemName(), false, null, null, "상품 이름은 필수입니다.")); // 🌟 파라미터 추가
}
if (item.getPrice()==null || item.getPrice()<1000 || item.getPrice()>1000000){
bindingResult.addError(new FieldError("item","price", item.getPrice(), false, null, null, "가격은 1,000 ~ 1,000,000 까지 허용합니다.")); // 🌟 파라미터 추가
}
if (item.getQuantity()==null || item.getQuantity()>=9999){
bindingResult.addError(new FieldError("item","quantity", item.getQuantity(), false, null, null, "수량은 최대 9,999 까지 허용합니다.")); // 🌟 파라미터 추가
}
// 특정 필드가 아닌 복합 룰 검증
if (item.getPrice()!=null && item.getQuantity()!=null){
int resultPrice = item.getPrice() * item.getQuantity();
if (resultPrice<10000){
bindingResult.addError(new ObjectError("item", null, null, "가격 * 수량의 합은 10,000원 이상이어야 합니다. 현재 값 = " + resultPrice));
}
}
// 검증에 실패하면 다시 입력 폼으로
if (bindingResult.hasErrors()){
log.info("errors={}", bindingResult);
return "validation/v2/addForm";
}
// 성공 로직
Item savedItem = itemRepository.save(item);
redirectAttributes.addAttribute("itemId", savedItem.getId());
redirectAttributes.addAttribute("status", true);
return "redirect:/validation/v2/items/{itemId}";
}
...
}
```
#### FieldError 생성자
FieldError 는 두 가지 생성자를 제공한다.
```
public FieldError(String objectName, String field, String defaultMessage);
public FieldError(String objectName, String field, @Nullable Object rejectedValue, boolean bindingFailure, @Nullable String[] codes, @Nullable Object[] arguments, @Nullable String defaultMessage)
```
- 파라미터 목록<br>
• `objectName` : 오류가 발생한 객체 이름<br>
• `field` : 오류 필드<br>
• `rejectedValue` : 사용자가 입력한 값(거절된 값)<br>
• `bindingFailure` : 타입 오류 같은 바인딩 실패인지, 검증 실패인지 구분 값
• `codes` : 메시지 코드<br>
• `arguments` : 메시지에서 사용하는 인자<br>
• `defaultMessage` : 기본 오류 메시지<br><br>
ObjectError 도 유사하게 두 가지 생성자를 제공한다. 코드를 참고하자.
#### 오류 발생 시 사용자 입력 값 유지
```java
new FieldError("item", "price", item.getPrice(), false, null, null, "가격은 1,000 ~ 1,000,000 까지 허용합니다.")
```
사용자의 입력 데이터가 컨트롤러의 `@ModelAttribute` 에 바인딩되는 시점에 오류가 발생하면 모델 객체에 사용자 입력 값을 유지하기 어렵다. <br>
예를 들어서 가격에 숫자가 아닌 문자가 입력된다면 가격은 `Integer` 타입이므로 문자를 보관할 수 있는 방법이 없다. <br>
그래서 오류가 발생한 경우 사용자 입력 값을 보관하는 별도의 방법이 필요하다. 그리고 이렇게 보관한 사용자 입력 값을 검증 오류 발생시 화면에 다시 출력하면 된다.<br>
`FieldError` 는 오류 발생시 사용자 입력 값을 저장하는 기능을 제공한다.<br><br>
여기서 `rejectedValue` 가 바로 오류 발생시 사용자 입력 값을 저장하는 필드다.<br>
`bindingFailure` 는 타입 오류 같은 바인딩이 실패했는지 여부를 적어주면 된다. 여기서는 바인딩이 실패한 것은 아니기 때문에 `false` 를 사용한다.
#### 타임리프의 사용자 입력 값 유지
`th:field="*{price}"`<br>
타임리프의 `th:field` 는 매우 똑똑하게 동작하는데, <br>
정상 상황에는 모델 객체의 값을 사용하지만, 오류가 발생하면 `FieldError` 에서 보관한 값을 사용해서 값을 출력한다.
#### 스프링의 바인딩 오류 처리
타입 오류로 바인딩에 실패하면 스프링은 `FieldError` 를 생성하면서 사용자가 입력한 값을 넣어둔다. <br>
그리고 해당 오류를 `BindingResult` 에 담아서 컨트롤러를 호출한다. <br>
따라서 타입 오류 같은 바인딩 실패시에도 사용자의 오류 메시지를 정상 출력할 수 있다.
***
<br>
💛 개인 공부 기록용 블로그입니다. 👻
[맨 위로 이동하기](#){: .btn .btn--primary }{: .align-right} |
/*
*
*
* FILL IN EACH FUNCTIONAL TEST BELOW COMPLETELY
* -----[Keep the tests in the same order!]-----
* (if additional are added, keep them at the very end!)
*/
var chaiHttp = require('chai-http');
var chai = require('chai');
var assert = chai.assert;
var server = require('../server');
chai.use(chaiHttp);
suite('Functional Tests', function() {
suite('GET /api/stock-prices => stockData object', function() {
test('1 stock', function(done) {
chai.request(server)
.get('/api/stock-prices')
.query({stock: 'cah'})
.end(function(err, res) {
assert.equal(res.status, 200);
assert.isObject(res.body, 'response should be an object');
assert.property(res.body.stockData, 'stock', 'stock property missing');
assert.property(res.body.stockData, 'price', 'price property missing');
assert.property(res.body.stockData, 'likes', 'likes property missing');
assert.equal(res.body.stockData.stock, 'cah', 'stock name missing');
done();
});
});
test('1 stock with like', function(done) {
chai.request(server)
.get('/api/stock-prices')
.query({stock: 'cbl', like: true})
.end(function(err, res){
assert.equal(res.status, 200);
assert.isObject(res.body, 'response should be an object');
assert.property(res.body.stockData, 'stock', 'stock property missing');
assert.property(res.body.stockData, 'price', 'price property missing');
assert.property(res.body.stockData, 'likes', 'likes property missing');
assert.equal(res.body.stockData.stock, 'cbl', 'stock name missing');
assert.isAtLeast(res.body.stockData.likes, 1, 'like is missing');
done();
});
});
test('1 stock with like again (ensure likes arent double counted)', function(done) {
chai.request(server)
.get('/api/stock-prices')
.query({stock: 'cbm', like: true})
.end(function(err, res){
assert.equal(res.status, 200);
assert.isObject(res.body, 'response should be an object');
assert.property(res.body.stockData, 'stock', 'stock property missing');
assert.property(res.body.stockData, 'price', 'price property missing');
assert.property(res.body.stockData, 'likes', 'likes property missing');
assert.equal(res.body.stockData.stock, 'cbm', 'stock name missing');
assert.isAtLeast(res.body.stockData.likes, 1, 'like is missing');
done();
});
});
test('2 stocks', function(done) {
chai.request(server)
.get('/api/stock-prices')
.query({stock: ['cc','cci']})
.end(function(err, res){
assert.equal(res.status, 200);
assert.isObject(res.body, 'response should be an object');
assert.property(res.body.stockData[0], 'stock', 'stock property missing');
assert.property(res.body.stockData[0], 'price', 'price property missing');
assert.property(res.body.stockData[0], 'rel_likes', 'likes property missing');
assert.equal(res.body.stockData[0].stock, 'cc', 'stock name missing');
assert.property(res.body.stockData[1], 'stock', 'stock property missing');
assert.property(res.body.stockData[1], 'price', 'price property missing');
assert.property(res.body.stockData[1], 'rel_likes', 'likes property missing');
assert.equal(res.body.stockData[1].stock, 'cci', 'stock name missing');
done();
});
});
test('2 stocks with like', function(done) {
chai.request(server)
.get('/api/stock-prices')
.query({stock: ['ccj','cck'], like: true})
.end(function(err, res){
assert.equal(res.status, 200);
assert.isObject(res.body, 'response should be an object');
assert.property(res.body.stockData[0], 'stock', 'stock property missing');
assert.property(res.body.stockData[0], 'price', 'price property missing');
assert.property(res.body.stockData[0], 'rel_likes', 'likes property missing');
assert.equal(res.body.stockData[0].stock, 'ccj', 'stock name missing');
assert.property(res.body.stockData[1], 'stock', 'stock property missing');
assert.property(res.body.stockData[1], 'price', 'price property missing');
assert.property(res.body.stockData[1], 'rel_likes', 'likes property missing');
assert.equal(res.body.stockData[1].stock, 'cck', 'stock name missing');
done();
});
});
});
}); |
# <form action="/users" method="post" accept-charset="UTF-8">
# <input type="hidden" name="authenticity_token" value="<%= form_authenticity_token %>">
# <label for="username">Username:</label>
# <input type="text" id="username" name="user[username]"><br><br>
# <label for="email">Email:</label>
# <input type="email" id="email" name="user[email]"><br><br>
# <label for="password">Password:</label>
# <input type="password" id="password" name="user[password]"><br><br>
# <input type="submit" value="Submit">
# </form>
# <%= form_tag users_path :method => 'post' do |f| %>
# <%= label_tag(:username, "username:") %>
# <%= text_field_tag ':post[username]' %> <br><br>
# <%= label_tag(:email, "enter your e-email:") %>
# <%= text_field_tag ':post[email]' %> <br><br>
# <%= label_tag(:password, "password:") %>
# <%= password_field_tag 'password' %> <br><br>
# <div> <%= submit_tag("Sign up") %> <div>
# <% end %>
<%= form_with model: @user do |form| %>
<div>
<%= form.label :username %><br>
<%= form.text_field :username %>
<% @user.errors.full_messages_for(:username).each do |message| %>
<div><%= message %></div>
<% end %>
</div>
<div>
<%= form.label :email %><br>
<%= form.text_field :email %>
<% @user.errors.full_messages_for(:email).each do |message| %>
<div><%= message %></div>
<% end %>
</div>
<div>
<%= form.label :password %><br>
<%= form.password_field :password %>
<% @user.errors.full_messages_for(:password).each do |message| %>
<div><%= message %></div>
<% end %>
</div>
<div>
<%= form.submit %>
</div>
<% end %> |
// Дана некоторая строка, например, вот такая: '023m0df0dfg0'
// Получите массив позиций всех нулей в этой в строке.
let string = '023m0df0dfg0';
let array = string.split('').map((elem, index) => elem == 0 ? index : -1).filter(elem => elem != -1);
console.log(array.join(''));
// Дана некоторая строка: 'abcdefg'
// Удалите из этой строки каждый третий символ. В нашем случае должно получится следующее: 'abdeg'
let str = 'abcdefg';
let result = str.split('').filter(function(elem, index){
if ((index + 1) % 3 !== 0) {
return elem;
}
}).join('');
console.log(result);
// Дан некоторый массив, например, вот такой:[1, 2, 3, 4, 5, 6]
// Поделите сумму элементов, стоящих на четных позициях, на сумму элементов, стоящих на нечетных позициях.
let arr = [1, 2, 3, 4, 5, 6];
let sumEven = arr.reduce(function(sum, elem, index){
if(index % 2 == 0) {
return sum += elem;
} else {
return sum;
}
}, 0)
let sumOdd = arr.reduce(function(sum, elem, index) {
if (index %2 != 0) {
return sum += elem;
} else {
return sum;
}
}, 0)
let res = sumEven / sumOdd;
console.log(res); |
<!DOCTYPE html>
<html lang="pl">
<head>
<meta charset="utf-8" />
<title>Moduł 2 - Model blokowy - Typy elementów</title>
<!-- 1. Stała szerokość -->
<!-- <style>
.box {
width: 240px;
height: 100px;
background-color: orange;
}
.box-2 {
background-color: olive;
}
</style> -->
<!-- 2. Wyrównanie elementów za pomocą margin -->
<!-- <style>
.box {
width: 240px;
height: 100px;
background-color: orange;
margin-left: auto;
margin-right: auto;
margin: 0 auto;
}
</style> -->
<!-- 3. Container, Wrapper -->
<!-- <style>
.container {
width: 1200px;
margin: 0 auto;
padding: 0 20px;
}
.wrapper {
width: 980px;
margin: 0 auto;
padding: 0 20px;
}
</style> -->
<!-- 4. Inline -->
<!-- <style>
.link {
background-color: pink;
padding-left: 20px;
padding-right: 20px;
margin-right: 50px;
margin-left: 50px;
width: 1000px;
height: 1000px;
margin-top: 100px;
margin-bottom: 100px;
}
</style> -->
<!-- 5. Inline block -->
<!-- <style>
.link {
display: inline-block;
background-color: pink;
padding-left: 20px;
padding-right: 20px;
margin-right: 50px;
margin-left: 50px;
width: 100px;
height: 100px;
margin-top: 100px;
margin-bottom: 100px;
}
</style> -->
<!-- 6. Display: inline, block, inline-block, none -->
<!-- <style>
.hidden {
display: none;
}
</style> -->
</head>
<body>
<!-- 1. Stała szerokość -->
<!-- <div class="box"></div>
<div class="box box-2"></div> -->
<!-- 3. Container, Wrapper -->
<!-- <div class="container">
<h1>Moja strona</h1>
</div>
<div class="wrapper"></div> -->
<!-- 4. Inline -->
<!-- <a class="link">Link</a> -->
<!-- 4.1 Inline przerwa -->
<!-- <img src="https://picsum.photos/640/480" alt="Losowy obraz z generatora obrazów" width="320" height="240" />
<img src="https://picsum.photos/640/480" alt="Losowy obraz z generatora obrazów" width="320" height="240" />
<img src="https://picsum.photos/640/480" alt="Losowy obraz z generatora obrazów" width="320" height="240" />
<img src="https://picsum.photos/640/480" alt="Losowy obraz z generatora obrazów" width="320" height="240" /> -->
<!-- 5. Inline block -->
<!-- <a class="inline-block">https://onet.pl</div> -->
</body>
</html> |
import 'package:flutter/widgets.dart';
import 'package:hooks_riverpod/hooks_riverpod.dart';
import 'package:supabase_flutter/supabase_flutter.dart';
import 'package:flagger_app/domain/entity/member.dart';
import 'package:flagger_app/domain/repository/member_repository.dart';
import 'package:flagger_app/foundation/supabase_client_provider.dart';
class AuthUseCase {
static Future<void> signUpWithEmailAndPassword({
required WidgetRef ref,
required String email,
required String password,
}) async {
final instance = ref.read(supabaseAuthProvider);
try {
// emailによるサインアップ
await instance.signUp(
email: email,
password: password,
);
final repo = ref.watch(memberRepositoryProvider);
// DBへMemberを登録
// プロフィール作成は別途処理を行う
await repo.createMember(
MemberRequest(
email: email,
updatedAt: DateTime.now(),
createdAt: DateTime.now(),
),
);
} on AuthException catch (e) {
debugPrint(e.message);
return;
}
}
} |
<template>
<el-form :model="form" ref="form" :rules="rules" class="form">
<el-form-item class="form-item" prop="username">
<el-input placeholder="用户名/手机" v-model="form.username"></el-input>
</el-form-item>
<el-form-item class="form-item" prop="password">
<el-input placeholder="密码" type="password" v-model="form.password"></el-input>
</el-form-item>
<p class="form-text">
<nuxt-link to="#">忘记密码</nuxt-link>
</p>
<el-button class="submit" type="primary" @click="handleLoginSubmit">登录</el-button>
</el-form>
</template>
<script>
import { async } from "q";
export default {
data() {
return {
// 表单数据
form: {
username: "",
password: ""
},
// 表单规则
rules: {
username: [
{
required: true,
message: "请输入用户名/手机号",
trigger: "blur"
}
],
password: [
{
required: true,
message: "请输入账户密码",
trigger: "blur"
}
]
}
};
},
methods: {
// 提交登录
handleLoginSubmit() {
this.$refs.form.validate(valid => {
// vaild为true则验证通过
if (valid) {
// 登录接口请求
// this.$axios({
// url: "/accounts/login",
// method: "POST",
// data: this.form
// }).then(res=>{
// if(res.status === 200) {
// this.$message.success('登录成功')
// }
// })
this.$store.dispatch("user/login", this.form).then(res => {
// 成功提示
this.$message({
message: "登录成功,正在跳转",
type: "success"
});
// 跳转到首页
setTimeout(() => {
// 返回上一页
this.$router.back();
}, 1000);
});
}
});
}
}
};
</script>
<style scoped lang="less">
.form {
padding: 25px;
}
.form-item {
margin-bottom: 20px;
}
.form-text {
font-size: 12px;
color: #409eff;
text-align: right;
line-height: 1;
}
.submit {
width: 100%;
margin-top: 10px;
}
</style> |
import java.util.ArrayList;
public class CoffeeKiosk {
private ArrayList<Item> menu;
private ArrayList<Order> orders;
//Constructor
public CoffeeKiosk(){
this.menu = new ArrayList<Item>();
this.orders = new ArrayList<Order>();
}
//Methods
public void addMenuItem(String name,Double price){
int index = this.getMenuItems().size();
this.menu.add(new Item(name,price,index));
}
public void displayMenu(){
for(Item item : this.menu){
int index = item.getItemIndex();
String name = item.getItemName();
double price = item.getItemPrice();
System.out.printf("%x %s -- $%.2f\n",index,name,price);
}
}
public ArrayList<Item> getMenuItems(){
return this.menu;
}
public ArrayList<Order> getOrders(){
return this.orders;
}
public void newOrder() {
// Shows the user a message prompt and then sets their input to a variable, name
System.out.println("Please enter customer name for new order:");
String name = System.console().readLine();
// Your code:
// Create a new order with the given input string
Order order = new Order(name);
// Show the user the menu, so they can choose items to add.
displayMenu();
// Prompts the user to enter an item number
System.out.println("Please enter a menu item index or q to quit:");
String itemNumber = System.console().readLine();
// Write a while loop to collect all user's order items
while (!itemNumber.equals("q")) {
int id = Integer.parseInt(itemNumber);
for(Item item : menu){
int index = item.getItemIndex();
if(id == index){
order.addItem(item);
System.out.println("Please enter a menu item index or q to quit:");
itemNumber = System.console().readLine();
}
}
// Get the item object from the menu, and add the item to the order
// Ask them to enter a new item index or q again, and take their input
}
// After you have collected their order, print the order details
// as the example below. You may use the order's display method.
order.display();
}
public void addMenuItemByInput() {
System.out.println("Please enter name of item you wish to add to the menu:");
String name = System.console().readLine();
System.out.printf("How much does a %s cost?\n",name);
String priceTag = System.console().readLine();
double price = Double.parseDouble(priceTag);
System.out.printf("Item Name: %s || Price: $%.2f\n",name,price);
System.out.println("Confirm Menu Entry (y for YES; else any key for NO):");
String confirmation = System.console().readLine();
if(confirmation.equals("y")){
addMenuItem(name,price);
}
System.out.println("__Current Menu__");
displayMenu();
}
} |
import { Suspense, useState } from "react";
import SearchResult from "./SearchResult";
const Lesson8_1 = () => {
const [query, setQuery] = useState("");
return (
<div>
<label>
アルバム検索
<input
type="text"
value={query}
onChange={(e) => setQuery(e.target.value)}
className={`border-2 px-3 py-3 rounded-md`}
/>
<Suspense fallback={<h2>Loading...</h2>}>
<SearchResult query={query} />
</Suspense>
</label>
</div>
);
};
export default Lesson8_1; |
// SPDX-License-Identifier: MIT
// Matic Contract
pragma solidity ^0.7.0;
import "./libraries/LibDiamond.sol";
abstract contract EIP712Base {
struct EIP712Domain {
string name;
string version;
uint256 chainId;
address verifyingContract;
}
bytes32 public constant EIP712_DOMAIN_TYPEHASH =
keccak256(
bytes(
"EIP712Domain(string name,string version,uint256 chainId,address verifyingContract)"
)
);
function getChainId() public pure returns (uint256) {
uint256 id;
assembly {
id := chainid()
}
return id;
}
function getDomainSeperator() public view returns (bytes32) {
LibDiamond.DiamondStorage storage ds = LibDiamond.diamondStorage();
return encodeDomainSeperator(ds.CONTRACT_ERC712_NAME, ds.CONTRACT_ERC712_VERSION);
}
function getWorkerDomainSeperator() public view returns (bytes32) {
LibDiamond.DiamondStorage storage ds = LibDiamond.diamondStorage();
return encodeWorkerDomainSeperator(ds.CONTRACT_ERC712_NAME, ds.CONTRACT_ERC712_VERSION);
}
function encodeDomainSeperator(string memory name, string memory version)
public
view
returns (bytes32)
{
uint256 chainId = getChainId();
require(chainId != 0, "chain ID must not be zero");
return
keccak256(
abi.encode(
EIP712_DOMAIN_TYPEHASH,
keccak256(bytes(name)),
keccak256(bytes(version)),
chainId,
address(this)
)
);
}
// This encodes the domain separator to the root chain, rather than the main chain.
function encodeWorkerDomainSeperator(
string memory name,
string memory version
) public view returns (bytes32) {
uint256 chainId = getChainId();
// 1 == truffle test; 1 == Ethereum
// 137 == matic mainnet; 1 == Ethereum
// 80001 == matic mumbai; 5 == Goerli
chainId = chainId == 137 || chainId == 1 ? 1 : chainId == 80001
? 5
: 31337;
require(chainId != 0, "chain ID must not be zero");
return
keccak256(
abi.encode(
EIP712_DOMAIN_TYPEHASH,
keccak256(bytes(name)),
keccak256(bytes(version)),
chainId,
address(this)
)
);
}
/**
* Accept message hash and returns hash message in EIP712 compatible form
* So that it can be used to recover signer from signature signed using EIP712 formatted data
* https://eips.ethereum.org/EIPS/eip-712
* "\\x19" makes the encoding deterministic
* "\\x01" is the version byte to make it compatible to EIP-191
*/
function toTypedMessageHash(bytes32 messageHash)
internal
view
returns (bytes32)
{
return
keccak256(
abi.encodePacked("\x19\x01", getDomainSeperator(), messageHash)
);
}
} |
import React, { useEffect, useRef, useState } from 'react';
import {Link, useNavigate } from 'react-router-dom';
import {TfiWrite} from 'react-icons/tfi'
import {VscGraph} from 'react-icons/vsc'
import {BsFillFileEarmarkPersonFill, BsMenuDown} from 'react-icons/bs'
import {BiHomeCircle} from 'react-icons/bi'
function NavBar (props){
const user = useRef()
const [autenticado, setautenticado] = useState();
const [bienvenida, setbienvenida] = useState();
const navigate = useNavigate()
useEffect(() => {
if(sessionStorage.getItem('nombre')){
setautenticado(true)
user.current='/home'
}
else{
setautenticado(false)
user.current='/login'
}
});
useEffect(() => {
saludo()
}, [autenticado]);
const logout=()=>{
sessionStorage.removeItem('nombre')
user.current=('/login')
navigate('/login')
}
const saludo=()=>{
if(autenticado){
setbienvenida(
<div className='text-white font-bold'>
Bienvenido {sessionStorage.getItem('nombre')}
<button onClick={logout} className='bg-red-700 w-5 mx-3 rounded-lg'>X</button>
</div>
)
}else{
setbienvenida(<div className='text-white font-bold flex justify-center w-auto'>Identificate</div>)
}
}
return (
<div className='grid grid-cols-3 w-auto justify-center -z-10'>
<h1 className='items-start text-white font-bold px-2 text-4xl justify-start hidden md:block'>Peña El Botijo</h1>
<img src='../src/imagenes/botijo.png' className='my-2 mx-2 w-12 h-12 md:hidden'/>
<div className=' flex justify-center text-xs items-center'>{bienvenida}</div>
<div className='hidden font-bold text-white px-2 md:block'>
<ul className='flex my-2 items-end justify-end '>
<li>
<Link to={user.current}>
<BiHomeCircle className='w-12 h-12 ml-5 hover:text-green-500'/>
</Link>
</li>
<li>
<Link to='/jugadores'>
<BsFillFileEarmarkPersonFill className='w-12 h-12 ml-5 hover:text-green-500'/>
</Link>
</li>
<li>
<Link to='/crearJugador'>
<TfiWrite className='w-12 h-12 ml-5 hover:text-green-500'/>
</Link>
</li>
<li>
<Link to='/resultados'>
<VscGraph className='w-12 h-12 ml-5 hover:text-green-500'/>
</Link>
</li>
</ul>
</div>
<div className='flex justify-end text-white cursor-pointer md:hidden'>
<BsMenuDown abrirCerrar={true} onClick={props.abrirCerrar} className='w-10 h-10 mr-5 mt-2'/>
</div>
</div>
);
}
export default NavBar; |
import AppLayout from 'layout/app-layout';
import React, { useState } from 'react';
import {
FormControl,
FormLabel,
Input,
Button,
Text,
Box,
Spinner,
FormErrorMessage,
Switch,
NumberInputStepper,
NumberDecrementStepper,
NumberInputField,
NumberIncrementStepper,
NumberInput,
} from '@chakra-ui/react';
import * as yup from 'yup';
import DatePicker from 'react-datepicker';
import { useFormik, FormikHelpers } from 'formik';
import { getUserStartupById, updateUserStartupById } from 'apiSdk/user-startups';
import { Error } from 'components/error';
import { userStartupValidationSchema } from 'validationSchema/user-startups';
import { UserStartupInterface } from 'interfaces/user-startup';
import useSWR from 'swr';
import { useRouter } from 'next/router';
import { AsyncSelect } from 'components/async-select';
import { ArrayFormField } from 'components/array-form-field';
import { AccessOperationEnum, AccessServiceEnum, withAuthorization } from '@roq/nextjs';
import { UserInterface } from 'interfaces/user';
import { StartupInterface } from 'interfaces/startup';
import { getUsers } from 'apiSdk/users';
import { getStartups } from 'apiSdk/startups';
function UserStartupEditPage() {
const router = useRouter();
const id = router.query.id as string;
const { data, error, isLoading, mutate } = useSWR<UserStartupInterface>(
() => (id ? `/user-startups/${id}` : null),
() => getUserStartupById(id),
);
const [formError, setFormError] = useState(null);
const handleSubmit = async (values: UserStartupInterface, { resetForm }: FormikHelpers<any>) => {
setFormError(null);
try {
const updated = await updateUserStartupById(id, values);
mutate(updated);
resetForm();
} catch (error) {
setFormError(error);
}
};
const formik = useFormik<UserStartupInterface>({
initialValues: data,
validationSchema: userStartupValidationSchema,
onSubmit: handleSubmit,
enableReinitialize: true,
});
return (
<AppLayout>
<Text as="h1" fontSize="2xl" fontWeight="bold">
Edit User Startup
</Text>
<Box bg="white" p={4} rounded="md" shadow="md">
{error && <Error error={error} />}
{formError && <Error error={formError} />}
{isLoading || (!formik.values && !error) ? (
<Spinner />
) : (
<form onSubmit={formik.handleSubmit}>
<AsyncSelect<UserInterface>
formik={formik}
name={'user_id'}
label={'user_id'}
placeholder={'Select User'}
fetcher={getUsers}
renderOption={(record) => (
<option key={record.id} value={record.id}>
{record?.id}
</option>
)}
/>
<AsyncSelect<StartupInterface>
formik={formik}
name={'startup_id'}
label={'startup_id'}
placeholder={'Select Startup'}
fetcher={getStartups}
renderOption={(record) => (
<option key={record.id} value={record.id}>
{record?.id}
</option>
)}
/>
<Button isDisabled={!formik.isValid || formik?.isSubmitting} colorScheme="blue" type="submit" mr="4">
Submit
</Button>
</form>
)}
</Box>
</AppLayout>
);
}
export default withAuthorization({
service: AccessServiceEnum.PROJECT,
entity: 'user_startup',
operation: AccessOperationEnum.UPDATE,
})(UserStartupEditPage); |
#!/usr/bin/env lua
-- free
local args, opts, usage = require("getopt").process {
{ "Display this help message", false, "help" },
{ "Output in kibibytes", false, "k", "kibi", "kilo" },
{ "Output in mebibytes", false, "m", "mebi", "mega" },
{ "Use powers of 1000, not 1024", false, "si" },
{ "Human-readable output (like -k)", false, "h", "human" },
{ "Repeat every N seconds", "N", "s", "seconds" },
{ "Repeat N times, then exit", "N", "c", "count"},
exit_on_bad_opt = true,
help_message = "pass '--help' for help\n",
args = arg
}
if #args > 0 or opts.h then
io.stderr:write(([[
usage: free [options]
options:
%s
Copyright (c) 2023 ULOS Developers under the GNU GPLv3
]]):format(usage))
os.exit(1)
end
local total, free, used = 0, 0, 0
local hand, err = io.open("/proc/meminfo", "r")
if not hand then
io.stderr:write("could not open /proc/meminfo: ", err, "\n")
os.exit(1)
-- good god LSP, is `os.exit()` not good enough?
error()
end
for line in hand:lines() do
if line:match("MemTotal") then
total = tonumber((line:match("(%d+) kB"))) * 1024
elseif line:match("MemAvailable") then
free = tonumber((line:match("(%d+) kB"))) * 1024
elseif line:match("MemUsed") then
used = tonumber((line:match("(%d+) kB"))) * 1024
end
end
hand:close()
-- some systems (i.e. Linux) don't provide this
if used == 0 then used = total - free end
local power = opts.si and 1000 or 1024
local suffix = opts.si and "" or "b"
if opts.h or opts.k or not next(opts) then
total = string.format("%.1f", total / power) .. " K" .. suffix
used = string.format("%.1f", used / power) .. " K" .. suffix
free = string.format("%.1f", free / power) .. "K" .. suffix
elseif opts.m then
total = string.format("%.1f", total / power / power) .. " M" .. suffix
used = string.format("%.1f", used / power / power) .. " M" .. suffix
free = string.format("%.1f", free / power / power) .. " M" .. suffix
end
io.write(" total / used / free\n")
io.write(("%10s / %10s / %10s\n"):format(total, used, free)) |
package com.bilik.playground.scala.mixins
object MixInIterator {
def main(args: Array[String]): Unit = {
val richStringIter = new RichStringIter
richStringIter.foreach(println)
} // END OF MAIN
}
// in this case, withotu additional functionality in abstract class, we can calmly replace this for trait and it all still works
// also change 'type T' for generics
abstract class AbsIterator {
type T
def hasNext: Boolean
def next(): T
}
// implementation
//StringIterator takes a String and can be used to iterate over the String (e.g. to see if a String contains a certain character).
class StringIterator(s: String) extends AbsIterator {
type T = Char
private var i = 0
def hasNext = i < s.length
def next() = {
val ch = s.charAt(i)
i += 1
ch
}
}
// Now let’s create a trait which also extends AbsIterator.
trait RichIterator extends AbsIterator {
def foreach(f: T => Unit): Unit = while (hasNext) f(next())
}
// We would like to combine the functionality of StringIterator and RichIterator into a single class.
class RichStringIter extends StringIterator("Scala") with RichIterator
// so what is the difference ? -> https://stackoverflow.com/questions/36732357/what-is-the-difference-between-mixins-and-inheritance |
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Mail\Mailables\Content;
use Illuminate\Mail\Mailables\Envelope;
use Illuminate\Queue\SerializesModels;
class RegOnlineCourseFormMail extends Mailable
{
use Queueable, SerializesModels;
public $regOnlineCourseForm;
public function __construct($arr)
{
$this->regOnlineCourseForm = $arr;
}
/**
* Get the message envelope.
*
* @return \Illuminate\Mail\Mailables\Envelope
*/
public function envelope()
{
return new Envelope(
from: "[email protected]",
subject: 'Регистрация на онлайн-курс',
);
}
/**
* Get the message content definition.
*
* @return \Illuminate\Mail\Mailables\Content
*/
public function content()
{
return new Content(
view: 'mail.regOnlineCourseForm',
with: [
'name' => $this->regOnlineCourseForm['name'],
'email' => $this->regOnlineCourseForm['email'],
'phone' => $this->regOnlineCourseForm['phone'],
],
);
}
/**
* Get the attachments for the message.
*
* @return array
*/
public function attachments()
{
return [];
}
} |
package com.github.sbroekhuis.interactiveschemainferrer.strategy
import com.fasterxml.jackson.databind.JsonNode
import com.saasquatch.jsonschemainferrer.GenericSchemaFeatureInput
import com.saasquatch.jsonschemainferrer.MultipleOfPolicies
import com.saasquatch.jsonschemainferrer.MultipleOfPolicy
import javafx.scene.control.ButtonBar
import javafx.scene.layout.Priority
import javafx.scene.text.Font
import tornadofx.*
/**
* # Strategy: MultipleOfStrategy
* This strategy implements the [MultipleOf](https://json-schema.org/understanding-json-schema/reference/numeric.html#multiples)
* keyword.
*
* We use the [MultipleOfPolicies.gcd] to get the greatest common divider, and if it is not 1, we ask the user to confirm.
*
*/
class MultipleOfStrategy : AbstractStrategy(), MultipleOfPolicy {
override fun getMultipleOf(input: GenericSchemaFeatureInput): JsonNode? {
if (input.samples.distinct().size <= 1) {
logger.info("Distinct Samples Size is 1, therefor we should not ask for a multipleOf")
return null
}
val result: JsonNode = MultipleOfPolicies.gcd().getMultipleOf(input) ?: return null
val multipleOf: Number = result.numberValue()
if (multipleOf == 1) {
logger.info("The GCD is 1, therefor we should not ask anything, ignore it")
return null
}
val response = askUserWith(Form(input.path, multipleOf))
return if (response) {
logger.info("Potential multipleOf accepted")
result
} else {
logger.info("Potential multipleOf declined")
null
}
}
private class Form(val path: String, val multipleOf: Number) :
StrategyFragment<Boolean>("Inferring - Possible MultipleOf Found") {
override val root =
strategyroot("https://json-schema.org/understanding-json-schema/reference/numeric.html#multiples") {
// Add the multiline description label
label {
graphic = textflow {
text("The field with path: ")
text(path) { font = Font.font("Monospace") }
text(" seems to be a number with a greatest common divider of ")
text(multipleOf.toString()) { font = Font.font("Monospace") }
text(".\nShould we add this constraint?")
}
isWrapText = true
}
separator()
// Button Bar
region { vgrow = Priority.ALWAYS }
buttonbar {
button("Yes", ButtonBar.ButtonData.YES) {
enableWhen(validator.valid)
action {
done(true)
}
}
button("No", ButtonBar.ButtonData.NO) {
action {
done(false)
}
}
}
}
}
} |
# Instagram-Clone-Using-Next.JS
This repository contains the code for an Instagram clone, a frontend web application built using Next.js, Tailwind CSS, Redux Toolkit, and React Query. It aims to replicate the core features and design of the popular social media platform, Instagram.
# Features
User authentication: Sign up, log in, and log out functionalities.
User profile: Display user information, profile picture, and the ability to update the profile picture.
Home feed: Display posts from the users that the logged-in user follows.
Explore page: Discover new users and posts.
Create post: Upload images with captions and post them.
Like and comment: Interact with posts by liking and commenting on them.
Responsive design: Mobile-friendly layout for a seamless experience on different devices.
# Technologies Used
Next.js: A React framework for building server-side rendered and static websites.
Tailwind CSS: A utility-first CSS framework for building custom user interfaces.
Redux Toolkit: A simplified state management library for React applications.
React Query: A library for managing server state and caching data in React applications.
Firebase: A platform for building web and mobile applications.
Cloud Firestore: A NoSQL cloud database for storing and syncing data.
Cloud Storage: A service for storing user-uploaded images.
# Getting Started
To get a local copy of the project up and running, follow these steps:
1)Clone the repository:
git clone https://github.com/MANOJPATIL143/Instagram-Clone-Using-Next.JS
2)Install the dependencies:
cd instagram-clone
npm install
3)Set up environment variables:
Create a .env file in the root directory.
Add the following variables to the .env file:
NEXT_PUBLIC_API_URL=http://localhost:8080/api/
4)Start the development server:
npm run dev
The web application should now be running at http://localhost:3000. |
import { useEffect } from "react";
import { Button, ButtonGroup, FormControl, Spinner } from "react-bootstrap";
import { FaSyncAlt } from "react-icons/fa";
import { ImUserPlus } from "react-icons/im";
import { IoCalendarNumber } from "react-icons/io5";
import { useQuery } from "react-query";
import { Link } from "react-router-dom";
import {
People,
setFind,
setPeople,
setPeopleOrigen,
} from "../features/people/peopleSlice";
import { useAppDispatch, useAppSelector } from "../hooks";
import { notify } from "../libs/toast";
import * as peopleService from "../services/people.service";
import { THandleChangeI } from "../types/THandleChanges";
import TablePeople from "./TablePeople";
export default function Searcher(): React.JSX.Element {
const dispatch = useAppDispatch();
const peopleOrigenState: People[] = useAppSelector(
({ people }) => people.peopleOrigen
);
const findState: string = useAppSelector(({ people }) => people.find);
const { data, error, isLoading, refetch } = useQuery<[], any>(
"people",
peopleService.getPeople
);
const filterPeople = (): void => {
dispatch(
setPeople(
peopleOrigenState.filter(
({ name, document_number }) =>
name.toLowerCase().startsWith(findState) ||
document_number.startsWith(findState)
)
)
);
};
useEffect(() => {
if (data) {
dispatch(setPeopleOrigen(data));
if (findState === "") dispatch(setPeople(data));
else filterPeople();
}
if (error) notify(error.response.data.error, { type: "error" });
}, [data, error]);
const handleChange = (e: THandleChangeI) =>
dispatch(setFind(e.target.value.toLocaleLowerCase()));
const handleClick = () => {
dispatch(setFind(""));
refetch();
};
useEffect(() => {
filterPeople();
}, [findState]);
return (
<>
<ButtonGroup className="position-fixed bottom-px">
<FormControl
name="find"
className="bg-white"
onChange={handleChange}
value={findState}
type="search"
size="sm"
placeholder="Buscar"
spellCheck={false}
autoComplete="off"
autoFocus
/>
<Button
variant="dark"
onClick={handleClick}
title="Refrescar datos"
>
{isLoading ? (
<Spinner size="sm" />
) : (
<FaSyncAlt className="mb-1" />
)}
</Button>
<Link
to="/people/add"
className="btn btn-dark"
title="Agendamiento por día"
>
<ImUserPlus className="mb-1" />
</Link>
<Link
to="/people/schedule"
className="btn btn-dark"
title="Agendamiento programado"
>
<IoCalendarNumber className="mb-1" />
</Link>
</ButtonGroup>
<TablePeople />
</>
);
} |
package il.example.weatherapp.utils
import android.Manifest
import android.app.NotificationChannel
import android.app.NotificationManager
import android.app.PendingIntent
import android.content.Context
import android.content.Intent
import android.content.pm.PackageManager
import android.os.Build
import androidx.appcompat.app.AppCompatActivity
import androidx.core.app.ActivityCompat
import androidx.core.app.NotificationCompat
import androidx.core.app.NotificationManagerCompat
import il.example.weatherapp.R
import il.example.weatherapp.ui.MainActivity
import il.example.weatherapp.utils.receivers.AlarmManagerReceiver
class AppUtils(private val activity: AppCompatActivity) {
companion object {
const val NOTIFICATION_ID = 123
const val NOTIFICATION_CHANNEL_ID = "10"
const val NOTIFICATION_CHANNEL_NAME = "NotificationChannel1"
fun notify(
context: Context,
title: String,
msg: String,
icon: Int? = null
) {
// Create an intent to launch your app's main activity
val intent = Intent(context, MainActivity::class.java).apply {
flags = Intent.FLAG_ACTIVITY_NEW_TASK or Intent.FLAG_ACTIVITY_CLEAR_TASK
}
// Create a PendingIntent to start your app's main activity
val pendingIntent = PendingIntent.getActivity(
context,
2,
intent,
PendingIntent.FLAG_UPDATE_CURRENT or PendingIntent.FLAG_IMMUTABLE
)
val build = NotificationCompat.Builder(context, NOTIFICATION_CHANNEL_ID)
.setContentTitle(title)
.setContentText(msg)
.setPriority(NotificationCompat.PRIORITY_HIGH)
.setContentIntent(pendingIntent) // Set the PendingIntent
.setAutoCancel(true)
if (icon != null) {
build.setSmallIcon(icon)
} else {
build.setSmallIcon(R.drawable.baseline_home_24)
}
if (ActivityCompat.checkSelfPermission(
context,
Manifest.permission.POST_NOTIFICATIONS
) == PackageManager.PERMISSION_GRANTED
)
{
NotificationManagerCompat.from(context).notify(NOTIFICATION_ID,build.build())
}
}
fun createNotificationChannel(context: Context) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
val channel = NotificationChannel(
NOTIFICATION_CHANNEL_ID,
NOTIFICATION_CHANNEL_NAME,
NotificationManager.IMPORTANCE_HIGH
)
NotificationManagerCompat.from(context).createNotificationChannel(channel)
}
}
//The notification will disappear as soon as we are lunching the app if any showed before.
fun cancelNotification(context: Context) {
NotificationManagerCompat.from(context).cancel(NOTIFICATION_ID)
}
}
} |
package org.jaqpot.core.service.resource;
//import io.swagger.annotations.*;
import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.Parameter;
import io.swagger.v3.oas.annotations.Parameters;
import io.swagger.v3.oas.annotations.enums.ParameterIn;
import io.swagger.v3.oas.annotations.enums.ParameterStyle;
import io.swagger.v3.oas.annotations.enums.SecuritySchemeIn;
import io.swagger.v3.oas.annotations.enums.SecuritySchemeType;
import io.swagger.v3.oas.annotations.extensions.Extension;
import io.swagger.v3.oas.annotations.extensions.ExtensionProperty;
import io.swagger.v3.oas.annotations.responses.ApiResponse;
import io.swagger.v3.oas.annotations.media.Schema;
import io.swagger.v3.oas.annotations.media.ArraySchema;
import io.swagger.v3.oas.annotations.media.Content;
import io.swagger.v3.oas.annotations.parameters.RequestBody;
import io.swagger.v3.oas.annotations.security.SecurityScheme;
import io.swagger.v3.oas.annotations.tags.Tag;
import org.apache.commons.validator.routines.UrlValidator;
import org.jaqpot.core.annotations.Jackson;
import org.jaqpot.core.data.AlgorithmHandler;
import org.jaqpot.core.data.ModelHandler;
import org.jaqpot.core.data.UserHandler;
import org.jaqpot.core.data.serialize.JSONSerializer;
import org.jaqpot.core.model.Algorithm;
import org.jaqpot.core.model.Task;
import org.jaqpot.core.model.facades.UserFacade;
import org.jaqpot.core.service.annotations.Authorize;
import org.jaqpot.core.service.authentication.AAService;
import org.jaqpot.core.service.data.TrainingService;
import org.jaqpot.core.service.exceptions.JaqpotDocumentSizeExceededException;
import org.jaqpot.core.service.exceptions.JaqpotDocumentSizeExceededException;
import org.jaqpot.core.service.exceptions.QuotaExceededException;
import org.jaqpot.core.service.exceptions.parameter.*;
import org.jaqpot.core.service.validator.ParameterValidator;
import org.jboss.resteasy.plugins.providers.multipart.InputPart;
import org.jboss.resteasy.plugins.providers.multipart.MultipartFormDataInput;
import javax.ejb.EJB;
import javax.inject.Inject;
import javax.ws.rs.*;
import javax.ws.rs.core.*;
import java.io.*;
import java.security.GeneralSecurityException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
import java.util.Base64;
import java.util.LinkedHashMap;
import java.util.logging.Level;
import org.jaqpot.core.data.DatasetHandler;
import org.jaqpot.core.model.ErrorReport;
import org.jaqpot.core.model.Model;
import org.jaqpot.core.model.dto.dataset.Dataset;
import org.jaqpot.core.service.annotations.TokenSecured;
import org.jaqpot.core.service.authentication.RoleEnum;
import org.jaqpot.core.service.data.PredictionService;
import xyz.euclia.euclia.accounts.client.models.User;
/**
* Created by Angelos Valsamis on 23/10/2017.
*/
@Path("/biokinetics")
//@Api(value = "/biokinetics", description = "Biokinetics API")
@Produces({"application/json", "text/uri-list"})
@Authorize
@Tag(name = "biokinetics")
@SecurityScheme(name = "bearerAuth",
type = SecuritySchemeType.HTTP,
in = SecuritySchemeIn.HEADER,
scheme = "bearer",
description = "add the token retreived from oidc. Example: Bearer <API_KEY>"
)
@io.swagger.v3.oas.annotations.security.SecurityRequirement(name = "bearerAuth")
public class BiokineticsResource {
@EJB
AAService aaService;
@Context
SecurityContext securityContext;
@EJB
AlgorithmHandler algorithmHandler;
@EJB
UserHandler userHandler;
@EJB
ModelHandler modelHandler;
@Inject
ParameterValidator parameterValidator;
@EJB
DatasetHandler datasetHandler;
@EJB
PredictionService predictionService;
@EJB
TrainingService trainingService;
@Context
UriInfo uriInfo;
@Inject
@Jackson
JSONSerializer serializer;
private static final Logger LOG = Logger.getLogger(BiokineticsResource.class.getName());
@POST
@TokenSecured({RoleEnum.DEFAULT_USER})
@Produces({MediaType.APPLICATION_JSON, "text/uri-list"})
@Consumes(MediaType.MULTIPART_FORM_DATA)
@Path("/pksim/createmodel")
@Operation(summary = "Creates Biokinetics model with PkSim",
description = "Creates a biokinetics model given a pksim .xml file and demographic data",
responses = {
@ApiResponse(responseCode = "200", content = @Content(schema = @Schema(implementation = Task.class)))
})
@org.jaqpot.core.service.annotations.Task
public Response trainBiokineticsModel(
@Parameter(name = "Authorization", description = "Authorization token", schema = @Schema(type = "string")) @HeaderParam("Authorization") String api_key,
@Parameter(name = "file", description = "xml[m,x] file", required = true, schema = @Schema(type = "string", format = "binary")) @FormParam("file") String file,
@Parameter(name = "dataset-uri", description = "Dataset uri to be trained upon", required = true, schema = @Schema(type = "string")) @FormParam("dataset-uri") String datasetUri,
@Parameter(name = "title", description = "Title of model", required = true, schema = @Schema(type = "string")) @FormParam("title") String title,
@Parameter(name = "description", description = "Description of model", required = true, schema = @Schema(type = "string")) @FormParam("description") String description,
@Parameter(name = "algorithm-uri", description = "Algorithm URI", required = true, schema = @Schema(type = "string")) @FormParam("algorithm-uri") String algorithmURI,
@Parameter(name = "parameters", description = "Parameters for algorithm", required = false, schema = @Schema(type = "string")) @FormParam("parameters") String parameters,
@Parameter(description = "multipartFormData input", hidden = true) MultipartFormDataInput input)
throws ParameterIsNullException, ParameterInvalidURIException, QuotaExceededException, IOException, ParameterScopeException, ParameterRangeException, ParameterTypeException, JaqpotDocumentSizeExceededException {
String[] apiA = api_key.split("\\s+");
String apiKey = apiA[1];
UrlValidator urlValidator = new UrlValidator(UrlValidator.ALLOW_LOCAL_URLS);
byte[] bytes;
Map<String, List<InputPart>> uploadForm = input.getFormDataMap();
List<InputPart> inputParts = uploadForm.get("file");
title = uploadForm.get("title").get(0).getBody(String.class, null);
//String title = uploadForm.get("title").get(0).getBody(String.class, null);
description = uploadForm.get("description").get(0).getBody(String.class, null);
//String description = uploadForm.get("description").get(0).getBody(String.class, null);
algorithmURI = uploadForm.get("algorithm-uri").get(0).getBody(String.class, null);
//String algorithmURI = uploadForm.get("algorithm-uri").get(0).getBody(String.class, null);
datasetUri = uploadForm.get("dataset-uri").get(0).getBody(String.class, null);
//String datasetUri = uploadForm.get("dataset-uri").get(0).getBody(String.class, null);
User user = userHandler.find(securityContext.getUserPrincipal().getName(), apiKey);
long modelCount = modelHandler.countAllOfCreator(user.get_id());
parameters = null;
//String parameters = null;
if (uploadForm.get("parameters") != null) {
parameters = uploadForm.get("parameters").get(0).getBody(String.class, null);
}
if (algorithmURI == null) {
throw new ParameterIsNullException("algorithmURI");
}
if (!urlValidator.isValid(algorithmURI)) {
throw new ParameterInvalidURIException("Not valid Algorithm URI.");
}
String algorithmId = algorithmURI.split("algorithm/")[1];
Algorithm algorithm = algorithmHandler.find(algorithmId);
if (algorithm == null) {
throw new NotFoundException("Could not find Algorithm with id:" + algorithmId);
}
parameterValidator.validate(parameters, algorithm.getParameters());
String encodedString = "";
for (InputPart inputPart : inputParts) {
try {
//Convert the uploaded file to inputstream
InputStream inputStream = inputPart.getBody(InputStream.class, null);
bytes = getBytesFromInputStream(inputStream);
//Base64 encode
byte[] encoded = java.util.Base64.getEncoder().encode(bytes);
encodedString = new String(encoded);
System.out.println(encodedString);
} catch (IOException e) {
e.printStackTrace();
}
}
//Append XML file (Base64 string) in parameters
parameters = parameters.substring(0, parameters.length() - 1);
parameters += ",\"xml_file\":[\"" + encodedString + "\"]}";
Map<String, Object> options = new HashMap<>();
options.put("title", title);
options.put("description", description);
options.put("api_key", apiKey);
options.put("parameters", parameters);
options.put("base_uri", uriInfo.getBaseUri().toString());
options.put("dataset_uri", datasetUri);
options.put("algorithmId", algorithmId);
options.put("creator", securityContext.getUserPrincipal().getName());
Task task = trainingService.initiateTraining(options, securityContext.getUserPrincipal().getName());
return Response.ok(task).build();
}
@POST
@TokenSecured({RoleEnum.DEFAULT_USER})
@Produces({MediaType.APPLICATION_JSON, "text/uri-list"})
@Consumes({MediaType.APPLICATION_FORM_URLENCODED})
@Path("httk/createmodel")
@Operation(summary = "Creates an httk biocinetics Model",
description = "Creates an httk biocinetics Model",
responses = {
@ApiResponse(responseCode = "200", content = @Content(schema = @Schema(implementation = Task.class)),
description = "The process has successfully been started. A task URI is returned."),
@ApiResponse(responseCode = "400", content = @Content(schema = @Schema(implementation = ErrorReport.class)), description = "Bad request. More info can be found in details of Error Report."),
@ApiResponse(responseCode = "401", content = @Content(schema = @Schema(implementation = ErrorReport.class)), description = "Wrong, missing or insufficient credentials. Error report is produced."),
@ApiResponse(responseCode = "404", content = @Content(schema = @Schema(implementation = ErrorReport.class)), description = "Algorithm was not found."),
@ApiResponse(responseCode = "500", content = @Content(schema = @Schema(implementation = ErrorReport.class)), description = "Internal server error - this request cannot be served.")
})
@org.jaqpot.core.service.annotations.Task
public Response trainHttk(
@Parameter(name = "title", required = true, schema = @Schema(implementation = String.class)) @FormParam("title") String title,
@Parameter(name = "description", required = true, schema = @Schema(implementation = String.class)) @FormParam("description") String description,
@Parameter(name = "parameters", schema = @Schema(implementation = String.class)) @FormParam("parameters") String parameters,
@Parameter(name = "Authorization", description = "Authorization token", schema = @Schema(implementation = String.class)) @HeaderParam("Authorization") String api_key) throws QuotaExceededException, ParameterIsNullException, ParameterInvalidURIException, ParameterTypeException, ParameterRangeException, ParameterScopeException, JaqpotDocumentSizeExceededException {
UrlValidator urlValidator = new UrlValidator(UrlValidator.ALLOW_LOCAL_URLS);
String[] apiA = api_key.split("\\s+");
String apiKey = apiA[1];
String algorithmId = "httk";
Algorithm algorithm = algorithmHandler.find(algorithmId);
if (algorithm == null) {
throw new NotFoundException("Could not find Algorithm with id:" + algorithmId);
}
if (title == null) {
throw new ParameterIsNullException("title");
}
if (description == null) {
throw new ParameterIsNullException("description");
}
User user = userHandler.find(securityContext.getUserPrincipal().getName(), apiKey);
Map<String, Object> options = new HashMap<>();
options.put("title", title);
options.put("description", description);
options.put("dataset_uri", null);
options.put("prediction_feature", null);
options.put("api_key", apiKey);
options.put("algorithmId", algorithmId);
options.put("parameters", parameters);
options.put("base_uri", uriInfo.getBaseUri().toString());
options.put("creator", securityContext.getUserPrincipal().getName());
Map<String, String> transformationAlgorithms = new LinkedHashMap<>();
if (!transformationAlgorithms.isEmpty()) {
String transformationAlgorithmsString = serializer.write(transformationAlgorithms);
LOG.log(Level.INFO, "Transformations:{0}", transformationAlgorithmsString);
options.put("transformations", transformationAlgorithmsString);
}
parameterValidator.validate(parameters, algorithm.getParameters());
Task task = trainingService.initiateTraining(options, securityContext.getUserPrincipal().getName());
return Response.ok(task).build();
}
@POST
@TokenSecured({RoleEnum.DEFAULT_USER})
@Consumes({MediaType.APPLICATION_FORM_URLENCODED})
@Produces({MediaType.APPLICATION_JSON})
@Path("httk/model/{id}")
@Operation(summary = "Creates prediction with httk model",
description = "Creates prediction with Httk model",
responses = {
@ApiResponse(content = @Content(schema = @Schema(implementation = Task.class))),},
extensions = {
@Extension(properties = {
@ExtensionProperty(name = "orn-@type", value = "x-orn:JaqpotPredictionTaskId"),}
),
@Extension(name = "orn:expects", properties = {
@ExtensionProperty(name = "x-orn-@id", value = "x-orn:AcessToken"),
@ExtensionProperty(name = "x-orn-@id", value = "x-orn:JaqpotModelId")
}),
@Extension(name = "orn:returns", properties = {
@ExtensionProperty(name = "x-orn-@id", value = "x-orn:JaqpotHttkPredictionTaskId")
})
})
@org.jaqpot.core.service.annotations.Task
public Response makeHttkPrediction(
@FormParam("visible") Boolean visible,
@PathParam("id") String id,
@Parameter(description = "Authorization token") @HeaderParam("Authorization") String api_key) throws GeneralSecurityException, QuotaExceededException, ParameterIsNullException, ParameterInvalidURIException, JaqpotDocumentSizeExceededException {
if (id == null) {
throw new ParameterIsNullException("id");
}
String[] apiA = api_key.split("\\s+");
String apiKey = apiA[1];
UrlValidator urlValidator = new UrlValidator(UrlValidator.ALLOW_LOCAL_URLS);
User user = userHandler.find(securityContext.getUserPrincipal().getName(), apiKey);
// long datasetCount = datasetHandler.countAllOfCreator(user.getId());
// int maxAllowedDatasets = new UserFacade(user).getMaxDatasets();
//
// if (datasetCount > maxAllowedDatasets) {
// LOG.info(String.format("User %s has %d datasets while maximum is %d",
// user.getId(), datasetCount, maxAllowedDatasets));
// throw new QuotaExceededException("Dear " + user.getId()
// + ", your quota has been exceeded; you already have " + datasetCount + " datasets. "
// + "No more than " + maxAllowedDatasets + " are allowed with your subscription.");
// }
Model model = modelHandler.find(id);
if (model == null) {
throw new NotFoundException("Model not found.");
}
if (!model.getAlgorithm().getId().equals("httk")) {
throw new NotFoundException("Model is not created from httk");
}
List<String> requiredFeatures = retrieveRequiredFeatures(model);
Map<String, Object> options = new HashMap<>();
options.put("dataset_uri", null);
options.put("api_key", apiKey);
options.put("modelId", id);
options.put("creator", securityContext.getUserPrincipal().getName());
options.put("base_uri", uriInfo.getBaseUri().toString());
Task task = predictionService.initiatePrediction(options);
return Response.ok(task).build();
}
private List<String> retrieveRequiredFeatures(Model model) {
if (model.getTransformationModels() != null && !model.getTransformationModels().isEmpty()) {
String transModelId = model.getTransformationModels().get(0).split("model/")[1];
Model transformationModel = modelHandler.findModelIndependentFeatures(transModelId);
if (transformationModel != null && transformationModel.getIndependentFeatures() != null) {
return transformationModel.getIndependentFeatures();
}
}
return model.getIndependentFeatures();
}
private static byte[] getBytesFromInputStream(InputStream is) throws IOException {
ByteArrayOutputStream os = new ByteArrayOutputStream();
byte[] buffer = new byte[0xFFFF];
for (int len; (len = is.read(buffer)) != -1;) {
os.write(buffer, 0, len);
}
os.flush();
return os.toByteArray();
}
} |
#include "Shader.h"
#include "glad/glad.h"
#include <fstream>
#include <sstream>
#include <filesystem>
#include <algorithm>
#include <iostream>
void Shader::Bind()
{
glUseProgram(m_ID);
}
void Shader::Reload()
{
//Release GL handle
glDeleteProgram(m_ID);
//Reset member variables
m_ID = 0;
m_UniformCache.clear();
//Rebuild
Build();
}
unsigned int Shader::getUniformLocation(const std::string& name)
{
auto search_res = std::find_if(
m_UniformCache.begin(), m_UniformCache.end(),
[&name](const std::pair<std::string, unsigned int> element)
{
return element.first == name;
}
);
if (search_res != m_UniformCache.end())
return search_res->second;
const unsigned int location = glGetUniformLocation(m_ID, name.c_str());
m_UniformCache.push_back(std::make_pair(name, location));
return location;
}
//Program type is assumed to be gl enum: {GL_VERTEX_SHADER, GL_FRAGMENT_SHADER, GL_COMPUTE_SHADER}
void compileShaderCode(const std::string& source, unsigned int& id, int program_type)
{
const char* source_c = source.c_str();
int success = 0;
char info_log[512];
id = glCreateShader(program_type);
glShaderSource(id, 1, &source_c, NULL);
glCompileShader(id);
glGetShaderiv(id, GL_COMPILE_STATUS, &success);
if (!success)
{
glGetShaderInfoLog(id, 512, NULL, info_log);
glDeleteShader(id);
throw std::runtime_error(info_log);
}
}
std::string loadSource(std::filesystem::path filepath, bool recursive_call = false)
{
const std::string include_token{"#include"};
std::ifstream input{ filepath };
if (!input)
{
throw std::runtime_error(
"Could not open shader source file:\n" + filepath.string()
);
}
std::string full_source, current_line;
while (std::getline(input, current_line))
{
if (current_line.find(include_token) != std::string::npos)
{
auto start_id = current_line.find_first_of('\"') + 1;
auto end_id = current_line.find_last_of('\"');
auto length = end_id - start_id;
std::string filename = current_line.substr(start_id, length);
std::filesystem::path new_path = filepath.remove_filename() / filename;
full_source += loadSource(new_path, true);
}
else
{
full_source += current_line + "\n";
}
}
if (!recursive_call)
full_source += "\0";
return full_source;
}
void VertFragShader::Build()
{
//Get source
std::filesystem::path current_path{ std::filesystem::current_path() };
std::string vert_code = loadSource(current_path / m_VertPath);
std::string frag_code = loadSource(current_path / m_FragPath);
//Compile shaders
unsigned int vert_id = 0, frag_id = 0;
try
{
compileShaderCode(vert_code, vert_id, GL_VERTEX_SHADER);
}
catch (const std::runtime_error& e)
{
std::cerr << "Vertex Shader compilation failed: \n"
<< "filepath: " << m_VertPath << '\n'
<< e.what() << '\n';
return;
}
try
{
compileShaderCode(frag_code, frag_id, GL_FRAGMENT_SHADER);
}
catch (const std::runtime_error& e)
{
std::cerr << "Fragment Shader compilation failed: \n"
<< "filepath: " << m_FragPath << '\n'
<< e.what() << '\n';
return;
}
//Link program
m_ID = glCreateProgram();
glAttachShader(m_ID, vert_id);
glAttachShader(m_ID, frag_id);
glLinkProgram(m_ID);
int success = 0;
char info_log[512];
glGetProgramiv(m_ID, GL_LINK_STATUS, &success);
if (!success)
{
glGetProgramInfoLog(m_ID, 512, NULL, info_log);
std::cerr << "Error: Shader program linking failed: \n"
<< "filepaths: " << m_VertPath << ", " << m_FragPath << '\n'
<< info_log << '\n';
}
glDeleteShader(vert_id);
glDeleteShader(frag_id);
}
VertFragShader::VertFragShader(const std::string& vert_path, const std::string& frag_path)
: m_VertPath(vert_path), m_FragPath(frag_path)
{
Build();
}
VertFragShader::~VertFragShader()
{
glDeleteProgram(m_ID);
}
void ComputeShader::Build()
{
//Get source
std::filesystem::path current_path{ std::filesystem::current_path() };
std::string compute_code = loadSource(current_path / m_ComputePath);
//Initialize local sizes
RetrieveLocalSizes(compute_code);
//Compile shader
unsigned int compute_id = 0;
try
{
compileShaderCode(compute_code, compute_id, GL_COMPUTE_SHADER);
}
catch (const std::runtime_error& e)
{
std::cerr << "Compute Shader compilation failed: \n"
<< "filepath: " << m_ComputePath << '\n'
<< e.what() << '\n';
return;
}
//Link program
m_ID = glCreateProgram();
glAttachShader(m_ID, compute_id);
glLinkProgram(m_ID);
int success = 0;
char info_log[512];
glGetProgramiv(m_ID, GL_LINK_STATUS, &success);
if (!success)
{
glGetProgramInfoLog(m_ID, 512, NULL, info_log);
std::cerr << "Error: Shader program linking failed: \n"
<< "filepath: " << m_ComputePath << '\n'
<< info_log << '\n';
}
glDeleteShader(compute_id);
}
ComputeShader::ComputeShader(const std::string& compute_path)
: m_ComputePath(compute_path)
{
Build();
}
ComputeShader::~ComputeShader()
{
glDeleteProgram(m_ID);
}
void ComputeShader::Dispatch(uint32_t size_x, uint32_t size_y, uint32_t size_z)
{
glDispatchCompute(size_x/m_LocalSizeX, size_y/m_LocalSizeY, size_z/m_LocalSizeZ);
}
void ComputeShader::RetrieveLocalSizes(const std::string& source_code)
{
auto retrieveInt = [source_code](const std::string& name) -> int
{
auto id = source_code.find(name);
if (id == std::string::npos)
throw std::runtime_error("Unable to find " + name);
//Id was before the beggining of the name, so shift it one past the end
id += name.size() + 2;
//Skip whitespaces
while (source_code[id] == ' ' || source_code[id] == '\n' || source_code[id] == '\t')
{
id++;
}
//Create a string
std::string value;
//Load all digits into the string
while (isdigit(source_code[id]))
{
value += source_code[id];
id++;
}
if (value.empty())
throw std::runtime_error("Unable to parse value of " + name);
return std::stoi(value);
};
auto setSize = [this, retrieveInt](uint32_t& value, const std::string& name) {
try
{
value = retrieveInt(name);
}
catch (const std::exception& e)
{
std::cerr << "Exception thrown when parsing: " << m_ComputePath << '\n';
std::cerr << e.what() << '\n';
//Default to setting sizes as 1 if something goes wrong
value = 1;
}
};
setSize(m_LocalSizeX, "local_size_x");
setSize(m_LocalSizeY, "local_size_y");
setSize(m_LocalSizeZ, "local_size_z");
}
void Shader::setUniform1i(const std::string& name, int x) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform1i(location, x);
}
void Shader::setUniform2i(const std::string& name, int x, int y) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform2i(location, x, y);
}
void Shader::setUniform3i(const std::string& name, int x, int y, int z) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform3i(location, x, y, z);
}
void Shader::setUniform4i(const std::string& name, int x, int y, int z, int w) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform4i(location, x, y, z, w);
}
void Shader::setUniform1f(const std::string& name, float x) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform1f(location, x);
}
void Shader::setUniform2f(const std::string& name, float x, float y) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform2f(location, x, y);
}
void Shader::setUniform3f(const std::string& name, float x, float y, float z) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform3f(location, x, y, z);
}
void Shader::setUniform4f(const std::string& name, float x, float y, float z, float w) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform4f(location, x, y, z, w);
}
void Shader::setUniformMatrix4fv(const std::string& name, float data[16]) {
const unsigned int location = getUniformLocation(name.c_str());
glUniformMatrix4fv(location, 1, GL_FALSE, data);
}
void Shader::setUniform2i(const std::string& name, glm::ivec2 v) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform2i(location, v.x, v.y);
}
void Shader::setUniform3i(const std::string& name, glm::ivec3 v) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform3i(location, v.x, v.y, v.z);
}
void Shader::setUniform4i(const std::string& name, glm::ivec4 v) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform4i(location, v.x, v.y, v.z, v.w);
}
void Shader::setUniform2f(const std::string& name, glm::vec2 v) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform2f(location, v.x, v.y);
}
void Shader::setUniform3f(const std::string& name, glm::vec3 v) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform3f(location, v.x, v.y, v.z);
}
void Shader::setUniform4f(const std::string& name, glm::vec4 v) {
const unsigned int location = getUniformLocation(name.c_str());
glUniform4f(location, v.x, v.y, v.z, v.w);
}
void Shader::setUniformMatrix4fv(const std::string& name, glm::mat4 mat) {
const unsigned int location = getUniformLocation(name.c_str());
glUniformMatrix4fv(location, 1, GL_FALSE, glm::value_ptr(mat));
} |
<?php
namespace App\Http\Controllers;
class SessionsController extends Controller
{
public function create()
{
return view('sessions.create');
}
public function store()
{
$attributes = request()->validate([
'email' => 'required|email',
'password' => 'required',
]);
if(auth()->attempt($attributes)){
session()->regenerate();
return redirect('/',)->with('success', 'Welcome Back!');
}
return back()
->withInput()
->withErrors(['email' => 'Error email']);
}
public function destroy()
{
auth()->logout();
return redirect('/');
}
} |
import Vue from 'vue'
import Vuex from 'vuex'
import firebase from "firebase/app";
import { db } from "../main";
import router from '../router'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
uid: null,
infouser: {},
signupform: {
email: "",
password: "",
name: "",
date: null,
sex: '',
alert: null,
},
loginForm: {
email: "",
password: "",
alert: null,
},
},
getters: {
},
mutations: {
GET_CURRENT_USER(state, uid) {
state.uid = uid
},
GET_LOGIN_FORM(state, loginForm) {
state.loginForm = loginForm
},
GET_SIGNUP_FORM(state, signupform) {
state.signupform = signupform
},
GET_INFO_USER(state, infouser) {
state.infouser = infouser
},
},
actions: {
getcurrentUser(context) {
firebase.auth().onAuthStateChanged((user) => {
if (user) {
context.commit('GET_CURRENT_USER', user.uid)
} else {
context.commit('GET_CURRENT_USER', null)
}
});
},
signup(context, signupform) {
delete signupform.alerts;
firebase
.auth()
.createUserWithEmailAndPassword(signupform.email, signupform.password)
.then((cred) => {
db.collection("users").doc(cred.user.uid).set(signupform);
firebase
.auth()
.currentUser.sendEmailVerification()
.then(() => {
});
router.push({ name: 'UserPrivate', params: { userid: userCredential.user.uid } })
})
.catch((error) => {
signupform.alert = error
context.commit('GET_SIGNUP_FORM', signupform)
});
},
login(context, loginForm) {
firebase
.auth()
.signInWithEmailAndPassword(loginForm.email, loginForm.password)
.then((userCredential) => {
context.commit('GET_CURRENT_USER', userCredential.user)
router.push({ name: 'UserPrivate', params: { userid: userCredential.user.uid } })
})
.catch((error) => {
loginForm.alert = error
context.commit('GET_LOGIN_FORM', loginForm)
});
},
logout(context) {
firebase.auth().signOut().then(() => {
context.commit('GET_CURRENT_USER', null)
router.push({ name: 'Login' })
console.log(123123)
}).catch(() => {
});
},
getinfouser(context, uid) {
var docRef = db.collection("users").doc(uid)
docRef
.get()
.then((doc) => {
if (doc.exists) {
context.commit('GET_INFO_USER', doc.data())
} else {
console.log("No such document!");
}
})
.catch((error) => {
console.log("Error getting document:", error);
});
var docRef1 = db.collection("users").doc(uid).collection("category").doc('nganhang')
console.log("Cached document data:", docRef1.get());
}
},
modules: {
}
}) |
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.IdentityModel.Tokens;
using senai_gufi_webAPI.Domains;
using senai_gufi_webAPI.Interfaces;
using senai_gufi_webAPI.Repositories;
using senai_gufi_webAPI.ViewModels;
using System;
using System.Collections.Generic;
using System.IdentityModel.Tokens.Jwt;
using System.Linq;
using System.Security.Claims;
using System.Threading.Tasks;
namespace senai_gufi_webAPI.Controllers
{
[Produces("application/json")]
[Route("api/[controller]")]
[ApiController]
public class LoginController : ControllerBase
{
private IUsuarioRepository _usuarioRepository { get; set; }
public LoginController()
{
_usuarioRepository = new UsuarioRepository();
}
/// <summary>
/// Valida o usuário
/// </summary>
/// <param name="login">Objeto login com e-mail e senha do usuário</param>
/// <returns>Status Code 200 (OK) com token do usuário autenticado</returns>
[HttpPost]
public IActionResult Login(LoginViewModel login)
{
try
{
Usuario usuarioBuscado = _usuarioRepository.Login(login.Email, login.Senha);
if (usuarioBuscado == null)
{
return NotFound("E-mail ou senha inválidos!");
}
// Caso o usuário seja encontrado, prossegue para a criação do token:
var clamzinha = new[]
{
new Claim(JwtRegisteredClaimNames.Email, usuarioBuscado.Email),
new Claim(JwtRegisteredClaimNames.Jti, usuarioBuscado.IdUsuario.ToString()),
new Claim(ClaimTypes.Role, usuarioBuscado.IdTipo.ToString())
};
var key = new SymmetricSecurityKey(System.Text.Encoding.UTF8.GetBytes("gufi-chave-autenticacao"));
var creds = new SigningCredentials(key, SecurityAlgorithms.HmacSha256);
var meuToken = new JwtSecurityToken(
issuer: "gufi.webAPI",
audience: "gufi.webAPI",
claims: clamzinha,
expires: DateTime.Now.AddMinutes(30),
signingCredentials: creds
);
return Ok(
new
{
tokenGerado = new JwtSecurityTokenHandler().WriteToken(meuToken)
} );
}
catch (Exception ex)
{
return BadRequest(ex);
}
}
}
} |
/*
* Copyright (C) 2012 Sistemas Operativos - UTN FRBA. All rights reserved.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
#include <stdlib.h>
#include "bitarray.h"
/*
* @NAME: bitarray_create
* @DESC: Crea y devuelve un puntero a una estructura t_bitarray
* @PARAMS:
* bitarray
* size - Tamaño en bytes del bit array
*/
t_bitarray *bitarray_create(char *bitarray, size_t size) {
t_bitarray *self = malloc(sizeof(t_bitarray));
self->bitarray = bitarray;
self->size = size;
return self;
}
/*
* @NAME: bitarray_test_bit
* @DESC: Devuelve el valor del bit de la posicion indicada
*/
bool bitarray_test_bit(t_bitarray *self, off_t bit_index) {
return((self->bitarray[BIT_CHAR(bit_index)] & BIT_IN_CHAR(bit_index)) != 0);
}
/*
* @NAME: bitarray_set_bit
* @DESC: Setea el valor del bit de la posicion indicada
*/
void bitarray_set_bit(t_bitarray *self, off_t bit_index) {
self->bitarray[BIT_CHAR(bit_index)] |= BIT_IN_CHAR(bit_index);
}
/*
* @NAME: bitarray_clean_bit
* @DESC: Limpia el valor del bit de la posicion indicada
*/
void bitarray_clean_bit(t_bitarray *self, off_t bit_index){
unsigned char mask;
/* create a mask to zero out desired bit */
mask = BIT_IN_CHAR(bit_index);
mask = ~mask;
self->bitarray[BIT_CHAR(bit_index)] &= mask;
}
/*
* @NAME: bitarray_get_max_bit
* @DESC: Devuelve la cantidad de bits en el bitarray
*/
size_t bitarray_get_max_bit(t_bitarray *self) {
return self->size * CHAR_BIT;
}
/*
* @NAME: bitarray_destroy
* @DESC: Destruye el bit array
*/
void bitarray_destroy(t_bitarray *self) {
free(self);
} |
/*
CSCI335 Fall 2023
Assignment 1 – Card Game
Name: Kazi Anwar
Date: November 2nd, 2023
PointCard.cpp defines the member functions for the PointCard class.
*/
#include "PointCard.hpp"
PointCard::PointCard() : Card()
{
setInstruction("");
setType(POINT_CARD);
setImageData(nullptr);
setDrawn(false);
//point card is a typa card so u inherit it from card class and defualt is point so u dont even need to change the cardtype_
//UPDATE yea u do now cause in ur card.cpp constructor u dont have it default to point card
}
bool PointCard::isPlayable() //check if the point card is playable
{
//if the card is not drawn yet, its not playable
if (getDrawn() == false)
{
return false;
}
//V1 works
//convert instruction to integer and then check if its greater than 99 or less than 1 which means its not within the range so not playable
// if (stoi(getInstruction()) > 99 || stoi(getInstruction()) < 1)
// {
// return false;
// }
// return true; //if ur here it means card has been drawn and the points are withing 1 and 99 meaning it is playable
//V2 works but efficienter?
const std::string& x = getInstruction(); //x is gonna hold the instruction string thats returned from get isntruction
for (char c : x) //iterate thru each character c in string x
{
if (isdigit(c) == false) //for each charachter u encounter, check if its a digit. only enter the ifstatement if it is not a digit
{
return false; //return false if its not a digit
}
}
return true; //if ur here it means all the digits are digits and its playbale
}
void PointCard::Print() const
{
//shows card type, points of the point card then the card image data but first u check if there isss data. If no then say no, if yes then show it
std::cout << "Type: " << getType() << "\n" << "Points: " << getInstruction() << "\n" << "Card: " << "\n";
if (getImageData() == nullptr)
{
std::cout << "No image data" << std::endl;
}
if (getImageData() != nullptr)
{
for (int i = 0; i < 80; ++i) //prof said ++i is better than i++ cuz in i++ u do a copy
{
std::cout << getImageData()[i];
}
}
} |
import React, { useEffect, useRef } from "react";
import bb, { bar } from "billboard.js";
const StackedBarPlot = () => {
const ref = useRef(null);
useEffect(() => {
bb.generate({
size: {
height: ref.current.clientHeight,
width: 400,
},
axis: {
rotated: true,
x: {
type: "category",
categories: [
"bmw",
"chevrolet",
"chrysler",
"dodge",
"ford",
"gmc",
"honda",
"hyundai",
"jeep",
"kia",
"lexus",
"mercedes-benz",
"nissan",
"ram",
"subaru",
"toyota",
"volkswagen",
],
height: 80,
},
y: {},
},
data: {
columns: [
[
"sedan",
1388,
2795,
839,
1027,
2511,
0,
2639,
1513,
0,
847,
1134,
1212,
2197,
0,
724,
2687,
1078,
],
[
"SUV",
0,
2754,
0,
0,
3487,
1334,
1567,
0,
3515,
0,
0,
0,
1492,
0,
727,
2022,
0,
],
[
"coupe",
0,
1394,
0,
836,
1133,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
],
[
"pickup",
0,
2711,
0,
0,
3038,
1457,
0,
0,
0,
0,
0,
0,
0,
1770,
0,
1177,
0,
],
[
"truck",
0,
3109,
0,
0,
4853,
1192,
0,
0,
0,
0,
0,
0,
0,
1710,
0,
1105,
0,
],
[
"van",
0,
0,
0,
0,
1187,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
],
[
"other",
0,
0,
0,
0,
0,
0,
0,
0,
861,
0,
0,
0,
0,
0,
0,
0,
0,
],
[
"wagon",
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
748,
0,
0,
],
[
"hatchback",
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
766,
0,
],
],
type: bar(),
groups: [
[
"sedan",
"SUV",
"coupe",
"pickup",
"truck",
"van",
"other",
"wagon",
"hatchback",
],
],
},
bar: {
width: {
ratio: 0.5,
},
},
legend: {
show: false,
},
bindto: "#stacked_bar_plot",
});
}, []);
return (
<div style={{ height: "100%" }} ref={ref} id="stacked_bar_plot"></div>
);
};
export default StackedBarPlot; |
---
layout: post
title: JavaScript Arrays
tags: phase-1 javascript js arrays
---
## 🗓️Today's topics
- Using arrays
- Iteration: using loops with arrays
- Transforming arrays with map, reduce, and filter
## 🎯 Project
[JS Calculator Part 1](https://classroom.github.com/a/dhNA3tEy)
## 🔖 Resources
- [MDN Loops and Iteration](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Loops_and_iteration)
- [MDN Array](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array)
- [MDN Bucles e iteración, Arrays en español](https://developer.mozilla.org/es/docs/Web/JavaScript/Guide/Loops_and_iteration)
- [A pretty good explanation of map, filter, and reduce](https://dev.to/chrisachard/map-filter-reduce-crash-course-5gan)
- [map, filter, and reduce in a tweet](https://twitter.com/steveluscher/status/741089564329054208)
- [A Visualization of What Reduce Does](http://reduce.surge.sh/)
### ⭐️ EXTRA/TMI
- [Eloquent JavaScript Chapter 5: Higher Order Functions](https://eloquentjavascript.net/05_higher_order.html) - This is a pretty intense read but it includes examples of map, filter, and reduce.
## 🦉 Code, Notes & Video
- [JavaScript Arrays notes](https://github.com/Momentum-Team-13/notes/blob/main/js-arrays.md)
- [Array examples from notes codepen](https://codepen.io/rlconley/pen/eYVeyJx)
- [Rebecca's video on for loops & while loops](https://vimeo.com/426872183)
- [Rebecca's video on for of loops](https://vimeo.com/426872217) |
# Data Validation with Data Annotations
In this chapter we will use Data Annotations to:
1. Restrict Chore name to a maximum length of 100 characters
1. Restrict Chore difficulties to a range of 1-5
1. Validate a user's registration information
## Using the `DataAnnotations` namespace
The attributes you will be using in this chapter come from the `System.ComponentModel.DataAnnotations`, so you will need to add a `using` directive for that namespace in each file in which you wish to use them.
## Maximum Length for Chore Names
The class definition for `Chore` and `ChoreDto` should look something like this up to this point:
>Chore.cs
``` csharp
namespace HouseRules.Models;
public class Chore
{
public int Id { get; set; }
public string Name { get; set; }
public int Difficulty { get; set; }
public int ChoreFrequencyDays { get; set; }
public List<ChoreAssignment> ChoreAssignments { get; set; }
public List<ChoreCompletion> ChoreCompletions { get; set; }
}
```
You can use the `MaxLength` Attribute to limit the length of `Chore` and `ChoreDto` names by adding it to the property like this:
``` csharp
[MaxLength(100)]
public string Name { get; set; }
```
You can also describe a range for numeric values:
``` csharp
[Range(1,5)]
public int Difficulty { get; set; }
```
Restart your API when you have made these updates (if it was already running) and test out these validators. Try to add chores with very long names or with difficulties that are too high. The API should return a `400 Bad Request` response, and the chore will not be added to the database.
## Giving the User Feedback
While it is good to protect our database from data that we don't want, it is a better UX to give the user feedback that their attempt failed, and why.
If you look at one of the failed `POST` HTTP requests in the dev tools, you can see that the API actually sends back meaningful feedback with validation error messages:

We can use these messages in the front end, but first, at least one of these messages isn't very useful. Your users might not (probably don't actually) know what an "array type" is, so let's define a better message in the `Chore` class like this:
``` csharp
[MaxLength(100, ErrorMessage = "Chore names must be 100 characters or less")]
public string Name { get; set; }
```
### Displaying Errors to the User
Now that the error message is more helpful, you can update the handler to check for the 400 response, and act accordingly:
1. Add a state variable called `errors` to the `CreateChore` component
1. Update the handler to check for an `errors` property in the response. If it is there, then set the `errors` state variable to the value of the response's `errors` value. Your code might look different, but here is an example:
``` javascript
const handleCreateChore = (evt) => {
evt.preventDefault();
const newChore = {
name,
difficulty,
choreFrequencyDays,
};
createChore(newChore).then((res) => {
if (res.errors) {
setErrors(res.errors);
} else {
navigate("/chores");
}
});
};
```
1. All that is left is to create some UI elements that will display the errors when they are set:
```jsx
<div style={{ color: "red" }}>
{Object.keys(errors).map((key) => (
<p key={key}>
{key}: {errors[key].join(",")}
</p>
))}
</div>
```
At this point in the course, though you haven't necessarily been shown code like this, you should be able to figure out what each part of it is doing. Look up `Object.keys` and `join` if you forget what those do in Javascript. Ask an instructor if any of the code here is unclear.
1. Test the form again to make sure you get appropriate feedback for bad input.
## Practice
1. Update the `Chore` class and the `CreateChore` component to enforce a minimum `ChoreFrequencyDays` of 1, and a maximum of 14
- bonus challenge: use the [datalist](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input/number#offering_suggested_values) element to suggest 1,3,7,10, and 14 as options (note that this will have no effect on the allowed values on the server side)
1. Use the `EmailAddress` data annotation to validate that a registering user has input a valid email for their registration, and use the `MaxLength` attribute to ensure that the username submitted is less than 50 characters.
1. You have already seen the `Required` data annotation used in data models to make nullable types `NOT NULL` in the database. You can also use `Required` to make data properties required when submitted to the API. Try adding it to properties on the Chore and Registration models to further improve model validation for these types.
Up Next: [More features for House Rules](./house-rules-more-features.md)
## 🔍 Additional Materials
1. [ Available Data Annotations](https://learn.microsoft.com/en-us/dotnet/api/system.componentmodel.dataannotations?view=net-6.0) |
import React, { useContext, useEffect, useState } from "react";
import { Row, Col } from "react-bootstrap";
import { LabelField } from "../../../components/fields";
import { CardLayout } from "../../../components/cards";
import PageLayout from "../../../layouts/PageLayout";
import data from "../../../data/master/updateEvent.json";
import { ThemeContext } from "../../../context/Themes";
import axios from "axios";
import { useNavigate } from "react-router-dom";
import EventImageUploader from "../imgUploader/ImageUploader";
import { ToastContainer, toast } from "react-toastify";
import "react-toastify/dist/ReactToastify.css";
import { Box, Anchor, Label } from "../../../components/elements";
import Breadcrumb from "../../../components/Breadcrumb";
export default function EditEvent() {
const navigate = useNavigate();
const serverUrl = process.env.REACT_APP_BASE_URL;
const token = localStorage.getItem("token");
const eventId = localStorage.getItem("eventId");
const redirectedFrom = localStorage.getItem("redirectedFrom");
const { theme } = useContext(ThemeContext);
const [eventData, setEventData] = useState({
name: "",
description: "",
img: [],
address: "",
type: "",
start_time: "",
end_time: "",
start_date: "",
end_date: "",
capacity: "",
lat: "",
long: "",
price: "",
status :"",
});
const [submitter, setSubmitter] = useState("Submit");
const [errorMessage, setErrorMessage] = useState({
name: "",
description: "",
img: "",
address: "",
type: "",
start_time: "",
end_time: "",
start_date: "",
end_date: "",
capacity: "",
lat: "",
long: "",
price: "",
});
useEffect(() => {
const fetchEventDetails = async () => {
try {
const response = await axios.get(`${serverUrl}/event/fetch/${eventId}`);
if (response.status === 200) {
console.log("fetched event data for selected event", response.data);
const commonEventData = {
name: response.data.name,
description: response.data.description,
img: response.data.image,
address: response.data.address,
type: response.data.type,
status : response.data.status,
start_time: response.data.start_time,
end_time: response.data.end_time,
start_date: response.data.start_date,
end_date: response.data.end_date,
capacity: response.data.capacity,
lat: response.data.lat,
long: response.data.long,
};
const eventDataWithPrice = {
...commonEventData,
price: response.data.price,
};
setEventData(
response.data.price ? eventDataWithPrice : commonEventData
);
}
} catch (error) {
navigate(`/${redirectedFrom}`);
console.log(error, "error");
}
};
fetchEventDetails();
}, []);
const handleOnChange = (e) => {
const { name } = e.target;
setErrorMessage((prevErrors) => ({ ...prevErrors, [name]: "" }));
setEventData((prevValue) => ({ ...prevValue, [name]: e.target.value }));
};
console.log(eventData, "event data");
//// STYLING
const crossBtn_style = {
color: theme === "dark_mode" ? "white" : "black",
fontWeight: "900",
fontSize: "15px",
padding: "2px 6px",
};
const errorTxt = {
fontSize: "11px",
padding: "2px 0px",
color: "#940e1d",
position: "absolute",
};
const validateForm = () => {
const errors = {};
if (!eventData.name) {
errors.name = "Event Name is required.";
}
if (eventData.description.length < 10) {
errors.description = "Description is required.";
}
if (!eventData.address) {
errors.address = "Address is required";
}
if (!eventData.type) {
errors.type = "Event Type is required";
}
if (!eventData.start_time) {
errors.start_time = "Open Time is required.";
}
if (!eventData.end_time) {
errors.end_time = "Close Time is required.";
}
if (!eventData.start_date) {
errors.start_date = "Start Date is required.";
}
if (!eventData.end_date) {
errors.end_date = "End Data is required.";
}
if (!eventData.capacity) {
errors.capacity = "Capacity is required.";
}
if (!eventData.lat) {
errors.lat = "Latitude is required.";
}
if (!eventData.long) {
errors.long = "Longitude is required.";
}
if (eventData.type === "paid") {
if (!eventData.price) {
errors.price = "Price is required.";
}
}
if (!eventData.img.length > 0) {
errors.img = "Event Image required.";
}
setErrorMessage(errors);
return Object.keys(errors).length === 0;
};
///// TO CHANGE THE STATUS OF EVENT
// const data = {
// Id : event_id,
// status : "status", ( "upcommin", ongoing , expired)
// }
// const response = await axios.get(
// `${serverUrl}/event/changeEventStatus`,
// { headers }
// );
const handleUpdataEvent = async (e) => {
e.preventDefault();
const isFormValid = validateForm();
console.log(isFormValid);
if (isFormValid) {
setSubmitter("Submitting ....");
console.log("form submitted");
try {
const headers = {
token: token,
};
const Id = eventId;
console.log(Id, "id");
const formData = new FormData();
formData.append("name", eventData.name);
formData.append("description", eventData.description);
for (let i = 0; i < eventData.img.length; i++) {
formData.append("img", eventData.img[i]);
}
formData.append("address", eventData.address);
formData.append("type", eventData.type);
formData.append("status", eventData.status);
formData.append("start_time", eventData.start_time);
formData.append("end_time", eventData.end_time);
formData.append("start_date", eventData.start_date);
formData.append("end_date", eventData.end_date);
formData.append("capacity", eventData.capacity);
formData.append("lat", eventData.lat);
formData.append("long", eventData.long);
if (eventData.price) {
formData.append("price", eventData.price);
}
formData.append("Id", eventId);
console.log(formData, " data to be sent");
const response = await axios.patch(
`${serverUrl}/event/update`,
formData,
{ headers }
);
if (response.status === 200) {
toast.success(response.data.result);
setTimeout(() => {
console.log("Message", response.data);
navigate(`/${redirectedFrom}`);
}, 2000);
}
} catch (error) {
setSubmitter("Submit");
console.error("Error:", error);
toast.error("An error occurred");
}
} else {
toast.warn("Please fill the All required fields");
}
};
const getUploadedImageFile = (imageAsInput) => {
setEventData((prevData) => ({ ...prevData, img: imageAsInput }));
};
return (
<PageLayout>
<Row>
<Col xl={12} style={{ margin: "20px auto" }}>
<CardLayout>
<Breadcrumb title={data?.pageTitle}>
{data?.breadcrumb.map((item, index) => (
<li key={index} className="mc-breadcrumb-item">
{item.path ? (
<Anchor className="mc-breadcrumb-link" href={item.path}>
{item.text}
</Anchor>
) : (
item.text
)}
</li>
))}
</Breadcrumb>
</CardLayout>
</Col>
<Col xl={7} style={{ margin: "0 auto" }}>
<CardLayout>
<div className="header d-flex justify-content-between align-items-center">
<h5 className="opacity-0">Add Event</h5>
<button
className="btn btn-sm btn-circle absolute"
style={crossBtn_style}
onClick={() => navigate(`/${redirectedFrom}`)}
>
✕
</button>
</div>
<Row>
<Col xl={6}>
<LabelField
onChange={handleOnChange}
type="text"
label="Event Name"
name="name"
value={eventData.name}
placeholder="Enter Event Name"
fieldSize="w-100 h-md"
/>
{errorMessage.name && (
<p style={errorTxt}>{errorMessage.name}</p>
)}
</Col>
<Col xl={6}>
<LabelField
onChange={handleOnChange}
type="number"
label="Capacity"
name="capacity"
value={eventData.capacity}
onKeyPress={(event) =>
!/[0-9]/.test(event.key) && event.preventDefault()
}
placeholder="Enter Capacity"
fieldSize="w-100 h-md"
/>
{errorMessage.capacity && (
<p style={errorTxt}>{errorMessage.capacity}</p>
)}
</Col>
<Col xl={12}>
<Box className=" d-flex flex-column justify-content-start gap-2">
<Label className="mc-label-field-title">description</Label>
<textarea
placeholder="Input your text...."
name="description"
rows="4"
value={eventData.description}
className="mc-label-field-textarea "
onChange={handleOnChange}
></textarea>
</Box>
{errorMessage.description && (
<p style={errorTxt}>{errorMessage.description}</p>
)}
</Col>
<Col xl={6}>
<LabelField
onChange={handleOnChange}
type="time"
label="Open Time"
name="start_time"
value={eventData.start_time}
placeholder="Enter address"
fieldSize="w-100 h-md"
/>
{errorMessage.start_time && (
<p style={errorTxt}>{errorMessage.start_time}</p>
)}
</Col>
<Col xl={6}>
<LabelField
onChange={handleOnChange}
type="time"
label="Closing Time"
name="end_time"
value={eventData.end_time}
placeholder="Enter address"
fieldSize="w-100 h-md"
/>
{errorMessage.end_time && (
<p style={errorTxt}>{errorMessage.end_time}</p>
)}
</Col>
<Col xl={6}>
<span className="mc-label-field-title">Start Date</span>
<div
style={{ height: "45px" }}
className="checkbox-container d-flex justify-content-between align-items-center mt-2 mc-label-field-input"
>
<input
type="date"
value={eventData.start_date}
name="start_date"
onChange={handleOnChange}
/>
</div>
{errorMessage.start_date && (
<p style={errorTxt}>{errorMessage.start_date}</p>
)}
</Col>
<Col xl={6}>
<span className="mc-label-field-title">End Date</span>
<div
style={{ height: "45px" }}
className="checkbox-container d-flex justify-content-between align-items-center mt-2 mc-label-field-input"
>
<input
type="date"
value={eventData.end_date}
name="end_date"
onChange={handleOnChange}
/>
</div>
{errorMessage.end_date && (
<p style={errorTxt}>{errorMessage.end_date}</p>
)}
</Col>
<Col xl={6}>
<LabelField
name="type"
onChange={handleOnChange}
value={eventData.type}
label="Event Type"
option={["free", "paid"]}
fieldSize="w-100 h-md"
/>
{errorMessage.type && (
<p style={errorTxt}>{errorMessage.type}</p>
)}
</Col>
{eventData.type === "paid" && (
<Col xl={6}>
<LabelField
onChange={handleOnChange}
type="number"
label="Ticket Price"
name="price"
onKeyPress={(event) =>
!/[0-9]/.test(event.key) && event.preventDefault()
}
value={eventData.price}
placeholder="Enter Price"
fieldSize="w-100 h-md"
/>
{errorMessage.price && (
<p style={errorTxt}>{errorMessage.price}</p>
)}
</Col>
)}
<Col xl={6}>
<LabelField
name="status"
onChange={handleOnChange}
value={eventData.status}
label="Status"
option={["ongoing", "upcomming", "expired"]}
fieldSize="w-100 h-md"
/>
{errorMessage.type && (
<p style={errorTxt}>{errorMessage.type}</p>
)}
</Col>
<Col xl={eventData.type === "paid" ? 6 : 12}>
<LabelField
onChange={handleOnChange}
type="text"
label="address"
name="address"
value={eventData.address}
placeholder="Enter Address"
fieldSize="w-100 h-md"
/>
{errorMessage.address && (
<p style={errorTxt}>{errorMessage.address}</p>
)}
</Col>
<Col xl={6}>
<LabelField
onChange={handleOnChange}
type="number"
label="Latitude"
name="lat"
value={eventData.lat}
placeholder="Enter latitude "
fieldSize="w-100 h-md"
onKeyPress={(event) =>
!/^[0-9.]$/.test(event.key) && event.preventDefault()
}
/>
{errorMessage.lat && <p style={errorTxt}>{errorMessage.lat}</p>}
</Col>
<Col xl={6}>
<LabelField
onChange={handleOnChange}
type="number"
label="Longitude"
name="long"
value={eventData.long}
placeholder="Enter Longitude"
fieldSize="w-100 h-md"
onKeyPress={(event) =>
!/^[0-9.]$/.test(event.key) && event.preventDefault()
}
/>
{errorMessage.long && (
<p style={errorTxt}>{errorMessage.long}</p>
)}
</Col>
<Col xl={12}>
<span className="mc-label-field-title">Event Images </span>
<div
style={{ height: "45px" }}
className="checkbox-container d-flex gap-3 flex-row-reverse align-items-center mt-2 mc-label-field-input"
>
<EventImageUploader
fetchImage={eventData.img}
imgArrayLimit={6}
getUploadedImageFile={getUploadedImageFile}
/>
</div>
{errorMessage.img && <p style={errorTxt}>{errorMessage.img}</p>}
</Col>
<Col xl={12}>
<div
style={{ height: "45px" }}
className=" d-flex justify-content-center "
>
<button
className="btn normal-case btn-primary "
style={{
height: "35px",
display: "flex",
alignItems: "center",
justifyContent: "center",
margin: "10px auto 20px auto",
animation: "none",
}}
onClick={handleUpdataEvent}
>
{submitter}
</button>
</div>
</Col>
</Row>
</CardLayout>
</Col>
</Row>
<ToastContainer
oastContainer
// position="bottom-center"
autoClose={5000}
hideProgressBar={false}
newestOnTop={false}
closeOnClick
rtl={false}
pauseOnFocusLoss
draggable
pauseOnHover
theme="light"
/>
</PageLayout>
);
} |
<%@taglib prefix="t" tagdir="/WEB-INF/tags"%>
<%@taglib prefix="spring" uri="http://www.springframework.org/tags"%>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%@page contentType="text/html;charset=UTF-8" language="java"%>
<t:template>
<jsp:body>
<!-- top main content -->
<%@include file="topMainContent.jsp"%>
<!-- [END] top main content -->
<!-- Breadcrumb -->
<div class="container padded">
<div class="row">
<div id="breadcrumbs">
<div class="breadcrumb-button blue">
<span class="breadcrumb-label"><i class="icon-home"></i>Home</span>
<span class="breadcrumb-arrow"><span></span></span>
</div>
<div class="breadcrumb-button">
<span class="breadcrumb-label"> <i class="icon-envelope-alt"></i> <spring:message code="label.campaigns" />
</span> <span class="breadcrumb-arrow"><span></span></span>
</div>
</div>
</div>
</div>
<!-- [END] Breadcrumb -->
<!-- main content -->
<div class="container">
<div class="box">
<div class="box-header">
<span class="title"><spring:message code="label.mailingLists" /></span>
<ul class="box-toolbar">
<li>
<a href="${pageContext.request.contextPath}/campaign/create">
<button class="btn btn-green">
<i class="icon-envelope-alt"></i>  <spring:message code="label.newCampaign" />
</button>
</a>
</li>
</ul>
</div><!-- box-header -->
<div class="box-content">
<div id="dataTables">
<table cellpadding="0" cellspacing="0" border="0" class="dTable responsive">
<thead>
<tr>
<th style="width:50px;">
</th>
<th>
<spring:message code="label.name" />
</th>
<th>
<spring:message code="label.description" />
</th>
<th>
<spring:message code="label.createdOn" />
</th>
<th>
<spring:message code="label.status" />
</th>
<th>
<spring:message code="label.submittedOn" />
</th>
</tr>
</thead>
<tbody>
<c:forEach var="campaign" items="${campaigns}">
<tr>
<td class="center">
<div class="btn-group">
<button class="btn btn-xs btn-default dropdown-toggle"
data-toggle="dropdown">
<i class="icon-cog"></i>
</button>
<ul class="dropdown-menu">
<li>
<a href="${pageContext.request.contextPath}/campaign/edit/${campaign.id}"><spring:message code="label.edit"/></a>
</li>
<li class="divider" />
<li>
<a href="${pageContext.request.contextPath}/campaign/delete/${campaign.id}"><spring:message code="label.delete"/></a>
</li>
</ul>
</div>
</td>
<td><a href="${pageContext.request.contextPath}/campaign/edit/${campaign.id}">${campaign.name}</a></td>
<td>${campaign.description}</td>
<td>${campaign.formatedCreatedOn}</td>
<td>
<spring:message code="${campaign.status.description}" />
<%-- <c:choose>
<c:when test="${campaign.submitted}">
<spring:message code="status.submitted"/>
</c:when>
<c:otherwise>
<spring:message code="status.NotSubmitted"/>
</c:otherwise>
</c:choose> --%>
</td>
<td>${campaign.formatedSubmittedDate}</td>
</tr>
</c:forEach>
</tbody>
</table>
</div>
</div>
</div>
</div>
<!-- [END] main content -->
</jsp:body>
</t:template> |
// Напишите приложение, которое выводит квадраты натуральных чисел на экран, а после получения сигнала ^С обрабатывает этот сигнал, пишет «выхожу из программы» и выходит.
//Для реализации данного паттерна воспользуйтесь каналами и оператором select с default-кейсом.
package main
import (
"fmt"
"os"
"os/signal"
"syscall"
"time"
)
func square(ch chan int, quit chan os.Signal) {
x := 1
for {
select {
case <-quit:
fmt.Println("выхожу из программы")
return
default:
ch <- x * x
x++
time.Sleep(1 * time.Second)
}
}
}
func main() {
ch := make(chan int)
quit := make(chan os.Signal, 1)
signal.Notify(quit, os.Interrupt, syscall.SIGTERM) //Буферизированный канал используется в этом коде для обеспечения того, что сигналы операционной системы (os.Interrupt, syscall.SIGTERM) могут быть корректно обработаны, даже если главная горутина занята и не может немедленно принять сигнал.
go func() {
for {
fmt.Println(<-ch)
}
}()
square(ch, quit)
} |
from django import forms
from django.db import models
datTyps = (
('dryBulbTemperature', 'Dry Bulb Temperature'),
('dewPointTemperature', 'Dew Point Temperature'),
('relativeHumidity', 'Relative Humidity'),
('windSpeed', 'Wind Speed'),
('globalHorizontalRadiation', 'Global Horizontal Radiation'),
('directNormalRadiation', 'Direct Normal Radiation'),
('diffuseHorizontalRadiation', 'Diffuse Horizontal Radiation'),
('horizontalInfraredRadiationIntensity', 'Horizontal Infrared Radiation'),
('globalHorizontalIlluminance', 'Global Horizontal Illuminance'),
('directNormalIlluminance', 'Direct Normal Illuminance'),
('diffuseHorizontalIlluminance', 'Diffuse Horizontal Illuminance'),)
legColors = (
('plasma', 'Plasma'),
('inferno', 'Inferno'),
('magma', 'Magma'),
('Greys', 'Greys'),
('Purples', 'Purples'),
('Blues', 'Blues'),
('Greens', 'Greens'),
('Oranges', 'Oranges'),
('Reds', 'Reds'),
('YlOrBr', 'YellowOrangeBrown'),
('YlOrRd', 'OrangeRed'),
('PuRd', 'PurpleRed'),
('RdPu', 'Red Purple'),
('BuPu', 'BluePurple'),
('GnBu', 'GreenBlue'),
('PuBu', 'PurplBlue'),
('YlGnBu', 'YellowGreenBlue'),
('PuBuGn', 'PurpleBlueGreen'),
('BuGn', 'BlueGreen'),
('YlGn', 'YellowGreen'),)
class DatTyp(models.Model):
datTyp = models.CharField(max_length=25,choices=datTyps)
class LegColor(models.Model):
legColor = models.CharField(max_length=25,choices=legColors)
class CityForm(forms.Form):
city_name = forms.CharField(label='City Name', max_length=100)
data_type = forms.ChoiceField(label='Data Type', choices=datTyps)
color = forms.ChoiceField(label='Color', choices=legColors) |
package com.jeffdisher.cacophony.caches;
import org.junit.Assert;
import org.junit.Test;
import com.jeffdisher.cacophony.data.global.AbstractDescription;
import com.jeffdisher.cacophony.testutils.MockKeys;
import com.jeffdisher.cacophony.testutils.MockSingleNode;
import com.jeffdisher.cacophony.types.IpfsFile;
public class TestLocalUserInfoCache
{
public static final IpfsFile F1 = MockSingleNode.generateHash(new byte[] {1});
public static final IpfsFile F2 = MockSingleNode.generateHash(new byte[] {2});
@Test
public void testEmpty() throws Throwable
{
LocalUserInfoCache cache = new LocalUserInfoCache();
ILocalUserInfoCache.Element elt = cache.getUserInfo(MockKeys.K1);
Assert.assertNull(elt);
}
@Test
public void testBasicRead() throws Throwable
{
LocalUserInfoCache cache = new LocalUserInfoCache();
cache.setUserInfo(MockKeys.K1, _createDescription("name", "description", F1, null, null, null));
ILocalUserInfoCache.Element elt = cache.getUserInfo(MockKeys.K1);
Assert.assertEquals("name", elt.name());
Assert.assertEquals("description", elt.description());
Assert.assertEquals(F1, elt.userPicCid());
Assert.assertNull(elt.emailOrNull());
Assert.assertNull(elt.websiteOrNull());
}
@Test
public void testReadAfterUpdate() throws Throwable
{
LocalUserInfoCache cache = new LocalUserInfoCache();
cache.setUserInfo(MockKeys.K1, _createDescription("name", "description", F1, null, null, null));
ILocalUserInfoCache.Element elt1 = cache.getUserInfo(MockKeys.K1);
cache.setUserInfo(MockKeys.K1, _createDescription("name2", "description2", F2, "email", "site", null));
ILocalUserInfoCache.Element elt2 = cache.getUserInfo(MockKeys.K1);
Assert.assertEquals("name", elt1.name());
Assert.assertEquals("description", elt1.description());
Assert.assertEquals(F1, elt1.userPicCid());
Assert.assertNull(elt1.emailOrNull());
Assert.assertNull(elt1.websiteOrNull());
Assert.assertEquals("name2", elt2.name());
Assert.assertEquals("description2", elt2.description());
Assert.assertEquals(F2, elt2.userPicCid());
Assert.assertEquals("email", elt2.emailOrNull());
Assert.assertEquals("site", elt2.websiteOrNull());
}
private static AbstractDescription _createDescription(String name, String description, IpfsFile userPicCid, String emailOrNull, String websiteOrNull, IpfsFile featureOrNull)
{
AbstractDescription instance = AbstractDescription.createNew();
instance.setName(name);
instance.setDescription(description);
instance.setUserPic("image/jpeg", userPicCid);
instance.setEmail(emailOrNull);
instance.setWebsite(websiteOrNull);
instance.setFeature(featureOrNull);
return instance;
}
} |
<template>
<div class="webFile">
<h1 style="margin-top: 3%">请输入可访问的FileUrl地址</h1>
<div style="margin: 30px 0;" />
<el-input
class="cl-textarea"
type="textarea"
:autosize="{ minRows: 3, maxRows: 4 }"
placeholder="请输入内容,保证文档可访问,如http://www.file.cn/a.docx"
v-model="textarea"
/>
<div style="margin: 40px 0;" />
<br />
<el-button icon="el-icon-delete" class="el-button" @click="clClean"
>clean</el-button
>
<el-button
icon="el-icon-check"
class="el-button"
@click="clSubmit"
v-loading.fullscreen.lock="loading"
>view</el-button
>
<el-button
icon="el-icon-refresh-right"
class="el-button"
@click="clConvert"
v-loading.fullscreen.lock="loading"
>
convert
</el-button>
<div
@mouseenter="mouseenter(i)"
@mouseleave="mouseleave(i)"
v-for="i in 2"
:key="i"
class="template"
style="margin-top:20px;position:relative;width: 200px; height: 200px;left:50%;transform: translateX(-100px);"
>
<el-image
class="template-img"
src="https://fuss10.elemecdn.com/e/5d/4a731a90594a4af544c0c25941171jpeg.jpeg"
></el-image>
<div v-if="chooseIndex !== i" class="template-title">
文件标题文件标题文件标题文件标题
</div>
<div v-if="chooseIndex === i" class="template-shadow">
<div class="template-shadow-title">
哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈
</div>
<el-button type="primary" class="template-shadow-btn">查看</el-button>
<div class="template-shadow-collect">收藏</div>
</div>
</div>
</div>
</template>
<script>
import { getViewUrlWebPath, convertReq, convertRes } from "../api/index";
import { fileSuffix } from "@/utils/common-data";
import { throttle } from "../utils/index";
export default {
name: "webFile",
data() {
return {
loading: false,
textarea: "",
convertResIndex: 0,
convertRes: "",
chooseIndex: "",
};
},
methods: {
mouseenter(i) {
this.chooseIndex = i;
console.log("鼠标移入了", i);
},
mouseleave: throttle(function(i) {
this.chooseIndex = "";
console.log("鼠标移出了", i);
}, 1000),
checkFileUrl(e) {
if (!this.textarea) {
this.showErrMeg("输入内容不能为空!");
return false;
} else {
let reg = /^((https|http|ftp|rtsp|mms)?:\/\/)[^\s]+/;
if (!reg.test(this.textarea)) {
this.showErrMeg("请输入正确的file url!");
return false;
}
const fileName = this.textarea;
const fileStrArr = fileName.split(".");
const suffix = fileStrArr[fileStrArr.length - 1];
let result = fileSuffix.some((item) => {
return item === suffix;
});
if (!result) {
this.showErrMeg("不支持该文件类型");
return false;
}
}
return true;
},
clClean() {
this.textarea = "";
this.console.success("cleaned textarea value");
},
clSubmit() {
this.getViewUrlWebPath();
},
clConvert() {
if (!this.checkFileUrl()) {
return;
}
this.loading = true;
const fileUrl = {
fileUrl: this.textarea,
};
if (fileUrl) {
const params = {
taskId: this.getUUId(),
srcUri: this.textarea,
// 参数可调,详见 https://open.wps.cn/docs/doc-format-conversion/api-list
exportType: "pdf",
};
// convert
convertReq(params)
.then((res) => {
if (res.data) {
// convert res
this.loading = true;
this.getConvertRes(params.taskId);
} else {
this.showErrMeg("请求错误!");
this.loading = false;
}
})
.catch(() => {
this.showErrMeg("请求错误!");
this.loading = false;
});
}
},
getConvertRes: async function(taskId) {
const params = { taskId };
await convertRes(params).then((res) => {
this.convertRes = res.data.data;
});
if (!this.convertRes && this.convertResIndex <= 5) {
setTimeout(() => {
this.convertResIndex += 1;
this.getConvertRes(taskId);
}, 1000);
}
if (this.convertRes) {
this.loading = false;
// 下载转换结果
let a = document.createElement("a");
a.href = this.convertRes;
a.click();
}
},
getViewUrlWebPath() {
if (!this.checkFileUrl()) {
return;
}
this.loading = true;
const params = {
fileUrl: this.textarea,
};
getViewUrlWebPath(params)
.then((res) => {
if (res.data) {
let r = res.data.data;
// 跳转 使用sessionStorage,避免关键信息在ip中暴露
// 使用push会停留当前页面,故不采纳
// params 传递参数,子组件无法渲染iframe组件,故不采纳
sessionStorage.wpsUrl = r.wpsUrl;
sessionStorage.token = r.token;
const jump = this.$router.resolve({ name: "viewFile" });
window.open(jump.href, "_blank");
} else {
this.showErrMeg("请求错误!");
}
this.loading = false;
})
.catch(() => {
this.showErrMeg("请求错误!");
this.loading = false;
});
},
},
};
</script>
<style scoped>
.cl-textarea {
width: 55%;
height: 40%;
}
.template {
margin-top: 20px;
position: relative;
width: 200px;
height: 200px;
left: 50%;
transform: translateX(-100px);
}
.template-img {
width: 200px;
height: 200px;
}
.template-title {
position: absolute;
bottom: 0;
left: 0;
width: 200px;
height: 50px;
background-color: #000;
z-index: 99;
opacity: 0.7;
text-align: center;
line-height: 50px;
color: #fff;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
}
@keyframes templateon {
0% {
background-color: rgba(0, 0, 0, 0.2);
}
50% {
background-color: rgba(0, 0, 0, 0.4);
}
75% {
background-color: rgba(0, 0, 0, 0.6);
}
100% {
background-color: rgba(0, 0, 0, 0.8);
}
}
@-webkit-keyframes templateon {
0% {
background-color: rgba(0, 0, 0, 0.2);
}
50% {
background-color: rgba(0, 0, 0, 0.4);
}
75% {
background-color: rgba(0, 0, 0, 0.6);
}
100% {
background-color: rgba(0, 0, 0, 0.8);
}
}
.template-shadow {
box-sizing: border-box;
position: absolute;
top: 0;
left: 0;
width: 200px;
height: 200px;
z-index: 99;
animation: templateon .2s;
background-color: rgba(0, 0, 0, 0.8);
}
.template-shadow-title {
position: absolute;
top: 15%;
color: #fff;
width: 100%;
height: 50px;
display: flex;
justify-content: center;
align-items: center;
font-size: 12px;
box-sizing: border-box;
padding: 0 10px;
word-break: break-all;
text-overflow: ellipsis;
overflow: hidden;
display: -webkit-box;
-webkit-line-clamp: 3;
-webkit-box-orient: vertical;
}
.template-shadow-btn {
position: absolute;
top: 55%;
left: 50%;
transform: translate(-50%, -50%);
}
.template-shadow-collect {
font-size: 14px;
color: rgb(19, 150, 236);
position: absolute;
bottom: 20px;
width: 100%;
text-align: center;
letter-spacing: 2px;
cursor: pointer;
}
</style> |
// API-defined (external) interfaces are prefixed with API_ and should not
// be used outside of the WeatherAPI module.
export interface API_Response_Current {
location: API_Location;
current: API_Current;
}
interface API_Location {
name: string;
region: string;
country: string;
lat: number;
lon: number;
tz_id: string;
localtime_epoch: number;
localtime: string;
}
interface API_Current {
last_updated_epoch: number;
last_updated: string;
temp_f: number;
temp_c: number;
feelslike_f: number;
feelslike_c: number;
condition: API_Condition;
is_day: number;
// additional data is available but unused
}
interface API_Condition {
text: string;
icon: string;
code: number;
}
export interface API_Response_Forecast {
location: API_Location;
current: API_Current;
forecast: API_Forecast;
}
interface API_Forecast {
forecastday: API_ForecastDay[];
}
interface API_ForecastDay {
date: string;
date_epoch: number;
day: API_Day;
astro: API_Astro;
hour: API_Hour[];
}
interface API_Day {
maxtemp_f: number;
mintemp_f: number;
avgtemp_f: number;
maxtemp_c: number;
mintemp_c: number;
avgtemp_c: number;
daily_chance_of_rain: number;
daily_chance_of_snow: number;
condition: API_Condition;
// additional data is available but unused
}
interface API_Astro {
sunrise: string;
sunset: string;
// additional data is available but unused
}
interface API_Hour {
time_epoch: number;
time: string;
temp_f: number;
feelslike_f: number;
chance_of_rain: number;
chance_of_snow: number;
condition: API_Condition;
// additional data is available but unused
}
export type API_Response_Search = API_SearchResult[];
interface API_SearchResult {
id: number;
name: string;
region: string;
country: string;
lat: number;
lon: number;
url: string;
} |
/* eslint-disable react-refresh/only-export-components */
import { Dispatch, PropsWithChildren, SetStateAction, createContext, useContext, useMemo, useState } from "react";
import { Point3D } from "../../domain/fld/FldFile";
export interface CursorContextProps {
// state
hoveredPoint?: number;
meshPoint?: Point3D;
// functions
setHoveredPoint: Dispatch<SetStateAction<number | undefined>>;
setMeshPoint: Dispatch<SetStateAction<Point3D | undefined>>;
}
export const CursorContext = createContext<CursorContextProps | undefined>(undefined);
export const useCursorContext = (): CursorContextProps => {
const context = useContext(CursorContext);
if (!context) {
throw new Error("CursorContext not initialized");
}
return context;
};
export const CursorContextProvider: React.FC<PropsWithChildren> = ({ children }: PropsWithChildren) => {
const [hoveredPoint, setHoveredPoint] = useState<number>();
const [meshPoint, setMeshPoint] = useState<Point3D>();
const contextValue: CursorContextProps = useMemo(() => {
return { hoveredPoint, meshPoint, setHoveredPoint, setMeshPoint };
}, [hoveredPoint, meshPoint]);
return <CursorContext.Provider value={contextValue}>{children}</CursorContext.Provider>;
}; |
package pt.ipp.estg.assistenteviagens.navigation.authNavigationScreens.screens
import androidx.compose.foundation.BorderStroke
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.material.*
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.ArrowBack
import androidx.compose.material.icons.filled.Email
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.res.colorResource
import androidx.compose.ui.text.font.FontWeight
import androidx.compose.ui.text.style.TextAlign
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import androidx.lifecycle.viewmodel.compose.viewModel
import androidx.navigation.NavHostController
import androidx.navigation.compose.rememberNavController
import pt.ipp.estg.assistenteviagens.R
import pt.ipp.estg.assistenteviagens.navigation.authNavigationScreens.models.FirestoreUserViewModel
import pt.ipp.estg.assistenteviagens.navigation.authNavigationScreens.models.entity.AuthNavigationItems
import pt.ipp.estg.assistenteviagens.ui.theme.AssistenteViagensTheme
@Composable
fun ForgotScreen(navController: NavHostController) {
val firestoreUserViewModel: FirestoreUserViewModel = viewModel()
Box(
modifier = Modifier
.fillMaxWidth()
.height(56.dp)
.background(colorResource(id = R.color.color_background_Drawer)),
) {
Row(verticalAlignment = Alignment.CenterVertically) {
IconButton(onClick = { navController.navigate(AuthNavigationItems.Login.route) }) {
Icon(
Icons.Filled.ArrowBack,
contentDescription = "ArrowBackIcon",
tint = Color.Black,
modifier = Modifier
.size(40.dp)
.padding(start = 5.dp, top = 5.dp)
)
}
}
Row(
modifier = Modifier.fillMaxWidth(),
verticalAlignment = Alignment.CenterVertically
) {
Text(
text = "Forgot Password",
fontSize = 30.sp,
fontWeight = FontWeight.Bold,
modifier = Modifier.fillMaxWidth(),
textAlign = TextAlign.Center
)
}
}
Column(
modifier = Modifier
.fillMaxSize(),
verticalArrangement = Arrangement.Center
) {
Text(
text = "Reset Password",
fontSize = 18.sp,
fontWeight = FontWeight.Bold,
color = colorResource(id = R.color.color_text_login),
modifier = Modifier.align(Alignment.CenterHorizontally)
)
Spacer(modifier = Modifier.size(30.dp))
Text(
text = "Enter your email to reset Password",
fontSize = 18.sp,
fontWeight = FontWeight.Bold,
color = colorResource(id = R.color.color_text_login),
modifier = Modifier.align(Alignment.CenterHorizontally)
)
Spacer(modifier = Modifier.size(20.dp))
OutlinedTextField(
label = { Text(text = "Email Address") },
value = firestoreUserViewModel.email.value,
onValueChange = { firestoreUserViewModel.email.value = it },
leadingIcon = {
Icon(
imageVector = Icons.Default.Email,
contentDescription = "IconEmail"
)
},
shape = RoundedCornerShape(10.dp),
modifier = Modifier
.width(286.dp)
.size(60.dp)
.align(Alignment.CenterHorizontally),
)
if (firestoreUserViewModel.isLoading.value) {
CircularProgressIndicator()
} else {
Button(
modifier = Modifier
.width(200.dp)
.size(60.dp)
.align(Alignment.CenterHorizontally)
.padding(top = 15.dp),
colors = ButtonDefaults.buttonColors(backgroundColor = colorResource(id = R.color.color_buttons)),
border = BorderStroke(1.dp, Color.Black),
shape = RoundedCornerShape(10.dp),
onClick = {
firestoreUserViewModel.sendPasswordResetEmail()
navController.navigate(AuthNavigationItems.Home.route)
},
) {
Text("Send reset link")
}
}
}
}
@Preview(showBackground = true)
@Composable
fun PreviewForgotScreen() {
AssistenteViagensTheme {
val navController = rememberNavController()
ForgotScreen(navController)
}
} |
import {ComponentFixture, TestBed} from '@angular/core/testing';
import {TicketDetailsComponent} from './ticket-details.component';
import {HttpClientModule} from '@angular/common/http';
import {UiModule} from '@acme/ui';
import {ApiService} from '@acme/shared-services';
import {TicketsComponent} from '../tickets/tickets.component';
import {ActivatedRoute, RouterModule} from '@angular/router';
import {APP_BASE_HREF} from '@angular/common';
import {of, timeout} from 'rxjs';
import {DebugElement} from '@angular/core';
describe('TicketDetailsComponent', () => {
let component: TicketDetailsComponent;
let fixture: ComponentFixture<TicketDetailsComponent>;
let apiService: ApiService;
let activatedRoute: ActivatedRoute;
const fakeRouteParams = new Map();
fakeRouteParams.set('id', 13);
// A fake ticket we will use
const fakeTicket = {
id: 13,
description: 'test1',
assigneeId: 1,
completed: false,
};
// Fake ApiService
class ApiServiceStub {
ticket(id: number) {
return of(fakeTicket);
}
}
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientModule, UiModule, RouterModule.forRoot([])],
providers: [
{provide: ApiService, useValue: new ApiServiceStub()},
{
provide: ActivatedRoute,
useValue: {
// We will test route parameter with id=13
snapshot: {paramMap: fakeRouteParams}
}
},
{provide: APP_BASE_HREF, useValue: '/'}
],
declarations: [TicketsComponent],
});
fixture = TestBed.createComponent(TicketDetailsComponent);
component = fixture.componentInstance;
activatedRoute = TestBed.inject(ActivatedRoute);
});
it('should create', () => {
expect(component).toBeTruthy();
});
it('can get url param', async() => {
expect(activatedRoute.snapshot.paramMap.get('id')).toEqual(13);
});
}); |
/**
* This file is part of the LineCoverage-library.
* The file contains class for parsing and storing configuration. Uses yaml-cpp.
*
* @author Saurav Agarwal
* @contact [email protected]
* @contact [email protected]
* Repository: https://github.com/UNCCharlotte-Robotics/LineCoverage-library
*
* Copyright (C) 2020--2022 University of North Carolina at Charlotte.
* The LineCoverage-library is owned by the University of North Carolina at Charlotte and is protected by United States copyright laws and applicable international treaties and/or conventions.
*
* The LineCoverage-library is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version.
*
* DISCLAIMER OF WARRANTIES: THE SOFTWARE IS PROVIDED "AS-IS" WITHOUT WARRANTY OF ANY KIND INCLUDING ANY WARRANTIES OF PERFORMANCE OR MERCHANTABILITY OR FITNESS FOR A PARTICULAR USE OR PURPOSE OR OF NON-INFRINGEMENT. YOU BEAR ALL RISK RELATING TO QUALITY AND PERFORMANCE OF THE SOFTWARE OR HARDWARE.
*
* SUPPORT AND MAINTENANCE: No support, installation, or training is provided.
*
* You should have received a copy of the GNU General Public License along with LineCoverage-library. If not, see <https://www.gnu.org/licenses/>.
*/
#ifndef LCLIBRARY_CORE_CONFIG_H_
#define LCLIBRARY_CORE_CONFIG_H_
#include <lclibrary/core/constants.h>
#include <yaml-cpp/yaml.h>
#include <string>
#include <iostream>
#include <fstream>
#include <filesystem>
#include <cmath>
namespace lclibrary {
class Config {
public:
std::string config_file_;
struct Database {
std::string path;
std::string data_dir;
std::string dir;
bool arg = false;
} database;
std::string sol_dir;
struct Filenames {
std::string map_json;
std::string nodes_ll;
std::string nodes_data;
std::string req_edges;
std::string nonreq_edges;
} filenames;
struct InputGraph {
bool lla = true;
bool costs = false;
} input_graph;
bool convert_osm_graph = false;
bool add_pairwise_nonreq_edges = false;
struct PlotInGraph {
bool plot = false;
bool plot_nreq_edges = false;
std::string name;
} plot_input_graph;
struct WriteGeoJSON {
bool write = false;
bool non_req_edges = false;
std::string filename;
std::string var_name;
} writeGeoJSON;
std::string problem;
std::string solver_slc;
std::string solver_mlc;
std::string solver_mlc_md;
bool use_2opt;
double ilp_time_limit;
double capacity;
bool cap_arg = false;
std::string cost_function;
struct TravelTime {
double service_speed;
double deadhead_speed;
double wind_speed;
double wind_dir;
} travel_time;
struct Ramp {
double speed;
double acceleration;
} ramp;
struct TravelTimeCircTurns {
double service_speed;
double deadhead_speed;
double wind_speed;
double wind_dir;
double angular_vel;
double acc;
double delta;
} travel_time_circ_turns;
struct RouteOutput {
bool plot = false;
bool kml = false;
bool data = false;
bool edge_data = false;
bool geojson = false;
bool agg_results = false;
bool append = false;
bool clear_dir = false;
} route_output;
enum DepotMode {mean, custom, none } depot_mode;
enum DepotsMode {cluster_auto, cluster, user } depots_mode;
size_t depot_ID = 0;
std::vector <size_t> depot_IDs;
size_t num_depots;
size_t num_runs;
bool use_seed = false;
double seed = 0;
bool nd_arg = false;
Config () {}
Config (const std::string &config_file) {
config_file_ = config_file;
}
Config (const std::string &config_file, const std::string &data_dir):Config(config_file) {
database.data_dir = data_dir;
database.arg = true;
}
Config (const std::string &config_file, const std::string &data_dir, const double cap):Config(config_file, data_dir) {
capacity = cap;
cap_arg = true;
}
Config (const std::string &config_file, const std::string &data_dir, const double cap, const size_t n):Config(config_file, data_dir, cap){
num_depots = n;
nd_arg = true;
}
void CopyConfig(const std::string &filename) const {
std::filesystem::copy(config_file_, filename, std::filesystem::copy_options::overwrite_existing);
}
int WriteConfig(const std::string &filename) const {
YAML::Node yaml_config_ = YAML::LoadFile(config_file_);
if(database.arg == true) {
yaml_config_["database"]["data_dir"] = database.data_dir;
}
if(cap_arg == true) {
yaml_config_["capacity"] = capacity;
}
if(nd_arg == true) {
yaml_config_["depots"]["num_depts"] = num_depots;
}
std::ofstream fout(filename);
fout << yaml_config_;
fout.close();
return kSuccess;
}
int ParseConfig () {
std::cout << "Using config file: " << config_file_ << std::endl;
if(not std::filesystem::exists(config_file_)) {
std::cerr << "Could not find config file " << config_file_ << std::endl;
return kFail;
}
YAML::Node yaml_config_ = YAML::LoadFile(config_file_);
if(database.arg == true) {
yaml_config_["database"]["data_dir"] = database.data_dir;
}
problem = yaml_config_["problem"].as<std::string>();
if(cap_arg == true) {
yaml_config_["capacity"] = capacity;
}
if(nd_arg == true) {
yaml_config_["depots"]["num_depts"] = num_depots;
}
if(problem == "slc" or problem == "mlc") {
std::string depot_config = yaml_config_["depot"]["mode"].as<std::string>();
if(depot_config == "mean") {
depot_mode = mean;
} else if (depot_config == "custom"){
depot_mode = custom;
depot_ID = yaml_config_["depot"]["ID"].as<size_t>();
} else if (depot_config == "none") {
depot_mode = none;
}
}
if(problem == "mlc_md") {
std::string depots_config = yaml_config_["depots"]["mode"].as<std::string>();
if(depots_config == "cluster_auto") {
depots_mode = cluster_auto;
} else if (depots_config == "cluster"){
depots_mode = cluster;
num_depots = yaml_config_["depots"]["num_depots"].as<size_t>();
} else if (depots_config == "user") {
depots_mode = user;
auto yaml_depots = yaml_config_["depots"]["IDs"];
for(YAML::const_iterator it = yaml_depots.begin(); it != yaml_depots.end(); ++it) {
depot_IDs.push_back(it->as<size_t>());
}
}
use_seed = yaml_config_["depots"]["use_seed"].as<bool>();
if(use_seed) {
seed = yaml_config_["depots"]["seed"].as<double>();
}
num_runs = yaml_config_["depots"]["num_runs"].as<size_t>();
}
auto database_yaml = yaml_config_["database"];
database.path = database_yaml["path"].as<std::string>();
database.data_dir = database_yaml["data_dir"].as<std::string>();
database.dir = database.path + "/" + database.data_dir + "/";
if(not std::filesystem::exists(database.dir)) {
std::cerr << "Database does not exist\n";
std::cerr << database.dir << std::endl;
return kFail;
}
auto filenames_yaml = yaml_config_["filenames"];
filenames.map_json = filenames_yaml["map_json"].as<std::string>();
filenames.nodes_ll = filenames_yaml["nodes_ll"].as<std::string>();
filenames.nodes_data = filenames_yaml["nodes_data"].as<std::string>();
filenames.req_edges = filenames_yaml["req_edges"].as<std::string>();
filenames.nonreq_edges = filenames_yaml["nonreq_edges"].as<std::string>();
convert_osm_graph = yaml_config_["convert_osm_json"].as<bool>();
add_pairwise_nonreq_edges = yaml_config_["add_pairwise_nonreq_edges"].as<bool>();
plot_input_graph.plot = yaml_config_["plot_input_graph"]["plot"].as<bool>();
plot_input_graph.plot_nreq_edges = yaml_config_["plot_input_graph"]["plot_nreq_edges"].as<bool>();
plot_input_graph.name = yaml_config_["plot_input_graph"]["name"].as<std::string>();
input_graph.lla = yaml_config_["input_graph"]["lla"].as<bool>();
input_graph.costs = yaml_config_["input_graph"]["costs"].as<bool>();
auto writeGeoJSON_yaml = yaml_config_["writeGeoJSON"];
writeGeoJSON.write = writeGeoJSON_yaml["write"].as<bool>();
writeGeoJSON.non_req_edges = writeGeoJSON_yaml["non_req_edges"].as<bool>();
writeGeoJSON.filename = writeGeoJSON_yaml["filename"].as<std::string>();
writeGeoJSON.var_name = writeGeoJSON_yaml["var_name"].as<std::string>();
if(problem == "slc") {
solver_slc = yaml_config_["solver_slc"].as<std::string>();
sol_dir = database.dir + problem + "_" + solver_slc + "/";
}
if(problem == "mlc") {
solver_mlc = yaml_config_["solver_mlc"].as<std::string>();
sol_dir = database.dir + problem + "_" + solver_mlc + "/";
}
if(problem == "mlc_md") {
solver_mlc_md = yaml_config_["solver_mlc_md"].as<std::string>();
sol_dir = database.dir + problem + "_" + solver_mlc_md + "/";
}
use_2opt = yaml_config_["use_2opt"].as<bool>();
ilp_time_limit = yaml_config_["ilp_time_limit"].as<double>();
capacity = yaml_config_["capacity"].as<double>();
cost_function = yaml_config_["cost_function"].as<std::string>();
if(cost_function == "travel_time") {
auto travel_time_yaml = yaml_config_["travel_time_config"];
travel_time.service_speed = travel_time_yaml["service_speed"].as<double>();
travel_time.deadhead_speed = travel_time_yaml["deadhead_speed"].as<double>();
travel_time.wind_speed = travel_time_yaml["wind_speed"].as<double>();
travel_time.wind_dir = travel_time_yaml["wind_dir"].as<double>() * M_PI/180.;
}
if(cost_function == "ramp") {
auto ramp_yaml = yaml_config_["ramp_config"];
ramp.speed = ramp_yaml["speed"].as<double>();
ramp.acceleration = ramp_yaml["acceleration"].as<double>();
}
if(cost_function == "travel_time_circturns") {
auto travel_time_yaml = yaml_config_["travel_time_circturns_config"];
travel_time_circ_turns.service_speed = travel_time_yaml["service_speed"].as<double>();
travel_time_circ_turns.deadhead_speed = travel_time_yaml["deadhead_speed"].as<double>();
travel_time_circ_turns.wind_speed = travel_time_yaml["wind_speed"].as<double>();
travel_time_circ_turns.wind_dir = travel_time_yaml["wind_dir"].as<double>() * M_PI/180.;
travel_time_circ_turns.acc = travel_time_yaml["acceleration"].as<double>();
travel_time_circ_turns.angular_vel = travel_time_yaml["angular_vel"].as<double>() * M_PI/180.;
travel_time_circ_turns.delta = travel_time_yaml["delta"].as<double>();
}
auto route_output_yaml = yaml_config_["route_output"];
route_output.plot = route_output_yaml["plot"].as<bool>();
route_output.kml = route_output_yaml["kml"].as<bool>();
route_output.data = route_output_yaml["data"].as<bool>();
route_output.edge_data = route_output_yaml["edge_data"].as<bool>();
route_output.geojson = route_output_yaml["geojson"].as<bool>();
route_output.agg_results = route_output_yaml["agg_results"].as<bool>();
route_output.append = route_output_yaml["append"].as<bool>();
route_output.clear_dir = route_output_yaml["clear_dir"].as<bool>();
if(std::filesystem::exists(sol_dir)) {
if(route_output.clear_dir) {
std::filesystem::remove_all(sol_dir);
}
}
return kSuccess;
}
};
} // namespace lclibrary
#endif /* LCLIBRARY_CORE_CONFIG_H_ */ |
#ifndef _PETSC_SOLVER_H_
#define _PETSC_SOLVER_H_
#include <petscksp.h>
#include <petscdm.h>
#include <petscdmda.h>
#include "../FlowField.h"
#include "../DataStructures.h"
#include "../Parameters.h"
#include "../LinearSolver.h"
namespace nseof {
const unsigned char LEFT_WALL_BIT = 1 << 0;
const unsigned char RIGHT_WALL_BIT = 1 << 1;
const unsigned char BOTTOM_WALL_BIT = 1 << 2;
const unsigned char TOP_WALL_BIT = 1 << 3;
const unsigned char FRONT_WALL_BIT = 1 << 4;
const unsigned char BACK_WALL_BIT = 1 << 5;
/** A class to encapsulate information the Petsc builder functions
* Petsc used so called context objects to give information to its routines.
* We need them to pass the flow field and the parameters in a single argument.
*/
class PetscUserCtx {
private:
Parameters &_parameters; //! Reference to parameters
FlowField &_flowField; //! Reference to the flow field
int *_limitsX, *_limitsY, *_limitsZ;
int _rank;
public:
/** Constructor
*
* @param parameters A parameters instance
* @param flowField The corresponding flow field
*/
PetscUserCtx(Parameters ¶meters, FlowField &flowField);
/** Returns the parameters */
Parameters &getParameters();
/** Returns the flow field */
FlowField &getFlowField();
void setLimits(int *limitsX, int *limitsY, int *limitsZ);
void getLimits(int **limitsX, int **limitsY, int **limitsZ);
void setRank(int rank);
int getRank() const;
unsigned char
setAsBoundary; // if set as boundary in the linear system. Use bits
int displacement[6]; // Displacements for the boundary treatment
};
class PetscSolver : public LinearSolver {
private:
Vec _x; //! Petsc vectors for solution and RHS
DM _da; //! Topology manager
KSP _ksp; //! Solver context
PC _pc; //! Preconditioner
PetscUserCtx _ctx; // Capsule for Petsc builders
// Indices for filling the matrices and right hand side
int _limitsX[2], _limitsY[2], _limitsZ[2];
PetscInt _firstX, _lengthX, _firstY, _lengthY, _firstZ, _lengthZ;
// Additional variables used to determine where to write back the results
int _offsetX, _offsetY, _offsetZ;
public:
/** Constructor */
PetscSolver(FlowField &flowField, Parameters ¶meters);
/** Uses petsc to solve the linear system for the pressure */
void solve();
/** Returns the grid */
const DM &getGrid() const;
/** Reinit the matrix so that it uses the right flag field */
void reInitMatrix();
void init();
};
}
#endif |
//
// DailyWeatherForecastTableViewCell.swift
// WeatherApp
//
// Created by Aslan Murat on 16.06.2022.
//
import UIKit
struct DailyWeatherForecastTableViewCellViewModel {
var weekDay: String
var iconTitle: String
var dayTemp: Int
var nightTemp: Int
}
class DailyWeatherForecastTableViewCell: UITableViewCell {
static let identifier = "DailyWeatherForecastTableViewCell"
private let dayLabel: UILabel = {
let label = UILabel()
label.font = .preferredFont(forTextStyle: .body)
label.translatesAutoresizingMaskIntoConstraints = false
return label
}()
private let weatherIconImageView: UIImageView = {
let imageView = UIImageView()
imageView.translatesAutoresizingMaskIntoConstraints = false
imageView.contentMode = .center
return imageView
}()
private let dayTempLabel: UILabel = {
let label = UILabel()
label.font = .preferredFont(forTextStyle: .body)
label.translatesAutoresizingMaskIntoConstraints = false
return label
}()
private let nightTempLabel: UILabel = {
let label = UILabel()
label.font = .preferredFont(forTextStyle: .body)
label.translatesAutoresizingMaskIntoConstraints = false
label.textColor = .secondaryLabel
return label
}()
override func awakeFromNib() {
super.awakeFromNib()
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
}
override init(style: UITableViewCell.CellStyle, reuseIdentifier: String?) {
super.init(style: style, reuseIdentifier: reuseIdentifier)
contentView.addSubview(dayLabel)
contentView.addSubview(weatherIconImageView)
contentView.addSubview(dayTempLabel)
contentView.addSubview(nightTempLabel)
}
required init?(coder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
override func layoutSubviews() {
super.layoutSubviews()
let constraints = [
dayLabel.centerYAnchor.constraint(equalTo: contentView.centerYAnchor),
dayLabel.leadingAnchor.constraint(equalTo: contentView.leadingAnchor, constant: 15),
weatherIconImageView.trailingAnchor.constraint(equalTo: dayTempLabel.leadingAnchor, constant: -10),
weatherIconImageView.centerYAnchor.constraint(equalTo: contentView.centerYAnchor),
weatherIconImageView.widthAnchor.constraint(equalToConstant: contentView.frame.width * 0.45),
dayTempLabel.trailingAnchor.constraint(equalTo: nightTempLabel.leadingAnchor, constant: -10),
dayTempLabel.centerYAnchor.constraint(equalTo: contentView.centerYAnchor),
dayTempLabel.widthAnchor.constraint(equalToConstant: contentView.frame.width * 0.10),
nightTempLabel.trailingAnchor.constraint(equalTo: contentView.trailingAnchor, constant: -10),
nightTempLabel.centerYAnchor.constraint(equalTo: contentView.centerYAnchor),
nightTempLabel.widthAnchor.constraint(equalToConstant: contentView.frame.width * 0.10),
]
NSLayoutConstraint.activate(constraints)
}
func configure(with viewModel: DailyWeatherForecastTableViewCellViewModel) {
let df = DateFormatter()
df.dateFormat = "yyyy-MM-dd"
let df1 = DateFormatter()
df1.dateFormat = "EEEE"
let date = df.date(from: viewModel.weekDay) ?? Date()
let dateStr = df1.string(from: date)
dayLabel.text = dateStr
weatherIconImageView.image = Icon.init(rawValue: viewModel.iconTitle)?.image
dayTempLabel.text = String(viewModel.dayTemp)+"°"
nightTempLabel.text = String(viewModel.nightTemp)+"°"
}
} |
/**
* This program generates 250 random numbers in an array
* and allows the user to search the array for a number.
*
* By: Rodas Nega
* Version: 1.0
* Since: 2022-11-15
*/
import promptSync from 'prompt-sync'
const prompt = promptSync()
/**
* Binary Search Function.
*
* @param {number[]} numArray - all numbers to be searched through.
* @param {number} target - number being requested by the user.
* @param {number} min - lowest point in the array.
* @param {number} max - highest point in the array.
* @returns {number} the array index that matches the target.
*/
function binarySearch(
numArray: number[],
target: number,
min: number,
max: number
): number {
// base case to prevent infinite loop
if (min > max) {
return -1
}
const mid = Math.floor((min + max) / 2)
// true if target equals the matching index
if (numArray[mid] === target) {
return mid
// searches in the lower half if middle > target
} else if (numArray[mid] > target) {
return binarySearch(numArray, target, min, mid - 1)
// searches in the upper half if middle < target
} else {
return binarySearch(numArray, target, mid + 1, max)
}
}
// declares constants
const MIN = 1
const MAX = 999
const ARRAY_SIZE = 250
const randomNumArray = new Array(ARRAY_SIZE)
for (let counter = 0; counter < randomNumArray.length; counter++) {
randomNumArray[counter] = Math.floor(Math.random() * MAX + MIN)
}
randomNumArray.sort(function (a, b) {
return a - b
})
console.log('Sorted Array: ')
for (let counter = 0; counter < randomNumArray.length; counter++) {
process.stdout.write(`${String(randomNumArray[counter])}, `)
}
console.log('\n')
const numInput = Number(prompt('Enter a number to search for (0 - 999): '))
console.log(
`Your number is in index ${binarySearch(
randomNumArray,
numInput,
0,
ARRAY_SIZE - 1
)}.`
)
console.log('\nDone.') |
# ESLint 警告是一种反模式
> 原文:<https://levelup.gitconnected.com/eslint-warnings-are-an-anti-pattern-78eb93aa9928>

斯科特·罗杰斯在 [Unsplash](https://unsplash.com?utm_source=medium&utm_medium=referral) 上拍摄的照片
ESLint 为任何给定的规则提供了三种设置:`error`、`warn`和`off`。`error`设置将使 ESLint 在遇到任何违反规则的情况下失败,`warn`设置将使 ESLint 报告发现的问题,但在遇到任何违反规则的情况下不会失败,`off`设置禁用规则。
我想说使用`warn`是一种反模式。为什么?因为要么你在乎什么,要么你不在乎。规则要么很重要,应该遵守,违反规则的地方应该修复,要么规则不重要,开发人员不应该担心。因此,使用`error`或`off`。
## 警告的问题是
现在,使用`warn`设置没有真正的问题。但问题是,当违反 ESLint 规则的行为没有得到执行时,开发人员往往会忽略它们。这意味着警告将堆积在 ESLint 输出中,产生大量噪声和混乱。
所以,你在乎那些被违反的规则吗?如果没有,为什么要启用该规则?如果一个规则没有任何用处,开发人员没有处理警告,那么就放弃这个规则。如果规则很重要,就把它设置为`error`,这样开发者就不会忽略它。
## 一个警告
我认为`warn`设置有一个用例是有效的,所以让我们来解决它。记住,只有西斯才处理绝对的事情。
当向您的代码库引入一个新的 ESLint 规则时,您可能会发现有太多的违规情况需要一次解决。在这种情况下,你该怎么办?您希望让您的 ESLint 脚本通过,特别是如果您在 CI 管道中强制执行它(您应该这样做!).为每一个违反该规则的行为添加一个`eslint-disable-next-line`注释和一个`TODO`注释可能会适得其反。
因此,如果您正在添加一个新的 ESLint 规则,并且发现由于某种原因您不能一次清除所有的违规,那么将新规则设置为`warn`,至少现在是这样。请理解,这是一个临时设置,您的目标应该是尽快清除警告。然后,一旦处理了违反规则的情况,您就可以将规则设置更改为`error`。
# 结论
ESLint 警告是一种反模式。仅使用`error`或`off`设置,保留使用`warn`设置仅作为临时权宜之计。
# 分级编码
```
Thanks for being a part of our community! More content in the [Level Up Coding publication](https://levelup.gitconnected.com/).Follow: [Twitter](https://twitter.com/gitconnected), [LinkedIn](https://www.linkedin.com/company/gitconnected), [Newsletter](https://newsletter.levelup.dev/)Level Up is transforming tech recruiting 👉 [**Join our talent collective**](https://jobs.levelup.dev/talent/welcome?referral=true)
``` |
import { View, Text, StyleSheet, TouchableOpacity, TextInput, ScrollView, Image } from 'react-native'
import React, { useState } from 'react'
import Animated, { FadeIn, FadeInDown, FadeInUp, FadeOutDown, FadeOutUp } from 'react-native-reanimated'
import { Ionicons } from '@expo/vector-icons'
import Colors from '@/constants/Colors'
import { places } from '@/assets/data/places'
import { bookingStyles } from '@/constants/Styles'
const AnimatedTouchableOpacity = Animated.createAnimatedComponent(TouchableOpacity)
interface Props {
openCard: number
setOpenCard: React.Dispatch<React.SetStateAction<number>>
selectedPlace: number
setSelectedPlace: React.Dispatch<React.SetStateAction<number>>
}
const Where = ({ openCard, setOpenCard, selectedPlace, setSelectedPlace }: Props) => {
return (
<View style={bookingStyles.card} >
{openCard != 0 ? (
<AnimatedTouchableOpacity onPress={() => setOpenCard(0)}
style={bookingStyles.cardPreview}
entering={FadeInUp.duration(150)}
exiting={FadeOutDown.duration(150)}
>
<Text style={bookingStyles.previewText}>Where</Text>
<Text style={bookingStyles.previewdData}>I'm flexible</Text>
</AnimatedTouchableOpacity>
) : (
<Animated.View style={bookingStyles.cardBody} entering={FadeInDown.duration(150)} exiting={FadeOutUp.duration(150)}>
<View style={{ paddingHorizontal: 20 }}>
<Text style={bookingStyles.cardHeader}>Where to?</Text>
<View style={bookingStyles.searchSection}>
<Ionicons style={bookingStyles.searchIcon} name='ios-search' size={20} />
<TextInput
style={bookingStyles.inputField}
placeholder='Search destination'
placeholderTextColor={Colors.grey}
/>
</View>
</View>
<ScrollView
horizontal
showsHorizontalScrollIndicator={false}
contentContainerStyle={{ gap: 25, paddingHorizontal: 20 }}
>
{places.map((item, index) => (
<TouchableOpacity key={index} onPress={() => setSelectedPlace(index)}>
<Image source={item.img} style={selectedPlace === index ? bookingStyles.placeSelected : bookingStyles.place} />
<Text
style={[
{ paddingTop: 6, textAlign: 'center' },
selectedPlace === index ? { fontFamily: 'mon-sb' } : { fontFamily: 'mon' }
]}>
{item.title}
</Text>
</TouchableOpacity>
))}
</ScrollView>
</Animated.View>
)
}
</View >
)
}
export default Where |
package com.example.alarm.domain.alarmManagerRing
import android.Manifest
import android.app.PendingIntent
import android.content.BroadcastReceiver
import android.content.Context
import android.content.Intent
import android.content.pm.PackageManager
import android.media.AudioManager
import android.media.RingtoneManager
import android.net.Uri
import android.util.Log
import androidx.core.app.ActivityCompat
import androidx.core.app.NotificationCompat
import androidx.core.app.NotificationManagerCompat
import com.example.alarm.R
import com.example.alarm.data.Constants
import com.example.alarm.data.local.Alarm
import com.example.alarm.di.App
import com.example.alarm.domain.repositorys.AlarmRepository
import dagger.hilt.android.AndroidEntryPoint
import kotlinx.coroutines.CoroutineScope
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.launch
import javax.inject.Inject
@AndroidEntryPoint
class BroadcastForAlarm : BroadcastReceiver() {
private lateinit var app:App
private val tag:String = "BroadcastForAlarm"
private lateinit var alarm:Alarm
@Inject
lateinit var repo: AlarmRepository
private val coroutineScope= CoroutineScope(Dispatchers.IO)
override fun onReceive(context: Context?, intent: Intent?) {
val ringtoneUriString = intent?.getStringExtra(Constants.IntentAlarm)
val alarmId = intent?.getStringExtra(Constants.IntentAlarmId)?.toInt()
Log.d(tag,"onHandleIntent: 1111111111111111111111111 $alarmId" )
try {
coroutineScope.launch {
if (alarmId != null) {
// get alarm from database
alarm = repo.getAlarm(alarmId)
}
}
// Parse the ringtone Uri string to a Uri
val ringtoneUri: Uri = Uri.parse(ringtoneUriString)
Log.d(tag,"onHandleIntent: 222222222222222222222222" )
// Now you have a Uri object representing the ringtone
// You can use this Uri with RingtoneManager to get a Ringtone instance if needed
app = context?.applicationContext as App
app.ringtone = RingtoneManager.getRingtone(context, ringtoneUri)
} catch (e: Exception) {
// Handle any parsing or other exceptions that may occur
Log.d(tag,"onHandleIntent: 3333333333333333333333333333" )
}
if(context != null && ringtoneUriString != null && alarmId != null){
// Get the AudioManager
val audioManager = context.getSystemService(Context.AUDIO_SERVICE) as AudioManager
// Set the volume level for the ringtone stream to maximum
val maxVolume = audioManager.getStreamMaxVolume(AudioManager.STREAM_RING)
audioManager.setStreamVolume(AudioManager.STREAM_RING, maxVolume, 0)
// start notification and sound
createNotification(context,alarm)
}
// if (ringtoneUriString!=null){
// val window = Window(context, ringtoneUriString,alarmId,repo)
// window.open()
// }
}
// this notification with actions
private fun createNotification(context: Context, alarm: Alarm) {
var newHour = if(alarm.hour > 12) alarm.hour - 12 else alarm.hour
if (newHour == 0){
newHour = 12
}
val amOrPm = if(alarm.hour > 12)"PM" else "AM"
// Create two actions
val action1Intent = Intent(context, OffAlarmReceiver::class.java)
action1Intent.putExtra(Constants.IntentAlarmId,alarm.id.toString())
val action1PendingIntent = PendingIntent.getBroadcast(context, alarm.id, action1Intent,
PendingIntent.FLAG_IMMUTABLE)
val action2Intent = Intent(context, SnoozeAlarmReceiver::class.java)
action1Intent.putExtra(Constants.IntentAlarmId,alarm.id.toString())
val action2PendingIntent = PendingIntent.getBroadcast(context, alarm.id, action2Intent,
PendingIntent.FLAG_IMMUTABLE)
// Start the ringtone
app.ringtone?.play()
app.ringtone?.isLooping = true
app.ringtone?.volume
val notification = NotificationCompat.Builder(context, Constants.channelId)
.setOngoing(true)
.setSmallIcon(R.mipmap.ic_launcher)
.setContentTitle("Alarm Clock")
.setContentText("Alarm ${newHour}:${alarm.minute} $amOrPm Ring Now")
.setVisibility(NotificationCompat.VISIBILITY_PUBLIC)
.setPriority(NotificationCompat.PRIORITY_HIGH)
.setCategory(NotificationCompat.CATEGORY_RECOMMENDATION)
.addAction(R.drawable.baseline_snooze_24, "Snooze", action2PendingIntent)
.addAction(R.drawable.baseline_alarm_off_24, "Off", action1PendingIntent)
.build()
val notificationManager = NotificationManagerCompat.from(context)
if (ActivityCompat.checkSelfPermission(
context,
Manifest.permission.POST_NOTIFICATIONS
) != PackageManager.PERMISSION_GRANTED
) { return }
notificationManager.notify(1, notification)
}
} |
import DataLoader from 'dataloader'
import pLimit from 'p-limit'
import type { Options } from '..'
import type { Clients } from '../clients'
import type {
ShippingItem,
Simulation,
SimulationArgs,
} from '../clients/commerce/types/Simulation'
// Limits concurrent requests to the API per request cycle
const CONCURRENT_REQUESTS_MAX = 1
export const getSimulationLoader = (_: Options, clients: Clients) => {
const limit = pLimit(CONCURRENT_REQUESTS_MAX)
const loader = async (simulationArgs: readonly SimulationArgs[]) => {
const allItems = simulationArgs.reduce((acc, { items }: SimulationArgs) => {
return [...acc, items]
}, [] as ShippingItem[][])
const items = [...allItems.flat()]
const simulation = await clients.commerce.checkout.simulation({
country: simulationArgs[0].country,
postalCode: simulationArgs[0].postalCode,
items,
})
// Sort and filter simulation since Checkout API may return
// items that we didn't ask for
const simulated = simulation.items.reduce((acc, item) => {
const index = item.requestIndex
if (typeof index === 'number' && index < acc.length) {
acc[index] = item
}
return acc
}, Array(items.length).fill(null) as Simulation['items'])
const itemsIndices = allItems.reduce(
(acc, curr) => [...acc, curr.length + acc[acc.length - 1]],
[0]
)
return allItems.map((__, index) => ({
...simulation,
items: simulated
.slice(itemsIndices[index], itemsIndices[index + 1])
.filter((item) => Boolean(item)),
}))
}
const limited = async (allItems: readonly SimulationArgs[]) =>
limit(loader, allItems)
return new DataLoader<SimulationArgs, Simulation>(limited, {
maxBatchSize: 50,
})
} |
// ignore_for_file: unnecessary_getters_setters
import '/backend/schema/util/schema_util.dart';
import 'index.dart';
import '/flutter_flow/flutter_flow_util.dart';
class ConversationStruct extends BaseStruct {
ConversationStruct({
String? question,
String? answer,
String? audioUrl,
}) : _question = question,
_answer = answer,
_audioUrl = audioUrl;
// "question" field.
String? _question;
String get question => _question ?? '';
set question(String? val) => _question = val;
bool hasQuestion() => _question != null;
// "answer" field.
String? _answer;
String get answer => _answer ?? '';
set answer(String? val) => _answer = val;
bool hasAnswer() => _answer != null;
// "audioUrl" field.
String? _audioUrl;
String get audioUrl => _audioUrl ?? '';
set audioUrl(String? val) => _audioUrl = val;
bool hasAudioUrl() => _audioUrl != null;
static ConversationStruct fromMap(Map<String, dynamic> data) =>
ConversationStruct(
question: data['question'] as String?,
answer: data['answer'] as String?,
audioUrl: data['audioUrl'] as String?,
);
static ConversationStruct? maybeFromMap(dynamic data) =>
data is Map<String, dynamic> ? ConversationStruct.fromMap(data) : null;
Map<String, dynamic> toMap() => {
'question': _question,
'answer': _answer,
'audioUrl': _audioUrl,
}.withoutNulls;
@override
Map<String, dynamic> toSerializableMap() => {
'question': serializeParam(
_question,
ParamType.String,
),
'answer': serializeParam(
_answer,
ParamType.String,
),
'audioUrl': serializeParam(
_audioUrl,
ParamType.String,
),
}.withoutNulls;
static ConversationStruct fromSerializableMap(Map<String, dynamic> data) =>
ConversationStruct(
question: deserializeParam(
data['question'],
ParamType.String,
false,
),
answer: deserializeParam(
data['answer'],
ParamType.String,
false,
),
audioUrl: deserializeParam(
data['audioUrl'],
ParamType.String,
false,
),
);
@override
String toString() => 'ConversationStruct(${toMap()})';
@override
bool operator ==(Object other) {
return other is ConversationStruct &&
question == other.question &&
answer == other.answer &&
audioUrl == other.audioUrl;
}
@override
int get hashCode => const ListEquality().hash([question, answer, audioUrl]);
}
ConversationStruct createConversationStruct({
String? question,
String? answer,
String? audioUrl,
}) =>
ConversationStruct(
question: question,
answer: answer,
audioUrl: audioUrl,
); |
<script>
import Color from "dojo/_base/Color";
import touch from "dojo/touch";
import on from "dojo/on";
import css from "dojo/css";
import lang from "dojo/_base/lang";
export default {
data: function() {
return {
colors_75: ["#F4BF00", "#FF1A00"],
colors_50: ["#ff9105", "#ffbd21"],
colors_25: ["#00A4E0", "#99cc00"],
colors_0: ["#0099cc", "#2cb1e1"],
redToYellow: ["#ff4444", "#ffae18"],
yellowToGreen: ["#ffae18", "#83b600"],
greenToGreen: ["#b6db49", "#75a800"],
mini_palette: [
"#ffffff", "#808080", "#000000",
"#e2f4fb", "#33b5e5", "#0099cc",
"#e5caf2", "#c182e0", "#9933cc",
"#e2f0b6", "#92c500", "#669900",
"#ffecc0", "#ffb61c", "#ff8a00",
"#ffcaca", "#f83a3a", "#cc0000"],
shadow_palette: [
"#ffffff", "rgba(0,0,0,0.25)", "rgba(0,0,0,0.5)",
"#e2f4fb", "#33b5e5", "#0099cc",
"#e5caf2", "#c182e0", "#9933cc",
"#e2f0b6", "#92c500", "#669900",
"#ffecc0", "#ffb61c", "#ff8a00",
"#ffcaca", "#f83a3a", "#cc0000"]
};
},
methods: {
mixColor(value) {
if (value < 0.25) {
let color = Color.blendColors(
new Color(this.colors_0[0]),
new Color(this.colors_0[1]),
value
);
return color.toHex();
} else if (value < 0.5) {
let color = Color.blendColors(
new Color(this.colors_25[0]),
new Color(this.colors_25[1]),
value
);
return color.toHex();
} else if (value < 0.75) {
let color = Color.blendColors(
new Color(this.colors_50[0]),
new Color(this.colors_50[1]),
value
);
return color.toHex();
} else {
let color = Color.blendColors(
new Color(this.colors_75[0]),
new Color(this.colors_75[1]),
value
);
return color.toHex();
}
},
greenToRed(value) {
if (value < 0.75) {
let color = Color.blendColors(
new Color(this.redToYellow[0]),
new Color(this.redToYellow[1]),
this.getPercentage(value, 0, 0.75)
);
return color.toHex();
} else {
let color = Color.blendColors(
new Color(this.greenToGreen[0]),
new Color(this.greenToGreen[1]),
this.getPercentage(value, 0.75, 1)
);
return color.toHex();
}
},
getPercentage(value, from, to) {
var dif = to - from;
var offSet = value - from;
return offSet / dif;
},
renderColorBoxes(colors, parent, columns, callback) {
var colorBoxes = {};
var table = document.createElement("table");
var tbody = document.createElement("tbody");
table.appendChild(tbody);
var tr = null;
for (var i = 0; i < colors.length; i++) {
if (i % columns == 0 || tr == null) {
tr = document.createElement("tr");
tbody.appendChild(tr);
}
var color = colors[i];
var td = document.createElement("td");
css.add(td, "MatcColorBox MatcColorBox" + (i % columns));
var span = document.createElement("span");
span.style.backgroundColor = color;
if (this.hasAlpha(color)) {
css.add(span, "MatcColorAlphaBox");
}
colorBoxes[color] = span;
this.tempOwn(on(span, touch.press, lang.hitch(this, callback, color)));
td.appendChild(span);
tr.appendChild(td);
}
parent.appendChild(table);
return colorBoxes;
},
hasAlpha (color) {
if (color.indexOf('0.') > 0) {
return true
}
return false
}
}
};
</script> |
<!DOCTYPE html>
<html lang="pt-br">
<head>
<!--fontes do google-->
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Angkor&display=swap" rel="stylesheet">
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name="description" content="Metodologias ágeis e scrum">
<meta name="keywords" content="ágil, scrum">
<meta name="author" content="Tati Muniz">
<title>Metodologia software ágil</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<!--Cabeçario-->
<header>
<!--barra de navegação-->
<nav>
<a href="index.html">Home</a> |
<a href="">Noticias</a> |
<a href="">Esporte</a> |
<a href="">Tecnologia</a>
</nav>
<h1>Metodologias ágeis de software</h1>
</header>
<!--Seção 1-->
<section>
<main>
<h2 class="subtitulo">*O que são metodologias ágeis de software?</h2>
<p class="texto">Metodologia ágil é um conjunto de técnicas e práticas para gestão de projetos
que oferece mais rapidez, eficiência e flexibilidade. Seu objetivo inicial era agilizar
o desenvolvimento de softwares, mas esses métodos extrapolaram o setor de tecnologia e
hoje revolucionam a gestão de empresas de todas as áreas.</p>
<figure>
<img src="metodologia-ágil.png" alt="analise de desenvolvimento">
<figcaption class="legenda">Pessoas analisando e discutindo o desenvolvimento de um gráfico</figcaption>
</figure>
</main>
</section>
<!--Seção 2-->
<section>
<!--artigo1-->
<article>
<h2>*A metodologia ágil SCRUM</h2>
<p class="verde">Scrum é uma metodologia ágil desenvolvida para potencializar equipes
no ambiente de trabalho.<br>
O Scrum se baseia em princípios que podem ser encontrados dentro do Manifesto Ágil,
um manifesto<br> criado por 17 autores que discorrem sobre a utilização de ferramentas,
contratos e planos para o sucesso<br> de um projeto.<br>
Inicialmente, o método foi desenvolvido com o objetivo de atender demandas de empresas
de software,<br> mas, hoje em dia, tem se difundido em empresas de diversos segmentos.</p>
</article>
<!--artigo 2-->
<article>
<h2 id="tit1">*Reunião diaria SCRUM</h2>
<p>Uma reunião de daily Scrum, também conhecida como reunião diária em pé (daily standup)
ou carinhosamente<br> apelidada como "daily”, é um evento com duração de 15 minutos por times
que seguem metodologias Agile<br> para discutir o que será feiro no dia. Como o nome sugere,
ela acontece todos os dias durante um sprint.</p>
</article>
</section>
<aside>
<a href="https://neilpatel.com/br/blog/metodologia-agil/">Metodologia ágeis de software</a> <br>
<a href="https://www.alura.com.br/empresas/artigos/scrum">Metodologia ágil SCRUM</a> <br>
<a href="https://miro.com/pt/agile/o-que-e-reuniao-daily-scrum/">Reunião diaria SCRUM</a><br>
</aside>
<!--rodapé--> <br>
<footer>Tati Muniz </footer>
<!--link para o arquivo JS-->
<script src="script.js"></script>
</body>
</html> |
import Diabetes from "@/components/Diabetes";
import Footer from "@/components/Footer";
import Navbar from "@/components/Navbar";
const About = () => {
return (
<>
<Navbar />
<section id="about">
<div className="container-lg">
<div className="row justify-content-center align-items-center">
<h1>About</h1>
<p>
The Female Diabetes Predictor website is a web application
developed as a final semester project for the Machine Learning and
Web Services module of GDSE in IJSE. The objective of the project
is to provide a predictive model for assessing the risk of
diabetes in females based on various input parameters. The website
comprises two main components: the back-end and the front-end.
</p>
<ul>
<li>
<strong>Back-end:</strong>
<dl>
The back-end of the website is built using Flask, a Python web
framework. It is hosted on an AWS EC2 instance, which offers
reliable and scalable compute resources in the cloud. The
back-end receives input data from the front-end, typically
through HTTP requests. The data includes parameters such as
age, BMI (Body Mass Index), blood pressure, insulin levels,
and other relevant features. This data is then processed and
passed through a machine learning model. The machine learning
model used in the back-end is trained on a dataset containing
historical data related to diabetes in females. The model
applies appropriate algorithms to analyze the input data and
generate a prediction regarding the likelihood of diabetes.
Once the prediction is obtained, the back-end sends the result
back to the front-end as a response to the initial request.
This prediction can be in the form of a binary classification
(e.g., high risk or low risk) or a numerical value
representing the probability of having diabetes.
</dl>
</li>
<li>
<strong>Front-end:</strong>
<dl>
The front-end of the website is developed using Next.js, a
React-based framework for server-rendered React applications.
Bootstrap, a popular CSS framework, is utilized for styling
and responsive design. The front-end is hosted on Hostinger, a
web hosting service. Users access the website through their
web browsers, interacting with the intuitive user interface
presented by the front-end. They enter their personal
information and health parameters into the input form. The
front-end then sends this data to the back-end for processing
and prediction. The prediction result received from the
back-end is displayed to the user, providing an assessment of
their diabetes risk based on the input provided. The front-end
ensures an engaging and user-friendly experience, making it
easy for individuals to understand and interpret the
prediction.
</dl>
</li>
</ul>
<p>
By integrating a machine learning model into the back-end and
presenting the prediction through the front-end, the Female
Diabetes Predictor website empowers users to gain insights into
their potential diabetes risk. This project showcases the fusion
of machine learning concepts, web development technologies, and
cloud hosting services to create an impactful application aimed at
assisting individuals in assessing their health condition.
</p>
<div className="mt-3">
<strong>Vimukthi Bandaragoda</strong>
<br />
<strong>GDSE 54</strong>
<br />
<strong>2019/G/1037</strong>
</div>
</div>
</div>
</section>
<Footer />
</>
);
};
export default About; |
public class B01_Operator01 {
/*
# 연산자 (Operator)
- 계산할 때 값 사이에 넣어서 쓰는 것
- +, -, *, /, <, &, ...
# 산술 연산자
+ : 더하기
- : 빼기
* : 곱하기
/ : 나누기
※ 정수끼리 나무면 몫만 구하고
실수가 포함되어 있으면 정확한 결과를 구한다
※ 정수끼리의 산술 연산 결과는 정수이고
정수와 실수의 산술 연산 결과는 실수다
% : 나머지
*/
public static void main(String[] args) {
int a = 10;
int b = 7;
double c = 7.0;
// 문자열과 숫자를 더하면 결과는 문자열
// (a + b) 형태로 해야 원하는 결과(정수값 더하기)가 나옴
System.out.println("a + b: " + (a + b));
System.out.println("a - b: " + (a - b));
System.out.println("a * b: " + (a * b));
System.out.println("a / b: " + (a / b));
System.out.println("a % b: " + (a % b));
// n으로 나눈 나머지 연산의 결과는 0 ~ (n-1) 이다.
System.out.println(10 % 5);
System.out.println(11 % 5);
System.out.println(12 % 5);
System.out.println(13 % 5);
System.out.println(14 % 5);
System.out.println(15 % 5);
// char 타입은 중수를 문자로 보여주는 것처럼
// double/float 타입은 정수를 .0을 붙여 보여준다
// 정수와 실수의 연산 결과 확인
System.out.println("a + c: " + (a + c));
System.out.println("a - c: " + (a - c));
System.out.println("a * c: " + (a * c));
System.out.println("a / c: " + (a / c));
System.out.println("a % c: " + (a % c));
// Math.pow(a, b): a 제곱 b의 결과를 반환
// 결과는 double 타입으로 나옴
double result = Math.pow(2, 10);
System.out.println("pow의 결과: " + result);
// Math.sqrt(a) : a의 제곱근을 반환 => √(root)
double result2 = Math.sqrt(25);
System.out.println("sqrt의 결과: " + result2);
// Math.abs(a) : a의 절댓값 반환
// 파라미터 타입에 따라 리턴 타입이 결정됨
// => Java에는 template 개념이 없나?
int abs1 = Math.abs(-7);
float abs2 = Math.abs(-1.23f);
double abs3 = Math.abs(-2.34);
System.out.println(abs1);
System.out.println(abs2);
System.out.println(abs3);
// Math.round(a) : a를 소수 첫 째 자리에서 반올림
// => 실수를 정수로 만듦
double roundValue = 123.456;
double roundResult = Math.round(roundValue);
System.out.printf("[Math.round()] roundValue: %f, roundResult: %f \n",
roundValue, roundResult);
// Math.ceil(a) : a를 소수 첫 째 자리에서 강제 올림
double ceilValue = 123.111;
double ceilResult = Math.ceil(ceilValue);
System.out.printf("[math.ceil()] ceilValue: %f, ceilResult: %f \n",
ceilValue, ceilResult);
// Math.floor(a) : a를 소수 첫 째 자리에서 강제 내림
double floorValue = 123.111;
double floorResult = Math.ceil(floorValue);
System.out.printf("[Math.ceil()] floorValue: %f, floorResult: %f \n",
floorValue, floorResult);
/*
# 원하는 자리에서 반올림 하는 방법
1. 반올림하고 싶은 자리를 소수 첫 번째 자리로 만듦
2. 반올림하고 다시 원래대로 돌림
*/
double value = 123.123456;
double unit = Math.pow(10, 4);
System.out.printf("[원하는 자리 반올림] value: %f, unit: %f \n", value, unit);
System.out.println(value * unit);
System.out.println(Math.round(value * unit));
System.out.println(Math.round(value * unit) / unit);
System.out.println("연습1 : 12500을 반올림하여 13000으로 만드시오");
double dbl1Value= 12500;
double dbl1Result = Math.round(dbl1Value / 1000) * 1000;
int int1Result = (int)dbl1Result;
System.out.printf(">> dbl1Value: %f, dbl1Result: %f, int1Result: %d \n",
dbl1Value, dbl1Result, int1Result);
System.out.println("연습2 : 1.138을 내림하여 1.13으로 만드시오");
double dbl2Value = 1.138;
double dbl2Result = Math.floor(dbl2Value * 100) / 100;
System.out.printf(">> dbl2Value: %f, dbl2Result: %f \n",
dbl2Value, dbl2Result);
}
}
/*
a + b: 17
a - b: 3
a * b: 70
a / b: 1
a % b: 3
0
1
2
3
4
0
a + c: 17.0
a - c: 3.0
a * c: 70.0
a / c: 1.4285714285714286
a % c: 3.0
pow의 결과: 1024.0
sqrt의 결과: 5.0
7
1.23
2.34
[Math.round()] roundValue: 123.456000, roundResult: 123.000000
[math.ceil()] ceilValue: 123.111000, ceilResult: 124.000000
[Math.ceil()] floorValue: 123.111000, floorResult: 124.000000
[원하는 자리 반올림] value: 123.123456, unit: 10000.000000
1231234.56
1231235
123.1235
연습1 : 12500을 반올림하여 13000으로 만드시오
>> dbl1Value: 12500.000000, dbl1Result: 13000.000000, int1Result: 13000
연습2 : 1.138을 내림하여 1.13으로 만드시오
>> dbl2Value: 1.138000, dbl2Result: 1.130000
*/ |
import { Routes } from '@angular/router';
import { LoginComponent } from "./core/auth/login/login.component";
import { DashboardComponent } from "./features/dashboard/dashboard.component";
import {ErrorComponent} from "./core/error/error.component";
import {SalesComponent} from "./features/sales/sales.component";
import {AccountingComponent} from "./features/accounting/accounting.component";
import {ContactsComponent} from "./features/contacts/contacts.component";
import {RegisterComponent} from "./core/auth/register/register.component";
import {ForgotPwdComponent} from "./core/auth/forgot-pwd/forgot-pwd.component";
import { AuthGuard } from "./core/auth/authGuard";
import {ResetPwdComponent} from "./core/auth/reset-pwd/reset-pwd.component";
import {TeamManagementComponent} from "./features/team-management/team-management.component";
import {SettingsComponent} from "./features/settings/settings.component";
import {ProductComponent} from "./features/sales/product/product.component";
import {CreateSalesComponent} from "./features/sales/create-sales/create-sales.component";
import {CreateProductComponent} from "./features/sales/product/create-product/create-product.component";
import {EditProductComponent} from "./features/sales/product/edit-product/edit-product.component";
import {CanDeactivateCreateInvoiceGuard} from "./features/sales/create-sales/CanDeactivateCreateInvoiceGuard";
import {InvoiceDisplayComponent} from "./features/sales/invoice-display/invoice-display.component";
import {AccountingCreateComponent} from "./features/accounting/accounting-create/accounting-create.component";
import {
AccountingCreateCashComponent
} from "./features/accounting/accounting-create/cash/accounting-create-cash.component";
import {
AccountingCreateCreditComponent
} from "./features/accounting/accounting-create/credit/accounting-create-credit.component";
export const routes: Routes = [
{ path: '', component: DashboardComponent, canActivate: [AuthGuard] },
{ path: 'dashboard', component: DashboardComponent, canActivate: [AuthGuard] },
{ path: 'login', component: LoginComponent },
{ path: 'sales', component: SalesComponent, canActivate: [AuthGuard] },
{ path: 'accounting', component: AccountingComponent, canActivate: [AuthGuard] },
{ path: 'accounting/create', component: AccountingCreateComponent, canActivate: [AuthGuard] },
{ path: 'accounting/create/cash/:id', component: AccountingCreateCashComponent, canActivate: [AuthGuard] },
{ path: 'accounting/create/credit/:id', component: AccountingCreateCreditComponent, canActivate: [AuthGuard] },
{ path: 'contacts', component: ContactsComponent, canActivate: [AuthGuard] },
{ path: 'register', component: RegisterComponent},
{ path: 'forgot-password', component: ForgotPwdComponent},
{ path: 'reset-password/:reset-token', component: ResetPwdComponent},
{ path: 'team-management', component: TeamManagementComponent, canActivate: [AuthGuard] },
{ path: 'settings', component: SettingsComponent, canActivate: [AuthGuard] },
{ path: 'sales/product',component: ProductComponent, canActivate: [AuthGuard] },
{ path: 'sales/product/create', component: CreateProductComponent, canActivate: [AuthGuard] },
{ path: 'sales/product/edit/:productId', component: EditProductComponent, canActivate: [AuthGuard] },
{ path: 'sales/create/:invoiceId', component: CreateSalesComponent, canActivate: [AuthGuard], canDeactivate: [CanDeactivateCreateInvoiceGuard] },
{ path: 'sales/invoice/:invoiceId', component: InvoiceDisplayComponent, canActivate: [AuthGuard] },
{ path: '**', component: ErrorComponent},
]; |
struct Node {
Node *links[26];
bool flag = false;
bool containsKey(char ch)
{
return (links[ch - 'a'] != NULL);
}
void put(char ch, Node* node)
{
links[ch - 'a'] = node;
}
Node* get(char ch)
{
return (links[ch - 'a']);
}
void setEnd()
{
flag = true;
}
bool isEnd()
{
return flag;
}
};
class Trie {
private:
Node* root;
public:
Trie() {
root = new Node();
}
// T.C = O(N) - length of the word
void insert(string word) {
Node* node = root;
for(int i = 0; i < word.size(); i++)
{
if(!node->containsKey(word[i]))
{
node->put(word[i], new Node());
}
// moves to the reference trie
node = node->get(word[i]);
}
node->setEnd();
}
bool search(string word) {
Node* node = root;
for(int i = 0; i < word.size(); i++)
{
if(!node->containsKey(word[i]))
return false;
node = node->get(word[i]);
}
return (node->isEnd());
}
bool startsWith(string prefix) {
Node* node = root;
for(int i = 0; i < prefix.size(); i++)
{
if(!node->containsKey(prefix[i]))
return false;
node = node->get(prefix[i]);
}
return true;
}
};
/**
* Your Trie object will be instantiated and called as such:
* Trie* obj = new Trie();
* obj->insert(word);
* bool param_2 = obj->search(word);
* bool param_3 = obj->startsWith(prefix);
*/ |
import { cn } from '@/lib/cn';
import { useTranslation } from 'react-i18next';
import { Props } from '.';
import Chip from './Chip';
const unitToSymbol: Record<string, string> = {
USD: '$',
TRY: '₺',
};
function Chips({ service, className }: Props): React.ReactElement {
const { t } = useTranslation('common', {
keyPrefix: 'recommendation-grid',
});
const fmtPrice = `${service.price}${unitToSymbol[service.priceUnit]}`;
return (
<div className={cn('flex flex-wrap justify-end gap-2', className)}>
<Chip
type="price"
text={t('price', { price: fmtPrice })}
/>
{service.isPopular && (
<Chip
type="topRated"
text={t('top-rated')}
/>
)}
{service.isNew && !service.isPopular && (
<Chip
type="new"
text={t('new')}
/>
)}
{service.user.isVerifiedAccount &&
!service.isPopular &&
!service.isNew && (
<Chip
type="pro"
text={t('pro')}
/>
)}
</div>
);
}
export default Chips; |
import React, { useEffect, useState } from 'react'
import { useDispatch } from 'react-redux';
import { useSelector } from 'react-redux';
import { uiCloseModal } from '../../actions/ui';
import {customStyles} from './customStyles';
import Modal from 'react-modal';
import DateTimePicker from 'react-datetime-picker';
import moment from 'moment'
import Swal from 'sweetalert2';
import { eventClearActiveEvent, eventStartAddNew, startEventUpdate } from '../../actions/calendar';
Modal.setAppElement('#root');
const startDate = moment().minutes(0).seconds(0).add(1,"hours");
const endDate = startDate.clone().add(1,"hours")
const initEvent = {
title: '',
notes: '',
start: startDate.toDate(),
end: endDate.toDate()
}
export const CalendarModal = () => {
const [dateStart, setDateStart] = useState(startDate.toDate());
const [dateEnd, setDateEnd] = useState(endDate.toDate());
const [titleValid, setTitleValid] = useState(true);
const dispatch = useDispatch();
const {modalOpen} = useSelector(state => state.ui)
const {activeEvent} = useSelector(state => state.calendar)
const [formValues, setFormValues] = useState(initEvent)
const {title, notes, start, end} = formValues;
useEffect(() => {
if (activeEvent){
setFormValues(activeEvent)
}else{
setFormValues(initEvent)
}
}, [activeEvent, setFormValues])
const handleInputChange = ({target})=>{
setFormValues({
...formValues,
[target.name]: target.value
})
}
const closeModal = ()=>{
dispatch(uiCloseModal());
dispatch(eventClearActiveEvent());
setFormValues(initEvent);
}
const handleStartDateChange = (e)=>{
setDateStart(e);
setFormValues({
...formValues,
start:e
})
}
const handleEndDateChange = (e)=>{
setDateEnd(e);
setFormValues({
...formValues,
end:e
})
}
const handleSubmit = (e)=>{
e.preventDefault();
const momentStart = moment(start);
const momentEnd = moment(end);
if(momentStart.isSameOrAfter(momentEnd)){
return Swal.fire('Error' , 'La fecha fin debe ser mayor a la inicial', 'error')
}
if(title.trim().length<2){
return setTitleValid(false)
}
if (activeEvent){
dispatch(startEventUpdate(formValues))
}else{
dispatch(eventStartAddNew(formValues));
}
setTitleValid(true);
closeModal();
}
return (
<Modal
isOpen={modalOpen}
//onAfterOpen={afterOpenModal}
onRequestClose={closeModal}
style={customStyles}
closeTimeoutMS={200}
contentLabel="Example Modal"
className='modal'
>
{activeEvent ?<h1>Actualizar Evento</h1>:<h1>Nuevo Evento</h1>}
<hr />
<form
className="container"
onSubmit={handleSubmit}
>
<div className="form-group">
<label>Fecha y hora inicio</label>
<DateTimePicker
onChange={handleStartDateChange}
value={dateStart}
className="form-control"
placeholder='Fecha Inicio'
/>
</div>
<div className="form-group">
<label>Fecha y hora fin</label>
<DateTimePicker
onChange={handleEndDateChange}
value={dateEnd}
minDate={dateStart}
className="form-control"
placeholder='Fecha y Hora Fin'
/>
</div>
<hr />
<div className="form-group">
<label>Titulo y notas</label>
<input
type="text"
className= {`form-control ${!titleValid&&'is-invalid'}`}
placeholder="Título del evento"
name="title"
autoComplete="off"
value={title}
onChange={handleInputChange}
/>
<small id="emailHelp" className="form-text text-muted">Una descripción corta</small>
</div>
<div className="form-group">
<textarea
type="text"
className="form-control"
placeholder="Notas"
rows="5"
name="notes"
value={notes}
onChange={handleInputChange}
></textarea>
<small id="emailHelp" className="form-text text-muted">Información adicional</small>
</div>
<button
type="submit"
className="btn btn-outline-primary btn-block"
>
<i className="far fa-save"></i>
<span> Guardar</span>
</button>
</form>
</Modal>
)
} |
/*
* Copyright 2011 Mozilla Foundation
*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mozilla.grouperfish.text.filter;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.util.Map;
import java.util.regex.Pattern;
import org.codehaus.jackson.map.ObjectMapper;
import org.codehaus.jackson.type.TypeReference;
import com.cybozu.labs.langdetect.Detector;
import com.cybozu.labs.langdetect.DetectorFactory;
import com.cybozu.labs.langdetect.LangDetectException;
public class LanguageFilter {
public LanguageFilter(String profileDirectory) throws LangDetectException {
DetectorFactory.loadProfile(profileDirectory);
}
// isLanguage("The quick brown fox.", "en")
public boolean isLanguage(String text, String langAbbr) {
Detector detector;
boolean isLang = false;
try {
detector = DetectorFactory.create();
detector.append(text);
isLang = detector.detect().startsWith(langAbbr);
} catch (LangDetectException e) {
//System.out.println("Could not detect language on: " + text);
}
return isLang;
}
public static void main(String[] args) throws IOException {
String inputPath = args[0];
String profilesPath = args[1];
String desiredLang = args[2];
String outputPath = args[3];
ObjectMapper jsonMapper = new ObjectMapper();
BufferedReader reader = null;
BufferedWriter writer = null;
try {
LanguageFilter langFilter = new LanguageFilter(profilesPath);
reader = new BufferedReader(new InputStreamReader(new FileInputStream(inputPath), "UTF-8"));
writer = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(outputPath), "UTF-8"));
String line = null;
Pattern tabPattern = Pattern.compile("\t");
long total = 0;
long passed = 0;
while ((line = reader.readLine()) != null) {
total++;
String[] fields = tabPattern.split(line);
// Skip if we have more tabs (work around this later)
if (fields.length != 2) continue;
Map<String,Object> jsonMap = jsonMapper.readValue(fields[1], new TypeReference<Map<String,Object>>() { });
// Filter lang. detection
// !fields[6].toLowerCase().startsWith(desiredLang) &&
if (!langFilter.isLanguage((String)jsonMap.get("text"), desiredLang)) continue;
// Write original line back to output
writer.write(line);
writer.write('\n');
passed++;
if (total % 1000 == 0) {
System.out.printf("Processed %d lines\n", total);
}
}
System.out.printf("Percentage that passed: %.2f%%\n", ((float)passed/(float)total)*100.0f);
} catch (LangDetectException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if (writer != null) {
try {
writer.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
if (reader != null) {
try {
reader.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
} |
var core_1 = require('@angular/core');
var router_deprecated_1 = require('@angular/router-deprecated');
var trace_1 = require("../trace");
/**
* The NSRouterLink directive lets you link to specific parts of your app.
*
* Consider the following route configuration:
* ```
* @RouteConfig([
* { path: '/user', component: UserCmp, as: 'User' }
* ]);
* class MyComp {}
* ```
*
* When linking to this `User` route, you can write:
*
* ```
* <a [nsRouterLink]="['./User']">link to user component</a>
* ```
*
* RouterLink expects the value to be an array of route names, followed by the params
* for that level of routing. For instance `['/Team', {teamId: 1}, 'User', {userId: 2}]`
* means that we want to generate a link for the `Team` route with params `{teamId: 1}`,
* and with a child route `User` with params `{userId: 2}`.
*
* The first route name should be prepended with `/`, `./`, or `../`.
* If the route begins with `/`, the router will look up the route from the root of the app.
* If the route begins with `./`, the router will instead look in the current component's
* children for the route. And if the route begins with `../`, the router will look at the
* current component's parent.
*/
var NSRouterLink = (function () {
function NSRouterLink(_router) {
this._router = _router;
}
Object.defineProperty(NSRouterLink.prototype, "isRouteActive", {
get: function () { return this._router.isRouteActive(this._navigationInstruction); },
enumerable: true,
configurable: true
});
Object.defineProperty(NSRouterLink.prototype, "params", {
set: function (changes) {
this._routeParams = changes;
this._navigationInstruction = this._router.generate(this._routeParams);
},
enumerable: true,
configurable: true
});
NSRouterLink.prototype.onTap = function () {
trace_1.routerLog("NSRouterLink onTap() instruction: " + JSON.stringify(this._navigationInstruction));
this._router.navigateByInstruction(this._navigationInstruction);
};
NSRouterLink = __decorate([
core_1.Directive({
selector: '[nsRouterLink]',
inputs: ['params: nsRouterLink'],
host: {
'(tap)': 'onTap()',
'[class.router-link-active]': 'isRouteActive'
}
}),
__metadata('design:paramtypes', [router_deprecated_1.Router])
], NSRouterLink);
return NSRouterLink;
}());
exports.NSRouterLink = NSRouterLink;
//# sourceMappingURL=ns-router-link.js.map |
import { IsEnum, IsNumber, IsOptional, IsString } from 'class-validator';
import { IsDateFormat } from '../common/class-validators/date-format.validator';
import { PaymentType } from '../domain/enums/payment-type.enum';
export class WalletTransactionQueryDto {
@IsNumber()
@IsOptional()
limit?: number;
@IsNumber()
@IsOptional()
offset?: number;
@IsOptional()
@IsNumber()
minAmountInMinorUnit?: number;
@IsOptional()
@IsNumber()
maxAmountInMinorUnit?: number;
@IsDateFormat('DD/MM/YYYY')
dateCreatedBefore?: number;
@IsDateFormat('DD/MM/YYYY')
dateCreatedAfter?: number;
@IsOptional()
@IsEnum(PaymentType)
type?: PaymentType;
@IsOptional()
membershipIdentifier?: string;
} |
/*
* Copyright (C) 2005-2010 Alfresco Software Limited.
*
* This file is part of Alfresco
*
* Alfresco is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Alfresco is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Alfresco. If not, see <http://www.gnu.org/licenses/>.
*/
package org.alfresco.repo.content.transform;
import java.io.File;
import java.util.Iterator;
import java.util.List;
import org.alfresco.error.AlfrescoRuntimeException;
import org.alfresco.repo.content.filestore.FileContentWriter;
import org.alfresco.service.cmr.repository.ContentReader;
import org.alfresco.service.cmr.repository.ContentWriter;
import org.alfresco.service.cmr.repository.TransformationOptions;
import org.alfresco.util.TempFileProvider;
import org.springframework.beans.factory.InitializingBean;
/**
* Transformer that passes a document through several nested transformations
* in order to accomplish its goal.
*
* @author Derek Hulley
*/
public class ComplexContentTransformer extends AbstractContentTransformer2 implements InitializingBean
{
private List<ContentTransformer> transformers;
private List<String> intermediateMimetypes;
public ComplexContentTransformer()
{
}
/**
* The list of transformers to use.
* <p>
* If a single transformer is supplied, then it will still be used.
*
* @param transformers list of <b>at least one</b> transformer
*/
public void setTransformers(List<ContentTransformer> transformers)
{
this.transformers = transformers;
}
/**
* Set the intermediate mimetypes that the transformer must take the content
* through. If the transformation <b>A..B..C</b> is performed in order to
* simulate <b>A..C</b>, then <b>B</b> is the intermediate mimetype. There
* must always be <b>n-1</b> intermediate mimetypes, where <b>n</b> is the
* number of {@link #setTransformers(List) transformers} taking part in the
* transformation.
*
* @param intermediateMimetypes intermediate mimetypes to transition the content
* through.
*/
public void setIntermediateMimetypes(List<String> intermediateMimetypes)
{
this.intermediateMimetypes = intermediateMimetypes;
}
/**
* Ensures that required properties have been set
*/
public void afterPropertiesSet() throws Exception
{
if (transformers == null || transformers.size() == 0)
{
throw new AlfrescoRuntimeException("At least one inner transformer must be supplied: " + this);
}
if (intermediateMimetypes == null || intermediateMimetypes.size() != transformers.size() - 1)
{
throw new AlfrescoRuntimeException(
"There must be n-1 intermediate mimetypes, where n is the number of transformers");
}
if (getMimetypeService() == null)
{
throw new AlfrescoRuntimeException("'mimetypeService' is a required property");
}
}
/**
* Check we can transform all the way along the chain of mimetypes
*
* @see org.alfresco.repo.content.transform.ContentTransformer#isTransformable(java.lang.String, java.lang.String, org.alfresco.service.cmr.repository.TransformationOptions)
*/
public boolean isTransformable(String sourceMimetype, String targetMimetype, TransformationOptions options)
{
boolean result = true;
String currentSourceMimetype = sourceMimetype;
Iterator<ContentTransformer> transformerIterator = transformers.iterator();
Iterator<String> intermediateMimetypeIterator = intermediateMimetypes.iterator();
while (transformerIterator.hasNext())
{
ContentTransformer transformer = transformerIterator.next();
// determine the target mimetype. This is the final target if we are on the last transformation
String currentTargetMimetype = null;
if (!transformerIterator.hasNext())
{
currentTargetMimetype = targetMimetype;
}
else
{
// use an intermediate transformation mimetype
currentTargetMimetype = intermediateMimetypeIterator.next();
}
// check we can tranform the current stage
if (transformer.isTransformable(currentSourceMimetype, currentTargetMimetype, options) == false)
{
result = false;
break;
}
// move on
currentSourceMimetype = currentTargetMimetype;
}
return result;
}
/**
* @see org.alfresco.repo.content.transform.AbstractContentTransformer2#transformInternal(org.alfresco.service.cmr.repository.ContentReader, org.alfresco.service.cmr.repository.ContentWriter, org.alfresco.service.cmr.repository.TransformationOptions)
*/
@Override
public void transformInternal(
ContentReader reader,
ContentWriter writer,
TransformationOptions options) throws Exception
{
ContentReader currentReader = reader;
Iterator<ContentTransformer> transformerIterator = transformers.iterator();
Iterator<String> intermediateMimetypeIterator = intermediateMimetypes.iterator();
while (transformerIterator.hasNext())
{
ContentTransformer transformer = transformerIterator.next();
// determine the target mimetype. This is the final target if we are on the last transformation
ContentWriter currentWriter = null;
if (!transformerIterator.hasNext())
{
currentWriter = writer;
}
else
{
String nextMimetype = intermediateMimetypeIterator.next();
// make a temp file writer with the correct extension
String sourceExt = getMimetypeService().getExtension(currentReader.getMimetype());
String targetExt = getMimetypeService().getExtension(nextMimetype);
File tempFile = TempFileProvider.createTempFile(
"ComplextTransformer_intermediate_" + sourceExt + "_",
"." + targetExt);
currentWriter = new FileContentWriter(tempFile);
currentWriter.setMimetype(nextMimetype);
}
// transform
transformer.transform(currentReader, currentWriter, options);
// move the source on
currentReader = currentWriter.getReader();
}
// done
}
} |
// Copyright (c) 2015 Fredrik Kuivinen, [email protected].
#include <assert.h>
#include <unordered_map>
#include "TreeNode.h"
using namespace std;
// Tests for the TreeNode class.
using T = TreeNode;
class TreeNodeTester {
public:
static int depth(unsigned UniverseBits, T t)
{
if (t.x == 0)
return 0;
else
return 1 + depth(UniverseBits, t.parent(UniverseBits));
}
static T leftmost_leaf(unsigned UniverseBits, T t)
{
while (!t.is_leaf(UniverseBits))
t = t.left(UniverseBits);
return t;
}
static T parent_k(unsigned UniverseBits, T t, int k)
{
for (; k > 0; k--) {
t = t.parent(UniverseBits);
}
return t;
}
static void test()
{
cout << "Running TreeNode tests... " << flush;
if (!SBQ_TEST)
abort();
unsigned UniverseBits = 10;
T root(UniverseBits, 0);
TEST_ASSERT(root.left(UniverseBits).x == 1);
TEST_ASSERT(root.right(UniverseBits).left(UniverseBits).x == 5);
TEST_ASSERT(T(UniverseBits, 13).parent(UniverseBits).x == 6);
TEST_ASSERT(T::u_size(UniverseBits) == 1024);
TEST_ASSERT(!T(UniverseBits, 15).is_leaf(UniverseBits));
TEST_ASSERT(T(UniverseBits, 1024).is_leaf(UniverseBits));
for (int i = 0; i < T::u_size(UniverseBits)*2-1; i++) {
T t(UniverseBits, i);
TEST_ASSERT(T::in_tree(UniverseBits, i));
TEST_ASSERT(t.depth() == depth(UniverseBits, t));
TEST_ASSERT(t.leftmost_leaf(UniverseBits) == leftmost_leaf(UniverseBits, t));
for (int k = 0; k <= t.depth(); k++)
TEST_ASSERT(t.parent_k(UniverseBits, k) == parent_k(UniverseBits, t, k));
}
TEST_ASSERT(!T::in_tree(UniverseBits, T::u_size(UniverseBits)*2-1));
unordered_map<T, int> m;
m[T(UniverseBits, 0)] = 0;
m[T(UniverseBits, 15)] = 15;
m[T(UniverseBits, 16)] = 16;
TEST_ASSERT(m[T(UniverseBits, 0)] == 0);
TEST_ASSERT(m[T(UniverseBits, 15)] == 15);
TEST_ASSERT(m[T(UniverseBits, 16)] == 16);
TEST_ASSERT(m.size() == 3);
using T3 = TreeNode;
UniverseBits = 3;
T3 root3(UniverseBits, 0);
TEST_ASSERT(root3.leftmost_leaf(UniverseBits).x == root3.left(UniverseBits).left(UniverseBits).left(UniverseBits).x);
TEST_ASSERT(T3(UniverseBits, 2).leftmost_leaf(UniverseBits).x == T3(UniverseBits, 11).x);
cout << "ok." << endl;
}
};
void testTreeNode() {
TreeNodeTester::test();
} |
package com.deedeesdesignloft.deedeesjavaprojectsandquiz;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.content.ContextCompat;
import androidx.core.content.res.ResourcesCompat;
import android.content.Context;
import android.content.Intent;
import android.os.Bundle;
import android.text.method.ScrollingMovementMethod;
import android.view.MenuItem;
import android.view.View;
import android.view.inputmethod.InputMethodManager;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import java.util.Objects;
public class PigLatin extends AppCompatActivity {
private EditText editTextUserInput;
private Button btnCalculate, btnReset, btnGetCode;
private TextView textViewLabel, textViewResult;
private String codePigLatin;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_layout_common);
initViews();
Objects.requireNonNull(getSupportActionBar()).setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setBackgroundDrawable(ResourcesCompat.getDrawable(getResources(), R.drawable.project_actionbar, null));
textViewResult.setMovementMethod(new ScrollingMovementMethod());
Intent intent = getIntent();
if (intent.hasExtra("pigLatin")){
textViewLabel.setText("Convert your input to Pig Latin");
} else {textViewLabel.setText("Error: 404");}
btnCalculate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (editTextUserInput.getText().toString().isEmpty()) {
Toast toast = Toast.makeText(getApplicationContext(), getResources().getString(R.string.input_something), Toast.LENGTH_SHORT);
View view = toast.getView();
TextView toastMessage = view.findViewById(android.R.id.message);
toastMessage.setTextColor(ContextCompat.getColor(getApplicationContext(), R.color.colorYellow));
view.setBackground(ContextCompat.getDrawable(getApplicationContext(), R.drawable.toast_shape_black));
toast.show();
} else {
getPigLatin();
//btnCalculate.setEnabled(false);
textViewResult.setVisibility(View.VISIBLE);
InputMethodManager imm = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
Objects.requireNonNull(imm).hideSoftInputFromWindow(v.getWindowToken(), 0);
}
}
});
btnReset.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
editTextUserInput.getText().clear();
textViewResult.setText("");
textViewResult.setVisibility(View.INVISIBLE);
//btnCalculate.setEnabled(true);
}
});
btnGetCode.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intentCode = new Intent(PigLatin.this, ActivityCodeCommon.class);
intentCode.putExtra("codePigLatin", codePigLatin);
startActivity(intentCode);
}
});
}
private void getPigLatin(){
String word = "", word2 = "";
char ch;
String userPigLatin = editTextUserInput.getText().toString();
userPigLatin.trim();
for (int i = 0; i < userPigLatin.length(); i++) {
ch = userPigLatin.charAt(i);
if (userPigLatin.charAt(i) == 'a' || userPigLatin.charAt(i) == 'e' ||
userPigLatin.charAt(i) == 'i' || userPigLatin.charAt(i) == 'o' ||
userPigLatin.charAt(i) == 'u') {
for (int j = 1; j < userPigLatin.length(); j++) {
word += userPigLatin.charAt(j);
}
break;
}
if (userPigLatin.charAt(i) != 'a' || userPigLatin.charAt(i) != 'e' ||
userPigLatin.charAt(i) != 'i' || userPigLatin.charAt(i) != 'o' ||
userPigLatin.charAt(i) != 'u') {
word2 += ch;
}
}
textViewResult.setText("Your text in Pig Latin:" + "\n" + "\n" + word + word2 + "ay");
}
private void initViews(){
editTextUserInput = findViewById(R.id.editTextUserInput);
btnCalculate = findViewById(R.id.btn_calculate);
btnReset = findViewById(R.id.btnReset);
textViewResult = findViewById(R.id.textView_result);
textViewLabel = findViewById(R.id.textView_label);
btnGetCode = findViewById(R.id.btnGetCode);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case android.R.id.home:
super.onBackPressed();
break;
default:
break;
}
return super.onOptionsItemSelected(item);
}
} |
package com.pthw.data.network.feature.home.mapper
import com.pthw.data.network.feature.home.response.ActorResponse
import com.pthw.data.network.ktor.IMAGE_BASE_URL
import com.pthw.domain.home.model.ActorVo
import com.pthw.shared.extension.orFalse
import com.pthw.shared.extension.orZero
import com.pthw.shared.mapper.UnidirectionalMap
import javax.inject.Inject
/**
* Created by P.T.H.W on 03/04/2024.
*/
class ActorVoMapper @Inject constructor() : UnidirectionalMap<ActorResponse?, ActorVo> {
override fun map(item: ActorResponse?): ActorVo {
return ActorVo(
adult = item?.adult.orFalse(),
gender = item?.gender.orZero(),
id = item?.id.orZero(),
knownForDepartment = item?.knownForDepartment.orEmpty(),
name = item?.name.orEmpty(),
originalName = item?.originalName.orEmpty(),
popularity = item?.popularity.orZero(),
profilePath = IMAGE_BASE_URL + item?.profilePath.orEmpty()
)
}
} |
// we want to use getStaticProps
import Head from "next/head";
import Image from "next/image";
export default function Dogs({ data }) {
const { content } = data;
// console.log("content", content);
return (
<>
<Head>{data.title}</Head>
<h1>{content.heading}</h1>
<p>{content.text}</p>
<Image
src={content.image.src}
alt={content.image.alt}
width={content.image.width}
height={content.image.height}
sizes="(max-width:750px) 100vw, 750px"
/>
</>
);
}
//it's preferred to use getStaticProps
export async function getStaticProps(context) {
//error at first: we need to inform which routes should be build
//console.log(context);
//console.log("context.param.slug", context.params.slug); //see terminal
const slug = context.params.slug;
const api = "https://bucolic-bombolone-857476.netlify.app/api/dogs/" + slug;
const res = await fetch(api);
// console.log(res.status);
if (res.status != 200) {
return {
notFound: true,
};
}
const data = await res.json();
// console.log(data);
return {
props: {
data: data,
},
};
}
//maybe this should come first?
//list all pages that should be build
//copy-paste moment untill it works as needed
export async function getStaticPaths() {
const api = "https://bucolic-bombolone-857476.netlify.app/api/dogs/";
const res = await fetch(api);
const data = await res.json();
const paths = data.map((object) => {
//bliver vist nok lavet med `npm run build`
console.log("sluggy", object.slug);
return { params: { slug: object.slug } };
});
return {
// paths: [{ params: { slug: "henry" } }],
paths,
fallback: false,
};
}
//http://localhost:3000/dogs/bufas or snoopy - see: https://bucolic-bombolone-857476.netlify.app/api/dogs |
import React from 'react'
import {
Card,
CardMedia,
CardContent,
Typography,
Box,
Rating,
CardActions,
Button,
CardActionArea,
} from '@mui/material'
import {BookType} from '../dataproviders/BookDataprovider'
import AddIcon from '@mui/icons-material/Add'
import {useDispatch, useSelector} from 'react-redux'
import {addToBasket} from '../redux/BasketSlice'
import {useNotification} from '@refinedev/core'
import {RootState} from '../redux/store'
import {useNavigate} from 'react-router-dom'
type BookItemProps = {
book: BookType
}
export const BookItem: React.FC<BookItemProps> = ({book}) => {
const dispatch = useDispatch()
const navigate = useNavigate()
const basket = useSelector((state: RootState) => state.basket)
const {open} = useNotification()
function add() {
if (open) {
for (const bookItem of basket) {
if (bookItem.id == book.id) {
open({
message: 'this item already exists',
type: 'error',
})
return
}
}
dispatch(addToBasket(book))
open({
message: `add ${book.name} to basket`,
type: 'success',
})
}
}
return (
<Card>
<CardActionArea onClick={() => navigate('/book/' + book.id)}>
<CardMedia
component="img"
image={
book.image ?? 'https://edit.org/images/cat/book-covers-big-2019101610.jpg'
}
alt={book.name}
height={'400px'}
/>
</CardActionArea>
<CardContent>
<Typography variant="h5" component="div">
{book.name}
</Typography>
<Typography variant="body2" color="text.secondary">
{book.writers.join(', ')}
</Typography>
<Box>
<Rating value={book.rating} readOnly />
</Box>
</CardContent>
<CardActions onClick={add}>
<Button startIcon={<AddIcon />}>ADD</Button>
</CardActions>
</Card>
)
} |
import { Play } from 'phosphor-react'
import { useState } from 'react'
import { zodResolver } from '@hookform/resolvers/zod'
// usamos essa forma de importação quando a biblioteca que estamos utilizando não tem o export default
import * as zod from 'zod'
import { useForm } from 'react-hook-form'
import {
CountdownContainer,
FormContainer,
HomeContainer,
MinutesAmountInput,
Separator,
StartCountdownButton,
TaskInput,
} from './styles'
const newCycleFormValidationSchema = zod.object({
task: zod.string().min(1, 'Informe a tarefa'),
minutesAmount: zod
.number()
.min(5, 'O ciclo precisa ser de no máximo 60 minutos')
.max(60, 'O ciclo precisa ser de no máximo 60 minutos'),
})
export function Home() {
// controlled / uncontrolled
// const [task, setTask] = useState('')
// na propriedade do form: onSubmit={handleSubmit}
// function handleSubmit(event) {
// event.target.task.value
// }
const { register, handleSubmit, watch } = useForm({
resolver: zodResolver(newCycleFormValidationSchema),
})
function handleCreateNewCycle(data: any) {
console.log(data)
}
// console.log(formState.errors) - para mostrar erros
const task = watch('task')
const isSubmitDisabled = !task
return (
<HomeContainer>
<form onSubmit={handleSubmit(handleCreateNewCycle)} action="">
<FormContainer>
<label htmlFor="task">Vou trabalhar em</label>
<TaskInput
list="task-suggestions"
id="task"
placeholder="Dê um nome para o seu projeto"
{...register('task')}
// controlled:
// onChange={(e) => setTask(e.target.value)}
// value={task}
/>
<datalist id="task-suggestions">
<option value="Projeto 1" />
<option value="Projeto 2" />
<option value="Projeto 3" />
<option value="Projeto 4" />
</datalist>
<label htmlFor="minutesAmout">Durante</label>
<MinutesAmountInput
type="number"
id="minutesAmount"
placeholder="00"
max={60}
step="5"
min={5}
{...register('minutesAmount', { valueAsNumber: true })}
/>
<span>minutos</span>
</FormContainer>
<CountdownContainer>
<span>0</span>
<span>0</span>
<Separator>:</Separator>
<span>0</span>
<span>0</span>
</CountdownContainer>
<StartCountdownButton disabled={isSubmitDisabled} type="submit">
<Play size={24} />
Começar
</StartCountdownButton>
</form>
</HomeContainer>
)
} |
package ai.corca.adcio_android_plugins.placement.utils
import ai.corca.adcio_android_plugins.databinding.ItemProductionBinding
import android.view.LayoutInflater
import android.view.ViewGroup
import androidx.recyclerview.widget.DiffUtil
import androidx.recyclerview.widget.ListAdapter
import androidx.recyclerview.widget.RecyclerView.ViewHolder
import com.bumptech.glide.Glide
class SuggestionListAdapter : ListAdapter<Production, SuggestionListAdapter.SuggestionViewHolder>(
ProductionDiffUtilCallback
) {
inner class SuggestionViewHolder(private var binding: ItemProductionBinding) : ViewHolder(binding.root) {
fun bind(item: Production) = with(binding) {
Glide.with(binding.root)
.load(item.image)
.circleCrop()
.into(binding.ivImage)
binding.tvTitle.text = item.name
}
}
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): SuggestionViewHolder {
return SuggestionViewHolder(
ItemProductionBinding.inflate(
LayoutInflater.from(parent.context),
parent,
false,
)
)
}
override fun onBindViewHolder(holder: SuggestionViewHolder, position: Int) {
holder.bind(getItem(position))
}
}
object ProductionDiffUtilCallback : DiffUtil.ItemCallback<Production>() {
override fun areItemsTheSame(oldItem: Production, newItem: Production): Boolean {
return oldItem.name == newItem.name
}
override fun areContentsTheSame(oldItem: Production, newItem: Production): Boolean {
return oldItem == newItem
}
} |
function [timestamps, hiConfValley_idx] = analyzeScannerVideo(videoFileName, roi)
arguments
videoFileName char
% roi is defined as X (horizontal), Y (vertical), width, height
roi (1, 4) double
end
% for debugging
debug = false;
% Calculate frame-to-frame change using ROI
[changeMetric, frameRate] = measureFrameChange(videoFileName, roi);
% Do some center-preserving averaging - basically reducing to peaks and
% valleys
changeMetricSmoothed=movmean(abs(hilbert(changeMetric)), 15);
highSmooth = max(changeMetricSmoothed);
AmpThreshFractionValley = 0.09; % For findvalley's AmpThreshold
AmpThreshFractionPeak = 0.45; % For findpeak's AmpThreshold
% from: 'Pragmatic Introduction to Signal Processing 2023' Thomas
% O'Haver
% [round(peak) valleyX valleyY MeasuredWidth 0] = findvalleys(x,y,SlopeThreshold,AmpThreshold,smoothwidth,peakgroup,smoothtype)
valley_info = findvalleys(1:length(changeMetricSmoothed), changeMetricSmoothed, 0.017368, highSmooth*AmpThreshFractionValley,3,8,3);
% from: 'Pragmatic Introduction to Signal Processing 2023' Thomas
% O'Haver
% [round(peak) PeakX PeakY MeasuredWidth 1.0646.*PeakY*MeasuredWidth] = findvalleys(x,y,SlopeThreshold,AmpThreshold,smoothwidth,peakgroup,smoothtype)
peak_info = findpeaksG(1:length(changeMetricSmoothed), changeMetricSmoothed,2000, highSmooth*AmpThreshFractionPeak,3,10,3);
% Warn if fewer than four high-change regions are detected
if size(peak_info,1) < 4
disp("ERROR: fewer than four high-change regions detected!");
end
if size(peak_info,1) > 4
disp("WARNING: more than four high-change regions detected!");
end
% Hopefully, combining peak and valley information can lead to
% high-confidence detection of low-noise regions
% In a perfect world, every peak will have low-change region before it,
% snd the last peak will have a low-change region _after_ it
valleyIdxOffset = 0;
hiConfValley_idx = [];
% Used to synthesize first (or last) valley position (if needed)
avgIdxBetwixtPeaks = mean(diff(peak_info(:,2)));
% Start looping to pair up valleys preceding peaks
minMatchesPossible = min(size(valley_info,1), size(peak_info,1));
if debug
disp("Number of valleys: " + string(size(valley_info,1)) + " Number of peaks: " + string(size(peak_info,1)))
outStr = "valley indices: ";
for i=1:size(valley_info,1)
outStr = outStr + string(valley_info(i, 2)) + ", ";
end
disp(outStr);
outStr = "peak indices: ";
for i=1:size(peak_info,1)
outStr = outStr + string(peak_info(i, 2)) + ", ";
end
disp(outStr);
figure;
t = tiledlayout(1,1);
ax1 = axes(t);
plot(ax1,changeMetricSmoothed, 'r');
hold on;
grid on;
plot(ax1,changeMetric, 'g');
end
for i=1:minMatchesPossible
% Is valley _before_ peak in question _and_ after previous peak
if i > 1
% May need to shift index more than one
while ~((valley_info(i - valleyIdxOffset, 2) < peak_info(i, 2)) && ...
(valley_info(i - valleyIdxOffset, 2) > peak_info(i - 1, 2)))
% valley _not_ between peaks push index deeper
valleyIdxOffset = valleyIdxOffset - 1;
if debug
disp("INFO: i: " + string(i) + " shifting valleyIdxOffset. Now at: " + string(valleyIdxOffset))
end
end
end
if valley_info(i - valleyIdxOffset, 2) < peak_info(i, 2)
% may be a good pre-peak valley
hiConfValley_idx = [hiConfValley_idx, valley_info(i - valleyIdxOffset, 2) ];
else
% Don't have a good pre-peak valley - need to try to synthesize
if i == 1
% First peak, check to make sure there is enough space for
% a valley
if peak_info(i, 2) > avgIdxBetwixtPeaks/2
% take pre-peak valley half-way between peak and start
hiConfValley_idx = [hiConfValley_idx, peak_info(i, 2)/2 ];
if debug
disp("INFO: synthesizing leading valley at index: " + string(peak_info(i, 2)/2))
end
else
disp("ERROR: not enough quiet time before first high-change");
end
% Since we synthesized first valley, we need to offset the
% rest
valleyIdxOffset = valleyIdxOffset + 1;
else
% we are on a peak after the first, but there is no valley
% before
disp("ERROR: no valley before peak # " + string(i) + " index: " + string(peak_info(i, 2)));
end
end
lastPeakSeen_idx = i;
lastValleySeen_idx = i - valleyIdxOffset;
end
% If we are short valley information on both start _and_ end, we might
% not have processed last valley and peak
if lastPeakSeen_idx < size(peak_info,1)
hiConfValley_idx = [hiConfValley_idx, valley_info(lastValleySeen_idx + 1, 2) ];
if debug
disp("INFO: adding valley at index: " + valley_info(lastValleySeen_idx + 1, 2))
end
end
% We probably came out of the preceding processing with valleys only
% _preceding_ peaks. If we do actually have valley data _after_ the
% last peak, let's harvest it, otherwize synthesize it
lastValleyAlreadyFound = false;
lastPeak_idx = size(peak_info,1);
for i=1:size(valley_info,1)
if valley_info(i, 2) > peak_info(lastPeak_idx, 2)
% we have valley data _after_ the last peak
hiConfValley_idx = [hiConfValley_idx, valley_info(i, 2) ];
lastValleyAlreadyFound = true;
% stop looking
break;
end
end
if ~lastValleyAlreadyFound
% no calculated last valley value, need to synthesize from last
% peak value, and inter-peak durations
if (peak_info(lastPeak_idx, 2) + avgIdxBetwixtPeaks) > size(changeMetricSmoothed, 1)
% Yay! we have enough points to get a valley after the last
% peak
hiConfValley_idx = [hiConfValley_idx, peak_info(lastPeak_idx, 2) + (avgIdxBetwixtPeaks/2)];
if debug
disp("INFO: synthesizing last valley at index: " + string(peak_info(lastPeak_idx, 2) + (avgIdxBetwixtPeaks/2)))
end
else
% dang! don't have enough points after the last high-change
% peak to take data
disp("ERROR: not enough data after peak # " + string(size(hiConfValley_idx, 1)) + " index: " + string(lastPeakSeen_idx));
end
end
% Convert float indices to integers
hiConfValley_idx = round(hiConfValley_idx);
% Convert good valley indices to time
timestamps = hiConfValley_idx / frameRate;
if debug
% pick up and add more to plot
ax2 = axes(t);
timeTics = zeros(size(changeMetric,2), 1);
timeTics(hiConfValley_idx) = 1;
plot(ax2, linspace(1, size(changeMetric,2) / frameRate, size(changeMetric,2)), timeTics, 'b');
ax2.XAxisLocation = 'top';
ax2.YAxisLocation = 'right';
ax2.Color = 'none';
ax1.Box = 'off';
ax2.Box = 'off';
[~,name,~] = fileparts(videoFileName);
title(name + " Frame-to-Frame change", "Interpreter","none");
legend(ax1, ["smoothed", "raw"]);
legend(ax2, ["window center"], 'Location', 'northwest');
xlabel(ax1, "Video frame index");
xlabel(ax2, "Video frame (seconds)");
end
end |
const { Router } = require("express");
const contenedor = require("../contenedor");
const moment = require('moment');
const router = Router();
const productos = new contenedor("productos.txt");
const admin = true;
function serverProductsRoutes(app) {
app.use("/api/productos", router);
// this.ok
router.get('/:id?', (req, res) => {
const {id} = req.params;
if (id) {
const object = productos.getById(id);
object.then(response => {
if (response) {
res.json({
admin,
product: response
});
} else {
res.send("Producto no encontrado");
}
}).catch(err => console.log("Error en router.get()", err));
} else {
const allProducts = productos.getAll();
allProducts.then(response => {
res.json({
admin,
products: response
});
}).catch(err => console.log("Error en router.get()", err));
}
})
// this is ok
router.post('/', (req, res) => {
if (admin) {
const timestamp = moment().format('DD/MM/YYYY hh:mm:ss a');
const product = {timestamp, ...req.body};
const savedProduct = productos.save(product);
savedProduct.then(response => res.json(response)).catch(err => console.log("Error en router.post()", err));
} else {
res.send("Solo disponible para administradores");
}
})
router.put('/:id', (req, res) => {
if (admin) {
const {id} = req.params;
const product = req.body;
const updatedProduct = productos.updateById(id, product);
updatedProduct.then(response => {
if (response) {
res.json(response);
} else {
res.send("Producto no encontrado");
}
}).catch(err => console.log("Error en router.put()", err));
} else {
res.send("Solo disponible para administradores");
}
})
//this ok
router.delete('/:id', (req, res) => {
if (admin) {
const {id} = req.params;
const deleteProduct = productos.deleteById(id);
deleteProduct.then(response => {
if (response) {
res.json(response);
} else {
res.send("Producto no encontrado");
}
}).catch(err => console.log("Error en router.get()", err));
} else {
res.send("Solo disponible para administradores");
}
})
}
module.exports = serverProductsRoutes; |
library(rvest);library(magrittr);library(stringr);library(data.table)
start_date <- as.Date('2010-01-01')
end_date <- as.Date('2019-12-31')
all_dates <- seq(start_date, end_date, by = 'day')
dates <- all_dates[weekdays(all_dates) %in% c("星期六")]
url <- paste0("https://www.billboard.com/charts/hot-100/",dates)
getdata <- function(url){
html <- tryCatch(read_html(url), error=function(e) NULL)
rows <- html %>% html_elements(".chart-results-list .o-chart-results-list-row")
# scrape data
rank <- rows %>%
xml_find_all("//span[contains(@class, 'c-label a-font-primary-bold-l u-font-size-32@tablet u-letter-spacing-0080@tablet')]") %>%
html_text()
artist <- rows %>%
xml_find_all("//span[contains(@class, 'c-label a-no-trucate a-font-primary-s lrv-u-font-size-14@mobile-max u-line-height-normal@mobile-max u-letter-spacing-0021 lrv-u-display-block a-truncate-ellipsis-2line u-max-width-330 u-max-width-230@tablet-only')]") %>%
html_text()
song <- rows %>%
html_elements(".c-title") %>%
html_text(trim = T)
lastweek<- rows %>%
html_nodes(xpath='//ul/li[4]/ul/li[7]/ul/li[3]') %>%
html_text()
peakpos <- rows %>%
html_nodes(xpath='//ul/li[4]/ul/li[7]/ul/li[4]') %>%
html_text()
weekson <- rows %>%
html_nodes(xpath='//ul/li[4]/ul/li[7]/ul/li[5]') %>%
html_text()
date <- gsub("https://www.billboard.com/charts/hot-100/", "", url)
#彙總dataset
df <- data.frame(date, rank, song, artist, lastweek, peakpos, weekson)
}
data <- rbindlist(parallel::mclapply(url, getdata))
data
#匯出
write.csv(data,file="Dataset/billboard.csv",row.names = FALSE) |
import {createSlice, Reducer} from '@reduxjs/toolkit';
import {persistReducer} from 'redux-persist';
import AsyncStorage from '@react-native-async-storage/async-storage';
import {createOfflineAsyncThunk} from '../utils/offline';
import {offlineActionTypes} from 'react-native-offline';
import {sleep} from '../utils/promise';
import {darkTheme} from '../themes/darkTheme';
export const key = 'app';
export interface AppState {
isThemeDark: boolean;
theme: typeof darkTheme;
emulateOffline: boolean;
}
const initialState: AppState = {
isThemeDark: true,
theme: darkTheme,
emulateOffline: false,
};
export const offlineQueueTest = createOfflineAsyncThunk(
`${key}/offlineQueueTest`,
async () => {
await sleep(100);
console.log('QUEUED?');
return 'QUEUED?';
},
{
offlineOptions: {
meta: {
dismiss: [`${key}/offlineCancelTest`],
},
},
},
);
export const slice = createSlice({
name: key,
initialState,
reducers: {
offlineCancelTest: state => {
console.log('CANCEL!');
return state;
},
toggleTheme: state => {
state.isThemeDark = !state.isThemeDark;
},
toggleOffline: state => {
state.emulateOffline = !state.emulateOffline;
},
},
extraReducers: builder => {
builder.addCase(offlineActionTypes.FETCH_OFFLINE_MODE, state => {
return state;
});
},
});
const typedReducer: Reducer<typeof initialState> = slice.reducer;
const appReducer = persistReducer(
{
key,
storage: AsyncStorage,
blacklist: ['password', 'isLogin'],
},
typedReducer,
);
export const {offlineCancelTest, toggleOffline, toggleTheme} = slice.actions;
export default appReducer; |
import React, { useEffect, useState } from 'react'
import Container from '../../components/Container'
import { FaLongArrowAltRight } from 'react-icons/fa';
import { Link } from 'react-router-dom'
import { AiFillHeart, AiOutlineHeart } from "react-icons/ai";
import assistanceImg from '../../assets/images/AssistanceMessage.jpg'
import Testimonial from '../../components/Testimonial';
import { localCars } from '../../assets/data/DataItem';
import LocalCarsCard from '../../components/cards/LocalCarsCard';
import Gallery from '../../components/Gallery';
import GalleryOverlay from '../../components/GalleryOverlay ';
const carPageNavbar = [
{
label: 'نظرة عامة'
},
{
label: 'المميزات والمساحات'
},
// {
// label: 'التاريخ والفحص'
// },
{
label: 'الموصى به'
},
]
const overviewItems = [
{
type: 'معدل الأميال لكل غالون',
label: '22 في المدينة / 26 في الطرق السريعة'
},
{
type: 'المحرك',
label: '4 سلندر، بنزين، 2.5 لتر'
},
{
type: 'نوع الدفع',
label: 'دفع رباعي'
},
{
type: 'ناقل الحركة',
label: 'أوتوماتيك'
},
{
type: 'اللون',
label: 'أحمر / أسود'
},
]
const featureItems = [
{
label: 'نظام الدفع الرباعي/نظام الدفع الكلي'
},
{
label: 'مقاعد قماشية'
},
{
label: 'صوت سيدي'
},
{
label: 'مزيل الضباب الخلفي'
},
{
label: 'راديو AM/FM'
},
{
label: 'جناح خلفي'
},
{
label: 'مثبت السرعة'
},
{
label: 'مدخل صوتي إضافي'
},
{
label: 'تكييف الهواء'
},
{
label: 'مرايا كهربائية'
},
]
const MotorDetailPage = () => {
const [activeSection, setActiveSection] = useState('')
const [openGalleryOverlay, setOpenGalleryOverlay] = useState(false)
const [mainImgIndex, setMainImgIndex] = useState(0)
carPageNavbar.map(item => {
if (item.label === activeSection) {
console.log('is equal', item.label)
}
})
useEffect(() => {
const handleScroll = () => {
const sections = document.querySelectorAll('div.section')
const carNavbar = document.querySelector('.carPageNavbar');
let currentSection = ''
if (carNavbar && sections) {
const carNavbarTop = carNavbar.offsetTop
const carNavbarHeight = carNavbar.clientHeight
sections.forEach((section) => {
const sectionTop = section.offsetTop
const sectionHeight = section.clientHeight
if (carNavbarTop + carNavbarHeight >= sectionTop && carNavbarTop + carNavbarHeight < sectionTop + sectionHeight) {
currentSection = section.id
}
})
setActiveSection(currentSection)
}
}
window.addEventListener('scroll', handleScroll)
return () => {
window.removeEventListener('scroll', handleScroll)
}
}, [])
if (openGalleryOverlay) {
return <GalleryOverlay
mainImgIndex= {mainImgIndex}
setMainImgIndex= {setMainImgIndex}
setOpenGalleryOverlay= {setOpenGalleryOverlay}/>
}
return (
<div className='mt-6'>
<Container>
<div className='flex items-center gap-4 text-lg font-semibold text-blue-800'>
<Link to={'/cars/moterFilter'} className='flex items-center gap-2 p-2 hover:bg-bgLightBlue'>
<FaLongArrowAltRight/>
<p>رجوع</p>
</Link>
•
<a href={`#${carPageNavbar[2].label}`} className='p-2 hover:bg-bgLightBlue'>
الموصى به
</a>
</div>
<div className='flex justify-between items-center '>
<div className=''>
<h1 className='text-[2.5rem] text-bold text-brandBlue font-bold mb-2'>
هونداي 2011
</h1>
<p className='text-[#2A343D] font-semibold text-[1.1rem] '>
30,000,000 ل.س
<span className='mx-3 text-neutral-300'>|</span>
106 كيلو متر
</p>
</div>
<div className="flex items-center gap-2 text-blue-500 ">
<div className=' '>123</div>
<div className=' relative transition cursor-pointer'>
<AiOutlineHeart
size={28}
className="absolute -top-[2px] -right-[2px] fill-blue-500 hover:fill-red-500"/>
<AiFillHeart
size={24}
className= 'fill-white'/>
</div>
</div>
</div>
</Container>
<div className=' mt-6'>
{/* <img src={assistanceImg} alt="assistance-img" className='w-full' /> */}
<Gallery
setMainImgIndex= {setMainImgIndex}
setOpenGalleryOverlay={setOpenGalleryOverlay}/>
</div>
<div className='carPageNavbar mt-2 shadow-lg bg-white sticky top-0 z-10'>
<Container>
<div className=' flex items-center'>
{carPageNavbar.map((item, index) => (
<h1 key={index}
className={`px-4 py-2 border-b-[4px] hover:border-lighBlue
${item.label === activeSection ? 'border-lighBlue ' : 'border-white' }`}>
<a href={`#${item.label}`}
className='px-3 py-4 block hover:bg-bgLightBlue cursor-pointer'>
{item.label}
</a>
</h1>
))}
</div>
</Container>
</div>
<Container>
<div id={`${carPageNavbar[0].label}`} className='section pt-[5rem]'>
<h1 className='text-[2rem] text-bold text-brandBlue font-bold mb-4'>
{carPageNavbar[0].label}
</h1>
<div className="grid grid-cols-4 gap-4">
{overviewItems.map((item, index) => (
<div key={index}>
<p className='font-[700] text-brandBlue'>{item.type}</p>
<p className=' text-neutral-500 font-[400]'>{item.label}</p>
</div>
))}
</div>
</div>
<div id={`${carPageNavbar[1].label}`} className='section pt-[5rem]'>
<h1 className='text-[2rem] text-bold text-brandBlue font-bold mb-4'>
{carPageNavbar[1].label}
</h1>
<div className="grid grid-cols-2 gap-4">
{featureItems.map((item, index) => (
<div key={index}>
<p className='text-neutral-500 font-[500]'>{item.label}</p>
</div>
))}
</div>
</div>
<div id={`${carPageNavbar[2].label}`} className='section pt-[5rem] mb-10'>
<h1 className='text-[2rem] text-bold text-brandBlue font-bold mb-4'>
{carPageNavbar[2].label}
</h1>
<Testimonial
testimonials={localCars}
cardComponent={LocalCarsCard}
testimonialID={'recommanded-car-carousel'}
useShadow={false}
buttonPadding={' py-12'}
testimonialPadding={' '}/>
</div>
{/* <div id="section-3" className='section pt-[5rem] h-[600px]'>
<h1 className='text-[2rem] text-bold text-brandBlue font-bold mb-4'>
{carPageNavbar[3].label}
</h1>
</div> */}
</Container>
</div>
)
}
export default MotorDetailPage |
<template>
<div class="footer-container">
<div >
<el-input class="input-container" v-model="input" placeholder="请输入" type="textarea" rows="1" @focus="resizeElInput" @blur="handleInputBlur" ref="textArea" />
<div v-if="!isRecording" class="cursor" @click="handleRecordStart">
<el-icon class="recording">
<img src="../../assets/icons/recording.svg" alt="" />
</el-icon>
</div>
<div v-else class="cursor" @click="handleRecordEnd">
<el-icon class="recording">
<img src="../../assets/icons/cancel-recording.svg" alt="" />
</el-icon>
</div>
<el-button type="primary" @click="handleInputClick">发送</el-button>
<el-button type="warning" @click="handlePreview">预览</el-button>
</div>
</div>
</template>
<script lang="ts" setup>
import { ref, defineEmits,getCurrentInstance, onMounted } from 'vue'
import { iatrtcrecord } from '@/utils/index.js'
import { ElMessage } from 'element-plus'
const input = ref('')
const emit = defineEmits(['push-message','preview','toggle-recording-panel'])
const isRecording = ref(false);
// 处理发送消息方法
const handleInputClick = () => {
const inputArea = document.querySelector('.input-container')?.children[0];
inputArea.rows = 1;
// footer-panel 元素
const footEle = document.querySelector<HTMLDivElement>('.footer-container');
if (footEle && footEle !== null) footEle.style.height = '32px';
emit('push-message', {
content: input.value,
id: window.context.getRoom().observerId,
date: new Date().getTime(),
type: 1
})
input.value = ''
}
// 从讯飞接口获取数据后触发更新字幕看板
const emitMessage = (data) => {
const reg = /[.,?。,?]/
if(data.length===1 && reg.test(data) ){
return;
}
emit('push-message', {
content: data,
id: window.context.getRoom().observerId,
date: new Date().getTime(),
type: 0
})
}
// 全局挂载的一个方法,用在讯飞的调用脚本中使用
window.emitMessage = emitMessage;
// 点击开始录音按钮
const handleRecordStart = () => {
iatrtcrecord.start()
isRecording.value = true;
window.context.dispatchMagixEvent('changeRecordingShow',{isRecording:true})
emit('toggle-recording-panel',true);
ElMessage({
message: '正在录音,点击底部红色按钮取消录音',
type: 'success',
})
}
// 点击结束录音按钮
const handleRecordEnd = ()=> {
iatrtcrecord.stop()
isRecording.value = false;
emit('toggle-recording-panel',false);
window.context.dispatchMagixEvent('changeRecordingShow',{isRecording:false})
ElMessage({
message: '已结束录音',
})
}
// 将聊天内容以及字幕文本输出为文档
const handlePreview = () => {
emit('preview')
}
// 输入框focus后让它高度增加为10行
const resizeElInput = (e) => {
e.preventDefault();
const footEle = document.querySelector<HTMLDivElement>('.footer-container');
if (footEle && footEle !== null) footEle.style.height = '200px';
e.target.rows = 9;
}
// 输入框blur后恢复行数为1行显示
const handleInputBlur = (e) => {
e.preventDefault();
// footer-panel 元素
setTimeout(()=>{
const footEle = document.querySelector<HTMLDivElement>('.footer-container');
if (footEle && footEle !== null) footEle.style.height = '32px';
e.target.rows = 1;
},100)
}
onMounted(()=>{
// 当发起人修改本地字幕看板的显隐后,其他人监听字幕看板的显示隐藏触发本地看板显隐事件
window.context.addMagixEventListener('changeRecordingShow',({payload})=>{
isRecording.value = payload.isRecording;
if(isRecording.value){
emit('toggle-recording-panel',true);
}
else {
emit('toggle-recording-panel',false);
}
})
})
</script>
<style lang="scss">
.cursor {
cursor: pointer;
}
.footer-container {
/* position: fixed; */
/* bottom: 0; */
// TODO: 输入框修正
width: 100%;
height: 32px;
width: 100%;
.input-container {
.el-textarea__inner {
resize: none;
}
}
div {
display: flex;
.recording {
display: block;
width: fit-content;
img {
width: 32px;
}
}
.input-container {
display: inline;
}
}
}
</style> |
import AddIcon from "@mui/icons-material/Add";
import RemoveIcon from "@mui/icons-material/Remove";
import { IconButton, Skeleton } from "@mui/material";
import moment from "moment";
import { useState } from "react";
import { CommentItem } from "../../api/hackerNews";
import { useHNItem } from "../../hooks/useHNItem";
import { getTimeDifference } from "../../util/util";
import styles from "./_comment.module.scss";
// To be used in item page below any items that contain kids, will add kids of this kid recursively
type Props = {
itemId: number;
depth: number;
};
export const CommentSkeleton = ({ isExpanded }: { isExpanded: boolean }) => {
if (!isExpanded) {
return (
<div className={styles.container}>
<Skeleton width="100%" />
</div>
);
}
return (
<div className={styles.container}>
<Skeleton width="100%" />
<Skeleton variant="rectangular" height={120} width="100%" />
<Skeleton width="30%" />
</div>
);
};
export const CommentKid = ({ itemId, depth }: Props) => {
const { data: itemData } = useHNItem<CommentItem>(itemId);
const [isExpanded, setIsExpanded] = useState(depth < 2 ? true : false);
if (!itemData) {
return <CommentSkeleton isExpanded={isExpanded} />;
}
return (
<div className={styles.container} style={{ marginLeft: depth > 0 ? "20px" : undefined }}>
<div className={`${styles.header} ${isExpanded ? styles.expanded : styles.collapsed}`}>
<IconButton className={styles["expand-button"]} onClick={() => setIsExpanded(!isExpanded)}>
[{isExpanded ? <RemoveIcon /> : <AddIcon />}]
</IconButton>
{itemData.by} | {getTimeDifference(moment.unix(itemData.time))}
</div>
<div className={`${styles.body} ${isExpanded ? "" : styles.collapsed}`}>
<div className={styles.content} dangerouslySetInnerHTML={{ __html: itemData.text }}></div>
<div className={`${styles.kids}`}>
{itemData.kids?.map((kidId, index) => {
return <CommentKid key={index} itemId={kidId} depth={depth + 1} />;
})}
</div>
</div>
</div>
);
}; |
<?php
namespace app\controllers;
use app\models\Cliente;
use app\models\ClienteSearch;
use yii\web\Controller;
use yii\web\NotFoundHttpException;
use yii\filters\VerbFilter;
/**
* ClientesController implements the CRUD actions for Cliente model.
*/
class ClientesController extends Controller
{
/**
* @inheritDoc
*/
public function behaviors()
{
return array_merge(
parent::behaviors(),
[
'verbs' => [
'class' => VerbFilter::className(),
'actions' => [
'delete' => ['POST'],
],
],
]
);
}
/**
* Lists all Cliente models.
*
* @return string
*/
public function actionIndex()
{
$searchModel = new ClienteSearch();
$dataProvider = $searchModel->search($this->request->queryParams);
return $this->render('index', [
'searchModel' => $searchModel,
'dataProvider' => $dataProvider,
]);
}
/**
* Displays a single Cliente model.
* @param int $ID_EXTERNO Id Externo
* @return string
* @throws NotFoundHttpException if the model cannot be found
*/
public function actionView($ID_EXTERNO)
{
return $this->render('view', [
'model' => $this->findModel($ID_EXTERNO),
]);
}
/**
* Creates a new Cliente model.
* If creation is successful, the browser will be redirected to the 'view' page.
* @return string|\yii\web\Response
*/
public function actionCreate()
{
$model = new Cliente();
if ($this->request->isPost) {
if ($model->load($this->request->post())) {
$existingModel = Cliente::findOne(['Cedula' => $model->Cedula]);
if ($existingModel !== null) {
$model->addError('Cedula', 'La cédula ya existe en la base de datos');
}else
{
if($model->save()){
return $this->redirect(['/camposextra/view', 'ID_EXTERNO' => $model->ID_EXTERNO]);
}
}
}
} else {
$model->loadDefaultValues();
}
return $this->render('create', [
'model' => $model,
//'ID_EXTERNO' => $model->ID_EXTERNO,
]);
}
/*public function actionCreate2()
{
$model = new Cliente();
if ($this->request->isPost) {
if ($model->load($this->request->post()) && $model->save()) {
return $this->redirect(['view', 'ID_EXTERNO' => $model->ID_EXTERNO]);
}
} else {
$model->loadDefaultValues();
}
return $this->render('create2', [
'model' => $model,
'ID_EXTERNO' => $model->ID_EXTERNO,
]);
}*/
/**
* Updates an existing Cliente model.
* If update is successful, the browser will be redirected to the 'view' page.
* @param int $ID_EXTERNO Id Externo
* @return string|\yii\web\Response
* @throws NotFoundHttpException if the model cannot be found
*/
/*public function actionUpdate($ID_EXTERNO)
{
$model = $this->findModel($ID_EXTERNO);
if ($this->request->isPost && $model->load($this->request->post()) && $model->save()) {
return $this->redirect(['view', 'ID_EXTERNO' => $model->ID_EXTERNO]);
}
return $this->render('update', [
'model' => $model,
]);
}*/
/**
* Deletes an existing Cliente model.
* If deletion is successful, the browser will be redirected to the 'index' page.
* @param int $ID_EXTERNO Id Externo
* @return \yii\web\Response
* @throws NotFoundHttpException if the model cannot be found
*/
/*public function actionDelete($ID_EXTERNO)
{
$this->findModel($ID_EXTERNO)->delete();
return $this->redirect(['index']);
}*/
/**
* Finds the Cliente model based on its primary key value.
* If the model is not found, a 404 HTTP exception will be thrown.
* @param int $ID_EXTERNO Id Externo
* @return Cliente the loaded model
* @throws NotFoundHttpException if the model cannot be found
*/
protected function findModel($ID_EXTERNO)
{
if (($model = Cliente::findOne(['ID_EXTERNO' => $ID_EXTERNO])) !== null) {
return $model;
}
throw new NotFoundHttpException('The requested page does not exist.');
}
} |
package testMyHealthCareSystem;
import myHealthCareSystem.*;
/**
* This is a simple test demo class for the health care system.
* @author Haoran Zu
* @version 1.0
* @since 2024-01-29
* Student Name: Haoran Zu
* Student Number: 041119896
* Professor: Natalie Gluzman
* Time: 2024-02-14
*/
public class TestDemo {
/**
* Main entry point for testing the health care assignment.
* @param args Command-line arguments passed to the program.
*/
public static void main(String[] args) {
/*
* TODO: You must use the System.out.printf, formatted prints to show all health
* data as found in the EMRecord class (includes the parent class EMHRecord). Do not miss any item (includes any
* attributes which are complex objects, name, address etc.
*
* Use the following standards guide to discover what field entries might look like for demo purposes.
*
* Field values and constraints can be found in the Standards guide
* Acute and Ambulatory Care Data Content Standard, https://secure.cihi.ca/estore/productSeries.htm?pc=PCC1428
*
*/
// sample test of BirthDate class
BirthDate bd = new BirthDate(1970,12,01);
System.out.printf("BirtDate is:" + bd);
System.out.println();
// patient's address
Address address = new Address("westbury RD","2375","Ottawa","k2c1g7");
System.out.printf("Address is:" + address);
System.out.println();
// patient's name
Name name = new Name("David Smith");
System.out.printf("Name is:" + name);
System.out.println();
/* TODO: Calculate the BMI for your patient record here.
* use the static method in the MedicalCalculator class along with instance variables from the
* EMHRecord class.
*/
// patient's EMRecord
EMRecord Record = new EMRecord("Ontario","011","1101","0010",60,120);
Record.setAddress(address);
Record.setName(name);
// patient's EMHRecord
Record.setFuncitonalCentreAccount("ACC001100");
Record.setEncounterSequence(1100);
Record.setIssuingProvince("Ontario");
Record.setResidenceCode("12345");
Record.setGender("Female");
Record.setSubmissionYear(2023);
Record.setAdminViaAmbulance("None");
Record.setRegistrationDate(20230101);
Record.setRegistrationTime(1800);
System.out.printf("Record is:" + Record);
System.out.println();
// patient's BMI
System.out.println("My patient record BMI is:" + MedicalCalculator.calculateBMI(Record.getWeight(), Record.getHeight()));
/* You do not need to modify this piece of code, it is fine as it is. */
System.out.println("\nBMI VALUES");
System.out.println("Underweight: less than 18.5");
System.out.println("Normal: between 18.5 and 24.9");
System.out.println("Overweight: between 25 and 29.9");
System.out.println("Obese: 30 or greater");
}
} |
import { useMemo, useState } from 'react';
import { Box, Grid } from '@mui/material';
import { useQuery } from '@apollo/client';
import HeadText from '../../component/HeadText';
import Input from '../../component/Input';
import MyCard from '../../component/MyCard';
import { GET_ALL_COUNTRY } from './query';
import CustomCard from '../../utils/interfaces/CustomCard';
export default () => {
const [search, setSearch] = useState<string>('');
const { data, loading } = useQuery(GET_ALL_COUNTRY);
const memoizedValue = useMemo(() => {
let filtered;
if (search !== '') {
filtered = data?.countries.filter(
(country: any) => country?.code === search.toUpperCase()
);
} else {
filtered = data?.countries;
}
return filtered;
}, [search, data]);
if (loading) {
return <div className='loader' />;
}
return (
<div className='container'>
<HeadText text='Countries' type='h4' />
<Input setValue={setSearch} />
<Box sx={{ flexGrow: 1 }}>
<Grid container spacing={2}>
{data &&
memoizedValue.map((item: CustomCard, ind: number) => {
return (
<Grid key={ind + 1} item xs={6} md={4} lg={3}>
<MyCard
code={item.code}
name={item.name}
native={item.native}
phone={item.phone}
capital={item.capital}
currency={item.currency}
languages={item.languages}
continent={item.continent}
emoji={item.emoji}
states={item.states}
/>
</Grid>
);
})}
</Grid>
</Box>
</div>
);
}; |
import json
import os
import sys
import tempfile
import time
from contextlib import contextmanager
from typing import Callable, Dict, List, Optional
import torch
import torch.distributed as dist
import torch.multiprocessing as mp
import vllm.envs as envs
from vllm.distributed.parallel_state import get_cpu_world_group, get_local_rank
from vllm.logger import init_logger
logger = init_logger(__name__)
@contextmanager
def mute_output():
with open(os.devnull, "w") as f:
sys.stderr = f
sys.stdout = f
yield
def producer(i: int,
init_method: str,
cuda_visible_devices: Optional[str] = None):
if cuda_visible_devices is not None:
os.environ["CUDA_VISIBLE_DEVICES"] = cuda_visible_devices
with mute_output():
dist.init_process_group(
backend="gloo",
init_method=init_method,
world_size=2,
rank=0,
)
# produce a tensor in GPU i
data = torch.zeros((128, ), device=f"cuda:{i}")
# get the information to reconstruct the shared tensor
func, args = torch.multiprocessing.reductions.reduce_tensor(data)
args = list(args)
dist.broadcast_object_list([(func, args)], src=0)
dist.barrier()
torch.cuda.synchronize()
assert torch.all(data == 1).item()
def consumer(j: int,
init_method: str,
cuda_visible_devices: Optional[str] = None):
if cuda_visible_devices is not None:
os.environ["CUDA_VISIBLE_DEVICES"] = cuda_visible_devices
with mute_output():
dist.init_process_group(
backend="gloo",
init_method=init_method,
world_size=2,
rank=1,
)
torch.cuda.set_device(j)
recv = [None]
dist.broadcast_object_list(recv, src=0)
func: Callable
args: List
func, args = recv[0] # type: ignore
# `args[6]` is the device id
# by default pytorch will use `i` from the producer
# here we need to set it to `j` to test P2P access
args[6] = j
data = func(*args)
data += 1
dist.barrier()
torch.cuda.synchronize()
assert torch.all(data == 1).item()
def can_actually_p2p(i, j):
"""
Usually, checking if P2P access is enabled can be done by
`torch.cuda.can_device_access_peer(i, j)`. However, sometimes
the driver might be broken, and `torch.cuda.can_device_access_peer(i, j)`
returns `True` even if P2P access is not actually possible.
See https://github.com/vllm-project/vllm/issues/2728 and
https://forums.developer.nvidia.com/t/direct-gpu-gpu-communication-does-not-seem-to-work-properly/283264/10
Therefore, we have to perform a real P2P access to check if it is actually
possible.
Note on p2p and cuda IPC:
Usually, one process uses one GPU:
GPU i --> cuda context i --> tensor i --> process i
We need to combine p2p and cuda IPC, so that:
GPU i --> cuda context i --> tensor i --> process i
|shared|
GPU j --> cuda context j --> tensor j --> process j
That is to say, process i creates a tensor in GPU i, passes IPC handle to
process j, and process j accesses the tensor in GPU j. Any operation on the
tensor in process j will be reflected in the tensor in process i, because
they are the same memory segment.
It is important to note that process j accesses the tensor in GPU j, not
GPU i. That's why we need p2p access. # noqa
"""
cuda_visible_devices = os.getenv('CUDA_VISIBLE_DEVICES', None)
# pass the CUDA_VISIBLE_DEVICES to the child process
# to make sure they see the same set of GPUs
# make sure the temp file is not the same across different calls
temp_path = tempfile.mktemp() + str(time.time())
# create an empty file
with open(temp_path, "w"):
pass
init_method = f"file://{temp_path}"
# make sure the processes are spawned
smp = mp.get_context("spawn")
pi = smp.Process(target=producer,
args=(i, init_method, cuda_visible_devices))
pj = smp.Process(target=consumer,
args=(j, init_method, cuda_visible_devices))
pi.start()
pj.start()
pi.join()
pj.join()
return pi.exitcode == 0 and pj.exitcode == 0
# why do we need this cache?
# we are testing peer-to-peer (p2p) access between GPUs,across processes.
# if we test it every time, it will be very slow, because we need to create
# N * N * 2 processes, where N is the world size. This is very slow.
# to reduce the time, we use a cache file to store the p2p access status.
# the cache file is generated by the master process if it does not exist.
# then all the processes can read the cache file to check the p2p access status.
# Note that the cache file is suffixed by the CUDA_VISIBLE_DEVICES, so that we
# can have different cache files for different CUDA_VISIBLE_DEVICES settings,
# e.g. used by different vllm engines. The device id in the cache file is a
# **local** device id, i.e. from 0 to num_dev-1, where num_dev is the number
# of visible devices in the vllm engine.
_gpu_p2p_access_cache: Optional[Dict[str, bool]] = None
def gpu_p2p_access_check(i: int, j: int) -> bool:
"""Check if GPU i can access GPU j."""
# if the cache variable is already calculated,
# read from the cache instead of checking it again
global _gpu_p2p_access_cache
if _gpu_p2p_access_cache is not None:
return _gpu_p2p_access_cache[f"{i}->{j}"]
is_distributed = dist.is_initialized()
num_dev = torch.cuda.device_count()
cuda_visible_devices = envs.CUDA_VISIBLE_DEVICES
if cuda_visible_devices is None:
cuda_visible_devices = ",".join(str(i) for i in range(num_dev))
VLLM_CONFIG_ROOT = envs.VLLM_CONFIG_ROOT
path = os.path.expanduser(
f"{VLLM_CONFIG_ROOT}/vllm/gpu_p2p_access_cache_for_{cuda_visible_devices}.json"
)
os.makedirs(os.path.dirname(path), exist_ok=True)
if ((not is_distributed or get_local_rank() == 0)
and (not os.path.exists(path))):
# only the local master process (with local_rank == 0) can
# enter this block to calculate the cache
logger.info("generating GPU P2P access cache in %s", path)
cache = {}
for _i in range(num_dev):
for _j in range(num_dev):
cache[f"{_i}->{_j}"] = can_actually_p2p(_i, _j)
with open(path, "w") as f:
json.dump(cache, f, indent=4)
if is_distributed:
cpu_world_group = get_cpu_world_group()
dist.barrier(cpu_world_group)
logger.info("reading GPU P2P access cache from %s", path)
with open(path, "r") as f:
cache = json.load(f)
_gpu_p2p_access_cache = cache
return _gpu_p2p_access_cache[f"{i}->{j}"]
__all__ = ["gpu_p2p_access_check"] |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.